TI社のBLDCモータードライバDRV8301を コントロールするためのユーティリティのようなクラスです。 できるだけユーザーにわかりやすく レジスタの設定・読み取りなどを行います。 尚、データシートは必須の模様。
Dependents: BLDC1Axis_DRV8301CTRL
DRV8301CTRL.h
00001 /* Copyright (c) 2016 Yajirushi(Cursor) 00002 * 00003 * Released under the MIT license 00004 * http://opensource.org/licenses/mit-license.php 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 #include "mbed.h" 00025 00026 #ifndef DRV8301CTRL_H 00027 #define DRV8301CTRL_H 00028 00029 // ##### BEGIN:ここからユーザーが変更する部分(Change setting, if you need.) #### 00030 00031 //DRV8301 SPI FREQUENCY(SPIの周波数:Max=5MHz) 00032 #define DRV8301CTRL_FREQ 1000000 00033 00034 // ##### END:ここまで ##### 00035 00036 //STATUS & CONTROL REGISTERS 00037 #define DRV8301REG_WRITEMODE 0x0000 00038 #define DRV8301REG_READMODE 0x8000 00039 #define DRV8301REG_STATUS1 0x0000 00040 #define DRV8301REG_STATUS2 0x0800 00041 #define DRV8301REG_CTRL1 0x1000 00042 #define DRV8301REG_CTRL2 0x1800 00043 00044 //ENUM STATUS & CONTROL VALUES 00045 enum CTRL1_GATE_CURRENT{ 00046 GATE_CURRENT_PEAKCURRENT_1_7A = 0x0000, 00047 GATE_CURRENT_PEAKCURRENT_0_7A = 0x0001, 00048 GATE_CURRENT_PEAKCURRENT_0_25A = 0x0002, 00049 GATE_CURRENT_PEAKCURRENT_RESERVED = 0x0003 00050 }; 00051 enum CTRL1_GATE_RESET{ 00052 GATE_RESET_NORMAL = 0x0000, 00053 GATE_RESET_RESETGATE_LATCHED_FAULT = 0x0004 00054 }; 00055 enum CTRL1_PWM_MODE{ 00056 PWM_MODE_PWMLINES_6 = 0x0000, 00057 PWM_MODE_PWMLINES_3 = 0x0008 00058 }; 00059 enum CTRL1_OCP_MODE{ 00060 OCP_MODE_CURRENTLIMIT = 0x0000, 00061 OCP_MODE_OC_LATCH_SHUTDOWN = 0x0010, 00062 OCP_MODE_REPORTONLY = 0x0020, 00063 OCP_MODE_OC_DISABLE = 0x0030 00064 }; 00065 enum CTRL1_OC_ADJ{ 00066 OC_ADJ_SET_ADJUST_0_060=0x0000, 00067 OC_ADJ_SET_ADJUST_0_068=0x0040, 00068 OC_ADJ_SET_ADJUST_0_076=0x0080, 00069 OC_ADJ_SET_ADJUST_0_086=0x00C0, 00070 OC_ADJ_SET_ADJUST_0_097=0x0100, 00071 OC_ADJ_SET_ADJUST_0_109=0x0140, 00072 OC_ADJ_SET_ADJUST_0_123=0x0180, 00073 OC_ADJ_SET_ADJUST_0_138=0x01C0, 00074 OC_ADJ_SET_ADJUST_0_155=0x0200, 00075 OC_ADJ_SET_ADJUST_0_175=0x0240, 00076 OC_ADJ_SET_ADJUST_0_197=0x0280, 00077 OC_ADJ_SET_ADJUST_0_222=0x02C0, 00078 OC_ADJ_SET_ADJUST_0_250=0x0300, 00079 OC_ADJ_SET_ADJUST_0_282=0x0340, 00080 OC_ADJ_SET_ADJUST_0_317=0x0380, 00081 OC_ADJ_SET_ADJUST_0_358=0x03C0, 00082 OC_ADJ_SET_ADJUST_0_403=0x0400, 00083 OC_ADJ_SET_ADJUST_0_454=0x0440, 00084 OC_ADJ_SET_ADJUST_0_511=0x0480, 00085 OC_ADJ_SET_ADJUST_0_576=0x04C0, 00086 OC_ADJ_SET_ADJUST_0_648=0x0500, 00087 OC_ADJ_SET_ADJUST_0_730=0x0540, 00088 OC_ADJ_SET_ADJUST_0_822=0x0580, 00089 OC_ADJ_SET_ADJUST_0_926=0x05C0, 00090 OC_ADJ_SET_ADJUST_1_043=0x0600, 00091 OC_ADJ_SET_ADJUST_1_175=0x0640, 00092 OC_ADJ_SET_ADJUST_1_324=0x0680, 00093 OC_ADJ_SET_ADJUST_1_491=0x06C0, 00094 OC_ADJ_SET_ADJUST_1_679=0x0700, 00095 OC_ADJ_SET_ADJUST_1_892=0x0740, 00096 OC_ADJ_SET_ADJUST_2_131=0x0780, 00097 OC_ADJ_SET_ADJUST_2_400=0x07C0 00098 }; 00099 enum CTRL2_OCTW_MODE{ 00100 OCTW_MODE_REPORT_OT_OC_BOTH = 0x0000, 00101 OCTW_MODE_REPORT_OVERTEMP_ONLY = 0x0001, 00102 OCTW_MODE_REPORT_OVERCURRENT_ONLY = 0x0002, 00103 OCTW_MODE_REPORT_RESERVED = 0x0003 00104 }; 00105 enum CTRL2_SHUNTGAIN{ 00106 SHUNTGAIN_GAIN_10V_PER_V = 0x0000, 00107 SHUNTGAIN_GAIN_20V_PER_V = 0x0004, 00108 SHUNTGAIN_GAIN_40V_PER_V = 0x0008, 00109 SHUNTGAIN_GAIN_80V_PER_V = 0x000C 00110 }; 00111 enum CTRL2_DC_CAL_CH1{ 00112 DC_CAL_CH1_ENABLE = 0x0000, 00113 DC_CAL_CH1_DISABLE = 0x0010 00114 }; 00115 enum CTRL2_DC_CAL_CH2{ 00116 DC_CAL_CH2_ENABLE = 0x0000, 00117 DC_CAL_CH2_DISABLE = 0x0020 00118 }; 00119 enum CTRL2_OC_TOFF{ 00120 OC_TOFF_CYCLE_BY_CYCLE = 0x0000, 00121 OC_TOFF_OFF_TIME_CONTROL = 0x0040 00122 }; 00123 00124 //DRV8301 Control Class ======================================================== 00125 class drv8301ctrl{ 00126 public: 00127 //Constructor (Overload +3) 00128 drv8301ctrl(Serial *serial, SPI *spi, PinName csel, PinName en_gate); 00129 drv8301ctrl(Serial *serial, SPI *spi, DigitalOut *csel, DigitalOut *en_gate); 00130 drv8301ctrl(SPI *spi, PinName csel, PinName en_gate); 00131 drv8301ctrl(SPI *spi, DigitalOut *csel, DigitalOut *en_gate); 00132 00133 //Destructor 00134 ~drv8301ctrl(); 00135 00136 private: 00137 Serial *pc; 00138 SPI *si; 00139 DigitalOut *cs, *gate; 00140 00141 bool hasSerial; 00142 unsigned short writeValue1, writeValue2; 00143 unsigned char devID; 00144 00145 //SPI read/write command 00146 int spi_cmd(int val, bool debug=true); 00147 00148 public: 00149 00150 //initialize:初期化 00151 void init(bool reset_iface=true); 00152 00153 //read status register1:(ステータスレジスタ1の読み取り) 00154 //FAULT, GVDD_UV, PVDD_UV, OTSD, OTW, 00155 //FETHA_OC, FETLA_OC, FETHB_OC, FETLB_OC, FETHC_OC, FETLC_OC 00156 unsigned short readStatus1(); 00157 00158 //read status register2:(ステータスレジスタ2の読み取り) 00159 //GVDD_OV, DEVICE_ID 00160 unsigned short readStatus2(); 00161 00162 //read status:FAULT 00163 bool readFault(); 00164 //read status:GVDD_UV(GVDD under voltage) 00165 bool readGVDD_UV(); 00166 //read status:PVDD_UV(PVDD under voltage) 00167 bool readPVDD_UV(); 00168 //read status:OTSD(Over Temperature ShutDown: over 150 degree C) 00169 bool readOTSD(); 00170 //read status:OTW(Over Temperature Warning: over 130 degree C) 00171 bool readOTW(); 00172 //read status:FETHA_OC(MOSFET A High-Side Over Current: ref OC_ADJ) 00173 bool readFETHA_OC(); 00174 //read status:FETLA_OC(MOSFET A Low-Side Over Current: ref OC_ADJ) 00175 bool readFETLA_OC(); 00176 //read status:FETHB_OC(MOSFET B High-Side Over Current: ref OC_ADJ) 00177 bool readFETHB_OC(); 00178 //read status:FETLB_OC(MOSFET B Low-Side Over Current: ref OC_ADJ) 00179 bool readFETLB_OC(); 00180 //read status:FETHC_OC(MOSFET C High-Side Over Current: ref OC_ADJ) 00181 bool readFETHC_OC(); 00182 //read status:FETLC_OC(MOSFET C Low-Side Over Current: ref OC_ADJ) 00183 bool readFETLC_OC(); 00184 //read status:GVDD_OV(GVDD over voltage) 00185 bool readGVDD_OV(); 00186 //read status:DEVICE_ID 00187 unsigned char readDEVICE_ID(); 00188 00189 //read control register1:(コントロールレジスタ1の読み取り) 00190 //GATE_CURRENT, GATE_RESET, PWM_MODE, OCP_MODE, OC_ADJ_SET 00191 unsigned short readCtrl1(); 00192 00193 //read control register2:(コントロールレジスタ2の読み取り) 00194 //OCTW_MODE, GAIN, DC_CAL_CH1, DC_CAL_CH2, OC_TOFF 00195 unsigned short readCtrl2(); 00196 00197 //read settings:GATE_CURRENT(MOSFET Gate drive peak current) 00198 unsigned char readGATE_CURRENT(); //HexValue 00199 float readValGATE_CURRENT(); //RealValue 00200 //read settings:GATE_RESET(MOSFET Gate reset mode) 00201 bool readGATE_RESETisNormal(); 00202 //read settings:PWM_MODE(6-pwm or 3-pwm) 00203 bool readPWM_MODEis6PWM(); 00204 //read settings:OCP_MODE(Over Current Protection mode) 00205 unsigned char readOCP_MODE(); 00206 //read settings:OC_ADJ_SET(Over Curent Adjust set) 00207 unsigned char readOC_ADJ_SET(); //HexValue 00208 float readValOC_ADJ_SET(); //RealValue 00209 //read settings:OCTW_MODE(Over Current and Temperature Warning mode) 00210 unsigned char readOCTW_MODE(); 00211 //read settings:GAIN(Shunt amp gain) 00212 unsigned char readGAIN(); 00213 //read settings:DC_CAL_CH1(Calibration Shunt amp CH-1) 00214 bool readDC_CAL_CH1isEnabled(); 00215 //read settings:DC_CAL_CH2(Calibration Shunt amp CH-2) 00216 bool readDC_CAL_CH2isEnabled(); 00217 //read settings:OC_TOFF(Over Current MOSFET gate off mode) 00218 bool readOC_TOFFisCycleByCycle(); 00219 00220 //write control register1:(コントロールレジスタ1へ書き込み) 00221 bool writeCtrl1(unsigned short val=0xffff); 00222 00223 //write control register2:(コントロールレジスタ2へ書き込み) 00224 bool writeCtrl2(unsigned short val=0xffff); 00225 00226 //writeValue clear:(内部に保持しているレジスタの仮の値を消去) 00227 void resetWriteValue1(); 00228 void resetWriteValue2(); 00229 00230 //writeValue direct update:(内部に保持しているレジスタの仮の値を更新) 00231 void updateWriteValue1(unsigned short val); 00232 void updateWriteValue2(unsigned short val); 00233 00234 //set value:GATE_CURRENT(MOSFET Gate drive peak current) 00235 void setGATE_CURRENT(unsigned char hexVal); 00236 void setGATE_CURRENT(float realVal); 00237 //set value:GATE_RESET(MOSFET Gate reset mode) 00238 void setGATE_RESET(bool isNormal); 00239 //set value:PWM_MODE(6-pwm or 3-pwm) 00240 void setPWM_MODE(bool is6PWM); 00241 //set value:OCP_MODE(Over Current Protection mode) 00242 void setOCP_MODE(unsigned char val); 00243 //set value:OC_ADJ_SET(Over Curent Adjust set) 00244 void setOC_ADJ(unsigned char hexVal); 00245 void setOC_ADJ(float realVal); 00246 //set value:OCTW_MODE(Over Current and Temperature Warning mode) 00247 void setOCTW_MODE(unsigned char val); 00248 //set value:GAIN(Shunt amp gain) 00249 void setGAIN(unsigned char val); 00250 //set value:DC_CAL_CH1(Calibration Shunt amp CH-1) 00251 void setDC_CAL_CH1_Enabled(bool enable); 00252 //set value:DC_CAL_CH2(Calibration Shunt amp CH-2) 00253 void setDC_CAL_CH2_Enabled(bool enable); 00254 //set value:OC_TOFF(Over Current MOSFET gate off mode) 00255 void setOC_TOFF_CycleByCycle(bool enable); 00256 00257 //EN_GATE on,off:(EN_GATEピンの状態セット) 00258 void gateEnable(); 00259 void gateDisable(); 00260 void gateReset(); 00261 }; 00262 00263 #endif
Generated on Mon Jul 18 2022 11:39:46 by
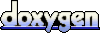