
Port from Avnet's Internet Of Things full WiGo demo: SmartConfig - WebServer - Exosite - Android sensor Fusion App
Dependencies: NVIC_set_all_priorities mbed cc3000_hostdriver_mbedsocket TEMT6200 TSI Wi-Go_eCompass_Lib_V3 WiGo_BattCharger
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "cc3000.h" 00018 #include "main.h" 00019 #include "TCPSocketConnection.h" 00020 #include "TCPSocketServer.h" 00021 00022 using namespace mbed_cc3000; 00023 00024 tUserFS user_info; 00025 00026 /* cc3000 module declaration specific for user's board. Check also init() */ 00027 #if (MY_BOARD == WIGO) 00028 00029 #include "I2C_busreset.h" 00030 #include "defLED.h" 00031 #include "TSISensor.h" 00032 #include "TEMT6200.h" 00033 #include "WiGo_BattCharger.h" 00034 #include "MMA8451Q.h" 00035 #include "MAG3110.h" 00036 #include "MPL3115A2.h" 00037 #include "Wi-Go_eCompass_Lib_V3.h" 00038 #include "demo.h" 00039 #include "doTCPIP.h" 00040 #include "run_exosite.h" 00041 00042 #define FCOUNTSPERG 4096.0F // sensor specific: MMA8451 provide 4096 counts / g in 2g mode 00043 #define FCOUNTSPERUT 10.0F // sensor specific: MAG3110 provide 10 counts / uT 00044 00045 #define BATT_0 0.53 00046 #define BATT_100 0.63 00047 00048 DigitalOut ledr (LED_RED); 00049 DigitalOut ledg (LED_GREEN); 00050 DigitalOut ledb (LED_BLUE); 00051 DigitalOut led1 (PTB8); 00052 DigitalOut led2 (PTB9); 00053 DigitalOut led3 (PTB10); 00054 00055 cc3000 wifi(PTA16, PTA13, PTD0, SPI(PTD2, PTD3, PTC5), "", "", NONE, true); 00056 TCPSocketConnection socket; 00057 00058 Serial pc(USBTX, USBRX); 00059 00060 // Slide sensor 00061 TSISensor tsi; 00062 00063 // Systick 00064 Ticker systick; 00065 // Variable checked in the systick handler 00066 // Code in the systick handler is only processed when Systick_Allowed = 1 00067 bool Systick_Allowed = 1; 00068 00069 // Ambient light sensor : PTD5 = enable, PTB0 = analog input 00070 TEMT6200 ambi(PTD5, PTB0); 00071 00072 //Wi-Go battery charger control 00073 WiGo_BattCharger Batt(CHRG_EN1, CHRG_EN2, CHRG_SNS_EN, CHRG_SNS, CHRG_POK, CHRG_CHG); 00074 00075 // Accelerometer 00076 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00077 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00078 00079 // Magnetometer 00080 #define MAG3110_I2C_ADDRESS (0x0e<<1) 00081 MAG3110 mag(PTE0, PTE1, MAG3110_I2C_ADDRESS); 00082 00083 // altimeter-Pressure-Temperature (apt) 00084 #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00085 MPL3115A2 apt( PTE0, PTE1, MPL3115A2_I2C_ADDRESS); 00086 00087 volatile int secondFlag; 00088 volatile int HsecondFlag; 00089 unsigned int seconds; 00090 unsigned int compass_type; 00091 unsigned short adc_sample3; 00092 float fcountperg = 1.0F / FCOUNTSPERG; 00093 float fcountperut = 1.0F / FCOUNTSPERUT; 00094 volatile unsigned char newData; 00095 volatile int server_running; 00096 axis6_t axis6; 00097 int do_mDNS = 0; 00098 //Device name - used for Smart config in order to stop the Smart phone configuration process 00099 char DevServname[] = "CC3000"; 00100 00101 void initLEDs(void) 00102 { 00103 RED_OFF; 00104 GREEN_OFF; 00105 BLUE_OFF; 00106 LED_D1_OFF; 00107 LED_D2_OFF; 00108 LED_D3_OFF; 00109 } 00110 00111 void GreenStop(void) 00112 { 00113 RED_OFF; GREEN_OFF; BLUE_OFF; 00114 while(1) 00115 { 00116 GREEN_ON; 00117 secondFlag = 0; 00118 while(!secondFlag); 00119 GREEN_OFF; 00120 secondFlag = 0; 00121 while(!secondFlag); 00122 } 00123 } 00124 00125 void accel_read(void) 00126 { 00127 signed short resultx, resulty, resultz; 00128 if(acc.isDataAvailable()) 00129 { 00130 resultx = acc.readReg(0x01)<<8; 00131 resultx |= acc.readReg(0x02); 00132 resultx = resultx >> 2; 00133 resulty = acc.readReg(0x03)<<8; 00134 resulty |= acc.readReg(0x04); 00135 resulty = resulty >> 2; 00136 resultz = acc.readReg(0x05)<<8; 00137 resultz |= acc.readReg(0x06); 00138 resultz = resultz >> 2; 00139 if(compass_type == NED_COMPASS) 00140 { 00141 axis6.acc_x = resultx; 00142 axis6.acc_y = -1 * resulty; // multiple by -1 to compensate for PCB layout 00143 axis6.acc_z = resultz; 00144 } 00145 if(compass_type == ANDROID_COMPASS) 00146 { 00147 axis6.acc_x = resulty; // 00148 axis6.acc_y = -1 * resultx; 00149 axis6.acc_z = resultz; 00150 } 00151 if(compass_type == WINDOWS_COMPASS) 00152 { 00153 axis6.acc_x = -1 * resulty; // 00154 axis6.acc_y = resultx; 00155 axis6.acc_z = resultz; 00156 } 00157 axis6.fax = axis6.acc_x; 00158 axis6.fay = axis6.acc_y; 00159 axis6.faz = axis6.acc_z; 00160 axis6.fGax = axis6.fax * fcountperg; 00161 axis6.fGay = axis6.fay * fcountperg; 00162 axis6.fGaz = axis6.faz * fcountperg; 00163 } 00164 } 00165 00166 void readTempAlt(void) // We don't use the fractional data 00167 { 00168 unsigned char raw_data[2]; 00169 if(apt.getAltimeterRaw(&raw_data[0])) 00170 axis6.alt = ((raw_data[0] << 8) | raw_data[1]); 00171 if(apt.getTemperatureRaw(&raw_data[0])) 00172 axis6.temp = raw_data[0]; 00173 } 00174 00175 void readCompass( void ) 00176 { 00177 if(mag.isDataAvailable()) 00178 { 00179 uint8_t mx_msb, my_msb, mz_msb; 00180 uint8_t mx_lsb, my_lsb, mz_lsb; 00181 00182 mx_msb = mag.readReg(0x01); 00183 mx_lsb = mag.readReg(0x02); 00184 my_msb = mag.readReg(0x03); 00185 my_lsb = mag.readReg(0x04); 00186 mz_msb = mag.readReg(0x05); 00187 mz_lsb = mag.readReg(0x06); 00188 00189 if(compass_type == NED_COMPASS) 00190 { 00191 axis6.mag_y = (((mx_msb << 8) | mx_lsb)); // x & y swapped to compensate for PCB layout 00192 axis6.mag_x = (((my_msb << 8) | my_lsb)); 00193 axis6.mag_z = (((mz_msb << 8) | mz_lsb)); 00194 } 00195 if(compass_type == ANDROID_COMPASS) 00196 { 00197 axis6.mag_x = (((mx_msb << 8) | mx_lsb)); 00198 axis6.mag_y = (((my_msb << 8) | my_lsb)); 00199 axis6.mag_z = -1 * (((mz_msb << 8) | mz_lsb)); // negate to reverse axis of Z to conform to Android coordinate system 00200 } 00201 if(compass_type == WINDOWS_COMPASS) 00202 { 00203 axis6.mag_x = (((mx_msb << 8) | mx_lsb)); 00204 axis6.mag_y = (((my_msb << 8) | my_lsb)); 00205 axis6.mag_z = -1 * (((mz_msb << 8) | mz_lsb)); 00206 } 00207 axis6.fmx = axis6.mag_x; 00208 axis6.fmy = axis6.mag_y; 00209 axis6.fmz = axis6.mag_z; 00210 axis6.fUTmx = axis6.fmx * fcountperut; 00211 axis6.fUTmy = axis6.fmy * fcountperut; 00212 axis6.fUTmz = axis6.fmz * fcountperut; 00213 } 00214 } 00215 00216 void axis6Print(void) 00217 { 00218 char *compass_points[9] = {"North", "N-East", "East", "S-East", "South", "S-West", "West", "N-West", "North"}; 00219 signed short compass_bearing = (axis6.compass + 23) / 45; 00220 printf("Compass : Roll=%-d Pitch=%-d Yaw=%-d [%s]\r\n", axis6.roll, axis6.pitch, axis6.yaw, compass_points[compass_bearing]); 00221 printf("Accel : X= %1.2f, Y= %1.2f, Z= %1.2f\r\n", axis6.fGax, axis6.fGay, axis6.fGaz); 00222 printf("Magneto : X= %3.1f, Y= %3.1f, Z= %3.1f\r\n\r\n", axis6.fUTmx, axis6.fUTmy, axis6.fUTmz); 00223 } 00224 00225 void set_dir_LED(void) 00226 { 00227 RED_OFF; GREEN_OFF; BLUE_OFF; 00228 00229 if((axis6.compass >= 353) || (axis6.compass <= 7)) 00230 { 00231 GREEN_ON; 00232 } 00233 else 00234 { 00235 GREEN_OFF; 00236 } 00237 if(((axis6.compass >= 348) && (axis6.compass <= 357)) || ((axis6.compass >= 3) && (axis6.compass <= 12))) 00238 { 00239 BLUE_ON; 00240 } 00241 else 00242 { 00243 BLUE_OFF; 00244 } 00245 if((axis6.compass >= 348) || (axis6.compass <= 12)) return; 00246 if(((axis6.compass >= 268) && (axis6.compass <= 272)) || ((axis6.compass >= 88) && (axis6.compass <= 92))) 00247 { 00248 RED_ON; 00249 return; 00250 } 00251 if((axis6.compass >= 178) && (axis6.compass <= 182)) 00252 { 00253 BLUE_ON; 00254 RED_ON; 00255 return; 00256 } 00257 } 00258 00259 void SysTick_Handler(void) 00260 { 00261 static unsigned int ttt = 1; 00262 int ts; 00263 if(Systick_Allowed) 00264 { 00265 ts = ttt & 0x1; 00266 if(ts == 0) 00267 { 00268 accel_read(); 00269 readCompass(); 00270 } 00271 if(ts == 1) 00272 { 00273 run_eCompass(); 00274 newData = 1; // a general purpose flag for things that need to synch to the ISR 00275 axis6.timestamp++; 00276 if(!server_running) set_dir_LED(); // Set the LEDs based on direction when nothing else is usng them 00277 } 00278 if(ttt == 50) 00279 { 00280 LED_D1_ON; 00281 if(seconds && (seconds < 15)) calibrate_eCompass(); 00282 readTempAlt(); 00283 axis6.light = ambi.readRaw(); // Light Sensor 00284 HsecondFlag = 1; // A general purpose flag for things that need to happen every 500ms 00285 } 00286 if(ttt >= 100) 00287 { 00288 LED_D1_OFF; 00289 ttt = 1; 00290 calibrate_eCompass(); 00291 Batt.sense_en(1); 00292 adc_sample3 = Batt.level(); 00293 Batt.sense_en(0); 00294 secondFlag = 1; // A general purpose flag for things that need to happen once a second 00295 HsecondFlag = 1; 00296 seconds++; 00297 if(!(seconds & 0x1F)) do_mDNS = 1; 00298 } else ttt++; 00299 } 00300 } 00301 /*void SysTick_Handler(void) 00302 { 00303 static unsigned int ttt = 1; 00304 int ts; 00305 ts = ttt & 0x3; 00306 if(ts == 2) readCompass(); 00307 if(ts == 1) accel_read(); 00308 if(ts == 3) 00309 { 00310 run_eCompass(); 00311 newData = 1; // a general purpose flag for things that need to synch to the ISR 00312 axis6.timestamp++; 00313 if(!server_running) set_dir_LED(); // Set the LEDs based on direction when nothing else is usng them 00314 } 00315 if(ttt == 100)//systick = 0.005 : 100 - systick = 0.025 : 20 00316 { 00317 LED_D1_ON; 00318 if(seconds && (seconds < 15)) calibrate_eCompass(); 00319 readTempAlt(); 00320 axis6.light = ambi.readRaw(); // Light Sensor 00321 HsecondFlag = 1; // A general purpose flag for things that need to happen every 500ms 00322 } 00323 if(ttt >= 200)//systick = 0.005 : 200 - systick = 0.025 : 40 00324 { 00325 LED_D1_OFF; 00326 ttt = 1; 00327 calibrate_eCompass(); 00328 Batt.sense_en(1); 00329 adc_sample3 = Batt.level(); 00330 Batt.sense_en(0); 00331 secondFlag = 1; // A general purpose flag for things that need to happen once a second 00332 HsecondFlag = 1; 00333 seconds++; 00334 if(!(seconds & 0x1F)) do_mDNS = 1; 00335 } else ttt++; 00336 }*/ 00337 00338 #elif (MY_BOARD == WIFI_DIPCORTEX) 00339 cc3000 wifi(p28, p27, p30, SPI(p21, p14, p37), PIN_INT0_IRQn); 00340 Serial pc(UART_TX, UART_RX); 00341 #else 00342 00343 #endif 00344 00345 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 00346 const uint8_t smartconfigkey[] = {0x73,0x6d,0x61,0x72,0x74,0x63,0x6f,0x6e,0x66,0x69,0x67,0x41,0x45,0x53,0x31,0x36}; 00347 #else 00348 const uint8_t smartconfigkey = 0; 00349 #endif 00350 00351 /** 00352 * \brief Print cc3000 information 00353 * \param none 00354 * \return none 00355 */ 00356 void print_cc3000_info() { 00357 uint8_t myMAC[8]; 00358 uint8_t spVER[5]; 00359 wifi._nvmem.read_sp_version(spVER); 00360 printf("SP Version (TI) : %d %d %d %d %d\r\n", spVER[0], spVER[1], spVER[2], spVER[3], spVER[4]); 00361 printf("MAC address + cc3000 info \r\n"); 00362 wifi.get_user_file_info((uint8_t *)&user_info, sizeof(user_info)); 00363 wifi.get_mac_address(myMAC); 00364 printf(" MAC address %02x:%02x:%02x:%02x:%02x:%02x \r\n \r\n", myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00365 00366 printf(" FTC %i \r\n",user_info.FTC); 00367 printf(" PP_version %i.%i \r\n",user_info.PP_version[0], user_info.PP_version[1]); 00368 printf(" SERV_PACK %i.%i \r\n",user_info.SERV_PACK[0], user_info.SERV_PACK[1]); 00369 printf(" DRV_VER %i.%i.%i \r\n",user_info.DRV_VER[0], user_info.DRV_VER[1], user_info.DRV_VER[2]); 00370 printf(" FW_VER %i.%i.%i \r\n",user_info.FW_VER[0], user_info.FW_VER[1], user_info.FW_VER[2]); 00371 } 00372 00373 /** 00374 * \brief Connect to SSID with a timeout 00375 * \param ssid Name of SSID 00376 * \param key Password 00377 * \param sec_mode Security mode 00378 * \return none 00379 */ 00380 void connect_to_ssid(char *ssid, char *key, unsigned char sec_mode) { 00381 printf("Connecting to SSID: %s. Timeout is 10s. \r\n",ssid); 00382 if (wifi.connect_to_AP((uint8_t *)ssid, (uint8_t *)key, sec_mode) == true) { 00383 printf(" Connected. \r\n"); 00384 } else { 00385 printf(" Connection timed-out (error). Please restart. \r\n"); 00386 while(1); 00387 } 00388 } 00389 00390 /** 00391 * \brief Connect to SSID without security 00392 * \param ssid Name of SSID 00393 * \return none 00394 */ 00395 void connect_to_ssid(char *ssid) { 00396 wifi.connect_open((uint8_t *)ssid); 00397 } 00398 00399 /** 00400 * \brief First time configuration 00401 * \param none 00402 * \return none 00403 */ 00404 void do_FTC(void) { 00405 printf("Running First Time Configuration \r\n"); 00406 wifi.start_smart_config(smartconfigkey); 00407 while (wifi.is_dhcp_configured() == false) { 00408 wait_ms(500); 00409 printf("Waiting for dhcp to be set. \r\n"); 00410 } 00411 user_info.FTC = 1; 00412 wifi.set_user_file_info((uint8_t *)&user_info, sizeof(user_info)); 00413 wifi._wlan.stop(); 00414 printf("FTC finished. \r\n"); 00415 } 00416 00417 /** 00418 * \brief TCP server demo 00419 * \param none 00420 * \return int 00421 */ 00422 int main() { 00423 int loop; 00424 int temp; 00425 unsigned int oldseconds; 00426 00427 //Board dependent init 00428 init(); 00429 00430 // Initalize global variables 00431 axis6.packet_id = 1; 00432 axis6.timestamp = 0; 00433 axis6.acc_x = 0; 00434 axis6.acc_y = 0; 00435 axis6.acc_z = 0; 00436 axis6.mag_x = 0; 00437 axis6.mag_y = 0; 00438 axis6.mag_z = 0; 00439 axis6.roll = 0; 00440 axis6.pitch = 0; 00441 axis6.yaw = 0; 00442 axis6.compass = 0; 00443 axis6.alt = 0; 00444 axis6.temp = 0; 00445 axis6.light = 0; 00446 compass_type = ANDROID_COMPASS; 00447 seconds = 0; 00448 server_running = 1; 00449 newData = 0; 00450 secondFlag = 0; 00451 HsecondFlag = 0; 00452 GREEN_ON; 00453 00454 // Unlock I2C bus if blocked by a device 00455 I2C_busreset(); 00456 00457 pc.baud(115200); 00458 00459 // set current to 500mA since we're turning on the Wi-Fi 00460 Batt.init(CHRG_500MA); 00461 00462 //Init LEDs 00463 initLEDs(); 00464 // Read the Magnetometer a couple of times to initalize 00465 for(loop=0 ; loop < 5 ; loop++) 00466 { 00467 temp = mag.readReg(0x01); 00468 temp = mag.readReg(0x02); 00469 temp = mag.readReg(0x03); 00470 temp = mag.readReg(0x04); 00471 temp = mag.readReg(0x05); 00472 temp = mag.readReg(0x06); 00473 wait_ms(50); 00474 } 00475 00476 init_eCompass(); 00477 00478 // Start Ticker 00479 systick.attach(&SysTick_Handler, 0.01); 00480 // Trigger a WLAN device 00481 wifi.init(); 00482 //wifi.start(0); 00483 printf("CC3000 Wi-Go IOT demo.\r\n"); 00484 print_cc3000_info(); 00485 server_running = 1; 00486 newData = 0; 00487 GREEN_ON; 00488 00489 if(!user_info.FTC) 00490 { 00491 do_FTC(); // Call First Time Configuration if SmartConfig has not been run 00492 printf("Please restart your board. \r\n"); 00493 GreenStop(); 00494 } 00495 00496 // Wait for slider touch 00497 printf("\r\nUse the slider to start an application.\r\n"); 00498 printf("Releasing the slider for more than 3 seconds\r\nwill start the chosen application.\r\n"); 00499 printf("Touching the slider within the 3 seconds\ntimeframe allows you to re-select an application.\r\n"); 00500 printf("\r\nThe RGB LED indicates the selection:\r\n"); 00501 printf("ORANGE - Erase all profiles.\r\n"); 00502 printf("PURPLE - Force SmartConfig.\r\n"); 00503 printf("BLUE - Webserver displaying live sensor data.\r\n"); 00504 printf("RED - Exosite data client.\r\n"); 00505 printf("GREEN - Android sensor fusion app.\r\n"); 00506 while( tsi.readPercentage() == 0 ) 00507 { 00508 RED_ON; 00509 wait(0.2); 00510 RED_OFF; 00511 wait(0.2); 00512 } 00513 RED_OFF; 00514 00515 oldseconds = seconds; 00516 loop = 100; 00517 temp = 0; 00518 // Read slider as long as it is touched. 00519 // If released for more than 3 seconds, exit 00520 while((loop != 0) || ((seconds - oldseconds) < 3)) 00521 { 00522 loop = tsi.readPercentage() * 100; 00523 if(loop != 0) 00524 { 00525 oldseconds = seconds; 00526 temp = loop; 00527 } 00528 if(temp > 80) 00529 { 00530 RED_ON; GREEN_ON; BLUE_OFF; //Orange 00531 } 00532 else if(temp > 60) 00533 { 00534 RED_ON; GREEN_OFF; BLUE_ON; //Purple 00535 } 00536 else if(temp > 40) 00537 { 00538 RED_OFF; GREEN_OFF; BLUE_ON; //Blue 00539 } 00540 else if(temp > 20) 00541 { 00542 RED_ON; GREEN_OFF; BLUE_OFF; //Red 00543 } 00544 else 00545 { 00546 RED_OFF; GREEN_ON; BLUE_OFF; //Green 00547 } 00548 } 00549 RED_OFF; GREEN_OFF; BLUE_OFF; 00550 00551 // Execute the user selected application 00552 if(temp > 80) // Erase all profiles 00553 { 00554 server_running = 1; 00555 RED_OFF; GREEN_OFF; BLUE_OFF; 00556 printf("\r\nErasing all wireless profiles. \r\n"); 00557 wifi.delete_profiles(); 00558 wifi.stop(); 00559 printf("Finished. Please restart your board. \r\n"); 00560 GreenStop(); 00561 } 00562 00563 if(temp > 60) // Force SmartConfig 00564 { 00565 server_running = 1; 00566 RED_OFF; GREEN_OFF; BLUE_OFF; 00567 printf("\r\nStarting Smart Config configuration. \r\n"); 00568 wifi.start_smart_config(smartconfigkey); 00569 while (wifi.is_dhcp_configured() == false) 00570 { 00571 wait_ms(500); 00572 printf("Waiting for dhcp to be set. \r\n"); 00573 } 00574 printf("Finished. Please restart your board. \r\n"); 00575 GreenStop(); 00576 } 00577 00578 RED_OFF; GREEN_OFF; BLUE_OFF; 00579 00580 printf("\r\nAttempting SSID Connection. \r\n"); 00581 if (wifi.connect() == -1) { 00582 printf("Failed to connect. Please verify connection details and try again. \r\n"); 00583 } else { 00584 printf("Connected - IP address: %s \r\n",wifi.getIPAddress()); 00585 } 00586 LED_D3_ON; 00587 00588 server_running = 0; 00589 00590 // Start the selected application 00591 if(temp > 40) // Run Webserver 00592 { 00593 compass_type = NED_COMPASS; 00594 init_eCompass(); 00595 seconds = 0; 00596 demo_wifi_main(); 00597 } 00598 if(temp > 20) // Send data to Exosite 00599 { 00600 compass_type = NED_COMPASS; 00601 init_eCompass(); 00602 seconds = 0; 00603 run_exosite(&socket); 00604 } 00605 init_eCompass(); 00606 seconds = 0; 00607 // Run TCP/IP Connection to host - Sensor Fusion App 00608 runTCPIPserver(); 00609 00610 } 00611
Generated on Thu Jul 14 2022 00:58:11 by
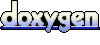