A basic Library for the 16 bit Differential A/D in the K64F Freedom platform
Embed:
(wiki syntax)
Show/hide line numbers
AnalohIn_Diff.cpp
00001 #include "mbed.h" 00002 #include "AnalogIn_Diff.h" 00003 00004 00005 AnalogIn_Diff::AnalogIn_Diff(int a2d_number) : ch(a2d_number) { 00006 if(ch) BW_SIM_SCGC3_ADC1(1); // Turn on clock as needed 00007 else BW_SIM_SCGC6_ADC0(1); 00008 BW_ADC_SC1n_DIFF(ch, 0, 1); // Differential Mode 00009 BW_ADC_CFG1_ADICLK(ch, 0); // Bus Clock 00010 BW_ADC_CFG1_MODE(ch, 3); // 16Bit differential mode 00011 BW_ADC_CFG1_ADLSMP(ch, 0); // Short Sample Window 00012 BW_ADC_CFG1_ADIV(ch, 3); // Clock / 8 00013 BW_ADC_CFG1_ADLPC(ch, 0); // Normal Power Mode 00014 } 00015 00016 AnalogIn_Diff::~AnalogIn_Diff() { } 00017 00018 int16_t AnalogIn_Diff::read_16(int channel) { // Returns a 16bit signed integer 00019 BW_ADC_SC1n_ADCH(ch, 0, channel); // Trigger Conversion 00020 while(!BR_ADC_SC1n_COCO(ch, 0)); // Wait for conversion to finish 00021 return(BR_ADC_Rn_D(ch, 0)); // Return the result 00022 } 00023 00024 float AnalogIn_Diff::read(int channel) { 00025 int16_t i; 00026 float t; 00027 BW_ADC_SC1n_ADCH(ch, 0, channel); 00028 while(!BR_ADC_SC1n_COCO(ch, 0)); 00029 i = BR_ADC_Rn_D(ch, 0); 00030 t = ((float) i); 00031 t = t / 32768.0f; 00032 return(t); 00033 }
Generated on Thu Jul 14 2022 20:58:59 by
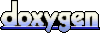