
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
flag.c
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file flag.c 00004 * @version V1.1.4 00005 * @date 2011.04.20 00006 * @brief Flag management implementation code of coocox CoOS kernel. 00007 ******************************************************************************* 00008 * @copy 00009 * 00010 * INTERNAL FILE,DON'T PUBLIC. 00011 * 00012 * <h2><center>© COPYRIGHT 2009 CooCox </center></h2> 00013 ******************************************************************************* 00014 */ 00015 00016 00017 00018 /*---------------------------- Include ---------------------------------------*/ 00019 #include <coocox.h> 00020 00021 #if CFG_FLAG_EN > 0 00022 /*---------------------------- Variable Define -------------------------------*/ 00023 #define FLAG_MAX_NUM 32 /*!< Define max flag number. */ 00024 FCB FlagCrl = {0}; /*!< Flags list struct */ 00025 00026 00027 /*---------------------------- Function Declare ------------------------------*/ 00028 static void FlagBlock(P_FLAG_NODE pnode,U32 flags,U8 waitType); 00029 static P_FLAG_NODE RemoveFromLink(P_FLAG_NODE pnode); 00030 00031 /** 00032 ******************************************************************************* 00033 * @brief Create a flag 00034 * @param[in] bAutoReset Reset mode,Co_TRUE(Auto Reset) FLASE(Manual Reset). 00035 * @param[in] bInitialState Initial state. 00036 * @param[out] None 00037 * @retval E_CREATE_FAIL Create flag fail. 00038 * @retval others Create flag successful. 00039 * 00040 * @par Description 00041 * @details This function use to create a event flag. 00042 * @note 00043 ******************************************************************************* 00044 */ 00045 OS_FlagID CoCreateFlag(BOOL bAutoReset,BOOL bInitialState) 00046 { 00047 U8 i; 00048 OsSchedLock(); 00049 00050 for(i=0;i<FLAG_MAX_NUM;i++) 00051 { 00052 /* Assign a free flag control block */ 00053 if((FlagCrl.flagActive &(1<<i)) == 0 ) 00054 { 00055 FlagCrl.flagActive |= (1<<i); /* Initialize active flag */ 00056 FlagCrl.flagRdy |= (bInitialState<<i);/* Initialize ready flag */ 00057 FlagCrl.resetOpt |= (bAutoReset<<i);/* Initialize reset option */ 00058 OsSchedUnlock(); 00059 return i ; /* Return Flag ID */ 00060 } 00061 } 00062 OsSchedUnlock(); 00063 00064 return E_CREATE_FAIL; /* There is no free flag control block*/ 00065 } 00066 00067 00068 /** 00069 ******************************************************************************* 00070 * @brief Delete a flag 00071 * @param[in] id Flag ID. 00072 * @param[in] opt Delete option. 00073 * @param[out] None 00074 * @retval E_CALL Error call in ISR. 00075 * @retval E_INVALID_ID Invalid event ID. 00076 * @retval E_TASK_WAITTING Tasks waitting for the event,delete fail. 00077 * @retval E_OK Event deleted successful. 00078 * 00079 * @par Description 00080 * @details This function is called to delete a event flag. 00081 * @note 00082 ******************************************************************************* 00083 */ 00084 StatusType CoDelFlag(OS_FlagID id,U8 opt) 00085 { 00086 P_FLAG_NODE pnode; 00087 P_FCB pfcb; 00088 pfcb = &FlagCrl ; 00089 if(OSIntNesting > 0) /* If be called from ISR */ 00090 { 00091 return E_CALL; 00092 } 00093 #if CFG_PAR_CHECKOUT_EN >0 00094 if((pfcb->flagActive &(1<<id)) == 0) /* Flag is valid or not */ 00095 { 00096 return E_INVALID_ID; 00097 } 00098 #endif 00099 OsSchedLock(); 00100 pnode = pfcb->headNode ; 00101 00102 while(pnode != Co_NULL) /* Ready all tasks waiting for flags */ 00103 { 00104 if((pnode->waitFlags &(1<<id)) != 0) /* If no task is waiting on flags */ 00105 { 00106 if(opt == OPT_DEL_NO_PEND) /* Delete flag if no task waiting */ 00107 { 00108 OsSchedUnlock(); 00109 return E_TASK_WAITING; 00110 } 00111 else if (opt == OPT_DEL_ANYWAY) /* Always delete the flag */ 00112 { 00113 if(pnode->waitType == OPT_WAIT_ALL) 00114 { 00115 /* If the flag is only required by NODE */ 00116 if( pnode->waitFlags == (1<<id) ) 00117 { 00118 /* Remove the NODE from waiting list */ 00119 pnode = RemoveFromLink(pnode); 00120 continue; 00121 } 00122 else 00123 { 00124 pnode->waitFlags &= ~(1<<id); /* Update waitflags */ 00125 } 00126 } 00127 else 00128 { 00129 pnode = RemoveFromLink(pnode); 00130 continue; 00131 } 00132 } 00133 } 00134 pnode = pnode->nextNode ; 00135 } 00136 00137 /* Remove the flag from the flags list */ 00138 pfcb->flagActive &= ~(1<<id); 00139 pfcb->flagRdy &= ~(1<<id); 00140 pfcb->resetOpt &= ~(1<<id); 00141 OsSchedUnlock(); 00142 return E_OK; 00143 } 00144 00145 00146 /** 00147 ******************************************************************************* 00148 * @brief AcceptSingleFlag 00149 * @param[in] id Flag ID. 00150 * @param[out] None 00151 * @retval E_INVALID_ID Invalid event ID. 00152 * @retval E_FLAG_NOT_READY Flag is not in ready state. 00153 * @retval E_OK The call was successful and your task owns the Flag. 00154 * 00155 * @par Description 00156 * @details This fucntion is called to accept single flag 00157 * @note 00158 ******************************************************************************* 00159 */ 00160 StatusType CoAcceptSingleFlag(OS_FlagID id) 00161 { 00162 P_FCB pfcb; 00163 pfcb = &FlagCrl ; 00164 #if CFG_PAR_CHECKOUT_EN >0 00165 if(id >= FLAG_MAX_NUM) 00166 { 00167 return E_INVALID_ID; /* Invalid 'id',return error */ 00168 } 00169 if((pfcb->flagActive &(1<<id)) == 0) 00170 { 00171 return E_INVALID_ID; /* Flag is deactive,return error */ 00172 } 00173 #endif 00174 if((pfcb->flagRdy &(1<<id)) != 0) /* If the required flag is set */ 00175 { 00176 OsSchedLock() 00177 pfcb->flagRdy &= ~((FlagCrl.resetOpt )&(1<<id)); /* Clear the flag */ 00178 OsSchedUnlock(); 00179 return E_OK; 00180 } 00181 else /* If the required flag is not set */ 00182 { 00183 return E_FLAG_NOT_READY; 00184 } 00185 } 00186 00187 00188 /** 00189 ******************************************************************************* 00190 * @brief AcceptMultipleFlags 00191 * @param[in] flags Flags that waiting to active task. 00192 * @param[in] waitType Flags wait type. 00193 * @param[out] perr A pointer to error code. 00194 * @retval 0 00195 * @retval springFlag 00196 * 00197 * @par Description 00198 * @details This fucntion is called to accept multiple flags. 00199 * @note 00200 ******************************************************************************* 00201 */ 00202 U32 CoAcceptMultipleFlags(U32 flags,U8 waitType,StatusType *perr) 00203 { 00204 U32 springFlag; 00205 P_FCB pfcb; 00206 pfcb = &FlagCrl ; 00207 00208 #if CFG_PAR_CHECKOUT_EN >0 00209 if((flags&pfcb->flagActive ) != flags ) /* Judge flag is active or not? */ 00210 { 00211 *perr = E_INVALID_PARAMETER; /* Invalid flags */ 00212 return 0; 00213 } 00214 #endif 00215 00216 springFlag = flags & pfcb->flagRdy ; 00217 00218 OsSchedLock(); 00219 /* If any required flags are set */ 00220 if( (springFlag != 0) && (waitType == OPT_WAIT_ANY) ) 00221 { 00222 00223 pfcb->flagRdy &= ~(springFlag & pfcb->resetOpt ); /* Clear the flags */ 00224 OsSchedUnlock(); 00225 *perr = E_OK; 00226 return springFlag; 00227 } 00228 00229 /* If all required flags are set */ 00230 if((springFlag == flags) && (waitType == OPT_WAIT_ALL)) 00231 { 00232 pfcb->flagRdy &= ~(springFlag&pfcb->resetOpt ); /* Clear the flags */ 00233 OsSchedUnlock(); 00234 *perr = E_OK; 00235 return springFlag; 00236 } 00237 OsSchedUnlock(); 00238 *perr = E_FLAG_NOT_READY; 00239 return 0; 00240 } 00241 00242 00243 00244 00245 /** 00246 ******************************************************************************* 00247 * @brief WaitForSingleFlag 00248 * @param[in] id Flag ID. 00249 * @param[in] timeout The longest time for writting flag. 00250 * @param[out] None 00251 * @retval E_CALL Error call in ISR. 00252 * @retval E_INVALID_ID Invalid event ID. 00253 * @retval E_TIMEOUT Flag wasn't received within 'timeout' time. 00254 * @retval E_OK The call was successful and your task owns the Flag, 00255 * or the event you are waiting for occurred. 00256 * 00257 * @par Description 00258 * @details This function is called to wait for only one flag, 00259 * (1) if parameter "timeout" == 0,waiting until flag be set; 00260 * (2) when "timeout" != 0,if flag was set or wasn't set but timeout 00261 * occured,the task will exit the waiting list,convert to READY 00262 * or RUNNING state. 00263 * @note 00264 ******************************************************************************* 00265 */ 00266 StatusType CoWaitForSingleFlag(OS_FlagID id,U32 timeout) 00267 { 00268 FLAG_NODE flagNode; 00269 P_FCB pfcb; 00270 P_OSTCB curTCB; 00271 00272 if(OSIntNesting > 0) /* See if the caller is ISR */ 00273 { 00274 return E_CALL; 00275 } 00276 if(OSSchedLock != 0) /* Schedule is lock? */ 00277 { 00278 return E_OS_IN_LOCK; /* Yes,error return */ 00279 } 00280 00281 #if CFG_PAR_CHECKOUT_EN >0 00282 if(id >= FLAG_MAX_NUM) /* Judge id is valid or not? */ 00283 { 00284 return E_INVALID_ID; /* Invalid 'id' */ 00285 } 00286 if((FlagCrl.flagActive &(1<<id)) == 0 )/* Judge flag is active or not? */ 00287 { 00288 return E_INVALID_ID; /* Flag is deactive ,return error */ 00289 } 00290 #endif 00291 00292 OsSchedLock(); 00293 pfcb = &FlagCrl ; 00294 /* See if the required flag is set */ 00295 if((pfcb->flagRdy &(1<<id)) != 0) /* If the required flag is set */ 00296 { 00297 pfcb->flagRdy &= ~((pfcb->resetOpt &(1<<id))); /* Clear the flag */ 00298 OsSchedUnlock(); 00299 } 00300 else /* If the required flag is not set */ 00301 { 00302 curTCB = TCBRunning ; 00303 if(timeout == 0) /* If time-out is not configured */ 00304 { 00305 /* Block task until the required flag is set */ 00306 FlagBlock (&flagNode,(1<<id),OPT_WAIT_ONE); 00307 curTCB->state = TASK_WAITING; 00308 TaskSchedReq = Co_TRUE; 00309 OsSchedUnlock(); 00310 00311 /* The required flag is set and the task is in running state */ 00312 curTCB->pnode = Co_NULL; 00313 OsSchedLock(); 00314 00315 /* Clear the required flag or not */ 00316 pfcb->flagRdy &= ~((1<<id)&(pfcb->resetOpt )); 00317 OsSchedUnlock(); 00318 } 00319 else /* If time-out is configured */ 00320 { 00321 /* Block task until the required flag is set or time-out occurs */ 00322 FlagBlock(&flagNode,(1<<id),OPT_WAIT_ONE); 00323 InsertDelayList(curTCB,timeout); 00324 00325 OsSchedUnlock(); 00326 if(curTCB->pnode == Co_NULL) /* If time-out occurred */ 00327 { 00328 return E_TIMEOUT; 00329 } 00330 else /* If flag is set */ 00331 { 00332 curTCB->pnode = Co_NULL; 00333 OsSchedLock(); 00334 00335 /* Clear the required flag or not */ 00336 pfcb->flagRdy &= ~((1<<id)&(pfcb->resetOpt )); 00337 OsSchedUnlock(); 00338 } 00339 } 00340 } 00341 return E_OK; 00342 } 00343 00344 00345 /** 00346 ******************************************************************************* 00347 * @brief WaitForMultipleFlags 00348 * @param[in] flags Flags that waiting to active task. 00349 * @param[in] waitType Flags wait type. 00350 * @param[in] timeout The longest time for writting flag. 00351 * @param[out] perr A pointer to error code. 00352 * @retval 0 00353 * @retval springFlag 00354 * 00355 * @par Description 00356 * @details This function is called to pend a task for waitting multiple flag. 00357 * @note 00358 ******************************************************************************* 00359 */ 00360 U32 CoWaitForMultipleFlags(U32 flags,U8 waitType,U32 timeout,StatusType *perr) 00361 { 00362 U32 springFlag; 00363 P_FCB pfcb; 00364 FLAG_NODE flagNode; 00365 P_OSTCB curTCB; 00366 00367 00368 if(OSIntNesting > 0) /* If the caller is ISR */ 00369 { 00370 *perr = E_CALL; 00371 return 0; 00372 } 00373 if(OSSchedLock != 0) /* Schedule is lock? */ 00374 { 00375 *perr = E_OS_IN_LOCK; 00376 return 0; /* Yes,error return */ 00377 } 00378 #if CFG_PAR_CHECKOUT_EN >0 00379 if( (flags&FlagCrl.flagActive ) != flags ) 00380 { 00381 *perr = E_INVALID_PARAMETER; /* Invalid 'flags' */ 00382 return 0; 00383 } 00384 #endif 00385 OsSchedLock(); 00386 pfcb = &FlagCrl ; 00387 springFlag = flags & pfcb->flagRdy ; 00388 00389 /* If any required flags are set */ 00390 if((springFlag != 0) && (waitType == OPT_WAIT_ANY)) 00391 { 00392 pfcb->flagRdy &= ~(springFlag & pfcb->resetOpt ); /* Clear the flag */ 00393 OsSchedUnlock(); 00394 *perr = E_OK; 00395 return springFlag; 00396 } 00397 00398 /* If all required flags are set */ 00399 if( (springFlag == flags) && (waitType == OPT_WAIT_ALL) ) 00400 { 00401 pfcb->flagRdy &= ~(springFlag & pfcb->resetOpt ); /* Clear the flags */ 00402 OsSchedUnlock(); 00403 *perr = E_OK; 00404 return springFlag; 00405 } 00406 00407 curTCB = TCBRunning ; 00408 if(timeout == 0) /* If time-out is not configured */ 00409 { 00410 /* Block task until the required flag are set */ 00411 FlagBlock(&flagNode,flags,waitType); 00412 curTCB->state = TASK_WAITING; 00413 TaskSchedReq = Co_TRUE; 00414 OsSchedUnlock(); 00415 00416 curTCB->pnode = Co_NULL; 00417 OsSchedLock(); 00418 springFlag = flags & pfcb->flagRdy ; 00419 pfcb->flagRdy &= ~(springFlag & pfcb->resetOpt );/* Clear the flags */ 00420 OsSchedUnlock(); 00421 *perr = E_OK; 00422 return springFlag; 00423 } 00424 else /* If time-out is configured */ 00425 { 00426 /* Block task until the required flag are set or time-out occurred */ 00427 FlagBlock(&flagNode,flags,waitType); 00428 InsertDelayList(curTCB,timeout); 00429 00430 OsSchedUnlock(); 00431 if(curTCB->pnode == Co_NULL) /* If time-out occurred */ 00432 { 00433 *perr = E_TIMEOUT; 00434 return 0; 00435 } 00436 else /* If the required flags are set */ 00437 { 00438 curTCB->pnode = Co_NULL; 00439 OsSchedLock(); 00440 springFlag = flags & FlagCrl.flagRdy ; 00441 00442 /* Clear the required ready flags or not */ 00443 pfcb->flagRdy &= ~(springFlag & pfcb->resetOpt ); 00444 OsSchedUnlock(); 00445 *perr = E_OK; 00446 return springFlag; 00447 } 00448 } 00449 } 00450 00451 00452 /** 00453 ******************************************************************************* 00454 * @brief Clear a Flag 00455 * @param[in] id Flag ID. 00456 * @param[out] None 00457 * @retval E_OK Event deleted successful. 00458 * @retval E_INVALID_ID Invalid event ID. 00459 * 00460 * @par Description 00461 * @details This function is called to clear a flag. 00462 * 00463 * @note 00464 ******************************************************************************* 00465 */ 00466 StatusType CoClearFlag(OS_FlagID id) 00467 { 00468 P_FCB pfcb; 00469 pfcb = &FlagCrl ; 00470 #if CFG_PAR_CHECKOUT_EN >0 00471 if(id >= FLAG_MAX_NUM) 00472 { 00473 return E_INVALID_ID; /* Invalid id */ 00474 } 00475 if((pfcb->flagActive &(1<<id)) == 0) 00476 { 00477 return E_INVALID_ID; /* Invalid flag */ 00478 } 00479 #endif 00480 00481 pfcb->flagRdy &= ~(1<<id); /* Clear the flag */ 00482 return E_OK; 00483 } 00484 00485 00486 /** 00487 ******************************************************************************* 00488 * @brief Set a flag 00489 * @param[in] id Flag ID. 00490 * @param[out] None 00491 * @retval E_INVALID_ID Invalid event ID. 00492 * @retval E_OK Event deleted successful. 00493 * 00494 * @par Description 00495 * @details This function is called to set a flag. 00496 * @note 00497 ******************************************************************************* 00498 */ 00499 StatusType CoSetFlag(OS_FlagID id) 00500 { 00501 P_FLAG_NODE pnode; 00502 P_FCB pfcb; 00503 pfcb = &FlagCrl ; 00504 00505 #if CFG_PAR_CHECKOUT_EN >0 00506 if(id >= FLAG_MAX_NUM) /* Flag is valid or not */ 00507 { 00508 return E_INVALID_ID; /* Invalid flag id */ 00509 } 00510 if((pfcb->flagActive &(1<<id)) == 0) 00511 { 00512 return E_INVALID_ID; /* Flag is not exist */ 00513 } 00514 #endif 00515 00516 if((pfcb->flagRdy &(1<<id)) != 0) /* Flag had already been set */ 00517 { 00518 return E_OK; 00519 } 00520 00521 pfcb->flagRdy |= (1<<id); /* Update the flags ready list */ 00522 00523 OsSchedLock(); 00524 pnode = pfcb->headNode ; 00525 while(pnode != Co_NULL) 00526 { 00527 if(pnode->waitType == OPT_WAIT_ALL) /* Extract all the bits we want */ 00528 { 00529 if((pnode->waitFlags &pfcb->flagRdy ) == pnode->waitFlags ) 00530 { 00531 /* Remove the flag node from the wait list */ 00532 pnode = RemoveFromLink(pnode); 00533 if((pfcb->resetOpt &(1<<id)) != 0)/* If the flags is auto-reset*/ 00534 { 00535 break; 00536 } 00537 continue; 00538 } 00539 } 00540 else /* Extract only the bits we want */ 00541 { 00542 if( (pnode->waitFlags & pfcb->flagRdy ) != 0) 00543 { 00544 /* Remove the flag node from the wait list */ 00545 pnode = RemoveFromLink(pnode); 00546 if((pfcb->resetOpt &(1<<id)) != 0) 00547 { 00548 break; /* The flags is auto-reset */ 00549 } 00550 continue; 00551 } 00552 } 00553 pnode = pnode->nextNode ; 00554 } 00555 OsSchedUnlock(); 00556 return E_OK; 00557 } 00558 00559 00560 00561 /** 00562 ******************************************************************************* 00563 * @brief Set a flag in ISR 00564 * @param[in] id Flag ID. 00565 * @param[out] None 00566 * @retval E_INVALID_ID Invalid event ID. 00567 * @retval E_OK Event deleted successful. 00568 * 00569 * @par Description 00570 * @details This function is called in ISR to set a flag. 00571 * @note 00572 ******************************************************************************* 00573 */ 00574 #if CFG_MAX_SERVICE_REQUEST > 0 00575 StatusType isr_SetFlag(OS_FlagID id) 00576 { 00577 if(OSSchedLock > 0) /* If scheduler is locked,(the caller is ISR) */ 00578 { 00579 /* Insert the request into service request queue */ 00580 if(InsertInSRQ(FLAG_REQ,id,Co_NULL) == Co_FALSE) 00581 { 00582 return E_SEV_REQ_FULL; /* The service requst queue is full */ 00583 } 00584 else 00585 { 00586 return E_OK; 00587 } 00588 } 00589 else 00590 { 00591 return(CoSetFlag(id)); /* The caller is not ISR, set the flag*/ 00592 } 00593 } 00594 #endif 00595 00596 /** 00597 ******************************************************************************* 00598 * @brief Block a task to wait a flag event 00599 * @param[in] pnode A node that will link into flag waiting list. 00600 * @param[in] flags Flag(s) that the node waiting for. 00601 * @param[in] waitType Waiting type of the node. 00602 * @param[out] None 00603 * @retval None 00604 * 00605 * @par Description 00606 * @details This function is called to block a task to wait a flag event. 00607 * @note 00608 ******************************************************************************* 00609 */ 00610 static void FlagBlock(P_FLAG_NODE pnode,U32 flags,U8 waitType) 00611 { 00612 P_FCB pfcb; 00613 pfcb = &FlagCrl ; 00614 00615 TCBRunning ->pnode = pnode; 00616 pnode->waitTask = TCBRunning ; 00617 pnode->waitFlags = flags; /* Save the flags that we need to wait for*/ 00618 pnode->waitType = waitType; /* Save the type of wait */ 00619 00620 if(pfcb->tailNode == 0) /* If this is the first NODE to insert? */ 00621 { 00622 pnode->nextNode = 0; 00623 pnode->prevNode = 0; 00624 pfcb->headNode = pnode; /* Insert the NODE to the head */ 00625 } 00626 else /* If it is not the first NODE to insert? */ 00627 { 00628 pfcb->tailNode ->nextNode = pnode; /* Insert the NODE to the tail */ 00629 pnode->prevNode = pfcb->tailNode ; 00630 pnode->nextNode = 0; 00631 } 00632 pfcb->tailNode = pnode; 00633 } 00634 00635 00636 /** 00637 ******************************************************************************* 00638 * @brief Remove a flag node from list 00639 * @param[in] pnode A node that will remove from flag waiting list. 00640 * @param[out] None 00641 * @retval pnode Next node of the node that have removed out. 00642 * 00643 * @par Description 00644 * @details This function is called to remove a flag node from the wait list. 00645 * @note 00646 ******************************************************************************* 00647 */ 00648 static P_FLAG_NODE RemoveFromLink(P_FLAG_NODE pnode) 00649 { 00650 P_OSTCB ptcb; 00651 00652 RemoveLinkNode(pnode); /* Remove the flag node from wait list. */ 00653 ptcb = pnode->waitTask ; 00654 00655 /* The task in the delay list */ 00656 if(ptcb->delayTick != INVALID_VALUE)/* If the task is in tick delay list */ 00657 { 00658 RemoveDelayList(ptcb); /* Remove the task from tick delay list */ 00659 } 00660 00661 ptcb->pnode = (void*)0xffffffff; 00662 00663 if(ptcb == TCBRunning ) 00664 { 00665 ptcb->state = TASK_RUNNING; 00666 } 00667 else 00668 { 00669 InsertToTCBRdyList(ptcb); /* Insert the task to ready list */ 00670 } 00671 return (pnode->nextNode ); 00672 } 00673 00674 /** 00675 ******************************************************************************* 00676 * @brief Remove a flag node from list 00677 * @param[in] pnode A node that will remove from flag waiting list. 00678 * @param[out] None 00679 * @retval None 00680 * 00681 * @par Description 00682 * @details This function is called to remove a flag node from the wait list. 00683 * @note 00684 ******************************************************************************* 00685 */ 00686 void RemoveLinkNode(P_FLAG_NODE pnode) 00687 { 00688 /* If only one NODE in the list*/ 00689 if((pnode->nextNode == 0) && (pnode->prevNode == 0)) 00690 { 00691 FlagCrl.headNode = 0; 00692 FlagCrl.tailNode = 0; 00693 } 00694 else if(pnode->nextNode == 0) /* If the NODE is tail */ 00695 { 00696 FlagCrl.tailNode = pnode->prevNode ; 00697 pnode->prevNode ->nextNode = 0; 00698 } 00699 else if(pnode->prevNode == 0) /* If the NODE is head */ 00700 { 00701 FlagCrl.headNode = pnode->nextNode ; 00702 pnode->nextNode ->prevNode = 0; 00703 } 00704 else /* The NODE is in the middle */ 00705 { 00706 pnode->nextNode ->prevNode = pnode->prevNode ; 00707 pnode->prevNode ->nextNode = pnode->nextNode ; 00708 } 00709 pnode->waitTask ->pnode = 0; 00710 } 00711 00712 #endif
Generated on Tue Jul 12 2022 18:19:10 by
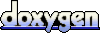