TextLCD compatible wrapper for NOKIA_5110 library
Fork of TextLCD by
TextLCD_NOKIA_5110.h
00001 /* mbed TextLCD5110 Library, for Nokia 5110 LCDs 00002 * Original Copyright (c) 2007-2010, sford, http://mbed.org 00003 * Revisions Copyright (c) 2014, David R. Van Wagner, https://mbed.org/users/davervw/ 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef MBED_TEXTLCD_H 00025 #define MBED_TEXTLCD_H 00026 00027 #include "mbed.h" 00028 #include "NOKIA_5110.h" 00029 00030 /** A TextLCD compatible interface for driving Nokia 5110 LCDs 00031 * 00032 * @code 00033 * #include "mbed.h" 00034 * #include "TextLCD5110.h" 00035 * 00036 * TextLCD5110 lcd(p5, p7, p24, p25, p26); 00037 * 00038 * int main() { 00039 * lcd.printf("Hello World!\n"); 00040 * } 00041 * @endcode 00042 */ 00043 class TextLCD5110 : public Stream { 00044 public: 00045 00046 /** Create a TextLCD5110 interface 00047 * 00048 * @param mosi serial in line (din) 00049 * @param sclk serial clock line (sclk) 00050 * @param dc data/command select line 00051 * @param cse Enable line (chip select) 00052 * @param reset Reset line 00053 */ 00054 TextLCD5110(PinName mosi, PinName sclk, PinName dc, PinName cse, PinName reset); 00055 00056 #if DOXYGEN_ONLY 00057 /** Write a character to the LCD 00058 * 00059 * @param c The character to write to the display 00060 */ 00061 int putc(int c); 00062 00063 /** Write a formated string to the LCD 00064 * 00065 * @param format A printf-style format string, followed by the 00066 * variables to use in formating the string. 00067 */ 00068 int printf(const char* format, ...); 00069 #endif 00070 00071 /** Locate to a screen column and row 00072 * 00073 * @param column The horizontal position from the left, indexed from 0 00074 * @param row The vertical position from the top, indexed from 0 00075 */ 00076 void locate(int column, int row); 00077 00078 /** Clear the screen and locate to 0,0 */ 00079 void cls(); 00080 00081 int rows(); 00082 int columns(); 00083 00084 int get_row(); 00085 int get_column(); 00086 00087 protected: 00088 00089 // Stream implementation functions 00090 virtual int _putc(int value); 00091 virtual int _getc(); 00092 00093 int _column; 00094 int _row; 00095 00096 NokiaLcd *pLcd; 00097 00098 void character(int column, int row, int c); 00099 00100 }; 00101 00102 #endif
Generated on Tue Jul 12 2022 17:30:04 by
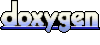