
test for the SPI_TFT Lib
Dependencies: SPI_TFT TFT_fonts mbed
Fork of TFT_Test1 by
main.cpp
00001 // example to test the TFT Display 00002 // Thanks to the GraphicsDisplay and TextDisplay classes from 00003 00004 #include "stdio.h" 00005 #include "mbed.h" 00006 #include "SPI_TFT.h" 00007 #include "string" 00008 #include "Arial12x12.h" 00009 #include "Arial24x23.h" 00010 #include "Arial28x28.h" 00011 #include "font_big.h" 00012 00013 extern unsigned char p1[]; // the mbed logo 00014 00015 // the TFT is connected to SPI pin 5-7 00016 //SPI_TFT TFT(p11, p12, p13, p14, p15,"TFT"); // mosi, miso, sclk, cs, reset 00017 SPI_TFT TFT(CLCD_MOSI, CLCD_MISO, CLCD_SCLK, CLCD_SSEL , CLCD_RESET, "TFT"); // mosi, miso, sclk, cs, reset 00018 Serial pc(USBTX, USBRX); 00019 00020 const uint16_t colorTable[18] = { 00021 Black, 00022 Navy, 00023 DarkGreen, 00024 DarkCyan, 00025 Maroon, 00026 Purple, 00027 Olive, 00028 LightGrey, 00029 DarkGrey, 00030 Blue, 00031 Green, 00032 Cyan, 00033 Red, 00034 Magenta, 00035 Yellow, 00036 White, 00037 Orange, 00038 GreenYellow 00039 }; 00040 00041 void screen2(void) // Graphics 00042 { 00043 //Draw some graphics 00044 int i, x[2], y[2] ; 00045 00046 TFT.background(Black); 00047 TFT.foreground(White); 00048 TFT.cls() ; 00049 TFT.set_font((unsigned char*) Arial12x12); 00050 TFT.locate(90,0); 00051 TFT.printf("Graphics"); 00052 00053 x[0] = 25 ; 00054 x[1] = 224 ; 00055 y[0] = 20 ; 00056 y[1] = 219 ; 00057 for (i = 20 ; i < 220 ; i += 10) { 00058 TFT.line(i+5, y[0], i+5, y[1], Blue) ; 00059 TFT.line(x[0], i, x[1], i, Blue) ; 00060 } 00061 TFT.line(125, y[0], 125, y[1], Green) ; 00062 TFT.line(x[0], 120, x[1], 120, Green) ; 00063 TFT.rect(x[0],y[0], x[1], y[1], Green) ; 00064 TFT.locate(10, 20) ; 00065 TFT.printf("V") ; 00066 TFT.locate(0, 115) ; 00067 TFT.printf("0.0") ; 00068 TFT.locate(115, 225) ; 00069 TFT.printf("0.0") ; 00070 TFT.locate(215, 225) ; 00071 TFT.printf("T") ; 00072 00073 double s; 00074 for (int i = x[0]; i < 225; i++) { 00075 s = 40 * sin((long double)i / 20); 00076 TFT.pixel(i, 120 + (int)s, White); 00077 } 00078 #if 0 00079 TFT.fillrect(10, 240, 229, 309, White) ; 00080 TFT.rect(10, 240, 229, 309, Red) ; 00081 TFT.rect(11, 241, 228, 308, Red) ; 00082 00083 TFT.background(White) ; 00084 TFT.foreground(Black) ; 00085 TFT.locate(20, 250) ; 00086 TFT.printf("With QVGA resolution") ; 00087 TFT.locate(20, 270) ; 00088 TFT.printf("simple graphics drawing") ; 00089 TFT.locate(20, 290) ; 00090 TFT.printf("capability is provided") ; 00091 #endif 00092 } 00093 00094 int main() 00095 { 00096 pc.baud(38400); 00097 pc.printf("Hello, mbed world.\n"); 00098 pc.printf("System core lock : %d\n", SystemCoreClock); 00099 00100 TFT.claim(stdout); // send stdout to the TFT display 00101 //TFT.claim(stderr); // send stderr to the TFT display 00102 00103 while(1) { 00104 TFT.background(Black); // set background to black 00105 TFT.foreground(White); // set chars to white 00106 TFT.cls(); // clear the screen 00107 TFT.set_font((unsigned char*) Arial12x12); // select the font 00108 00109 // first show the 4 directions 00110 TFT.set_orientation(0); 00111 TFT.locate(0,0); 00112 printf(" Hello Mbed 0"); 00113 TFT.set_orientation(1); 00114 TFT.locate(0,0); 00115 printf(" Hello Mbed 1"); 00116 TFT.set_orientation(2); 00117 TFT.locate(0,0); 00118 printf(" Hello Mbed 2"); 00119 TFT.set_orientation(3); 00120 TFT.locate(0,0); 00121 printf(" Hello Mbed 3"); 00122 TFT.set_orientation(1); 00123 TFT.set_font((unsigned char*) Arial24x23); 00124 TFT.locate(50,100); 00125 TFT.printf("TFT orientation"); 00126 00127 wait(5); // wait two seconds 00128 00129 screen2(); 00130 wait(5); 00131 00132 // draw some graphics 00133 TFT.background(Black); // set background to black 00134 TFT.foreground(White); // set chars to white 00135 TFT.cls(); 00136 TFT.set_orientation(1); 00137 TFT.set_font((unsigned char*) Arial24x23); 00138 TFT.locate(120,30); 00139 TFT.printf("Graphic"); 00140 00141 /* 00142 TFT.line(0,0,100,200,Green); 00143 TFT.rect(100,50,150,100,Red); 00144 TFT.fillrect(180,25,220,70,Blue); 00145 TFT.circle(80,150,33,White); 00146 */ 00147 00148 int c = 0; 00149 for(int i = 0; i < 32; i++) { 00150 TFT.fillrect(i*10, i*7, i*10 + 20, i*7 + 20, colorTable[c++]); 00151 TFT.rect(i*10, 240 - i*7,i*10 + 20, 240 - i*7 - 20, colorTable[c++]); 00152 TFT.circle(i*10, 120, 20, colorTable[c++]); 00153 if (c > 18) 00154 c = 0; 00155 } 00156 00157 wait(5); // wait two seconds 00158 00159 // bigger text 00160 TFT.foreground(White); 00161 TFT.background(Blue); 00162 TFT.cls(); 00163 TFT.set_font((unsigned char*) Arial24x23); 00164 TFT.locate(0,0); 00165 TFT.printf("Different Fonts :"); 00166 00167 TFT.set_font((unsigned char*) Neu42x35); 00168 TFT.locate(0,30); 00169 TFT.printf("Hello Mbed 1"); 00170 TFT.set_font((unsigned char*) Arial24x23); 00171 TFT.locate(20,80); 00172 TFT.printf("Hello Mbed 2"); 00173 TFT.set_font((unsigned char*) Arial12x12); 00174 TFT.locate(35,120); 00175 TFT.printf("Hello Mbed 3"); 00176 wait(5); 00177 00178 // mbed logo 00179 TFT.set_orientation(1); 00180 TFT.background(Black); 00181 TFT.cls(); 00182 TFT.Bitmap(90,90,172,55,p1); 00183 wait(5); 00184 } 00185 } 00186
Generated on Sat Jul 16 2022 05:30:48 by
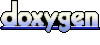