
Port from Avnet's Internet Of Things full WiGo demo: SmartConfig - WebServer - Exosite - Android sensor Fusion App
Dependencies: NVIC_set_all_priorities mbed cc3000_hostdriver_mbedsocket TEMT6200 TSI Wi-Go_eCompass_Lib_V3 WiGo_BattCharger
run_exosite.cpp
00001 /***************************************************************************** 00002 * 00003 * demo.c - CC3000 Main Demo Application 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #include "run_exosite.h" 00037 #include "Wi-Go_eCompass_Lib_V3.h" 00038 #include "mbed.h" 00039 #include "defLED.h" 00040 #include "strlib.h" 00041 00042 extern DigitalOut ledr; 00043 extern DigitalOut ledg; 00044 extern DigitalOut ledb; 00045 extern DigitalOut led1; 00046 extern DigitalOut led2; 00047 extern DigitalOut led3; 00048 00049 // local defines 00050 #define WRITE_INTERVAL 5 00051 #define EXO_BUFFER_SIZE 300 //reserve 300 bytes for our output buffer 00052 00053 typedef struct 00054 { 00055 float *p; 00056 char *s; 00057 } exo_data_ft; 00058 00059 typedef struct 00060 { 00061 int16_t *p; 00062 char *s; 00063 } exo_data_it; 00064 00065 //extern tUserFS user_info; 00066 00067 extern char requestBuffer[]; 00068 00069 extern unsigned int compass_type, seconds; 00070 00071 char exo_meta[META_SIZE]; 00072 00073 #define RX_SIZE 60 00074 #define MAC_LEN 6 00075 00076 typedef enum 00077 { 00078 CIK_LINE, 00079 HOST_LINE, 00080 CONTENT_LINE, 00081 ACCEPT_LINE, 00082 LENGTH_LINE, 00083 GETDATA_LINE, 00084 POSTDATA_LINE, 00085 VENDOR_LINE, 00086 EMPTY_LINE 00087 } lineTypes; 00088 00089 #define STR_CIK_HEADER "X-Exosite-CIK: c3c675e0601551c6d4d3230e162d62cc8bba3311\r\n" 00090 #define STR_CONTENT_LENGTH "Content-Length: " 00091 #define STR_GET_URL "GET /api:v1/stack/alias?" 00092 #define STR_HTTP " HTTP/1.1\r\n" 00093 #define STR_HOST "Host: avnet.m2.exosite.com\r\n" 00094 #define STR_ACCEPT "Accept: application/x-www-form-urlencoded; charset=utf-8\r\n" 00095 #define STR_CONTENT "Content-Type: application/x-www-form-urlencoded; charset=utf-8\r\n" 00096 #define STR_VENDOR "vendor=avnet&model=wigosmartconfig&sn=" 00097 #define STR_CRLF "\r\n" 00098 #define MY_CIK "27b9684751332589be52c8e5819e7c438f7e7479" 00099 char myCIK[] = "c3c675e0601551c6d4d3230e162d62cc8bba3311"; 00100 00101 // local functions 00102 void activate_device(TCPSocketConnection *socket); 00103 int readResponse(TCPSocketConnection *socket, char * expectedCode); 00104 long connect_to_exosite(TCPSocketConnection *socket); 00105 void sendLine(TCPSocketConnection *socket, unsigned char LINE, char * payload); 00106 void exosite_meta_write(unsigned char * write_buffer, unsigned short srcBytes, unsigned char element); 00107 void exosite_meta_read(unsigned char * read_buffer, unsigned short destBytes, unsigned char element); 00108 int Exosite_Write(TCPSocketConnection *socket, char * pbuf, unsigned char bufsize); 00109 int Exosite_Read(char * palias, char * pbuf, unsigned char bufsize); 00110 int Exosite_Init(TCPSocketConnection *socket); 00111 00112 // global variables 00113 static unsigned char exositeWriteFailures = 0; 00114 00115 // exported functions 00116 00117 // externs 00118 extern char *itoa(int n, char *s, int b); 00119 00120 extern axis6_t axis6; 00121 extern int secondFlag; 00122 extern int server_running; 00123 00124 /** \brief Definition of data packet to be sent by server */ 00125 unsigned char dataPacket[] = { '\r', 0xBE, 128, 128, 128, 70, 36, 0xEF }; 00126 char activeCIK[41]; 00127 00128 exo_data_ft exo_fdata[] = { 00129 &axis6.fGax, "acc_x=%f&", 00130 &axis6.fGay, "acc_y=%f&", 00131 &axis6.fGaz, "acc_z=%f&", 00132 &axis6.fUTmx, "mag_x=%f&", 00133 &axis6.fUTmy, "mag_y=%f&", 00134 &axis6.fUTmz, "mag_z=%f&", 00135 &axis6.q0, "q_w=%f&", 00136 &axis6.q1, "q_x=%f&", 00137 &axis6.q2, "q_y=%f&", 00138 &axis6.q3, "q_z=%f&", 00139 0, 0 }; 00140 00141 exo_data_it exo_idata[] = { 00142 &axis6.roll, "roll=%d&", 00143 &axis6.pitch, "pitch=%d&", 00144 &axis6.yaw, "yaw=%d&", 00145 &axis6.alt, "alt=%d&", 00146 &axis6.temp, "temp=%d&", 00147 &axis6.light, "light=%d&", 00148 0, 0 00149 }; 00150 00151 //***************************************************************************** 00152 // 00153 //! main 00154 //! 00155 //! \param None 00156 //! 00157 //! \return none 00158 //! 00159 //! \brief The main loop is executed here 00160 // 00161 //***************************************************************************** 00162 void run_exosite(TCPSocketConnection *socket) 00163 { 00164 int value; 00165 exo_data_it *tsp; 00166 exo_data_ft *tspf; 00167 00168 printf("\nConnecting to Exosite\n"); 00169 //user_info.validCIK = 0; // uncomment this to force provisioning every time, only used for debug 00170 00171 if(!user_info.validCIK) 00172 { 00173 printf("Activating Wi-Go System on Exosite\n"); 00174 uint8_t myMAC[8]; 00175 wifi.get_mac_address(myMAC); 00176 printf(" MAC address %02x:%02x:%02x:%02x:%02x:%02x \r\n \r\n", myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00177 wait_ms(100); 00178 printf("Enter the device MAC on your Exosite device portal to prepare\n"); 00179 wait_ms(100); 00180 printf("the system for provisioning your Wi-Go system onto Exosite\n"); 00181 wait_ms(100); 00182 printf("Hit any key to continue.....\n"); 00183 getchar(); 00184 Exosite_Init(socket); 00185 } 00186 00187 for(value=0 ;value < CIK_LENGTH ; value++) activeCIK[value] = user_info.CIK[value]; 00188 activeCIK[value] = NULL; 00189 // Main Loop 00190 while (1) 00191 { 00192 while(!secondFlag) /*__wfi()*/; 00193 secondFlag = 0; 00194 LED_D2_ON; 00195 00196 // Build string 00197 requestBuffer[0] = 0; 00198 tsp = &exo_idata[0]; 00199 tspf = &exo_fdata[0]; 00200 while(tsp->p) 00201 { 00202 sprintf( requestBuffer + strlen(requestBuffer), tsp->s, *tsp->p); 00203 tsp++; 00204 } 00205 while(tspf->p) 00206 { 00207 sprintf( requestBuffer + strlen(requestBuffer), tspf->s, *tspf->p); 00208 tspf++; 00209 } 00210 sprintf( requestBuffer + strlen(requestBuffer), "time=%d\r\n", axis6.timestamp); 00211 00212 if(strlen(requestBuffer) > 300) printf("Buffer size= %d too small!!!", strlen(requestBuffer)); 00213 //printf("Buffer size= %d\n", strlen(requestBuffer)); 00214 value = Exosite_Write(socket, requestBuffer, strlen(requestBuffer)); //write all sensor values to the cloud 00215 LED_D2_OFF; 00216 if(value == -1) 00217 { 00218 LED_D3_OFF; 00219 wifi.stop(); 00220 if (wifi.connect() == -1) 00221 printf("Failed to connect. Please verify connection details and try again. \r\n"); 00222 else 00223 { 00224 printf("Connected - IP address: %s \r\n",wifi.getIPAddress()); 00225 LED_D3_ON; 00226 } 00227 } 00228 } 00229 } 00230 00231 //***************************************************************************** 00232 // 00233 //! Exosite_Init 00234 //! 00235 //! \param None 00236 //! 00237 //! \return 0 success; -1 failure 00238 //! 00239 //! \brief The function initializes the cloud connection to Exosite 00240 // 00241 //***************************************************************************** 00242 int Exosite_Init(TCPSocketConnection *socket) 00243 { 00244 char strBuf[META_MARK_SIZE]; 00245 const unsigned char meta_server_ip[6] = {173,255,209,28,0,80}; 00246 uint8_t mac_status = 1; 00247 uint8_t myMAC[8]; 00248 int i; 00249 char tempCIK[CIK_LENGTH]; 00250 00251 //check our meta mark - if it isn't there, we wipe the meta structure 00252 exosite_meta_read((unsigned char *)strBuf, META_MARK_SIZE, META_MARK); 00253 if (strncmp(strBuf, EXOMARK, META_MARK_SIZE)) 00254 { 00255 memset(exo_meta, 0, META_SIZE); //erase the information currently in meta 00256 exosite_meta_write((unsigned char *)meta_server_ip, 6, META_SERVER); //store server IP 00257 exosite_meta_write((unsigned char *)EXOMARK, META_MARK_SIZE, META_MARK); //store exosite mark 00258 } 00259 00260 while(mac_status) mac_status = wifi.get_mac_address(myMAC); 00261 exosite_meta_write((unsigned char *)myMAC, 17, META_UUID); 00262 00263 //setup some of our globals for operation 00264 exositeWriteFailures = 0; 00265 00266 //exosite Re-init : Called after Init has been ran in the past, but maybe 00267 // comms were down and we have to keep trying... 00268 activate_device(socket); //the moment of truth - can this device provision with the Exosite cloud?... 00269 exosite_meta_read((unsigned char *)tempCIK, CIK_LENGTH, META_CIK); //sanity check on the CIK 00270 for (i = 0; i < CIK_LENGTH; i++) 00271 { 00272 if (!(tempCIK[i] >= 'a' && tempCIK[i] <= 'f' || tempCIK[i] >= '0' && tempCIK[i] <= '9')) 00273 { 00274 return -1; 00275 } 00276 } 00277 for(i=0;i<CIK_LENGTH;i++) user_info.CIK[i] = tempCIK[i]; 00278 user_info.validCIK = 1; 00279 wifi._nvmem.write( NVMEM_USER_FILE_1_FILEID, sizeof(user_info), 0, (unsigned char *) &user_info); 00280 return 0; 00281 } 00282 00283 //***************************************************************************** 00284 // 00285 //! Exosite_Write 00286 //! 00287 //! \param pbuf - string buffer containing data to be sent 00288 //! bufsize - number of bytes to send 00289 //! 00290 //! \return 0 success; -1 failure 00291 //! 00292 //! \brief The function writes data to Exosite 00293 // 00294 //***************************************************************************** 00295 int Exosite_Write(TCPSocketConnection *socket, char * pbuf, unsigned char bufsize) 00296 { 00297 char strBuf[10]; 00298 long sock = -1; 00299 00300 sock = connect_to_exosite(socket); 00301 if(sock == -1) return(sock); 00302 00303 // This is an example write POST... 00304 // s.send('POST /api:v1/stack/alias HTTP/1.1\r\n') 00305 // s.send('Host: m2.exosite.com\r\n') 00306 // s.send('X-Exosite-CIK: 5046454a9a1666c3acfae63bc854ec1367167815\r\n') 00307 // s.send('Content-Type: application/x-www-form-urlencoded; charset=utf-8\r\n') 00308 // s.send('Content-Length: 6\r\n\r\n') 00309 // s.send('temp=2') 00310 00311 sprintf(strBuf, "%d", (int)bufsize); //make a string for length 00312 00313 sendLine(socket, POSTDATA_LINE, "/onep:v1/stack/alias"); 00314 sendLine(socket, HOST_LINE, NULL); 00315 sendLine(socket, CIK_LINE, NULL); 00316 sendLine(socket, CONTENT_LINE, NULL); 00317 sendLine(socket, LENGTH_LINE, strBuf); 00318 //printf("Data=%s\n", pbuf); 00319 socket->send_all(pbuf, bufsize); //alias=value 00320 wifi._event.hci_unsolicited_event_handler(); 00321 00322 if (0 == readResponse(socket, "204")) 00323 { 00324 exositeWriteFailures = 0; 00325 } 00326 else 00327 { 00328 exositeWriteFailures++; 00329 } 00330 00331 socket->close(); 00332 00333 if (exositeWriteFailures > 5) 00334 { 00335 // sometimes transport connect works even if no connection... 00336 printf("ERROR %d\r\n", EXO_ERROR_WRITE); 00337 } 00338 00339 if (!exositeWriteFailures) 00340 { 00341 //printf("ShowUIcode %d\n", EXO_CLIENT_RW); 00342 return 0; // success 00343 } 00344 return 0; 00345 } 00346 00347 00348 //***************************************************************************** 00349 // 00350 //! activate_device 00351 //! 00352 //! \param none 00353 //! 00354 //! \return none 00355 //! 00356 //! \brief Calls activation API - if successful, it saves the returned 00357 //! CIK to non-volatile 00358 // 00359 //***************************************************************************** 00360 void activate_device(TCPSocketConnection *socket) 00361 { 00362 long sock = -1; 00363 volatile int length; 00364 char strLen[5]; 00365 00366 while (sock < 0) 00367 sock = connect_to_exosite(socket); 00368 printf("Activating Device\n"); 00369 00370 length = strlen(STR_VENDOR) + META_UUID_SIZE; 00371 itoa(length, strLen, 10); //make a string for length 00372 00373 sendLine(socket, POSTDATA_LINE, "/provision/activate"); 00374 sendLine(socket, HOST_LINE, NULL); 00375 sendLine(socket, CONTENT_LINE, NULL); 00376 sendLine(socket, LENGTH_LINE, strLen); 00377 sendLine(socket, VENDOR_LINE, NULL); 00378 00379 if (0 == readResponse(socket, "200")) 00380 { 00381 char strBuf[RX_SIZE]; 00382 unsigned char strLen, len; 00383 char *p; 00384 unsigned char crlf = 0; 00385 unsigned char ciklen = 0; 00386 char NCIK[CIK_LENGTH]; 00387 00388 do 00389 { 00390 strLen = socket->receive(strBuf, RX_SIZE); 00391 len = strLen; 00392 p = strBuf; 00393 // Find 4 consecutive \r or \n - should be: \r\n\r\n 00394 while (0 < len && 4 > crlf) 00395 { 00396 if ('\r' == *p || '\n' == *p) 00397 { 00398 ++crlf; 00399 } 00400 else 00401 { 00402 crlf = 0; 00403 } 00404 ++p; 00405 --len; 00406 } 00407 00408 // The body is the CIK 00409 if (0 < len && 4 == crlf && CIK_LENGTH > ciklen) 00410 { 00411 // TODO, be more robust - match Content-Length header value to CIK_LENGTH 00412 unsigned char need, part; 00413 need = CIK_LENGTH - ciklen; 00414 part = need < len ? need : len; 00415 strncpy(NCIK + ciklen, p, part); 00416 ciklen += part; 00417 } 00418 } while (RX_SIZE == strLen); 00419 00420 if (CIK_LENGTH == ciklen) 00421 { 00422 exosite_meta_write((unsigned char *)NCIK, CIK_LENGTH, META_CIK); 00423 } 00424 } 00425 else 00426 { 00427 printf("Activation failed\n"); 00428 getchar(); 00429 } 00430 socket->close(); 00431 } 00432 00433 //***************************************************************************** 00434 // 00435 //! connect_to_exosite 00436 //! 00437 //! \param None 00438 //! 00439 //! \return socket handle 00440 //! 00441 //! \brief Establishes a connection with the Exosite API server 00442 // 00443 //***************************************************************************** 00444 long connect_to_exosite(TCPSocketConnection *socket) 00445 { 00446 static unsigned char connectRetries = 0; 00447 long sock; 00448 00449 if (connectRetries++ > 5) 00450 { 00451 connectRetries = 0; 00452 printf("ERROR %d\r\n", EXO_ERROR_CONNECT); 00453 } 00454 00455 const char* ECHO_SERVER_ADDRESS = "173.255.209.28"; //TODO - use DNS or check m2.exosite.com/ip to check for updates 00456 const int ECHO_SERVER_PORT = 80; 00457 00458 unsigned char server[META_SERVER_SIZE]; 00459 exosite_meta_read(server, META_SERVER_SIZE, META_SERVER); 00460 00461 sock = socket->connect(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT); 00462 if(sock < 0) 00463 { 00464 printf("Connection to server failed\n"); 00465 socket->close(); 00466 wait_ms(200); 00467 return -1; 00468 } 00469 else 00470 { 00471 connectRetries = 0; 00472 //printf("ShowUIcode %d\n", EXO_SERVER_CONNECTED); 00473 } 00474 00475 // Success 00476 //printf("success\n"); 00477 return sock; 00478 } 00479 00480 00481 //***************************************************************************** 00482 // 00483 //! readResponse 00484 //! 00485 //! \param socket handle, pointer to expected HTTP response code 00486 //! 00487 //! \return 0 if match, -1 if no match 00488 //! 00489 //! \brief Reads first 12 bytes of HTTP response and extracts the 3 byte code 00490 // 00491 //***************************************************************************** 00492 int readResponse(TCPSocketConnection *socket, char * code) 00493 { 00494 char rxBuf[12]; 00495 unsigned char rxLen; 00496 rxLen = socket->receive(rxBuf, 12); 00497 //PROBLEM : more than 12 chars are printed 00498 printf("rec %s\n", rxBuf); 00499 if (12 == rxLen && code[0] == rxBuf[9] && code[1] == rxBuf[10] && code[2] == rxBuf[11]) 00500 { 00501 return 0; 00502 } 00503 00504 return -1; 00505 } 00506 00507 00508 //***************************************************************************** 00509 // 00510 //! sendLine 00511 //! 00512 //! \param Which line type 00513 //! 00514 //! \return socket handle 00515 //! 00516 //! \brief Sends data out the socket 00517 // 00518 //***************************************************************************** 00519 void sendLine(TCPSocketConnection *socket, unsigned char LINE, char * payload) 00520 { 00521 char strBuf[80]; 00522 unsigned char strLen = 0; 00523 00524 switch(LINE) 00525 { 00526 case CIK_LINE: 00527 sprintf(strBuf, "X-Exosite-CIK: %s\r\n", activeCIK); 00528 strLen = strlen(strBuf); 00529 //strLen = 57; 00530 //memcpy(strBuf,STR_CIK_HEADER,strLen); 00531 //exosite_meta_read((unsigned char *)&strBuf[strLen], CIK_LENGTH, META_CIK); 00532 //strLen += CIK_LENGTH; 00533 //memcpy(&strBuf[strLen],STR_CRLF, 2); 00534 //strLen += 2; 00535 break; 00536 case HOST_LINE: 00537 strLen = 28; 00538 memcpy(strBuf,STR_HOST,strLen); 00539 strBuf[strLen] = NULL; 00540 break; 00541 case CONTENT_LINE: 00542 strLen = 64; 00543 memcpy(strBuf,STR_CONTENT,strLen); 00544 strBuf[strLen] = NULL; 00545 break; 00546 case ACCEPT_LINE: 00547 strLen = 58; 00548 memcpy(strBuf,STR_ACCEPT,strLen); 00549 memcpy(&strBuf[strLen],payload, strlen(payload)); 00550 strLen += strlen(payload); 00551 break; 00552 case LENGTH_LINE: // Content-Length: NN 00553 strLen = 16; 00554 memcpy(strBuf,STR_CONTENT_LENGTH,strLen); 00555 memcpy(&strBuf[strLen],payload, strlen(payload)); 00556 strLen += strlen(payload); 00557 memcpy(&strBuf[strLen],STR_CRLF, 2); 00558 strLen += 2; 00559 memcpy(&strBuf[strLen],STR_CRLF, 2); 00560 strLen += 2; 00561 strBuf[strLen] = NULL; 00562 break; 00563 case GETDATA_LINE: 00564 strLen = 24; 00565 memcpy(strBuf,STR_GET_URL,strLen); 00566 memcpy(&strBuf[strLen],payload, strlen(payload)); 00567 strLen += strlen(payload); 00568 memcpy(&strBuf[strLen],STR_HTTP, 12); 00569 strLen += 12; 00570 break; 00571 case VENDOR_LINE: 00572 strLen = strlen(STR_VENDOR); 00573 memcpy(strBuf, STR_VENDOR, strLen); 00574 exosite_meta_read((unsigned char *)&strBuf[strLen], META_UUID_SIZE, META_UUID); 00575 strLen += META_UUID_SIZE; 00576 strBuf[strLen] = NULL; 00577 break; 00578 case POSTDATA_LINE: 00579 strLen = 5; 00580 memcpy(strBuf,"POST ", strLen); 00581 memcpy(&strBuf[strLen],payload, strlen(payload)); 00582 strLen += strlen(payload); 00583 memcpy(&strBuf[strLen],STR_HTTP, 12); 00584 strLen += 12; 00585 strBuf[strLen] = NULL; 00586 break; 00587 case EMPTY_LINE: 00588 strLen = 2; 00589 memcpy(strBuf,STR_CRLF,strLen); 00590 break; 00591 default: 00592 break; 00593 } 00594 //printf("sendLine: %s\n", strBuf); 00595 socket->send_all(strBuf, strLen); 00596 wifi._event.hci_unsolicited_event_handler(); 00597 return; 00598 } 00599 00600 //***************************************************************************** 00601 // 00602 //! exosite_meta_write 00603 //! 00604 //! \param write_buffer - string buffer containing info to write to meta; 00605 //! srcBytes - size of string in bytes; element - item from 00606 //! MetaElements enum. 00607 //! 00608 //! \return None 00609 //! 00610 //! \brief Writes specific meta information to meta memory. 00611 // 00612 //***************************************************************************** 00613 void exosite_meta_write(unsigned char * write_buffer, unsigned short srcBytes, unsigned char element) 00614 { 00615 exosite_meta * meta_info = 0; 00616 00617 //TODO - do not write if the data already there is identical... 00618 00619 switch (element) 00620 { 00621 case META_CIK: 00622 if (srcBytes > META_CIK_SIZE) return; 00623 memcpy((char *)(exo_meta + (int)meta_info->cik), write_buffer, srcBytes); //store CIK 00624 break; 00625 case META_SERVER: 00626 if (srcBytes > META_SERVER_SIZE) return; 00627 memcpy((char *)(exo_meta + (int)meta_info->server), write_buffer, srcBytes); //store server IP 00628 break; 00629 case META_MARK: 00630 if (srcBytes > META_MARK_SIZE) return; 00631 memcpy((char *)(exo_meta + (int)meta_info->mark), write_buffer, srcBytes); //store exosite mark 00632 break; 00633 case META_UUID: 00634 if (srcBytes > META_UUID_SIZE) return; 00635 memcpy((char *)(exo_meta + (int)meta_info->uuid), write_buffer, srcBytes); //store UUID 00636 break; 00637 case META_MFR: 00638 if (srcBytes > META_MFR_SIZE) return; 00639 memcpy((char *)(exo_meta + (int)meta_info->mfr), write_buffer, srcBytes); //store manufacturing info 00640 break; 00641 case META_NONE: 00642 default: 00643 break; 00644 } 00645 return; 00646 } 00647 00648 00649 //***************************************************************************** 00650 // 00651 //! exosite_meta_read 00652 //! 00653 //! \param read_buffer - string buffer to receive element data; destBytes - 00654 //! size of buffer in bytes; element - item from MetaElements enum. 00655 //! 00656 //! \return None 00657 //! 00658 //! \brief Writes specific meta information to meta memory. 00659 // 00660 //***************************************************************************** 00661 void exosite_meta_read(unsigned char * read_buffer, unsigned short destBytes, unsigned char element) 00662 { 00663 exosite_meta * meta_info = 0; 00664 00665 switch (element) 00666 { 00667 case META_CIK: 00668 if (destBytes < META_CIK_SIZE) return; 00669 memcpy(read_buffer, (char *)(exo_meta + (int)meta_info->cik), destBytes); //read CIK 00670 break; 00671 case META_SERVER: 00672 if (destBytes < META_SERVER_SIZE) return; 00673 memcpy(read_buffer, (char *)(exo_meta + (int)meta_info->server), destBytes); //read server IP 00674 break; 00675 case META_MARK: 00676 if (destBytes < META_MARK_SIZE) return; 00677 memcpy(read_buffer, (char *)(exo_meta + (int)meta_info->mark), destBytes); //read exosite mark 00678 break; 00679 case META_UUID: 00680 if (destBytes < META_UUID_SIZE) return; 00681 memcpy(read_buffer, (char *)(exo_meta + (int)meta_info->uuid), destBytes); //read exosite mark 00682 break; 00683 case META_MFR: 00684 if (destBytes < META_MFR_SIZE) return; 00685 memcpy(read_buffer, (char *)(exo_meta + (int)meta_info->mfr), destBytes); //read exosite mark 00686 break; 00687 case META_NONE: 00688 default: 00689 break; 00690 } 00691 return; 00692 }
Generated on Thu Jul 14 2022 00:58:11 by
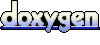