Note! This project has moved to github.com/armmbed/mbed-events
Embed:
(wiki syntax)
Show/hide line numbers
EventLoop.cpp
00001 #include "EventLoop.h" 00002 #include "rtos.h" 00003 00004 00005 EventLoop::EventLoop(osPriority priority, 00006 unsigned event_count, 00007 unsigned event_context, 00008 unsigned char *event_pointer, 00009 uint32_t stack_size, 00010 unsigned char *stack_pointer) 00011 : EventQueue(event_count, event_context, event_pointer) { 00012 _running = false; 00013 _priority = priority; 00014 _stack_size = stack_size; 00015 _stack_pointer = stack_pointer; 00016 } 00017 00018 EventLoop::EventLoop(bool start, 00019 osPriority priority, 00020 unsigned event_count, 00021 unsigned event_context, 00022 unsigned char *event_pointer, 00023 uint32_t stack_size, 00024 unsigned char *stack_pointer) 00025 : EventQueue(event_count, event_context, event_pointer) { 00026 _running = false; 00027 _priority = priority; 00028 _stack_size = stack_size; 00029 _stack_pointer = stack_pointer; 00030 00031 if (start) { 00032 EventLoop::start(); 00033 } 00034 } 00035 00036 EventLoop::~EventLoop() { 00037 stop(); 00038 } 00039 00040 osStatus EventLoop::start() { 00041 if (_running) { 00042 return osOK; 00043 } 00044 00045 _running = true; 00046 _thread = new Thread(run, this, _priority, _stack_size, _stack_pointer); 00047 if (!_thread) { 00048 return osErrorNoMemory; 00049 } 00050 00051 return osOK; 00052 } 00053 00054 osStatus EventLoop::stop() { 00055 if (!_running) { 00056 return osOK; 00057 } 00058 00059 Thread *thread = _thread; 00060 _thread = 0; 00061 00062 _running = false; 00063 while (true) { 00064 uint8_t state = thread->get_state(); 00065 if (state == Thread::Inactive || state == osErrorParameter) { 00066 break; 00067 } 00068 00069 osStatus status = Thread::yield(); 00070 if (status != osOK) { 00071 break; 00072 } 00073 } 00074 00075 delete thread; 00076 return osOK; 00077 } 00078 00079 void EventLoop::run(const void *p) { 00080 EventLoop *loop = (EventLoop *)p; 00081 00082 while (loop->_running) { 00083 loop->dispatch(0); 00084 Thread::yield(); 00085 } 00086 } 00087
Generated on Wed Jul 13 2022 19:57:07 by
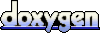