
Just4Trionic - CAN and BDM FLASH programmer for Saab cars
Embed:
(wiki syntax)
Show/hide line numbers
bdmcpu32.h
00001 /******************************************************************************* 00002 00003 bdmcpu32.h 00004 (c) 2010 by Sophie Dexter 00005 00006 A derivative work based on: 00007 //----------------------------------------------------------------------------- 00008 // CAN/BDM adapter firmware 00009 // (C) Janis Silins, 2010 00010 // $id$ 00011 //----------------------------------------------------------------------------- 00012 00013 ******************************************************************************** 00014 00015 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00016 This software is provided 'free' and in good faith, but the author does not 00017 accept liability for any damage arising from its use. 00018 00019 *******************************************************************************/ 00020 00021 #ifndef __BDMCPU32_H__ 00022 #define __BDMCPU32_H__ 00023 00024 #include "mbed.h" 00025 00026 #include "common.h" 00027 #include "bdmtrionic.h" 00028 //#include "BDM.h" 00029 00030 00031 // MCU status macros 00032 #ifndef IGNORE_VCC_PIN 00033 // #define IS_CONNECTED (PIN_PWR) 00034 #define IS_CONNECTED (bool)((LPC_GPIO1->FIOPIN) & (1 << 30)) // PIN_POWER is p19 p1.30 00035 #else 00036 #define IS_CONNECTED true 00037 #endif // IGNORE_VCC_PIN 00038 00039 //#define IN_BDM (PIN_FREEZE) 00040 #define IN_BDM (bool)((LPC_GPIO2->FIOPIN) & (1 << 0)) // FREEZE is p26 P2.0 00041 //#define IS_RUNNING (PIN_RESET && !IN_BDM) 00042 #define IS_RUNNING ((bool)((LPC_GPIO2->FIOPIN) & (1 << 3)) && !IN_BDM) // PIN_RESET is P23 P2.3 00043 00044 // MCU management 00045 uint8_t stop_chip(); 00046 uint8_t reset_chip(); 00047 uint8_t run_chip(const uint32_t* addr); 00048 uint8_t restart_chip(); 00049 uint8_t step_chip(); 00050 uint8_t bkpt_low(); 00051 uint8_t bkpt_high(); 00052 uint8_t reset_low(); 00053 uint8_t reset_high(); 00054 uint8_t berr_low(); 00055 uint8_t berr_high(); 00056 uint8_t berr_input(); 00057 00058 // BDM Clock speed 00059 enum bdm_speed { 00060 SLOW, 00061 FAST, 00062 TURBO, 00063 NITROUS 00064 }; 00065 void bdm_clk_mode(bdm_speed mode); 00066 00067 // memory 00068 uint8_t memread_byte(uint8_t* result, const uint32_t* addr); 00069 uint8_t memread_word(uint16_t* result, const uint32_t* addr); 00070 uint8_t memread_long(uint32_t* result, const uint32_t* addr); 00071 uint8_t memdump_byte(uint8_t* result); 00072 uint8_t memdump_word(uint16_t* result); 00073 uint8_t memdump_long(uint32_t* result); 00074 uint8_t memwrite_byte(const uint32_t* addr, uint8_t value); 00075 uint8_t memwrite_word(const uint32_t* addr, uint16_t value); 00076 uint8_t memwrite_long(const uint32_t* addr, const uint32_t* value); 00077 uint8_t memfill_byte(uint8_t value); 00078 uint8_t memfill_word(uint16_t value); 00079 uint8_t memfill_long(const uint32_t* value); 00080 00081 // memory split commands 00082 // Setup a start of a sequence of BDM operations 00083 // read commands 00084 uint8_t memread_byte_cmd(const uint32_t* addr); 00085 uint8_t memread_word_cmd(const uint32_t* addr); 00086 uint8_t memread_long_cmd(const uint32_t* addr); 00087 // write commands 00088 uint8_t memwrite_byte_cmd(const uint32_t* addr); 00089 uint8_t memwrite_word_cmd(const uint32_t* addr); 00090 uint8_t memwrite_long_cmd(const uint32_t* addr); 00091 // follow on commands 00092 // dump bytes/words/longs 00093 uint8_t memget_word(uint16_t* result); 00094 uint8_t memget_long(uint32_t* result); 00095 // read and write bytes 00096 uint8_t memwrite_write_byte(const uint32_t* addr, const uint8_t value); 00097 uint8_t memwrite_read_byte(const uint32_t* addr, const uint8_t value); 00098 uint8_t memwrite_nop_byte(const uint32_t* addr, const uint8_t value); 00099 uint8_t memread_read_byte(uint8_t* result, const uint32_t* addr); 00100 uint8_t memread_write_byte(uint8_t* result, const uint32_t* addr); 00101 uint8_t memread_nop_byte(uint8_t* result, const uint32_t* addr); 00102 // 00103 uint8_t memwrite_word_write_word(const uint32_t* addr, const uint16_t value1, const uint16_t value2); 00104 uint8_t memwrite_word_read_word(uint16_t* result, const uint32_t* addr, const uint16_t value); 00105 00106 00107 // registers 00108 uint8_t sysreg_read(uint32_t* result, uint8_t reg); 00109 uint8_t sysreg_write(uint8_t reg, const uint32_t* value); 00110 uint8_t adreg_read(uint32_t* result, uint8_t reg); 00111 uint8_t adreg_write(uint8_t reg, const uint32_t* value); 00112 00113 // bdm part commands 00114 bool bdm_command (uint16_t cmd); 00115 bool bdm_address (const uint32_t* addr); 00116 bool bdm_get (uint32_t* result, uint8_t size, uint16_t next_cmd); 00117 bool bdm_put (const uint32_t* value, uint8_t size); 00118 bool bdm_ready (uint16_t next_cmd); 00119 00120 #endif // __BDMCPU32_H__ 00121 //----------------------------------------------------------------------------- 00122 // EOF 00123 //-----------------------------------------------------------------------------
Generated on Fri Jul 15 2022 00:43:05 by
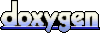