WIZwikiREST-io Reference code for WIZnet Academy
Dependencies: DHT MbedJSONValue_v102 WIZnetInterface mbed-src
Fork of WIZwiki-REST-io_v103 by
main.cpp
00001 #include "mbed.h" 00002 #include "HTTPServer.h" 00003 #include "RequestHandler.h" 00004 #include "EthernetInterface.h" 00005 #include "MbedJSONValue.h" 00006 #include "DHT.h" 00007 00008 #define SERVER_PORT 80 00009 //#define DHCP 00010 00011 00012 //-- GPIO LED -- 00013 DigitalOut GP09(D9); 00014 DigitalOut GP10(D10); 00015 DigitalOut GP11(D11); 00016 00017 //-- ADC -- 00018 AnalogIn ain(A0); 00019 00020 //-- DHT -- 00021 DHT sensor(D4, DHT11); 00022 00023 00024 EthernetInterface eth; 00025 HTTPServer WIZwikiWebSvr; 00026 MbedJSONValue WIZwikiREST; 00027 00028 GetRequestHandler myGetReq; 00029 //PostRequestHandler myPostReq; 00030 PutRequestHandler myPutReq; 00031 00032 // Enter a MAC address for your controller below. 00033 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x00, 0x01, 0xFE}; 00034 char mac_str[20]; 00035 char ip_addr[] = "192.168.100.100"; 00036 char subnet_mask[] = "255.255.255.0"; 00037 char gateway_addr[] = "192.168.100.1"; 00038 00039 float c = 0.0f, h = 0.0f; 00040 00041 00042 //-- GPIO -- 00043 bool p9_set(void* param) 00044 { 00045 if(!param) return false; 00046 GP09.write(*(int*)param); 00047 return true; 00048 } 00049 bool p10_set(void* param) 00050 { 00051 if(!param) return false; 00052 GP10.write(*(int*)param); 00053 return true; 00054 } 00055 bool p11_set(void* param) 00056 { 00057 if(!param) return false; 00058 GP11.write(*(int*)param); 00059 return true; 00060 } 00061 00062 //-- ADC -- 00063 bool ain_read(void* param) 00064 { 00065 ((MbedJSONValue*)param)->_value.asDouble = ain.read(); 00066 return true; 00067 } 00068 00069 //-- DHT -- 00070 bool tmp_read(void* param) 00071 { 00072 wait(0.5); 00073 ((MbedJSONValue*)param)->_value.asDouble = (sensor.readData()==0) ? sensor.ReadTemperature(CELCIUS) : 0; 00074 return true; 00075 } 00076 bool hum_read(void* param) 00077 { 00078 wait(0.5); 00079 ((MbedJSONValue*)param)->_value.asDouble = (sensor.readData()==0) ? sensor.ReadHumidity() : 0; 00080 return true; 00081 } 00082 00083 00084 void WIZwiki_REST_init(); 00085 00086 int main(void) 00087 { 00088 00089 sprintf(mac_str, "%02X:%02X:%02X:%02X:%02X:%02X",mac_addr[0],mac_addr[1],mac_addr[2],mac_addr[3],mac_addr[4],mac_addr[5]); 00090 00091 WIZwiki_REST_init(); 00092 00093 // Serialize it into a JSON string 00094 printf("---------------------WIZwikiREST-------------------- \r\n"); 00095 printf("\r\n%s\r\n", WIZwikiREST.serialize().c_str()); 00096 printf("---------------------------------------------------- \r\n"); 00097 00098 WIZwikiWebSvr.add_request_handler("GET", &myGetReq); 00099 //WIZwikiWebSvr.add_request_handler("POST", &myPostReq); 00100 WIZwikiWebSvr.add_request_handler("PUT", &myPutReq); 00101 //WIZwikiWebSvr.add_request_handler("DELETE", new PostRequestHandler()); 00102 00103 #ifdef DHCP 00104 eth.init(mac_addr); //Use DHCP 00105 #else 00106 eth.init(mac_addr, ip_addr, subnet_mask, gateway_addr); //Not Use DHCP 00107 #endif 00108 00109 00110 printf("Check Ethernet Link\r\n"); 00111 00112 do{ 00113 printf(" Link - Wait... \r\n"); 00114 wait(1); 00115 }while(!eth.ethernet_link()); 00116 printf("-- Ethetnet PHY Link - Done -- \r\n"); 00117 00118 if (eth.connect() < 0 ) 00119 printf("-- EThernet Connect - Fail -- \r\n"); 00120 else 00121 { 00122 printf("-- Assigned Network Information -- \r\n"); 00123 printf(" IP : %s\r\n\r\n", eth.getIPAddress()); 00124 printf(" MASK : %s\r\n\r\n", eth.getNetworkMask()); 00125 printf(" GW : %s\r\n\r\n", eth.getGateway()); 00126 } 00127 00128 printf("Link up\r\n"); 00129 printf("IP Address is %s\r\n", eth.getIPAddress()); 00130 00131 00132 if(!WIZwikiWebSvr.init(SERVER_PORT)) 00133 { 00134 eth.disconnect(); 00135 return -1; 00136 } 00137 00138 while(1) 00139 { 00140 WIZwikiWebSvr.run(); 00141 } 00142 } 00143 00144 void WIZwiki_REST_init(void) 00145 { 00146 //Fill the object 00147 WIZwikiREST["Name"] = "WIZwikiREST-io WIZnet Academy"; 00148 WIZwikiREST["Name"].accessible = false; 00149 00150 //Network 00151 WIZwikiREST["Network"]["MAC"] = mac_str; 00152 WIZwikiREST["Network"]["IP"] = ip_addr; 00153 WIZwikiREST["Network"]["IP"].accessible = true; 00154 WIZwikiREST["Network"]["SN"] = subnet_mask; 00155 WIZwikiREST["Network"]["SN"].accessible = true; 00156 WIZwikiREST["Network"]["GW"] = gateway_addr; 00157 WIZwikiREST["Network"]["GW"].accessible = true; 00158 00159 // GPIO 00160 WIZwikiREST["GPIOs"]["P09"] = 0; 00161 WIZwikiREST["GPIOs"]["P09"].accessible = true; 00162 WIZwikiREST["GPIOs"]["P09"].cb_action = p9_set; 00163 WIZwikiREST["GPIOs"]["P10"] = 0; 00164 WIZwikiREST["GPIOs"]["P10"].accessible = true; 00165 WIZwikiREST["GPIOs"]["P10"].cb_action = p10_set; 00166 WIZwikiREST["GPIOs"]["P11"] = 0; 00167 WIZwikiREST["GPIOs"]["P11"].accessible = true; 00168 WIZwikiREST["GPIOs"]["P11"].cb_action = p11_set; 00169 00170 // ADC 00171 WIZwikiREST["ADC"]["A0"] = 0.0f; 00172 WIZwikiREST["ADC"]["A0"].accessible = false; 00173 WIZwikiREST["ADC"]["A0"].cb_action = ain_read; 00174 00175 // DHT11 00176 WIZwikiREST["DHT"]["tmp"] = 0.0f; 00177 WIZwikiREST["DHT"]["tmp"].accessible = false; 00178 WIZwikiREST["DHT"]["tmp"].cb_action = tmp_read; 00179 WIZwikiREST["DHT"]["hum"] = 0.0f; 00180 WIZwikiREST["DHT"]["hum"].accessible = false; 00181 WIZwikiREST["DHT"]["hum"].cb_action = hum_read; 00182 00183 }
Generated on Wed Jul 27 2022 10:11:29 by
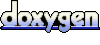