
A demo program for DOGL-128 LCD module. Based on Mike Sheldon's 3D Tie Fighter demo.
Embed:
(wiki syntax)
Show/hide line numbers
DogLCD.h
00001 #ifndef MBED_DOGLCD_H 00002 #define MBED_DOGLCD_H 00003 00004 #include "AbstractLCD.h" 00005 00006 /*********** 00007 * Module for Electronic Assembly's DOGL128-6 display module 00008 * Should be compatible with other modules using ST7565 controller 00009 ***********/ 00010 00011 #define LCDWIDTH 128 00012 #define LCDHEIGHT 64 00013 #define LCDPAGES (LCDHEIGHT+7)/8 00014 00015 /* 00016 00017 Each page is 8 lines, one byte per column 00018 00019 Col0 00020 +---+-- 00021 | 0 | 00022 Page 0 | 1 | 00023 | 2 | 00024 | 3 | 00025 | 4 | 00026 | 5 | 00027 | 6 | 00028 | 7 | 00029 +---+-- 00030 */ 00031 00032 /* 00033 LCD interface class. 00034 Usage: 00035 DogLCD dog(spi, pin_power, pin_cs, pin_a0, pin_reset); 00036 where spi is an instance of SPI class 00037 */ 00038 00039 class DogLCD: public AbstractLCD 00040 { 00041 SPI& _spi; 00042 DigitalOut _cs, _a0, _reset, _power; 00043 int _updating; 00044 void _send_commands(const unsigned char* buf, size_t size); 00045 void _send_data(const unsigned char* buf, size_t size); 00046 void _set_xy(int x, int y); 00047 unsigned char _framebuffer[LCDWIDTH*LCDPAGES]; 00048 public: 00049 DogLCD(SPI& spi, PinName power, PinName cs, PinName a0, PinName reset): 00050 _spi(spi), _cs(cs), _a0(a0), _reset(reset), _power(power), _updating(0) 00051 { 00052 } 00053 // initialize and turn on the display 00054 void init(); 00055 // send a 128x64 picture for the whole screen 00056 void send_pic(const unsigned char* data); 00057 // clear screen 00058 void clear_screen(); 00059 // turn all pixels on 00060 void all_on(bool on = true); 00061 00062 // AbstractLCD methods 00063 virtual int width() {return LCDWIDTH;}; 00064 virtual int height() {return LCDHEIGHT;}; 00065 virtual void pixel(int x, int y, int colour); 00066 virtual void fill(int x, int y, int width, int height, int colour); 00067 virtual void beginupdate(); 00068 virtual void endupdate(); 00069 }; 00070 00071 #endif
Generated on Fri Jul 15 2022 20:49:26 by
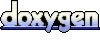