
A minimal library for the DHT11.
Dependents: EXP10_DHT11_LCD Sushil_MODSERIAL Core1000_SmartFarm idd_summer17_hw3_evey_jenny_seiyoung ... more
Dht11.h
00001 #ifndef DHT11_H 00002 #define DHT11_H 00003 00004 #include "mbed.h" 00005 00006 #define DHTLIB_OK 0 00007 #define DHTLIB_ERROR_CHECKSUM -1 00008 #define DHTLIB_ERROR_TIMEOUT -2 00009 00010 /** Class for the DHT11 sensor. 00011 * 00012 * Example: 00013 * @code 00014 * #include "mbed.h" 00015 * #include "Dht11.h" 00016 * 00017 * Serial pc(USBTX, USBRX); 00018 * Dht11 sensor(PTD7); 00019 * 00020 * int main() { 00021 * sensor.read() 00022 * pc.printf("T: %f, H: %d\r\n", sensor.getFahrenheit(), sensor.getHumidity()); 00023 * } 00024 * @endcode 00025 */ 00026 class Dht11 00027 { 00028 public: 00029 /** Construct the sensor object. 00030 * 00031 * @param pin PinName for the sensor pin. 00032 */ 00033 Dht11(PinName const &p); 00034 00035 /** Update the humidity and temp from the sensor. 00036 * 00037 * @returns 00038 * 0 on success, otherwise error. 00039 */ 00040 int read(); 00041 00042 /** Get the temp(f) from the saved object. 00043 * 00044 * @returns 00045 * Fahrenheit float 00046 */ 00047 float getFahrenheit(); 00048 00049 /** Get the temp(c) from the saved object. 00050 * 00051 * @returns 00052 * Celsius int 00053 */ 00054 int getCelsius(); 00055 00056 /** Get the humidity from the saved object. 00057 * 00058 * @returns 00059 * Humidity percent int 00060 */ 00061 int getHumidity(); 00062 00063 private: 00064 /// percentage of humidity 00065 int _humidity; 00066 /// celsius 00067 int _temperature; 00068 /// pin to read the sensor info on 00069 DigitalInOut _pin; 00070 /// times startup (must settle for at least a second) 00071 Timer _timer; 00072 }; 00073 00074 #endif
Generated on Wed Jul 13 2022 01:42:32 by
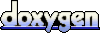