
Final firmware for ConnectorBox TPH 1.0 (NXP LPC1768).
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * ------------------------------- 00003 * ConnectorBoxFW 2.0 (2016-09-26) 00004 * ------------------------------- 00005 * Firmware for ConnectorBox TPH 1.0 (NXP LPC1768). 00006 * 00007 * Copyright (c) 2016, Martin Wolker (neolker@gmail.com) 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without 00011 * modification, are permitted provided that the following conditions are met: 00012 * - Redistributions of source code must retain the above copyright 00013 * notice, this list of conditions and the following disclaimer. 00014 * - Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the distribution. 00017 * - Neither the name of Martin Wolker nor the 00018 * names of its contributors may be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00022 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00023 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL MARTIN WOLKER BE LIABLE FOR ANY 00025 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00026 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00027 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00028 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00029 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 */ 00032 00033 #include "mbed.h" 00034 #include "USBSerial.h" 00035 00036 #define ROT_SPEED 19200 00037 #define ROT_TIMEOUT 250 00038 #define ROT_INTERVAL 350 00039 #define TEMPERATURE_START 29 00040 #define TEMPERATURE_END 35 00041 #define HUMIDITY_START 12 00042 #define HUMIDITY_END 18 00043 #define SERIAL_START 77 00044 #define SERIAL_END 85 00045 #define LED_DELAY 100 00046 00047 USBSerial PC; 00048 Serial ROT_01(p28, p27); 00049 Serial ROT_02(p9, p10); 00050 DigitalInOut ID_01(p11); 00051 DigitalInOut ID_02(p12); 00052 DigitalInOut ID_03(p13); 00053 DigitalInOut ID_04(p14); 00054 DigitalInOut ID_05(p21); 00055 DigitalInOut ID_06(p22); 00056 DigitalInOut ID_07(p25); 00057 DigitalInOut ID_08(p26); 00058 DigitalInOut ID_09(p5); 00059 DigitalInOut ID_10(p6); 00060 DigitalInOut ID_11(p7); 00061 DigitalInOut ID_12(p8); 00062 DigitalInOut ID_13(p29); 00063 DigitalInOut ID_14(p30); 00064 DigitalInOut ID_15(p18); 00065 DigitalInOut ID_16(p17); 00066 DigitalInOut ID_17(p16); 00067 DigitalInOut ID_18(p15); 00068 DigitalInOut ID_19(p20); 00069 DigitalInOut ID_20(p19); 00070 DigitalOut LED(LED1); 00071 00072 enum DELAY {A = 6, B = 64, C = 60, D = 10, E = 9, F = 55, G = 0, H = 480, I = 70, J = 410}; 00073 00074 void GetHelp() 00075 { 00076 PC.printf("+-----------------------------------+\r\n"); 00077 PC.printf("| HELP - List of commands |\r\n"); 00078 PC.printf("+-----------------------------------+\r\n"); 00079 PC.printf("| a -> Read all IDs and all ROTs |\r\n"); 00080 PC.printf("| d -> Debug of ROT_01 (RAW DATA) |\r\n"); 00081 PC.printf("| h -> Get this HELP |\r\n"); 00082 PC.printf("| i -> Read all IDs |\r\n"); 00083 PC.printf("| p -> Periodic reading of all ROTs |\r\n"); 00084 PC.printf("| r -> Read all ROTs |\r\n"); 00085 PC.printf("| s -> Signalization by USB_LED |\r\n"); 00086 PC.printf("| t -> Test of ID_01 and ROT_01 |\r\n"); 00087 PC.printf("| v -> Get version of firmware |\r\n"); 00088 PC.printf("+-----------------------------------+\r\n"); 00089 } 00090 00091 void Blink(int info) 00092 { 00093 if (info) PC.printf("LED blinking\r\n"); 00094 for (int i = 0; i < 2; i++) { 00095 LED = 1; 00096 wait_ms(LED_DELAY); 00097 LED = 0; 00098 wait_ms(LED_DELAY); 00099 } 00100 } 00101 00102 int Reset(DigitalInOut& pin) 00103 { 00104 pin.output(); 00105 pin = 0; 00106 wait_us(H); 00107 pin.input(); 00108 wait_us(I); 00109 uint32_t result = pin; 00110 wait_us(J); 00111 return result; 00112 } 00113 00114 void WriteBit(DigitalInOut& pin, uint32_t bit) 00115 { 00116 pin.output(); 00117 if (bit) { 00118 pin = 0; 00119 wait_us(A); 00120 pin.input(); 00121 wait_us(B); 00122 } else { 00123 pin = 0; 00124 wait_us(C); 00125 pin.input(); 00126 wait_us(D); 00127 } 00128 } 00129 00130 uint32_t ReadBit(DigitalInOut& pin) 00131 { 00132 uint32_t bit_value; 00133 pin.output(); 00134 pin = 0; 00135 wait_us(A); 00136 pin.input(); 00137 wait_us(E); 00138 bit_value = pin; 00139 wait_us(F); 00140 return bit_value; 00141 } 00142 00143 void WriteByte(DigitalInOut& pin, uint32_t byte) 00144 { 00145 for (uint32_t bit = 0; bit < 8; ++bit) { 00146 WriteBit(pin, byte & 0x01); 00147 byte >>= 1; 00148 } 00149 } 00150 00151 uint32_t ReadByte(DigitalInOut& pin) 00152 { 00153 uint32_t byte = 0; 00154 for (uint32_t bit = 0; bit < 8; ++bit) { 00155 byte |= (ReadBit(pin) << bit); 00156 } 00157 return byte; 00158 } 00159 00160 void GetID(DigitalInOut& pin, int number) 00161 { 00162 Reset(pin); 00163 WriteByte(pin, 0x33); 00164 int B8 = ReadByte(pin); 00165 int B7 = ReadByte(pin); 00166 int B6 = ReadByte(pin); 00167 int B5 = ReadByte(pin); 00168 int B4 = ReadByte(pin); 00169 int B3 = ReadByte(pin); 00170 int B2 = ReadByte(pin); 00171 int B1 = ReadByte(pin); 00172 PC.printf("ID_%02d: %02X%02X%02X%02X%02X%02X%02X%02X\r\n", number, B1, B2, B3, B4, B5, B6, B7, B8); 00173 } 00174 00175 void GetROT(Serial& port, int number, int debug) 00176 { 00177 Timer t; 00178 int position = 0; 00179 char symbol = '0'; 00180 char buffer[255] = ""; 00181 t.start(); 00182 port.printf("{F99RDD-\r"); 00183 while((symbol != '\r') && (t.read_ms() < ROT_TIMEOUT)) { 00184 if(port.readable()) { 00185 symbol = port.getc(); 00186 buffer[position] = symbol; 00187 position++; 00188 } 00189 } 00190 t.stop(); 00191 if (debug) { 00192 PC.printf("------DEBUG_START-------\r\n"); 00193 PC.printf("Reading time is: %d ms\r\n", t.read_ms()); 00194 PC.printf("Count of characters: %d\r\n", position); 00195 PC.printf("ROT_%02d (RAW DATA):\r\n", number); 00196 if (position == 0) { 00197 PC.printf("N/A\r\n"); 00198 } else { 00199 for (int d = 0; d < 105; d++) { 00200 PC.printf("%c", buffer[d]); 00201 } 00202 PC.printf("\n"); 00203 } 00204 PC.printf("ROT_%02d (PARSED DATA):\r\n", number); 00205 } 00206 t.reset(); 00207 if ((position == 105) && (buffer[16] != '-')) { 00208 PC.printf("ROT_%02d: ", number); 00209 for (int i = TEMPERATURE_START; i < TEMPERATURE_END; i++) { 00210 if (buffer[i] == '.') { 00211 PC.printf(","); 00212 } else { 00213 PC.printf("%c", buffer[i]); 00214 } 00215 } 00216 PC.printf("; "); 00217 for (int j = HUMIDITY_START; j < HUMIDITY_END; j++) { 00218 if (buffer[j] == '.') { 00219 PC.printf(","); 00220 } else { 00221 PC.printf("%c", buffer[j]); 00222 } 00223 } 00224 PC.printf("; "); 00225 for (int k = SERIAL_START; k < SERIAL_END; k++) { 00226 PC.printf("%c", buffer[k]); 00227 } 00228 PC.printf("\r\n"); 00229 } else { 00230 PC.printf("ROT_%02d: 0,00; 0,00; 00000000\r\n", number); 00231 } 00232 if (debug) PC.printf("-------DEBUG_END--------\r\n"); 00233 } 00234 00235 void GetVersion() 00236 { 00237 PC.printf("+--------------------------------+\r\n"); 00238 PC.printf("| ConnectorBox TPH 1.0 |\r\n"); 00239 PC.printf("+--------------------------------+\r\n"); 00240 PC.printf("| FW version: 2.0 (2016-09-26) |\r\n"); 00241 PC.printf("| Author: Martin Wolker |\r\n"); 00242 PC.printf("| Contact: neolker@gmail.com |\r\n"); 00243 PC.printf("+--------------------------------+\r\n"); 00244 } 00245 00246 int main(void) 00247 { 00248 LED = 0; 00249 int flag = 1; 00250 ROT_01.baud(ROT_SPEED); 00251 ROT_02.baud(ROT_SPEED); 00252 Blink(0); 00253 wait_ms(100); 00254 while(1) { 00255 if (PC.readable()) { 00256 switch(PC.getc()) { 00257 case 'A': 00258 case 'a': 00259 GetID(ID_01, 1); 00260 GetID(ID_02, 2); 00261 GetID(ID_03, 3); 00262 GetID(ID_04, 4); 00263 GetID(ID_05, 5); 00264 GetID(ID_06, 6); 00265 GetID(ID_07, 7); 00266 GetID(ID_08, 8); 00267 GetID(ID_09, 9); 00268 GetID(ID_10, 10); 00269 GetID(ID_11, 11); 00270 GetID(ID_12, 12); 00271 GetID(ID_13, 13); 00272 GetID(ID_14, 14); 00273 GetID(ID_15, 15); 00274 GetID(ID_16, 16); 00275 GetID(ID_17, 17); 00276 GetID(ID_18, 18); 00277 GetID(ID_19, 19); 00278 GetID(ID_20, 20); 00279 GetROT(ROT_01, 1, 0); 00280 GetROT(ROT_02, 2, 0); 00281 Blink(0); 00282 break; 00283 case 'D': 00284 case 'd': 00285 GetROT(ROT_01, 1, 1); 00286 Blink(0); 00287 break; 00288 case 'H': 00289 case 'h': 00290 GetHelp(); 00291 Blink(0); 00292 break; 00293 case 'I': 00294 case 'i': 00295 GetID(ID_01, 1); 00296 GetID(ID_02, 2); 00297 GetID(ID_03, 3); 00298 GetID(ID_04, 4); 00299 GetID(ID_05, 5); 00300 GetID(ID_06, 6); 00301 GetID(ID_07, 7); 00302 GetID(ID_08, 8); 00303 GetID(ID_09, 9); 00304 GetID(ID_10, 10); 00305 GetID(ID_11, 11); 00306 GetID(ID_12, 12); 00307 GetID(ID_13, 13); 00308 GetID(ID_14, 14); 00309 GetID(ID_15, 15); 00310 GetID(ID_16, 16); 00311 GetID(ID_17, 17); 00312 GetID(ID_18, 18); 00313 GetID(ID_19, 19); 00314 GetID(ID_20, 20); 00315 Blink(0); 00316 break; 00317 case 'P': 00318 case 'p': 00319 PC.printf("-------------START--------------\r\n"); 00320 while(flag) { 00321 GetROT(ROT_01, 1, 0); 00322 GetROT(ROT_02, 2, 0); 00323 Blink(0); 00324 wait_ms(ROT_INTERVAL); 00325 if (PC.readable()) { 00326 PC.getc(); 00327 PC.printf("--------------END---------------\r\n"); 00328 flag = 0; 00329 } 00330 } 00331 flag = 1; 00332 break; 00333 case 'R': 00334 case 'r': 00335 GetROT(ROT_01, 1, 0); 00336 GetROT(ROT_02, 2, 0); 00337 Blink(0); 00338 break; 00339 case 'S': 00340 case 's': 00341 Blink(1); 00342 break; 00343 case 'T': 00344 case 't': 00345 GetID(ID_01, 1); 00346 GetROT(ROT_01, 1, 0); 00347 Blink(0); 00348 break; 00349 case 'V': 00350 case 'v': 00351 GetVersion(); 00352 Blink(0); 00353 break; 00354 default: 00355 PC.printf("Unknown command, try \"h\" for HELP...\r\n"); 00356 Blink(0); 00357 break; 00358 } 00359 } 00360 } 00361 }
Generated on Sat Jul 23 2022 16:13:51 by
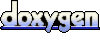