
Fujitsu MB85RSxx serial FRAM test program
Dependencies: mbed MB85RSxx_SPI
main.cpp
00001 #include "mbed.h" 00002 #include "MB85RSxx_SPI.h" 00003 00004 DigitalOut myled(LED1); 00005 Serial pc(USBTX, USBRX); 00006 00007 #if defined(TARGET_LPC1768) 00008 MB85RSxx_SPI fram(p5, p6, p7, p8); // mosi, miso, sclk, cs 00009 #elif defined(TARGET_LPC1114) 00010 MB85RSxx_SPI fram(dp2, dp1, dp6, dp9); // mosi, miso, sclk, cs 00011 #else // Arduino R3 Shield form factor 00012 MB85RSxx_SPI fram(D11, D12, D13, D10); // mosi, miso, sclk, cs 00013 #endif 00014 00015 int main() 00016 { 00017 uint8_t buf[16]; 00018 uint32_t address; 00019 00020 pc.baud(115200); 00021 pc.printf("\nFujitsu MB85RSxxx FRAM test program\n\n"); 00022 00023 // Read device ID and detect memory density for addressing 00024 fram.read_device_id(buf); 00025 pc.printf("read device ID = 0x%x 0x%x 0x%x 0x%x\n", buf[0], buf[1], buf[2], buf[3]); 00026 00027 fram.write_enable(); 00028 pc.printf("read status (WREN) = 0x%x\n", fram.read_status()); 00029 00030 fram.write_disable(); 00031 pc.printf("read status (WRDI) = 0x%x\n", fram.read_status()); 00032 00033 // Write 0 data 00034 fram.write_enable(); 00035 fram.fill(0, 0, 256); 00036 00037 // Prepare write data 00038 for (int i = 0; i < 16; i++) { 00039 buf[i] = i; 00040 } 00041 00042 // Write data with write enable 00043 fram.write_enable(); 00044 fram.write(0x00, buf, 16); 00045 00046 // Attempt to write data (not written) 00047 fram.write(0x10, buf, 16); 00048 00049 // Write data with write enable 00050 fram.write_enable(); 00051 fram.write(0x20, buf, 16); 00052 00053 // Read data 00054 for (address = 0; address < 0x80; address += 16) { 00055 fram.read(address, buf, 16); 00056 pc.printf("%08X : ", address); 00057 for (int i = 0; i < 16; i++) { 00058 pc.printf("%02X ", buf[i]); 00059 } 00060 pc.printf("\n"); 00061 } 00062 00063 // Write number from 0 to 255 00064 pc.printf("\n"); 00065 for (address = 0; address < 0x100; address++) { 00066 fram.write_enable(); 00067 fram.write(address, (uint8_t)address); 00068 } 00069 // Read data 00070 for (address = 0; address < 0x100; address += 16) { 00071 fram.read(address, buf, 16); 00072 pc.printf("%08X : ", address); 00073 for (int i = 0; i < 16; i++) { 00074 pc.printf("%02X ", buf[i]); 00075 } 00076 pc.printf("\n"); 00077 } 00078 00079 while(1) { 00080 myled = 1; 00081 wait(0.2); 00082 myled = 0; 00083 wait(0.2); 00084 } 00085 } 00086
Generated on Tue Jul 19 2022 03:13:08 by
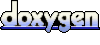