
ロボコンマガジン 2013年1月号に掲載されている 「第3回お手軽mbedではじめるロボットプロトタイピング」 で紹介しているうおーるぼっとをマウスで動かすプログラムです。
Dependencies: FatFileSystem TB6612FNG2 mbed
Fork of BlueUSB by
AutoEvents.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "USBHost.h" 00026 #include "Utils.h" 00027 #include "TB6612.h" 00028 00029 #define AUTOEVT(_class,_subclass,_protocol) (((_class) << 16) | ((_subclass) << 8) | _protocol) 00030 #define AUTO_KEYBOARD AUTOEVT(CLASS_HID,1,1) 00031 #define AUTO_MOUSE AUTOEVT(CLASS_HID,1,2) 00032 00033 u8 auto_mouse[4]; // buttons,dx,dy,scroll 00034 u8 auto_keyboard[8]; // modifiers,reserved,keycode1..keycode6 00035 u8 auto_joystick[4]; // x,y,buttons,throttle 00036 00037 TB6612 left(p21,p12,p11); 00038 TB6612 right(p22,p14,p13); 00039 00040 void AutoEventCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00041 { 00042 static int speed = 100; 00043 int evt = (int)userData; 00044 switch (evt) 00045 { 00046 case AUTO_KEYBOARD: 00047 printf("AUTO_KEYBOARD "); 00048 break; 00049 case AUTO_MOUSE: 00050 printf("AUTO_MOUSE "); 00051 break; 00052 default: 00053 printf("HUH "); 00054 } 00055 00056 if( (data[0] & 0x01) != 0 ) 00057 { 00058 left = speed; 00059 } 00060 else 00061 { 00062 left = 0; 00063 } 00064 if( (data[0] & 0x02) != 0 ) 00065 { 00066 right = speed; 00067 } 00068 else 00069 { 00070 right = 0; 00071 } 00072 00073 speed = speed + (signed char)data[3]; 00074 00075 if( speed > 100 ) speed = 100; 00076 if( speed < -100 ) speed = -100; 00077 00078 printf("speed = %d ",speed); 00079 00080 printfBytes("data",data,len); 00081 USBInterruptTransfer(device,endpoint,data,len,AutoEventCallback,userData); 00082 } 00083 00084 // Establish transfers for interrupt events 00085 void AddAutoEvent(int device, InterfaceDescriptor* id, EndpointDescriptor* ed) 00086 { 00087 if ((ed->bmAttributes & 3) != ENDPOINT_INTERRUPT || !(ed->bEndpointAddress & 0x80)) 00088 return; 00089 00090 // Make automatic interrupt enpoints for known devices 00091 u32 evt = AUTOEVT(id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00092 u8* dst = 0; 00093 int len; 00094 switch (evt) 00095 { 00096 case AUTO_MOUSE: 00097 dst = auto_mouse; 00098 len = sizeof(auto_mouse); 00099 break; 00100 case AUTO_KEYBOARD: 00101 dst = auto_keyboard; 00102 len = sizeof(auto_keyboard); 00103 break; 00104 default: 00105 printf("Interrupt endpoint %02X %08X\n",ed->bEndpointAddress,evt); 00106 break; 00107 } 00108 if (dst) 00109 { 00110 printf("Auto Event for %02X %08X\n",ed->bEndpointAddress,evt); 00111 USBInterruptTransfer(device,ed->bEndpointAddress,dst,len,AutoEventCallback,(void*)evt); 00112 } 00113 } 00114 00115 void PrintString(int device, int i) 00116 { 00117 u8 buffer[256]; 00118 int le = GetDescriptor(device,DESCRIPTOR_TYPE_STRING,i,buffer,255); 00119 if (le < 0) 00120 return; 00121 char* dst = (char*)buffer; 00122 for (int j = 2; j < le; j += 2) 00123 *dst++ = buffer[j]; 00124 *dst = 0; 00125 printf("%d:%s\n",i,(const char*)buffer); 00126 } 00127 00128 // Walk descriptors and create endpoints for a given device 00129 int StartAutoEvent(int device, int configuration, int interfaceNumber) 00130 { 00131 u8 buffer[255]; 00132 int err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00133 if (err < 0) 00134 return err; 00135 00136 int len = buffer[2] | (buffer[3] << 8); 00137 u8* d = buffer; 00138 u8* end = d + len; 00139 while (d < end) 00140 { 00141 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) 00142 { 00143 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00144 if (id->bInterfaceNumber == interfaceNumber) 00145 { 00146 d += d[0]; 00147 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) 00148 { 00149 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00150 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00151 d += d[0]; 00152 } 00153 } 00154 } 00155 d += d[0]; 00156 } 00157 return 0; 00158 } 00159 00160 // Implemented in main.cpp 00161 int OnDiskInsert(int device); 00162 00163 // Implemented in TestShell.cpp 00164 int OnBluetoothInsert(int device); 00165 00166 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc) 00167 { 00168 printf("LoadDevice %d %02X:%02X:%02X\n",device,interfaceDesc->bInterfaceClass,interfaceDesc->bInterfaceSubClass,interfaceDesc->bInterfaceProtocol); 00169 char s[128]; 00170 for (int i = 1; i < 3; i++) 00171 { 00172 if (GetString(device,i,s,sizeof(s)) < 0) 00173 break; 00174 printf("%d: %s\n",i,s); 00175 } 00176 00177 switch (interfaceDesc->bInterfaceClass) 00178 { 00179 case CLASS_MASS_STORAGE: 00180 if (interfaceDesc->bInterfaceSubClass == 0x06 && interfaceDesc->bInterfaceProtocol == 0x50) 00181 OnDiskInsert(device); // it's SCSI! 00182 break; 00183 case CLASS_WIRELESS_CONTROLLER: 00184 if (interfaceDesc->bInterfaceSubClass == 0x01 && interfaceDesc->bInterfaceProtocol == 0x01) 00185 OnBluetoothInsert(device); // it's bluetooth! 00186 break; 00187 default: 00188 StartAutoEvent(device,1,0); 00189 break; 00190 } 00191 }
Generated on Wed Jul 13 2022 21:50:50 by
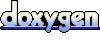