Class library for a polling-based 4x4 keypad.
Dependents: LCD kbdlcdkl25z control_onoff control_onoff ... more
Keypad.cpp
00001 #include "Keypad.h" 00002 #include "mbed.h" 00003 00004 00005 // Keypad layout: 00006 // [row][col] Col0 Col1 Col2 Col3 00007 char const kpdLayout[4][4] = {{'1' ,'2' ,'3' ,'A'}, //row0 00008 {'4' ,'5' ,'6' ,'B'}, //row1 00009 {'7' ,'8' ,'9' ,'C'}, //row2 00010 {'*' ,'0' ,'#' ,'D'}}; //row3 00011 00012 //NIBBLE LOW=0000, HIGH= 0111 1011 1101 1110 Col (x) 00013 //const char KpdInMask[4] ={0xe0,0xd0,0xb0,0x70}; 00014 const char KpdInMask[4] ={0x0e,0x0d,0x0b,0x07}; 00015 00016 //NIBBLE HIGH=1111, LOW= 0111 1011 1101 1110 Rows (y) 00017 //const char KpdOutMask[4]={0xfe,0xfd,0xfb,0xf7}; 00018 const char KpdOutMask[4]={0x0e,0x0d,0x0b,0x07}; 00019 00020 00021 Keypad::Keypad(PinName col1, PinName col2, PinName col3, PinName col4, PinName row1, PinName row2, PinName row3, PinName row4) : _cols(col1,col2,col3,col4), _rows(row1,row2,row3,row4) { } 00022 00023 char Keypad::ReadKey() { 00024 char KeyValue, Done=0; 00025 uint16_t y, x; 00026 00027 //delay_ms(ContactBounceTime); //warning no contact bounce protection 00028 //call read_key more than once with delay 00029 //between if key stay constant then key is pressed 00030 y = 0; 00031 while((y < 4) && (!Done)) 00032 { 00033 _cols = KpdOutMask[y]; //write mask value to the column outputs 00034 wait(0.01); 00035 00036 KeyValue = _rows; //read mask value from the row inputs 00037 00038 if(KeyValue == KpdInMask[0]) 00039 x = 0; 00040 else if(KeyValue == KpdInMask[1]) 00041 x = 1; 00042 else if(KeyValue == KpdInMask[2]) 00043 x = 2; 00044 else if(KeyValue == KpdInMask[3]) 00045 x = 3; 00046 else 00047 { 00048 KeyValue='\0'; //more than one key was pressed or no key in this row. 00049 x=9; 00050 } 00051 if(x != 9) 00052 { 00053 Done = 1; //valid key found 00054 KeyValue = kpdLayout[x][y]; //convert to a character eg. '1','2','3','#','*' 00055 } 00056 y++; 00057 } 00058 return(KeyValue); 00059 }
Generated on Wed Jul 13 2022 14:39:05 by
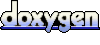