Cypress F-RAM FM25W256 library
Dependents: Hello-FM25W256 Hello-FM25W256
FM25W256.cpp
00001 /* Cypress FM25W256 F-RAM component library 00002 * 00003 * Copyright (c) 2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * * 00017 * @author Toyomasa Watarai 00018 * @version 1.0 00019 * @date 5-March-2016 00020 * 00021 * http://www.cypress.com/products/nonvolatile-ram 00022 * http://www.cypress.com/documentation/datasheets/fm25w256-256-kbit-32-k-8-serial-spi-f-ram?source=search&keywords=FM25W256&cat=technical_documents 00023 * 00024 */ 00025 00026 #include "mbed.h" 00027 #include "FM25W256.h" 00028 00029 FM25W256::FM25W256(PinName mosi, PinName miso, PinName clk, PinName cs) 00030 : _spi(mosi, miso, clk), _cs(cs) 00031 { 00032 _spi.format(8, 0); 00033 _spi.frequency(FM25W256_CLK); 00034 _cs = 1; 00035 wait_ms(1); // tPU = 1ms (min.) 00036 } 00037 00038 FM25W256::FM25W256(SPI &spi, PinName cs) 00039 : _spi(spi), _cs(cs) 00040 { 00041 _spi.format(8, 0); 00042 _spi.frequency(FM25W256_CLK); 00043 _cs = 1; 00044 wait_ms(1); // tPU = 1ms (min.) 00045 } 00046 00047 void FM25W256::write(uint16_t address, uint8_t data) 00048 { 00049 _cs = 0; 00050 _spi.write(CMD_WREN); 00051 _cs = 1; 00052 00053 _cs = 0; 00054 _spi.write(CMD_WRITE); 00055 _spi.write(address >> 8); 00056 _spi.write(address & 0xFF); 00057 _spi.write(data); 00058 _cs = 1; 00059 } 00060 00061 void FM25W256::write(uint16_t address, uint8_t *data, uint16_t size) 00062 { 00063 _cs = 0; 00064 _spi.write(CMD_WREN); 00065 _cs = 1; 00066 00067 _cs = 0; 00068 _spi.write(CMD_WRITE); 00069 _spi.write(address >> 8); 00070 _spi.write(address & 0xFF); 00071 while(size--) { 00072 _spi.write(*data++); 00073 } 00074 _cs = 1; 00075 } 00076 00077 uint8_t FM25W256::read(uint16_t address) 00078 { 00079 uint8_t data; 00080 00081 _cs = 0; 00082 _spi.write(CMD_READ); 00083 _spi.write(address >> 8); 00084 _spi.write(address & 0xFF); 00085 data = _spi.write(0); 00086 _cs = 1; 00087 00088 return data; 00089 } 00090 00091 void FM25W256::read(uint16_t address, uint8_t *buf, uint16_t size) 00092 { 00093 _cs = 0; 00094 _spi.write(CMD_READ); 00095 _spi.write(address >> 8); 00096 _spi.write(address & 0xFF); 00097 while (size--) { 00098 *buf++ = _spi.write(0); 00099 } 00100 _cs = 1; 00101 } 00102 00103 void FM25W256::wirte_status(uint8_t data) 00104 { 00105 _cs = 0; 00106 _spi.write(CMD_WREN); 00107 _cs = 1; 00108 00109 _cs = 0; 00110 _spi.write(CMD_WRSR); 00111 _spi.write(data); 00112 _cs = 1; 00113 } 00114 00115 uint8_t FM25W256::read_status() 00116 { 00117 uint8_t data; 00118 _cs = 0; 00119 _spi.write(CMD_RDSR); 00120 data = _spi.write(0); 00121 _cs = 1; 00122 00123 return data; 00124 } 00125 00126 void FM25W256::set_write_protect(E_WP bp) 00127 { 00128 // Set WPEN, BP0 and BP1 00129 wirte_status((1 << 7) | (bp << 2)); 00130 } 00131 00132 void FM25W256::clear_write_protect() 00133 { 00134 wirte_status(0); 00135 } 00136
Generated on Wed Jul 13 2022 07:21:54 by
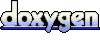