Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_spi.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_spi.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief SPI HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Serial Peripheral Interface (SPI) peripheral: 00010 * + Initialization and de-initialization functions 00011 * + IO operation functions 00012 * + Peripheral Control functions 00013 * + Peripheral State functions 00014 * 00015 @verbatim 00016 ============================================================================== 00017 ##### How to use this driver ##### 00018 ============================================================================== 00019 [..] 00020 The SPI HAL driver can be used as follows: 00021 00022 (#) Declare a SPI_HandleTypeDef handle structure, for example: 00023 SPI_HandleTypeDef hspi; 00024 00025 (#)Initialize the SPI low level resources by implementing the HAL_SPI_MspInit() API: 00026 (##) Enable the SPIx interface clock 00027 (##) SPI pins configuration 00028 (+++) Enable the clock for the SPI GPIOs 00029 (+++) Configure these SPI pins as alternate function push-pull 00030 (##) NVIC configuration if you need to use interrupt process 00031 (+++) Configure the SPIx interrupt priority 00032 (+++) Enable the NVIC SPI IRQ handle 00033 (##) DMA Configuration if you need to use DMA process 00034 (+++) Declare a DMA_HandleTypeDef handle structure for the transmit or receive channel 00035 (+++) Enable the DMAx clock 00036 (+++) Configure the DMA handle parameters 00037 (+++) Configure the DMA Tx or Rx channel 00038 (+++) Associate the initialized hdma_tx handle to the hspi DMA Tx or Rx handle 00039 (+++) Configure the priority and enable the NVIC for the transfer complete interrupt on the DMA Tx or Rx channel 00040 00041 (#) Program the Mode, BidirectionalMode , Data size, Baudrate Prescaler, NSS 00042 management, Clock polarity and phase, FirstBit and CRC configuration in the hspi Init structure. 00043 00044 (#) Initialize the SPI registers by calling the HAL_SPI_Init() API: 00045 (++) This API configures also the low level Hardware GPIO, CLOCK, CORTEX...etc) 00046 by calling the customized HAL_SPI_MspInit() API. 00047 [..] 00048 Circular mode restriction: 00049 (#) The DMA circular mode cannot be used when the SPI is configured in these modes: 00050 (##) Master 2Lines RxOnly 00051 (##) Master 1Line Rx 00052 (#) The CRC feature is not managed when the DMA circular mode is enabled 00053 (#) When the SPI DMA Pause/Stop features are used, we must use the following APIs 00054 the HAL_SPI_DMAPause()/ HAL_SPI_DMAStop() only under the SPI callbacks 00055 00056 @endverbatim 00057 ****************************************************************************** 00058 * @attention 00059 * 00060 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00061 * 00062 * Redistribution and use in source and binary forms, with or without modification, 00063 * are permitted provided that the following conditions are met: 00064 * 1. Redistributions of source code must retain the above copyright notice, 00065 * this list of conditions and the following disclaimer. 00066 * 2. Redistributions in binary form must reproduce the above copyright notice, 00067 * this list of conditions and the following disclaimer in the documentation 00068 * and/or other materials provided with the distribution. 00069 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00070 * may be used to endorse or promote products derived from this software 00071 * without specific prior written permission. 00072 * 00073 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00074 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00075 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00076 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00077 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00078 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00079 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00080 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00081 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00082 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00083 * 00084 ****************************************************************************** 00085 */ 00086 00087 /* Includes ------------------------------------------------------------------*/ 00088 #include "stm32l4xx_hal.h" 00089 00090 /** @addtogroup STM32L4xx_HAL_Driver 00091 * @{ 00092 */ 00093 00094 /** @defgroup SPI SPI 00095 * @brief SPI HAL module driver 00096 * @{ 00097 */ 00098 #ifdef HAL_SPI_MODULE_ENABLED 00099 00100 /* Private typedef -----------------------------------------------------------*/ 00101 /* Private defines -----------------------------------------------------------*/ 00102 /** @defgroup SPI_Private_Constants SPI Private Constants 00103 * @{ 00104 */ 00105 #define SPI_DEFAULT_TIMEOUT 50 00106 /** 00107 * @} 00108 */ 00109 00110 /* Private macros ------------------------------------------------------------*/ 00111 /* Private variables ---------------------------------------------------------*/ 00112 /* Private function prototypes -----------------------------------------------*/ 00113 /** @defgroup SPI_Private_Functions SPI Private Functions 00114 * @{ 00115 */ 00116 static void SPI_DMATransmitCplt(DMA_HandleTypeDef *hdma); 00117 static void SPI_DMAReceiveCplt(DMA_HandleTypeDef *hdma); 00118 static void SPI_DMATransmitReceiveCplt(DMA_HandleTypeDef *hdma); 00119 static void SPI_DMAHalfTransmitCplt(DMA_HandleTypeDef *hdma); 00120 static void SPI_DMAHalfReceiveCplt(DMA_HandleTypeDef *hdma); 00121 static void SPI_DMAHalfTransmitReceiveCplt(DMA_HandleTypeDef *hdma); 00122 static void SPI_DMAError(DMA_HandleTypeDef *hdma); 00123 static HAL_StatusTypeDef SPI_WaitFlagStateUntilTimeout(SPI_HandleTypeDef *hspi, uint32_t Flag, uint32_t State, uint32_t Timeout); 00124 static HAL_StatusTypeDef SPI_WaitFifoStateUntilTimeout(SPI_HandleTypeDef *hspi, uint32_t Fifo, uint32_t State, uint32_t Timeout); 00125 static void SPI_TxISR_8BIT(struct __SPI_HandleTypeDef *hspi); 00126 static void SPI_TxISR_16BIT(struct __SPI_HandleTypeDef *hspi); 00127 static void SPI_RxISR_8BIT(struct __SPI_HandleTypeDef *hspi); 00128 static void SPI_RxISR_8BITCRC(struct __SPI_HandleTypeDef *hspi); 00129 static void SPI_RxISR_16BIT(struct __SPI_HandleTypeDef *hspi); 00130 static void SPI_RxISR_16BITCRC(struct __SPI_HandleTypeDef *hspi); 00131 static void SPI_2linesRxISR_8BIT(struct __SPI_HandleTypeDef *hspi); 00132 static void SPI_2linesRxISR_8BITCRC(struct __SPI_HandleTypeDef *hspi); 00133 static void SPI_2linesTxISR_8BIT(struct __SPI_HandleTypeDef *hspi); 00134 static void SPI_2linesTxISR_16BIT(struct __SPI_HandleTypeDef *hspi); 00135 static void SPI_2linesRxISR_16BIT(struct __SPI_HandleTypeDef *hspi); 00136 static void SPI_2linesRxISR_16BITCRC(struct __SPI_HandleTypeDef *hspi); 00137 static void SPI_CloseRxTx_ISR(SPI_HandleTypeDef *hspi); 00138 static void SPI_CloseRx_ISR(SPI_HandleTypeDef *hspi); 00139 static void SPI_CloseTx_ISR(SPI_HandleTypeDef *hspi); 00140 static HAL_StatusTypeDef SPI_EndRxTransaction(SPI_HandleTypeDef *hspi, uint32_t Timeout); 00141 static HAL_StatusTypeDef SPI_EndRxTxTransaction(SPI_HandleTypeDef *hspi, uint32_t Timeout); 00142 /** 00143 * @} 00144 */ 00145 00146 /* Exported functions ---------------------------------------------------------*/ 00147 00148 /** @defgroup SPI_Exported_Functions SPI Exported Functions 00149 * @{ 00150 */ 00151 00152 /** @defgroup SPI_Exported_Functions_Group1 Initialization and de-initialization functions 00153 * @brief Initialization and Configuration functions 00154 * 00155 @verbatim 00156 =============================================================================== 00157 ##### Initialization and de-initialization functions ##### 00158 =============================================================================== 00159 [..] This subsection provides a set of functions allowing to initialize and 00160 de-initialize the SPIx peripheral: 00161 00162 (+) User must implement HAL_SPI_MspInit() function in which he configures 00163 all related peripherals resources (CLOCK, GPIO, DMA, IT and NVIC ). 00164 00165 (+) Call the function HAL_SPI_Init() to configure the selected device with 00166 the selected configuration: 00167 (++) Mode 00168 (++) Direction 00169 (++) Data Size 00170 (++) Clock Polarity and Phase 00171 (++) NSS Management 00172 (++) BaudRate Prescaler 00173 (++) FirstBit 00174 (++) TIMode 00175 (++) CRC Calculation 00176 (++) CRC Polynomial if CRC enabled 00177 (++) CRC Length, used only with Data8 and Data16 00178 (++) FIFO reception threshold 00179 00180 (+) Call the function HAL_SPI_DeInit() to restore the default configuration 00181 of the selected SPIx peripheral. 00182 00183 @endverbatim 00184 * @{ 00185 */ 00186 00187 /** 00188 * @brief Initialize the SPI according to the specified parameters 00189 * in the SPI_InitTypeDef and initialize the associated handle. 00190 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00191 * the configuration information for SPI module. 00192 * @retval HAL status 00193 */ 00194 HAL_StatusTypeDef HAL_SPI_Init(SPI_HandleTypeDef *hspi) 00195 { 00196 uint32_t frxth; 00197 00198 /* Check the SPI handle allocation */ 00199 if(hspi == NULL) 00200 { 00201 return HAL_ERROR; 00202 } 00203 00204 /* Check the parameters */ 00205 assert_param(IS_SPI_ALL_INSTANCE(hspi->Instance)); 00206 assert_param(IS_SPI_MODE(hspi->Init.Mode)); 00207 assert_param(IS_SPI_DIRECTION(hspi->Init.Direction)); 00208 assert_param(IS_SPI_DATASIZE(hspi->Init.DataSize)); 00209 assert_param(IS_SPI_CPOL(hspi->Init.CLKPolarity)); 00210 assert_param(IS_SPI_CPHA(hspi->Init.CLKPhase)); 00211 assert_param(IS_SPI_NSS(hspi->Init.NSS)); 00212 assert_param(IS_SPI_NSSP(hspi->Init.NSSPMode)); 00213 assert_param(IS_SPI_BAUDRATE_PRESCALER(hspi->Init.BaudRatePrescaler)); 00214 assert_param(IS_SPI_FIRST_BIT(hspi->Init.FirstBit)); 00215 assert_param(IS_SPI_TIMODE(hspi->Init.TIMode)); 00216 assert_param(IS_SPI_CRC_CALCULATION(hspi->Init.CRCCalculation)); 00217 assert_param(IS_SPI_CRC_POLYNOMIAL(hspi->Init.CRCPolynomial)); 00218 assert_param(IS_SPI_CRC_LENGTH(hspi->Init.CRCLength)); 00219 00220 if(hspi->State == HAL_SPI_STATE_RESET) 00221 { 00222 /* Allocate lock resource and initialize it */ 00223 hspi->Lock = HAL_UNLOCKED; 00224 00225 /* Init the low level hardware : GPIO, CLOCK, NVIC... */ 00226 HAL_SPI_MspInit(hspi); 00227 } 00228 00229 hspi->State = HAL_SPI_STATE_BUSY; 00230 00231 /* Disable the selected SPI peripheral */ 00232 __HAL_SPI_DISABLE(hspi); 00233 00234 /* Align by default the rs fifo threshold on the data size */ 00235 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00236 { 00237 frxth = SPI_RXFIFO_THRESHOLD_HF; 00238 } 00239 else 00240 { 00241 frxth = SPI_RXFIFO_THRESHOLD_QF; 00242 } 00243 00244 /* CRC calculation is valid only for 16Bit and 8 Bit */ 00245 if(( hspi->Init.DataSize != SPI_DATASIZE_16BIT ) && ( hspi->Init.DataSize != SPI_DATASIZE_8BIT )) 00246 { 00247 /* CRC must be disabled */ 00248 hspi->Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00249 } 00250 00251 /* Align the CRC Length on the data size */ 00252 if( hspi->Init.CRCLength == SPI_CRC_LENGTH_DATASIZE) 00253 { 00254 /* CRC Length aligned on the data size : value set by default */ 00255 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00256 { 00257 hspi->Init.CRCLength = SPI_CRC_LENGTH_16BIT; 00258 } 00259 else 00260 { 00261 hspi->Init.CRCLength = SPI_CRC_LENGTH_8BIT; 00262 } 00263 } 00264 00265 /*---------------------------- SPIx CR1 & CR2 Configuration ------------------------*/ 00266 /* Configure : SPI Mode, Communication Mode, Clock polarity and phase, NSS management, 00267 Communication speed, First bit, CRC calculation state, CRC Length */ 00268 hspi->Instance->CR1 = (hspi->Init.Mode | hspi->Init.Direction | 00269 hspi->Init.CLKPolarity | hspi->Init.CLKPhase | (hspi->Init.NSS & SPI_CR1_SSM) | 00270 hspi->Init.BaudRatePrescaler | hspi->Init.FirstBit | hspi->Init.CRCCalculation); 00271 00272 if( hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT) 00273 { 00274 hspi->Instance->CR1|= SPI_CR1_CRCL; 00275 } 00276 00277 /* Configure : NSS management */ 00278 /* Configure : Rx Fifo Threshold */ 00279 hspi->Instance->CR2 = (((hspi->Init.NSS >> 16) & SPI_CR2_SSOE) | hspi->Init.TIMode | hspi->Init.NSSPMode | 00280 hspi->Init.DataSize ) | frxth; 00281 00282 /*---------------------------- SPIx CRCPOLY Configuration --------------------*/ 00283 /* Configure : CRC Polynomial */ 00284 hspi->Instance->CRCPR = hspi->Init.CRCPolynomial; 00285 00286 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00287 hspi->State= HAL_SPI_STATE_READY; 00288 00289 return HAL_OK; 00290 } 00291 00292 /** 00293 * @brief DeInitialize the SPI peripheral. 00294 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00295 * the configuration information for SPI module. 00296 * @retval HAL status 00297 */ 00298 HAL_StatusTypeDef HAL_SPI_DeInit(SPI_HandleTypeDef *hspi) 00299 { 00300 /* Check the SPI handle allocation */ 00301 if(hspi == NULL) 00302 { 00303 return HAL_ERROR; 00304 } 00305 00306 /* Check the parameters */ 00307 assert_param(IS_SPI_ALL_INSTANCE(hspi->Instance)); 00308 hspi->State = HAL_SPI_STATE_BUSY; 00309 00310 /* Disable the SPI Peripheral Clock */ 00311 __HAL_SPI_DISABLE(hspi); 00312 00313 /* DeInit the low level hardware: GPIO, CLOCK, NVIC... */ 00314 HAL_SPI_MspDeInit(hspi); 00315 00316 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00317 hspi->State = HAL_SPI_STATE_RESET; 00318 00319 __HAL_UNLOCK(hspi); 00320 00321 return HAL_OK; 00322 } 00323 00324 /** 00325 * @brief Initialize the SPI MSP. 00326 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00327 * the configuration information for SPI module. 00328 * @retval None 00329 */ 00330 __weak void HAL_SPI_MspInit(SPI_HandleTypeDef *hspi) 00331 { 00332 /* NOTE : This function should not be modified, when the callback is needed, 00333 the HAL_SPI_MspInit should be implemented in the user file 00334 */ 00335 } 00336 00337 /** 00338 * @brief DeInitialize the SPI MSP. 00339 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00340 * the configuration information for SPI module. 00341 * @retval None 00342 */ 00343 __weak void HAL_SPI_MspDeInit(SPI_HandleTypeDef *hspi) 00344 { 00345 /* NOTE : This function should not be modified, when the callback is needed, 00346 the HAL_SPI_MspDeInit should be implemented in the user file 00347 */ 00348 } 00349 00350 /** 00351 * @} 00352 */ 00353 00354 /** @defgroup SPI_Exported_Functions_Group2 IO operation functions 00355 * @brief Data transfers functions 00356 * 00357 @verbatim 00358 ============================================================================== 00359 ##### IO operation functions ##### 00360 =============================================================================== 00361 [..] 00362 This subsection provides a set of functions allowing to manage the SPI 00363 data transfers. 00364 00365 [..] The SPI supports master and slave mode : 00366 00367 (#) There are two modes of transfer: 00368 (++) Blocking mode: The communication is performed in polling mode. 00369 The HAL status of all data processing is returned by the same function 00370 after finishing transfer. 00371 (++) No-Blocking mode: The communication is performed using Interrupts 00372 or DMA, These APIs return the HAL status. 00373 The end of the data processing will be indicated through the 00374 dedicated SPI IRQ when using Interrupt mode or the DMA IRQ when 00375 using DMA mode. 00376 The HAL_SPI_TxCpltCallback(), HAL_SPI_RxCpltCallback() and HAL_SPI_TxRxCpltCallback() user callbacks 00377 will be executed respectively at the end of the transmit or Receive process 00378 The HAL_SPI_ErrorCallback()user callback will be executed when a communication error is detected 00379 00380 (#) APIs provided for these 2 transfer modes (Blocking mode or Non blocking mode using either Interrupt or DMA) 00381 exist for 1Line (simplex) and 2Lines (full duplex) modes. 00382 00383 @endverbatim 00384 * @{ 00385 */ 00386 00387 /** 00388 * @brief Transmit an amount of data in blocking mode. 00389 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00390 * the configuration information for SPI module. 00391 * @param pData: pointer to data buffer 00392 * @param Size: amount of data to be sent 00393 * @param Timeout: Timeout duration 00394 * @retval HAL status 00395 */ 00396 HAL_StatusTypeDef HAL_SPI_Transmit(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00397 { 00398 uint32_t tickstart = HAL_GetTick(); 00399 HAL_StatusTypeDef errorcode = HAL_OK; 00400 00401 assert_param(IS_SPI_DIRECTION_2LINES_OR_1LINE(hspi->Init.Direction)); 00402 00403 /* Process Locked */ 00404 __HAL_LOCK(hspi); 00405 00406 if(hspi->State != HAL_SPI_STATE_READY) 00407 { 00408 errorcode = HAL_BUSY; 00409 goto error; 00410 } 00411 00412 if((pData == NULL ) || (Size == 0)) 00413 { 00414 errorcode = HAL_ERROR; 00415 goto error; 00416 } 00417 00418 /* Set the transaction information */ 00419 hspi->State = HAL_SPI_STATE_BUSY_TX; 00420 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00421 hspi->pTxBuffPtr = pData; 00422 hspi->TxXferSize = Size; 00423 hspi->TxXferCount = Size; 00424 hspi->pRxBuffPtr = (uint8_t *)NULL; 00425 hspi->RxXferSize = 0; 00426 hspi->RxXferCount = 0; 00427 00428 /* Configure communication direction : 1Line */ 00429 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 00430 { 00431 SPI_1LINE_TX(hspi); 00432 } 00433 00434 /* Reset CRC Calculation */ 00435 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00436 { 00437 SPI_RESET_CRC(hspi); 00438 } 00439 00440 /* Check if the SPI is already enabled */ 00441 if((hspi->Instance->CR1 & SPI_CR1_SPE) != SPI_CR1_SPE) 00442 { 00443 /* Enable SPI peripheral */ 00444 __HAL_SPI_ENABLE(hspi); 00445 } 00446 00447 /* Transmit data in 16 Bit mode */ 00448 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00449 { 00450 /* Transmit data in 16 Bit mode */ 00451 while (hspi->TxXferCount > 0) 00452 { 00453 /* Wait until TXE flag is set to send data */ 00454 if((hspi->Instance->SR & SPI_FLAG_TXE) == SPI_FLAG_TXE) 00455 { 00456 hspi->Instance->DR = *((uint16_t *)hspi->pTxBuffPtr); 00457 hspi->pTxBuffPtr += sizeof(uint16_t); 00458 hspi->TxXferCount--; 00459 } 00460 else 00461 { 00462 /* Timeout management */ 00463 if((Timeout == 0) || ((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout))) 00464 { 00465 errorcode = HAL_TIMEOUT; 00466 goto error; 00467 } 00468 } 00469 } 00470 } 00471 /* Transmit data in 8 Bit mode */ 00472 else 00473 { 00474 while (hspi->TxXferCount > 0) 00475 { 00476 /* Wait until TXE flag is set to send data */ 00477 if((hspi->Instance->SR & SPI_FLAG_TXE) == SPI_FLAG_TXE) 00478 { 00479 if(hspi->TxXferCount > 1) 00480 { 00481 /* write on the data register in packing mode */ 00482 hspi->Instance->DR = *((uint16_t*)hspi->pTxBuffPtr); 00483 hspi->pTxBuffPtr += sizeof(uint16_t); 00484 hspi->TxXferCount -= 2; 00485 } 00486 else 00487 { 00488 *((__IO uint8_t*)&hspi->Instance->DR) = (*hspi->pTxBuffPtr++); 00489 hspi->TxXferCount--; 00490 } 00491 } 00492 else 00493 { 00494 /* Timeout management */ 00495 if((Timeout == 0) || ((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout))) 00496 { 00497 errorcode = HAL_TIMEOUT; 00498 goto error; 00499 } 00500 } 00501 } 00502 } 00503 00504 /* Enable CRC Transmission */ 00505 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00506 { 00507 hspi->Instance->CR1|= SPI_CR1_CRCNEXT; 00508 } 00509 00510 /* Check the end of the transaction */ 00511 if(SPI_EndRxTxTransaction(hspi,Timeout) != HAL_OK) 00512 { 00513 hspi->ErrorCode = HAL_SPI_ERROR_FLAG; 00514 } 00515 00516 /* Clear overrun flag in 2 Lines communication mode because received is not read */ 00517 if(hspi->Init.Direction == SPI_DIRECTION_2LINES) 00518 { 00519 __HAL_SPI_CLEAR_OVRFLAG(hspi); 00520 } 00521 00522 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 00523 { 00524 errorcode = HAL_ERROR; 00525 } 00526 00527 error: 00528 hspi->State = HAL_SPI_STATE_READY; 00529 /* Process Unlocked */ 00530 __HAL_UNLOCK(hspi); 00531 return errorcode; 00532 } 00533 00534 /** 00535 * @brief Receive an amount of data in blocking mode. 00536 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00537 * the configuration information for SPI module. 00538 * @param pData: pointer to data buffer 00539 * @param Size: amount of data to be received 00540 * @param Timeout: Timeout duration 00541 * @retval HAL status 00542 */ 00543 HAL_StatusTypeDef HAL_SPI_Receive(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00544 { 00545 __IO uint16_t tmpreg; 00546 uint32_t tickstart = HAL_GetTick(); 00547 HAL_StatusTypeDef errorcode = HAL_OK; 00548 00549 if((hspi->Init.Mode == SPI_MODE_MASTER) && (hspi->Init.Direction == SPI_DIRECTION_2LINES)) 00550 { 00551 /* the receive process is not supported in 2Lines direction master mode */ 00552 /* in this case we call the TransmitReceive process */ 00553 /* Process Locked */ 00554 return HAL_SPI_TransmitReceive(hspi,pData,pData,Size,Timeout); 00555 } 00556 00557 /* Process Locked */ 00558 __HAL_LOCK(hspi); 00559 00560 if(hspi->State != HAL_SPI_STATE_READY) 00561 { 00562 errorcode = HAL_BUSY; 00563 goto error; 00564 } 00565 00566 if((pData == NULL ) || (Size == 0)) 00567 { 00568 errorcode = HAL_ERROR; 00569 goto error; 00570 } 00571 00572 hspi->State = HAL_SPI_STATE_BUSY_RX; 00573 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00574 hspi->pRxBuffPtr = pData; 00575 hspi->RxXferSize = Size; 00576 hspi->RxXferCount = Size; 00577 hspi->pTxBuffPtr = (uint8_t *)NULL; 00578 hspi->TxXferSize = 0; 00579 hspi->TxXferCount = 0; 00580 00581 /* Reset CRC Calculation */ 00582 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00583 { 00584 SPI_RESET_CRC(hspi); 00585 /* this is done to handle the CRCNEXT before the latest data */ 00586 hspi->RxXferCount--; 00587 } 00588 00589 /* Set the Rx Fido threshold */ 00590 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00591 { 00592 /* set fiforxthresold according the reception data length: 16bit */ 00593 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 00594 } 00595 else 00596 { 00597 /* set fiforxthresold according the reception data length: 8bit */ 00598 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 00599 } 00600 00601 /* Configure communication direction 1Line and enabled SPI if needed */ 00602 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 00603 { 00604 SPI_1LINE_RX(hspi); 00605 } 00606 00607 /* Check if the SPI is already enabled */ 00608 if((hspi->Instance->CR1 & SPI_CR1_SPE) != SPI_CR1_SPE) 00609 { 00610 /* Enable SPI peripheral */ 00611 __HAL_SPI_ENABLE(hspi); 00612 } 00613 00614 if(hspi->Init.DataSize <= SPI_DATASIZE_8BIT) 00615 { 00616 /* Transfer loop */ 00617 while(hspi->RxXferCount > 0) 00618 { 00619 /* Check the RXNE flag */ 00620 if((hspi->Instance->SR & SPI_FLAG_RXNE) == SPI_FLAG_RXNE) 00621 { 00622 /* read the received data */ 00623 (*hspi->pRxBuffPtr++)= *(__IO uint8_t *)&hspi->Instance->DR; 00624 hspi->RxXferCount--; 00625 } 00626 else 00627 { 00628 /* Timeout management */ 00629 if((Timeout == 0) || ((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout))) 00630 { 00631 errorcode = HAL_TIMEOUT; 00632 goto error; 00633 } 00634 } 00635 } 00636 } 00637 else 00638 { 00639 /* Transfer loop */ 00640 while(hspi->RxXferCount > 0) 00641 { 00642 /* Check the RXNE flag */ 00643 if((hspi->Instance->SR & SPI_FLAG_RXNE) == SPI_FLAG_RXNE) 00644 { 00645 *((uint16_t*)hspi->pRxBuffPtr) = hspi->Instance->DR; 00646 hspi->pRxBuffPtr += sizeof(uint16_t); 00647 hspi->RxXferCount--; 00648 } 00649 else 00650 { 00651 /* Timeout management */ 00652 if((Timeout == 0) || ((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout))) 00653 { 00654 errorcode = HAL_TIMEOUT; 00655 goto error; 00656 } 00657 } 00658 } 00659 } 00660 00661 /* Handle the CRC Transmission */ 00662 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00663 { 00664 /* freeze the CRC before the latest data */ 00665 hspi->Instance->CR1|= SPI_CR1_CRCNEXT; 00666 00667 /* Read the latest data */ 00668 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, Timeout) != HAL_OK) 00669 { 00670 /* the latest data has not been received */ 00671 errorcode = HAL_TIMEOUT; 00672 goto error; 00673 } 00674 00675 /* Receive last data in 16 Bit mode */ 00676 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00677 { 00678 *((uint16_t*)hspi->pRxBuffPtr) = hspi->Instance->DR; 00679 } 00680 /* Receive last data in 8 Bit mode */ 00681 else 00682 { 00683 *hspi->pRxBuffPtr = *(__IO uint8_t *)&hspi->Instance->DR; 00684 } 00685 00686 /* Wait until TXE flag */ 00687 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, Timeout) != HAL_OK) 00688 { 00689 /* Flag Error*/ 00690 hspi->ErrorCode = HAL_SPI_ERROR_CRC; 00691 errorcode = HAL_TIMEOUT; 00692 goto error; 00693 } 00694 00695 if(hspi->Init.DataSize == SPI_DATASIZE_16BIT) 00696 { 00697 tmpreg = hspi->Instance->DR; 00698 UNUSED(tmpreg); /* To avoid GCC warning */ 00699 } 00700 else 00701 { 00702 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 00703 UNUSED(tmpreg); /* To avoid GCC warning */ 00704 00705 if((hspi->Init.DataSize == SPI_DATASIZE_8BIT) && (hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT)) 00706 { 00707 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, Timeout) != HAL_OK) 00708 { 00709 /* Error on the CRC reception */ 00710 hspi->ErrorCode = HAL_SPI_ERROR_CRC; 00711 errorcode = HAL_TIMEOUT; 00712 goto error; 00713 } 00714 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 00715 UNUSED(tmpreg); /* To avoid GCC warning */ 00716 } 00717 } 00718 } 00719 00720 /* Check the end of the transaction */ 00721 if(SPI_EndRxTransaction(hspi,Timeout) != HAL_OK) 00722 { 00723 hspi->ErrorCode = HAL_SPI_ERROR_FLAG; 00724 } 00725 00726 /* Check if CRC error occurred */ 00727 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 00728 { 00729 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 00730 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 00731 } 00732 00733 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 00734 { 00735 errorcode = HAL_ERROR; 00736 } 00737 00738 error : 00739 hspi->State = HAL_SPI_STATE_READY; 00740 __HAL_UNLOCK(hspi); 00741 return errorcode; 00742 } 00743 00744 /** 00745 * @brief Transmit and Receive an amount of data in blocking mode. 00746 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00747 * the configuration information for SPI module. 00748 * @param pTxData: pointer to transmission data buffer 00749 * @param pRxData: pointer to reception data buffer 00750 * @param Size: amount of data to be sent and received 00751 * @param Timeout: Timeout duration 00752 * @retval HAL status 00753 */ 00754 HAL_StatusTypeDef HAL_SPI_TransmitReceive(SPI_HandleTypeDef *hspi, uint8_t *pTxData, uint8_t *pRxData, uint16_t Size, uint32_t Timeout) 00755 { 00756 __IO uint16_t tmpreg; 00757 uint32_t tickstart = HAL_GetTick(); 00758 HAL_StatusTypeDef errorcode = HAL_OK; 00759 00760 assert_param(IS_SPI_DIRECTION_2LINES(hspi->Init.Direction)); 00761 00762 /* Process Locked */ 00763 __HAL_LOCK(hspi); 00764 00765 if(hspi->State != HAL_SPI_STATE_READY) 00766 { 00767 errorcode = HAL_BUSY; 00768 goto error; 00769 } 00770 00771 if((pTxData == NULL) || (pRxData == NULL) || (Size == 0)) 00772 { 00773 errorcode = HAL_ERROR; 00774 goto error; 00775 } 00776 00777 hspi->State = HAL_SPI_STATE_BUSY_TX_RX; 00778 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00779 hspi->pRxBuffPtr = pRxData; 00780 hspi->RxXferCount = Size; 00781 hspi->RxXferSize = Size; 00782 hspi->pTxBuffPtr = pTxData; 00783 hspi->TxXferCount = Size; 00784 hspi->TxXferSize = Size; 00785 00786 /* Reset CRC Calculation */ 00787 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00788 { 00789 SPI_RESET_CRC(hspi); 00790 } 00791 00792 /* Set the Rx Fido threshold */ 00793 if((hspi->Init.DataSize > SPI_DATASIZE_8BIT) || (hspi->RxXferCount > 1)) 00794 { 00795 /* set fiforxthreshold according the reception data length: 16bit */ 00796 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 00797 } 00798 else 00799 { 00800 /* set fiforxthreshold according the reception data length: 8bit */ 00801 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 00802 } 00803 00804 /* Check if the SPI is already enabled */ 00805 if((hspi->Instance->CR1 &SPI_CR1_SPE) != SPI_CR1_SPE) 00806 { 00807 /* Enable SPI peripheral */ 00808 __HAL_SPI_ENABLE(hspi); 00809 } 00810 00811 /* Transmit and Receive data in 16 Bit mode */ 00812 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 00813 { 00814 while ((hspi->TxXferCount > 0 ) || (hspi->RxXferCount > 0)) 00815 { 00816 /* Check TXE flag */ 00817 if((hspi->TxXferCount > 0) && ((hspi->Instance->SR & SPI_FLAG_TXE) == SPI_FLAG_TXE)) 00818 { 00819 hspi->Instance->DR = *((uint16_t *)hspi->pTxBuffPtr); 00820 hspi->pTxBuffPtr += sizeof(uint16_t); 00821 hspi->TxXferCount--; 00822 00823 /* Enable CRC Transmission */ 00824 if((hspi->TxXferCount == 0) && (hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE)) 00825 { 00826 hspi->Instance->CR1|= SPI_CR1_CRCNEXT; 00827 } 00828 } 00829 00830 /* Check RXNE flag */ 00831 if((hspi->RxXferCount > 0) && ((hspi->Instance->SR & SPI_FLAG_RXNE) == SPI_FLAG_RXNE)) 00832 { 00833 *((uint16_t *)hspi->pRxBuffPtr) = hspi->Instance->DR; 00834 hspi->pRxBuffPtr += sizeof(uint16_t); 00835 hspi->RxXferCount--; 00836 } 00837 if((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout)) 00838 { 00839 errorcode = HAL_TIMEOUT; 00840 goto error; 00841 } 00842 } 00843 } 00844 /* Transmit and Receive data in 8 Bit mode */ 00845 else 00846 { 00847 while((hspi->TxXferCount > 0) || (hspi->RxXferCount > 0)) 00848 { 00849 /* check TXE flag */ 00850 if((hspi->TxXferCount > 0) && ((hspi->Instance->SR & SPI_FLAG_TXE) == SPI_FLAG_TXE)) 00851 { 00852 if(hspi->TxXferCount > 1) 00853 { 00854 hspi->Instance->DR = *((uint16_t*)hspi->pTxBuffPtr); 00855 hspi->pTxBuffPtr += sizeof(uint16_t); 00856 hspi->TxXferCount -= 2; 00857 } 00858 else 00859 { 00860 *(__IO uint8_t *)&hspi->Instance->DR = (*hspi->pTxBuffPtr++); 00861 hspi->TxXferCount--; 00862 } 00863 00864 /* Enable CRC Transmission */ 00865 if((hspi->TxXferCount == 0) && (hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE)) 00866 { 00867 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 00868 } 00869 } 00870 00871 /* Wait until RXNE flag is reset */ 00872 if((hspi->RxXferCount > 0) && ((hspi->Instance->SR & SPI_FLAG_RXNE) == SPI_FLAG_RXNE)) 00873 { 00874 if(hspi->RxXferCount > 1) 00875 { 00876 *((uint16_t*)hspi->pRxBuffPtr) = hspi->Instance->DR; 00877 hspi->pRxBuffPtr += sizeof(uint16_t); 00878 hspi->RxXferCount -= 2; 00879 if(hspi->RxXferCount <= 1) 00880 { 00881 /* set fiforxthresold before to switch on 8 bit data size */ 00882 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 00883 } 00884 } 00885 else 00886 { 00887 (*hspi->pRxBuffPtr++) = *(__IO uint8_t *)&hspi->Instance->DR; 00888 hspi->RxXferCount--; 00889 } 00890 } 00891 if((Timeout != HAL_MAX_DELAY) && ((HAL_GetTick()-tickstart) >= Timeout)) 00892 { 00893 errorcode = HAL_TIMEOUT; 00894 goto error; 00895 } 00896 } 00897 } 00898 00899 /* Read CRC from DR to close CRC calculation process */ 00900 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 00901 { 00902 /* Wait until TXE flag */ 00903 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, Timeout) != HAL_OK) 00904 { 00905 /* Error on the CRC reception */ 00906 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 00907 errorcode = HAL_TIMEOUT; 00908 goto error; 00909 } 00910 00911 if(hspi->Init.DataSize == SPI_DATASIZE_16BIT) 00912 { 00913 tmpreg = hspi->Instance->DR; 00914 UNUSED(tmpreg); /* To avoid GCC warning */ 00915 } 00916 else 00917 { 00918 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 00919 UNUSED(tmpreg); /* To avoid GCC warning */ 00920 00921 if(hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT) 00922 { 00923 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, Timeout) != HAL_OK) 00924 { 00925 /* Error on the CRC reception */ 00926 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 00927 errorcode = HAL_TIMEOUT; 00928 goto error; 00929 } 00930 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 00931 UNUSED(tmpreg); /* To avoid GCC warning */ 00932 } 00933 } 00934 } 00935 00936 /* Check if CRC error occurred */ 00937 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 00938 { 00939 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 00940 /* Clear CRC Flag */ 00941 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 00942 00943 errorcode = HAL_ERROR; 00944 } 00945 00946 /* Check the end of the transaction */ 00947 if(SPI_EndRxTxTransaction(hspi,Timeout) != HAL_OK) 00948 { 00949 hspi->ErrorCode = HAL_SPI_ERROR_FLAG; 00950 } 00951 00952 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 00953 { 00954 errorcode = HAL_ERROR; 00955 } 00956 00957 error : 00958 hspi->State = HAL_SPI_STATE_READY; 00959 __HAL_UNLOCK(hspi); 00960 return errorcode; 00961 } 00962 00963 /** 00964 * @brief Transmit an amount of data in non-blocking mode with Interrupt. 00965 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 00966 * the configuration information for SPI module. 00967 * @param pData: pointer to data buffer 00968 * @param Size: amount of data to be sent 00969 * @retval HAL status 00970 */ 00971 HAL_StatusTypeDef HAL_SPI_Transmit_IT(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size) 00972 { 00973 HAL_StatusTypeDef errorcode = HAL_OK; 00974 assert_param(IS_SPI_DIRECTION_2LINES_OR_1LINE(hspi->Init.Direction)); 00975 00976 /* Process Locked */ 00977 __HAL_LOCK(hspi); 00978 00979 if((pData == NULL) || (Size == 0)) 00980 { 00981 errorcode = HAL_ERROR; 00982 goto error; 00983 } 00984 00985 if(hspi->State != HAL_SPI_STATE_READY) 00986 { 00987 errorcode = HAL_BUSY; 00988 goto error; 00989 } 00990 00991 /* prepare the transfer */ 00992 hspi->State = HAL_SPI_STATE_BUSY_TX; 00993 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 00994 hspi->pTxBuffPtr = pData; 00995 hspi->TxXferSize = Size; 00996 hspi->TxXferCount = Size; 00997 hspi->pRxBuffPtr = (uint8_t *)NULL; 00998 hspi->RxXferSize = 0; 00999 hspi->RxXferCount = 0; 01000 hspi->RxISR = NULL; 01001 01002 /* Set the function for IT treatment */ 01003 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT ) 01004 { 01005 hspi->TxISR = SPI_TxISR_16BIT; 01006 } 01007 else 01008 { 01009 hspi->TxISR = SPI_TxISR_8BIT; 01010 } 01011 01012 /* Configure communication direction : 1Line */ 01013 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 01014 { 01015 SPI_1LINE_TX(hspi); 01016 } 01017 01018 /* Reset CRC Calculation */ 01019 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01020 { 01021 SPI_RESET_CRC(hspi); 01022 } 01023 01024 /* Enable TXE and ERR interrupt */ 01025 __HAL_SPI_ENABLE_IT(hspi,(SPI_IT_TXE)); 01026 01027 01028 /* Check if the SPI is already enabled */ 01029 if((hspi->Instance->CR1 &SPI_CR1_SPE) != SPI_CR1_SPE) 01030 { 01031 /* Enable SPI peripheral */ 01032 __HAL_SPI_ENABLE(hspi); 01033 } 01034 01035 error : 01036 __HAL_UNLOCK(hspi); 01037 return errorcode; 01038 } 01039 01040 /** 01041 * @brief Receive an amount of data in non-blocking mode with Interrupt. 01042 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01043 * the configuration information for SPI module. 01044 * @param pData: pointer to data buffer 01045 * @param Size: amount of data to be sent 01046 * @retval HAL status 01047 */ 01048 HAL_StatusTypeDef HAL_SPI_Receive_IT(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size) 01049 { 01050 HAL_StatusTypeDef errorcode = HAL_OK; 01051 01052 /* Process Locked */ 01053 __HAL_LOCK(hspi); 01054 01055 if(hspi->State != HAL_SPI_STATE_READY) 01056 { 01057 errorcode = HAL_BUSY; 01058 goto error; 01059 } 01060 if((pData == NULL) || (Size == 0)) 01061 { 01062 errorcode = HAL_ERROR; 01063 goto error; 01064 } 01065 01066 /* Configure communication */ 01067 hspi->State = HAL_SPI_STATE_BUSY_RX; 01068 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 01069 hspi->pRxBuffPtr = pData; 01070 hspi->RxXferSize = Size; 01071 hspi->RxXferCount = Size; 01072 hspi->pTxBuffPtr = (uint8_t *)NULL; 01073 hspi->TxXferSize = 0; 01074 hspi->TxXferCount = 0; 01075 01076 if((hspi->Init.Mode == SPI_MODE_MASTER) && (hspi->Init.Direction == SPI_DIRECTION_2LINES)) 01077 { 01078 /* Process Unlocked */ 01079 __HAL_UNLOCK(hspi); 01080 /* the receive process is not supported in 2Lines direction master mode */ 01081 /* in this we call the TransmitReceive process */ 01082 return HAL_SPI_TransmitReceive_IT(hspi,pData,pData,Size); 01083 } 01084 01085 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01086 { 01087 hspi->CRCSize = 1; 01088 if((hspi->Init.DataSize <= SPI_DATASIZE_8BIT) && (hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT)) 01089 { 01090 hspi->CRCSize = 2; 01091 } 01092 } 01093 else 01094 { 01095 hspi->CRCSize = 0; 01096 } 01097 01098 hspi->TxISR = NULL; 01099 /* check the data size to adapt Rx threshold and the set the function for IT treatment */ 01100 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT ) 01101 { 01102 /* set fiforxthresold according the reception data length: 16 bit */ 01103 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01104 hspi->RxISR = SPI_RxISR_16BIT; 01105 } 01106 else 01107 { 01108 /* set fiforxthresold according the reception data length: 8 bit */ 01109 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01110 hspi->RxISR = SPI_RxISR_8BIT; 01111 } 01112 01113 /* Configure communication direction : 1Line */ 01114 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 01115 { 01116 SPI_1LINE_RX(hspi); 01117 } 01118 01119 /* Reset CRC Calculation */ 01120 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01121 { 01122 SPI_RESET_CRC(hspi); 01123 } 01124 01125 /* Enable TXE and ERR interrupt */ 01126 __HAL_SPI_ENABLE_IT(hspi, (SPI_IT_RXNE | SPI_IT_ERR)); 01127 01128 /* Check if the SPI is already enabled */ 01129 if((hspi->Instance->CR1 & SPI_CR1_SPE) != SPI_CR1_SPE) 01130 { 01131 /* Enable SPI peripheral */ 01132 __HAL_SPI_ENABLE(hspi); 01133 } 01134 01135 error : 01136 /* Process Unlocked */ 01137 __HAL_UNLOCK(hspi); 01138 return errorcode; 01139 } 01140 01141 /** 01142 * @brief Transmit and Receive an amount of data in non-blocking mode with Interrupt. 01143 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01144 * the configuration information for SPI module. 01145 * @param pTxData: pointer to transmission data buffer 01146 * @param pRxData: pointer to reception data buffer 01147 * @param Size: amount of data to be sent and received 01148 * @retval HAL status 01149 */ 01150 HAL_StatusTypeDef HAL_SPI_TransmitReceive_IT(SPI_HandleTypeDef *hspi, uint8_t *pTxData, uint8_t *pRxData, uint16_t Size) 01151 { 01152 HAL_StatusTypeDef errorcode = HAL_OK; 01153 assert_param(IS_SPI_DIRECTION_2LINES(hspi->Init.Direction)); 01154 01155 /* Process locked */ 01156 __HAL_LOCK(hspi); 01157 01158 if(!((hspi->State == HAL_SPI_STATE_READY) || \ 01159 ((hspi->Init.Mode == SPI_MODE_MASTER) && (hspi->Init.Direction == SPI_DIRECTION_2LINES) && (hspi->State == HAL_SPI_STATE_BUSY_RX)))) 01160 { 01161 errorcode = HAL_BUSY; 01162 goto error; 01163 } 01164 01165 if((pTxData == NULL ) || (pRxData == NULL ) || (Size == 0)) 01166 { 01167 errorcode = HAL_ERROR; 01168 goto error; 01169 } 01170 01171 hspi->CRCSize = 0; 01172 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01173 { 01174 hspi->CRCSize = 1; 01175 if((hspi->Init.DataSize <= SPI_DATASIZE_8BIT) && (hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT)) 01176 { 01177 hspi->CRCSize = 2; 01178 } 01179 } 01180 01181 if(hspi->State != HAL_SPI_STATE_BUSY_RX) 01182 { 01183 hspi->State = HAL_SPI_STATE_BUSY_TX_RX; 01184 } 01185 01186 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 01187 hspi->pTxBuffPtr = pTxData; 01188 hspi->TxXferSize = Size; 01189 hspi->TxXferCount = Size; 01190 hspi->pRxBuffPtr = pRxData; 01191 hspi->RxXferSize = Size; 01192 hspi->RxXferCount = Size; 01193 01194 /* Set the function for IT treatment */ 01195 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT ) 01196 { 01197 hspi->RxISR = SPI_2linesRxISR_16BIT; 01198 hspi->TxISR = SPI_2linesTxISR_16BIT; 01199 } 01200 else 01201 { 01202 hspi->RxISR = SPI_2linesRxISR_8BIT; 01203 hspi->TxISR = SPI_2linesTxISR_8BIT; 01204 } 01205 01206 /* Reset CRC Calculation */ 01207 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01208 { 01209 SPI_RESET_CRC(hspi); 01210 } 01211 01212 /* check if packing mode is enabled and if there is more than 2 data to receive */ 01213 if((hspi->Init.DataSize > SPI_DATASIZE_8BIT) || (hspi->RxXferCount >= 2)) 01214 { 01215 /* set fiforxthresold according the reception data length: 16 bit */ 01216 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01217 } 01218 else 01219 { 01220 /* set fiforxthresold according the reception data length: 8 bit */ 01221 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01222 } 01223 01224 /* Enable TXE, RXNE and ERR interrupt */ 01225 __HAL_SPI_ENABLE_IT(hspi, (SPI_IT_TXE | SPI_IT_RXNE | SPI_IT_ERR)); 01226 01227 /* Check if the SPI is already enabled */ 01228 if((hspi->Instance->CR1 & SPI_CR1_SPE) != SPI_CR1_SPE) 01229 { 01230 /* Enable SPI peripheral */ 01231 __HAL_SPI_ENABLE(hspi); 01232 } 01233 01234 error : 01235 /* Process Unlocked */ 01236 __HAL_UNLOCK(hspi); 01237 return errorcode; 01238 } 01239 01240 /** 01241 * @brief Transmit an amount of data in non-blocking mode with DMA. 01242 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01243 * the configuration information for SPI module. 01244 * @param pData: pointer to data buffer 01245 * @param Size: amount of data to be sent 01246 * @retval HAL status 01247 */ 01248 HAL_StatusTypeDef HAL_SPI_Transmit_DMA(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size) 01249 { 01250 HAL_StatusTypeDef errorcode = HAL_OK; 01251 assert_param(IS_SPI_DIRECTION_2LINES_OR_1LINE(hspi->Init.Direction)); 01252 01253 /* Process Locked */ 01254 __HAL_LOCK(hspi); 01255 01256 if(hspi->State != HAL_SPI_STATE_READY) 01257 { 01258 errorcode = HAL_BUSY; 01259 goto error; 01260 } 01261 01262 if((pData == NULL) || (Size == 0)) 01263 { 01264 errorcode = HAL_ERROR; 01265 goto error; 01266 } 01267 01268 hspi->State = HAL_SPI_STATE_BUSY_TX; 01269 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 01270 hspi->pTxBuffPtr = pData; 01271 hspi->TxXferSize = Size; 01272 hspi->TxXferCount = Size; 01273 hspi->pRxBuffPtr = (uint8_t *)NULL; 01274 hspi->RxXferSize = 0; 01275 hspi->RxXferCount = 0; 01276 01277 /* Configure communication direction : 1Line */ 01278 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 01279 { 01280 SPI_1LINE_TX(hspi); 01281 } 01282 01283 /* Reset CRC Calculation */ 01284 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01285 { 01286 SPI_RESET_CRC(hspi); 01287 } 01288 01289 /* Set the SPI TxDMA Half transfer complete callback */ 01290 hspi->hdmatx->XferHalfCpltCallback = SPI_DMAHalfTransmitCplt; 01291 01292 /* Set the SPI TxDMA transfer complete callback */ 01293 hspi->hdmatx->XferCpltCallback = SPI_DMATransmitCplt; 01294 01295 /* Set the DMA error callback */ 01296 hspi->hdmatx->XferErrorCallback = SPI_DMAError; 01297 01298 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX); 01299 /* packing mode is enabled only if the DMA setting is HALWORD */ 01300 if((hspi->Init.DataSize <= SPI_DATASIZE_8BIT) && (hspi->hdmatx->Init.MemDataAlignment == DMA_MDATAALIGN_HALFWORD)) 01301 { 01302 /* Check the even/odd of the data size + crc if enabled */ 01303 if((hspi->TxXferCount & 0x1) == 0) 01304 { 01305 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX); 01306 hspi->TxXferCount = (hspi->TxXferCount >> 1); 01307 } 01308 else 01309 { 01310 SET_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX); 01311 hspi->TxXferCount = (hspi->TxXferCount >> 1) + 1; 01312 } 01313 } 01314 01315 /* Enable the Tx DMA channel */ 01316 HAL_DMA_Start_IT(hspi->hdmatx, (uint32_t)hspi->pTxBuffPtr, (uint32_t)&hspi->Instance->DR, hspi->TxXferCount); 01317 01318 /* Check if the SPI is already enabled */ 01319 if((hspi->Instance->CR1 &SPI_CR1_SPE) != SPI_CR1_SPE) 01320 { 01321 /* Enable SPI peripheral */ 01322 __HAL_SPI_ENABLE(hspi); 01323 } 01324 01325 /* Enable Tx DMA Request */ 01326 SET_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN); 01327 01328 error : 01329 /* Process Unlocked */ 01330 __HAL_UNLOCK(hspi); 01331 return errorcode; 01332 } 01333 01334 /** 01335 * @brief Receive an amount of data in non-blocking mode with DMA. 01336 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01337 * the configuration information for SPI module. 01338 * @param pData: pointer to data buffer 01339 * @note When the CRC feature is enabled the pData Length must be Size + 1. 01340 * @param Size: amount of data to be sent 01341 * @retval HAL status 01342 */ 01343 HAL_StatusTypeDef HAL_SPI_Receive_DMA(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size) 01344 { 01345 HAL_StatusTypeDef errorcode = HAL_OK; 01346 01347 /* Process Locked */ 01348 __HAL_LOCK(hspi); 01349 01350 if(hspi->State != HAL_SPI_STATE_READY) 01351 { 01352 errorcode = HAL_BUSY; 01353 goto error; 01354 } 01355 01356 if((pData == NULL) || (Size == 0)) 01357 { 01358 errorcode = HAL_ERROR; 01359 goto error; 01360 } 01361 01362 hspi->State = HAL_SPI_STATE_BUSY_RX; 01363 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 01364 hspi->pRxBuffPtr = pData; 01365 hspi->RxXferSize = Size; 01366 hspi->RxXferCount = Size; 01367 hspi->pTxBuffPtr = (uint8_t *)NULL; 01368 hspi->TxXferSize = 0; 01369 hspi->TxXferCount = 0; 01370 01371 if((hspi->Init.Mode == SPI_MODE_MASTER) && (hspi->Init.Direction == SPI_DIRECTION_2LINES)) 01372 { 01373 /* Process Unlocked */ 01374 __HAL_UNLOCK(hspi); 01375 /* the receive process is not supported in 2Lines direction master mode */ 01376 /* in this case we call the TransmitReceive process */ 01377 return HAL_SPI_TransmitReceive_DMA(hspi,pData,pData,Size); 01378 } 01379 01380 /* Configure communication direction : 1Line */ 01381 if(hspi->Init.Direction == SPI_DIRECTION_1LINE) 01382 { 01383 SPI_1LINE_RX(hspi); 01384 } 01385 01386 /* Reset CRC Calculation */ 01387 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01388 { 01389 SPI_RESET_CRC(hspi); 01390 } 01391 01392 /* packing mode management is enabled by the DMA settings */ 01393 if((hspi->Init.DataSize <= SPI_DATASIZE_8BIT) && (hspi->hdmarx->Init.MemDataAlignment == DMA_MDATAALIGN_HALFWORD)) 01394 { 01395 /* Restriction the DMA data received is not allowed in this mode */ 01396 errorcode = HAL_ERROR; 01397 goto error; 01398 } 01399 01400 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMARX); 01401 if( hspi->Init.DataSize > SPI_DATASIZE_8BIT) 01402 { 01403 /* set fiforxthresold according the reception data length: 16bit */ 01404 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01405 } 01406 else 01407 { 01408 /* set fiforxthresold according the reception data length: 8bit */ 01409 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01410 } 01411 01412 /* Set the SPI RxDMA Half transfer complete callback */ 01413 hspi->hdmarx->XferHalfCpltCallback = SPI_DMAHalfReceiveCplt; 01414 01415 /* Set the SPI Rx DMA transfer complete callback */ 01416 hspi->hdmarx->XferCpltCallback = SPI_DMAReceiveCplt; 01417 01418 /* Set the DMA error callback */ 01419 hspi->hdmarx->XferErrorCallback = SPI_DMAError; 01420 01421 /* Enable Rx DMA Request */ 01422 SET_BIT(hspi->Instance->CR2, SPI_CR2_RXDMAEN); 01423 01424 /* Enable the Rx DMA channel */ 01425 HAL_DMA_Start_IT(hspi->hdmarx, (uint32_t)&hspi->Instance->DR, (uint32_t)hspi->pRxBuffPtr, hspi->RxXferCount); 01426 01427 /* Check if the SPI is already enabled */ 01428 if((hspi->Instance->CR1 & SPI_CR1_SPE) != SPI_CR1_SPE) 01429 { 01430 /* Enable SPI peripheral */ 01431 __HAL_SPI_ENABLE(hspi); 01432 } 01433 01434 error: 01435 /* Process Unlocked */ 01436 __HAL_UNLOCK(hspi); 01437 return errorcode; 01438 } 01439 01440 /** 01441 * @brief Transmit and Receive an amount of data in non-blocking mode with DMA. 01442 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01443 * the configuration information for SPI module. 01444 * @param pTxData: pointer to transmission data buffer 01445 * @param pRxData: pointer to reception data buffer 01446 * @note When the CRC feature is enabled the pRxData Length must be Size + 1 01447 * @param Size: amount of data to be sent 01448 * @retval HAL status 01449 */ 01450 HAL_StatusTypeDef HAL_SPI_TransmitReceive_DMA(SPI_HandleTypeDef *hspi, uint8_t *pTxData, uint8_t *pRxData, uint16_t Size) 01451 { 01452 HAL_StatusTypeDef errorcode = HAL_OK; 01453 assert_param(IS_SPI_DIRECTION_2LINES(hspi->Init.Direction)); 01454 01455 /* Process locked */ 01456 __HAL_LOCK(hspi); 01457 01458 if(!((hspi->State == HAL_SPI_STATE_READY) || 01459 ((hspi->Init.Mode == SPI_MODE_MASTER) && (hspi->Init.Direction == SPI_DIRECTION_2LINES) && (hspi->State == HAL_SPI_STATE_BUSY_RX)))) 01460 { 01461 errorcode = HAL_BUSY; 01462 goto error; 01463 } 01464 01465 if((pTxData == NULL ) || (pRxData == NULL ) || (Size == 0)) 01466 { 01467 errorcode = HAL_ERROR; 01468 goto error; 01469 } 01470 01471 /* check if the transmit Receive function is not called by a receive master */ 01472 if(hspi->State != HAL_SPI_STATE_BUSY_RX) 01473 { 01474 hspi->State = HAL_SPI_STATE_BUSY_TX_RX; 01475 } 01476 01477 hspi->ErrorCode = HAL_SPI_ERROR_NONE; 01478 hspi->pTxBuffPtr = (uint8_t *)pTxData; 01479 hspi->TxXferSize = Size; 01480 hspi->TxXferCount = Size; 01481 hspi->pRxBuffPtr = (uint8_t *)pRxData; 01482 hspi->RxXferSize = Size; 01483 hspi->RxXferCount = Size; 01484 01485 /* Reset CRC Calculation + increase the rxsize */ 01486 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01487 { 01488 SPI_RESET_CRC(hspi); 01489 } 01490 01491 /* Reset the threshold bit */ 01492 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX | SPI_CR2_LDMARX); 01493 01494 /* the packing mode management is enabled by the DMA settings according the spi data size */ 01495 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 01496 { 01497 /* set fiforxthreshold according the reception data length: 16bit */ 01498 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01499 } 01500 else 01501 { 01502 /* set fiforxthresold according the reception data length: 8bit */ 01503 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01504 01505 if(hspi->hdmatx->Init.MemDataAlignment == DMA_MDATAALIGN_HALFWORD) 01506 { 01507 if((hspi->TxXferSize & 0x1) == 0x0) 01508 { 01509 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX); 01510 hspi->TxXferCount = hspi->TxXferCount >> 1; 01511 } 01512 else 01513 { 01514 SET_BIT(hspi->Instance->CR2, SPI_CR2_LDMATX); 01515 hspi->TxXferCount = (hspi->TxXferCount >> 1) + 1; 01516 } 01517 } 01518 01519 if(hspi->hdmarx->Init.MemDataAlignment == DMA_MDATAALIGN_HALFWORD) 01520 { 01521 /* set fiforxthresold according the reception data length: 16bit */ 01522 CLEAR_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 01523 01524 if((hspi->RxXferCount & 0x1) == 0x0 ) 01525 { 01526 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_LDMARX); 01527 hspi->RxXferCount = hspi->RxXferCount >> 1; 01528 } 01529 else 01530 { 01531 SET_BIT(hspi->Instance->CR2, SPI_CR2_LDMARX); 01532 hspi->RxXferCount = (hspi->RxXferCount >> 1) + 1; 01533 } 01534 } 01535 } 01536 01537 /* Set the SPI Rx DMA transfer complete callback if the transfer request is a 01538 reception request (RXNE) */ 01539 if(hspi->State == HAL_SPI_STATE_BUSY_RX) 01540 { 01541 /* Set the SPI Rx DMA Half transfer complete callback */ 01542 hspi->hdmarx->XferHalfCpltCallback = SPI_DMAHalfReceiveCplt; 01543 hspi->hdmarx->XferCpltCallback = SPI_DMAReceiveCplt; 01544 } 01545 else 01546 { 01547 /* Set the SPI Rx DMA Half transfer complete callback */ 01548 hspi->hdmarx->XferHalfCpltCallback = SPI_DMAHalfTransmitReceiveCplt; 01549 hspi->hdmarx->XferCpltCallback = SPI_DMATransmitReceiveCplt; 01550 } 01551 01552 /* Set the DMA error callback */ 01553 hspi->hdmarx->XferErrorCallback = SPI_DMAError; 01554 01555 /* Enable Rx DMA Request */ 01556 SET_BIT(hspi->Instance->CR2, SPI_CR2_RXDMAEN); 01557 01558 /* Enable the Rx DMA channel */ 01559 HAL_DMA_Start_IT(hspi->hdmarx, (uint32_t)&hspi->Instance->DR, (uint32_t) hspi->pRxBuffPtr, hspi->RxXferCount); 01560 01561 /* Set the SPI Tx DMA transfer complete callback as NULL because the communication closing 01562 is performed in DMA reception complete callback */ 01563 hspi->hdmatx->XferHalfCpltCallback = NULL; 01564 hspi->hdmatx->XferCpltCallback = NULL; 01565 01566 /* Set the DMA error callback */ 01567 hspi->hdmatx->XferErrorCallback = SPI_DMAError; 01568 01569 /* Enable the Tx DMA channel */ 01570 HAL_DMA_Start_IT(hspi->hdmatx, (uint32_t)hspi->pTxBuffPtr, (uint32_t)&hspi->Instance->DR, hspi->TxXferCount); 01571 01572 /* Check if the SPI is already enabled */ 01573 if((hspi->Instance->CR1 &SPI_CR1_SPE) != SPI_CR1_SPE) 01574 { 01575 /* Enable SPI peripheral */ 01576 __HAL_SPI_ENABLE(hspi); 01577 } 01578 01579 /* Enable Tx DMA Request */ 01580 SET_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN); 01581 01582 error : 01583 /* Process Unlocked */ 01584 __HAL_UNLOCK(hspi); 01585 return errorcode; 01586 } 01587 01588 /** 01589 * @brief Pause the DMA Transfer. 01590 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01591 * the configuration information for the specified SPI module. 01592 * @retval HAL status 01593 */ 01594 HAL_StatusTypeDef HAL_SPI_DMAPause(SPI_HandleTypeDef *hspi) 01595 { 01596 /* Process Locked */ 01597 __HAL_LOCK(hspi); 01598 01599 /* Disable the SPI DMA Tx & Rx requests */ 01600 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 01601 01602 /* Process Unlocked */ 01603 __HAL_UNLOCK(hspi); 01604 01605 return HAL_OK; 01606 } 01607 01608 /** 01609 * @brief Resume the DMA Transfer. 01610 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01611 * the configuration information for the specified SPI module. 01612 * @retval HAL status 01613 */ 01614 HAL_StatusTypeDef HAL_SPI_DMAResume(SPI_HandleTypeDef *hspi) 01615 { 01616 /* Process Locked */ 01617 __HAL_LOCK(hspi); 01618 01619 /* Enable the SPI DMA Tx & Rx requests */ 01620 SET_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 01621 01622 /* Process Unlocked */ 01623 __HAL_UNLOCK(hspi); 01624 01625 return HAL_OK; 01626 } 01627 01628 /** 01629 * @brief Stop the DMA Transfer. 01630 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01631 * the configuration information for the specified SPI module. 01632 * @retval HAL status 01633 */ 01634 HAL_StatusTypeDef HAL_SPI_DMAStop(SPI_HandleTypeDef *hspi) 01635 { 01636 /* The Lock is not implemented on this API to allow the user application 01637 to call the HAL SPI API under callbacks HAL_SPI_TxCpltCallback() or HAL_SPI_RxCpltCallback() or HAL_SPI_TxRxCpltCallback(): 01638 when calling HAL_DMA_Abort() API the DMA TX/RX Transfer complete interrupt is generated 01639 and the correspond call back is executed HAL_SPI_TxCpltCallback() or HAL_SPI_RxCpltCallback() or HAL_SPI_TxRxCpltCallback() 01640 */ 01641 01642 /* Abort the SPI DMA tx channel */ 01643 if(hspi->hdmatx != NULL) 01644 { 01645 HAL_DMA_Abort(hspi->hdmatx); 01646 } 01647 /* Abort the SPI DMA rx channel */ 01648 if(hspi->hdmarx != NULL) 01649 { 01650 HAL_DMA_Abort(hspi->hdmarx); 01651 } 01652 01653 /* Disable the SPI DMA Tx & Rx requests */ 01654 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 01655 hspi->State = HAL_SPI_STATE_READY; 01656 return HAL_OK; 01657 } 01658 01659 /** 01660 * @brief Handle SPI interrupt request. 01661 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01662 * the configuration information for the specified SPI module. 01663 * @retval None 01664 */ 01665 void HAL_SPI_IRQHandler(SPI_HandleTypeDef *hspi) 01666 { 01667 uint32_t itsource = hspi->Instance->CR2; 01668 uint32_t itflag = hspi->Instance->SR; 01669 01670 /* SPI in mode Receiver ----------------------------------------------------*/ 01671 if(((itflag & SPI_FLAG_OVR) == RESET) && 01672 ((itflag & SPI_FLAG_RXNE) != RESET) && ((itsource & SPI_IT_RXNE) != RESET)) 01673 { 01674 hspi->RxISR(hspi); 01675 return; 01676 } 01677 01678 /* SPI in mode Transmitter ---------------------------------------------------*/ 01679 if(((itflag & SPI_FLAG_TXE) != RESET) && ((itsource & SPI_IT_TXE) != RESET)) 01680 { 01681 hspi->TxISR(hspi); 01682 return; 01683 } 01684 01685 /* SPI in Error Treatment ---------------------------------------------------*/ 01686 if((itflag & (SPI_FLAG_MODF | SPI_FLAG_OVR | SPI_FLAG_FRE)) != RESET) 01687 { 01688 /* SPI Overrun error interrupt occurred -------------------------------------*/ 01689 if((itflag & SPI_FLAG_OVR) != RESET) 01690 { 01691 if(hspi->State != HAL_SPI_STATE_BUSY_TX) 01692 { 01693 hspi->ErrorCode |= HAL_SPI_ERROR_OVR; 01694 __HAL_SPI_CLEAR_OVRFLAG(hspi); 01695 } 01696 else 01697 { 01698 return; 01699 } 01700 } 01701 01702 /* SPI Mode Fault error interrupt occurred -------------------------------------*/ 01703 if((itflag & SPI_FLAG_MODF) != RESET) 01704 { 01705 hspi->ErrorCode |= HAL_SPI_ERROR_MODF; 01706 __HAL_SPI_CLEAR_MODFFLAG(hspi); 01707 } 01708 01709 /* SPI Frame error interrupt occurred ----------------------------------------*/ 01710 if((itflag & SPI_FLAG_FRE) != RESET) 01711 { 01712 hspi->ErrorCode |= HAL_SPI_ERROR_FRE; 01713 __HAL_SPI_CLEAR_FREFLAG(hspi); 01714 } 01715 01716 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_RXNE | SPI_IT_TXE | SPI_IT_ERR); 01717 hspi->State = HAL_SPI_STATE_READY; 01718 HAL_SPI_ErrorCallback(hspi); 01719 return; 01720 } 01721 } 01722 01723 /** 01724 * @brief Tx Transfer completed callback. 01725 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01726 * the configuration information for SPI module. 01727 * @retval None 01728 */ 01729 __weak void HAL_SPI_TxCpltCallback(SPI_HandleTypeDef *hspi) 01730 { 01731 /* NOTE : This function should not be modified, when the callback is needed, 01732 the HAL_SPI_TxCpltCallback should be implemented in the user file 01733 */ 01734 } 01735 01736 /** 01737 * @brief Rx Transfer completed callback. 01738 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01739 * the configuration information for SPI module. 01740 * @retval None 01741 */ 01742 __weak void HAL_SPI_RxCpltCallback(SPI_HandleTypeDef *hspi) 01743 { 01744 /* NOTE : This function should not be modified, when the callback is needed, 01745 the HAL_SPI_RxCpltCallback should be implemented in the user file 01746 */ 01747 } 01748 01749 /** 01750 * @brief Tx and Rx Transfer completed callback. 01751 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01752 * the configuration information for SPI module. 01753 * @retval None 01754 */ 01755 __weak void HAL_SPI_TxRxCpltCallback(SPI_HandleTypeDef *hspi) 01756 { 01757 /* NOTE : This function should not be modified, when the callback is needed, 01758 the HAL_SPI_TxRxCpltCallback should be implemented in the user file 01759 */ 01760 } 01761 01762 /** 01763 * @brief Tx Half Transfer completed callback. 01764 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01765 * the configuration information for SPI module. 01766 * @retval None 01767 */ 01768 __weak void HAL_SPI_TxHalfCpltCallback(SPI_HandleTypeDef *hspi) 01769 { 01770 /* NOTE : This function should not be modified, when the callback is needed, 01771 the HAL_SPI_TxHalfCpltCallback should be implemented in the user file 01772 */ 01773 } 01774 01775 /** 01776 * @brief Rx Half Transfer completed callback. 01777 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01778 * the configuration information for SPI module. 01779 * @retval None 01780 */ 01781 __weak void HAL_SPI_RxHalfCpltCallback(SPI_HandleTypeDef *hspi) 01782 { 01783 /* NOTE : This function should not be modified, when the callback is needed, 01784 the HAL_SPI_RxHalfCpltCallback() should be implemented in the user file 01785 */ 01786 } 01787 01788 /** 01789 * @brief Tx and Rx Half Transfer callback. 01790 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01791 * the configuration information for SPI module. 01792 * @retval None 01793 */ 01794 __weak void HAL_SPI_TxRxHalfCpltCallback(SPI_HandleTypeDef *hspi) 01795 { 01796 /* NOTE : This function should not be modified, when the callback is needed, 01797 the HAL_SPI_TxRxHalfCpltCallback() should be implemented in the user file 01798 */ 01799 } 01800 01801 /** 01802 * @brief SPI error callback. 01803 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01804 * the configuration information for SPI module. 01805 * @retval None 01806 */ 01807 __weak void HAL_SPI_ErrorCallback(SPI_HandleTypeDef *hspi) 01808 { 01809 /* NOTE : This function should not be modified, when the callback is needed, 01810 the HAL_SPI_ErrorCallback should be implemented in the user file 01811 */ 01812 /* NOTE : The ErrorCode parameter in the hspi handle is updated by the SPI processes 01813 and user can use HAL_SPI_GetError() API to check the latest error occurred 01814 */ 01815 } 01816 01817 /** 01818 * @} 01819 */ 01820 01821 /** @defgroup SPI_Exported_Functions_Group3 Peripheral State and Errors functions 01822 * @brief SPI control functions 01823 * 01824 @verbatim 01825 =============================================================================== 01826 ##### Peripheral State and Errors functions ##### 01827 =============================================================================== 01828 [..] 01829 This subsection provides a set of functions allowing to control the SPI. 01830 (+) HAL_SPI_GetState() API can be helpful to check in run-time the state of the SPI peripheral 01831 (+) HAL_SPI_GetError() check in run-time Errors occurring during communication 01832 @endverbatim 01833 * @{ 01834 */ 01835 01836 /** 01837 * @brief Return the SPI handle state. 01838 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01839 * the configuration information for SPI module. 01840 * @retval SPI state 01841 */ 01842 HAL_SPI_StateTypeDef HAL_SPI_GetState(SPI_HandleTypeDef *hspi) 01843 { 01844 /* Return SPI handle state */ 01845 return hspi->State; 01846 } 01847 01848 /** 01849 * @brief Return the SPI error code. 01850 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 01851 * the configuration information for SPI module. 01852 * @retval SPI error code in bitmap format 01853 */ 01854 uint32_t HAL_SPI_GetError(SPI_HandleTypeDef *hspi) 01855 { 01856 return hspi->ErrorCode; 01857 } 01858 01859 /** 01860 * @} 01861 */ 01862 01863 01864 /** 01865 * @} 01866 */ 01867 01868 /** @addtogroup SPI_Private_Functions 01869 * @brief Private functions 01870 * @{ 01871 */ 01872 01873 /** 01874 * @brief DMA SPI transmit process complete callback. 01875 * @param hdma: pointer to a DMA_HandleTypeDef structure that contains 01876 * the configuration information for the specified DMA module. 01877 * @retval None 01878 */ 01879 static void SPI_DMATransmitCplt(DMA_HandleTypeDef *hdma) 01880 { 01881 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 01882 01883 if((hdma->Instance->CCR & DMA_CCR_CIRC) != DMA_CCR_CIRC) 01884 { 01885 /* Disable Tx DMA Request */ 01886 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN); 01887 01888 /* Check the end of the transaction */ 01889 if(SPI_EndRxTxTransaction(hspi,SPI_DEFAULT_TIMEOUT) != HAL_OK) 01890 { 01891 hspi->ErrorCode = HAL_SPI_ERROR_FLAG; 01892 } 01893 01894 /* Clear overrun flag in 2 Lines communication mode because received data is not read */ 01895 if(hspi->Init.Direction == SPI_DIRECTION_2LINES) 01896 { 01897 __HAL_SPI_CLEAR_OVRFLAG(hspi); 01898 } 01899 01900 hspi->TxXferCount = 0; 01901 hspi->State = HAL_SPI_STATE_READY; 01902 01903 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 01904 { 01905 HAL_SPI_ErrorCallback(hspi); 01906 return; 01907 } 01908 } 01909 HAL_SPI_TxCpltCallback(hspi); 01910 } 01911 01912 /** 01913 * @brief DMA SPI receive process complete callback. 01914 * @param hdma: pointer to a DMA_HandleTypeDef structure that contains 01915 * the configuration information for the specified DMA module. 01916 * @retval None 01917 */ 01918 static void SPI_DMAReceiveCplt(DMA_HandleTypeDef *hdma) 01919 { 01920 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 01921 01922 if((hdma->Instance->CCR & DMA_CCR_CIRC) != DMA_CCR_CIRC) 01923 { 01924 __IO uint16_t tmpreg; 01925 01926 /* CRC handling */ 01927 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 01928 { 01929 /* Wait until TXE flag */ 01930 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, SPI_DEFAULT_TIMEOUT) != HAL_OK) 01931 { 01932 /* Error on the CRC reception */ 01933 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 01934 } 01935 if(hspi->Init.DataSize > SPI_DATASIZE_8BIT) 01936 { 01937 tmpreg = hspi->Instance->DR; 01938 UNUSED(tmpreg); /* To avoid GCC warning */ 01939 } 01940 else 01941 { 01942 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 01943 UNUSED(tmpreg); /* To avoid GCC warning */ 01944 01945 if(hspi->Init.CRCLength == SPI_CRC_LENGTH_16BIT) 01946 { 01947 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_RXNE, SPI_FLAG_RXNE, SPI_DEFAULT_TIMEOUT) != HAL_OK) 01948 { 01949 /* Error on the CRC reception */ 01950 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 01951 } 01952 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 01953 UNUSED(tmpreg); /* To avoid GCC warning */ 01954 } 01955 } 01956 } 01957 01958 /* Disable Rx/Tx DMA Request (done by default to handle the case master rx direction 2 lines) */ 01959 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 01960 01961 /* Check the end of the transaction */ 01962 if(SPI_EndRxTransaction(hspi,SPI_DEFAULT_TIMEOUT)!=HAL_OK) 01963 { 01964 hspi->ErrorCode|= HAL_SPI_ERROR_FLAG; 01965 } 01966 01967 hspi->RxXferCount = 0; 01968 hspi->State = HAL_SPI_STATE_READY; 01969 01970 /* Check if CRC error occurred */ 01971 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 01972 { 01973 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 01974 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 01975 } 01976 01977 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 01978 { 01979 HAL_SPI_ErrorCallback(hspi); 01980 return; 01981 } 01982 } 01983 HAL_SPI_RxCpltCallback(hspi); 01984 } 01985 01986 /** 01987 * @brief DMA SPI transmit receive process complete callback. 01988 * @param hdma : pointer to a DMA_HandleTypeDef structure that contains 01989 * the configuration information for the specified DMA module. 01990 * @retval None 01991 */ 01992 static void SPI_DMATransmitReceiveCplt(DMA_HandleTypeDef *hdma) 01993 { 01994 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 01995 01996 if((hdma->Instance->CCR & DMA_CCR_CIRC) != DMA_CCR_CIRC) 01997 { 01998 __IO int16_t tmpreg; 01999 /* CRC handling */ 02000 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02001 { 02002 if((hspi->Init.DataSize == SPI_DATASIZE_8BIT) && (hspi->Init.CRCLength == SPI_CRC_LENGTH_8BIT)) 02003 { 02004 if(SPI_WaitFifoStateUntilTimeout(hspi, SPI_FLAG_FRLVL, SPI_FRLVL_QUARTER_FULL, SPI_DEFAULT_TIMEOUT) != HAL_OK) 02005 { 02006 /* Error on the CRC reception */ 02007 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 02008 } 02009 tmpreg = *(__IO uint8_t *)&hspi->Instance->DR; 02010 UNUSED(tmpreg); /* To avoid GCC warning */ 02011 } 02012 else 02013 { 02014 if(SPI_WaitFifoStateUntilTimeout(hspi, SPI_FLAG_FRLVL, SPI_FRLVL_HALF_FULL, SPI_DEFAULT_TIMEOUT) != HAL_OK) 02015 { 02016 /* Error on the CRC reception */ 02017 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 02018 } 02019 tmpreg = hspi->Instance->DR; 02020 UNUSED(tmpreg); /* To avoid GCC warning */ 02021 } 02022 } 02023 02024 /* Check the end of the transaction */ 02025 if(SPI_EndRxTxTransaction(hspi,SPI_DEFAULT_TIMEOUT) != HAL_OK) 02026 { 02027 hspi->ErrorCode = HAL_SPI_ERROR_FLAG; 02028 } 02029 02030 /* Disable Rx/Tx DMA Request */ 02031 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 02032 02033 hspi->TxXferCount = 0; 02034 hspi->RxXferCount = 0; 02035 hspi->State = HAL_SPI_STATE_READY; 02036 02037 /* Check if CRC error occurred */ 02038 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 02039 { 02040 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 02041 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 02042 } 02043 02044 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 02045 { 02046 HAL_SPI_ErrorCallback(hspi); 02047 return; 02048 } 02049 } 02050 HAL_SPI_TxRxCpltCallback(hspi); 02051 } 02052 02053 /** 02054 * @brief DMA SPI half transmit process complete callback. 02055 * @param hdma : pointer to a DMA_HandleTypeDef structure that contains 02056 * the configuration information for the specified DMA module. 02057 * @retval None 02058 */ 02059 static void SPI_DMAHalfTransmitCplt(DMA_HandleTypeDef *hdma) 02060 { 02061 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 02062 02063 HAL_SPI_TxHalfCpltCallback(hspi); 02064 } 02065 02066 /** 02067 * @brief DMA SPI half receive process complete callback. 02068 * @param hdma: pointer to a DMA_HandleTypeDef structure that contains 02069 * the configuration information for the specified DMA module. 02070 * @retval None 02071 */ 02072 static void SPI_DMAHalfReceiveCplt(DMA_HandleTypeDef *hdma) 02073 { 02074 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 02075 02076 HAL_SPI_RxHalfCpltCallback(hspi); 02077 } 02078 02079 /** 02080 * @brief DMA SPI half transmit receive process complete callback. 02081 * @param hdma : pointer to a DMA_HandleTypeDef structure that contains 02082 * the configuration information for the specified DMA module. 02083 * @retval None 02084 */ 02085 static void SPI_DMAHalfTransmitReceiveCplt(DMA_HandleTypeDef *hdma) 02086 { 02087 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 02088 02089 HAL_SPI_TxRxHalfCpltCallback(hspi); 02090 } 02091 02092 /** 02093 * @brief DMA SPI communication error callback. 02094 * @param hdma : pointer to a DMA_HandleTypeDef structure that contains 02095 * the configuration information for the specified DMA module. 02096 * @retval None 02097 */ 02098 static void SPI_DMAError(DMA_HandleTypeDef *hdma) 02099 { 02100 SPI_HandleTypeDef* hspi = ( SPI_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 02101 02102 /* Stop the disable DMA transfer on SPI side */ 02103 CLEAR_BIT(hspi->Instance->CR2, SPI_CR2_TXDMAEN | SPI_CR2_RXDMAEN); 02104 02105 hspi->ErrorCode|= HAL_SPI_ERROR_DMA; 02106 hspi->State = HAL_SPI_STATE_READY; 02107 HAL_SPI_ErrorCallback(hspi); 02108 } 02109 02110 /** 02111 * @brief Rx 8-bit handler for Transmit and Receive in Interrupt mode. 02112 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02113 * the configuration information for SPI module. 02114 * @retval None 02115 */ 02116 static void SPI_2linesRxISR_8BIT(struct __SPI_HandleTypeDef *hspi) 02117 { 02118 /* Receive data in packing mode */ 02119 if(hspi->RxXferCount > 1) 02120 { 02121 *((uint16_t*)hspi->pRxBuffPtr) = hspi->Instance->DR; 02122 hspi->pRxBuffPtr += sizeof(uint16_t); 02123 hspi->RxXferCount -= 2; 02124 if(hspi->RxXferCount == 1) 02125 { 02126 /* set fiforxthresold according the reception data length: 8bit */ 02127 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 02128 } 02129 } 02130 /* Receive data in 8 Bit mode */ 02131 else 02132 { 02133 *hspi->pRxBuffPtr++ = *((__IO uint8_t *)&hspi->Instance->DR); 02134 hspi->RxXferCount--; 02135 } 02136 02137 /* check end of the reception */ 02138 if(hspi->RxXferCount == 0) 02139 { 02140 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02141 { 02142 SET_BIT(hspi->Instance->CR2, SPI_RXFIFO_THRESHOLD); 02143 hspi->RxISR = SPI_2linesRxISR_8BITCRC; 02144 return; 02145 } 02146 02147 /* Disable RXNE interrupt */ 02148 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_RXNE); 02149 02150 if(hspi->TxXferCount == 0) 02151 { 02152 SPI_CloseRxTx_ISR(hspi); 02153 } 02154 } 02155 } 02156 02157 /** 02158 * @brief Rx 8-bit handler for Transmit and Receive in Interrupt mode. 02159 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02160 * the configuration information for SPI module. 02161 * @retval None 02162 */ 02163 static void SPI_2linesRxISR_8BITCRC(struct __SPI_HandleTypeDef *hspi) 02164 { 02165 __IO uint8_t tmpreg = *((__IO uint8_t *)&hspi->Instance->DR); 02166 UNUSED(tmpreg); /* To avoid GCC warning */ 02167 02168 hspi->CRCSize--; 02169 02170 /* check end of the reception */ 02171 if(hspi->CRCSize == 0) 02172 { 02173 /* Disable RXNE interrupt */ 02174 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_RXNE); 02175 02176 if(hspi->TxXferCount == 0) 02177 { 02178 SPI_CloseRxTx_ISR(hspi); 02179 } 02180 } 02181 } 02182 02183 /** 02184 * @brief Tx 8-bit handler for Transmit and Receive in Interrupt mode. 02185 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02186 * the configuration information for SPI module. 02187 * @retval None 02188 */ 02189 static void SPI_2linesTxISR_8BIT(struct __SPI_HandleTypeDef *hspi) 02190 { 02191 /* Transmit data in packing Bit mode */ 02192 if(hspi->TxXferCount >= 2) 02193 { 02194 hspi->Instance->DR = *((uint16_t *)hspi->pTxBuffPtr); 02195 hspi->pTxBuffPtr += sizeof(uint16_t); 02196 hspi->TxXferCount -= 2; 02197 } 02198 /* Transmit data in 8 Bit mode */ 02199 else 02200 { 02201 *(__IO uint8_t *)&hspi->Instance->DR = (*hspi->pTxBuffPtr++); 02202 hspi->TxXferCount--; 02203 } 02204 02205 /* check the end of the transmission */ 02206 if(hspi->TxXferCount == 0) 02207 { 02208 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02209 { 02210 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02211 } 02212 /* Disable TXE interrupt */ 02213 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_TXE); 02214 02215 if(hspi->RxXferCount == 0) 02216 { 02217 SPI_CloseRxTx_ISR(hspi); 02218 } 02219 } 02220 } 02221 02222 /** 02223 * @brief Rx 16-bit handler for Transmit and Receive in Interrupt mode. 02224 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02225 * the configuration information for SPI module. 02226 * @retval None 02227 */ 02228 static void SPI_2linesRxISR_16BIT(struct __SPI_HandleTypeDef *hspi) 02229 { 02230 /* Receive data in 16 Bit mode */ 02231 *((uint16_t*)hspi->pRxBuffPtr) = hspi->Instance->DR; 02232 hspi->pRxBuffPtr += sizeof(uint16_t); 02233 hspi->RxXferCount--; 02234 02235 if(hspi->RxXferCount == 0) 02236 { 02237 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02238 { 02239 hspi->RxISR = SPI_2linesRxISR_16BITCRC; 02240 return; 02241 } 02242 02243 /* Disable RXNE interrupt */ 02244 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_RXNE); 02245 02246 if(hspi->TxXferCount == 0) 02247 { 02248 SPI_CloseRxTx_ISR(hspi); 02249 } 02250 } 02251 } 02252 02253 /** 02254 * @brief Manage the CRC 16-bit receive for Transmit and Receive in Interrupt mode. 02255 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02256 * the configuration information for SPI module. 02257 * @retval None 02258 */ 02259 static void SPI_2linesRxISR_16BITCRC(struct __SPI_HandleTypeDef *hspi) 02260 { 02261 /* Receive data in 16 Bit mode */ 02262 __IO uint16_t tmpreg = hspi->Instance->DR; 02263 UNUSED(tmpreg); /* To avoid GCC warning */ 02264 02265 /* Disable RXNE interrupt */ 02266 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_RXNE); 02267 02268 SPI_CloseRxTx_ISR(hspi); 02269 } 02270 02271 /** 02272 * @brief Tx 16-bit handler for Transmit and Receive in Interrupt mode. 02273 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02274 * the configuration information for SPI module. 02275 * @retval None 02276 */ 02277 static void SPI_2linesTxISR_16BIT(struct __SPI_HandleTypeDef *hspi) 02278 { 02279 /* Transmit data in 16 Bit mode */ 02280 hspi->Instance->DR = *((uint16_t *)hspi->pTxBuffPtr); 02281 hspi->pTxBuffPtr += sizeof(uint16_t); 02282 hspi->TxXferCount--; 02283 02284 /* Enable CRC Transmission */ 02285 if(hspi->TxXferCount == 0) 02286 { 02287 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02288 { 02289 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02290 } 02291 /* Disable TXE interrupt */ 02292 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_TXE); 02293 02294 if(hspi->RxXferCount == 0) 02295 { 02296 SPI_CloseRxTx_ISR(hspi); 02297 } 02298 } 02299 } 02300 02301 /** 02302 * @brief Manage the CRC 8-bit receive in Interrupt context. 02303 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02304 * the configuration information for SPI module. 02305 * @retval None 02306 */ 02307 static void SPI_RxISR_8BITCRC(struct __SPI_HandleTypeDef *hspi) 02308 { 02309 __IO uint8_t tmpreg = *((uint8_t*)&hspi->Instance->DR); 02310 UNUSED(tmpreg); /* To avoid GCC warning */ 02311 02312 hspi->CRCSize--; 02313 02314 if(hspi->CRCSize == 0) 02315 { 02316 SPI_CloseRx_ISR(hspi); 02317 } 02318 } 02319 02320 /** 02321 * @brief Manage the receive 8-bit in Interrupt context. 02322 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02323 * the configuration information for SPI module. 02324 * @retval None 02325 */ 02326 static void SPI_RxISR_8BIT(struct __SPI_HandleTypeDef *hspi) 02327 { 02328 *hspi->pRxBuffPtr++ = (*(__IO uint8_t *)&hspi->Instance->DR); 02329 hspi->RxXferCount--; 02330 02331 /* Enable CRC Transmission */ 02332 if((hspi->RxXferCount == 1) && (hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE)) 02333 { 02334 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02335 } 02336 02337 if(hspi->RxXferCount == 0) 02338 { 02339 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02340 { 02341 hspi->RxISR = SPI_RxISR_8BITCRC; 02342 return; 02343 } 02344 SPI_CloseRx_ISR(hspi); 02345 } 02346 } 02347 02348 /** 02349 * @brief Manage the CRC 16-bit receive in Interrupt context. 02350 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02351 * the configuration information for SPI module. 02352 * @retval None 02353 */ 02354 static void SPI_RxISR_16BITCRC(struct __SPI_HandleTypeDef *hspi) 02355 { 02356 __IO uint16_t tmpreg; 02357 02358 tmpreg = hspi->Instance->DR; 02359 UNUSED(tmpreg); /* To avoid GCC warning */ 02360 02361 /* Disable RXNE and ERR interrupt */ 02362 __HAL_SPI_DISABLE_IT(hspi, (SPI_IT_RXNE | SPI_IT_ERR)); 02363 02364 SPI_CloseRx_ISR(hspi); 02365 } 02366 02367 /** 02368 * @brief Manage the 16-bit receive in Interrupt context. 02369 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02370 * the configuration information for SPI module. 02371 * @retval None 02372 */ 02373 static void SPI_RxISR_16BIT(struct __SPI_HandleTypeDef *hspi) 02374 { 02375 *((uint16_t *)hspi->pRxBuffPtr) = hspi->Instance->DR; 02376 hspi->pRxBuffPtr += sizeof(uint16_t); 02377 hspi->RxXferCount--; 02378 02379 /* Enable CRC Transmission */ 02380 if((hspi->RxXferCount == 1) && (hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE)) 02381 { 02382 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02383 } 02384 02385 if(hspi->RxXferCount == 0) 02386 { 02387 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02388 { 02389 hspi->RxISR = SPI_RxISR_16BITCRC; 02390 return; 02391 } 02392 SPI_CloseRx_ISR(hspi); 02393 } 02394 } 02395 02396 /** 02397 * @brief Handle the data 8-bit transmit in Interrupt mode. 02398 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02399 * the configuration information for SPI module. 02400 * @retval None 02401 */ 02402 static void SPI_TxISR_8BIT(struct __SPI_HandleTypeDef *hspi) 02403 { 02404 *(__IO uint8_t *)&hspi->Instance->DR = (*hspi->pTxBuffPtr++); 02405 hspi->TxXferCount--; 02406 02407 if(hspi->TxXferCount == 0) 02408 { 02409 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02410 { 02411 /* Enable CRC Transmission */ 02412 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02413 } 02414 02415 SPI_CloseTx_ISR(hspi); 02416 } 02417 } 02418 02419 /** 02420 * @brief Handle the data 16-bit transmit in Interrupt mode. 02421 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02422 * the configuration information for SPI module. 02423 * @retval None 02424 */ 02425 static void SPI_TxISR_16BIT(struct __SPI_HandleTypeDef *hspi) 02426 { 02427 /* Transmit data in 16 Bit mode */ 02428 hspi->Instance->DR = *((uint16_t *)hspi->pTxBuffPtr); 02429 hspi->pTxBuffPtr += sizeof(uint16_t); 02430 hspi->TxXferCount--; 02431 02432 if(hspi->TxXferCount == 0) 02433 { 02434 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02435 { 02436 /* Enable CRC Transmission */ 02437 hspi->Instance->CR1 |= SPI_CR1_CRCNEXT; 02438 } 02439 SPI_CloseTx_ISR(hspi); 02440 } 02441 } 02442 02443 /** 02444 * @brief Handle SPI Communication Timeout. 02445 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02446 * the configuration information for SPI module. 02447 * @param Flag : SPI flag to check 02448 * @param State : flag state to check 02449 * @param Timeout : Timeout duration 02450 * @retval HAL status 02451 */ 02452 static HAL_StatusTypeDef SPI_WaitFlagStateUntilTimeout(SPI_HandleTypeDef *hspi, uint32_t Flag, uint32_t State, uint32_t Timeout) 02453 { 02454 uint32_t tickstart = HAL_GetTick(); 02455 02456 while((hspi->Instance->SR & Flag) != State) 02457 { 02458 if(Timeout != HAL_MAX_DELAY) 02459 { 02460 if((Timeout == 0) || ((HAL_GetTick()-tickstart) >= Timeout)) 02461 { 02462 /* Disable the SPI and reset the CRC: the CRC value should be cleared 02463 on both master and slave sides in order to resynchronize the master 02464 and slave for their respective CRC calculation */ 02465 02466 /* Disable TXE, RXNE and ERR interrupts for the interrupt process */ 02467 __HAL_SPI_DISABLE_IT(hspi, (SPI_IT_TXE | SPI_IT_RXNE | SPI_IT_ERR)); 02468 02469 if((hspi->Init.Mode == SPI_MODE_MASTER)&&((hspi->Init.Direction == SPI_DIRECTION_1LINE)||(hspi->Init.Direction == SPI_DIRECTION_2LINES_RXONLY))) 02470 { 02471 /* Disable SPI peripheral */ 02472 __HAL_SPI_DISABLE(hspi); 02473 } 02474 02475 /* Reset CRC Calculation */ 02476 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02477 { 02478 SPI_RESET_CRC(hspi); 02479 } 02480 02481 hspi->State= HAL_SPI_STATE_READY; 02482 02483 /* Process Unlocked */ 02484 __HAL_UNLOCK(hspi); 02485 02486 return HAL_TIMEOUT; 02487 } 02488 } 02489 } 02490 02491 return HAL_OK; 02492 } 02493 02494 /** 02495 * @brief Handle SPI FIFO Communication Timeout. 02496 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02497 * the configuration information for SPI module. 02498 * @param Fifo : Fifo to check 02499 * @param State : Fifo state to check 02500 * @param Timeout : Timeout duration 02501 * @retval HAL status 02502 */ 02503 static HAL_StatusTypeDef SPI_WaitFifoStateUntilTimeout(SPI_HandleTypeDef *hspi, uint32_t Fifo, uint32_t State, uint32_t Timeout) 02504 { 02505 __IO uint8_t tmpreg; 02506 uint32_t tickstart = HAL_GetTick(); 02507 02508 while((hspi->Instance->SR & Fifo) != State) 02509 { 02510 if((Fifo == SPI_SR_FRLVL) && (State == SPI_FRLVL_EMPTY)) 02511 { 02512 tmpreg = *((__IO uint8_t*)&hspi->Instance->DR); 02513 UNUSED(tmpreg); /* To avoid GCC warning */ 02514 } 02515 02516 if(Timeout != HAL_MAX_DELAY) 02517 { 02518 if((Timeout == 0) || ((HAL_GetTick()-tickstart) >= Timeout)) 02519 { 02520 /* Disable the SPI and reset the CRC: the CRC value should be cleared 02521 on both master and slave sides in order to resynchronize the master 02522 and slave for their respective CRC calculation */ 02523 02524 /* Disable TXE, RXNE and ERR interrupts for the interrupt process */ 02525 __HAL_SPI_DISABLE_IT(hspi, (SPI_IT_TXE | SPI_IT_RXNE | SPI_IT_ERR)); 02526 02527 if((hspi->Init.Mode == SPI_MODE_MASTER)&&((hspi->Init.Direction == SPI_DIRECTION_1LINE)||(hspi->Init.Direction == SPI_DIRECTION_2LINES_RXONLY))) 02528 { 02529 /* Disable SPI peripheral */ 02530 __HAL_SPI_DISABLE(hspi); 02531 } 02532 02533 /* Reset CRC Calculation */ 02534 if(hspi->Init.CRCCalculation == SPI_CRCCALCULATION_ENABLE) 02535 { 02536 SPI_RESET_CRC(hspi); 02537 } 02538 02539 hspi->State = HAL_SPI_STATE_READY; 02540 02541 /* Process Unlocked */ 02542 __HAL_UNLOCK(hspi); 02543 02544 return HAL_TIMEOUT; 02545 } 02546 } 02547 } 02548 02549 return HAL_OK; 02550 } 02551 02552 /** 02553 * @brief Handle the check of the RX transaction complete. 02554 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02555 * the configuration information for SPI module. 02556 * @param Timeout : Timeout duration 02557 * @retval None 02558 */ 02559 static HAL_StatusTypeDef SPI_EndRxTransaction(SPI_HandleTypeDef *hspi, uint32_t Timeout) 02560 { 02561 if((hspi->Init.Mode == SPI_MODE_MASTER)&&((hspi->Init.Direction == SPI_DIRECTION_1LINE)||(hspi->Init.Direction == SPI_DIRECTION_2LINES_RXONLY))) 02562 { 02563 /* Disable SPI peripheral */ 02564 __HAL_SPI_DISABLE(hspi); 02565 } 02566 02567 /* Control the BSY flag */ 02568 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_BSY, RESET, Timeout) != HAL_OK) 02569 { 02570 hspi->ErrorCode |= HAL_SPI_ERROR_FLAG; 02571 return HAL_TIMEOUT; 02572 } 02573 02574 if((hspi->Init.Mode == SPI_MODE_MASTER)&&((hspi->Init.Direction == SPI_DIRECTION_1LINE)||(hspi->Init.Direction == SPI_DIRECTION_2LINES_RXONLY))) 02575 { 02576 /* Empty the FRLVL fifo */ 02577 if(SPI_WaitFifoStateUntilTimeout(hspi, SPI_FLAG_FRLVL, SPI_FRLVL_EMPTY, Timeout) != HAL_OK) 02578 { 02579 hspi->ErrorCode |= HAL_SPI_ERROR_FLAG; 02580 return HAL_TIMEOUT; 02581 } 02582 } 02583 return HAL_OK; 02584 } 02585 02586 /** 02587 * @brief Handle the check of the RXTX or TX transaction complete. 02588 * @param hspi: SPI handle 02589 * @param Timeout : Timeout duration 02590 */ 02591 static HAL_StatusTypeDef SPI_EndRxTxTransaction(SPI_HandleTypeDef *hspi, uint32_t Timeout) 02592 { 02593 /* Control if the TX fifo is empty */ 02594 if(SPI_WaitFifoStateUntilTimeout(hspi, SPI_FLAG_FTLVL, SPI_FTLVL_EMPTY, Timeout) != HAL_OK) 02595 { 02596 hspi->ErrorCode |= HAL_SPI_ERROR_FLAG; 02597 return HAL_TIMEOUT; 02598 } 02599 /* Control the BSY flag */ 02600 if(SPI_WaitFlagStateUntilTimeout(hspi, SPI_FLAG_BSY, RESET, Timeout) != HAL_OK) 02601 { 02602 hspi->ErrorCode |= HAL_SPI_ERROR_FLAG; 02603 return HAL_TIMEOUT; 02604 } 02605 return HAL_OK; 02606 } 02607 02608 /** 02609 * @brief Handle the end of the RXTX transaction. 02610 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02611 * the configuration information for SPI module. 02612 * @retval None 02613 */ 02614 static void SPI_CloseRxTx_ISR(SPI_HandleTypeDef *hspi) 02615 { 02616 /* Disable ERR interrupt */ 02617 __HAL_SPI_DISABLE_IT(hspi, SPI_IT_ERR); 02618 02619 /* Check the end of the transaction */ 02620 if(SPI_EndRxTxTransaction(hspi,SPI_DEFAULT_TIMEOUT)!=HAL_OK) 02621 { 02622 hspi->ErrorCode|= HAL_SPI_ERROR_FLAG; 02623 } 02624 02625 /* Check if CRC error occurred */ 02626 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 02627 { 02628 hspi->State = HAL_SPI_STATE_READY; 02629 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 02630 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 02631 HAL_SPI_ErrorCallback(hspi); 02632 } 02633 else 02634 { 02635 if(hspi->ErrorCode == HAL_SPI_ERROR_NONE) 02636 { 02637 if(hspi->State == HAL_SPI_STATE_BUSY_RX) 02638 { 02639 hspi->State = HAL_SPI_STATE_READY; 02640 HAL_SPI_RxCpltCallback(hspi); 02641 } 02642 else 02643 { 02644 hspi->State = HAL_SPI_STATE_READY; 02645 HAL_SPI_TxRxCpltCallback(hspi); 02646 } 02647 } 02648 else 02649 { 02650 hspi->State = HAL_SPI_STATE_READY; 02651 HAL_SPI_ErrorCallback(hspi); 02652 } 02653 } 02654 } 02655 02656 /** 02657 * @brief Handle the end of the RX transaction. 02658 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02659 * the configuration information for SPI module. 02660 * @retval None 02661 */ 02662 static void SPI_CloseRx_ISR(SPI_HandleTypeDef *hspi) 02663 { 02664 /* Disable RXNE and ERR interrupt */ 02665 __HAL_SPI_DISABLE_IT(hspi, (SPI_IT_RXNE | SPI_IT_ERR)); 02666 02667 /* Check the end of the transaction */ 02668 if(SPI_EndRxTransaction(hspi,SPI_DEFAULT_TIMEOUT)!=HAL_OK) 02669 { 02670 hspi->ErrorCode|= HAL_SPI_ERROR_FLAG; 02671 } 02672 hspi->State = HAL_SPI_STATE_READY; 02673 02674 /* Check if CRC error occurred */ 02675 if(__HAL_SPI_GET_FLAG(hspi, SPI_FLAG_CRCERR) != RESET) 02676 { 02677 hspi->ErrorCode|= HAL_SPI_ERROR_CRC; 02678 __HAL_SPI_CLEAR_CRCERRFLAG(hspi); 02679 HAL_SPI_ErrorCallback(hspi); 02680 } 02681 else 02682 { 02683 if(hspi->ErrorCode == HAL_SPI_ERROR_NONE) 02684 { 02685 HAL_SPI_RxCpltCallback(hspi); 02686 } 02687 else 02688 { 02689 HAL_SPI_ErrorCallback(hspi); 02690 } 02691 } 02692 } 02693 02694 /** 02695 * @brief Handle the end of the TX transaction. 02696 * @param hspi: pointer to a SPI_HandleTypeDef structure that contains 02697 * the configuration information for SPI module. 02698 * @retval None 02699 */ 02700 static void SPI_CloseTx_ISR(SPI_HandleTypeDef *hspi) 02701 { 02702 /* Disable TXE and ERR interrupt */ 02703 __HAL_SPI_DISABLE_IT(hspi, (SPI_IT_TXE | SPI_IT_ERR)); 02704 02705 /* Check the end of the transaction */ 02706 if(SPI_EndRxTxTransaction(hspi,SPI_DEFAULT_TIMEOUT)!=HAL_OK) 02707 { 02708 hspi->ErrorCode|= HAL_SPI_ERROR_FLAG; 02709 } 02710 02711 /* Clear overrun flag in 2 Lines communication mode because received is not read */ 02712 if(hspi->Init.Direction == SPI_DIRECTION_2LINES) 02713 { 02714 __HAL_SPI_CLEAR_OVRFLAG(hspi); 02715 } 02716 02717 hspi->State = HAL_SPI_STATE_READY; 02718 if(hspi->ErrorCode != HAL_SPI_ERROR_NONE) 02719 { 02720 HAL_SPI_ErrorCallback(hspi); 02721 } 02722 else 02723 { 02724 HAL_SPI_TxCpltCallback(hspi); 02725 } 02726 } 02727 02728 /** 02729 * @} 02730 */ 02731 02732 #endif /* HAL_SPI_MODULE_ENABLED */ 02733 02734 /** 02735 * @} 02736 */ 02737 02738 /** 02739 * @} 02740 */ 02741 02742 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 02743
Generated on Tue Jul 12 2022 11:35:15 by
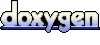