
I have ported my old project “pNesX” game console emulator to the nucleo.
Dependencies: SDFileSystem mbed
K6502.h
00001 /*===================================================================*/ 00002 /* */ 00003 /* K6502.h : Header file for K6502 */ 00004 /* */ 00005 /* 1999/10/19 Racoon New preparation */ 00006 /* */ 00007 /*===================================================================*/ 00008 00009 #ifndef K6502_H_INCLUDED 00010 #define K6502_H_INCLUDED 00011 00012 // Type definition 00013 #ifndef DWORD 00014 typedef unsigned long DWORD; 00015 //typedef unsigned int DWORD; 00016 #endif 00017 00018 #ifndef WORD 00019 typedef unsigned short WORD; 00020 //typedef unsigned short WORD; 00021 #endif 00022 00023 #ifndef BYTE 00024 typedef unsigned char BYTE; 00025 //typedef char BYTE; 00026 #endif 00027 00028 #ifndef NULL 00029 #define NULL 0 00030 #endif 00031 00032 /* 6502 Flags */ 00033 #define FLAG_C 0x01 00034 #define FLAG_Z 0x02 00035 #define FLAG_I 0x04 00036 #define FLAG_D 0x08 00037 #define FLAG_B 0x10 00038 #define FLAG_R 0x20 00039 #define FLAG_V 0x40 00040 #define FLAG_N 0x80 00041 00042 /* Stack Address */ 00043 #define BASE_STACK 0x100 00044 00045 /* Interrupt Vectors */ 00046 #define VECTOR_NMI 0xfffa 00047 #define VECTOR_RESET 0xfffc 00048 #define VECTOR_IRQ 0xfffe 00049 00050 // NMI Request 00051 #define NMI_REQ NMI_State = 0; 00052 00053 // IRQ Request 00054 #define IRQ_REQ IRQ_State = 0; 00055 00056 // Emulator Operation 00057 void K6502_Init(); 00058 void K6502_Reset(); 00059 void K6502_Set_Int_Wiring( BYTE byNMI_Wiring, BYTE byIRQ_Wiring ); 00060 void K6502_Step( register WORD wClocks ); 00061 00062 // I/O Operation (User definition) 00063 static inline BYTE K6502_Read( WORD wAddr); 00064 static inline WORD K6502_ReadW( WORD wAddr ); 00065 static inline BYTE K6502_ReadZp( BYTE byAddr ); 00066 static inline WORD K6502_ReadZpW( BYTE byAddr ); 00067 static inline BYTE K6502_ReadAbsX(); 00068 static inline BYTE K6502_ReadAbsY(); 00069 static inline BYTE K6502_ReadIY(); 00070 00071 static inline void K6502_Write( WORD wAddr, BYTE byData ); 00072 static inline void K6502_WriteW( WORD wAddr, WORD wData ); 00073 00074 // The state of the IRQ pin 00075 extern BYTE IRQ_State; 00076 00077 // The state of the NMI pin 00078 extern BYTE NMI_State; 00079 00080 #endif /* !K6502_H_INCLUDED */ 00081 00082
Generated on Tue Jul 12 2022 20:36:59 by
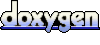