Note! This project has moved to github.com/armmbed/mbed-events
Embed:
(wiki syntax)
Show/hide line numbers
EventLoop.h
00001 /* EventLoop 00002 * 00003 * EventQueue wrapped in a thread 00004 */ 00005 #ifndef EVENT_LOOP_H 00006 #define EVENT_LOOP_H 00007 00008 #include "EventQueue.h" 00009 #include "Thread.h" 00010 00011 /** Managed queue coupled with its own thread 00012 */ 00013 class EventLoop : public EventQueue { 00014 public: 00015 /** Create an event loop without starting execution 00016 * @param priority Initial priority of the thread 00017 * (default: osPriorityNormal) 00018 * @param event_count Number of events to allow enqueueing at once 00019 * (default: 32) 00020 * @param event_context Max size of arguments passed with an event 00021 * (default: 0) 00022 * @param event_pointer Pointer to memory area to be used for events 00023 * (default: NULL) 00024 * @param stack_size Stack size (in bytes) requirements for the thread 00025 * (default: DEFAULT_STACK_SIZE) 00026 * @param stack_pointer Pointer to stack area to be used by the thread 00027 * (default: NULL) 00028 */ 00029 EventLoop(osPriority priority=osPriorityNormal, 00030 unsigned event_count=32, 00031 unsigned event_context=0, 00032 unsigned char *event_pointer=NULL, 00033 uint32_t stack_size=DEFAULT_STACK_SIZE, 00034 unsigned char *stack_pointer=NULL); 00035 00036 /** Create an event loop running in a dedicated thread 00037 * @param start True to start on construction 00038 * @param priority Initial priority of the thread 00039 * (default: osPriorityNormal) 00040 * @param event_count Number of events to allow enqueueing at once 00041 * (default: 32) 00042 * @param event_context Max size of arguments passed with an event 00043 * (default: 0) 00044 * @param event_pointer Pointer to memory area to be used for events 00045 * (default: NULL) 00046 * @param stack_size Stack size (in bytes) requirements for the thread 00047 * (default: DEFAULT_STACK_SIZE) 00048 * @param stack_pointer Pointer to stack area to be used by the thread 00049 * (default: NULL) 00050 */ 00051 EventLoop(bool start, 00052 osPriority priority=osPriorityNormal, 00053 unsigned event_count=32, 00054 unsigned event_context=0, 00055 unsigned char *event_pointer=NULL, 00056 uint32_t stack_size=DEFAULT_STACK_SIZE, 00057 unsigned char *stack_pointer=NULL); 00058 00059 /** Clean up event loop 00060 */ 00061 ~EventLoop(); 00062 00063 /** Starts an event loop running in a dedicated thread 00064 */ 00065 osStatus start(); 00066 00067 /** Stops an event loop cleanly, waiting for any currently executing events 00068 */ 00069 osStatus stop(); 00070 00071 private: 00072 static void run(const void *p); 00073 00074 bool _running; 00075 osPriority _priority; 00076 uint32_t _stack_size; 00077 unsigned char *_stack_pointer; 00078 rtos::Thread *_thread; 00079 }; 00080 00081 #endif
Generated on Wed Jul 13 2022 19:57:07 by
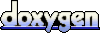