Fujitsu MB85RSxx SPI FRAM access library
Dependents: MB85RSxx_Hello TYBLE16_simple_data_logger
MB85RSxx_SPI.h
00001 /** 00002 ****************************************************************************** 00003 * @file MB85RSxx_SPI.h 00004 * @author Toyomasa Watarai 00005 * @version V1.0.0 00006 * @date 24 April 2017 00007 * @brief This file contains the class of an MB85RSxx FRAM library with SPI interface 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * Permission is hereby granted, free of charge, to any person obtaining a copy 00012 * of this software and associated documentation files (the "Software"), to deal 00013 * in the Software without restriction, including without limitation the rights 00014 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00015 * copies of the Software, and to permit persons to whom the Software is 00016 * furnished to do so, subject to the following conditions: 00017 * 00018 * The above copyright notice and this permission notice shall be included in 00019 * all copies or substantial portions of the Software. 00020 * 00021 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00022 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00023 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00024 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00025 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00026 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00027 * THE SOFTWARE. 00028 */ 00029 00030 #ifndef MBED_MB85RSxx_SPI_H 00031 #define MBED_MB85RSxx_SPI_H 00032 00033 #include "mbed.h" 00034 00035 #define MB85_WREN 0x06 00036 #define MB85_WRDI 0x04 00037 #define MB85_RDSR 0x05 00038 #define MB85_WRSR 0x01 00039 #define MB85_READ 0x03 00040 #define MB85_WRITE 0x02 00041 #define MB85_RDID 0x9F 00042 #define MB85_FSTRD 0x0B 00043 #define MB85_SLEEP 0xB9 00044 00045 #define MB85_DENSITY_64K 0x3 00046 #define MB85_DENSITY_256K 0x5 00047 #define MB85_DENSITY_512K 0x6 00048 #define MB85_DENSITY_1M 0x7 00049 #define MB85_DENSITY_2M 0x8 00050 00051 /** Interface for accessing Fujitsu MB85RSxx FRAM 00052 * 00053 * @code 00054 * #include "mbed.h" 00055 * #include "MB85RCxx_I2C.h" 00056 * 00057 * Serial pc(USBTX, USBRX); 00058 * 00059 * #if defined(TARGET_LPC1768) 00060 * MB85RSxx_SPI _spi(p5, p6, p7, p8); // mosi, miso, sclk, cs 00061 * #elif defined(TARGET_LPC1114) 00062 * MB85RSxx_SPI _spi(dp2, dp1, dp6, dp9); // mosi, miso, sclk, cs 00063 * #else // Arduino R3 Shield form factor 00064 * MB85RSxx_SPI fram(D11, D12, D13, D10); // mosi, miso, sclk, cs 00065 * #endif 00066 * 00067 * int main() 00068 * { 00069 * uint8_t buf[16]; 00070 * uint32_t address; 00071 * 00072 * fram.write_enable(); 00073 * fram.fill(0, 0, 256); 00074 * 00075 * for (int i = 0; i < 16; i++) { 00076 * buf[i] = i; 00077 * } 00078 * fram.write(0, buf, 16); 00079 * 00080 * for (address = 0; address < 0x80; address += 16) { 00081 * fram.read(address, buf, 16); 00082 * pc.printf("%08X : ", address); 00083 * for (int i = 0; i < 16; i++) { 00084 * pc.printf("%02X ", buf[i]); 00085 * } 00086 * pc.printf("\n"); 00087 * } 00088 * } 00089 * 00090 * @endcode 00091 */ 00092 00093 /** MB85RSxx_SPI class 00094 * 00095 * MB85RSxx_SPI: A library to access Fujitsu MB85RSxx FRAM 00096 * 00097 */ 00098 class MB85RSxx_SPI 00099 { 00100 public: 00101 00102 /** Create an MB85RSxx_SPI instance 00103 * which is connected to specified SPI pins and chip select pin 00104 * 00105 * @param mosi SPI Master Out, Slave In pin 00106 * @param miso SPI Master In, Slave Out pin 00107 * @param sclk SPI Clock pin 00108 * @param cs Chip Select pin 00109 */ 00110 MB85RSxx_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs); 00111 00112 /** Destructor of MB85RSxx_SPI 00113 */ 00114 virtual ~MB85RSxx_SPI(); 00115 00116 /** Set the SPI bus clock frequency 00117 * 00118 * @param hz SPI bus clock frequency 00119 * 00120 */ 00121 void frequency(int hz); 00122 00123 /** Read device ID from MB85RSxx FRAM 00124 * 00125 * @param device_id Pointer to the byte-array to read data in to 00126 * 00127 * @returns memory dencity 00128 */ 00129 void read_device_id(uint8_t* device_id); 00130 00131 /** Read status register from MB85RSxx FRAM 00132 * 00133 * @returns Status register value 00134 */ 00135 uint8_t read_status(); 00136 00137 /** Read data from memory address 00138 * 00139 * @param address Memory address 00140 * @param data Pointer to the byte-array to read data in to 00141 * @param length Number of bytes to read 00142 * 00143 */ 00144 void read(uint32_t address, uint8_t* data, uint32_t length); 00145 00146 /** Read byte data from memory address 00147 * 00148 * @param address Memory address 00149 * 00150 * @returns Read out data 00151 */ 00152 uint8_t read(uint32_t address); 00153 00154 /** Write data to memory address 00155 * 00156 * @param address Memory address 00157 * @param data Pointer to the byte-array data to write 00158 * @param length Number of bytes to write 00159 * 00160 */ 00161 void write(uint32_t address, uint8_t* data, uint32_t length); 00162 00163 /** Write data to memory address 00164 * 00165 * @param address Memory address 00166 * @param data data to write out to memory 00167 * 00168 */ 00169 void write(uint32_t address, uint8_t data); 00170 00171 /** Fill data to memory address 00172 * 00173 * @param address Memory address 00174 * @param data data to fill out to memory 00175 * @param length Number of bytes to write 00176 * 00177 */ 00178 void fill(uint32_t address, uint8_t data, uint32_t length); 00179 00180 /** Enable write access 00181 */ 00182 void write_enable(); 00183 00184 /** Disable write access 00185 */ 00186 void write_disable(); 00187 00188 private: 00189 SPI _spi; 00190 DigitalOut _cs; 00191 int _address_bits; 00192 00193 }; 00194 00195 #endif
Generated on Wed Jul 13 2022 19:22:52 by
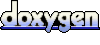