
This program implements a slave SPI in software for testing the SPI interface of protocoltool.
Fork of 8255_emulator by
main.cpp
00001 #include "mbed.h" 00002 00003 //#include <stdarg.h> 00004 #include <stdio.h> 00005 #include <stdlib.h> 00006 #include <string.h> 00007 00008 /* 00009 Instructions for use: connect the mbed to a parallel port using these connexions. 00010 use a terminal program to connect via USB to the mbed side. */ 00011 00012 /* This is for testing since it uses the serial port at 9600 bauds to connect to a PC */ 00013 00014 /* 00015 8255 Parallel Pin Bit 00016 PC7 /OBF -> /ACK 10 6 00017 PC6 /ACK <- /SLCTIN 17 3 00018 PC5 IBF -> BUSY 11 7 00019 PC4 /STB <- /STB 1 0 00020 00021 15 nError -> p9 not used 00022 13 Select -> p10 not used 00023 12 PE -> p11 not used 00024 11 Busy -> p12 MISO 00025 10 nAck -> p13 not used 00026 00027 1 nStrobe -> p14 MOSI 00028 14 nAutoFeed -> p15 SCLK 00029 16 nInit -> p16 not used 00030 17 nSelectIn -> p17 not used 00031 */ 00032 00033 DigitalOut MISO(p12); 00034 DigitalIn MOSI(p14); 00035 InterruptIn SCLK(p15); 00036 00037 /* 00038 CE0 p30 p0.4 00039 CE1 p29 p0.5 00040 CE2 p8 p0.6 00041 CE3 p7 p0.7 00042 CE4 p6 p0.8 00043 CE5 p5 p0.9 00044 CE6 p28 p0.10 00045 CE7 p27 p0.11 00046 */ 00047 BusInOut PtrData(p30,p29,p8,p7,p6,p5,p28,p27); 00048 00049 #define __DOUTBUFSIZE 256 00050 #define __DINBUFSIZE 256 00051 char __outstr[__DOUTBUFSIZE]; 00052 char __instr[__DINBUFSIZE]; 00053 00054 Serial pc(USBTX, USBRX); // tx, rx 00055 00056 unsigned char rx_data, tx_data; 00057 00058 bool msb_first = true; 00059 bool edge_falling = false; // false: CPOL = 0, CPHA = 0; true: CPOL = 1, CPHA = 1 00060 bool byte_ready; 00061 int bit_count; 00062 00063 void shift_bit(void) 00064 { 00065 if (msb_first) 00066 { 00067 rx_data = (rx_data << 1) | MOSI; 00068 MISO = (tx_data & 0x80) >> 7; 00069 tx_data <<= 1; 00070 } 00071 else 00072 { 00073 rx_data = (rx_data >> 1) | (MOSI << 7); 00074 MISO = tx_data & 1; 00075 tx_data >>= 1; 00076 } 00077 bit_count++; 00078 if (bit_count == 8) 00079 { 00080 byte_ready = true; 00081 bit_count = 0; 00082 } 00083 } 00084 00085 void sclk_fall(void) 00086 { 00087 if (edge_falling && (PtrData == 0xfe)) 00088 { 00089 shift_bit(); 00090 } 00091 } 00092 00093 void sclk_rise(void) 00094 { 00095 if (!edge_falling && (PtrData == 0xfe)) 00096 { 00097 shift_bit(); 00098 } 00099 } 00100 00101 int main() 00102 { 00103 unsigned char key; 00104 bool configure_end = false; 00105 00106 PtrData.input(); 00107 00108 /* 9600 baud serial port */ 00109 pc.printf("SPI tester on mbed\r\n\n"); 00110 00111 MISO = 0; 00112 SCLK.fall(&sclk_fall); 00113 SCLK.rise(&sclk_rise); 00114 SCLK.mode(PullUp); 00115 SCLK.enable_irq(); 00116 00117 byte_ready = false; 00118 bit_count = 0; 00119 00120 pc.printf("Actual configuration\r\n\n"); 00121 pc.printf("MSB first\r\n"); 00122 pc.printf("Rising edge clock\r\n\n"); 00123 00124 pc.printf("Configure\r\n\n"); 00125 pc.printf("M: MSB first\r\n"); 00126 pc.printf("L: LSB first\r\n"); 00127 pc.printf("F: Falling edge clock\r\n"); 00128 pc.printf("R: Rising edge clock\r\n"); 00129 pc.printf("G: Go\r\n\n"); 00130 00131 do 00132 { 00133 key = pc.getc(); 00134 00135 switch (key) 00136 { 00137 case 'M': 00138 case 'm': 00139 msb_first = true; 00140 pc.printf("MSB first\r\n"); 00141 break; 00142 00143 case 'L': 00144 case 'l': 00145 msb_first = false; 00146 pc.printf("LSB first\r\n"); 00147 break; 00148 00149 case 'F': 00150 case 'f': 00151 edge_falling = true; 00152 pc.printf("Falling edge clock\r\n"); 00153 break; 00154 00155 case 'R': 00156 case 'r': 00157 edge_falling = true; 00158 pc.printf("Rising edge clock\r\n"); 00159 break; 00160 00161 case 'G': 00162 case 'g': 00163 configure_end = true; 00164 break; 00165 00166 default: 00167 ; 00168 } 00169 } while (!configure_end); 00170 00171 pc.printf("Configure end, begin test\r\n\n"); 00172 00173 while(1) 00174 { 00175 if (pc.readable()) 00176 { 00177 tx_data = pc.getc(); 00178 } 00179 else 00180 { 00181 if (byte_ready) 00182 { 00183 pc.putc(rx_data); 00184 byte_ready = false; 00185 } 00186 } 00187 } 00188 }
Generated on Wed Jul 13 2022 01:00:20 by
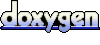