
Example demonstrating the use of the Vodafone USB Modem library with the HTTP Client
Dependencies: HTTPClient VodafoneUSBModem mbed-rtos mbed
Fork of VodafoneK3770HTTPClientTestBeta by
main.cpp
00001 #include "mbed.h" 00002 #include "VodafoneUSBModem.h" 00003 #include "HTTPClient.h" 00004 00005 void test(void const*) 00006 { 00007 VodafoneUSBModem modem; 00008 HTTPClient http; 00009 char str[512]; 00010 00011 int ret = modem.connect("pp.vodafone.co.uk"); 00012 if(ret) 00013 { 00014 printf("Could not connect\n"); 00015 return; 00016 } 00017 00018 //GET data 00019 printf("Trying to fetch page...\n"); 00020 ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00021 if (!ret) 00022 { 00023 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00024 printf("Result: %s\n", str); 00025 } 00026 else 00027 { 00028 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00029 } 00030 00031 //POST data 00032 HTTPMap map; 00033 HTTPText text(str, 512); 00034 map.put("Hello", "World"); 00035 map.put("test", "1234"); 00036 printf("Trying to post data...\n"); 00037 ret = http.post("http://httpbin.org/post", map, &text); 00038 if (!ret) 00039 { 00040 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00041 printf("Result: %s\n", str); 00042 } 00043 else 00044 { 00045 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00046 } 00047 00048 modem.disconnect(); 00049 00050 while(1) { 00051 } 00052 } 00053 00054 00055 int main() 00056 { 00057 Thread testTask(test, NULL, osPriorityNormal, 1024 * 4); 00058 DigitalOut led(LED1); 00059 while(1) 00060 { 00061 led=!led; 00062 Thread::wait(1000); 00063 } 00064 00065 return 0; 00066 }
Generated on Tue Jul 12 2022 12:11:05 by
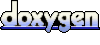