Class library for a polling-based 4x4 keypad.
Dependents: LCD kbdlcdkl25z control_onoff control_onoff ... more
Keypad.h
00001 /* Keypad Library v1.0 00002 * Copyright (c) 2016 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * This is a modified version of Riaan Ehlers' library which was written for the 00006 * Microchip PIC18 series microcontrollers. 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 */ 00026 00027 #ifndef Keypad_H 00028 #define Keypad_H 00029 00030 #include "mbed.h" 00031 00032 /** Class library for a polling-based 4x4 keypad. 00033 * 00034 * Example: 00035 * @code 00036 * #include "mbed.h" 00037 * #include "Keypad.h" 00038 * 00039 * Keypad kpad(PE_15, PE_14, PE_13, PE_12, PE_11, PE_10, PE_9, PE_8); 00040 * 00041 * int main() { 00042 * char key; 00043 * int released = 1; 00044 * 00045 * while(1){ 00046 * key = kpad.ReadKey(); //read the current key pressed 00047 * 00048 * if(key == '\0') 00049 * released = 1; //set the flag when all keys are released 00050 * 00051 * if((key != '\0') && (released == 1)) { //if a key is pressed AND previous key was released 00052 * printf("%c\n", key); 00053 * released = 0; //clear the flag to indicate that key is still pressed 00054 * } 00055 * } 00056 * } 00057 * @endcode 00058 */ 00059 00060 class Keypad { 00061 public: 00062 /** Create a Keypad object. 00063 * @param col1..4 DigitalOut (or BusOut) compatible pins for keypad Column data lines 00064 * @param row1..4 DigitalIn (or BusIn) compatible pins for keypad Row data lines 00065 */ 00066 Keypad(PinName col1, PinName col2, PinName col3, PinName col4, PinName row1, PinName row2, PinName row3, PinName row4); 00067 00068 /** Returns the letter of the key pressed. 00069 * @param 00070 * None 00071 * @return 00072 * The character of the key pressed. Returns '\0' if no key is pressed. 00073 */ 00074 char ReadKey(); 00075 00076 00077 private: 00078 BusOut _cols; 00079 BusIn _rows; 00080 }; 00081 00082 #endif
Generated on Wed Jul 13 2022 14:39:05 by
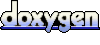