
This is a program for testing the 8255 interface on protocoltool. It implements a software emulation of the bidirectional mode 2 of a 8255 chip.
Fork of parallel_port_tester by
main.cpp
00001 #include "mbed.h" 00002 00003 //#include <stdarg.h> 00004 #include <stdio.h> 00005 #include <stdlib.h> 00006 #include <string.h> 00007 00008 /* 00009 Instructions for use: connect the mbed to a parallel port using these connexions. 00010 use a terminal program to connect via USB to the mbed side. */ 00011 00012 /* This is for testing since it uses the serial port at 9600 bauds to connect to a PC */ 00013 00014 #define PAR_INVERT_OBF_SIGNAL 00015 00016 #define PAR_8255_SEND_MASK 0x80 00017 #define PAR_8255_RECV_MASK 0x40 00018 00019 /* 00020 8255 Parallel Pin Bit 00021 PC7 /OBF -> /ACK 10 6 00022 PC6 /ACK <- /SLCTIN 17 3 00023 PC5 IBF -> BUSY 11 7 00024 PC4 /STB <- /STB 1 0 00025 00026 15 nError -> p9 not used 00027 13 Select -> p10 not used 00028 12 PE -> p11 not used 00029 11 Busy -> p12 IBF 00030 10 nAck -> p13 /OBF 00031 00032 1 nStrobe -> p14 /STB 00033 14 nAutoFeed -> p15 not used 00034 16 nInit -> p16 not used 00035 17 nSelectIn -> p17 /ACK 00036 */ 00037 00038 DigitalOut IBF(p12); // IBF 00039 DigitalOut nOBF(p13); // /OBF 00040 00041 InterruptIn nSTB(p14); 00042 InterruptIn nACK(p17); 00043 00044 /* 00045 D0 p30 p0.4 00046 D1 p29 p0.5 00047 D2 p8 p0.6 00048 D3 p7 p0.7 00049 D4 p6 p0.8 00050 D5 p5 p0.9 00051 D6 p28 p0.10 00052 D7 p27 p0.11 00053 */ 00054 BusInOut PtrData(p30,p29,p8,p7,p6,p5,p28,p27); 00055 00056 #define __DOUTBUFSIZE 256 00057 #define __DINBUFSIZE 256 00058 char __outstr[__DOUTBUFSIZE]; 00059 char __instr[__DINBUFSIZE]; 00060 00061 Serial pc(USBTX, USBRX); // tx, rx 00062 00063 unsigned char rx_data; 00064 00065 // Peripheral should check that there is no output pending from the 8255 to the peripheral before writing to the 8255 00066 00067 // When /STB is falling 00068 void perif2mbed(void) 00069 { 00070 // read byte from peripheral 00071 rx_data = PtrData; 00072 IBF = 1; 00073 } 00074 00075 // When /ACK is rising 00076 void mbed2perif(void) 00077 { 00078 nOBF = 1; 00079 PtrData.input(); 00080 } 00081 00082 void write_byte(unsigned char out) 00083 { 00084 // wait for /OBF = 1 and no read cycle in progress for politeness 00085 while ((nOBF == 0) || (IBF == 1)); 00086 00087 PtrData = out; 00088 PtrData.output(); 00089 nOBF = 0; 00090 } 00091 00092 unsigned char read_byte(void) 00093 { 00094 while (IBF == 0); 00095 IBF = 0; 00096 return rx_data; 00097 } 00098 00099 int main() 00100 { 00101 PtrData.input(); 00102 00103 /* 9600 baud serial port */ 00104 pc.printf("8255 emulator on mbed\r\n\n"); 00105 00106 IBF = 0; 00107 nOBF = 1; 00108 nSTB.fall(&perif2mbed); 00109 nACK.rise(&mbed2perif); 00110 00111 PtrData.input(); 00112 00113 while(1) 00114 { 00115 // bytes from peripherals to 8255 00116 if (IBF == 1) 00117 { 00118 IBF = 0; 00119 pc.putc(rx_data); 00120 } 00121 else 00122 { 00123 if (pc.readable()) 00124 { 00125 write_byte(pc.getc()); 00126 } 00127 } 00128 } 00129 }
Generated on Sat Jul 16 2022 18:46:09 by
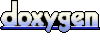