
HTTP_SDCard_File_Server_WIZwiki-W7500 avec gestion des extensions MIME
Dependencies: SDFileSystem WIZnetInterface mbed STATIC_COLORS
Fork of HTTP_SDCard_File_Server_WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "SDFileSystem.h" 00004 #include <stdio.h> 00005 #include <string.h> 00006 00007 #define USE_DHCP 1 00008 00009 #define MAC "\x00\x08\xDC\x11\x34\x78" 00010 #define IP "192.168.0.170" 00011 #define MASK "255.255.255.0" 00012 #define GATEWAY "192.168.0.254" 00013 00014 #define HTTPD_SERVER_PORT 80 00015 #define HTTPD_MAX_REQ_LENGTH 1023 00016 #define HTTPD_MAX_HDR_LENGTH 255 00017 #define HTTPD_MAX_FNAME_LENGTH 127 00018 #define HTTPD_MAX_DNAME_LENGTH 127 00019 00020 #if defined(TARGET_WIZwiki_W7500) 00021 Serial uart(USBTX, USBRX); 00022 SDFileSystem sd(PB_3, PB_2, PB_1, PB_0, "sd"); // WIZwiki-W7500 00023 #include "static_colors.h" 00024 // LED R : server listning status 00025 // LED GREEN : socket connecting status Ok 00026 // LED BLUE : socket connecting status Busy 00027 #endif 00028 00029 EthernetInterface eth; 00030 TCPSocketServer server; 00031 TCPSocketConnection client; 00032 00033 char buffer[HTTPD_MAX_REQ_LENGTH+1]; 00034 char httpHeader[HTTPD_MAX_HDR_LENGTH+1]; 00035 char fileName[HTTPD_MAX_FNAME_LENGTH+1]; 00036 char dirName[HTTPD_MAX_DNAME_LENGTH+1]; 00037 char *uristr; 00038 char *eou; 00039 char *qrystr; 00040 00041 FILE *fp; 00042 int rdCnt; 00043 00044 // Initialize a pins to perform analog input and digital output fucntions 00045 AnalogIn ain(A0); 00046 00047 Ticker ledTick; 00048 00049 //char str[] = "This is a sample string"; 00050 char *pch; 00051 char ext[5]; 00052 char ext_gif[] = "gif"; 00053 char ext_jpg[] = "jpg"; 00054 char ext_png[] = "png"; 00055 char ext_tiff[] = "tiff"; 00056 int pos_ext; 00057 int extLen; 00058 00059 void ledTickfunc() 00060 { 00061 led_r = !led_r; 00062 } 00063 00064 void get_file(char* uri) 00065 { 00066 int i, ext_j; 00067 00068 uart.printf("get_file %s\n", uri); 00069 char *lstchr = strrchr(uri, NULL) -1; 00070 if('/' == *lstchr) 00071 { 00072 uart.printf("Open directory /sd%s\n", uri); 00073 if( 0 == strcmp("/Images/", uri) ) uart.printf("(Directory OK)\n"); 00074 *lstchr = 0; 00075 sprintf(fileName, "/sd%s", uri); 00076 DIR *d = opendir(fileName); 00077 if(d != NULL) 00078 { 00079 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: text/html\r\nConnection: Close\r\n\r\n"); 00080 client.send(httpHeader,strlen(httpHeader)); 00081 sprintf(httpHeader,"<html><head><title>Directory Listing</title></head><body><h2>%s Directory Listing</h2><br><hr><br><ul>", uri); 00082 client.send(httpHeader,strlen(httpHeader)); 00083 struct dirent *p; 00084 while((p = readdir(d)) != NULL) 00085 { 00086 sprintf(dirName, "%s/%s", fileName, p->d_name); 00087 uart.printf("%s\n", dirName); 00088 DIR *subDir = opendir(dirName); 00089 if(subDir != NULL) 00090 { 00091 sprintf(httpHeader,"<li><a href=\"./%s/\">%s/</a></li>", p->d_name, p->d_name); 00092 } 00093 else 00094 { 00095 sprintf(httpHeader,"<li><a href=\"./%s\">%s</a></li>", p->d_name, p->d_name); 00096 } 00097 client.send(httpHeader,strlen(httpHeader)); 00098 } 00099 } 00100 closedir(d); 00101 uart.printf("Directory closed\n"); 00102 sprintf(httpHeader,"</ul></body></html>"); 00103 client.send(httpHeader,strlen(httpHeader)); 00104 } 00105 else 00106 { 00107 sprintf(fileName, "/sd%s", uri); 00108 fp = fopen(fileName, "r"); 00109 if(fp == NULL) 00110 { 00111 uart.printf("File not found\n"); 00112 if( 0 == strcmp("/AIN0.js", uri) ) 00113 { 00114 uart.printf("(File OK)\n"); 00115 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: application/javascript\r\nConnection: Close\r\n\r\n"); 00116 client.send(httpHeader,strlen(httpHeader)); 00117 00118 sprintf(httpHeader,"var AIN0 = %3.3f;\n", ain.read()*3.3f); 00119 client.send(httpHeader,strlen(httpHeader)); 00120 } 00121 else 00122 { 00123 sprintf(httpHeader,"HTTP/1.1 404 Not Found \r\nContent-Type: text\r\nConnection: Close\r\n\r\n"); 00124 client.send(httpHeader,strlen(httpHeader)); 00125 client.send(uri,strlen(uri)); 00126 } 00127 } 00128 else 00129 { 00130 pch = strrchr(fileName,'.'); 00131 00132 if( pch != NULL) 00133 { 00134 pos_ext = pch - fileName + 1; 00135 extLen = strlen(fileName) - pos_ext; 00136 00137 uart.printf("Last occurence of '.' found at %d , extLen : %d\n", pos_ext, extLen ); 00138 00139 for(i=0; i<extLen; i=i+1) 00140 { 00141 ext[i] = fileName[i+pos_ext]; 00142 } 00143 00144 ext[extLen] = '\0'; 00145 00146 uart.printf("Sending header of the : %s\n", fileName); 00147 uart.printf("extension : %s\n", ext); 00148 00149 if( strcmp(ext, ext_gif) == 0 ) ext_j = 1; 00150 00151 if( strcmp(ext, ext_jpg) == 0 ) ext_j = 2; 00152 00153 if( strcmp(ext, ext_png) == 0 ) ext_j = 3; 00154 00155 if( strcmp(ext, ext_tiff) == 0 ) ext_j = 4; 00156 } 00157 else 00158 { 00159 uart.printf("Sending header of the : %s\n", fileName); 00160 ext_j = 0; 00161 } 00162 00163 switch(ext_j) 00164 { 00165 case 1: // HTTP reply with GIF image mime type 00166 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: image/gif\r\nConnection: Close\r\n\r\n"); 00167 break; 00168 00169 case 2: // HTTP reply with JPG image mime type 00170 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: image/jpg\r\nConnection: Close\r\n\r\n"); 00171 break; 00172 00173 case 3: // HTTP reply with PNG image mime type 00174 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: image/jpg\r\nConnection: Close\r\n\r\n"); 00175 break; 00176 00177 case 4: // HTTP reply with TIFF image mime type 00178 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: image/tiff\r\nConnection: Close\r\n\r\n"); 00179 break; 00180 00181 default: // HTTP reply with HTML mime type 00182 sprintf(httpHeader,"HTTP/1.1 200 OK\r\nContent-Type: text/html\r\nConnection: Close\r\n\r\n"); 00183 } 00184 00185 client.send(httpHeader,strlen(httpHeader)); 00186 uart.printf(" file "); 00187 while((rdCnt = fread(buffer, sizeof( char ), 1024, fp)) == 1024) 00188 { 00189 client.send(buffer, rdCnt); 00190 uart.printf("."); 00191 } 00192 client.send(buffer, rdCnt); 00193 fclose(fp); 00194 uart.printf("done\n"); 00195 } 00196 } 00197 } 00198 00199 int main(void) 00200 { 00201 ledTick.attach(&ledTickfunc,0.5); 00202 // Serial Interface eth; 00203 // Serial port configuration (valeurs par defaut) : 9600 baud, 8-bit data, no parity, stop bit 00204 uart.baud(9600); 00205 uart.format(8, SerialBase::None, 1); 00206 uart.printf("Initializing\n"); 00207 wait(1.0); 00208 // Check File System 00209 uart.printf("Checking File System\n"); 00210 DIR *d = opendir("/sd/"); 00211 if(d != NULL) 00212 { 00213 uart.printf("SD Card Present\n"); 00214 } 00215 else 00216 { 00217 uart.printf("SD Card Root Directory Not Found\n"); 00218 } 00219 wait(1.0); 00220 // EthernetInterface eth; 00221 uart.printf("Initializing Ethernet\n"); 00222 #if USE_DHCP 00223 //eth.init Use DHCP 00224 int ret = eth.init((uint8_t*)MAC); // Use DHCP for WIZnetInterface 00225 uart.printf("Connecting DHCP\n"); 00226 #else 00227 int ret = eth.init((uint8_t*)MAC,IP,MASK,GATEWAY); //IP,mask,Gateway 00228 uart.printf("Connecting (IP,mask,Gateway)\n"); 00229 #endif 00230 wait(1.0); 00231 // Check Ethernet Link-Done 00232 uart.printf("Check Ethernet Link\r\n"); 00233 00234 if(eth.link() == true) 00235 { 00236 uart.printf("- Ethernet PHY Link - Done\r\n"); 00237 //led_r = LED_ON; 00238 COLOR(_RED_); 00239 } 00240 else 00241 { 00242 uart.printf("- Ethernet PHY Link - Fail\r\n"); 00243 //led_r = LED_OFF; 00244 COLOR(_BLACK_); 00245 } 00246 wait(1.0); 00247 if(!ret) 00248 { 00249 uart.printf("Initialized, MAC: %s\r\n", eth.getMACAddress()); 00250 ret = eth.connect(); 00251 00252 if(!ret) 00253 { 00254 uart.printf("IP: %s, MASK: %s, GW: %s\r\n", 00255 eth.getIPAddress(), eth.getNetworkMask(), eth.getGateway()); 00256 // led_b = LED_ON, led_g = LED_ON; 00257 COLOR(_CYAN_); 00258 } 00259 else 00260 { 00261 uart.printf("Error ethernet.connect() - ret = %d\r\n", ret); 00262 //led_b = LED_OFF; 00263 COLOR(_BLUE_); 00264 exit(0); 00265 } 00266 } 00267 else 00268 { 00269 uart.printf("Error ethernet.init() - ret = %d\r\n", ret); 00270 //led_b = LED_OFF; 00271 COLOR(_BLACK_); 00272 exit(0); 00273 } 00274 wait(1.0); 00275 // TCPSocketServer server; 00276 server.bind(HTTPD_SERVER_PORT); 00277 server.listen(); 00278 uart.printf("Server Listening\n"); 00279 00280 while(true) 00281 { 00282 uart.printf("\nWait for new connection...\r\n"); 00283 server.accept(client); 00284 client.set_blocking(false, 1500); // Timeout after (1.5)s 00285 00286 uart.printf("Connection from: %s\r\n", client.get_address()); 00287 while(true) 00288 { 00289 //led_g = LED_ON; 00290 COLOR(_GREEN_); 00291 int n = client.receive(buffer, sizeof(buffer)); 00292 if(n <= 0) break; 00293 uart.printf("Recieved Data: %d\r\n\r\n%.*s\r\n",n,n,buffer); 00294 if(n >= 1024) 00295 { 00296 sprintf(httpHeader,"HTTP/1.1 413 Request Entity Too Large \r\nContent-Type: text\r\nConnection: Close\r\n\r\n"); 00297 client.send(httpHeader,strlen(httpHeader)); 00298 client.send(buffer,n); 00299 break; 00300 } 00301 else 00302 { 00303 buffer[n]=0; 00304 } 00305 if(!strncmp(buffer, "GET ", 4)) 00306 { 00307 uristr = buffer + 4; 00308 eou = strstr(uristr, " "); 00309 if(eou == NULL) 00310 { 00311 sprintf(httpHeader,"HTTP/1.1 400 Bad Request \r\nContent-Type: text\r\nConnection: Close\r\n\r\n"); 00312 client.send(httpHeader,strlen(httpHeader)); 00313 client.send(buffer,n); 00314 } 00315 else 00316 { 00317 *eou = 0; 00318 get_file(uristr); 00319 } 00320 } 00321 } 00322 //led_g = LED_OFF; 00323 COLOR(_BLACK_); 00324 client.close(); 00325 } 00326 }
Generated on Sun Jul 17 2022 14:11:22 by
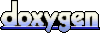