
I have ported my old project “pNesX” game console emulator to the nucleo.
Dependencies: SDFileSystem mbed
pspad.cpp
00001 /*===================================================================*/ 00002 /* */ 00003 /* pspad.cpp : PS Pad function */ 00004 /* */ 00005 /* 2016/1/20 Racoon */ 00006 /* */ 00007 /*===================================================================*/ 00008 00009 #include "mbed.h" 00010 #include "pspad.h" 00011 00012 // SPI interface 00013 SPI pad_spi(PC_12, PC_11, PC_10); // MOSI(should pullup), MISO, SCK 00014 DigitalOut pad1_cs(PD_2); 00015 00016 // PS pad initialize 00017 void pspad_init() 00018 { 00019 pad_spi.format(8, 3); 00020 pad_spi.frequency(250000); 00021 } 00022 00023 // Read PS Pad state 00024 // data1 SE -- -- ST U R D L 00025 // data2 L2 R2 L1 R1 TR O X SQ 00026 void pspad_read(unsigned short *pad1, unsigned short *pad2) 00027 { 00028 pad1_cs = 0; 00029 wait_us(500); 00030 00031 pad_spi.write(0x80); 00032 pad_spi.write(0x42); 00033 pad_spi.write(0); 00034 pad_spi.write(0); 00035 int d1 = pad_spi.write(0); 00036 int d2 = pad_spi.write(0); 00037 00038 pad1_cs = 1; 00039 00040 *pad1 = (char)~d1 << 8 | (char)~d2; 00041 00042 *pad2 = 0; 00043 } 00044
Generated on Tue Jul 12 2022 20:36:59 by
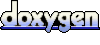