Simple piece of code for simulation sensor inputs of a hoverboard to do basic control of wheels.
Dependencies: WiiNunchuck mbed-dev
HallDecoder.cpp
00001 #include "HallDecoder.h" 00002 00003 00004 HallDecoder::HallDecoder(PinName pin1, PinName pin2, PinName pin3) : _int1(pin1), _int2(pin2), _int3(pin3) 00005 { 00006 _lastint = 0; 00007 _ticks = 0; 00008 _int1.rise(this, &HallDecoder::hall1_handler); 00009 _int1.fall(this, &HallDecoder::hall1_handler); 00010 _int2.rise(this, &HallDecoder::hall2_handler); 00011 _int2.fall(this, &HallDecoder::hall2_handler); 00012 _int3.rise(this, &HallDecoder::hall3_handler); 00013 _int3.fall(this, &HallDecoder::hall3_handler); 00014 } 00015 00016 00017 void HallDecoder::hall1_handler() 00018 { 00019 if(_lastint == 2) { 00020 _ticks++; 00021 } else if(_lastint == 3) { 00022 _ticks--; 00023 } 00024 _lastint = 1; 00025 } 00026 00027 void HallDecoder::hall2_handler() 00028 { 00029 if(_lastint == 3) { 00030 _ticks++; 00031 } else if(_lastint == 1) { 00032 _ticks--; 00033 } 00034 _lastint = 2; 00035 } 00036 00037 void HallDecoder::hall3_handler() 00038 { 00039 if(_lastint == 1) { 00040 _ticks++; 00041 } else if(_lastint == 2) { 00042 _ticks--; 00043 } 00044 _lastint = 3; 00045 } 00046 00047 00048 int32_t HallDecoder::getticks() 00049 { 00050 return _ticks; 00051 } 00052 void HallDecoder::resetticks() 00053 { 00054 _ticks = 0; 00055 }
Generated on Tue Jul 19 2022 23:48:06 by
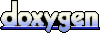