Racing Robots Session
Dependencies: MbedJSONValue m3pi
xbee.cpp
00001 #include "xbee.h" 00002 #include "MbedJSONValue.h" 00003 00004 Xbee::Xbee(PinName tx, PinName rx){ 00005 xbee = new Serial(tx, rx); 00006 xbee->baud(115200); 00007 xbee->attach(this, &Xbee::received, Serial::RxIrq); 00008 run = false; 00009 printf("xbee constructor\r\n"); 00010 rst = new DigitalOut(p26); 00011 reset(); 00012 setCode(-1); 00013 } 00014 00015 void Xbee::reset(){ 00016 rst->write(0); 00017 wait_ms(1); 00018 rst->write(1); 00019 wait_ms(1); 00020 } 00021 00022 void Xbee::setCode(int code){ 00023 if( code >= 0 && code < 1000){ 00024 error("Code must be between 0 and 999."); 00025 } 00026 this->code = code; 00027 } 00028 00029 int Xbee::hasCode(){ 00030 return code != -1; 00031 } 00032 00033 int Xbee::running(){ 00034 return run; 00035 } 00036 00037 int Xbee::stopped(){ 00038 return !running(); 00039 } 00040 00041 void Xbee::received(){ 00042 char c; 00043 while(xbee->readable()){ 00044 c = xbee->getc(); 00045 putc(c, stdout); 00046 buffer[buffer_pos] = c; 00047 buffer_pos++; 00048 if(c == '\n'){ 00049 buffer[buffer_pos] = '\0'; 00050 00051 printf("buffer: %s\r\n", buffer); 00052 00053 buffer_pos = 0; 00054 MbedJSONValue json; 00055 parse(json, buffer); 00056 00057 int code = -1; 00058 if(json.hasMember("start")){ 00059 code = json["start"].get<int>(); 00060 } else if(json.hasMember("stop")){ 00061 code = json["stop"].get<int>(); 00062 } else { 00063 break; 00064 } 00065 00066 printf("code: %d\r\n", code); 00067 00068 if(json.hasMember("start") && code == this->code){ 00069 run = true; 00070 printf("start\r\n"); 00071 } else if(code == this->code){ 00072 run = false; 00073 printf("stop\r\n"); 00074 } 00075 } 00076 } 00077 }
Generated on Sat Jul 16 2022 11:43:30 by
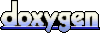