
Bathroom scale
Dependencies: Hexi_KW40Z Hexi_OLED_SSD1351 MPL3115A2
main.cpp
00001 #include "mbed.h" 00002 #include "Hexi_KW40Z.h" 00003 #include "Hexi_OLED_SSD1351.h" 00004 #include "OLED_types.h" 00005 #include "OpenSans_Font.h" 00006 #include "string.h" 00007 00008 #define LED_ON 0 00009 #define LED_OFF 1 00010 00011 00012 void StartHaptic(void); 00013 void StopHaptic(void const *n); 00014 void txTask(void); 00015 long ReadWeight(void); 00016 uint16_t getGram(); 00017 long offset = 0; 00018 long getAverageValue(int times); 00019 00020 // Pin connections for Hexiwear 00021 //MPL3115A2 MPL3115A2( PTC11, PTC10, MPL3115A2_I2C_ADDRESS); 00022 /* pos [0] = altimeter or pressure value */ 00023 /* pos [1] = temperature value */ 00024 float sensor_data[2]; 00025 00026 DigitalOut redLed(LED1,1); 00027 DigitalOut greenLed(LED2,1); 00028 DigitalOut blueLed(LED3,1); 00029 DigitalOut haptic(PTB9); 00030 00031 DigitalIn HX_DOUT(PTC3); 00032 DigitalOut HX_PD_SCK(PTC5); 00033 00034 /* Define timer for haptic feedback */ 00035 RtosTimer hapticTimer(StopHaptic, osTimerOnce); 00036 00037 /* Instantiate the Hexi KW40Z Driver (UART TX, UART RX) */ 00038 KW40Z kw40z_device(PTE24, PTE25); 00039 00040 /* Instantiate the SSD1351 OLED Driver */ 00041 SSD1351 oled(PTB22,PTB21,PTC13,PTB20,PTE6, PTD15); /* (MOSI,SCLK,POWER,CS,RST,DC) */ 00042 00043 /*Create a Thread to handle sending BLE Sensor Data */ 00044 Thread txThread; 00045 00046 /* Text Buffer */ 00047 char text[20]; 00048 00049 /****************************Call Back Functions*******************************/ 00050 void ButtonRight(void) 00051 { 00052 StartHaptic(); 00053 kw40z_device.ToggleAdvertisementMode(); 00054 } 00055 00056 void ButtonLeft(void) 00057 { 00058 StartHaptic(); 00059 kw40z_device.ToggleAdvertisementMode(); 00060 } 00061 00062 void PassKey(void) 00063 { 00064 StartHaptic(); 00065 strcpy((char *) text,"PAIR CODE"); 00066 oled.TextBox((uint8_t *)text,0,25,95,18); 00067 00068 /* Display Bond Pass Key in a 95px by 18px textbox at x=0,y=40 */ 00069 sprintf(text,"%d", kw40z_device.GetPassKey()); 00070 oled.TextBox((uint8_t *)text,0,40,95,18); 00071 } 00072 00073 /***********************End of Call Back Functions*****************************/ 00074 00075 /********************************Main******************************************/ 00076 00077 int main() 00078 { 00079 Thread::wait(10000); 00080 offset = getAverageValue(20); 00081 /* Get OLED Class Default Text Properties */ 00082 oled_text_properties_t textProperties = {0}; 00083 oled.GetTextProperties(&textProperties); 00084 00085 /* Turn on the backlight of the OLED Display */ 00086 oled.DimScreenON(); 00087 00088 /* Fills the screen with solid black */ 00089 oled.FillScreen(COLOR_BLACK); 00090 00091 /* Register callbacks to application functions */ 00092 kw40z_device.attach_buttonLeft(&ButtonLeft); 00093 kw40z_device.attach_buttonRight(&ButtonRight); 00094 kw40z_device.attach_passkey(&PassKey); 00095 00096 /* Change font color to white */ 00097 textProperties.fontColor = COLOR_WHITE; 00098 oled.SetTextProperties(&textProperties); 00099 00100 /* Display Bluetooth Label at x=17,y=30 */ 00101 strcpy((char *) text,"89.9kg"); 00102 oled.Label((uint8_t *)text,17,30); 00103 00104 00105 /* Change font color to Blue */ 00106 textProperties.fontColor = COLOR_BLUE; 00107 oled.SetTextProperties(&textProperties); 00108 00109 /* Display Bluetooth Label at x=17,y=65 */ 00110 strcpy((char *) text,"BLUETOOTH"); 00111 oled.Label((uint8_t *)text,17,65); 00112 00113 /* Change font color to white */ 00114 textProperties.fontColor = COLOR_WHITE; 00115 textProperties.alignParam = OLED_TEXT_ALIGN_CENTER; 00116 oled.SetTextProperties(&textProperties); 00117 00118 /* Display Label at x=22,y=80 */ 00119 strcpy((char *) text,"Tap Below"); 00120 oled.Label((uint8_t *)text,22,80); 00121 00122 uint8_t prevLinkState = 0; 00123 uint8_t currLinkState = 0; 00124 txThread.start(txTask); /*Start transmitting Sensor Tag Data */ 00125 00126 while (true) 00127 { 00128 blueLed = !kw40z_device.GetAdvertisementMode(); /*Indicate BLE Advertisment Mode*/ 00129 Thread::wait(50); 00130 } 00131 } 00132 00133 /******************************End of Main*************************************/ 00134 00135 00136 /* txTask() transmits the sensor data */ 00137 void txTask(void){ 00138 00139 while (true) 00140 { 00141 /* sets the gain of hx711 to 128*/ 00142 ReadWeight(); 00143 00144 /*Notify Hexiwear App that it is running Sensor Tag mode*/ 00145 kw40z_device.SendSetApplicationMode(GUI_CURRENT_APP_SENSOR_TAG); 00146 00147 /*Send weight using pressure service*/ 00148 kw40z_device.SendPressure(getGram()); 00149 Thread::wait(1000); 00150 } 00151 } 00152 00153 void StartHaptic(void) { 00154 hapticTimer.start(50); 00155 haptic = 1; 00156 } 00157 00158 void StopHaptic(void const *n) { 00159 haptic = 0; 00160 hapticTimer.stop(); 00161 } 00162 00163 long ReadWeight(void) 00164 { 00165 long Count; 00166 unsigned char i; 00167 HX_PD_SCK.write(0); 00168 Count = 0; 00169 while(HX_DOUT.read() == 1); 00170 for (i=0;i<24;i++) 00171 { 00172 HX_PD_SCK.write(1); 00173 Count=Count<<1; 00174 HX_PD_SCK.write(0); 00175 if(HX_DOUT.read() == 1) Count++; 00176 } 00177 HX_PD_SCK.write(1); 00178 Count=Count^0x800000; 00179 HX_PD_SCK.write(0); 00180 return(Count); 00181 } 00182 00183 uint16_t getGram(){ 00184 return ((getAverageValue(20) - offset))/1000; 00185 } 00186 00187 long getAverageValue(int times){ 00188 long sum = 0; 00189 for (int i = 1; i <= times; i++) 00190 { 00191 sum += ReadWeight(); 00192 } 00193 return sum / times; 00194 } 00195 00196 00197 00198 00199
Generated on Wed Jul 13 2022 17:10:46 by
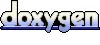