
Just4Trionic - CAN and BDM FLASH programmer for Saab cars
Embed:
(wiki syntax)
Show/hide line numbers
srecutils.cpp
00001 /******************************************************************************* 00002 00003 srecutils.h 00004 (c) 2010 by Sophie Dexter 00005 00006 Functions for manipulating Motorala S-records 00007 00008 ******************************************************************************** 00009 00010 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00011 This software is provided 'free' and in good faith, but the author does not 00012 accept liability for any damage arising from its use. 00013 00014 *******************************************************************************/ 00015 00016 #include "srecutils.h" 00017 00018 // SRecGetByte 00019 // 00020 // Returns an int which is a single byte made up from two ascii characters read from an S-record file 00021 // 00022 // inputs: a file pointer for the S-record file 00023 // return: an integer which is the byte in hex format 00024 00025 int SRecGetByte(FILE *fp) { 00026 int c = 0; 00027 int retbyte = 0; 00028 00029 for(int i=0; i<2; i++) { 00030 if ((c = fgetc(fp)) == EOF) return -1; 00031 c -= (c > '9') ? ('A' - 10) : '0'; 00032 retbyte = (retbyte << 4) + c; 00033 } 00034 return retbyte; 00035 } 00036 00037 // SRecGetAddress 00038 // 00039 // Returns an int which is the address part of the S-record line 00040 // The S-record type 1/2/3 or 9/8/7 determines if there are 2, 3 or 4 bytes in the address 00041 // 00042 // inputs: an integer which is the number of bytes that make up the address; 2, 3 or 4 bytes 00043 // a file pointer for the S-record file 00044 // return: an integer which is the load address for the S-record 00045 00046 int SRecGetAddress(int size, FILE *fp) { 00047 int address = 0; 00048 for (int i = 0; i<size; i++) 00049 { 00050 address <<= 8; 00051 address |= SRecGetByte (fp); 00052 } 00053 return address; 00054 }
Generated on Fri Jul 15 2022 00:43:05 by
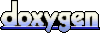