Library to switch 433MHz remote controlled sockets.
Embed:
(wiki syntax)
Show/hide line numbers
RCSwitch.h
Go to the documentation of this file.
00001 /** 00002 *@section DESCRIPTION 00003 * RCSwitch - Ported from the Arduino libary for remote control outlet switches 00004 * Contributors: 00005 * - Andre Koehler / info(at)tomate-online(dot)de 00006 * - Gordeev Andrey Vladimirovich / gordeev(at)openpyro(dot)com 00007 * - Skineffect / http://forum.ardumote.com/viewtopic.php?f=2&t=46 00008 * - Dominik Fischer / dom_fischer(at)web(dot)de 00009 * - Frank Oltmanns / <first name>.<last name>(at)gmail(dot)com 00010 * - Chris Dick / Porting to mbed 00011 * 00012 * Project home: http://code.google.com/p/rc-switch/ 00013 * @section LICENSE 00014 * Copyright (c) 2011 Suat Özgür. All right reserved. 00015 * 00016 * This library is free software; you can redistribute it and/or 00017 * modify it under the terms of the GNU Lesser General Public 00018 * License as published by the Free Software Foundation; either 00019 * version 2.1 of the License, or (at your option) any later version. 00020 * 00021 * This library is distributed in the hope that it will be useful, 00022 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00023 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00024 * Lesser General Public License for more details. 00025 * 00026 * You should have received a copy of the GNU Lesser General Public 00027 * License along with this library; if not, write to the Free Software 00028 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00029 * Permission is hereby granted, free of charge, to any person obtaining a copy 00030 * @file "RCSwitch.h" 00031 */ 00032 #ifndef _RCSwitch_h 00033 #define _RCSwitch_h 00034 #include "mbed.h" 00035 00036 // might need to change this... 00037 // We can handle up to (unsigned long) => 32 bit * 2 H/L changes per bit + 2 for sync 00038 #define RCSWITCH_MAX_CHANGES 67 /**< Number of maximum High/Low changes per packet. */ 00039 00040 #define PROTOCOL3_SYNC_FACTOR 71 /**< Protocol 3 Sync Factor */ 00041 #define PROTOCOL3_0_HIGH_CYCLES 4 /**< Protocol 3 number of high cycles in a 0 */ 00042 #define PROTOCOL3_0_LOW_CYCLES 11 /**< Protocol 3 number of low cycles in a 0*/ 00043 #define PROTOCOL3_1_HIGH_CYCLES 9 /**< Protocol 3 number of high cycles in a 1*/ 00044 #define PROTOCOL3_1_LOW_CYCLES 6 /**< Protocol 3 number of low cycles in a 1*/ 00045 /** RCSwitch Class 00046 * 00047 * Example: 00048 * @code 00049 * #include "mbed.h" 00050 * #include "RCSwitch.h" 00051 * 00052 * // This Example should only do one of either transmit or receive 00053 * //#define TRANSMIT 00054 * #define RECEIVE 00055 * 00056 * Serial pc(USBTX, USBRX); // tx, rx 00057 * RCSwitch mySwitch = RCSwitch( p11, p21 ); //tx, rx 00058 * 00059 * int main() 00060 * { 00061 * pc.printf("Setup"); 00062 * while(1) { 00063 * #ifdef RECEIVE 00064 * if (mySwitch.available()) { 00065 * 00066 * int value = mySwitch.getReceivedValue(); 00067 * 00068 * if (value == 0) { 00069 * pc.printf("Unknown encoding"); 00070 * } else { 00071 * pc.printf("Received %d \n\r", mySwitch.getReceivedValue()); 00072 * pc.printf(" bit %d \n\r", mySwitch.getReceivedBitlength()); 00073 * pc.printf(" Protocol: %d \n\r", mySwitch.getReceivedProtocol()); 00074 * } 00075 * mySwitch.resetAvailable(); 00076 * } 00077 * #endif 00078 * #ifdef TRANSMIT 00079 * // Example: TypeA_WithDIPSwitches 00080 * mySwitch.switchOn("11111", "00010"); 00081 * wait(1); 00082 * mySwitch.switchOn("11111", "00010"); 00083 * wait(1); 00084 * 00085 * // Same switch as above, but using decimal code 00086 * mySwitch.send(5393, 24); 00087 * wait(1); 00088 * mySwitch.send(5396, 24); 00089 * wait(1); 00090 * 00091 * // Same switch as above, but using binary code 00092 * mySwitch.send("000000000001010100010001"); 00093 * wait(1); 00094 * mySwitch.send("000000000001010100010100"); 00095 * wait(1); 00096 * 00097 * // Same switch as above, but tri-state code 00098 * mySwitch.sendTriState("00000FFF0F0F"); 00099 * wait(1); 00100 * mySwitch.sendTriState("00000FFF0FF0"); 00101 * wait(1); 00102 * #endif 00103 * } 00104 * } 00105 * 00106 * @endcode 00107 */ 00108 00109 /** 00110 * 00111 * 00112 */ 00113 class RCSwitch { 00114 00115 public: 00116 /** Class constructor. 00117 * The constructor assigns the specified pinout, attatches 00118 * an Interrupt to the receive pin. for the LPC1768 this must not 00119 * be pins 19 and 20. For the KL25Z, the pin must be on ports A or C 00120 * @param tx Transmitter pin of the RF module. 00121 * @param rx Receiver pin of the RF module. 00122 */ 00123 RCSwitch(PinName tx, PinName rx); 00124 /** Class constructor. 00125 * The constructor assigns the specified pinout, attatches 00126 * an Interrupt to the receive pin. for the LPC1768 this must not 00127 * be pins 19 and 20. For the KL25Z, the pin must be on ports A or C 00128 * @param tx Transmitter pin of the RF module. 00129 * @param rx Receiver pin of the RF module. 00130 * @param tx_en Enable pin of the transmitter 00131 */ 00132 RCSwitch(PinName tx, PinName rx, PinName rx_en); 00133 /** 00134 * Set protocol to be used in transmission 00135 * @param nProtocol Protocol type ot transmit 00136 */ 00137 void setProtocol(int nProtocol); 00138 /** 00139 * Set protocol to be used in transmission 00140 * @param nProtocol Protocol type ot transmit 00141 * @param nPulseLength Length of each pulse 00142 */ 00143 void setProtocol(int nProtocol, int nPulseLength); 00144 /** 00145 * Switch a remote switch on (Type A with 10 pole DIP switches) 00146 * 00147 * @param sGroup Code of the switch group (refers to DIP switches 1..5 where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00148 * @param sDevice Code of the switch device (refers to DIP switches 6..10 (A..E) where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00149 */ 00150 void switchOn(char* sGroup, char* sDevice); 00151 /** 00152 * Switch a remote switch off (Type A with 10 pole DIP switches) 00153 * 00154 * @param sGroup Code of the switch group (refers to DIP switches 1..5 where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00155 * @param sDevice Code of the switch device (refers to DIP switches 6..10 (A..E) where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00156 */ 00157 void switchOff(char* sGroup, char* sDevice); 00158 /** 00159 * Deprecated, use switchOn(char* sGroup, char* sDevice) instead! 00160 * Switch a remote switch on (Type A with 10 pole DIP switches) 00161 * 00162 * @param sGroup Code of the switch group (refers to DIP switches 1..5 where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00163 * @param nChannelCode Number of the switch itself (1..5) 00164 */ 00165 void switchOn(char* sGroup, int nChannelCode); 00166 /** 00167 * Deprecated, use switchOff(char* sGroup, char* sDevice) instead! 00168 * Switch a remote switch off (Type A with 10 pole DIP switches) 00169 * 00170 * @param sGroup Code of the switch group (refers to DIP switches 1..5 where "1" = on and "0" = off, if all DIP switches are on it's "11111") 00171 * @param nChannelCode Number of the switch itself (1..5) 00172 */ 00173 void switchOff(char* sGroup, int nChannelCode); 00174 /** 00175 * Switch a remote switch on (Type B with two rotary/sliding switches) 00176 * 00177 * @param nAddressCode Number of the switch group (1..4) 00178 * @param nChannelCode Number of the switch itself (1..4) 00179 */ 00180 void switchOn(int nAddressCode, int nChannelCode); 00181 /** 00182 * Switch a remote switch off (Type B with two rotary/sliding switches) 00183 * 00184 * @param nAddressCode Number of the switch group (1..4) 00185 * @param nChannelCode Number of the switch itself (1..4) 00186 */ 00187 void switchOff(int nAddressCode, int nChannelCode); 00188 /** 00189 * Switch a remote switch on (Type C Intertechno) 00190 * 00191 * @param sFamily Familycode (a..f) 00192 * @param nGroup Number of group (1..4) 00193 * @param nDevice Number of device (1..4) 00194 */ 00195 void switchOn(char sFamily, int nGroup, int nDevice); 00196 /** 00197 * Switch a remote switch off (Type C Intertechno) 00198 * 00199 * @param sFamily Familycode (a..f) 00200 * @param nGroup Number of group (1..4) 00201 * @param nDevice Number of device (1..4) 00202 */ 00203 void switchOff(char sFamily, int nGroup, int nDevice); 00204 00205 /** 00206 * Switch a remote switch off (Type D REV) 00207 * 00208 * @param sGroup Code of the switch group (A,B,C,D) 00209 * @param nDevice Number of the switch itself (1..3) 00210 */ 00211 void switchOn(char sGroup, int nDevice); 00212 /** 00213 * Switch a remote switch on (Type D REV) 00214 * 00215 * @param sGroup Code of the switch group (A,B,C,D) 00216 * @param nDevice Number of the switch itself (1..3) 00217 */ 00218 void switchOff(char sGroup, int nDevice); 00219 /** 00220 * Sends a codeword 00221 * @param sCodeWord Codeword to be sent 00222 */ 00223 void sendTriState(char* Code); 00224 /** 00225 * Converts a CodeWord to a set Length and sends it 00226 * @param Code CodeWord to be sent 00227 * @param length Length of CodeWord to send 00228 */ 00229 void send(unsigned long Code, unsigned int length); 00230 /** 00231 * Sends a CodeWord 00232 * @param Code CodeWord to send 00233 */ 00234 void send(char* Code); 00235 /** 00236 * Enable receiving data This clear message storage 00237 * and enables the interrupt, which may enable the port 00238 */ 00239 void enableReceive(); 00240 /** 00241 * Disable receiving data This disables the interrupt 00242 * which may disable the port 00243 */ 00244 void disableReceive(); 00245 /** 00246 * Message availiable 00247 * @return bool Message availiability 00248 */ 00249 bool available(); 00250 /** 00251 * Clear Messages 00252 */ 00253 void resetAvailable(); 00254 /** 00255 * Get Message Value 00256 * @return unsigned long The value of the message received 00257 */ 00258 unsigned long getReceivedValue(); 00259 /** 00260 * Get bit length 00261 * @return unsigned int Number of bits received 00262 */ 00263 unsigned int getReceivedBitlength(); 00264 /** 00265 * Get the delay 00266 * @Return unsigned int The delay 00267 */ 00268 unsigned int getReceivedDelay(); 00269 /** 00270 * Get Protocol 00271 * @return unsigned int The protocol used in the message 00272 */ 00273 unsigned int getReceivedProtocol(); 00274 /** 00275 * Get Raw data 00276 * @return unsinged int The raw data of the message recieved 00277 */ 00278 unsigned int* getReceivedRawdata(); 00279 /** 00280 * Enable the transmitter 00281 */ 00282 void enableTransmit(); 00283 /** 00284 * Disable the transmitter 00285 */ 00286 void disableTransmit(); 00287 /** 00288 * Set pulse length in micro seconds 00289 * @param nPulseLength the Length of the pulse 00290 */ 00291 void setPulseLength(int nPulseLength); 00292 /** 00293 * Set number of times to repeat transmission 00294 * @param nRepeat Number of repeats 00295 */ 00296 void setRepeatTransmit(int nRepeatTransmit); 00297 /** 00298 * Set receive tolerance 00299 * @param nPercent Percentage tolerance of the receiver 00300 */ 00301 void setReceiveTolerance(int nPercent); 00302 00303 static int nReceiveTolerance; /**< Tolerance of the receiver */ 00304 static unsigned long nReceivedValue; /**< Value Recieved */ 00305 static unsigned int nReceivedBitlength; /**< Length in bits of value reveived */ 00306 static unsigned int nReceivedDelay; /**< Delay in receive */ 00307 static unsigned int nReceivedProtocol; /**< Protocol of message recieved */ 00308 static bool ReceiveEnabled; /**< Receive enabled */ 00309 static bool TransmitEnable; /**< Transmit enabled */ 00310 static bool TransmitEnablePin; /**< Pin of transmitter enable pin */ 00311 static unsigned int timings[RCSWITCH_MAX_CHANGES]; /**< timings[0] contains sync timing, followed by a number of bits */ 00312 00313 private: 00314 DigitalOut _tx; 00315 InterruptIn _rx; 00316 DigitalOut _tx_en; 00317 00318 char* getCodeWordB(int nGroupNumber, int nSwitchNumber, bool bStatus); 00319 00320 char* getCodeWordA(char* sGroup, int nSwitchNumber, bool bStatus); 00321 00322 char* getCodeWordA(char* sGroup, char* sDevice, bool bStatus); 00323 00324 char* getCodeWordC(char sFamily, int nGroup, int nDevice, bool bStatus); 00325 00326 char* getCodeWordD(char group, int nDevice, bool bStatus); 00327 00328 void sendT0(); 00329 00330 void sendT1(); 00331 00332 void sendTF(); 00333 00334 void send0(); 00335 00336 void send1(); 00337 00338 void sendSync(); 00339 00340 void transmit(int nHighPulses, int nLowPulses); 00341 00342 void RCSwitchRxPinChange(); 00343 00344 static char* dec2binWzerofill(unsigned long dec, unsigned int length); 00345 00346 static char* dec2binWcharfill(unsigned long dec, unsigned int length, char fill); 00347 00348 static void handleInterrupt(); 00349 00350 static bool receiveProtocol1(unsigned int changeCount); 00351 00352 static bool receiveProtocol2(unsigned int changeCount); 00353 00354 static bool receiveProtocol3(unsigned int changeCount); 00355 00356 int nReceiverInterrupt; 00357 int nTransmitterPin; 00358 int nPulseLength; 00359 int nRepeatTransmit; 00360 char nProtocol; 00361 Timer timer; 00362 }; 00363 00364 #endif
Generated on Thu Jul 14 2022 01:54:18 by
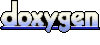