
Just4Trionic - CAN and BDM FLASH programmer for Saab cars
Embed:
(wiki syntax)
Show/hide line numbers
bdmtrionic.cpp
00001 /******************************************************************************* 00002 00003 bdmtrionic.cpp 00004 (c) 2010 by Sophie Dexter 00005 00006 General purpose BDM functions for Just4Trionic by Just4pLeisure 00007 00008 A derivative work based on: 00009 //----------------------------------------------------------------------------- 00010 // CAN/BDM adapter firmware 00011 // (C) Janis Silins, 2010 00012 // $id$ 00013 //----------------------------------------------------------------------------- 00014 00015 ******************************************************************************** 00016 00017 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00018 This software is provided 'free' and in good faith, but the author does not 00019 accept liability for any damage arising from its use. 00020 00021 *******************************************************************************/ 00022 00023 #include "bdmtrionic.h" 00024 00025 // structure for command address/value pairs 00026 struct mempair_t { 00027 uint32_t addr; ///< target address 00028 uint16_t val; ///< word value 00029 }; 00030 00031 // word write algorithm (29Fxxx) 00032 static const struct mempair_t am29_write [] = { 00033 {0xaaaa, 0xaaaa}, {0x5554, 0x5555}, {0xaaaa, 0xa0a0}, 00034 }; 00035 00036 // chip erase algorithms 00037 static const struct mempair_t am29_erase [] = { 00038 {0xaaaa, 0xaaaa}, {0x5554, 0x5555}, {0xaaaa, 0x8080}, 00039 {0xaaaa, 0xaaaa}, {0x5554, 0x5555}, {0xaaaa, 0x1010} 00040 }; 00041 00042 // reset algorithms 00043 //static const struct mempair_t am29_reset = {0xfffe, 0xf0f0}; 00044 static const struct mempair_t am29_reset [] = { 00045 {0xaaaa, 0xaaaa}, {0x5554, 0x5555}, {0xaaaa, 0xf0f0}, 00046 }; 00047 00048 // chip id algorithms 00049 static const struct mempair_t am29_id [] = { 00050 {0xaaaa, 0xaaaa}, {0x5554, 0x5555}, {0xaaaa, 0x9090}, 00051 }; 00052 00053 // ;-) 00054 static const struct mempair_t flash_tag [] = { 00055 {0x7fe00, 0xFF4A}, {0x7fe02, 0x7573}, {0x7fe04, 0x7434}, {0x7fe06, 0x704C}, 00056 {0x7fe08, 0x6569}, {0x7fe0a, 0x7375}, {0x7fe0c, 0x7265}, {0x7fe0e, 0x3B29}, 00057 }; 00058 00059 // local functions 00060 bool reset_am29(void); 00061 bool erase_am29(); 00062 bool flash_am29(const uint32_t* addr, uint16_t value); 00063 bool reset_am28(void); 00064 bool erase_am28(const uint32_t* start_addr, const uint32_t* end_addr); 00065 bool flash_am28(const uint32_t* addr, uint16_t value); 00066 bool get_flash_id(uint8_t* make, uint8_t* type); 00067 00068 bool run_bdm_driver(uint32_t addr, uint32_t maxtime); 00069 00070 //----------------------------------------------------------------------------- 00071 /** 00072 Dumps contents of a memory block from [start_addr] up to, but not including, 00073 the [end_addr] as long words (word-aligned addresses). MCU must be in 00074 background mode. The operation interrupts if the break character is 00075 received. 00076 00077 @param start_addr block start address 00078 @param end_addr block end address 00079 00080 @return status flag 00081 */ 00082 00083 00084 uint8_t dump_flash(const uint32_t* start_addr, const uint32_t* end_addr) 00085 { 00086 00087 // check parametres 00088 if (*start_addr > *end_addr) { 00089 return TERM_ERR; 00090 } 00091 00092 // dump memory contents 00093 uint32_t curr_addr = *start_addr; 00094 uint32_t value; 00095 00096 while ((curr_addr < *end_addr) && (pc.getc() != TERM_BREAK)) { 00097 // read long word 00098 if (curr_addr > *start_addr) { 00099 if (memdump_long(&value) != TERM_OK) { 00100 return TERM_ERR; 00101 } 00102 } else { 00103 if (memread_long(&value, &curr_addr) != TERM_OK) { 00104 return TERM_ERR; 00105 } 00106 } 00107 00108 // send memory value to host 00109 printf("%08X", value); 00110 printf("\r\n"); 00111 00112 // add the terminating character 00113 if (curr_addr < *end_addr - 4) { 00114 pc.putc(TERM_OK); 00115 // light up the activity LED 00116 ACTIVITYLEDON; 00117 } 00118 00119 curr_addr += 4; 00120 } 00121 00122 return TERM_OK; 00123 } 00124 00125 //----------------------------------------------------------------------------- 00126 /** 00127 Dumps the contents of a T5 ECU to a BIN file on the mbed 'disk' 00128 from [start_addr] up to, but not including, the [end_addr]. 00129 MCU must be in background mode. 00130 00131 @param start_addr block start address 00132 @param end_addr block end address 00133 00134 @return status flag 00135 */ 00136 00137 uint8_t dump_trionic() 00138 { 00139 00140 // Configure the MC68332 register values to prepare for flashing 00141 printf("I am trying to discover what type of Trionic ECU I am connected to...\r\n"); 00142 prep_t5_do(); 00143 // Work out what type of FLASH chips we want to make a dump file for 00144 uint8_t make; 00145 uint8_t type; 00146 get_flash_id(&make, &type); 00147 // set up chip-specific functions 00148 bool (*reset_func)(); 00149 uint32_t flash_size; 00150 00151 switch (type) { 00152 case AMD29BL802C: 00153 printf("I have found AMD29BL802C type FLASH chips; I must be connected to a T8 ECU :-)\r\n"); 00154 reset_func = &reset_am29; 00155 flash_size = T8FLASHSIZE; 00156 break; 00157 case AMD29F400B: 00158 case AMD29F400T: 00159 printf("I have found AMD29F400 type FLASH chips; I must be connected to a T7 ECU :-)\r\n"); 00160 reset_func = &reset_am29; 00161 flash_size = T7FLASHSIZE; 00162 break; 00163 case AMD29F010: 00164 case SST39SF010: 00165 case AMICA29010L: 00166 printf("I have found 29/39F010 type FLASH chips; I must be connected to a repaired T5.5 ECU :-)\r\n"); 00167 reset_func = &reset_am29; 00168 flash_size = T55FLASHSIZE; 00169 break; 00170 case ATMEL29C010: 00171 printf("I have found Atmel 29C010 type FLASH chips; I must be connected to a repaired T5.5 ECU :-)\r\n"); 00172 reset_func = &reset_am29; 00173 flash_size = T55FLASHSIZE; 00174 break; 00175 case AMD28F010: 00176 case INTEL28F010: 00177 printf("I have found 28F010 type FLASH chips; I must be connected to a T5.5 ECU :-)\r\n"); 00178 reset_func = &reset_am28; 00179 flash_size = T55FLASHSIZE; 00180 break; 00181 case AMD28F512: 00182 case INTEL28F512: 00183 printf("I have found 28F512 type FLASH chips; I must be connected to a T5.2 ECU :-)\r\n"); 00184 reset_func = &reset_am28; 00185 flash_size = T52FLASHSIZE; 00186 break; 00187 case ATMEL29C512: 00188 printf("I have found Atmel 29C512 type FLASH chips; I must be connected to a repaired T5.2 ECU :-)\r\n"); 00189 reset_func = &reset_am28; 00190 flash_size = T52FLASHSIZE; 00191 break; 00192 default: 00193 // unknown flash type 00194 printf("I could not work out what FLASH chips or TRIONIC ECU I am connected to :-(\r\n"); 00195 return TERM_ERR; 00196 } 00197 00198 // reset the FLASH chips 00199 if (!reset_func()) return TERM_ERR; 00200 00201 printf("Creating FLASH dump file...\r\n"); 00202 FILE *fp = fopen("/local/original.bin", "w"); // Open "original.bin" on the local file system for writing 00203 if (!fp) { 00204 perror ("The following error occured"); 00205 return TERM_ERR; 00206 } 00207 00208 // dump memory contents 00209 uint32_t addr = 0x00; 00210 uint32_t long_value; 00211 00212 // setup start address to dump from 00213 if (memread_long_cmd(&addr) != TERM_OK) return TERM_ERR; 00214 00215 timer.reset(); 00216 timer.start(); 00217 printf(" 0.00 %% complete.\r"); 00218 while (addr < flash_size) { 00219 uint16_t byte_count = 0; 00220 while (byte_count < FILE_BUF_LENGTH) { 00221 // get long word 00222 if (memget_long(&long_value) != TERM_OK) return TERM_ERR; 00223 // send memory value to file_buffer before saving to mbed 'disk' 00224 file_buffer[byte_count++] = ((uint8_t)(long_value >> 24)); 00225 file_buffer[byte_count++] = ((uint8_t)(long_value >> 16)); 00226 file_buffer[byte_count++] = ((uint8_t)(long_value >> 8)); 00227 file_buffer[byte_count++] = ((uint8_t)long_value); 00228 } 00229 fwrite(file_buffer, 1, FILE_BUF_LENGTH, fp); 00230 if (ferror (fp)) { 00231 fclose (fp); 00232 printf ("Error writing to the FLASH BIN file.\r\n"); 00233 return TERM_ERR; 00234 } 00235 printf("%6.2f\r", 100*(float)addr/(float)flash_size ); 00236 // make the activity led twinkle 00237 ACTIVITYLEDON; 00238 addr += FILE_BUF_LENGTH; 00239 } 00240 printf("100.00\r\n"); 00241 // should 'clear' the BDM connection here but bdm_clear won't compile from here 00242 // instead do a memread (or anything really) but ignore the result because it's not needed for anything 00243 memread_long(&long_value, &addr); 00244 timer.stop(); 00245 printf("Getting the FLASH dump took %#.1f seconds.\r\n",timer.read()); 00246 fclose(fp); 00247 return TERM_OK; 00248 } 00249 00250 //----------------------------------------------------------------------------- 00251 /** 00252 Erases the flash memory chip starting from [start_addr] up to, but not 00253 including [end_addr] and optionally verifies the result; MCU must be in 00254 background mode. 00255 00256 @param flash_type type of flash chip 00257 @param start_addr flash start address 00258 @param end_addr flash end address 00259 00260 @return status flag 00261 */ 00262 uint8_t erase_flash(const char* flash_type, const uint32_t* start_addr, 00263 const uint32_t* end_addr) 00264 { 00265 // AM29Fxxx chips (retrofitted to Trionic 5.x; original to T7) 00266 if (strncmp(flash_type, "29f010", 6) == 0 || 00267 strncmp(flash_type, "29f400", 6) == 0) { 00268 return erase_am29() ? TERM_OK : TERM_ERR; 00269 } 00270 00271 // AM28F010 chip (Trionic 5.x original) 00272 if (strncmp(flash_type, "28f010", 6) == 0) { 00273 return erase_am28(start_addr, end_addr) ? TERM_OK : TERM_ERR; 00274 } 00275 00276 return TERM_ERR; 00277 } 00278 00279 //----------------------------------------------------------------------------- 00280 /** 00281 Writes a batch of long words to the flash starting from [start_addr]. The 00282 operation interrupts if a break character is received. MCU must be in 00283 background mode. 00284 00285 @param flash_type type of flash chip 00286 @param start_addr block start address 00287 00288 @return status flag 00289 */ 00290 uint8_t write_flash(const char* flash_type, const uint32_t* start_addr) 00291 { 00292 // set up chip-specific functions 00293 bool (*reset_func)(void); 00294 bool (*flash_func)(const uint32_t*, uint16_t); 00295 00296 // AM29Fxxx chips (retrofitted to Trionic 5.x, original to T7) 00297 if (strncmp(flash_type, "29f010", 6) == 0 || 00298 strncmp(flash_type, "29f400", 6) == 0) { 00299 reset_func = &reset_am29; 00300 flash_func = &flash_am29; 00301 } else if (strncmp(flash_type, "28f010", 6) == 0) { 00302 // AM28F010 chip (Trionic 5.x original) 00303 reset_func = &reset_am28; 00304 flash_func = &flash_am28; 00305 } else { 00306 // unknown flash type 00307 return TERM_ERR; 00308 } 00309 00310 // reset the flash 00311 if (!reset_func()) { 00312 return TERM_ERR; 00313 } 00314 00315 uint32_t curr_addr = *start_addr; 00316 if (strncmp(flash_type, "29f010", 6) == 0) { 00317 curr_addr = 0; 00318 } 00319 00320 int rx_char = 0; 00321 char rx_buf[8]; 00322 char* rx_ptr; 00323 uint32_t long_value; 00324 bool ret = true; 00325 00326 // ready to receive data 00327 pc.putc(TERM_OK); 00328 00329 while (true) { 00330 // receive long words from USB 00331 printf("receive long words from USB\r\n"); 00332 rx_ptr = rx_buf; 00333 do { 00334 rx_char = pc.getc(); 00335 if (rx_char != EOF) { 00336 // have got all characters for one long word 00337 if (rx_ptr > &rx_buf[7]) { 00338 ret = (rx_char == TERM_OK); 00339 break; 00340 } 00341 00342 // save the character 00343 *rx_ptr++ = (char)rx_char; 00344 } 00345 } while (rx_char != TERM_OK && rx_char != TERM_BREAK); 00346 // end writing 00347 printf("end writing\r\n"); 00348 if (!ret || rx_char == TERM_BREAK) { 00349 break; 00350 } 00351 00352 // convert value to long word 00353 printf("convert value to long word\r\n"); 00354 if (!ascii2int(&long_value, rx_buf, 8)) { 00355 ret = false; 00356 break; 00357 } 00358 printf("long value %08x \r\n", long_value); 00359 00360 // write the first word 00361 printf("write the first word\r\n"); 00362 if (!flash_func(&curr_addr, (uint16_t)(long_value >> 16))) { 00363 ret = false; 00364 break; 00365 } 00366 curr_addr += 2; 00367 // write the second word 00368 printf("write the second word\r\n"); 00369 if (!flash_func(&curr_addr, (uint16_t)long_value)) { 00370 ret = false; 00371 break; 00372 } 00373 curr_addr += 2; 00374 00375 // light up the activity LED 00376 ACTIVITYLEDON; 00377 } 00378 00379 // reset flash 00380 return (reset_func() && ret) ? TERM_OK : TERM_ERR; 00381 } 00382 00383 //----------------------------------------------------------------------------- 00384 /** 00385 Writes a BIN file to the flash starting from [start_addr]. 00386 The operation ends when no more bytes can be read from the BIN file. 00387 MCU must be in background mode. 00388 00389 @param flash_type type of flash chip 00390 @param start_addr block start address 00391 00392 @return status flag 00393 */ 00394 uint8_t flash_trionic() 00395 { 00396 // Configure the MC68332 register values to prepare for flashing 00397 printf("I am trying to discover what type of Trionic ECU I am connected to...\r\n"); 00398 prep_t5_do(); 00399 // Work out what type of FLASH chips we want to program 00400 uint8_t make; 00401 uint8_t type; 00402 get_flash_id(&make, &type); 00403 // set up chip-specific functions 00404 bool (*reset_func)(); 00405 bool (*flash_func)(const uint32_t*, uint16_t); 00406 uint32_t flash_size; 00407 00408 switch (type) { 00409 case AMD29BL802C: 00410 printf("I have found AMD29BL802C type FLASH chips; I must be connected to a T8 ECU :-)\r\n"); 00411 reset_func = &reset_am29; 00412 flash_func = &flash_am29; 00413 flash_size = T8FLASHSIZE; 00414 break; 00415 case AMD29F400B: 00416 case AMD29F400T: 00417 printf("I have found AMD29F400 type FLASH chips; I must be connected to a T7 ECU :-)\r\n"); 00418 reset_func = &reset_am29; 00419 flash_func = &flash_am29; 00420 flash_size = T7FLASHSIZE; 00421 break; 00422 case AMD29F010: 00423 case SST39SF010: 00424 case AMICA29010L: 00425 printf("I have found 29/39F010 type FLASH chips; I must be connected to a repaired T5.5 ECU :-)\r\n"); 00426 reset_func = &reset_am29; 00427 flash_func = &flash_am29; 00428 flash_size = T55FLASHSIZE; 00429 break; 00430 case ATMEL29C010: 00431 printf("I have found Atmel 29C010 type FLASH chips; I must be connected to a repaired T5.5 ECU :-)\r\n"); 00432 reset_func = &reset_am29; 00433 flash_func = NULL; 00434 flash_size = T55FLASHSIZE; 00435 break; 00436 case AMD28F010: 00437 case INTEL28F010: 00438 printf("I have found 28F010 type FLASH chips; I must be connected to a T5.5 ECU :-)\r\n"); 00439 reset_func = &reset_am28; 00440 flash_func = &flash_am28; 00441 flash_size = T55FLASHSIZE; 00442 break; 00443 case AMD28F512: 00444 case INTEL28F512: 00445 printf("I have found 28F512 type FLASH chips; I must be connected to a T5.2 ECU :-)\r\n"); 00446 reset_func = &reset_am28; 00447 flash_func = &flash_am28; 00448 flash_size = T52FLASHSIZE; 00449 break; 00450 case ATMEL29C512: 00451 printf("I have found Atmel 29C512 type FLASH chips; I must be connected to a repaired T5.2 ECU :-)\r\n"); 00452 reset_func = &reset_am29; 00453 flash_func = NULL; 00454 flash_size = T52FLASHSIZE; 00455 break; 00456 default: 00457 // unknown flash type 00458 printf("I could not work out what FLASH chips or TRIONIC ECU I am connected to :-(\r\n"); 00459 return TERM_ERR; 00460 } 00461 // reset the FLASH chips 00462 if (!reset_func()) return TERM_ERR; 00463 00464 00465 printf("Checking the FLASH BIN file...\r\n"); 00466 FILE *fp = fopen("/local/modified.bin", "r"); // Open "modified.bin" on the local file system for reading 00467 // FILE *fp = fopen("/local/original.bin", "r"); // Open "original.bin" on the local file system for reading 00468 if (!fp) { 00469 printf("Error: I could not find the BIN file MODIFIED.BIN\r\n");; 00470 return TERM_ERR; 00471 } 00472 // obtain file size - it should match the size of the FLASH chips: 00473 fseek (fp , 0 , SEEK_END); 00474 uint32_t file_size = ftell (fp); 00475 rewind (fp); 00476 00477 // read the initial stack pointer value in the BIN file - it should match the value expected for the type of ECU 00478 uint8_t stack_bytes[4] = {0, 0, 0, 0}; 00479 uint32_t stack_long = 0; 00480 if (!fread(&stack_bytes[0],1,4,fp)) return TERM_ERR; 00481 rewind (fp); 00482 for(uint32_t i=0; i<4; i++) { 00483 (stack_long <<= 8) |= stack_bytes[i]; 00484 } 00485 00486 if (flash_size == T52FLASHSIZE && (file_size != T52FLASHSIZE || stack_long != T5POINTER)) { 00487 fclose(fp); 00488 printf("The BIN file does not appear to be for a T5.2 ECU :-(\r\n"); 00489 printf("BIN file size: %#10x, FLASH chip size: %#010x, Pointer: %#10x.\r\n", file_size, flash_size, stack_long); 00490 return TERM_ERR; 00491 } 00492 if (flash_size == T55FLASHSIZE && (file_size != T55FLASHSIZE || stack_long != T5POINTER)) { 00493 fclose(fp); 00494 printf("The BIN file does not appear to be for a T5.5 ECU :-(\r\n"); 00495 printf("BIN file size: %#10x, FLASH chip size: %#010x, Pointer: %#10x.\r\n", file_size, flash_size, stack_long); 00496 return TERM_ERR; 00497 } 00498 if (flash_size == T7FLASHSIZE && (file_size != T7FLASHSIZE || stack_long != T7POINTER)) { 00499 fclose(fp); 00500 printf("The BIN file does not appear to be for a T7 ECU :-(\r\n"); 00501 printf("BIN file size: %#10x, FLASH chip size: %#010x, Pointer: %#10x.\r\n", file_size, flash_size, stack_long); 00502 return TERM_ERR; 00503 } 00504 if (flash_size == T8FLASHSIZE && (file_size != T8FLASHSIZE || stack_long != T8POINTER)) { 00505 fclose(fp); 00506 printf("The BIN file does not appear to be for a T8 ECU :-(\r\n"); 00507 printf("BIN file size: %#10x, FLASH chip size: %#010x, Pointer: %#10x.\r\n", file_size, flash_size, stack_long); 00508 return TERM_ERR; 00509 } 00510 00511 uint32_t curr_addr = 0; 00512 00513 switch (type) { 00514 case AMD29BL802C: 00515 case AMD29F400T: 00516 case AMD29F010: 00517 case SST39SF010: 00518 case AMICA29010L: 00519 case ATMEL29C010: 00520 case AMD28F010: 00521 case INTEL28F010: 00522 case AMD28F512: 00523 case INTEL28F512: 00524 case ATMEL29C512: { 00525 // BDM FLASH Driver 00526 /* 00527 uint8_t flashDriver5[] = {\ 00528 0x60,0x00,0x04,0x08,\ 00529 0x7C,0x2F,0x2D,0x5C,0x2A,0x0D,0x00,0x00,\ 00530 0x02,0x03,0x00,0x03,0x41,0xFA,0xFF,0xF6,\ 00531 0x10,0xBB,0x30,0xEE,0x70,0x01,0x52,0x43,\ 00532 0x4E,0x75,\ 00533 0x20,0x7C,0x00,0xFF,0xFA,0x00,0x08,0x10,\ 00534 0x00,0x04,0x66,0x4E,0xD0,0xFC,0x00,0x04,\ 00535 0x10,0xFC,0x00,0x7F,0x08,0x10,0x00,0x03,\ 00536 0x67,0xFA,0xD0,0xFC,0x00,0x1C,0x42,0x10,\ 00537 0xD0,0xFC,0x00,0x23,0x30,0xBC,0x3F,0xFF,\ 00538 0xD0,0xFC,0x00,0x04,0x70,0x07,0x30,0xC0,\ 00539 0x30,0xBC,0x68,0x70,0xD0,0xFC,0x00,0x06,\ 00540 0x30,0xC0,0x30,0xFC,0x30,0x30,0x30,0xC0,\ 00541 0x30,0xBC,0x50,0x30,0xD0,0xFC,0x01,0xBE,\ 00542 0x70,0x40,0x30,0xC0,0x30,0x80,0x30,0x3C,\ 00543 0x55,0xF0,0x4E,0x71,0x51,0xC8,0xFF,0xFC,\ 00544 0x60,0x18,0xD0,0xFC,0x00,0x08,0x30,0xFC,\ 00545 0x69,0x08,0x08,0x10,0x00,0x09,0x67,0xFA,\ 00546 0x31,0x3C,0x68,0x08,0xD0,0xFC,0x00,0x48,\ 00547 0x42,0x50,0x4E,0x75,\ 00548 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,\ 00549 0x00,0x00,0x2C,0x3C,0x00,0x00,0x55,0x55,\ 00550 0x2E,0x3C,0x00,0x00,0xAA,0xAA,0x2A,0x46,\ 00551 0x53,0x8D,0x2C,0x47,0x45,0xF8,0x00,0x00,\ 00552 0x47,0xFA,0xFF,0xDE,0x3C,0x87,0x3A,0x86,\ 00553 0x3C,0xBC,0x90,0x90,0x36,0xDA,0x36,0x92,\ 00554 0x3C,0x87,0x3A,0x86,0x3C,0xBC,0xF0,0xF0,\ 00555 0x20,0x3A,0xFF,0xC6,0x72,0x02,0x48,0x41,\ 00556 0x74,0x01,0x0C,0x00,0x00,0x25,0x67,0x50,\ 00557 0x0C,0x00,0x00,0xB8,0x67,0x4A,0x74,0x04,\ 00558 0x0C,0x00,0x00,0x5D,0x67,0x42,0x74,0x01,\ 00559 0xE3,0x99,0x0C,0x00,0x00,0xA7,0x67,0x38,\ 00560 0x0C,0x00,0x00,0xB4,0x67,0x32,0x74,0x02,\ 00561 0x0C,0x00,0x00,0x20,0x67,0x2A,0x0C,0x00,\ 00562 0x00,0xA4,0x67,0x24,0x0C,0x00,0x00,0xB5,\ 00563 0x67,0x1E,0x74,0x04,0x0C,0x00,0x00,0xD5,\ 00564 0x67,0x16,0x74,0x03,0xE3,0x99,0x0C,0x00,\ 00565 0x00,0x23,0x67,0x0C,0xE3,0x99,0x0C,0x00,\ 00566 0x00,0x81,0x67,0x04,0x72,0x00,0x74,0x00,\ 00567 0x47,0xFA,0xFF,0x6A,0x26,0x81,0x47,0xFA,\ 00568 0xFF,0x68,0x16,0x82,0x4E,0x75,\ 00569 0x45,0x72,0x61,0x73,0x69,0x6E,0x67,0x20,\ 00570 0x46,0x4C,0x41,0x53,0x48,0x20,0x63,0x68,\ 00571 0x69,0x70,0x73,0x0D,0x0A,0x00,0x41,0xFA,\ 00572 0xFF,0xE8,0x70,0x01,0x12,0x3A,0xFF,0x44,\ 00573 0x53,0x01,0x67,0x16,0x53,0x01,0x67,0x00,\ 00574 0x00,0xB8,0x53,0x01,0x67,0x00,0x01,0x0A,\ 00575 0x53,0x01,0x67,0x00,0x01,0x3A,0x60,0x00,\ 00576 0x01,0x3A,0x4B,0xF8,0x00,0x00,0x24,0x3A,\ 00577 0xFF,0x1E,0x26,0x02,0x3A,0xBC,0xFF,0xFF,\ 00578 0x3A,0xBC,0xFF,0xFF,0x42,0x55,0x4A,0x35,\ 00579 0x28,0xFF,0x67,0x28,0x7A,0x19,0x1B,0xBC,\ 00580 0x00,0x40,0x28,0xFF,0x42,0x35,0x28,0xFF,\ 00581 0x72,0x15,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00582 0x1B,0xBC,0x00,0xC0,0x28,0xFF,0x72,0x0C,\ 00583 0x4E,0x71,0x51,0xC9,0xFF,0xFC,0x4A,0x35,\ 00584 0x28,0xFF,0x66,0x06,0x53,0x82,0x66,0xCC,\ 00585 0x60,0x04,0x53,0x45,0x66,0xD0,0x42,0x55,\ 00586 0x4A,0x55,0x4A,0x05,0x67,0x00,0x00,0xE4,\ 00587 0x24,0x03,0x50,0xC4,0x2A,0x3C,0x03,0xE8,\ 00588 0x03,0xE8,0x72,0x20,0x1B,0x81,0x28,0xFF,\ 00589 0x1B,0x81,0x28,0xFF,0x32,0x3C,0x55,0xF0,\ 00590 0x4E,0x71,0x51,0xC9,0xFF,0xFC,0x4E,0xBA,\ 00591 0xFE,0x24,0x1B,0xBC,0x00,0xA0,0x28,0xFF,\ 00592 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00593 0xB8,0x35,0x28,0xFF,0x66,0x08,0x48,0x45,\ 00594 0x53,0x82,0x66,0xE6,0x60,0x04,0x53,0x45,\ 00595 0x66,0xC8,0x42,0x55,0x4A,0x55,0x4A,0x45,\ 00596 0x67,0x00,0x00,0x98,0x60,0x00,0x00,0x90,\ 00597 0x70,0x01,0x42,0x83,0x1D,0x87,0x08,0x00,\ 00598 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0x80,\ 00599 0x08,0x00,0x1D,0x87,0x08,0x00,0x1B,0x86,\ 00600 0x08,0x00,0x1D,0xBC,0x00,0x10,0x08,0x00,\ 00601 0x2A,0x00,0x4E,0xBA,0xFD,0xD0,0x20,0x05,\ 00602 0x1A,0x30,0x09,0x90,0x08,0x05,0x00,0x07,\ 00603 0x66,0x20,0x08,0x05,0x00,0x05,0x67,0xE8,\ 00604 0x1A,0x30,0x09,0x90,0x08,0x05,0x00,0x07,\ 00605 0x66,0x10,0x1D,0x87,0x08,0x00,0x1B,0x86,\ 00606 0x08,0x00,0x1D,0xBC,0x00,0xF0,0x08,0x00,\ 00607 0x60,0x40,0x53,0x80,0x67,0xAE,0x60,0x36,\ 00608 0x42,0x83,0x3C,0x87,0x3A,0x86,0x3C,0xBC,\ 00609 0x00,0x80,0x3C,0x87,0x3A,0x86,0x3C,0xBC,\ 00610 0x00,0x10,0x4E,0xBA,0xFD,0x88,0x3A,0x15,\ 00611 0x08,0x05,0x00,0x07,0x66,0x18,0x08,0x05,\ 00612 0x00,0x05,0x67,0xEE,0x3A,0x15,0x08,0x05,\ 00613 0x00,0x07,0x66,0x0A,0x3C,0x87,0x3A,0x86,\ 00614 0x3C,0xBC,0x00,0xF0,0x60,0x04,0x42,0x80,\ 00615 0x60,0x02,0x70,0x01,0x4E,0x75,\ 00616 0x47,0xFB,0x01,0x70,0x00,0x00,0x04,0x50,\ 00617 0x28,0x49,0x24,0x3C,0x00,0x00,0x01,0x00,\ 00618 0x12,0x3A,0xFD,0xDA,0x53,0x01,0x67,0x14,\ 00619 0x53,0x01,0x67,0x5A,0x53,0x01,0x67,0x00,\ 00620 0x00,0xBC,0x53,0x01,0x67,0x00,0x01,0x00,\ 00621 0x60,0x00,0x01,0x2E,0x10,0x33,0x28,0xFF,\ 00622 0x0C,0x00,0x00,0xFF,0x67,0x28,0x7A,0x19,\ 00623 0x19,0xBC,0x00,0x40,0x28,0xFF,0x19,0x80,\ 00624 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 00625 0xFF,0xFC,0x19,0xBC,0x00,0xC0,0x28,0xFF,\ 00626 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00627 0xB0,0x34,0x28,0xFF,0x66,0x06,0x53,0x82,\ 00628 0x66,0xCA,0x60,0x04,0x53,0x05,0x66,0xD0,\ 00629 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 00630 0x00,0xE8,0x60,0x00,0x00,0xE0,0x20,0x0C,\ 00631 0xD0,0x82,0xC0,0xBC,0x00,0x00,0x00,0x01,\ 00632 0x08,0x40,0x00,0x00,0x16,0x33,0x28,0xFF,\ 00633 0x0C,0x03,0x00,0xFF,0x67,0x48,0x1D,0x87,\ 00634 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 00635 0x00,0xA0,0x08,0x00,0x19,0x83,0x28,0xFF,\ 00636 0xC6,0x3C,0x00,0x80,0x18,0x34,0x28,0xFF,\ 00637 0x1A,0x04,0xC8,0x3C,0x00,0x80,0xB8,0x03,\ 00638 0x67,0x24,0x08,0x05,0x00,0x05,0x67,0xEC,\ 00639 0x18,0x34,0x28,0xFF,0xC8,0x3C,0x00,0x80,\ 00640 0xB8,0x03,0x67,0x12,0x1D,0x87,0x08,0x00,\ 00641 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 00642 0x08,0x00,0x60,0x00,0x00,0x84,0x53,0x82,\ 00643 0x66,0xA6,0x60,0x78,0x36,0x33,0x28,0xFE,\ 00644 0x0C,0x43,0xFF,0xFF,0x67,0x3A,0x3C,0x87,\ 00645 0x3A,0x86,0x3C,0xBC,0x00,0xA0,0x39,0x83,\ 00646 0x28,0xFE,0xC6,0x7C,0x00,0x80,0x38,0x34,\ 00647 0x28,0xFE,0x3A,0x04,0xC8,0x7C,0x00,0x80,\ 00648 0xB8,0x43,0x67,0x1C,0x08,0x05,0x00,0x05,\ 00649 0x67,0xEC,0x38,0x34,0x28,0xFE,0xC8,0x7C,\ 00650 0x00,0x80,0xB8,0x43,0x67,0x0A,0x3C,0x87,\ 00651 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x38,\ 00652 0x55,0x82,0x66,0xB8,0x60,0x2E,0x3C,0x87,\ 00653 0x3A,0x86,0x3C,0xBC,0xA0,0xA0,0x39,0xB3,\ 00654 0x28,0xFE,0x28,0xFE,0x55,0x82,0x66,0xF6,\ 00655 0x32,0x3C,0x55,0xF0,0x4E,0x71,0x51,0xC9,\ 00656 0xFF,0xFC,0x34,0x3C,0x01,0x00,0x36,0x33,\ 00657 0x28,0xFE,0xB6,0x74,0x28,0xFE,0x66,0x08,\ 00658 0x55,0x82,0x66,0xF2,0x42,0x80,0x60,0x02,\ 00659 0x70,0x01,0x4E,0x75,\ 00660 0x4F,0xFB,0x01,0x70,0x00,0x00,0x02,0xF4,\ 00661 0x4E,0xBA,0xFC,0x0A,0x4E,0xBA,0xFC,0x84,\ 00662 0x4E,0xBA,0xFD,0x32,0x4A,0xFA,0x42,0x80,\ 00663 0x22,0x40,0x4E,0xBA,0xFE,0x88,0x4A,0xFA,\ 00664 0xD2,0xFC,0x01,0x00,0x60,0xF4 00665 }; 00666 00667 uint8_t flashDriver6[] = {\ 00668 0x60,0x00,0x04,0x0A,\ 00669 0x7C,0x2F,0x2D,0x5C,0x2A,0x0D,0x00,0x00,\ 00670 0x02,0x03,0x00,0x03,0x41,0xFA,0xFF,0xF6,\ 00671 0x10,0xBB,0x30,0xEE,0x70,0x01,0x4A,0xFA,\ 00672 0x52,0x43,0x4E,0x75,\ 00673 0x20,0x7C,0x00,0xFF,0xFA,0x00,0x08,0x10,\ 00674 0x00,0x04,0x66,0x4E,0xD0,0xFC,0x00,0x04,\ 00675 0x10,0xFC,0x00,0x7F,0x08,0x10,0x00,0x03,\ 00676 0x67,0xFA,0xD0,0xFC,0x00,0x1C,0x42,0x10,\ 00677 0xD0,0xFC,0x00,0x23,0x30,0xBC,0x3F,0xFF,\ 00678 0xD0,0xFC,0x00,0x04,0x70,0x07,0x30,0xC0,\ 00679 0x30,0xBC,0x68,0x70,0xD0,0xFC,0x00,0x06,\ 00680 0x30,0xC0,0x30,0xFC,0x30,0x30,0x30,0xC0,\ 00681 0x30,0xBC,0x50,0x30,0xD0,0xFC,0x01,0xBE,\ 00682 0x70,0x40,0x30,0xC0,0x30,0x80,0x30,0x3C,\ 00683 0x55,0xF0,0x4E,0x71,0x51,0xC8,0xFF,0xFC,\ 00684 0x60,0x18,0xD0,0xFC,0x00,0x08,0x30,0xFC,\ 00685 0x69,0x08,0x08,0x10,0x00,0x09,0x67,0xFA,\ 00686 0x31,0x3C,0x68,0x08,0xD0,0xFC,0x00,0x48,\ 00687 0x42,0x50,0x4E,0x75,\ 00688 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,\ 00689 0x00,0x00,0x2C,0x3C,0x00,0x00,0x55,0x55,\ 00690 0x2E,0x3C,0x00,0x00,0xAA,0xAA,0x2A,0x46,\ 00691 0x53,0x8D,0x2C,0x47,0x45,0xF8,0x00,0x00,\ 00692 0x47,0xFA,0xFF,0xDE,0x3C,0x87,0x3A,0x86,\ 00693 0x3C,0xBC,0x90,0x90,0x36,0xDA,0x36,0x92,\ 00694 0x3C,0x87,0x3A,0x86,0x3C,0xBC,0xF0,0xF0,\ 00695 0x20,0x3A,0xFF,0xC6,0x72,0x02,0x48,0x41,\ 00696 0x74,0x01,0x0C,0x00,0x00,0x25,0x67,0x50,\ 00697 0x0C,0x00,0x00,0xB8,0x67,0x4A,0x74,0x04,\ 00698 0x0C,0x00,0x00,0x5D,0x67,0x42,0x74,0x01,\ 00699 0xE3,0x99,0x0C,0x00,0x00,0xA7,0x67,0x38,\ 00700 0x0C,0x00,0x00,0xB4,0x67,0x32,0x74,0x02,\ 00701 0x0C,0x00,0x00,0x20,0x67,0x2A,0x0C,0x00,\ 00702 0x00,0xA4,0x67,0x24,0x0C,0x00,0x00,0xB5,\ 00703 0x67,0x1E,0x74,0x04,0x0C,0x00,0x00,0xD5,\ 00704 0x67,0x16,0x74,0x03,0xE3,0x99,0x0C,0x00,\ 00705 0x00,0x23,0x67,0x0C,0xE3,0x99,0x0C,0x00,\ 00706 0x00,0x81,0x67,0x04,0x72,0x00,0x74,0x00,\ 00707 0x47,0xFA,0xFF,0x6A,0x26,0x81,0x47,0xFA,\ 00708 0xFF,0x68,0x16,0x82,0x4E,0x75,\ 00709 0x45,0x72,0x61,0x73,0x69,0x6E,0x67,0x20,\ 00710 0x46,0x4C,0x41,0x53,0x48,0x20,0x63,0x68,\ 00711 0x69,0x70,0x73,0x0D,0x0A,0x00,0x41,0xFA,\ 00712 0xFF,0xE8,0x70,0x01,0x12,0x3A,0xFF,0x44,\ 00713 0x53,0x01,0x67,0x16,0x53,0x01,0x67,0x00,\ 00714 0x00,0xB8,0x53,0x01,0x67,0x00,0x01,0x0A,\ 00715 0x53,0x01,0x67,0x00,0x01,0x3A,0x60,0x00,\ 00716 0x01,0x3A,0x4B,0xF8,0x00,0x00,0x24,0x3A,\ 00717 0xFF,0x1E,0x26,0x02,0x3A,0xBC,0xFF,0xFF,\ 00718 0x3A,0xBC,0xFF,0xFF,0x42,0x55,0x4A,0x35,\ 00719 0x28,0xFF,0x67,0x28,0x7A,0x19,0x1B,0xBC,\ 00720 0x00,0x40,0x28,0xFF,0x42,0x35,0x28,0xFF,\ 00721 0x72,0x15,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00722 0x1B,0xBC,0x00,0xC0,0x28,0xFF,0x72,0x0C,\ 00723 0x4E,0x71,0x51,0xC9,0xFF,0xFC,0x4A,0x35,\ 00724 0x28,0xFF,0x66,0x06,0x53,0x82,0x66,0xCC,\ 00725 0x60,0x04,0x53,0x45,0x66,0xD0,0x42,0x55,\ 00726 0x4A,0x55,0x4A,0x05,0x67,0x00,0x00,0xE4,\ 00727 0x24,0x03,0x50,0xC4,0x2A,0x3C,0x03,0xE8,\ 00728 0x03,0xE8,0x72,0x20,0x1B,0x81,0x28,0xFF,\ 00729 0x1B,0x81,0x28,0xFF,0x32,0x3C,0x55,0xF0,\ 00730 0x4E,0x71,0x51,0xC9,0xFF,0xFC,0x4E,0xBA,\ 00731 0xFE,0x22,0x1B,0xBC,0x00,0xA0,0x28,0xFF,\ 00732 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00733 0xB8,0x35,0x28,0xFF,0x66,0x08,0x48,0x45,\ 00734 0x53,0x82,0x66,0xE6,0x60,0x04,0x53,0x45,\ 00735 0x66,0xC8,0x42,0x55,0x4A,0x55,0x4A,0x45,\ 00736 0x67,0x00,0x00,0x98,0x60,0x00,0x00,0x90,\ 00737 0x70,0x01,0x42,0x83,0x1D,0x87,0x08,0x00,\ 00738 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0x80,\ 00739 0x08,0x00,0x1D,0x87,0x08,0x00,0x1B,0x86,\ 00740 0x08,0x00,0x1D,0xBC,0x00,0x10,0x08,0x00,\ 00741 0x2A,0x00,0x4E,0xBA,0xFD,0xCE,0x20,0x05,\ 00742 0x1A,0x30,0x09,0x90,0x08,0x05,0x00,0x07,\ 00743 0x66,0x20,0x08,0x05,0x00,0x05,0x67,0xE8,\ 00744 0x1A,0x30,0x09,0x90,0x08,0x05,0x00,0x07,\ 00745 0x66,0x10,0x1D,0x87,0x08,0x00,0x1B,0x86,\ 00746 0x08,0x00,0x1D,0xBC,0x00,0xF0,0x08,0x00,\ 00747 0x60,0x40,0x53,0x80,0x67,0xAE,0x60,0x36,\ 00748 0x42,0x83,0x3C,0x87,0x3A,0x86,0x3C,0xBC,\ 00749 0x00,0x80,0x3C,0x87,0x3A,0x86,0x3C,0xBC,\ 00750 0x00,0x10,0x4E,0xBA,0xFD,0x86,0x3A,0x15,\ 00751 0x08,0x05,0x00,0x07,0x66,0x18,0x08,0x05,\ 00752 0x00,0x05,0x67,0xEE,0x3A,0x15,0x08,0x05,\ 00753 0x00,0x07,0x66,0x0A,0x3C,0x87,0x3A,0x86,\ 00754 0x3C,0xBC,0x00,0xF0,0x60,0x04,0x42,0x80,\ 00755 0x60,0x02,0x70,0x01,0x4E,0x75,\ 00756 0x47,0xFB,0x01,0x70,0x00,0x00,0x04,0x4E,\ 00757 0x28,0x49,0x24,0x3C,0x00,0x00,0x01,0x00,\ 00758 0x12,0x3A,0xFD,0xDA,0x53,0x01,0x67,0x14,\ 00759 0x53,0x01,0x67,0x5A,0x53,0x01,0x67,0x00,\ 00760 0x00,0xBC,0x53,0x01,0x67,0x00,0x01,0x00,\ 00761 0x60,0x00,0x01,0x2E,0x10,0x33,0x28,0xFF,\ 00762 0x0C,0x00,0x00,0xFF,0x67,0x28,0x7A,0x19,\ 00763 0x19,0xBC,0x00,0x40,0x28,0xFF,0x19,0x80,\ 00764 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 00765 0xFF,0xFC,0x19,0xBC,0x00,0xC0,0x28,0xFF,\ 00766 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00767 0xB0,0x34,0x28,0xFF,0x66,0x06,0x53,0x82,\ 00768 0x66,0xCA,0x60,0x04,0x53,0x05,0x66,0xD0,\ 00769 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 00770 0x00,0xE8,0x60,0x00,0x00,0xE0,0x20,0x0C,\ 00771 0xD0,0x82,0xC0,0xBC,0x00,0x00,0x00,0x01,\ 00772 0x08,0x40,0x00,0x00,0x16,0x33,0x28,0xFF,\ 00773 0x0C,0x03,0x00,0xFF,0x67,0x48,0x1D,0x87,\ 00774 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 00775 0x00,0xA0,0x08,0x00,0x19,0x83,0x28,0xFF,\ 00776 0xC6,0x3C,0x00,0x80,0x18,0x34,0x28,0xFF,\ 00777 0x1A,0x04,0xC8,0x3C,0x00,0x80,0xB8,0x03,\ 00778 0x67,0x24,0x08,0x05,0x00,0x05,0x67,0xEC,\ 00779 0x18,0x34,0x28,0xFF,0xC8,0x3C,0x00,0x80,\ 00780 0xB8,0x03,0x67,0x12,0x1D,0x87,0x08,0x00,\ 00781 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 00782 0x08,0x00,0x60,0x00,0x00,0x84,0x53,0x82,\ 00783 0x66,0xA6,0x60,0x78,0x36,0x33,0x28,0xFE,\ 00784 0x0C,0x43,0xFF,0xFF,0x67,0x3A,0x3C,0x87,\ 00785 0x3A,0x86,0x3C,0xBC,0x00,0xA0,0x39,0x83,\ 00786 0x28,0xFE,0xC6,0x7C,0x00,0x80,0x38,0x34,\ 00787 0x28,0xFE,0x3A,0x04,0xC8,0x7C,0x00,0x80,\ 00788 0xB8,0x43,0x67,0x1C,0x08,0x05,0x00,0x05,\ 00789 0x67,0xEC,0x38,0x34,0x28,0xFE,0xC8,0x7C,\ 00790 0x00,0x80,0xB8,0x43,0x67,0x0A,0x3C,0x87,\ 00791 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x38,\ 00792 0x55,0x82,0x66,0xB8,0x60,0x2E,0x3C,0x87,\ 00793 0x3A,0x86,0x3C,0xBC,0xA0,0xA0,0x39,0xB3,\ 00794 0x28,0xFE,0x28,0xFE,0x55,0x82,0x66,0xF6,\ 00795 0x32,0x3C,0x55,0xF0,0x4E,0x71,0x51,0xC9,\ 00796 0xFF,0xFC,0x34,0x3C,0x01,0x00,0x36,0x33,\ 00797 0x28,0xFE,0xB6,0x74,0x28,0xFE,0x66,0x08,\ 00798 0x55,0x82,0x66,0xF2,0x42,0x80,0x60,0x02,\ 00799 0x70,0x01,0x4E,0x75,\ 00800 0x4F,0xFB,0x01,0x70,0x00,0x00,0x02,0xF2,\ 00801 0x4E,0xBA,0xFC,0x0A,0x4E,0xBA,0xFC,0x84,\ 00802 0x4E,0xBA,0xFD,0x32,0x4A,0xFA,0x42,0x80,\ 00803 0x22,0x40,0x4E,0xBA,0xFE,0x88,0x4A,0xFA,\ 00804 0xD2,0xFC,0x01,0x00,0x60,0xF4,0x00,0x00 00805 }; 00806 */ 00807 uint8_t flashDriver[] = {\ 00808 0x60,0x00,0x04,0x0C,\ 00809 0x7C,0x2F,0x2D,0x5C,0x2A,0x0D,0x00,0x00,\ 00810 0x02,0x03,0x00,0x03,0x41,0xFA,0xFF,0xF6,\ 00811 0x10,0xBB,0x30,0xEE,0x70,0x01,0x4A,0xFA,\ 00812 0x52,0x43,0x4E,0x75,\ 00813 0x20,0x7C,0x00,0xFF,0xFA,0x00,0x08,0x10,\ 00814 0x00,0x04,0x66,0x4E,0xD0,0xFC,0x00,0x04,\ 00815 0x10,0xFC,0x00,0x7F,0x08,0x10,0x00,0x03,\ 00816 0x67,0xFA,0xD0,0xFC,0x00,0x1C,0x42,0x10,\ 00817 0xD0,0xFC,0x00,0x23,0x30,0xBC,0x3F,0xFF,\ 00818 0xD0,0xFC,0x00,0x04,0x70,0x07,0x30,0xC0,\ 00819 0x30,0xBC,0x68,0x70,0xD0,0xFC,0x00,0x06,\ 00820 0x30,0xC0,0x30,0xFC,0x30,0x30,0x30,0xC0,\ 00821 0x30,0xBC,0x50,0x30,0xD0,0xFC,0x01,0xBE,\ 00822 0x70,0x40,0x30,0xC0,0x30,0x80,0x30,0x3C,\ 00823 0x55,0xF0,0x4E,0x71,0x51,0xC8,0xFF,0xFC,\ 00824 0x60,0x18,0xD0,0xFC,0x00,0x08,0x30,0xFC,\ 00825 0x69,0x08,0x08,0x10,0x00,0x09,0x67,0xFA,\ 00826 0x31,0x3C,0x68,0x08,0xD0,0xFC,0x00,0x48,\ 00827 0x42,0x50,0x4E,0x75,\ 00828 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,\ 00829 0x00,0x00,0x2C,0x3C,0x00,0x00,0x55,0x55,\ 00830 0x2E,0x3C,0x00,0x00,0xAA,0xAA,0x2A,0x46,\ 00831 0x53,0x8D,0x2C,0x47,0x45,0xF8,0x00,0x00,\ 00832 0x47,0xFA,0xFF,0xDE,0x3C,0x87,0x3A,0x86,\ 00833 0x3C,0xBC,0x90,0x90,0x36,0xDA,0x36,0x92,\ 00834 0x3C,0x87,0x3A,0x86,0x3C,0xBC,0xF0,0xF0,\ 00835 0x20,0x3A,0xFF,0xC6,0x72,0x02,0x48,0x41,\ 00836 0x74,0x01,0x0C,0x00,0x00,0x25,0x67,0x50,\ 00837 0x0C,0x00,0x00,0xB8,0x67,0x4A,0x74,0x04,\ 00838 0x0C,0x00,0x00,0x5D,0x67,0x42,0x74,0x01,\ 00839 0xE3,0x99,0x0C,0x00,0x00,0xA7,0x67,0x38,\ 00840 0x0C,0x00,0x00,0xB4,0x67,0x32,0x74,0x02,\ 00841 0x0C,0x00,0x00,0x20,0x67,0x2A,0x0C,0x00,\ 00842 0x00,0xA4,0x67,0x24,0x0C,0x00,0x00,0xB5,\ 00843 0x67,0x1E,0x74,0x04,0x0C,0x00,0x00,0xD5,\ 00844 0x67,0x16,0x74,0x03,0xE3,0x99,0x0C,0x00,\ 00845 0x00,0x23,0x67,0x0C,0xE3,0x99,0x0C,0x00,\ 00846 0x00,0x81,0x67,0x04,0x72,0x00,0x74,0x00,\ 00847 0x47,0xFA,0xFF,0x6A,0x26,0x81,0x47,0xFA,\ 00848 0xFF,0x68,0x16,0x82,0x4E,0x75,\ 00849 0x45,0x72,0x61,0x73,0x69,0x6E,0x67,0x20,\ 00850 0x46,0x4C,0x41,0x53,0x48,0x20,0x63,0x68,\ 00851 0x69,0x70,0x73,0x0D,0x0A,0x00,0x41,0xFA,\ 00852 0xFF,0xE8,0x70,0x01,0x4A,0xFA,0x12,0x3A,\ 00853 0xFF,0x42,0x53,0x01,0x67,0x16,0x53,0x01,\ 00854 0x67,0x00,0x00,0xB8,0x53,0x01,0x67,0x00,\ 00855 0x01,0x0A,0x53,0x01,0x67,0x00,0x01,0x3A,\ 00856 0x60,0x00,0x01,0x3A,0x4B,0xF8,0x00,0x00,\ 00857 0x24,0x3A,0xFF,0x1C,0x26,0x02,0x3A,0xBC,\ 00858 0xFF,0xFF,0x3A,0xBC,0xFF,0xFF,0x42,0x55,\ 00859 0x4A,0x35,0x28,0xFF,0x67,0x28,0x7A,0x19,\ 00860 0x1B,0xBC,0x00,0x40,0x28,0xFF,0x42,0x35,\ 00861 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 00862 0xFF,0xFC,0x1B,0xBC,0x00,0xC0,0x28,0xFF,\ 00863 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00864 0x4A,0x35,0x28,0xFF,0x66,0x06,0x53,0x82,\ 00865 0x66,0xCC,0x60,0x04,0x53,0x45,0x66,0xD0,\ 00866 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 00867 0x00,0xE4,0x24,0x03,0x50,0xC4,0x2A,0x3C,\ 00868 0x03,0xE8,0x03,0xE8,0x72,0x20,0x1B,0x81,\ 00869 0x28,0xFF,0x1B,0x81,0x28,0xFF,0x32,0x3C,\ 00870 0x55,0xF0,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00871 0x4E,0xBA,0xFE,0x20,0x1B,0xBC,0x00,0xA0,\ 00872 0x28,0xFF,0x72,0x0C,0x4E,0x71,0x51,0xC9,\ 00873 0xFF,0xFC,0xB8,0x35,0x28,0xFF,0x66,0x08,\ 00874 0x48,0x45,0x53,0x82,0x66,0xE6,0x60,0x04,\ 00875 0x53,0x45,0x66,0xC8,0x42,0x55,0x4A,0x55,\ 00876 0x4A,0x45,0x67,0x00,0x00,0x98,0x60,0x00,\ 00877 0x00,0x90,0x70,0x01,0x42,0x83,0x1D,0x87,\ 00878 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 00879 0x00,0x80,0x08,0x00,0x1D,0x87,0x08,0x00,\ 00880 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0x10,\ 00881 0x08,0x00,0x2A,0x00,0x4E,0xBA,0xFD,0xCC,\ 00882 0x20,0x05,0x1A,0x30,0x09,0x90,0x08,0x05,\ 00883 0x00,0x07,0x66,0x20,0x08,0x05,0x00,0x05,\ 00884 0x67,0xE8,0x1A,0x30,0x09,0x90,0x08,0x05,\ 00885 0x00,0x07,0x66,0x10,0x1D,0x87,0x08,0x00,\ 00886 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 00887 0x08,0x00,0x60,0x40,0x53,0x80,0x67,0xAE,\ 00888 0x60,0x36,0x42,0x83,0x3C,0x87,0x3A,0x86,\ 00889 0x3C,0xBC,0x00,0x80,0x3C,0x87,0x3A,0x86,\ 00890 0x3C,0xBC,0x00,0x10,0x4E,0xBA,0xFD,0x84,\ 00891 0x3A,0x15,0x08,0x05,0x00,0x07,0x66,0x18,\ 00892 0x08,0x05,0x00,0x05,0x67,0xEE,0x3A,0x15,\ 00893 0x08,0x05,0x00,0x07,0x66,0x0A,0x3C,0x87,\ 00894 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x04,\ 00895 0x42,0x80,0x60,0x02,0x70,0x01,0x4E,0x75,\ 00896 0x47,0xFB,0x01,0x70,0x00,0x00,0x04,0x4C,\ 00897 0x28,0x49,0x24,0x3C,0x00,0x00,0x01,0x00,\ 00898 0x12,0x3A,0xFD,0xD8,0x53,0x01,0x67,0x14,\ 00899 0x53,0x01,0x67,0x5A,0x53,0x01,0x67,0x00,\ 00900 0x00,0xBC,0x53,0x01,0x67,0x00,0x01,0x00,\ 00901 0x60,0x00,0x01,0x2E,0x10,0x33,0x28,0xFF,\ 00902 0x0C,0x00,0x00,0xFF,0x67,0x28,0x7A,0x19,\ 00903 0x19,0xBC,0x00,0x40,0x28,0xFF,0x19,0x80,\ 00904 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 00905 0xFF,0xFC,0x19,0xBC,0x00,0xC0,0x28,0xFF,\ 00906 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 00907 0xB0,0x34,0x28,0xFF,0x66,0x06,0x53,0x82,\ 00908 0x66,0xCA,0x60,0x04,0x53,0x05,0x66,0xD0,\ 00909 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 00910 0x00,0xE8,0x60,0x00,0x00,0xE0,0x20,0x0C,\ 00911 0xD0,0x82,0xC0,0xBC,0x00,0x00,0x00,0x01,\ 00912 0x08,0x40,0x00,0x00,0x16,0x33,0x28,0xFF,\ 00913 0x0C,0x03,0x00,0xFF,0x67,0x48,0x1D,0x87,\ 00914 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 00915 0x00,0xA0,0x08,0x00,0x19,0x83,0x28,0xFF,\ 00916 0xC6,0x3C,0x00,0x80,0x18,0x34,0x28,0xFF,\ 00917 0x1A,0x04,0xC8,0x3C,0x00,0x80,0xB8,0x03,\ 00918 0x67,0x24,0x08,0x05,0x00,0x05,0x67,0xEC,\ 00919 0x18,0x34,0x28,0xFF,0xC8,0x3C,0x00,0x80,\ 00920 0xB8,0x03,0x67,0x12,0x1D,0x87,0x08,0x00,\ 00921 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 00922 0x08,0x00,0x60,0x00,0x00,0x84,0x53,0x82,\ 00923 0x66,0xA6,0x60,0x78,0x36,0x33,0x28,0xFE,\ 00924 0x0C,0x43,0xFF,0xFF,0x67,0x3A,0x3C,0x87,\ 00925 0x3A,0x86,0x3C,0xBC,0x00,0xA0,0x39,0x83,\ 00926 0x28,0xFE,0xC6,0x7C,0x00,0x80,0x38,0x34,\ 00927 0x28,0xFE,0x3A,0x04,0xC8,0x7C,0x00,0x80,\ 00928 0xB8,0x43,0x67,0x1C,0x08,0x05,0x00,0x05,\ 00929 0x67,0xEC,0x38,0x34,0x28,0xFE,0xC8,0x7C,\ 00930 0x00,0x80,0xB8,0x43,0x67,0x0A,0x3C,0x87,\ 00931 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x38,\ 00932 0x55,0x82,0x66,0xB8,0x60,0x2E,0x3C,0x87,\ 00933 0x3A,0x86,0x3C,0xBC,0xA0,0xA0,0x39,0xB3,\ 00934 0x28,0xFE,0x28,0xFE,0x55,0x82,0x66,0xF6,\ 00935 0x32,0x3C,0x55,0xF0,0x4E,0x71,0x51,0xC9,\ 00936 0xFF,0xFC,0x34,0x3C,0x01,0x00,0x36,0x33,\ 00937 0x28,0xFE,0xB6,0x74,0x28,0xFE,0x66,0x08,\ 00938 0x55,0x82,0x66,0xF2,0x42,0x80,0x60,0x02,\ 00939 0x70,0x01,0x4E,0x75,\ 00940 0x4F,0xFB,0x01,0x70,0x00,0x00,0x02,0xF0,\ 00941 0x4E,0xBA,0xFC,0x08,0x4E,0xBA,0xFC,0x82,\ 00942 0x4E,0xBA,0xFD,0x30,0x4A,0xFA,0x42,0x80,\ 00943 0x22,0x40,0x4E,0xBA,0xFE,0x88,0x4A,0xFA,\ 00944 0xD2,0xFC,0x01,0x00,0x60,0xF4 00945 }; 00946 00947 /* 00948 uint8_t flashDriver8[] = {\ 00949 0x60,0x00,0x05,0xC2,0x54,0x72,0x69,0x6F,\ 00950 0x6E,0x69,0x63,0x20,0x45,0x43,0x55,0x20,\ 00951 0x46,0x4C,0x41,0x53,0x48,0x20,0x73,0x63,\ 00952 0x72,0x69,0x70,0x74,0x0D,0x0A,0x00,0x50,\ 00953 0x72,0x6F,0x67,0x72,0x61,0x6D,0x6D,0x69,\ 00954 0x6E,0x67,0x20,0x46,0x4C,0x41,0x53,0x48,\ 00955 0x20,0x63,0x68,0x69,0x70,0x20,0x61,0x64,\ 00956 0x64,0x72,0x65,0x73,0x73,0x65,0x73,0x3A,\ 00957 0x0D,0x0A,0x00,0x54,0x72,0x69,0x6F,0x6E,\ 00958 0x69,0x63,0x20,0x45,0x43,0x55,0x20,0x46,\ 00959 0x4C,0x41,0x53,0x48,0x20,0x63,0x68,0x69,\ 00960 0x70,0x73,0x20,0x75,0x70,0x64,0x61,0x74,\ 00961 0x65,0x64,0x20,0x2D,0x20,0x65,0x6E,0x6A,\ 00962 0x6F,0x79,0x20,0x3A,0x2D,0x29,0x0D,0x0A,\ 00963 0x00,0x46,0x4C,0x41,0x53,0x48,0x20,0x73,\ 00964 0x69,0x7A,0x65,0x3A,0x20,0x30,0x78,0x30,\ 00965 0x46,0x61,0x64,0x65,0x30,0x20,0x42,0x79,\ 00966 0x74,0x65,0x73,0x0D,0x0A,0x00,0x30,0x78,\ 00967 0x30,0x43,0x61,0x66,0x65,0x30,0x2D,0x30,\ 00968 0x42,0x61,0x62,0x65,0x30,0x0D,0x00,0x72,\ 00969 0x62,0x00,\ 00970 0x30,0x31,0x32,0x33,0x34,0x35,0x36,0x37,\ 00971 0x38,0x39,0x41,0x42,0x43,0x44,0x45,0x46,\ 00972 0xE1,0x9A,0x76,0x05,0x42,0x84,0xE9,0x9A,\ 00973 0x18,0x02,0x02,0x04,0x00,0x0F,0x10,0xFB,\ 00974 0x40,0xE0,0x51,0xCB,0xFF,0xF2,0x4E,0x75,\ 00975 0x7C,0x2F,0x2D,0x5C,0x2A,0x0D,0x00,0x00,\ 00976 0x02,0x03,0x00,0x03,0x41,0xFA,0xFF,0xF6,\ 00977 0x10,0xBB,0x30,0xEE,0x70,0x01,0x4A,0xFA,\ 00978 0x52,0x43,0x4E,0x75,\ 00979 0x20,0x7C,0x00,0xFF,0xFA,0x00,0x08,0x10,\ 00980 0x00,0x04,0x66,0x4E,0xD0,0xFC,0x00,0x04,\ 00981 0x10,0xFC,0x00,0x7F,0x08,0x10,0x00,0x03,\ 00982 0x67,0xFA,0xD0,0xFC,0x00,0x1C,0x42,0x10,\ 00983 0xD0,0xFC,0x00,0x23,0x30,0xBC,0x3F,0xFF,\ 00984 0xD0,0xFC,0x00,0x04,0x70,0x07,0x30,0xC0,\ 00985 0x30,0xBC,0x68,0x70,0xD0,0xFC,0x00,0x06,\ 00986 0x30,0xC0,0x30,0xFC,0x30,0x30,0x30,0xC0,\ 00987 0x30,0xBC,0x50,0x30,0xD0,0xFC,0x01,0xBE,\ 00988 0x70,0x40,0x30,0xC0,0x30,0x80,0x30,0x3C,\ 00989 0x55,0xF0,0x4E,0x71,0x51,0xC8,0xFF,0xFC,\ 00990 0x60,0x18,0xD0,0xFC,0x00,0x08,0x30,0xFC,\ 00991 0x69,0x08,0x08,0x10,0x00,0x09,0x67,0xFA,\ 00992 0x31,0x3C,0x68,0x08,0xD0,0xFC,0x00,0x48,\ 00993 0x42,0x50,0x4E,0x75,\ 00994 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,\ 00995 0x00,0x00,0x2C,0x3C,0x00,0x00,0x55,0x55,\ 00996 0x2E,0x3C,0x00,0x00,0xAA,0xAA,0x2A,0x46,\ 00997 0x53,0x8D,0x2C,0x47,0x45,0xF8,0x00,0x00,\ 00998 0x47,0xFA,0xFF,0xDE,0x3C,0x87,0x3A,0x86,\ 00999 0x3C,0xBC,0x90,0x90,0x36,0xDA,0x36,0x92,\ 01000 0x3C,0x87,0x3A,0x86,0x3C,0xBC,0xF0,0xF0,\ 01001 0x20,0x3A,0xFF,0xC6,0x72,0x02,0x48,0x41,\ 01002 0x74,0x01,0x0C,0x00,0x00,0x25,0x67,0x50,\ 01003 0x0C,0x00,0x00,0xB8,0x67,0x4A,0x74,0x04,\ 01004 0x0C,0x00,0x00,0x5D,0x67,0x42,0x74,0x01,\ 01005 0xE3,0x99,0x0C,0x00,0x00,0xA7,0x67,0x38,\ 01006 0x0C,0x00,0x00,0xB4,0x67,0x32,0x74,0x02,\ 01007 0x0C,0x00,0x00,0x20,0x67,0x2A,0x0C,0x00,\ 01008 0x00,0xA4,0x67,0x24,0x0C,0x00,0x00,0xB5,\ 01009 0x67,0x1E,0x74,0x04,0x0C,0x00,0x00,0xD5,\ 01010 0x67,0x16,0x74,0x03,0xE3,0x99,0x0C,0x00,\ 01011 0x00,0x23,0x67,0x0C,0xE3,0x99,0x0C,0x00,\ 01012 0x00,0x81,0x67,0x04,0x72,0x00,0x74,0x00,\ 01013 0x47,0xFA,0xFF,0x6A,0x26,0x81,0x47,0xFA,\ 01014 0xFF,0x68,0x16,0x82,0x4E,0x75,\ 01015 0x46,0x6F,0x75,0x6E,0x64,0x20,0x61,0x20,\ 01016 0x54,0x20,0x20,0x20,0x0D,0x0A,0x00,0x00,\ 01017 0x20,0x3A,0xFF,0x4C,0x48,0x40,0x41,0xFA,\ 01018 0xFF,0xF1,0x72,0x38,0xE9,0x18,0x65,0x16,\ 01019 0x72,0x37,0xE3,0x18,0x65,0x10,0x72,0x35,\ 01020 0x10,0xC1,0x72,0x2E,0x10,0xC1,0x72,0x35,\ 01021 0xE3,0x18,0x65,0x02,0x72,0x32,0x10,0x81,\ 01022 0x41,0xFA,0xFF,0xC6,0x70,0x01,0x4A,0xFA,\ 01023 0x4E,0x75,\ 01024 0x46,0x49,0x4C,0x45,0x4E,0x41,0x4D,0x45,\ 01025 0x2E,0x42,0x49,0x4E,0x00,0x45,0x6E,0x74,\ 01026 0x65,0x72,0x20,0x61,0x20,0x66,0x69,0x6C,\ 01027 0x65,0x6E,0x61,0x6D,0x65,0x20,0x28,0x75,\ 01028 0x70,0x20,0x74,0x6F,0x20,0x38,0x20,0x63,\ 01029 0x68,0x61,0x72,0x61,0x63,0x74,0x65,0x72,\ 01030 0x73,0x29,0x3A,0x20,0x00,0x00,0x41,0xFA,\ 01031 0xFF,0xD5,0x70,0x01,0x4A,0xFA,0x41,0xFA,\ 01032 0xFF,0xC0,0x78,0x08,0x70,0x04,0x4A,0xFA,\ 01033 0x0C,0x00,0x00,0x0D,0x67,0x26,0x0C,0x00,\ 01034 0x00,0x2E,0x67,0x20,0x0C,0x00,0x00,0x08,\ 01035 0x66,0x0A,0xB8,0x00,0x67,0xE6,0x53,0x48,\ 01036 0x54,0x44,0x60,0x06,0x4A,0x04,0x67,0x0C,\ 01037 0x10,0xC0,0x12,0x00,0x70,0x02,0x4A,0xFA,\ 01038 0x51,0xCC,0xFF,0xD2,0x10,0xFC,0x00,0x2E,\ 01039 0x10,0xFC,0x00,0x42,0x10,0xFC,0x00,0x49,\ 01040 0x10,0xFC,0x00,0x4E,0x10,0xBC,0x00,0x00,\ 01041 0x59,0x88,0x70,0x01,0x4A,0xFA,0x41,0xFA,\ 01042 0xFD,0xB5,0x70,0x01,0x4A,0xFA,0x41,0xFA,\ 01043 0xFF,0x68,0x43,0xFA,0xFD,0xBD,0x70,0x06,\ 01044 0x4A,0xFA,0x41,0xFA,0xFF,0x58,0x20,0x80,\ 01045 0x4E,0x75,\ 01046 0x45,0x72,0x61,0x73,0x69,0x6E,0x67,0x20,\ 01047 0x46,0x4C,0x41,0x53,0x48,0x20,0x63,0x68,\ 01048 0x69,0x70,0x73,0x0D,0x0A,0x00,0x41,0xFA,\ 01049 0xFF,0xE8,0x70,0x01,0x4A,0xFA,0x12,0x3A,\ 01050 0xFE,0x52,0x53,0x01,0x67,0x16,0x53,0x01,\ 01051 0x67,0x00,0x00,0xB8,0x53,0x01,0x67,0x00,\ 01052 0x01,0x0A,0x53,0x01,0x67,0x00,0x01,0x3A,\ 01053 0x60,0x00,0x01,0x3A,0x4B,0xF8,0x00,0x00,\ 01054 0x24,0x3A,0xFE,0x2C,0x26,0x02,0x3A,0xBC,\ 01055 0xFF,0xFF,0x3A,0xBC,0xFF,0xFF,0x42,0x55,\ 01056 0x4A,0x35,0x28,0xFF,0x67,0x28,0x7A,0x19,\ 01057 0x1B,0xBC,0x00,0x40,0x28,0xFF,0x42,0x35,\ 01058 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 01059 0xFF,0xFC,0x1B,0xBC,0x00,0xC0,0x28,0xFF,\ 01060 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 01061 0x4A,0x35,0x28,0xFF,0x66,0x06,0x53,0x82,\ 01062 0x66,0xCC,0x60,0x04,0x53,0x45,0x66,0xD0,\ 01063 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 01064 0x00,0xE4,0x24,0x03,0x50,0xC4,0x2A,0x3C,\ 01065 0x03,0xE8,0x03,0xE8,0x72,0x20,0x1B,0x81,\ 01066 0x28,0xFF,0x1B,0x81,0x28,0xFF,0x32,0x3C,\ 01067 0x55,0xF0,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 01068 0x4E,0xBA,0xFD,0x30,0x1B,0xBC,0x00,0xA0,\ 01069 0x28,0xFF,0x72,0x0C,0x4E,0x71,0x51,0xC9,\ 01070 0xFF,0xFC,0xB8,0x35,0x28,0xFF,0x66,0x08,\ 01071 0x48,0x45,0x53,0x82,0x66,0xE6,0x60,0x04,\ 01072 0x53,0x45,0x66,0xC8,0x42,0x55,0x4A,0x55,\ 01073 0x4A,0x45,0x67,0x00,0x00,0x98,0x60,0x00,\ 01074 0x00,0x90,0x70,0x01,0x42,0x83,0x1D,0x87,\ 01075 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 01076 0x00,0x80,0x08,0x00,0x1D,0x87,0x08,0x00,\ 01077 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0x10,\ 01078 0x08,0x00,0x2A,0x00,0x4E,0xBA,0xFC,0xDC,\ 01079 0x20,0x05,0x1A,0x30,0x09,0x90,0x08,0x05,\ 01080 0x00,0x07,0x66,0x20,0x08,0x05,0x00,0x05,\ 01081 0x67,0xE8,0x1A,0x30,0x09,0x90,0x08,0x05,\ 01082 0x00,0x07,0x66,0x10,0x1D,0x87,0x08,0x00,\ 01083 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 01084 0x08,0x00,0x60,0x40,0x53,0x80,0x67,0xAE,\ 01085 0x60,0x36,0x42,0x83,0x3C,0x87,0x3A,0x86,\ 01086 0x3C,0xBC,0x00,0x80,0x3C,0x87,0x3A,0x86,\ 01087 0x3C,0xBC,0x00,0x10,0x4E,0xBA,0xFC,0x94,\ 01088 0x3A,0x15,0x08,0x05,0x00,0x07,0x66,0x18,\ 01089 0x08,0x05,0x00,0x05,0x67,0xEE,0x3A,0x15,\ 01090 0x08,0x05,0x00,0x07,0x66,0x0A,0x3C,0x87,\ 01091 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x04,\ 01092 0x42,0x80,0x60,0x02,0x70,0x01,0x4E,0x75,\ 01093 0x47,0xFB,0x01,0x70,0x00,0x00,0x02,0x96,\ 01094 0x28,0x49,0x24,0x3C,0x00,0x00,0x01,0x00,\ 01095 0x12,0x3A,0xFC,0xE8,0x53,0x01,0x67,0x14,\ 01096 0x53,0x01,0x67,0x5A,0x53,0x01,0x67,0x00,\ 01097 0x00,0xBC,0x53,0x01,0x67,0x00,0x01,0x00,\ 01098 0x60,0x00,0x01,0x2E,0x10,0x33,0x28,0xFF,\ 01099 0x0C,0x00,0x00,0xFF,0x67,0x28,0x7A,0x19,\ 01100 0x19,0xBC,0x00,0x40,0x28,0xFF,0x19,0x80,\ 01101 0x28,0xFF,0x72,0x15,0x4E,0x71,0x51,0xC9,\ 01102 0xFF,0xFC,0x19,0xBC,0x00,0xC0,0x28,0xFF,\ 01103 0x72,0x0C,0x4E,0x71,0x51,0xC9,0xFF,0xFC,\ 01104 0xB0,0x34,0x28,0xFF,0x66,0x06,0x53,0x82,\ 01105 0x66,0xCA,0x60,0x04,0x53,0x05,0x66,0xD0,\ 01106 0x42,0x55,0x4A,0x55,0x4A,0x05,0x67,0x00,\ 01107 0x00,0xE8,0x60,0x00,0x00,0xE0,0x20,0x0C,\ 01108 0xD0,0x82,0xC0,0xBC,0x00,0x00,0x00,0x01,\ 01109 0x08,0x40,0x00,0x00,0x16,0x33,0x28,0xFF,\ 01110 0x0C,0x03,0x00,0xFF,0x67,0x48,0x1D,0x87,\ 01111 0x08,0x00,0x1B,0x86,0x08,0x00,0x1D,0xBC,\ 01112 0x00,0xA0,0x08,0x00,0x19,0x83,0x28,0xFF,\ 01113 0xC6,0x3C,0x00,0x80,0x18,0x34,0x28,0xFF,\ 01114 0x1A,0x04,0xC8,0x3C,0x00,0x80,0xB8,0x03,\ 01115 0x67,0x24,0x08,0x05,0x00,0x05,0x67,0xEC,\ 01116 0x18,0x34,0x28,0xFF,0xC8,0x3C,0x00,0x80,\ 01117 0xB8,0x03,0x67,0x12,0x1D,0x87,0x08,0x00,\ 01118 0x1B,0x86,0x08,0x00,0x1D,0xBC,0x00,0xF0,\ 01119 0x08,0x00,0x60,0x00,0x00,0x84,0x53,0x82,\ 01120 0x66,0xA6,0x60,0x78,0x36,0x33,0x28,0xFE,\ 01121 0x0C,0x43,0xFF,0xFF,0x67,0x3A,0x3C,0x87,\ 01122 0x3A,0x86,0x3C,0xBC,0x00,0xA0,0x39,0x83,\ 01123 0x28,0xFE,0xC6,0x7C,0x00,0x80,0x38,0x34,\ 01124 0x28,0xFE,0x3A,0x04,0xC8,0x7C,0x00,0x80,\ 01125 0xB8,0x43,0x67,0x1C,0x08,0x05,0x00,0x05,\ 01126 0x67,0xEC,0x38,0x34,0x28,0xFE,0xC8,0x7C,\ 01127 0x00,0x80,0xB8,0x43,0x67,0x0A,0x3C,0x87,\ 01128 0x3A,0x86,0x3C,0xBC,0x00,0xF0,0x60,0x38,\ 01129 0x55,0x82,0x66,0xB8,0x60,0x2E,0x3C,0x87,\ 01130 0x3A,0x86,0x3C,0xBC,0xA0,0xA0,0x39,0xB3,\ 01131 0x28,0xFE,0x28,0xFE,0x55,0x82,0x66,0xF6,\ 01132 0x32,0x3C,0x55,0xF0,0x4E,0x71,0x51,0xC9,\ 01133 0xFF,0xFC,0x34,0x3C,0x01,0x00,0x36,0x33,\ 01134 0x28,0xFE,0xB6,0x74,0x28,0xFE,0x66,0x08,\ 01135 0x55,0x82,0x66,0xF2,0x42,0x80,0x60,0x02,\ 01136 0x70,0x01,0x4E,0x75,\ 01137 0x4F,0xFB,0x01,0x70,0x00,0x00,0x01,0x3A,\ 01138 0x41,0xFA,0xFA,0x36,0x70,0x01,0x4A,0xFA,\ 01139 0x4E,0xBA,0xFB,0x10,0x4E,0xBA,0xFB,0x8A,\ 01140 0x4A,0x42,0x66,0x06,0x74,0x01,0x60,0x00,\ 01141 0x00,0x42,0x4E,0xBA,0xFC,0x28,0x41,0xFA,\ 01142 0xFA,0x93,0x24,0x3A,0xFB,0x6E,0x4E,0xBA,\ 01143 0xFA,0xBE,0x41,0xFA,0xFA,0x79,0x70,0x01,\ 01144 0x4A,0xFA,0x4E,0xBA,0xFD,0x06,0x4A,0x00,\ 01145 0x67,0x06,0x74,0x05,0x60,0x00,0x00,0x1C,\ 01146 0x4A,0xFA,0x41,0xFA,0xFA,0x0F,0x70,0x01,\ 01147 0x4A,0xFA,0x42,0x80,0x22,0x40,0x4E,0xBA,\ 01148 0xFE,0x4C,0x4A,0xFA,0xD2,0xFC,0x01,0x00,\ 01149 0x60,0xF4,0x20,0x7C,0x00,0xFF,0xFA,0x00,\ 01150 0x08,0x10,0x00,0x04,0x66,0x08,0x02,0x79,\ 01151 0xFF,0xBF,0x00,0xFF,0xFC,0x14,0x22,0x02,\ 01152 0x70,0x00,0x4A,0xFA 01153 }; 01154 */ 01155 01156 01157 //if (prep_t5_do() != TERM_OK) return TERM_ERR; 01158 // Set Program counter to start of BDM driver code 01159 uint32_t driverAddress = 0x100000; 01160 if (sysreg_write(0x0, &driverAddress) != TERM_OK) break; 01161 for (uint32_t i = 0; i < sizeof(flashDriver); i++) { 01162 if(memwrite_byte(&driverAddress, flashDriver[i]) != TERM_OK) return false; 01163 driverAddress++; 01164 } 01165 // if (!bdmLoadMemory(flashDriver, driverAddress, sizeof(flashDriver))) break; 01166 01167 timer.reset(); 01168 timer.start(); 01169 printf("Erasing FLASH chips...\r\n"); 01170 printf("This can take up to a minute for a T8,\r\n"); 01171 printf("30s for a T7 or 15s for a T5 ECU.\r\n"); 01172 // execute the erase algorithm in the BDM driver 01173 // write the buffer - should complete within 200 milliseconds 01174 // Typical and Maximum Chip Programming times are 9 and 27 seconds for Am29BL802C 01175 // Typical Chip erase time for Am29BL802C is 45 secinds, not including 0x00 programming prior to erasure. 01176 // Allow for at least worst case 27 seconds programming to 0x00 + 3(?) * 45 typical erase time (162 seconds) 01177 // Allow at least 200 seconds erase time 2,000 * (100ms + BDM memread time) 01178 // NOTE: 29/39F010 and 29F400 erase times are considerably lower 01179 01180 // if (sysreg_write(0x0, &driverAddress) != TERM_OK) return TERM_ERR; 01181 // break; 01182 do { 01183 if (!bdmRunDriver(0x0, 200000)) { 01184 printf("WARNING: An error occured when I tried to erase the FLASH chips :-(\r\n"); 01185 return TERM_ERR; 01186 } 01187 } while (bdmProcessSyscall() == CONTINUE); 01188 01189 // if (!run_bdm_driver(0x0, 200000)) { 01190 // printf("WARNING: An error occured when I tried to erase the FLASH chips :-(\r\n"); 01191 // return TERM_ERR; 01192 // } 01193 printf("Erasing took %#.1f seconds.\r\n",timer.read()); 01194 01195 printf("Programming the FLASH chips...\r\n"); 01196 01197 // ready to receive data 01198 printf(" 0.00 %% complete.\r"); 01199 while (curr_addr < flash_size) { 01200 // receive bytes from BIN file - break if no more bytes to get 01201 if (!fread(file_buffer,1,0x100,fp)) { 01202 fclose(fp); 01203 printf("Error reading the BIN file MODIFIED.BIN"); 01204 break; 01205 } 01206 if (!bdmLoadMemory((uint8_t*)file_buffer, 0x100700, 0x100)) break; 01207 // write the buffer - should complete within 200 milliseconds 01208 if (!bdmRunDriver(0x0, 200)) break; 01209 // if (!run_bdm_driver(0x0, 200)) break; 01210 01211 printf("%6.2f\r", 100*(float)curr_addr/(float)flash_size ); 01212 // make the activity LED twinkle 01213 ACTIVITYLEDON; 01214 curr_addr += 0x100; 01215 } 01216 break; 01217 } 01218 // johnc's original method 01219 case AMD29F400B: /// a sort of dummy 'placeholder' as the 'B' chip isn't ever fitted to T7 ECUS 01220 default: { 01221 timer.reset(); 01222 timer.start(); 01223 01224 // reset the FLASH chips 01225 printf("Reset the FLASH chip(s) to prepare them for Erasing\r\n"); 01226 if (!reset_func()) return TERM_ERR; 01227 01228 switch (type) { 01229 // AM29Fxxx chips (retrofitted to Trionic 5.x; original to T7) 01230 case AMD29BL802C: 01231 case AMD29F400B: 01232 case AMD29F400T: 01233 case AMD29F010: 01234 case SST39SF010: 01235 case AMICA29010L: 01236 printf("Erasing 29BL802/F400/010 type FLASH chips...\r\n"); 01237 if (!erase_am29()) { 01238 printf("WARNING: An error occured when I tried to erase the FLASH chips :-(\r\n"); 01239 return TERM_ERR; 01240 } 01241 break; 01242 // AM28F010 chip (Trionic 5.x original) 01243 case AMD28F010: 01244 case INTEL28F010: 01245 case AMD28F512: 01246 case INTEL28F512: 01247 printf("Erasing 28F010/512 type FLASH chips...\r\n"); 01248 if (!erase_am28(&curr_addr, &flash_size)) { 01249 printf("WARNING: An error occured when I tried to erase the FLASH chips :-(\r\n"); 01250 return TERM_ERR; 01251 } 01252 break; 01253 case ATMEL29C010: 01254 case ATMEL29C512: 01255 printf("Atmel FLASH chips do not require ERASEing :-)\r\n"); 01256 break; 01257 default: 01258 // unknown flash type - shouldn't get here hence "Strange!" 01259 printf("Strange! I couldn't work out how to erase the FLASH chips in the TRIONIC ECU that I am connected to :-(\r\n"); 01260 return TERM_ERR; 01261 } 01262 01263 timer.stop(); 01264 printf("Erasing took %#.1f seconds.\r\n",timer.read()); 01265 01266 printf("Programming the FLASH chips...\r\n"); 01267 01268 timer.reset(); 01269 timer.start(); 01270 01271 uint16_t word_value = 0; 01272 01273 // ready to receive data 01274 printf(" 0.00 %% complete.\r"); 01275 while (curr_addr < flash_size) { 01276 // receive bytes from BIN file 01277 //Get a byte - break if no more bytes to get 01278 if (!fread(&file_buffer[0],1,0x2,fp)) { 01279 fclose(fp); 01280 printf("Error reading the BIN file MODIFIED.BIN"); 01281 break; 01282 } 01283 for(uint32_t i=0; i<2; i++) { 01284 (word_value <<= 8) |= file_buffer[i]; 01285 } 01286 01287 // write the word if it is not 0xffff 01288 if (word_value != 0xffff) { 01289 if (!flash_func(&curr_addr, word_value)) break; 01290 } 01291 01292 if (!(curr_addr % 0x80)) { 01293 printf("%6.2f\r", 100*(float)curr_addr/(float)flash_size ); 01294 // make the activity LED twinkle 01295 ACTIVITYLEDON; 01296 } 01297 curr_addr += 2; 01298 } 01299 } 01300 } 01301 timer.stop(); 01302 fclose(fp); 01303 01304 if (curr_addr == flash_size) { 01305 printf("100.00\r\n"); 01306 printf("Programming took %#.1f seconds.\r\n",timer.read()); 01307 01308 // "Just4pleisure;)" 'tag' in the empty space at the end of the FLASH chip 01309 // Removed for now because it conflicts with some information that Dilemma places in this empty space 01310 // and because it is unsafe for Trionic 8 ECUs which have much bigger BIN files and FLASH chips 01311 // reset_func(); 01312 // for (uint8_t i = 0; i < 8; ++i) { 01313 // memread_word(&word_value, &flash_tag[i].addr); 01314 // flash_func(&flash_tag[i].addr, (flash_tag[i].val & word_value)); 01315 // } 01316 01317 } else { 01318 printf("\r\n"); 01319 printf("Programming took %#.1f seconds.\r\n",timer.read()); 01320 printf("WARNING: Oh dear, I couldn't program the FLASH at address 0x%08x.\r\n", curr_addr); 01321 } 01322 01323 // reset flash 01324 return (reset_func() && (curr_addr == flash_size)) ? TERM_OK : TERM_ERR; 01325 } 01326 01327 //----------------------------------------------------------------------------- 01328 /** 01329 Resets an AM29Fxxx flash memory chip. MCU must be in background mode. 01330 01331 @param none 01332 01333 @return succ / fail 01334 */ 01335 bool reset_am29(void) 01336 { 01337 // execute the reset command 01338 // uint32_t addr = 0xfffe; 01339 // return (memwrite_word(&addr, 0xf0f0) == TERM_OK); 01340 // execute the algorithm 01341 for (uint8_t i = 0; i < 3; ++i) { 01342 if (memwrite_word(&am29_reset[i].addr, am29_reset[i].val) != TERM_OK) return false; 01343 } 01344 return true; 01345 } 01346 01347 //----------------------------------------------------------------------------- 01348 /** 01349 Erases an AM29Fxxx flash memory chip and verifies the result; MCU must be 01350 in background mode. 01351 01352 @return succ / fail 01353 */ 01354 bool erase_am29() 01355 { 01356 // reset flash 01357 if (!reset_am29()) { 01358 return false; 01359 } 01360 printf("Erasing AMD 29Fxxx FLASH chips.\r\n"); 01361 printf("This can take up to a minute for a T8,\r\n"); 01362 printf("30s for a T7 or 15s for a T5 ECU.\r\n"); 01363 // execute the algorithm 01364 for (uint8_t i = 0; i < 6; ++i) { 01365 if (memwrite_word(&am29_erase[i].addr, am29_erase[i].val) != TERM_OK) { 01366 reset_am29(); 01367 return false; 01368 } 01369 } 01370 01371 // verify the result 01372 uint32_t addr = 0x0; 01373 uint16_t verify_value; 01374 01375 printf(" 0.0 seconds.\r"); 01376 timeout.reset(); 01377 timeout.start(); 01378 while (timeout.read() < 200.0) { 01379 // Typical and Maximum Chip Programming times are 9 and 27 seconds for Am29BL802C 01380 // Typical Chip erase time for Am29BL802C is 45 secinds, not including 0x00 programming prior to erasure. 01381 // Allow for at least worst case 27 seconds programming to 0x00 + 3(?) * 45 typical erase time (162 seconds) 01382 // Allow at least 200 seconds erase time 2,000 * (100ms + BDM memread time) 01383 // NOTE: 29/39F010 and 29F400 erase times are considerably lower 01384 if (memread_word(&verify_value, &addr) == TERM_OK && verify_value == 0xffff) { 01385 // erase completed normally 01386 reset_am29(); 01387 printf("\n"); 01388 return true; 01389 } 01390 // make the activity LED twinkle 01391 ACTIVITYLEDON; 01392 printf("%5.1f\r", timeout.read()); 01393 } 01394 // erase failed 01395 printf("\n"); 01396 reset_am29(); 01397 return false; 01398 } 01399 01400 //----------------------------------------------------------------------------- 01401 /** 01402 Writes a word to AM29Fxxx flash memory chip and optionally verifies the 01403 result; MCU must be in background mode. 01404 01405 @param addr destination address 01406 @param val value 01407 01408 @return succ / fail 01409 */ 01410 bool flash_am29(const uint32_t* addr, uint16_t value) 01411 { 01412 01413 // execute the algorithm 01414 for (uint8_t i = 0; i < 3; ++i) { 01415 if (memwrite_word(&am29_write[i].addr, am29_write[i].val) != TERM_OK) { 01416 reset_am29(); 01417 return false; 01418 } 01419 } 01420 // write the value 01421 if (memwrite_word(addr, value) != TERM_OK) { 01422 reset_am29(); 01423 return false; 01424 } 01425 // verify the result 01426 timeout.reset(); 01427 timeout.start(); 01428 while (timeout.read_us() < 500) { 01429 // Typical and Maximum Word Programming times are 9us and 360us for Am29BL802C 01430 // Allow at least 500 microseconds program time 500 * (1us + BDM memread time) 01431 // NOTE: 29/39F010 and 29F400 programming times are considerably lower 01432 uint16_t verify_value; 01433 if ((memread_word(&verify_value, addr) == TERM_OK) && 01434 (verify_value == value)) { 01435 // flashing successful 01436 return true; 01437 } 01438 } 01439 // writing failed 01440 reset_am29(); 01441 return false; 01442 } 01443 01444 01445 //----------------------------------------------------------------------------- 01446 /** 01447 Writes a word to a FLASH memory chip and checks the result 01448 MCU must be in background mode. 01449 01450 @param addr BDM driver address (0 to continue) 01451 @param maxtime how long to allow driver to execute (milliseconds) 01452 01453 @return succ / fail 01454 */ 01455 bool run_bdm_driver(uint32_t addr, uint32_t maxtime) 01456 { 01457 // Start BDM driver and allow it up to 200 milliseconds to update 256 Bytes 01458 // Upto 25 pulses per byte, 16us per pulse, 256 Bytes 01459 // 25 * 16 * 256 = 102,400us plus overhead for driver code execution time 01460 // Allowing up to 200 milliseconds seems like a good allowance. 01461 uint32_t driverAddress = addr; 01462 if (run_chip(&driverAddress) != TERM_OK) { 01463 printf("Failed to start BDM driver.\r\n"); 01464 return false; 01465 } 01466 timeout.reset(); 01467 timeout.start(); 01468 // T5 ECUs' BDM interface seem to have problems when the running the CPU and 01469 // sometimes shows the CPU briefly switching between showing BDM mode or that 01470 // the CPU is running. 01471 // I 'debounce' the interface state to workaround this erratic bahaviour 01472 for (uint32_t debounce = 0; debounce < 5; debounce++) { 01473 while (IS_RUNNING) { 01474 debounce = 0; 01475 if (timeout.read_ms() > maxtime) { 01476 printf("Driver did not return to BDM mode.\r\n"); 01477 timeout.stop(); 01478 return false; 01479 } 01480 } 01481 wait_us(1); 01482 } 01483 timeout.stop(); 01484 // Check return code in D0 register (0 - OK, 1 - FAILED) 01485 uint32_t result = 1; 01486 if (adreg_read(&result, 0x0) != TERM_OK) { 01487 printf("Failed to read BDM register.\r\n"); 01488 return false; 01489 } 01490 return (result == 1) ? false : true; 01491 } 01492 01493 01494 //----------------------------------------------------------------------------- 01495 /** 01496 Resets a AM28Fxxx flash memory chip. MCU must be in background mode. 01497 01498 @param start_addr flash start address 01499 01500 @return succ / fail 01501 */ 01502 bool reset_am28(void) 01503 { 01504 uint32_t start_addr = 0x0; 01505 return (memwrite_word_write_word(&start_addr, 0xffff, 0xffff) == TERM_OK); 01506 } 01507 01508 //----------------------------------------------------------------------------- 01509 /** 01510 Erases an AM28Fxxx flash memory chip and verifies the result; MCU must be 01511 in background mode. 01512 01513 @param start_addr flash start address 01514 @param end_addr flash end address 01515 01516 @return succ / fail 01517 */ 01518 bool erase_am28(const uint32_t* start_addr, const uint32_t* end_addr) 01519 { 01520 01521 // check the addresses 01522 if (!start_addr || !end_addr) return false; 01523 01524 // reset flash 01525 if (!reset_am28()) return false; 01526 01527 // write zeroes over entire flash space 01528 uint32_t addr = *start_addr; 01529 01530 printf("First write 0x00 to all FLASH addresses.\r\n"); 01531 printf(" 0.00 %% complete.\r"); 01532 while (addr < *end_addr) { 01533 if (!flash_am28(&addr, 0x0000)) return false; 01534 addr += 2; 01535 // // feedback to host computer 01536 // pc.putc(TERM_OK); 01537 if (!(addr % 0x80)) { 01538 // make the activity LED twinkle 01539 ACTIVITYLEDON; 01540 printf("%6.2f\r", 100*(float)addr/(float)*end_addr ); 01541 } 01542 } 01543 printf("\n"); 01544 01545 // erase flash 01546 addr = *start_addr; 01547 uint8_t verify_value; 01548 01549 printf("Now erasing FLASH and verfiying that all addresses are 0xFF.\r\n"); 01550 printf(" 0.00 %% complete.\r"); 01551 uint16_t pulse_cnt = 0; 01552 if (memwrite_byte_cmd(NULL) != TERM_OK) { 01553 reset_am28(); 01554 return false; 01555 } 01556 while ((++pulse_cnt < 1000) && (addr < *end_addr)) { 01557 // issue the erase command 01558 if (memwrite_write_byte(&addr, 0x20) != TERM_OK || 01559 memwrite_write_byte(&addr, 0x20) != TERM_OK) break; 01560 wait_ms(10); 01561 01562 while (addr < *end_addr) { 01563 // issue the verify command 01564 if (memwrite_read_byte(&addr, 0xa0) != TERM_OK) break; 01565 // wait_us(6); 01566 // check the written value 01567 if (memread_write_byte(&verify_value, &addr) != TERM_OK) break; 01568 if (verify_value != 0xff) break; 01569 // succeeded need to check next address 01570 addr++; 01571 // make the activity LED twinkle 01572 ACTIVITYLEDON; 01573 if (!(addr % 0x80)) { 01574 // make the activity LED twinkle 01575 ACTIVITYLEDON; 01576 printf("%6.2f\r", 100*(float)addr/(float)*end_addr ); 01577 } 01578 } 01579 } 01580 printf("\n"); 01581 // the erase process ends with a BDM_WRITE + BDM_BYTESIZE command left in the BDM 01582 // it is safe to use it to put one of the FLASH chips into read mode and thereby 01583 // leave the BDM ready for the next command 01584 memwrite_nop_byte(start_addr, 0x00); 01585 01586 reset_am28(); 01587 // check for success 01588 return (addr == *end_addr) ? true : false; 01589 } 01590 01591 //----------------------------------------------------------------------------- 01592 /** 01593 Writes a byte to AM28Fxxx flash memory chip and verifies the result 01594 A so called 'mask' method checks the FLASH contents and only tries 01595 to program bytes that need to be programmed. 01596 MCU must be in background mode. 01597 01598 @param addr destination address 01599 @param val value 01600 01601 @return succ / fail 01602 */ 01603 bool flash_am28(const uint32_t* addr, uint16_t value) 01604 { 01605 01606 if (!addr) return false; 01607 01608 uint8_t pulse_cnt = 0; 01609 uint16_t verify_value = 0; 01610 uint16_t mask_value = 0xffff; 01611 01612 // put flash into read mode and read address 01613 if (memwrite_word_read_word(&verify_value, addr, 0x0000) != TERM_OK) return false; 01614 // return if FLASH already has the correct value - e.g. not all of the FLASH is used and is 0xff 01615 if (verify_value == value) return true; 01616 01617 while (++pulse_cnt < 25) { 01618 01619 // set a mask 01620 if ((uint8_t)verify_value == (uint8_t)value) 01621 mask_value &= 0xff00; 01622 if ((uint8_t)(verify_value >> 8) == (uint8_t)(value >> 8)) 01623 mask_value &= 0x00ff; 01624 01625 // write the new value 01626 if (memwrite_word_write_word(addr, (0x4040 & mask_value), value) != TERM_OK) break; 01627 // NOTE the BDM interface is slow enough that there is no need for a 10us delay before verifying 01628 // issue the verification command 01629 // NOTE the BDM interface is slow enough that there is no need for a 6us delay before reading back 01630 if (memwrite_word_read_word(&verify_value, addr, (0xc0c0 & mask_value)) != TERM_OK) break; 01631 // check if flashing was successful; 01632 if (verify_value == value) return true; 01633 } 01634 01635 // something went wrong; reset the flash chip and return failed 01636 reset_am28(); 01637 return false; 01638 } 01639 01640 //----------------------------------------------------------------------------- 01641 /** 01642 Does the equivalent of do prept5.do in BD32 01643 Sets up all of the control registers in the MC68332 so that we can program 01644 the FLASH chips 01645 01646 @param none 01647 01648 @return succ / fail 01649 */ 01650 01651 //uint8_t prep_t5_do(void) { 01652 uint8_t prep_t8_do(void) 01653 { 01654 01655 // reset and freeze the MC68332 chip 01656 if (restart_chip() != TERM_OK) return TERM_ERR; 01657 01658 // set the 'fc' registers to allow supervisor mode access 01659 uint32_t long_value = 0x05; 01660 if (sysreg_write(0x0e, &long_value) != TERM_OK) return TERM_ERR; 01661 if (sysreg_write(0x0f, &long_value) != TERM_OK) return TERM_ERR; 01662 01663 // Set MC68332 to 16 MHz (actually 16.78 MHz) (SYNCR) 01664 long_value = 0x00fffa04; 01665 if (memwrite_word(&long_value, 0x7f00) != TERM_OK) return TERM_ERR; 01666 01667 // Disable watchdog and monitors (SYPCR) 01668 long_value = 0x00fffa21; 01669 if (memwrite_byte(&long_value, 0x00) != TERM_OK) return TERM_ERR; 01670 01671 01672 // Chip select pin assignments (CSPAR0) 01673 long_value = 0x00fffa44; 01674 if (memwrite_word(&long_value, 0x3fff) != TERM_OK) return TERM_ERR; 01675 01676 // Boot Chip select read only, one wait state (CSBARBT) 01677 long_value = 0x00fffa48; 01678 if (memwrite_word(&long_value, 0x0007) != TERM_OK) return TERM_ERR; 01679 if (memfill_word(0x6870) != TERM_OK) return TERM_ERR; 01680 01681 // Chip select 1 and 2 upper lower bytes, zero wait states (CSBAR1, CSOR1, CSBAR2, CSBAR2) 01682 long_value = 0x00fffa50; 01683 if (memwrite_word(&long_value, 0x0007) != TERM_OK) return TERM_ERR; 01684 if (memfill_word(0x3030) != TERM_OK) return TERM_ERR; 01685 if (memfill_word(0x0007) != TERM_OK) return TERM_ERR; 01686 if (memfill_word(0x5030) != TERM_OK) return TERM_ERR; 01687 01688 // PQS Data - turn on VPPH (PORTQS) 01689 long_value = 0x00fffc14; 01690 if (memwrite_word(&long_value, 0x0040) != TERM_OK) return TERM_ERR; 01691 01692 // PQS Data Direction output (DDRQS) 01693 long_value = 0x00fffc17; 01694 if (memwrite_byte(&long_value, 0x40) != TERM_OK) return TERM_ERR; 01695 // wait for programming voltage to be ready 01696 wait_ms(10); 01697 01698 // // Enable internal 2kByte RAM of 68332 at address 0x00100000 (TRAMBAR) 01699 // long_value = 0x00fffb04; 01700 // if (memwrite_word(&long_value, 0x1000) != TERM_OK) return TERM_ERR; 01701 return TERM_OK; 01702 } 01703 01704 //----------------------------------------------------------------------------- 01705 /** 01706 Does the equivalent of do prept5/7/8.do in BD32 01707 Sets up all of the control registers in the MC68332/377 so that we can 01708 program the FLASH chips 01709 01710 @param none 01711 01712 @return succ / fail 01713 */ 01714 01715 uint8_t prep_t5_do(void) 01716 { 01717 // Make sure that BDM clock is SLOW 01718 bdm_clk_mode(SLOW); 01719 // reset and freeze the MC68332/377 chip 01720 if (restart_chip() != TERM_OK) return TERM_ERR; 01721 01722 // define some variables to store address and data values 01723 uint32_t long_value = 0x05; 01724 uint16_t verify_value; 01725 01726 // set the 'fc' registers to allow supervisor mode access 01727 if (sysreg_write(0x0e, &long_value) != TERM_OK) return TERM_ERR; 01728 if (sysreg_write(0x0f, &long_value) != TERM_OK) return TERM_ERR; 01729 01730 // Read MC68332/377 Module Control Register (SIMCR/MCR) 01731 // and use the value to work out if ECU is a T5/7 or a T8 01732 long_value = 0x00fffa00; 01733 if (memread_word(&verify_value, &long_value) != TERM_OK) return TERM_ERR; 01734 //////////////////////////////////////////////////////////////////////////////////////////////////////////////// 01735 // verify_value = 0x7E4F; 01736 //////////////////////////////////////////////////////////////////////////////////////////////////////////////// 01737 // MC68377 MCR = x111111x01001111 binary after a reset 01738 if ((verify_value & 0x7E4F) == 0x7E4F) { 01739 printf ("I have found a Trionic 8 ECU.\r\n"); 01740 // Stop system protection (MDR bit 0 STOP-SYS-PROT) 01741 long_value = 0x00fffa04; 01742 if (memwrite_byte(&long_value, 0x01) != TERM_OK) return TERM_ERR; 01743 // Set MC68377 to double it's default speed (16 MHz?) (SYNCR) 01744 long_value = 0x00fffa08; 01745 // First set the MFD part (change 4x to 8x) 01746 if (memwrite_word(&long_value, 0x6908) != TERM_OK) return TERM_ERR; 01747 // wait for everything to settle (should really check the PLL lock register) 01748 wait_ms(100); 01749 // Now set the RFD part (change /2 to /1) 01750 if (memwrite_word(&long_value, 0x6808) != TERM_OK) return TERM_ERR; 01751 // Disable watchdog and monitors (SYPCR) 01752 long_value = 0x00fffa50; 01753 if (memwrite_word(&long_value, 0x0000) != TERM_OK) return TERM_ERR; 01754 // Enable internal 6kByte RAM of 68377 at address 0x00100000 (DPTRAM) 01755 long_value = 0x00fff684; 01756 if (memwrite_word(&long_value, 0x1000) != TERM_OK) return TERM_ERR; 01757 // can use fast or turbo or nitrous BDM clock mode once ECU has been prepped and CPU clock is ??MHz 01758 // bdm_clk_mode(NITROUS); 01759 } 01760 // MC68332 SIMCR = 0000x00011001111 binary after a reset 01761 //if ((verify_value & 0x00CF) == 0x00CF) { 01762 else { 01763 printf ("I have found a Trionic 5 or 7 ECU.\r\n"); 01764 // Set MC68332 to 16 MHz (actually 16.78 MHz) (SYNCR) 01765 long_value = 0x00fffa04; 01766 if (memwrite_word(&long_value, 0x7f00) != TERM_OK) return TERM_ERR; 01767 // Disable watchdog and monitors (SYPCR) 01768 long_value = 0x00fffa21; 01769 if (memwrite_byte(&long_value, 0x00) != TERM_OK) return TERM_ERR; 01770 // Chip select pin assignments (CSPAR0) 01771 long_value = 0x00fffa44; 01772 if (memwrite_word(&long_value, 0x3fff) != TERM_OK) return TERM_ERR; 01773 // Boot Chip select read only, one wait state (CSBARBT) 01774 long_value = 0x00fffa48; 01775 if (memwrite_word(&long_value, 0x0007) != TERM_OK) return TERM_ERR; 01776 if (memfill_word(0x6870) != TERM_OK) return TERM_ERR; 01777 // Chip select 1 and 2 upper lower bytes, zero wait states (CSBAR1, CSOR1, CSBAR2, CSBAR2) 01778 long_value = 0x00fffa50; 01779 if (memwrite_word(&long_value, 0x0007) != TERM_OK) return TERM_ERR; 01780 if (memfill_word(0x3030) != TERM_OK) return TERM_ERR; 01781 if (memfill_word(0x0007) != TERM_OK) return TERM_ERR; 01782 if (memfill_word(0x5030) != TERM_OK) return TERM_ERR; 01783 // PQS Data - turn on VPPH (PORTQS) 01784 long_value = 0x00fffc14; 01785 if (memwrite_word(&long_value, 0x0040) != TERM_OK) return TERM_ERR; 01786 // PQS Data Direction output (DDRQS) 01787 long_value = 0x00fffc17; 01788 if (memwrite_byte(&long_value, 0x40) != TERM_OK) return TERM_ERR; 01789 // wait for programming voltage to be ready 01790 wait_ms(10); 01791 // Enable internal 2kByte RAM of 68332 at address 0x00100000 (TRAMBAR) 01792 long_value = 0x00fffb04; 01793 if (memwrite_word(&long_value, 0x1000) != TERM_OK) return TERM_ERR; 01794 // can use fast or turbo BDM clock mode once ECU has been prepped and CPU clock is 16MHz 01795 // bdm_clk_mode(TURBO); 01796 // bdm_clk_mode(FAST); 01797 } 01798 return TERM_OK; 01799 } 01800 01801 01802 //----------------------------------------------------------------------------- 01803 /** 01804 Works out what type of flash chip is fitted in the ECU by reading 01805 the manufacturer byte codes. 01806 It is enough to use the 29Fxxx flash id algorithm because 28Fxxx 01807 FLASH chips ignore the first few writes needed by the 29Fxxx chips 01808 MCU must be in background mode. 01809 01810 @param make (out) 01811 type (out) 01812 01813 @return succ / fail 01814 */ 01815 bool get_flash_id(uint8_t* make, uint8_t* type) 01816 { 01817 01818 uint32_t addr = 0x0; 01819 uint32_t value; 01820 bool ret; 01821 // read id bytes algorithm for 29F010/400 FLASH chips 01822 for (uint8_t i = 0; i < 3; ++i) { 01823 //printf("Getting FLASH chip ID.\r\n"); 01824 if (memwrite_word(&am29_id[i].addr, am29_id[i].val) != TERM_OK) { 01825 printf("There was an error when I tried to request the FLASH chip ID.\r\n"); 01826 return false; 01827 } 01828 } 01829 if (memread_long(&value, &addr) != TERM_OK) { 01830 printf("Error Reading FLASH chip types in get_flash_id\r\n"); 01831 return false; 01832 } 01833 // *make = (uint8_t)(value >> 24); 01834 // *type = (uint8_t)(value >> 8); 01835 *make = (uint8_t)(value >> 16); 01836 *type = (uint8_t)(value); 01837 printf("FLASH id bytes: %08x, make: %02x, type: %02x\r\n", value, *make, *type); 01838 switch (*type) { 01839 case AMD29BL802C: 01840 case AMD29F400B: 01841 case AMD29F400T: 01842 case AMD29F010: 01843 case AMD28F010: 01844 case INTEL28F010: 01845 case AMD28F512: 01846 case INTEL28F512: 01847 ret = true; 01848 default: 01849 ret = false; 01850 } 01851 return ret; 01852 } 01853 01854 //----------------------------------------------------------------------------- 01855 // EOF 01856 //-----------------------------------------------------------------------------
Generated on Fri Jul 15 2022 00:43:05 by
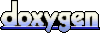