Class library for a LIS3DSH MEMS digital output motion sensor (acceleromoter).
Dependents: stm32f407 mbed_blinky STM32F407
LIS3DSH.h
00001 /* LIS3DSH Library v1.0 00002 * Copyright (c) 2016 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #ifndef LIS3DSH_H 00026 #define LIS3DSH_H 00027 00028 #include "mbed.h" 00029 00030 /** Class library for a LIS3DSH MEMS digital output motion sensor (acceleromoter). 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "LIS3DSH.h" 00036 * 00037 * LIS3DSH acc(PA_7, PA_6, PA_5, PE_3); 00038 * // mosi, miso, clk , cs 00039 * 00040 * int main() { 00041 * int16_t X, Y, Z; //signed integer variables for raw X,Y,Z values 00042 * float roll, pitch; //float variables for angles 00043 * 00044 * if(acc.Detect() != 1) { 00045 * printf("LIS3DSH Acceleromoter not detected!\n"); 00046 * while(1){ }; 00047 * } 00048 * 00049 * while(1) { 00050 * acc.ReadData(&X, &Y, &Z); //read X, Y, Z values 00051 * acc.ReadAngles(&roll, &pitch); //read roll and pitch angles 00052 * printf("X: %d Y: %d Z: %d\n", X, Y, Z); 00053 * printf("Roll: %f Pitch: %f\n", roll, pitch); 00054 * 00055 * wait(1.0); //delay before reading next values 00056 * } 00057 * } 00058 * @endcode 00059 */ 00060 00061 class LIS3DSH { 00062 public: 00063 /** Create a LIS3DSH object connected to the specified pins. 00064 * @param mosi SPI compatible pin used for the LIS3DSH's MOSI pin 00065 * @param miso SPI compatible pin used for the LIS3DSH's MISO pin 00066 * @param clk SPI compatible pin used for the LIS3DSH's CLK pin 00067 * @param cs DigitalOut compatible pin used for the LIS3DSH's CS pin 00068 */ 00069 LIS3DSH(PinName mosi, PinName miso, PinName clk, PinName cs); 00070 00071 /** Determines if the LIS3DSH acceleromoter can be detected. 00072 * @param 00073 * None 00074 * @return 00075 * 1 = detected; 0 = not detected. 00076 */ 00077 int Detect(void); 00078 00079 /** Write a byte of data to the LIS3DSH at a selected address. 00080 * @param addr 8-bit address to where the data should be written 00081 * @param data 8-bit data byte to write 00082 * @return 00083 * None 00084 */ 00085 void WriteReg(uint8_t addr, uint8_t data); 00086 00087 /** Read data from the LIS3DSH from a selected address. 00088 * @param 00089 * addr 8-bit address from where the data should be read 00090 * @return 00091 * Contents of the register as a 8-bit data byte. 00092 */ 00093 uint8_t ReadReg(uint8_t addr); 00094 00095 /** Reads the raw X, Y, Z values from the LIS3DSH as signed 16-bit values (int16_t). 00096 * @param X Reference to variable for the raw X value 00097 * @param Y Reference to variable for the raw Y value 00098 * @param Z Reference to variable for the raw Z value 00099 * @return 00100 * None 00101 */ 00102 void ReadData(int16_t *X, int16_t *Y, int16_t *Z); 00103 00104 /** Reads the roll angle and pitch angle from the LIS3DSH. 00105 * @param Roll Reference to variable for the roll angle (0.0 - 359.999999) 00106 * @param Pitch Reference to variable for the pitch angle (0.0 - 359.999999) 00107 * @return 00108 * None 00109 */ 00110 void ReadAngles(float *Roll, float *Pitch); 00111 00112 private: 00113 SPI _spi; 00114 DigitalOut _cs; 00115 float gToDegrees(float V, float H); 00116 }; 00117 00118 #endif
Generated on Tue Jul 12 2022 15:41:48 by
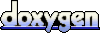