
Demo for GetTypeName library, using all defined types.
Dependencies: GetTypeName mbed
main.cpp
00001 #include "mbed.h" 00002 #include "GetTypeName.h" 00003 00004 int main() 00005 { 00006 char a = 65; 00007 uint8_t b = 20; 00008 signed char c = -30; 00009 int8_t d = -40; 00010 unsigned short e = 50; 00011 uint16_t f = 60; 00012 short g = -70; 00013 int16_t h = -80; 00014 unsigned int i = 90; 00015 uint32_t j = 100; 00016 int k = -110; 00017 int32_t l = -120; 00018 unsigned long long m = 130; 00019 uint64_t n = 140; 00020 long long o = -150; 00021 int64_t p = -160; 00022 float q = 1.7; 00023 double r = 1.8; 00024 bool s = 1; 00025 00026 printf("Type name for <char> '%c' is %s\r\n", a, GetTypeName(a)); 00027 printf("Type name for <uint8_t> %d is %s\r\n", b, GetTypeName(b)); 00028 printf("Type name for <signed char> %d is %s\r\n", c, GetTypeName(c)); 00029 printf("Type name for <int8_t> %d is %s\r\n", d, GetTypeName(d)); 00030 printf("Type name for <unsigned short> %d is %s\r\n", e, GetTypeName(e)); 00031 printf("Type name for <uint16_t> %d is %s\r\n", f, GetTypeName(f)); 00032 printf("Type name for <short> %d is %s\r\n", g, GetTypeName(g)); 00033 printf("Type name for <int16_t> %d is %s\r\n", h, GetTypeName(h)); 00034 printf("Type name for <unsigned int> %d is %s\r\n", i, GetTypeName(i)); 00035 printf("Type name for <uint32_t> %d is %s\r\n", j, GetTypeName(j)); 00036 printf("Type name for <int> %d is %s\r\n", k, GetTypeName(k)); 00037 printf("Type name for <int32_t> %d is %s\r\n", l, GetTypeName(l)); 00038 printf("Type name for <unsigned long long> %lld is %s\r\n", m, GetTypeName(m)); 00039 printf("Type name for <uint64_t> %lld is %s\r\n", n, GetTypeName(n)); 00040 printf("Type name for <long long> %lld is %s\r\n", o, GetTypeName(o)); 00041 printf("Type name for <int64_t> %lld is %s\r\n", p, GetTypeName(p)); 00042 printf("Type name for <float> %f is %s\r\n", q, GetTypeName(q)); 00043 printf("Type name for <double> %f is %s\r\n", r, GetTypeName(r)); 00044 printf("Type name for <bool> %d is %s\r\n", s, GetTypeName(s)); 00045 00046 // Store GetTypeName in a variable 00047 const char *VarType; 00048 VarType = GetTypeName(a); 00049 printf("'%c' is of '%s' type\r\n", a, VarType); 00050 00051 // Check whether GetTypeName is of 'char' type. 00052 // Note that strcmp returns 0 when both strings are equal. 00053 if(!strcmp(GetTypeName(a),"char")) printf("'%c' is of 'char' type\r\n", a); 00054 }
Generated on Fri Jul 22 2022 15:02:40 by
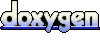