
Test HC05 Bluetooth adapter with NUCLEO F401RE. Includes serial command listener will echo commands from remote PC both on that connection and on local PC.
Dependencies: mbed multi-serial-command-listener
main.cpp
00001 /* xej-Nucleo-F401RE-and-HC05-Bluetooth.cpp 00002 Test Nucleo-F401RE with HC05 Bluetooth adapter 00003 00004 Wanted to use it for upload of telemetry 00005 type a command and hc053 port and it will be echoed back 00006 00007 00008 pin-HC05 Pin-MBed 00009 TX --- PA_12 00010 RX --- PA_11 00011 +5V --- +5 on CN6 00012 Also worked on 3.3V on CN6 00013 GND --- GND on CN6 00014 STATE--- NC - not connected 00015 EN --- NC - not connected 00016 00017 If you cycle power on mbed you may need to close 00018 and re-open the port connection which 00019 is pretty easy when using RealTerm. 00020 00021 tested with Honbay® Wireless Bluetooth Host Serial Transceiver Module 00022 Draws about 30mA when starting then drops back 00023 to 10mA when idle with a jump to about 20 mA 00024 when transmitting. 00025 00026 *** 00027 * By Joseph Ellsworth CTO of A2WH 00028 * Take a look at A2WH.com Producing Water from Air using Solar Energy 00029 * March-2016 License: https://developer.mbed.org/handbook/MIT-Licence 00030 * Please contact us http://a2wh.com for help with custom design projects. 00031 *** 00032 00033 */ 00034 #include "mbed.h" 00035 #include "multi-serial-command-listener.h" 00036 00037 char myCommand[SCMD_MAX_CMD_LEN+1]; 00038 00039 00040 //------------------------------------ 00041 // RealTerm or Teraterm config 00042 // 9600 bauds, 8-bit data, no parity 00043 //------------------------------------ 00044 00045 //Serial hc05(D1, D0); // PA_2, PA_3 This one does not work because redirected to USBTX, USBRX 00046 // // can be fixed by changing solder bridges 00047 Serial hc052(D10,D2); // PB_6, PA_10 This one works 00048 Serial hc053(PA_11, PA_12); // This one works 00049 Serial pc(USBTX, USBRX); 00050 00051 DigitalOut myled(LED1); 00052 00053 00054 void commandCallback(char *cmdIn, void *extraContext) { 00055 strcpy(myCommand, cmdIn); 00056 // all our commands will be recieved async in commandCallback 00057 // we don't want to do time consuming things since it could 00058 // block the reader and allow the uart to overflow so we simply 00059 // copy it out in the callback and then process it latter. 00060 00061 // See data_log one of dependants of this library for example 00062 // of using *extraContext 00063 } 00064 00065 int main() { 00066 pc.baud(9600); 00067 //hc05.baud(9600); 00068 hc052.baud(9600); 00069 hc053.baud(9600); 00070 int i = 1; 00071 struct SCMD *cmdProc = scMake(&hc053, commandCallback, NULL) ; 00072 00073 pc.printf("Test HC05 Bluetooth Connection !\r\n"); 00074 while(1) { 00075 wait(1); 00076 //hc05.printf("d1/do this program runs since %d seconds.\r\n", i); 00077 hc052.printf("d10/d2 %d seconds\r\n", i); 00078 hc053.printf("PA_11/PA_12 %d seconds\r\n", i); 00079 pc.printf("This program runs since %d seconds.\r\n", i++); 00080 myled = !myled; 00081 if (myCommand[0] != 0) { 00082 pc.printf("Command Recieved =%s\r\n", myCommand); 00083 hc053.printf("\r\nCommand Recieved =%s\r\n", myCommand); 00084 if (strcmp(myCommand,"clear") == 0) { 00085 i = 0; 00086 } 00087 myCommand[0] = 0; // clear until we recieve the next command 00088 } 00089 } 00090 } 00091
Generated on Mon Jul 18 2022 01:30:20 by
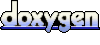