Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_i2c.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_i2c.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief I2C HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Inter Integrated Circuit (I2C) peripheral: 00010 * + Initialization and de-initialization functions 00011 * + IO operation functions 00012 * + Peripheral State and Errors functions 00013 * 00014 @verbatim 00015 ============================================================================== 00016 ##### How to use this driver ##### 00017 ============================================================================== 00018 [..] 00019 The I2C HAL driver can be used as follows: 00020 00021 (#) Declare a I2C_HandleTypeDef handle structure, for example: 00022 I2C_HandleTypeDef hi2c; 00023 00024 (#)Initialize the I2C low level resources by implementing the HAL_I2C_MspInit() API: 00025 (##) Enable the I2Cx interface clock 00026 (##) I2C pins configuration 00027 (+++) Enable the clock for the I2C GPIOs 00028 (+++) Configure I2C pins as alternate function open-drain 00029 (##) NVIC configuration if you need to use interrupt process 00030 (+++) Configure the I2Cx interrupt priority 00031 (+++) Enable the NVIC I2C IRQ Channel 00032 (##) DMA Configuration if you need to use DMA process 00033 (+++) Declare a DMA_HandleTypeDef handle structure for the transmit or receive channel 00034 (+++) Enable the DMAx interface clock using 00035 (+++) Configure the DMA handle parameters 00036 (+++) Configure the DMA Tx or Rx channel 00037 (+++) Associate the initialized DMA handle to the hi2c DMA Tx or Rx handle 00038 (+++) Configure the priority and enable the NVIC for the transfer complete interrupt on 00039 the DMA Tx or Rx channel 00040 00041 (#) Configure the Communication Clock Timing, Own Address1, Master Addressing Mode, Dual Addressing mode, 00042 Own Address2, Own Address2 Mask, General call and Nostretch mode in the hi2c Init structure. 00043 00044 (#) Initialize the I2C registers by calling the HAL_I2C_Init(), configures also the low level Hardware 00045 (GPIO, CLOCK, NVIC...etc) by calling the customized HAL_I2C_MspInit(&hi2c) API. 00046 00047 (#) To check if target device is ready for communication, use the function HAL_I2C_IsDeviceReady() 00048 00049 (#) For I2C IO and IO MEM operations, three operation modes are available within this driver : 00050 00051 *** Polling mode IO operation *** 00052 ================================= 00053 [..] 00054 (+) Transmit in master mode an amount of data in blocking mode using HAL_I2C_Master_Transmit() 00055 (+) Receive in master mode an amount of data in blocking mode using HAL_I2C_Master_Receive() 00056 (+) Transmit in slave mode an amount of data in blocking mode using HAL_I2C_Slave_Transmit() 00057 (+) Receive in slave mode an amount of data in blocking mode using HAL_I2C_Slave_Receive() 00058 00059 *** Polling mode IO MEM operation *** 00060 ===================================== 00061 [..] 00062 (+) Write an amount of data in blocking mode to a specific memory address using HAL_I2C_Mem_Write() 00063 (+) Read an amount of data in blocking mode from a specific memory address using HAL_I2C_Mem_Read() 00064 00065 00066 *** Interrupt mode IO operation *** 00067 =================================== 00068 [..] 00069 (+) Transmit in master mode an amount of data in non-blocking mode using HAL_I2C_Master_Transmit_IT() 00070 (+) At transmission end of transfer HAL_I2C_MasterTxCpltCallback() is executed and user can 00071 add his own code by customization of function pointer HAL_I2C_MasterTxCpltCallback() 00072 (+) Receive in master mode an amount of data in non-blocking mode using HAL_I2C_Master_Receive_IT() 00073 (+) At reception end of transfer HAL_I2C_MasterRxCpltCallback() is executed and user can 00074 add his own code by customization of function pointer HAL_I2C_MasterRxCpltCallback() 00075 (+) Transmit in slave mode an amount of data in non-blocking mode using HAL_I2C_Slave_Transmit_IT() 00076 (+) At transmission end of transfer HAL_I2C_SlaveTxCpltCallback() is executed and user can 00077 add his own code by customization of function pointer HAL_I2C_SlaveTxCpltCallback() 00078 (+) Receive in slave mode an amount of data in non-blocking mode using HAL_I2C_Slave_Receive_IT() 00079 (+) At reception end of transfer HAL_I2C_SlaveRxCpltCallback() is executed and user can 00080 add his own code by customization of function pointer HAL_I2C_SlaveRxCpltCallback() 00081 (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can 00082 add his own code by customization of function pointer HAL_I2C_ErrorCallback() 00083 00084 *** Interrupt mode IO MEM operation *** 00085 ======================================= 00086 [..] 00087 (+) Write an amount of data in non-blocking mode with Interrupt to a specific memory address using 00088 HAL_I2C_Mem_Write_IT() 00089 (+) At MEM end of write transfer HAL_I2C_MemTxCpltCallback() is executed and user can 00090 add his own code by customization of function pointer HAL_I2C_MemTxCpltCallback() 00091 (+) Read an amount of data in non-blocking mode with Interrupt from a specific memory address using 00092 HAL_I2C_Mem_Read_IT() 00093 (+) At MEM end of read transfer HAL_I2C_MemRxCpltCallback() is executed and user can 00094 add his own code by customization of function pointer HAL_I2C_MemRxCpltCallback() 00095 (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can 00096 add his own code by customization of function pointer HAL_I2C_ErrorCallback() 00097 00098 *** DMA mode IO operation *** 00099 ============================== 00100 [..] 00101 (+) Transmit in master mode an amount of data in non-blocking mode (DMA) using 00102 HAL_I2C_Master_Transmit_DMA() 00103 (+) At transmission end of transfer HAL_I2C_MasterTxCpltCallback() is executed and user can 00104 add his own code by customization of function pointer HAL_I2C_MasterTxCpltCallback() 00105 (+) Receive in master mode an amount of data in non-blocking mode (DMA) using 00106 HAL_I2C_Master_Receive_DMA() 00107 (+) At reception end of transfer HAL_I2C_MasterRxCpltCallback() is executed and user can 00108 add his own code by customization of function pointer HAL_I2C_MasterRxCpltCallback() 00109 (+) Transmit in slave mode an amount of data in non-blocking mode (DMA) using 00110 HAL_I2C_Slave_Transmit_DMA() 00111 (+) At transmission end of transfer HAL_I2C_SlaveTxCpltCallback() is executed and user can 00112 add his own code by customization of function pointer HAL_I2C_SlaveTxCpltCallback() 00113 (+) Receive in slave mode an amount of data in non-blocking mode (DMA) using 00114 HAL_I2C_Slave_Receive_DMA() 00115 (+) At reception end of transfer HAL_I2C_SlaveRxCpltCallback() is executed and user can 00116 add his own code by customization of function pointer HAL_I2C_SlaveRxCpltCallback() 00117 (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can 00118 add his own code by customization of function pointer HAL_I2C_ErrorCallback() 00119 00120 *** DMA mode IO MEM operation *** 00121 ================================= 00122 [..] 00123 (+) Write an amount of data in non-blocking mode with DMA to a specific memory address using 00124 HAL_I2C_Mem_Write_DMA() 00125 (+) At MEM end of write transfer HAL_I2C_MemTxCpltCallback() is executed and user can 00126 add his own code by customization of function pointer HAL_I2C_MemTxCpltCallback() 00127 (+) Read an amount of data in non-blocking mode with DMA from a specific memory address using 00128 HAL_I2C_Mem_Read_DMA() 00129 (+) At MEM end of read transfer HAL_I2C_MemRxCpltCallback() is executed and user can 00130 add his own code by customization of function pointer HAL_I2C_MemRxCpltCallback() 00131 (+) In case of transfer Error, HAL_I2C_ErrorCallback() function is executed and user can 00132 add his own code by customization of function pointer HAL_I2C_ErrorCallback() 00133 00134 00135 *** I2C HAL driver macros list *** 00136 ================================== 00137 [..] 00138 Below the list of most used macros in I2C HAL driver. 00139 00140 (+) __HAL_I2C_ENABLE: Enable the I2C peripheral 00141 (+) __HAL_I2C_DISABLE: Disable the I2C peripheral 00142 (+) __HAL_I2C_GET_FLAG : Checks whether the specified I2C flag is set or not 00143 (+) __HAL_I2C_CLEAR_FLAG : Clear the specified I2C pending flag 00144 (+) __HAL_I2C_ENABLE_IT: Enable the specified I2C interrupt 00145 (+) __HAL_I2C_DISABLE_IT: Disable the specified I2C interrupt 00146 00147 [..] 00148 (@) You can refer to the I2C HAL driver header file for more useful macros 00149 00150 @endverbatim 00151 ****************************************************************************** 00152 * @attention 00153 * 00154 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00155 * 00156 * Redistribution and use in source and binary forms, with or without modification, 00157 * are permitted provided that the following conditions are met: 00158 * 1. Redistributions of source code must retain the above copyright notice, 00159 * this list of conditions and the following disclaimer. 00160 * 2. Redistributions in binary form must reproduce the above copyright notice, 00161 * this list of conditions and the following disclaimer in the documentation 00162 * and/or other materials provided with the distribution. 00163 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00164 * may be used to endorse or promote products derived from this software 00165 * without specific prior written permission. 00166 * 00167 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00168 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00169 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00170 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00171 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00172 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00173 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00174 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00175 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00176 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00177 * 00178 ****************************************************************************** 00179 */ 00180 00181 /* Includes ------------------------------------------------------------------*/ 00182 #include "stm32l4xx_hal.h" 00183 00184 /** @addtogroup STM32L4xx_HAL_Driver 00185 * @{ 00186 */ 00187 00188 /** @defgroup I2C I2C 00189 * @brief I2C HAL module driver 00190 * @{ 00191 */ 00192 00193 #ifdef HAL_I2C_MODULE_ENABLED 00194 00195 /* Private typedef -----------------------------------------------------------*/ 00196 /* Private constants ---------------------------------------------------------*/ 00197 /** @addtogroup I2C_Private_Constants I2C Private Constants 00198 * @{ 00199 */ 00200 #define TIMING_CLEAR_MASK ((uint32_t)0xF0FFFFFF) /*<! I2C TIMING clear register Mask */ 00201 #define I2C_TIMEOUT_ADDR ((uint32_t)10000) /* 10 s */ 00202 #define I2C_TIMEOUT_BUSY ((uint32_t)25) /* 25 ms */ 00203 #define I2C_TIMEOUT_DIR ((uint32_t)25) /* 25 ms */ 00204 #define I2C_TIMEOUT_RXNE ((uint32_t)25) /* 25 ms */ 00205 #define I2C_TIMEOUT_STOPF ((uint32_t)25) /* 25 ms */ 00206 #define I2C_TIMEOUT_TC ((uint32_t)25) /* 25 ms */ 00207 #define I2C_TIMEOUT_TCR ((uint32_t)25) /* 25 ms */ 00208 #define I2C_TIMEOUT_TXIS ((uint32_t)25) /* 25 ms */ 00209 #define I2C_TIMEOUT_FLAG ((uint32_t)25) /* 25 ms */ 00210 /** 00211 * @} 00212 */ 00213 00214 /* Private macro -------------------------------------------------------------*/ 00215 /* Private variables ---------------------------------------------------------*/ 00216 /* Private function prototypes -----------------------------------------------*/ 00217 /** @addtogroup I2C_Private_Functions I2C Private Functions 00218 * @{ 00219 */ 00220 static void I2C_DMAMasterTransmitCplt(DMA_HandleTypeDef *hdma); 00221 static void I2C_DMAMasterReceiveCplt(DMA_HandleTypeDef *hdma); 00222 static void I2C_DMASlaveTransmitCplt(DMA_HandleTypeDef *hdma); 00223 static void I2C_DMASlaveReceiveCplt(DMA_HandleTypeDef *hdma); 00224 static void I2C_DMAMemTransmitCplt(DMA_HandleTypeDef *hdma); 00225 static void I2C_DMAMemReceiveCplt(DMA_HandleTypeDef *hdma); 00226 static void I2C_DMAError(DMA_HandleTypeDef *hdma); 00227 00228 static HAL_StatusTypeDef I2C_RequestMemoryWrite(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout); 00229 static HAL_StatusTypeDef I2C_RequestMemoryRead(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout); 00230 static HAL_StatusTypeDef I2C_WaitOnFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Flag, FlagStatus Status, uint32_t Timeout); 00231 static HAL_StatusTypeDef I2C_WaitOnTXISFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout); 00232 static HAL_StatusTypeDef I2C_WaitOnRXNEFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout); 00233 static HAL_StatusTypeDef I2C_WaitOnSTOPFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout); 00234 static HAL_StatusTypeDef I2C_IsAcknowledgeFailed(I2C_HandleTypeDef *hi2c, uint32_t Timeout); 00235 00236 static HAL_StatusTypeDef I2C_MasterTransmit_ISR(I2C_HandleTypeDef *hi2c); 00237 static HAL_StatusTypeDef I2C_MasterReceive_ISR(I2C_HandleTypeDef *hi2c); 00238 00239 static HAL_StatusTypeDef I2C_SlaveTransmit_ISR(I2C_HandleTypeDef *hi2c); 00240 static HAL_StatusTypeDef I2C_SlaveReceive_ISR(I2C_HandleTypeDef *hi2c); 00241 00242 static void I2C_TransferConfig(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t Size, uint32_t Mode, uint32_t Request); 00243 /** 00244 * @} 00245 */ 00246 00247 /* Exported functions --------------------------------------------------------*/ 00248 00249 /** @defgroup I2C_Exported_Functions I2C Exported Functions 00250 * @{ 00251 */ 00252 00253 /** @defgroup I2C_Exported_Functions_Group1 Initialization and de-initialization functions 00254 * @brief Initialization and Configuration functions 00255 * 00256 @verbatim 00257 =============================================================================== 00258 ##### Initialization and de-initialization functions ##### 00259 =============================================================================== 00260 [..] This subsection provides a set of functions allowing to initialize and 00261 deinitialize the I2Cx peripheral: 00262 00263 (+) User must Implement HAL_I2C_MspInit() function in which he configures 00264 all related peripherals resources (CLOCK, GPIO, DMA, IT and NVIC ). 00265 00266 (+) Call the function HAL_I2C_Init() to configure the selected device with 00267 the selected configuration: 00268 (++) Clock Timing 00269 (++) Own Address 1 00270 (++) Addressing mode (Master, Slave) 00271 (++) Dual Addressing mode 00272 (++) Own Address 2 00273 (++) Own Address 2 Mask 00274 (++) General call mode 00275 (++) Nostretch mode 00276 00277 (+) Call the function HAL_I2C_DeInit() to restore the default configuration 00278 of the selected I2Cx peripheral. 00279 00280 @endverbatim 00281 * @{ 00282 */ 00283 00284 /** 00285 * @brief Initializes the I2C according to the specified parameters 00286 * in the I2C_InitTypeDef and initialize the associated handle. 00287 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00288 * the configuration information for the specified I2C. 00289 * @retval HAL status 00290 */ 00291 HAL_StatusTypeDef HAL_I2C_Init(I2C_HandleTypeDef *hi2c) 00292 { 00293 /* Check the I2C handle allocation */ 00294 if(hi2c == NULL) 00295 { 00296 return HAL_ERROR; 00297 } 00298 00299 /* Check the parameters */ 00300 assert_param(IS_I2C_ALL_INSTANCE(hi2c->Instance)); 00301 assert_param(IS_I2C_OWN_ADDRESS1(hi2c->Init.OwnAddress1)); 00302 assert_param(IS_I2C_ADDRESSING_MODE(hi2c->Init.AddressingMode)); 00303 assert_param(IS_I2C_DUAL_ADDRESS(hi2c->Init.DualAddressMode)); 00304 assert_param(IS_I2C_OWN_ADDRESS2(hi2c->Init.OwnAddress2)); 00305 assert_param(IS_I2C_OWN_ADDRESS2_MASK(hi2c->Init.OwnAddress2Masks)); 00306 assert_param(IS_I2C_GENERAL_CALL(hi2c->Init.GeneralCallMode)); 00307 assert_param(IS_I2C_NO_STRETCH(hi2c->Init.NoStretchMode)); 00308 00309 if(hi2c->State == HAL_I2C_STATE_RESET) 00310 { 00311 /* Allocate lock resource and initialize it */ 00312 hi2c->Lock = HAL_UNLOCKED; 00313 00314 /* Init the low level hardware : GPIO, CLOCK, CORTEX...etc */ 00315 HAL_I2C_MspInit(hi2c); 00316 } 00317 00318 hi2c->State = HAL_I2C_STATE_BUSY; 00319 00320 /* Disable the selected I2C peripheral */ 00321 __HAL_I2C_DISABLE(hi2c); 00322 00323 /*---------------------------- I2Cx TIMINGR Configuration ------------------*/ 00324 /* Configure I2Cx: Frequency range */ 00325 hi2c->Instance->TIMINGR = hi2c->Init.Timing & TIMING_CLEAR_MASK; 00326 00327 /*---------------------------- I2Cx OAR1 Configuration ---------------------*/ 00328 /* Configure I2Cx: Own Address1 and ack own address1 mode */ 00329 hi2c->Instance->OAR1 &= ~I2C_OAR1_OA1EN; 00330 if(hi2c->Init.OwnAddress1 != 0) 00331 { 00332 if(hi2c->Init.AddressingMode == I2C_ADDRESSINGMODE_7BIT) 00333 { 00334 hi2c->Instance->OAR1 = (I2C_OAR1_OA1EN | hi2c->Init.OwnAddress1); 00335 } 00336 else /* I2C_ADDRESSINGMODE_10BIT */ 00337 { 00338 hi2c->Instance->OAR1 = (I2C_OAR1_OA1EN | I2C_OAR1_OA1MODE | hi2c->Init.OwnAddress1); 00339 } 00340 } 00341 00342 /*---------------------------- I2Cx CR2 Configuration ----------------------*/ 00343 /* Configure I2Cx: Addressing Master mode */ 00344 if(hi2c->Init.AddressingMode == I2C_ADDRESSINGMODE_10BIT) 00345 { 00346 hi2c->Instance->CR2 = (I2C_CR2_ADD10); 00347 } 00348 /* Enable the AUTOEND by default, and enable NACK (should be disable only during Slave process */ 00349 hi2c->Instance->CR2 |= (I2C_CR2_AUTOEND | I2C_CR2_NACK); 00350 00351 /*---------------------------- I2Cx OAR2 Configuration ---------------------*/ 00352 /* Configure I2Cx: Dual mode and Own Address2 */ 00353 hi2c->Instance->OAR2 = (hi2c->Init.DualAddressMode | hi2c->Init.OwnAddress2 | (hi2c->Init.OwnAddress2Masks << 8)); 00354 00355 /*---------------------------- I2Cx CR1 Configuration ----------------------*/ 00356 /* Configure I2Cx: Generalcall and NoStretch mode */ 00357 hi2c->Instance->CR1 = (hi2c->Init.GeneralCallMode | hi2c->Init.NoStretchMode); 00358 00359 /* Enable the selected I2C peripheral */ 00360 __HAL_I2C_ENABLE(hi2c); 00361 00362 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00363 hi2c->State = HAL_I2C_STATE_READY; 00364 00365 return HAL_OK; 00366 } 00367 00368 /** 00369 * @brief DeInitialize the I2C peripheral. 00370 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00371 * the configuration information for the specified I2C. 00372 * @retval HAL status 00373 */ 00374 HAL_StatusTypeDef HAL_I2C_DeInit(I2C_HandleTypeDef *hi2c) 00375 { 00376 /* Check the I2C handle allocation */ 00377 if(hi2c == NULL) 00378 { 00379 return HAL_ERROR; 00380 } 00381 00382 /* Check the parameters */ 00383 assert_param(IS_I2C_ALL_INSTANCE(hi2c->Instance)); 00384 00385 hi2c->State = HAL_I2C_STATE_BUSY; 00386 00387 /* Disable the I2C Peripheral Clock */ 00388 __HAL_I2C_DISABLE(hi2c); 00389 00390 /* DeInit the low level hardware: GPIO, CLOCK, NVIC */ 00391 HAL_I2C_MspDeInit(hi2c); 00392 00393 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00394 00395 hi2c->State = HAL_I2C_STATE_RESET; 00396 00397 /* Release Lock */ 00398 __HAL_UNLOCK(hi2c); 00399 00400 return HAL_OK; 00401 } 00402 00403 /** 00404 * @brief Initialize the I2C MSP. 00405 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00406 * the configuration information for the specified I2C. 00407 * @retval None 00408 */ 00409 __weak void HAL_I2C_MspInit(I2C_HandleTypeDef *hi2c) 00410 { 00411 /* NOTE : This function should not be modified, when the callback is needed, 00412 the HAL_I2C_MspInit could be implemented in the user file 00413 */ 00414 } 00415 00416 /** 00417 * @brief DeInitialize the I2C MSP. 00418 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00419 * the configuration information for the specified I2C. 00420 * @retval None 00421 */ 00422 __weak void HAL_I2C_MspDeInit(I2C_HandleTypeDef *hi2c) 00423 { 00424 /* NOTE : This function should not be modified, when the callback is needed, 00425 the HAL_I2C_MspDeInit could be implemented in the user file 00426 */ 00427 } 00428 00429 /** 00430 * @} 00431 */ 00432 00433 /** @defgroup I2C_Exported_Functions_Group2 Input and Output operation functions 00434 * @brief Data transfers functions 00435 * 00436 @verbatim 00437 =============================================================================== 00438 ##### IO operation functions ##### 00439 =============================================================================== 00440 [..] 00441 This subsection provides a set of functions allowing to manage the I2C data 00442 transfers. 00443 00444 (#) There are two modes of transfer: 00445 (++) Blocking mode : The communication is performed in the polling mode. 00446 The status of all data processing is returned by the same function 00447 after finishing transfer. 00448 (++) No-Blocking mode : The communication is performed using Interrupts 00449 or DMA. These functions return the status of the transfer startup. 00450 The end of the data processing will be indicated through the 00451 dedicated I2C IRQ when using Interrupt mode or the DMA IRQ when 00452 using DMA mode. 00453 00454 (#) Blocking mode functions are : 00455 (++) HAL_I2C_Master_Transmit() 00456 (++) HAL_I2C_Master_Receive() 00457 (++) HAL_I2C_Slave_Transmit() 00458 (++) HAL_I2C_Slave_Receive() 00459 (++) HAL_I2C_Mem_Write() 00460 (++) HAL_I2C_Mem_Read() 00461 (++) HAL_I2C_IsDeviceReady() 00462 00463 (#) No-Blocking mode functions with Interrupt are : 00464 (++) HAL_I2C_Master_Transmit_IT() 00465 (++) HAL_I2C_Master_Receive_IT() 00466 (++) HAL_I2C_Slave_Transmit_IT() 00467 (++) HAL_I2C_Slave_Receive_IT() 00468 (++) HAL_I2C_Mem_Write_IT() 00469 (++) HAL_I2C_Mem_Read_IT() 00470 00471 (#) No-Blocking mode functions with DMA are : 00472 (++) HAL_I2C_Master_Transmit_DMA() 00473 (++) HAL_I2C_Master_Receive_DMA() 00474 (++) HAL_I2C_Slave_Transmit_DMA() 00475 (++) HAL_I2C_Slave_Receive_DMA() 00476 (++) HAL_I2C_Mem_Write_DMA() 00477 (++) HAL_I2C_Mem_Read_DMA() 00478 00479 (#) A set of Transfer Complete Callbacks are provided in non Blocking mode: 00480 (++) HAL_I2C_MemTxCpltCallback() 00481 (++) HAL_I2C_MemRxCpltCallback() 00482 (++) HAL_I2C_MasterTxCpltCallback() 00483 (++) HAL_I2C_MasterRxCpltCallback() 00484 (++) HAL_I2C_SlaveTxCpltCallback() 00485 (++) HAL_I2C_SlaveRxCpltCallback() 00486 (++) HAL_I2C_ErrorCallback() 00487 00488 @endverbatim 00489 * @{ 00490 */ 00491 00492 /** 00493 * @brief Transmits in master mode an amount of data in blocking mode. 00494 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00495 * the configuration information for the specified I2C. 00496 * @param DevAddress: Target device address 00497 * @param pData: Pointer to data buffer 00498 * @param Size: Amount of data to be sent 00499 * @param Timeout: Timeout duration 00500 * @retval HAL status 00501 */ 00502 HAL_StatusTypeDef HAL_I2C_Master_Transmit(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00503 { 00504 uint32_t sizetmp = 0; 00505 00506 if(hi2c->State == HAL_I2C_STATE_READY) 00507 { 00508 if((pData == NULL ) || (Size == 0)) 00509 { 00510 return HAL_ERROR; 00511 } 00512 00513 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 00514 { 00515 return HAL_BUSY; 00516 } 00517 00518 /* Process Locked */ 00519 __HAL_LOCK(hi2c); 00520 00521 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_TX; 00522 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00523 00524 /* Send Slave Address */ 00525 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 00526 /* Size > 255, need to set RELOAD bit */ 00527 if(Size > 255) 00528 { 00529 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_GENERATE_START_WRITE); 00530 sizetmp = 255; 00531 } 00532 else 00533 { 00534 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_GENERATE_START_WRITE); 00535 sizetmp = Size; 00536 } 00537 00538 do 00539 { 00540 /* Wait until TXIS flag is set */ 00541 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 00542 { 00543 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00544 { 00545 return HAL_ERROR; 00546 } 00547 else 00548 { 00549 return HAL_TIMEOUT; 00550 } 00551 } 00552 /* Write data to TXDR */ 00553 hi2c->Instance->TXDR = (*pData++); 00554 sizetmp--; 00555 Size--; 00556 00557 if((sizetmp == 0)&&(Size!=0)) 00558 { 00559 /* Wait until TXE flag is set */ 00560 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, Timeout) != HAL_OK) 00561 { 00562 return HAL_TIMEOUT; 00563 } 00564 00565 if(Size > 255) 00566 { 00567 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 00568 sizetmp = 255; 00569 } 00570 else 00571 { 00572 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 00573 sizetmp = Size; 00574 } 00575 } 00576 00577 }while(Size > 0); 00578 00579 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 00580 /* Wait until STOPF flag is set */ 00581 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 00582 { 00583 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00584 { 00585 return HAL_ERROR; 00586 } 00587 else 00588 { 00589 return HAL_TIMEOUT; 00590 } 00591 } 00592 00593 /* Clear STOP Flag */ 00594 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 00595 00596 /* Clear Configuration Register 2 */ 00597 I2C_RESET_CR2(hi2c); 00598 00599 hi2c->State = HAL_I2C_STATE_READY; 00600 00601 /* Process Unlocked */ 00602 __HAL_UNLOCK(hi2c); 00603 00604 return HAL_OK; 00605 } 00606 else 00607 { 00608 return HAL_BUSY; 00609 } 00610 } 00611 00612 /** 00613 * @brief Receives in master mode an amount of data in blocking mode. 00614 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00615 * the configuration information for the specified I2C. 00616 * @param DevAddress: Target device address 00617 * @param pData: Pointer to data buffer 00618 * @param Size: Amount of data to be sent 00619 * @param Timeout: Timeout duration 00620 * @retval HAL status 00621 */ 00622 HAL_StatusTypeDef HAL_I2C_Master_Receive(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00623 { 00624 uint32_t sizetmp = 0; 00625 00626 if(hi2c->State == HAL_I2C_STATE_READY) 00627 { 00628 if((pData == NULL ) || (Size == 0)) 00629 { 00630 return HAL_ERROR; 00631 } 00632 00633 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 00634 { 00635 return HAL_BUSY; 00636 } 00637 00638 /* Process Locked */ 00639 __HAL_LOCK(hi2c); 00640 00641 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_RX; 00642 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00643 00644 /* Send Slave Address */ 00645 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 00646 /* Size > 255, need to set RELOAD bit */ 00647 if(Size > 255) 00648 { 00649 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 00650 sizetmp = 255; 00651 } 00652 else 00653 { 00654 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 00655 sizetmp = Size; 00656 } 00657 00658 do 00659 { 00660 /* Wait until RXNE flag is set */ 00661 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, Timeout) != HAL_OK) 00662 { 00663 return HAL_TIMEOUT; 00664 } 00665 00666 /* Write data to RXDR */ 00667 (*pData++) =hi2c->Instance->RXDR; 00668 sizetmp--; 00669 Size--; 00670 00671 if((sizetmp == 0)&&(Size!=0)) 00672 { 00673 /* Wait until TCR flag is set */ 00674 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, Timeout) != HAL_OK) 00675 { 00676 return HAL_TIMEOUT; 00677 } 00678 00679 if(Size > 255) 00680 { 00681 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 00682 sizetmp = 255; 00683 } 00684 else 00685 { 00686 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 00687 sizetmp = Size; 00688 } 00689 } 00690 00691 }while(Size > 0); 00692 00693 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 00694 /* Wait until STOPF flag is set */ 00695 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 00696 { 00697 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00698 { 00699 return HAL_ERROR; 00700 } 00701 else 00702 { 00703 return HAL_TIMEOUT; 00704 } 00705 } 00706 00707 /* Clear STOP Flag */ 00708 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 00709 00710 /* Clear Configuration Register 2 */ 00711 I2C_RESET_CR2(hi2c); 00712 00713 hi2c->State = HAL_I2C_STATE_READY; 00714 00715 /* Process Unlocked */ 00716 __HAL_UNLOCK(hi2c); 00717 00718 return HAL_OK; 00719 } 00720 else 00721 { 00722 return HAL_BUSY; 00723 } 00724 } 00725 00726 /** 00727 * @brief Transmits in slave mode an amount of data in blocking mode. 00728 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00729 * the configuration information for the specified I2C. 00730 * @param pData: Pointer to data buffer 00731 * @param Size: Amount of data to be sent 00732 * @param Timeout: Timeout duration 00733 * @retval HAL status 00734 */ 00735 HAL_StatusTypeDef HAL_I2C_Slave_Transmit(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00736 { 00737 if(hi2c->State == HAL_I2C_STATE_READY) 00738 { 00739 if((pData == NULL ) || (Size == 0)) 00740 { 00741 return HAL_ERROR; 00742 } 00743 00744 /* Process Locked */ 00745 __HAL_LOCK(hi2c); 00746 00747 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_RX; 00748 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00749 00750 /* Enable Address Acknowledge */ 00751 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 00752 00753 /* Wait until ADDR flag is set */ 00754 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, Timeout) != HAL_OK) 00755 { 00756 /* Disable Address Acknowledge */ 00757 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00758 return HAL_TIMEOUT; 00759 } 00760 00761 /* Clear ADDR flag */ 00762 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 00763 00764 /* If 10bit addressing mode is selected */ 00765 if(hi2c->Init.AddressingMode == I2C_ADDRESSINGMODE_10BIT) 00766 { 00767 /* Wait until ADDR flag is set */ 00768 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, Timeout) != HAL_OK) 00769 { 00770 /* Disable Address Acknowledge */ 00771 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00772 return HAL_TIMEOUT; 00773 } 00774 00775 /* Clear ADDR flag */ 00776 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 00777 } 00778 00779 /* Wait until DIR flag is set Transmitter mode */ 00780 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_DIR, RESET, Timeout) != HAL_OK) 00781 { 00782 /* Disable Address Acknowledge */ 00783 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00784 return HAL_TIMEOUT; 00785 } 00786 00787 do 00788 { 00789 /* Wait until TXIS flag is set */ 00790 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 00791 { 00792 /* Disable Address Acknowledge */ 00793 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00794 00795 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00796 { 00797 return HAL_ERROR; 00798 } 00799 else 00800 { 00801 return HAL_TIMEOUT; 00802 } 00803 } 00804 00805 /* Read data from TXDR */ 00806 hi2c->Instance->TXDR = (*pData++); 00807 Size--; 00808 }while(Size > 0); 00809 00810 /* Wait until STOP flag is set */ 00811 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 00812 { 00813 /* Disable Address Acknowledge */ 00814 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00815 00816 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00817 { 00818 /* Normal use case for Transmitter mode */ 00819 /* A NACK is generated to confirm the end of transfer */ 00820 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00821 } 00822 else 00823 { 00824 return HAL_TIMEOUT; 00825 } 00826 } 00827 00828 /* Clear STOP flag */ 00829 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_STOPF); 00830 00831 /* Wait until BUSY flag is reset */ 00832 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_BUSY, SET, Timeout) != HAL_OK) 00833 { 00834 /* Disable Address Acknowledge */ 00835 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00836 return HAL_TIMEOUT; 00837 } 00838 00839 /* Disable Address Acknowledge */ 00840 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00841 00842 hi2c->State = HAL_I2C_STATE_READY; 00843 00844 /* Process Unlocked */ 00845 __HAL_UNLOCK(hi2c); 00846 00847 return HAL_OK; 00848 } 00849 else 00850 { 00851 return HAL_BUSY; 00852 } 00853 } 00854 00855 /** 00856 * @brief Receive in slave mode an amount of data in blocking mode 00857 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00858 * the configuration information for the specified I2C. 00859 * @param pData: Pointer to data buffer 00860 * @param Size: Amount of data to be sent 00861 * @param Timeout: Timeout duration 00862 * @retval HAL status 00863 */ 00864 HAL_StatusTypeDef HAL_I2C_Slave_Receive(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size, uint32_t Timeout) 00865 { 00866 if(hi2c->State == HAL_I2C_STATE_READY) 00867 { 00868 if((pData == NULL ) || (Size == 0)) 00869 { 00870 return HAL_ERROR; 00871 } 00872 00873 /* Process Locked */ 00874 __HAL_LOCK(hi2c); 00875 00876 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_RX; 00877 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00878 00879 /* Enable Address Acknowledge */ 00880 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 00881 00882 /* Wait until ADDR flag is set */ 00883 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, Timeout) != HAL_OK) 00884 { 00885 /* Disable Address Acknowledge */ 00886 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00887 return HAL_TIMEOUT; 00888 } 00889 00890 /* Clear ADDR flag */ 00891 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 00892 00893 /* Wait until DIR flag is reset Receiver mode */ 00894 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_DIR, SET, Timeout) != HAL_OK) 00895 { 00896 /* Disable Address Acknowledge */ 00897 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00898 return HAL_TIMEOUT; 00899 } 00900 00901 while(Size > 0) 00902 { 00903 /* Wait until RXNE flag is set */ 00904 if(I2C_WaitOnRXNEFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 00905 { 00906 /* Disable Address Acknowledge */ 00907 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00908 if(hi2c->ErrorCode == HAL_I2C_ERROR_TIMEOUT) 00909 { 00910 return HAL_TIMEOUT; 00911 } 00912 else 00913 { 00914 return HAL_ERROR; 00915 } 00916 } 00917 00918 /* Read data from RXDR */ 00919 (*pData++) = hi2c->Instance->RXDR; 00920 Size--; 00921 } 00922 00923 /* Wait until STOP flag is set */ 00924 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 00925 { 00926 /* Disable Address Acknowledge */ 00927 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00928 00929 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 00930 { 00931 return HAL_ERROR; 00932 } 00933 else 00934 { 00935 return HAL_TIMEOUT; 00936 } 00937 } 00938 00939 /* Clear STOP flag */ 00940 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_STOPF); 00941 00942 /* Wait until BUSY flag is reset */ 00943 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_BUSY, SET, Timeout) != HAL_OK) 00944 { 00945 /* Disable Address Acknowledge */ 00946 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00947 return HAL_TIMEOUT; 00948 } 00949 00950 00951 /* Disable Address Acknowledge */ 00952 hi2c->Instance->CR2 |= I2C_CR2_NACK; 00953 00954 hi2c->State = HAL_I2C_STATE_READY; 00955 00956 /* Process Unlocked */ 00957 __HAL_UNLOCK(hi2c); 00958 00959 return HAL_OK; 00960 } 00961 else 00962 { 00963 return HAL_BUSY; 00964 } 00965 } 00966 00967 /** 00968 * @brief Transmit in master mode an amount of data in non-blocking mode with Interrupt 00969 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 00970 * the configuration information for the specified I2C. 00971 * @param DevAddress: Target device address 00972 * @param pData: Pointer to data buffer 00973 * @param Size: Amount of data to be sent 00974 * @retval HAL status 00975 */ 00976 HAL_StatusTypeDef HAL_I2C_Master_Transmit_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) 00977 { 00978 if(hi2c->State == HAL_I2C_STATE_READY) 00979 { 00980 if((pData == NULL) || (Size == 0)) 00981 { 00982 return HAL_ERROR; 00983 } 00984 00985 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 00986 { 00987 return HAL_BUSY; 00988 } 00989 00990 /* Process Locked */ 00991 __HAL_LOCK(hi2c); 00992 00993 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_TX; 00994 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 00995 00996 hi2c->pBuffPtr = pData; 00997 hi2c->XferCount = Size; 00998 if(Size > 255) 00999 { 01000 hi2c->XferSize = 255; 01001 } 01002 else 01003 { 01004 hi2c->XferSize = Size; 01005 } 01006 01007 /* Send Slave Address */ 01008 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01009 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01010 { 01011 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_WRITE); 01012 } 01013 else 01014 { 01015 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_WRITE); 01016 } 01017 01018 /* Process Unlocked */ 01019 __HAL_UNLOCK(hi2c); 01020 01021 /* Note : The I2C interrupts must be enabled after unlocking current process 01022 to avoid the risk of I2C interrupt handle execution before current 01023 process unlock */ 01024 01025 01026 /* Enable ERR, TC, STOP, NACK, TXI interrupt */ 01027 /* possible to enable all of these */ 01028 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01029 __HAL_I2C_ENABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_TXI ); 01030 01031 return HAL_OK; 01032 } 01033 else 01034 { 01035 return HAL_BUSY; 01036 } 01037 } 01038 01039 /** 01040 * @brief Receive in master mode an amount of data in non-blocking mode with Interrupt 01041 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01042 * the configuration information for the specified I2C. 01043 * @param DevAddress: Target device address 01044 * @param pData: Pointer to data buffer 01045 * @param Size: Amount of data to be sent 01046 * @retval HAL status 01047 */ 01048 HAL_StatusTypeDef HAL_I2C_Master_Receive_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) 01049 { 01050 if(hi2c->State == HAL_I2C_STATE_READY) 01051 { 01052 if((pData == NULL) || (Size == 0)) 01053 { 01054 return HAL_ERROR; 01055 } 01056 01057 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01058 { 01059 return HAL_BUSY; 01060 } 01061 01062 /* Process Locked */ 01063 __HAL_LOCK(hi2c); 01064 01065 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_RX; 01066 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01067 01068 hi2c->pBuffPtr = pData; 01069 hi2c->XferCount = Size; 01070 if(Size > 255) 01071 { 01072 hi2c->XferSize = 255; 01073 } 01074 else 01075 { 01076 hi2c->XferSize = Size; 01077 } 01078 01079 /* Send Slave Address */ 01080 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01081 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01082 { 01083 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 01084 } 01085 else 01086 { 01087 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 01088 } 01089 01090 /* Process Unlocked */ 01091 __HAL_UNLOCK(hi2c); 01092 01093 /* Note : The I2C interrupts must be enabled after unlocking current process 01094 to avoid the risk of I2C interrupt handle execution before current 01095 process unlock */ 01096 01097 /* Enable ERR, TC, STOP, NACK, RXI interrupt */ 01098 /* possible to enable all of these */ 01099 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01100 __HAL_I2C_ENABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI | I2C_IT_STOPI | I2C_IT_NACKI | I2C_IT_RXI ); 01101 01102 return HAL_OK; 01103 } 01104 else 01105 { 01106 return HAL_BUSY; 01107 } 01108 } 01109 01110 /** 01111 * @brief Transmit in slave mode an amount of data in non-blocking mode with Interrupt 01112 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01113 * the configuration information for the specified I2C. 01114 * @param pData: Pointer to data buffer 01115 * @param Size: Amount of data to be sent 01116 * @retval HAL status 01117 */ 01118 HAL_StatusTypeDef HAL_I2C_Slave_Transmit_IT(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) 01119 { 01120 if(hi2c->State == HAL_I2C_STATE_READY) 01121 { 01122 if((pData == NULL) || (Size == 0)) 01123 { 01124 return HAL_ERROR; 01125 } 01126 01127 /* Process Locked */ 01128 __HAL_LOCK(hi2c); 01129 01130 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_TX; 01131 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01132 01133 /* Enable Address Acknowledge */ 01134 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 01135 01136 hi2c->pBuffPtr = pData; 01137 hi2c->XferSize = Size; 01138 hi2c->XferCount = Size; 01139 01140 /* Process Unlocked */ 01141 __HAL_UNLOCK(hi2c); 01142 01143 /* Note : The I2C interrupts must be enabled after unlocking current process 01144 to avoid the risk of I2C interrupt handle execution before current 01145 process unlock */ 01146 01147 /* Enable ERR, TC, STOP, NACK, TXI interrupt */ 01148 /* possible to enable all of these */ 01149 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01150 __HAL_I2C_ENABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI | I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_TXI ); 01151 01152 return HAL_OK; 01153 } 01154 else 01155 { 01156 return HAL_BUSY; 01157 } 01158 } 01159 01160 /** 01161 * @brief Receive in slave mode an amount of data in non-blocking mode with Interrupt 01162 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01163 * the configuration information for the specified I2C. 01164 * @param pData: Pointer to data buffer 01165 * @param Size: Amount of data to be sent 01166 * @retval HAL status 01167 */ 01168 HAL_StatusTypeDef HAL_I2C_Slave_Receive_IT(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) 01169 { 01170 if(hi2c->State == HAL_I2C_STATE_READY) 01171 { 01172 if((pData == NULL) || (Size == 0)) 01173 { 01174 return HAL_ERROR; 01175 } 01176 01177 /* Process Locked */ 01178 __HAL_LOCK(hi2c); 01179 01180 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_RX; 01181 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01182 01183 /* Enable Address Acknowledge */ 01184 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 01185 01186 hi2c->pBuffPtr = pData; 01187 hi2c->XferSize = Size; 01188 hi2c->XferCount = Size; 01189 01190 /* Process Unlocked */ 01191 __HAL_UNLOCK(hi2c); 01192 01193 /* Note : The I2C interrupts must be enabled after unlocking current process 01194 to avoid the risk of I2C interrupt handle execution before current 01195 process unlock */ 01196 01197 /* Enable ERR, TC, STOP, NACK, RXI interrupt */ 01198 /* possible to enable all of these */ 01199 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01200 __HAL_I2C_ENABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI | I2C_IT_STOPI | I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI); 01201 01202 return HAL_OK; 01203 } 01204 else 01205 { 01206 return HAL_BUSY; 01207 } 01208 } 01209 01210 /** 01211 * @brief Transmit in master mode an amount of data in non-blocking mode with DMA 01212 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01213 * the configuration information for the specified I2C. 01214 * @param DevAddress: Target device address 01215 * @param pData: Pointer to data buffer 01216 * @param Size: Amount of data to be sent 01217 * @retval HAL status 01218 */ 01219 HAL_StatusTypeDef HAL_I2C_Master_Transmit_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) 01220 { 01221 if(hi2c->State == HAL_I2C_STATE_READY) 01222 { 01223 if((pData == NULL) || (Size == 0)) 01224 { 01225 return HAL_ERROR; 01226 } 01227 01228 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01229 { 01230 return HAL_BUSY; 01231 } 01232 01233 /* Process Locked */ 01234 __HAL_LOCK(hi2c); 01235 01236 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_TX; 01237 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01238 01239 hi2c->pBuffPtr = pData; 01240 hi2c->XferCount = Size; 01241 if(Size > 255) 01242 { 01243 hi2c->XferSize = 255; 01244 } 01245 else 01246 { 01247 hi2c->XferSize = Size; 01248 } 01249 01250 /* Set the I2C DMA transfer complete callback */ 01251 hi2c->hdmatx->XferCpltCallback = I2C_DMAMasterTransmitCplt; 01252 01253 /* Set the DMA error callback */ 01254 hi2c->hdmatx->XferErrorCallback = I2C_DMAError; 01255 01256 /* Enable the DMA channel */ 01257 HAL_DMA_Start_IT(hi2c->hdmatx, (uint32_t)pData, (uint32_t)&hi2c->Instance->TXDR, hi2c->XferSize); 01258 01259 /* Send Slave Address */ 01260 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01261 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01262 { 01263 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_WRITE); 01264 } 01265 else 01266 { 01267 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_WRITE); 01268 } 01269 01270 /* Wait until TXIS flag is set */ 01271 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, I2C_TIMEOUT_TXIS) != HAL_OK) 01272 { 01273 /* Disable Address Acknowledge */ 01274 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01275 01276 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01277 { 01278 return HAL_ERROR; 01279 } 01280 else 01281 { 01282 return HAL_TIMEOUT; 01283 } 01284 } 01285 01286 01287 /* Enable DMA Request */ 01288 hi2c->Instance->CR1 |= I2C_CR1_TXDMAEN; 01289 01290 /* Process Unlocked */ 01291 __HAL_UNLOCK(hi2c); 01292 01293 return HAL_OK; 01294 } 01295 else 01296 { 01297 return HAL_BUSY; 01298 } 01299 } 01300 01301 /** 01302 * @brief Receive in master mode an amount of data in non-blocking mode with DMA 01303 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01304 * the configuration information for the specified I2C. 01305 * @param DevAddress: Target device address 01306 * @param pData: Pointer to data buffer 01307 * @param Size: Amount of data to be sent 01308 * @retval HAL status 01309 */ 01310 HAL_StatusTypeDef HAL_I2C_Master_Receive_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t *pData, uint16_t Size) 01311 { 01312 if(hi2c->State == HAL_I2C_STATE_READY) 01313 { 01314 if((pData == NULL) || (Size == 0)) 01315 { 01316 return HAL_ERROR; 01317 } 01318 01319 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01320 { 01321 return HAL_BUSY; 01322 } 01323 01324 /* Process Locked */ 01325 __HAL_LOCK(hi2c); 01326 01327 hi2c->State = HAL_I2C_STATE_MASTER_BUSY_RX; 01328 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01329 01330 hi2c->pBuffPtr = pData; 01331 hi2c->XferCount = Size; 01332 if(Size > 255) 01333 { 01334 hi2c->XferSize = 255; 01335 } 01336 else 01337 { 01338 hi2c->XferSize = Size; 01339 } 01340 01341 /* Set the I2C DMA transfer complete callback */ 01342 hi2c->hdmarx->XferCpltCallback = I2C_DMAMasterReceiveCplt; 01343 01344 /* Set the DMA error callback */ 01345 hi2c->hdmarx->XferErrorCallback = I2C_DMAError; 01346 01347 /* Enable the DMA channel */ 01348 HAL_DMA_Start_IT(hi2c->hdmarx, (uint32_t)&hi2c->Instance->RXDR, (uint32_t)pData, hi2c->XferSize); 01349 01350 /* Send Slave Address */ 01351 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01352 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01353 { 01354 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 01355 } 01356 else 01357 { 01358 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 01359 } 01360 01361 /* Wait until RXNE flag is set */ 01362 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, I2C_TIMEOUT_RXNE) != HAL_OK) 01363 { 01364 return HAL_TIMEOUT; 01365 } 01366 01367 01368 /* Enable DMA Request */ 01369 hi2c->Instance->CR1 |= I2C_CR1_RXDMAEN; 01370 01371 /* Process Unlocked */ 01372 __HAL_UNLOCK(hi2c); 01373 01374 return HAL_OK; 01375 } 01376 else 01377 { 01378 return HAL_BUSY; 01379 } 01380 } 01381 01382 /** 01383 * @brief Transmit in slave mode an amount of data in non-blocking mode with DMA 01384 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01385 * the configuration information for the specified I2C. 01386 * @param pData: Pointer to data buffer 01387 * @param Size: Amount of data to be sent 01388 * @retval HAL status 01389 */ 01390 HAL_StatusTypeDef HAL_I2C_Slave_Transmit_DMA(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) 01391 { 01392 if(hi2c->State == HAL_I2C_STATE_READY) 01393 { 01394 if((pData == NULL) || (Size == 0)) 01395 { 01396 return HAL_ERROR; 01397 } 01398 /* Process Locked */ 01399 __HAL_LOCK(hi2c); 01400 01401 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_TX; 01402 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01403 01404 hi2c->pBuffPtr = pData; 01405 hi2c->XferCount = Size; 01406 hi2c->XferSize = Size; 01407 01408 /* Set the I2C DMA transfer complete callback */ 01409 hi2c->hdmatx->XferCpltCallback = I2C_DMASlaveTransmitCplt; 01410 01411 /* Set the DMA error callback */ 01412 hi2c->hdmatx->XferErrorCallback = I2C_DMAError; 01413 01414 /* Enable the DMA channel */ 01415 HAL_DMA_Start_IT(hi2c->hdmatx, (uint32_t)pData, (uint32_t)&hi2c->Instance->TXDR, hi2c->XferSize); 01416 01417 /* Enable Address Acknowledge */ 01418 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 01419 01420 /* Wait until ADDR flag is set */ 01421 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, I2C_TIMEOUT_ADDR) != HAL_OK) 01422 { 01423 /* Disable Address Acknowledge */ 01424 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01425 return HAL_TIMEOUT; 01426 } 01427 01428 /* Clear ADDR flag */ 01429 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 01430 01431 /* If 10bits addressing mode is selected */ 01432 if(hi2c->Init.AddressingMode == I2C_ADDRESSINGMODE_10BIT) 01433 { 01434 /* Wait until ADDR flag is set */ 01435 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, I2C_TIMEOUT_ADDR) != HAL_OK) 01436 { 01437 /* Disable Address Acknowledge */ 01438 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01439 return HAL_TIMEOUT; 01440 } 01441 01442 /* Clear ADDR flag */ 01443 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 01444 } 01445 01446 /* Wait until DIR flag is set Transmitter mode */ 01447 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_DIR, RESET, I2C_TIMEOUT_BUSY) != HAL_OK) 01448 { 01449 /* Disable Address Acknowledge */ 01450 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01451 return HAL_TIMEOUT; 01452 } 01453 01454 /* Enable DMA Request */ 01455 hi2c->Instance->CR1 |= I2C_CR1_TXDMAEN; 01456 01457 /* Process Unlocked */ 01458 __HAL_UNLOCK(hi2c); 01459 01460 return HAL_OK; 01461 } 01462 else 01463 { 01464 return HAL_BUSY; 01465 } 01466 } 01467 01468 /** 01469 * @brief Receive in slave mode an amount of data in non-blocking mode with DMA 01470 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01471 * the configuration information for the specified I2C. 01472 * @param pData: Pointer to data buffer 01473 * @param Size: Amount of data to be sent 01474 * @retval HAL status 01475 */ 01476 HAL_StatusTypeDef HAL_I2C_Slave_Receive_DMA(I2C_HandleTypeDef *hi2c, uint8_t *pData, uint16_t Size) 01477 { 01478 if(hi2c->State == HAL_I2C_STATE_READY) 01479 { 01480 if((pData == NULL) || (Size == 0)) 01481 { 01482 return HAL_ERROR; 01483 } 01484 /* Process Locked */ 01485 __HAL_LOCK(hi2c); 01486 01487 hi2c->State = HAL_I2C_STATE_SLAVE_BUSY_RX; 01488 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01489 01490 hi2c->pBuffPtr = pData; 01491 hi2c->XferSize = Size; 01492 hi2c->XferCount = Size; 01493 01494 /* Set the I2C DMA transfer complete callback */ 01495 hi2c->hdmarx->XferCpltCallback = I2C_DMASlaveReceiveCplt; 01496 01497 /* Set the DMA error callback */ 01498 hi2c->hdmarx->XferErrorCallback = I2C_DMAError; 01499 01500 /* Enable the DMA channel */ 01501 HAL_DMA_Start_IT(hi2c->hdmarx, (uint32_t)&hi2c->Instance->RXDR, (uint32_t)pData, Size); 01502 01503 /* Enable Address Acknowledge */ 01504 hi2c->Instance->CR2 &= ~I2C_CR2_NACK; 01505 01506 /* Wait until ADDR flag is set */ 01507 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_ADDR, RESET, I2C_TIMEOUT_ADDR) != HAL_OK) 01508 { 01509 /* Disable Address Acknowledge */ 01510 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01511 return HAL_TIMEOUT; 01512 } 01513 01514 /* Clear ADDR flag */ 01515 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_ADDR); 01516 01517 /* Wait until DIR flag is set Receiver mode */ 01518 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_DIR, SET, I2C_TIMEOUT_DIR) != HAL_OK) 01519 { 01520 /* Disable Address Acknowledge */ 01521 hi2c->Instance->CR2 |= I2C_CR2_NACK; 01522 return HAL_TIMEOUT; 01523 } 01524 01525 /* Enable DMA Request */ 01526 hi2c->Instance->CR1 |= I2C_CR1_RXDMAEN; 01527 01528 /* Process Unlocked */ 01529 __HAL_UNLOCK(hi2c); 01530 01531 return HAL_OK; 01532 } 01533 else 01534 { 01535 return HAL_BUSY; 01536 } 01537 } 01538 /** 01539 * @brief Write an amount of data in blocking mode to a specific memory address 01540 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01541 * the configuration information for the specified I2C. 01542 * @param DevAddress: Target device address 01543 * @param MemAddress: Internal memory address 01544 * @param MemAddSize: Size of internal memory address 01545 * @param pData: Pointer to data buffer 01546 * @param Size: Amount of data to be sent 01547 * @param Timeout: Timeout duration 01548 * @retval HAL status 01549 */ 01550 HAL_StatusTypeDef HAL_I2C_Mem_Write(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout) 01551 { 01552 uint32_t Sizetmp = 0; 01553 01554 /* Check the parameters */ 01555 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 01556 01557 if(hi2c->State == HAL_I2C_STATE_READY) 01558 { 01559 if((pData == NULL) || (Size == 0)) 01560 { 01561 return HAL_ERROR; 01562 } 01563 01564 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01565 { 01566 return HAL_BUSY; 01567 } 01568 01569 /* Process Locked */ 01570 __HAL_LOCK(hi2c); 01571 01572 hi2c->State = HAL_I2C_STATE_MEM_BUSY_TX; 01573 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01574 01575 /* Send Slave Address and Memory Address */ 01576 if(I2C_RequestMemoryWrite(hi2c, DevAddress, MemAddress, MemAddSize, Timeout) != HAL_OK) 01577 { 01578 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01579 { 01580 /* Process Unlocked */ 01581 __HAL_UNLOCK(hi2c); 01582 return HAL_ERROR; 01583 } 01584 else 01585 { 01586 /* Process Unlocked */ 01587 __HAL_UNLOCK(hi2c); 01588 return HAL_TIMEOUT; 01589 } 01590 } 01591 01592 /* Set NBYTES to write and reload if size > 255 */ 01593 /* Size > 255, need to set RELOAD bit */ 01594 if(Size > 255) 01595 { 01596 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 01597 Sizetmp = 255; 01598 } 01599 else 01600 { 01601 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 01602 Sizetmp = Size; 01603 } 01604 01605 do 01606 { 01607 /* Wait until TXIS flag is set */ 01608 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 01609 { 01610 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01611 { 01612 return HAL_ERROR; 01613 } 01614 else 01615 { 01616 return HAL_TIMEOUT; 01617 } 01618 } 01619 01620 /* Write data to DR */ 01621 hi2c->Instance->TXDR = (*pData++); 01622 Sizetmp--; 01623 Size--; 01624 01625 if((Sizetmp == 0)&&(Size!=0)) 01626 { 01627 /* Wait until TCR flag is set */ 01628 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, Timeout) != HAL_OK) 01629 { 01630 return HAL_TIMEOUT; 01631 } 01632 01633 01634 if(Size > 255) 01635 { 01636 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 01637 Sizetmp = 255; 01638 } 01639 else 01640 { 01641 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 01642 Sizetmp = Size; 01643 } 01644 } 01645 01646 }while(Size > 0); 01647 01648 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 01649 /* Wait until STOPF flag is reset */ 01650 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 01651 { 01652 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01653 { 01654 return HAL_ERROR; 01655 } 01656 else 01657 { 01658 return HAL_TIMEOUT; 01659 } 01660 } 01661 01662 /* Clear STOP Flag */ 01663 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 01664 01665 /* Clear Configuration Register 2 */ 01666 I2C_RESET_CR2(hi2c); 01667 01668 hi2c->State = HAL_I2C_STATE_READY; 01669 01670 /* Process Unlocked */ 01671 __HAL_UNLOCK(hi2c); 01672 01673 return HAL_OK; 01674 } 01675 else 01676 { 01677 return HAL_BUSY; 01678 } 01679 } 01680 01681 /** 01682 * @brief Read an amount of data in blocking mode from a specific memory address 01683 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01684 * the configuration information for the specified I2C. 01685 * @param DevAddress: Target device address 01686 * @param MemAddress: Internal memory address 01687 * @param MemAddSize: Size of internal memory address 01688 * @param pData: Pointer to data buffer 01689 * @param Size: Amount of data to be sent 01690 * @param Timeout: Timeout duration 01691 * @retval HAL status 01692 */ 01693 HAL_StatusTypeDef HAL_I2C_Mem_Read(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size, uint32_t Timeout) 01694 { 01695 uint32_t Sizetmp = 0; 01696 01697 /* Check the parameters */ 01698 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 01699 01700 if(hi2c->State == HAL_I2C_STATE_READY) 01701 { 01702 if((pData == NULL) || (Size == 0)) 01703 { 01704 return HAL_ERROR; 01705 } 01706 01707 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01708 { 01709 return HAL_BUSY; 01710 } 01711 01712 /* Process Locked */ 01713 __HAL_LOCK(hi2c); 01714 01715 hi2c->State = HAL_I2C_STATE_MEM_BUSY_RX; 01716 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01717 01718 /* Send Slave Address and Memory Address */ 01719 if(I2C_RequestMemoryRead(hi2c, DevAddress, MemAddress, MemAddSize, Timeout) != HAL_OK) 01720 { 01721 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01722 { 01723 /* Process Unlocked */ 01724 __HAL_UNLOCK(hi2c); 01725 return HAL_ERROR; 01726 } 01727 else 01728 { 01729 /* Process Unlocked */ 01730 __HAL_UNLOCK(hi2c); 01731 return HAL_TIMEOUT; 01732 } 01733 } 01734 01735 /* Send Slave Address */ 01736 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01737 /* Size > 255, need to set RELOAD bit */ 01738 if(Size > 255) 01739 { 01740 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 01741 Sizetmp = 255; 01742 } 01743 else 01744 { 01745 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 01746 Sizetmp = Size; 01747 } 01748 01749 do 01750 { 01751 /* Wait until RXNE flag is set */ 01752 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, Timeout) != HAL_OK) 01753 { 01754 return HAL_TIMEOUT; 01755 } 01756 01757 /* Read data from RXDR */ 01758 (*pData++) = hi2c->Instance->RXDR; 01759 01760 /* Decrement the Size counter */ 01761 Sizetmp--; 01762 Size--; 01763 01764 if((Sizetmp == 0)&&(Size!=0)) 01765 { 01766 /* Wait until TCR flag is set */ 01767 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, Timeout) != HAL_OK) 01768 { 01769 return HAL_TIMEOUT; 01770 } 01771 01772 if(Size > 255) 01773 { 01774 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 01775 Sizetmp = 255; 01776 } 01777 else 01778 { 01779 I2C_TransferConfig(hi2c,DevAddress,Size, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 01780 Sizetmp = Size; 01781 } 01782 } 01783 01784 }while(Size > 0); 01785 01786 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 01787 /* Wait until STOPF flag is reset */ 01788 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 01789 { 01790 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01791 { 01792 return HAL_ERROR; 01793 } 01794 else 01795 { 01796 return HAL_TIMEOUT; 01797 } 01798 } 01799 01800 /* Clear STOP Flag */ 01801 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 01802 01803 /* Clear Configuration Register 2 */ 01804 I2C_RESET_CR2(hi2c); 01805 01806 hi2c->State = HAL_I2C_STATE_READY; 01807 01808 /* Process Unlocked */ 01809 __HAL_UNLOCK(hi2c); 01810 01811 return HAL_OK; 01812 } 01813 else 01814 { 01815 return HAL_BUSY; 01816 } 01817 } 01818 /** 01819 * @brief Write an amount of data in non-blocking mode with Interrupt to a specific memory address 01820 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01821 * the configuration information for the specified I2C. 01822 * @param DevAddress: Target device address 01823 * @param MemAddress: Internal memory address 01824 * @param MemAddSize: Size of internal memory address 01825 * @param pData: Pointer to data buffer 01826 * @param Size: Amount of data to be sent 01827 * @retval HAL status 01828 */ 01829 HAL_StatusTypeDef HAL_I2C_Mem_Write_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) 01830 { 01831 /* Check the parameters */ 01832 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 01833 01834 if(hi2c->State == HAL_I2C_STATE_READY) 01835 { 01836 if((pData == NULL) || (Size == 0)) 01837 { 01838 return HAL_ERROR; 01839 } 01840 01841 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01842 { 01843 return HAL_BUSY; 01844 } 01845 01846 /* Process Locked */ 01847 __HAL_LOCK(hi2c); 01848 01849 hi2c->State = HAL_I2C_STATE_MEM_BUSY_TX; 01850 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 01851 01852 hi2c->pBuffPtr = pData; 01853 hi2c->XferCount = Size; 01854 if(Size > 255) 01855 { 01856 hi2c->XferSize = 255; 01857 } 01858 else 01859 { 01860 hi2c->XferSize = Size; 01861 } 01862 01863 /* Send Slave Address and Memory Address */ 01864 if(I2C_RequestMemoryWrite(hi2c, DevAddress, MemAddress, MemAddSize, I2C_TIMEOUT_FLAG) != HAL_OK) 01865 { 01866 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01867 { 01868 /* Process Unlocked */ 01869 __HAL_UNLOCK(hi2c); 01870 return HAL_ERROR; 01871 } 01872 else 01873 { 01874 /* Process Unlocked */ 01875 __HAL_UNLOCK(hi2c); 01876 return HAL_TIMEOUT; 01877 } 01878 } 01879 01880 /* Set NBYTES to write and reload if size > 255 */ 01881 /* Size > 255, need to set RELOAD bit */ 01882 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01883 { 01884 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 01885 } 01886 else 01887 { 01888 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 01889 } 01890 01891 /* Process Unlocked */ 01892 __HAL_UNLOCK(hi2c); 01893 01894 /* Note : The I2C interrupts must be enabled after unlocking current process 01895 to avoid the risk of I2C interrupt handle execution before current 01896 process unlock */ 01897 01898 /* Enable ERR, TC, STOP, NACK, TXI interrupt */ 01899 /* possible to enable all of these */ 01900 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01901 __HAL_I2C_ENABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_TXI ); 01902 01903 return HAL_OK; 01904 } 01905 else 01906 { 01907 return HAL_BUSY; 01908 } 01909 } 01910 01911 /** 01912 * @brief Read an amount of data in non-blocking mode with Interrupt from a specific memory address 01913 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 01914 * the configuration information for the specified I2C. 01915 * @param DevAddress: Target device address 01916 * @param MemAddress: Internal memory address 01917 * @param MemAddSize: Size of internal memory address 01918 * @param pData: Pointer to data buffer 01919 * @param Size: Amount of data to be sent 01920 * @retval HAL status 01921 */ 01922 HAL_StatusTypeDef HAL_I2C_Mem_Read_IT(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) 01923 { 01924 /* Check the parameters */ 01925 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 01926 01927 if(hi2c->State == HAL_I2C_STATE_READY) 01928 { 01929 if((pData == NULL) || (Size == 0)) 01930 { 01931 return HAL_ERROR; 01932 } 01933 01934 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 01935 { 01936 return HAL_BUSY; 01937 } 01938 01939 /* Process Locked */ 01940 __HAL_LOCK(hi2c); 01941 01942 hi2c->State = HAL_I2C_STATE_MEM_BUSY_RX; 01943 01944 hi2c->pBuffPtr = pData; 01945 hi2c->XferCount = Size; 01946 if(Size > 255) 01947 { 01948 hi2c->XferSize = 255; 01949 } 01950 else 01951 { 01952 hi2c->XferSize = Size; 01953 } 01954 01955 /* Send Slave Address and Memory Address */ 01956 if(I2C_RequestMemoryRead(hi2c, DevAddress, MemAddress, MemAddSize, I2C_TIMEOUT_FLAG) != HAL_OK) 01957 { 01958 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 01959 { 01960 /* Process Unlocked */ 01961 __HAL_UNLOCK(hi2c); 01962 return HAL_ERROR; 01963 } 01964 else 01965 { 01966 /* Process Unlocked */ 01967 __HAL_UNLOCK(hi2c); 01968 return HAL_TIMEOUT; 01969 } 01970 } 01971 01972 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 01973 /* Size > 255, need to set RELOAD bit */ 01974 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 01975 { 01976 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 01977 } 01978 else 01979 { 01980 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 01981 } 01982 01983 /* Process Unlocked */ 01984 __HAL_UNLOCK(hi2c); 01985 01986 /* Note : The I2C interrupts must be enabled after unlocking current process 01987 to avoid the risk of I2C interrupt handle execution before current 01988 process unlock */ 01989 01990 /* Enable ERR, TC, STOP, NACK, RXI interrupt */ 01991 /* possible to enable all of these */ 01992 /* I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI */ 01993 __HAL_I2C_ENABLE_IT(hi2c, I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_RXI ); 01994 01995 return HAL_OK; 01996 } 01997 else 01998 { 01999 return HAL_BUSY; 02000 } 02001 } 02002 /** 02003 * @brief Write an amount of data in non-blocking mode with DMA to a specific memory address 02004 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02005 * the configuration information for the specified I2C. 02006 * @param DevAddress: Target device address 02007 * @param MemAddress: Internal memory address 02008 * @param MemAddSize: Size of internal memory address 02009 * @param pData: Pointer to data buffer 02010 * @param Size: Amount of data to be sent 02011 * @retval HAL status 02012 */ 02013 HAL_StatusTypeDef HAL_I2C_Mem_Write_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) 02014 { 02015 /* Check the parameters */ 02016 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 02017 02018 if(hi2c->State == HAL_I2C_STATE_READY) 02019 { 02020 if((pData == NULL) || (Size == 0)) 02021 { 02022 return HAL_ERROR; 02023 } 02024 02025 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 02026 { 02027 return HAL_BUSY; 02028 } 02029 02030 /* Process Locked */ 02031 __HAL_LOCK(hi2c); 02032 02033 hi2c->State = HAL_I2C_STATE_MEM_BUSY_TX; 02034 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 02035 02036 hi2c->pBuffPtr = pData; 02037 hi2c->XferCount = Size; 02038 if(Size > 255) 02039 { 02040 hi2c->XferSize = 255; 02041 } 02042 else 02043 { 02044 hi2c->XferSize = Size; 02045 } 02046 02047 /* Set the I2C DMA transfer complete callback */ 02048 hi2c->hdmatx->XferCpltCallback = I2C_DMAMemTransmitCplt; 02049 02050 /* Set the DMA error callback */ 02051 hi2c->hdmatx->XferErrorCallback = I2C_DMAError; 02052 02053 /* Enable the DMA channel */ 02054 HAL_DMA_Start_IT(hi2c->hdmatx, (uint32_t)pData, (uint32_t)&hi2c->Instance->TXDR, hi2c->XferSize); 02055 02056 /* Send Slave Address and Memory Address */ 02057 if(I2C_RequestMemoryWrite(hi2c, DevAddress, MemAddress, MemAddSize, I2C_TIMEOUT_FLAG) != HAL_OK) 02058 { 02059 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 02060 { 02061 /* Process Unlocked */ 02062 __HAL_UNLOCK(hi2c); 02063 return HAL_ERROR; 02064 } 02065 else 02066 { 02067 /* Process Unlocked */ 02068 __HAL_UNLOCK(hi2c); 02069 return HAL_TIMEOUT; 02070 } 02071 } 02072 02073 /* Send Slave Address */ 02074 /* Set NBYTES to write and reload if size > 255 */ 02075 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 02076 { 02077 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 02078 } 02079 else 02080 { 02081 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 02082 } 02083 02084 /* Wait until TXIS flag is set */ 02085 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, I2C_TIMEOUT_TXIS) != HAL_OK) 02086 { 02087 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 02088 { 02089 return HAL_ERROR; 02090 } 02091 else 02092 { 02093 return HAL_TIMEOUT; 02094 } 02095 } 02096 02097 /* Enable DMA Request */ 02098 hi2c->Instance->CR1 |= I2C_CR1_TXDMAEN; 02099 02100 /* Process Unlocked */ 02101 __HAL_UNLOCK(hi2c); 02102 02103 return HAL_OK; 02104 } 02105 else 02106 { 02107 return HAL_BUSY; 02108 } 02109 } 02110 02111 /** 02112 * @brief Reads an amount of data in non-blocking mode with DMA from a specific memory address. 02113 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02114 * the configuration information for the specified I2C. 02115 * @param DevAddress: Target device address 02116 * @param MemAddress: Internal memory address 02117 * @param MemAddSize: Size of internal memory address 02118 * @param pData: Pointer to data buffer 02119 * @param Size: Amount of data to be read 02120 * @retval HAL status 02121 */ 02122 HAL_StatusTypeDef HAL_I2C_Mem_Read_DMA(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint8_t *pData, uint16_t Size) 02123 { 02124 /* Check the parameters */ 02125 assert_param(IS_I2C_MEMADD_SIZE(MemAddSize)); 02126 02127 if(hi2c->State == HAL_I2C_STATE_READY) 02128 { 02129 if((pData == NULL) || (Size == 0)) 02130 { 02131 return HAL_ERROR; 02132 } 02133 02134 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 02135 { 02136 return HAL_BUSY; 02137 } 02138 02139 /* Process Locked */ 02140 __HAL_LOCK(hi2c); 02141 02142 hi2c->State = HAL_I2C_STATE_MEM_BUSY_RX; 02143 02144 hi2c->pBuffPtr = pData; 02145 hi2c->XferCount = Size; 02146 if(Size > 255) 02147 { 02148 hi2c->XferSize = 255; 02149 } 02150 else 02151 { 02152 hi2c->XferSize = Size; 02153 } 02154 02155 /* Set the I2C DMA transfer complete callback */ 02156 hi2c->hdmarx->XferCpltCallback = I2C_DMAMemReceiveCplt; 02157 02158 /* Set the DMA error callback */ 02159 hi2c->hdmarx->XferErrorCallback = I2C_DMAError; 02160 02161 /* Enable the DMA channel */ 02162 HAL_DMA_Start_IT(hi2c->hdmarx, (uint32_t)&hi2c->Instance->RXDR, (uint32_t)pData, hi2c->XferSize); 02163 02164 /* Send Slave Address and Memory Address */ 02165 if(I2C_RequestMemoryRead(hi2c, DevAddress, MemAddress, MemAddSize, I2C_TIMEOUT_FLAG) != HAL_OK) 02166 { 02167 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 02168 { 02169 /* Process Unlocked */ 02170 __HAL_UNLOCK(hi2c); 02171 return HAL_ERROR; 02172 } 02173 else 02174 { 02175 /* Process Unlocked */ 02176 __HAL_UNLOCK(hi2c); 02177 return HAL_TIMEOUT; 02178 } 02179 } 02180 02181 /* Set NBYTES to write and reload if size > 255 and generate RESTART */ 02182 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 02183 { 02184 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_GENERATE_START_READ); 02185 } 02186 else 02187 { 02188 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_GENERATE_START_READ); 02189 } 02190 02191 /* Wait until RXNE flag is set */ 02192 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, I2C_TIMEOUT_RXNE) != HAL_OK) 02193 { 02194 return HAL_TIMEOUT; 02195 } 02196 02197 /* Enable DMA Request */ 02198 hi2c->Instance->CR1 |= I2C_CR1_RXDMAEN; 02199 02200 /* Process Unlocked */ 02201 __HAL_UNLOCK(hi2c); 02202 02203 return HAL_OK; 02204 } 02205 else 02206 { 02207 return HAL_BUSY; 02208 } 02209 } 02210 02211 /** 02212 * @brief Checks if target device is ready for communication. 02213 * @note This function is used with Memory devices 02214 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02215 * the configuration information for the specified I2C. 02216 * @param DevAddress: Target device address 02217 * @param Trials: Number of trials 02218 * @param Timeout: Timeout duration 02219 * @retval HAL status 02220 */ 02221 HAL_StatusTypeDef HAL_I2C_IsDeviceReady(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint32_t Trials, uint32_t Timeout) 02222 { 02223 uint32_t tickstart = 0; 02224 02225 __IO uint32_t I2C_Trials = 0; 02226 02227 if(hi2c->State == HAL_I2C_STATE_READY) 02228 { 02229 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BUSY) == SET) 02230 { 02231 return HAL_BUSY; 02232 } 02233 02234 /* Process Locked */ 02235 __HAL_LOCK(hi2c); 02236 02237 hi2c->State = HAL_I2C_STATE_BUSY; 02238 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 02239 02240 do 02241 { 02242 /* Generate Start */ 02243 hi2c->Instance->CR2 = I2C_GENERATE_START(hi2c->Init.AddressingMode,DevAddress); 02244 02245 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 02246 /* Wait until STOPF flag is set or a NACK flag is set*/ 02247 tickstart = HAL_GetTick(); 02248 while((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == RESET) && (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == RESET) && (hi2c->State != HAL_I2C_STATE_TIMEOUT)) 02249 { 02250 if(Timeout != HAL_MAX_DELAY) 02251 { 02252 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 02253 { 02254 /* Device is ready */ 02255 hi2c->State = HAL_I2C_STATE_READY; 02256 /* Process Unlocked */ 02257 __HAL_UNLOCK(hi2c); 02258 return HAL_TIMEOUT; 02259 } 02260 } 02261 } 02262 02263 /* Check if the NACKF flag has not been set */ 02264 if (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == RESET) 02265 { 02266 /* Wait until STOPF flag is reset */ 02267 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_STOPF, RESET, Timeout) != HAL_OK) 02268 { 02269 return HAL_TIMEOUT; 02270 } 02271 02272 /* Clear STOP Flag */ 02273 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02274 02275 /* Device is ready */ 02276 hi2c->State = HAL_I2C_STATE_READY; 02277 02278 /* Process Unlocked */ 02279 __HAL_UNLOCK(hi2c); 02280 02281 return HAL_OK; 02282 } 02283 else 02284 { 02285 /* Wait until STOPF flag is reset */ 02286 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_STOPF, RESET, Timeout) != HAL_OK) 02287 { 02288 return HAL_TIMEOUT; 02289 } 02290 02291 /* Clear NACK Flag */ 02292 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02293 02294 /* Clear STOP Flag, auto generated with autoend*/ 02295 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02296 } 02297 02298 /* Check if the maximum allowed number of trials has been reached */ 02299 if (I2C_Trials++ == Trials) 02300 { 02301 /* Generate Stop */ 02302 hi2c->Instance->CR2 |= I2C_CR2_STOP; 02303 02304 /* Wait until STOPF flag is reset */ 02305 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_STOPF, RESET, Timeout) != HAL_OK) 02306 { 02307 return HAL_TIMEOUT; 02308 } 02309 02310 /* Clear STOP Flag */ 02311 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02312 } 02313 }while(I2C_Trials < Trials); 02314 02315 hi2c->State = HAL_I2C_STATE_READY; 02316 02317 /* Process Unlocked */ 02318 __HAL_UNLOCK(hi2c); 02319 02320 return HAL_TIMEOUT; 02321 } 02322 else 02323 { 02324 return HAL_BUSY; 02325 } 02326 } 02327 /** 02328 * @} 02329 */ 02330 02331 /** @defgroup I2C_IRQ_Handler_and_Callbacks IRQ Handler and Callbacks 02332 * @{ 02333 */ 02334 02335 /** 02336 * @brief This function handles I2C event interrupt request. 02337 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02338 * the configuration information for the specified I2C. 02339 * @retval None 02340 */ 02341 void HAL_I2C_EV_IRQHandler(I2C_HandleTypeDef *hi2c) 02342 { 02343 /* I2C in mode Transmitter ---------------------------------------------------*/ 02344 if (((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TXIS) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_ADDR) == SET)) && (__HAL_I2C_GET_IT_SOURCE(hi2c, (I2C_IT_TCI | I2C_IT_STOPI | I2C_IT_NACKI | I2C_IT_TXI | I2C_IT_ADDRI)) == SET)) 02345 { 02346 /* Slave mode selected */ 02347 if (hi2c->State == HAL_I2C_STATE_SLAVE_BUSY_TX) 02348 { 02349 I2C_SlaveTransmit_ISR(hi2c); 02350 } 02351 } 02352 02353 if (((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TXIS) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET)) && (__HAL_I2C_GET_IT_SOURCE(hi2c, (I2C_IT_TCI | I2C_IT_STOPI | I2C_IT_NACKI | I2C_IT_TXI)) == SET)) 02354 { 02355 /* Master mode selected */ 02356 if ((hi2c->State == HAL_I2C_STATE_MASTER_BUSY_TX) || (hi2c->State == HAL_I2C_STATE_MEM_BUSY_TX)) 02357 { 02358 I2C_MasterTransmit_ISR(hi2c); 02359 } 02360 } 02361 02362 /* I2C in mode Receiver ----------------------------------------------------*/ 02363 if (((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_RXNE) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_ADDR) == SET)) && (__HAL_I2C_GET_IT_SOURCE(hi2c, (I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_RXI | I2C_IT_ADDRI)) == SET)) 02364 { 02365 /* Slave mode selected */ 02366 if (hi2c->State == HAL_I2C_STATE_SLAVE_BUSY_RX) 02367 { 02368 I2C_SlaveReceive_ISR(hi2c); 02369 } 02370 } 02371 if (((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_RXNE) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) || (__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET)) && (__HAL_I2C_GET_IT_SOURCE(hi2c, (I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_RXI)) == SET)) 02372 { 02373 /* Master mode selected */ 02374 if ((hi2c->State == HAL_I2C_STATE_MASTER_BUSY_RX) || (hi2c->State == HAL_I2C_STATE_MEM_BUSY_RX)) 02375 { 02376 I2C_MasterReceive_ISR(hi2c); 02377 } 02378 } 02379 } 02380 02381 /** 02382 * @brief This function handles I2C error interrupt request. 02383 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02384 * the configuration information for the specified I2C. 02385 * @retval None 02386 */ 02387 void HAL_I2C_ER_IRQHandler(I2C_HandleTypeDef *hi2c) 02388 { 02389 /* I2C Bus error interrupt occurred ------------------------------------*/ 02390 if((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_BERR) == SET) && (__HAL_I2C_GET_IT_SOURCE(hi2c, I2C_IT_ERRI) == SET)) 02391 { 02392 hi2c->ErrorCode |= HAL_I2C_ERROR_BERR; 02393 02394 /* Clear BERR flag */ 02395 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_BERR); 02396 } 02397 02398 /* I2C Over-Run/Under-Run interrupt occurred ----------------------------------------*/ 02399 if((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_OVR) == SET) && (__HAL_I2C_GET_IT_SOURCE(hi2c, I2C_IT_ERRI) == SET)) 02400 { 02401 hi2c->ErrorCode |= HAL_I2C_ERROR_OVR; 02402 02403 /* Clear OVR flag */ 02404 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_OVR); 02405 } 02406 02407 /* I2C Arbitration Loss error interrupt occurred -------------------------------------*/ 02408 if((__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_ARLO) == SET) && (__HAL_I2C_GET_IT_SOURCE(hi2c, I2C_IT_ERRI) == SET)) 02409 { 02410 hi2c->ErrorCode |= HAL_I2C_ERROR_ARLO; 02411 02412 /* Clear ARLO flag */ 02413 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_ARLO); 02414 } 02415 02416 /* Call the Error Callback in case of Error detected */ 02417 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 02418 { 02419 hi2c->State = HAL_I2C_STATE_READY; 02420 02421 HAL_I2C_ErrorCallback(hi2c); 02422 } 02423 } 02424 02425 /** 02426 * @brief Master Tx Transfer completed callbacks. 02427 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02428 * the configuration information for the specified I2C. 02429 * @retval None 02430 */ 02431 __weak void HAL_I2C_MasterTxCpltCallback(I2C_HandleTypeDef *hi2c) 02432 { 02433 /* NOTE : This function should not be modified, when the callback is needed, 02434 the HAL_I2C_TxCpltCallback could be implemented in the user file 02435 */ 02436 } 02437 02438 /** 02439 * @brief Master Rx Transfer completed callbacks. 02440 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02441 * the configuration information for the specified I2C. 02442 * @retval None 02443 */ 02444 __weak void HAL_I2C_MasterRxCpltCallback(I2C_HandleTypeDef *hi2c) 02445 { 02446 /* NOTE : This function should not be modified, when the callback is needed, 02447 the HAL_I2C_TxCpltCallback could be implemented in the user file 02448 */ 02449 } 02450 02451 /** @brief Slave Tx Transfer completed callbacks. 02452 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02453 * the configuration information for the specified I2C. 02454 * @retval None 02455 */ 02456 __weak void HAL_I2C_SlaveTxCpltCallback(I2C_HandleTypeDef *hi2c) 02457 { 02458 /* NOTE : This function should not be modified, when the callback is needed, 02459 the HAL_I2C_TxCpltCallback could be implemented in the user file 02460 */ 02461 } 02462 02463 /** 02464 * @brief Slave Rx Transfer completed callbacks. 02465 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02466 * the configuration information for the specified I2C. 02467 * @retval None 02468 */ 02469 __weak void HAL_I2C_SlaveRxCpltCallback(I2C_HandleTypeDef *hi2c) 02470 { 02471 /* NOTE : This function should not be modified, when the callback is needed, 02472 the HAL_I2C_TxCpltCallback could be implemented in the user file 02473 */ 02474 } 02475 02476 /** 02477 * @brief Memory Tx Transfer completed callbacks. 02478 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02479 * the configuration information for the specified I2C. 02480 * @retval None 02481 */ 02482 __weak void HAL_I2C_MemTxCpltCallback(I2C_HandleTypeDef *hi2c) 02483 { 02484 /* NOTE : This function should not be modified, when the callback is needed, 02485 the HAL_I2C_TxCpltCallback could be implemented in the user file 02486 */ 02487 } 02488 02489 /** 02490 * @brief Memory Rx Transfer completed callbacks. 02491 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02492 * the configuration information for the specified I2C. 02493 * @retval None 02494 */ 02495 __weak void HAL_I2C_MemRxCpltCallback(I2C_HandleTypeDef *hi2c) 02496 { 02497 /* NOTE : This function should not be modified, when the callback is needed, 02498 the HAL_I2C_TxCpltCallback could be implemented in the user file 02499 */ 02500 } 02501 02502 /** 02503 * @brief I2C error callbacks. 02504 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02505 * the configuration information for the specified I2C. 02506 * @retval None 02507 */ 02508 __weak void HAL_I2C_ErrorCallback(I2C_HandleTypeDef *hi2c) 02509 { 02510 /* NOTE : This function should not be modified, when the callback is needed, 02511 the HAL_I2C_ErrorCallback could be implemented in the user file 02512 */ 02513 } 02514 02515 /** 02516 * @} 02517 */ 02518 02519 02520 /** @defgroup I2C_Exported_Functions_Group3 Peripheral State and Errors functions 02521 * @brief Peripheral State and Errors functions 02522 * 02523 @verbatim 02524 =============================================================================== 02525 ##### Peripheral State and Errors functions ##### 02526 =============================================================================== 02527 [..] 02528 This subsection permits to get in run-time the status of the peripheral 02529 and the data flow. 02530 02531 @endverbatim 02532 * @{ 02533 */ 02534 02535 /** 02536 * @brief Return the I2C handle state. 02537 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02538 * the configuration information for the specified I2C. 02539 * @retval HAL state 02540 */ 02541 HAL_I2C_StateTypeDef HAL_I2C_GetState(I2C_HandleTypeDef *hi2c) 02542 { 02543 /* Return I2C handle state */ 02544 return hi2c->State; 02545 } 02546 02547 /** 02548 * @brief Return the I2C error code. 02549 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02550 * the configuration information for the specified I2C. 02551 * @retval I2C Error Code 02552 */ 02553 uint32_t HAL_I2C_GetError(I2C_HandleTypeDef *hi2c) 02554 { 02555 return hi2c->ErrorCode; 02556 } 02557 02558 /** 02559 * @} 02560 */ 02561 02562 /** 02563 * @} 02564 */ 02565 02566 /** @addtogroup I2C_Private_Functions 02567 * @{ 02568 */ 02569 02570 /** 02571 * @brief Handle Interrupt Flags Master Transmit Mode 02572 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02573 * the configuration information for the specified I2C. 02574 * @retval HAL status 02575 */ 02576 static HAL_StatusTypeDef I2C_MasterTransmit_ISR(I2C_HandleTypeDef *hi2c) 02577 { 02578 uint16_t DevAddress; 02579 02580 /* Process Locked */ 02581 __HAL_LOCK(hi2c); 02582 02583 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TXIS) == SET) 02584 { 02585 /* Write data to TXDR */ 02586 hi2c->Instance->TXDR = (*hi2c->pBuffPtr++); 02587 hi2c->XferSize--; 02588 hi2c->XferCount--; 02589 } 02590 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) 02591 { 02592 if((hi2c->XferSize == 0)&&(hi2c->XferCount!=0)) 02593 { 02594 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 02595 02596 if(hi2c->XferCount > 255) 02597 { 02598 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 02599 hi2c->XferSize = 255; 02600 } 02601 else 02602 { 02603 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferCount, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 02604 hi2c->XferSize = hi2c->XferCount; 02605 } 02606 } 02607 else 02608 { 02609 /* Process Unlocked */ 02610 __HAL_UNLOCK(hi2c); 02611 02612 /* Wrong size Status regarding TCR flag event */ 02613 hi2c->ErrorCode |= HAL_I2C_ERROR_SIZE; 02614 HAL_I2C_ErrorCallback(hi2c); 02615 } 02616 } 02617 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) 02618 { 02619 if(hi2c->XferCount == 0) 02620 { 02621 /* Generate Stop */ 02622 hi2c->Instance->CR2 |= I2C_CR2_STOP; 02623 } 02624 else 02625 { 02626 /* Process Unlocked */ 02627 __HAL_UNLOCK(hi2c); 02628 02629 /* Wrong size Status regarding TCR flag event */ 02630 hi2c->ErrorCode |= HAL_I2C_ERROR_SIZE; 02631 HAL_I2C_ErrorCallback(hi2c); 02632 } 02633 } 02634 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) 02635 { 02636 /* Disable ERR, TC, STOP, NACK, TXI interrupt */ 02637 __HAL_I2C_DISABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_TXI ); 02638 02639 /* Clear STOP Flag */ 02640 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02641 02642 /* Clear Configuration Register 2 */ 02643 I2C_RESET_CR2(hi2c); 02644 02645 hi2c->State = HAL_I2C_STATE_READY; 02646 02647 /* Process Unlocked */ 02648 __HAL_UNLOCK(hi2c); 02649 02650 if(hi2c->State == HAL_I2C_STATE_MEM_BUSY_TX) 02651 { 02652 HAL_I2C_MemTxCpltCallback(hi2c); 02653 } 02654 else 02655 { 02656 HAL_I2C_MasterTxCpltCallback(hi2c); 02657 } 02658 } 02659 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET) 02660 { 02661 /* Clear NACK Flag */ 02662 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02663 02664 /* Process Unlocked */ 02665 __HAL_UNLOCK(hi2c); 02666 02667 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 02668 HAL_I2C_ErrorCallback(hi2c); 02669 } 02670 02671 /* Process Unlocked */ 02672 __HAL_UNLOCK(hi2c); 02673 02674 return HAL_OK; 02675 } 02676 02677 /** 02678 * @brief Handle Interrupt Flags Master Receive Mode 02679 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02680 * the configuration information for the specified I2C. 02681 * @retval HAL status 02682 */ 02683 static HAL_StatusTypeDef I2C_MasterReceive_ISR(I2C_HandleTypeDef *hi2c) 02684 { 02685 uint16_t DevAddress; 02686 02687 /* Process Locked */ 02688 __HAL_LOCK(hi2c); 02689 02690 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_RXNE) == SET) 02691 { 02692 /* Read data from RXDR */ 02693 (*hi2c->pBuffPtr++) = hi2c->Instance->RXDR; 02694 hi2c->XferSize--; 02695 hi2c->XferCount--; 02696 } 02697 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TCR) == SET) 02698 { 02699 if((hi2c->XferSize == 0)&&(hi2c->XferCount!=0)) 02700 { 02701 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 02702 02703 if(hi2c->XferCount > 255) 02704 { 02705 I2C_TransferConfig(hi2c,DevAddress,255, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 02706 hi2c->XferSize = 255; 02707 } 02708 else 02709 { 02710 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferCount, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 02711 hi2c->XferSize = hi2c->XferCount; 02712 } 02713 } 02714 else 02715 { 02716 /* Process Unlocked */ 02717 __HAL_UNLOCK(hi2c); 02718 02719 /* Wrong size Status regarding TCR flag event */ 02720 hi2c->ErrorCode |= HAL_I2C_ERROR_SIZE; 02721 HAL_I2C_ErrorCallback(hi2c); 02722 } 02723 } 02724 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TC) == SET) 02725 { 02726 if(hi2c->XferCount == 0) 02727 { 02728 /* Generate Stop */ 02729 hi2c->Instance->CR2 |= I2C_CR2_STOP; 02730 } 02731 else 02732 { 02733 /* Process Unlocked */ 02734 __HAL_UNLOCK(hi2c); 02735 02736 /* Wrong size Status regarding TCR flag event */ 02737 hi2c->ErrorCode |= HAL_I2C_ERROR_SIZE; 02738 HAL_I2C_ErrorCallback(hi2c); 02739 } 02740 } 02741 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) 02742 { 02743 /* Disable ERR, TC, STOP, NACK, TXI interrupt */ 02744 __HAL_I2C_DISABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_RXI ); 02745 02746 /* Clear STOP Flag */ 02747 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02748 02749 /* Clear Configuration Register 2 */ 02750 I2C_RESET_CR2(hi2c); 02751 02752 hi2c->State = HAL_I2C_STATE_READY; 02753 02754 /* Process Unlocked */ 02755 __HAL_UNLOCK(hi2c); 02756 02757 if(hi2c->State == HAL_I2C_STATE_MEM_BUSY_RX) 02758 { 02759 HAL_I2C_MemRxCpltCallback(hi2c); 02760 } 02761 else 02762 { 02763 HAL_I2C_MasterRxCpltCallback(hi2c); 02764 } 02765 } 02766 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET) 02767 { 02768 /* Clear NACK Flag */ 02769 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02770 02771 /* Process Unlocked */ 02772 __HAL_UNLOCK(hi2c); 02773 02774 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 02775 HAL_I2C_ErrorCallback(hi2c); 02776 } 02777 02778 /* Process Unlocked */ 02779 __HAL_UNLOCK(hi2c); 02780 02781 return HAL_OK; 02782 02783 } 02784 02785 /** 02786 * @brief Handle Interrupt Flags Slave Transmit Mode 02787 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02788 * the configuration information for the specified I2C. 02789 * @retval HAL status 02790 */ 02791 static HAL_StatusTypeDef I2C_SlaveTransmit_ISR(I2C_HandleTypeDef *hi2c) 02792 { 02793 /* Process locked */ 02794 __HAL_LOCK(hi2c); 02795 02796 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) != RESET) 02797 { 02798 /* Check that I2C transfer finished */ 02799 /* if yes, normal use case, a NACK is sent by the MASTER when Transfer is finished */ 02800 /* Mean XferCount == 0*/ 02801 /* So clear Flag NACKF only */ 02802 if(hi2c->XferCount == 0) 02803 { 02804 /* Clear NACK Flag */ 02805 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02806 02807 /* Process Unlocked */ 02808 __HAL_UNLOCK(hi2c); 02809 } 02810 else 02811 { 02812 /* if no, error use case, a Non-Acknowledge of last Data is generated by the MASTER*/ 02813 /* Clear NACK Flag */ 02814 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02815 02816 /* Set ErrorCode corresponding to a Non-Acknowledge */ 02817 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 02818 02819 /* Process Unlocked */ 02820 __HAL_UNLOCK(hi2c); 02821 02822 /* Call the Error callback to prevent upper layer */ 02823 HAL_I2C_ErrorCallback(hi2c); 02824 } 02825 } 02826 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_ADDR) == SET) 02827 { 02828 /* Clear ADDR flag */ 02829 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_ADDR); 02830 } 02831 /* Check first if STOPF is set */ 02832 /* to prevent a Write Data in TX buffer */ 02833 /* which is stuck in TXDR until next */ 02834 /* communication with Master */ 02835 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) 02836 { 02837 /* Disable ERRI, TCI, STOPI, NACKI, ADDRI, RXI, TXI interrupt */ 02838 __HAL_I2C_DISABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_TXI ); 02839 02840 /* Disable Address Acknowledge */ 02841 hi2c->Instance->CR2 |= I2C_CR2_NACK; 02842 02843 /* Clear STOP Flag */ 02844 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02845 02846 hi2c->State = HAL_I2C_STATE_READY; 02847 02848 /* Process Unlocked */ 02849 __HAL_UNLOCK(hi2c); 02850 02851 HAL_I2C_SlaveTxCpltCallback(hi2c); 02852 } 02853 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TXIS) == SET) 02854 { 02855 /* Write data to TXDR only if XferCount not reach "0" */ 02856 /* A TXIS flag can be set, during STOP treatment */ 02857 if(hi2c->XferCount > 0) 02858 { 02859 /* Write data to TXDR */ 02860 hi2c->Instance->TXDR = (*hi2c->pBuffPtr++); 02861 hi2c->XferCount--; 02862 } 02863 } 02864 02865 /* Process Unlocked */ 02866 __HAL_UNLOCK(hi2c); 02867 02868 return HAL_OK; 02869 } 02870 02871 /** 02872 * @brief Handle Interrupt Flags Slave Receive Mode 02873 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02874 * the configuration information for the specified I2C. 02875 * @retval HAL status 02876 */ 02877 static HAL_StatusTypeDef I2C_SlaveReceive_ISR(I2C_HandleTypeDef *hi2c) 02878 { 02879 /* Process Locked */ 02880 __HAL_LOCK(hi2c); 02881 02882 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) != RESET) 02883 { 02884 /* Clear NACK Flag */ 02885 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 02886 02887 /* Process Unlocked */ 02888 __HAL_UNLOCK(hi2c); 02889 02890 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 02891 HAL_I2C_ErrorCallback(hi2c); 02892 } 02893 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_ADDR) == SET) 02894 { 02895 /* Clear ADDR flag */ 02896 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_ADDR); 02897 } 02898 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_RXNE) == SET) 02899 { 02900 /* Read data from RXDR */ 02901 (*hi2c->pBuffPtr++) = hi2c->Instance->RXDR; 02902 hi2c->XferSize--; 02903 hi2c->XferCount--; 02904 } 02905 else if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) 02906 { 02907 /* Disable ERRI, TCI, STOPI, NACKI, ADDRI, RXI, TXI interrupt */ 02908 __HAL_I2C_DISABLE_IT(hi2c,I2C_IT_ERRI | I2C_IT_TCI| I2C_IT_STOPI| I2C_IT_NACKI | I2C_IT_ADDRI | I2C_IT_RXI | I2C_IT_RXI ); 02909 02910 /* Disable Address Acknowledge */ 02911 hi2c->Instance->CR2 |= I2C_CR2_NACK; 02912 02913 /* Clear STOP Flag */ 02914 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 02915 02916 hi2c->State = HAL_I2C_STATE_READY; 02917 02918 /* Process Unlocked */ 02919 __HAL_UNLOCK(hi2c); 02920 02921 HAL_I2C_SlaveRxCpltCallback(hi2c); 02922 } 02923 02924 /* Process Unlocked */ 02925 __HAL_UNLOCK(hi2c); 02926 02927 return HAL_OK; 02928 } 02929 02930 /** 02931 * @brief Master sends target device address followed by internal memory address for write request. 02932 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02933 * the configuration information for the specified I2C. 02934 * @param DevAddress: Target device address 02935 * @param MemAddress: Internal memory address 02936 * @param MemAddSize: Size of internal memory address 02937 * @param Timeout: Timeout duration 02938 * @retval HAL status 02939 */ 02940 static HAL_StatusTypeDef I2C_RequestMemoryWrite(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout) 02941 { 02942 I2C_TransferConfig(hi2c,DevAddress,MemAddSize, I2C_RELOAD_MODE, I2C_GENERATE_START_WRITE); 02943 02944 /* Wait until TXIS flag is set */ 02945 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 02946 { 02947 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 02948 { 02949 return HAL_ERROR; 02950 } 02951 else 02952 { 02953 return HAL_TIMEOUT; 02954 } 02955 } 02956 02957 /* If Memory address size is 8Bit */ 02958 if(MemAddSize == I2C_MEMADD_SIZE_8BIT) 02959 { 02960 /* Send Memory Address */ 02961 hi2c->Instance->TXDR = I2C_MEM_ADD_LSB(MemAddress); 02962 } 02963 /* If Memory address size is 16Bit */ 02964 else 02965 { 02966 /* Send MSB of Memory Address */ 02967 hi2c->Instance->TXDR = I2C_MEM_ADD_MSB(MemAddress); 02968 02969 /* Wait until TXIS flag is set */ 02970 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 02971 { 02972 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 02973 { 02974 return HAL_ERROR; 02975 } 02976 else 02977 { 02978 return HAL_TIMEOUT; 02979 } 02980 } 02981 02982 /* Send LSB of Memory Address */ 02983 hi2c->Instance->TXDR = I2C_MEM_ADD_LSB(MemAddress); 02984 } 02985 02986 /* Wait until TCR flag is set */ 02987 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, Timeout) != HAL_OK) 02988 { 02989 return HAL_TIMEOUT; 02990 } 02991 02992 return HAL_OK; 02993 } 02994 02995 /** 02996 * @brief Master sends target device address followed by internal memory address for read request. 02997 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 02998 * the configuration information for the specified I2C. 02999 * @param DevAddress: Target device address 03000 * @param MemAddress: Internal memory address 03001 * @param MemAddSize: Size of internal memory address 03002 * @param Timeout: Timeout duration 03003 * @retval HAL status 03004 */ 03005 static HAL_StatusTypeDef I2C_RequestMemoryRead(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint16_t MemAddress, uint16_t MemAddSize, uint32_t Timeout) 03006 { 03007 I2C_TransferConfig(hi2c,DevAddress,MemAddSize, I2C_SOFTEND_MODE, I2C_GENERATE_START_WRITE); 03008 03009 /* Wait until TXIS flag is set */ 03010 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 03011 { 03012 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03013 { 03014 return HAL_ERROR; 03015 } 03016 else 03017 { 03018 return HAL_TIMEOUT; 03019 } 03020 } 03021 03022 /* If Memory address size is 8Bit */ 03023 if(MemAddSize == I2C_MEMADD_SIZE_8BIT) 03024 { 03025 /* Send Memory Address */ 03026 hi2c->Instance->TXDR = I2C_MEM_ADD_LSB(MemAddress); 03027 } 03028 /* If Memory address size is 16Bit */ 03029 else 03030 { 03031 /* Send MSB of Memory Address */ 03032 hi2c->Instance->TXDR = I2C_MEM_ADD_MSB(MemAddress); 03033 03034 /* Wait until TXIS flag is set */ 03035 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, Timeout) != HAL_OK) 03036 { 03037 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03038 { 03039 return HAL_ERROR; 03040 } 03041 else 03042 { 03043 return HAL_TIMEOUT; 03044 } 03045 } 03046 03047 /* Send LSB of Memory Address */ 03048 hi2c->Instance->TXDR = I2C_MEM_ADD_LSB(MemAddress); 03049 } 03050 03051 /* Wait until TC flag is set */ 03052 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TC, RESET, Timeout) != HAL_OK) 03053 { 03054 return HAL_TIMEOUT; 03055 } 03056 03057 return HAL_OK; 03058 } 03059 03060 /** 03061 * @brief DMA I2C master transmit process complete callback. 03062 * @param hdma: DMA handle 03063 * @retval None 03064 */ 03065 static void I2C_DMAMasterTransmitCplt(DMA_HandleTypeDef *hdma) 03066 { 03067 uint16_t DevAddress; 03068 I2C_HandleTypeDef* hi2c = (I2C_HandleTypeDef*)((DMA_HandleTypeDef*)hdma)->Parent; 03069 03070 /* Check if last DMA request was done with RELOAD */ 03071 /* Set NBYTES to write and reload if size > 255 */ 03072 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03073 { 03074 /* Wait until TCR flag is set */ 03075 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, I2C_TIMEOUT_TCR) != HAL_OK) 03076 { 03077 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03078 } 03079 03080 /* Disable DMA Request */ 03081 hi2c->Instance->CR1 &= ~I2C_CR1_TXDMAEN; 03082 03083 /* Check if Errors has been detected during transfer */ 03084 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03085 { 03086 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03087 /* Wait until STOPF flag is reset */ 03088 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03089 { 03090 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03091 { 03092 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03093 } 03094 else 03095 { 03096 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03097 } 03098 } 03099 03100 /* Clear STOP Flag */ 03101 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03102 03103 /* Clear Configuration Register 2 */ 03104 I2C_RESET_CR2(hi2c); 03105 03106 hi2c->XferCount = 0; 03107 03108 hi2c->State = HAL_I2C_STATE_READY; 03109 HAL_I2C_ErrorCallback(hi2c); 03110 } 03111 else 03112 { 03113 hi2c->pBuffPtr += hi2c->XferSize; 03114 hi2c->XferCount -= hi2c->XferSize; 03115 if(hi2c->XferCount > 255) 03116 { 03117 hi2c->XferSize = 255; 03118 } 03119 else 03120 { 03121 hi2c->XferSize = hi2c->XferCount; 03122 } 03123 03124 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 03125 03126 /* Enable the DMA channel */ 03127 HAL_DMA_Start_IT(hi2c->hdmatx, (uint32_t)hi2c->pBuffPtr, (uint32_t)&hi2c->Instance->TXDR, hi2c->XferSize); 03128 03129 /* Send Slave Address */ 03130 /* Set NBYTES to write and reload if size > 255 */ 03131 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03132 { 03133 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 03134 } 03135 else 03136 { 03137 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 03138 } 03139 03140 /* Wait until TXIS flag is set */ 03141 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, I2C_TIMEOUT_TXIS) != HAL_OK) 03142 { 03143 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03144 /* Wait until STOPF flag is reset */ 03145 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03146 { 03147 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03148 { 03149 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03150 } 03151 else 03152 { 03153 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03154 } 03155 } 03156 03157 /* Clear STOP Flag */ 03158 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03159 03160 /* Clear Configuration Register 2 */ 03161 I2C_RESET_CR2(hi2c); 03162 03163 hi2c->XferCount = 0; 03164 03165 hi2c->State = HAL_I2C_STATE_READY; 03166 HAL_I2C_ErrorCallback(hi2c); 03167 } 03168 else 03169 { 03170 /* Enable DMA Request */ 03171 hi2c->Instance->CR1 |= I2C_CR1_TXDMAEN; 03172 } 03173 } 03174 } 03175 else 03176 { 03177 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03178 /* Wait until STOPF flag is reset */ 03179 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03180 { 03181 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03182 { 03183 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03184 } 03185 else 03186 { 03187 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03188 } 03189 } 03190 03191 /* Clear STOP Flag */ 03192 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03193 03194 /* Clear Configuration Register 2 */ 03195 I2C_RESET_CR2(hi2c); 03196 03197 /* Disable DMA Request */ 03198 hi2c->Instance->CR1 &= ~I2C_CR1_TXDMAEN; 03199 03200 hi2c->XferCount = 0; 03201 03202 hi2c->State = HAL_I2C_STATE_READY; 03203 03204 /* Check if Errors has been detected during transfer */ 03205 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03206 { 03207 HAL_I2C_ErrorCallback(hi2c); 03208 } 03209 else 03210 { 03211 HAL_I2C_MasterTxCpltCallback(hi2c); 03212 } 03213 } 03214 } 03215 03216 /** 03217 * @brief DMA I2C slave transmit process complete callback. 03218 * @param hdma: DMA handle 03219 * @retval None 03220 */ 03221 static void I2C_DMASlaveTransmitCplt(DMA_HandleTypeDef *hdma) 03222 { 03223 I2C_HandleTypeDef* hi2c = (I2C_HandleTypeDef*)((DMA_HandleTypeDef*)hdma)->Parent; 03224 03225 /* Wait until STOP flag is set */ 03226 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03227 { 03228 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03229 { 03230 /* Normal Use case, a AF is generated by master */ 03231 /* to inform slave the end of transfer */ 03232 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 03233 } 03234 else 03235 { 03236 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03237 } 03238 } 03239 03240 /* Clear STOP flag */ 03241 __HAL_I2C_CLEAR_FLAG(hi2c,I2C_FLAG_STOPF); 03242 03243 /* Wait until BUSY flag is reset */ 03244 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_BUSY, SET, I2C_TIMEOUT_BUSY) != HAL_OK) 03245 { 03246 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03247 } 03248 03249 /* Disable DMA Request */ 03250 hi2c->Instance->CR1 &= ~I2C_CR1_TXDMAEN; 03251 03252 hi2c->XferCount = 0; 03253 03254 hi2c->State = HAL_I2C_STATE_READY; 03255 03256 /* Check if Errors has been detected during transfer */ 03257 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03258 { 03259 HAL_I2C_ErrorCallback(hi2c); 03260 } 03261 else 03262 { 03263 HAL_I2C_SlaveTxCpltCallback(hi2c); 03264 } 03265 } 03266 03267 /** 03268 * @brief DMA I2C master receive process complete callback 03269 * @param hdma: DMA handle 03270 * @retval None 03271 */ 03272 static void I2C_DMAMasterReceiveCplt(DMA_HandleTypeDef *hdma) 03273 { 03274 I2C_HandleTypeDef* hi2c = (I2C_HandleTypeDef*)((DMA_HandleTypeDef*)hdma)->Parent; 03275 uint16_t DevAddress; 03276 03277 /* Check if last DMA request was done with RELOAD */ 03278 /* Set NBYTES to write and reload if size > 255 */ 03279 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03280 { 03281 /* Wait until TCR flag is set */ 03282 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, I2C_TIMEOUT_TCR) != HAL_OK) 03283 { 03284 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03285 } 03286 03287 /* Disable DMA Request */ 03288 hi2c->Instance->CR1 &= ~I2C_CR1_RXDMAEN; 03289 03290 /* Check if Errors has been detected during transfer */ 03291 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03292 { 03293 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03294 /* Wait until STOPF flag is reset */ 03295 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03296 { 03297 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03298 { 03299 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03300 } 03301 else 03302 { 03303 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03304 } 03305 } 03306 03307 /* Clear STOP Flag */ 03308 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03309 03310 /* Clear Configuration Register 2 */ 03311 I2C_RESET_CR2(hi2c); 03312 03313 hi2c->XferCount = 0; 03314 03315 hi2c->State = HAL_I2C_STATE_READY; 03316 HAL_I2C_ErrorCallback(hi2c); 03317 } 03318 else 03319 { 03320 hi2c->pBuffPtr += hi2c->XferSize; 03321 hi2c->XferCount -= hi2c->XferSize; 03322 if(hi2c->XferCount > 255) 03323 { 03324 hi2c->XferSize = 255; 03325 } 03326 else 03327 { 03328 hi2c->XferSize = hi2c->XferCount; 03329 } 03330 03331 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 03332 03333 /* Enable the DMA channel */ 03334 HAL_DMA_Start_IT(hi2c->hdmarx, (uint32_t)&hi2c->Instance->RXDR, (uint32_t)hi2c->pBuffPtr, hi2c->XferSize); 03335 03336 /* Send Slave Address */ 03337 /* Set NBYTES to write and reload if size > 255 */ 03338 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03339 { 03340 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 03341 } 03342 else 03343 { 03344 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 03345 } 03346 03347 /* Wait until RXNE flag is set */ 03348 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, I2C_TIMEOUT_RXNE) != HAL_OK) 03349 { 03350 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03351 } 03352 03353 /* Check if Errors has been detected during transfer */ 03354 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03355 { 03356 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03357 /* Wait until STOPF flag is reset */ 03358 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03359 { 03360 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03361 { 03362 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03363 } 03364 else 03365 { 03366 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03367 } 03368 } 03369 03370 /* Clear STOP Flag */ 03371 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03372 03373 /* Clear Configuration Register 2 */ 03374 I2C_RESET_CR2(hi2c); 03375 03376 hi2c->XferCount = 0; 03377 03378 hi2c->State = HAL_I2C_STATE_READY; 03379 03380 HAL_I2C_ErrorCallback(hi2c); 03381 } 03382 else 03383 { 03384 /* Enable DMA Request */ 03385 hi2c->Instance->CR1 |= I2C_CR1_RXDMAEN; 03386 } 03387 } 03388 } 03389 else 03390 { 03391 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03392 /* Wait until STOPF flag is reset */ 03393 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03394 { 03395 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03396 { 03397 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03398 } 03399 else 03400 { 03401 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03402 } 03403 } 03404 03405 /* Clear STOP Flag */ 03406 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03407 03408 /* Clear Configuration Register 2 */ 03409 I2C_RESET_CR2(hi2c); 03410 03411 /* Disable DMA Request */ 03412 hi2c->Instance->CR1 &= ~I2C_CR1_RXDMAEN; 03413 03414 hi2c->XferCount = 0; 03415 03416 hi2c->State = HAL_I2C_STATE_READY; 03417 03418 /* Check if Errors has been detected during transfer */ 03419 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03420 { 03421 HAL_I2C_ErrorCallback(hi2c); 03422 } 03423 else 03424 { 03425 HAL_I2C_MasterRxCpltCallback(hi2c); 03426 } 03427 } 03428 } 03429 03430 /** 03431 * @brief DMA I2C slave receive process complete callback. 03432 * @param hdma: DMA handle 03433 * @retval None 03434 */ 03435 static void I2C_DMASlaveReceiveCplt(DMA_HandleTypeDef *hdma) 03436 { 03437 I2C_HandleTypeDef* hi2c = (I2C_HandleTypeDef*)((DMA_HandleTypeDef*)hdma)->Parent; 03438 03439 /* Wait until STOPF flag is reset */ 03440 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03441 { 03442 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03443 { 03444 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03445 } 03446 else 03447 { 03448 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03449 } 03450 } 03451 03452 /* Clear STOPF flag */ 03453 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03454 03455 /* Wait until BUSY flag is reset */ 03456 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_BUSY, SET, I2C_TIMEOUT_BUSY) != HAL_OK) 03457 { 03458 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03459 } 03460 03461 /* Disable DMA Request */ 03462 hi2c->Instance->CR1 &= ~I2C_CR1_RXDMAEN; 03463 03464 /* Disable Address Acknowledge */ 03465 hi2c->Instance->CR2 |= I2C_CR2_NACK; 03466 03467 hi2c->XferCount = 0; 03468 03469 hi2c->State = HAL_I2C_STATE_READY; 03470 03471 /* Check if Errors has been detected during transfer */ 03472 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03473 { 03474 HAL_I2C_ErrorCallback(hi2c); 03475 } 03476 else 03477 { 03478 HAL_I2C_SlaveRxCpltCallback(hi2c); 03479 } 03480 } 03481 03482 /** 03483 * @brief DMA I2C Memory Write process complete callback 03484 * @param hdma : DMA handle 03485 * @retval None 03486 */ 03487 static void I2C_DMAMemTransmitCplt(DMA_HandleTypeDef *hdma) 03488 { 03489 uint16_t DevAddress; 03490 I2C_HandleTypeDef* hi2c = ( I2C_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 03491 03492 /* Check if last DMA request was done with RELOAD */ 03493 /* Set NBYTES to write and reload if size > 255 */ 03494 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03495 { 03496 /* Wait until TCR flag is set */ 03497 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, I2C_TIMEOUT_TCR) != HAL_OK) 03498 { 03499 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03500 } 03501 03502 /* Disable DMA Request */ 03503 hi2c->Instance->CR1 &= ~I2C_CR1_TXDMAEN; 03504 03505 /* Check if Errors has been detected during transfer */ 03506 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03507 { 03508 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03509 /* Wait until STOPF flag is reset */ 03510 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03511 { 03512 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03513 { 03514 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03515 } 03516 else 03517 { 03518 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03519 } 03520 } 03521 03522 /* Clear STOP Flag */ 03523 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03524 03525 /* Clear Configuration Register 2 */ 03526 I2C_RESET_CR2(hi2c); 03527 03528 hi2c->XferCount = 0; 03529 03530 hi2c->State = HAL_I2C_STATE_READY; 03531 HAL_I2C_ErrorCallback(hi2c); 03532 } 03533 else 03534 { 03535 hi2c->pBuffPtr += hi2c->XferSize; 03536 hi2c->XferCount -= hi2c->XferSize; 03537 if(hi2c->XferCount > 255) 03538 { 03539 hi2c->XferSize = 255; 03540 } 03541 else 03542 { 03543 hi2c->XferSize = hi2c->XferCount; 03544 } 03545 03546 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 03547 03548 /* Enable the DMA channel */ 03549 HAL_DMA_Start_IT(hi2c->hdmatx, (uint32_t)hi2c->pBuffPtr, (uint32_t)&hi2c->Instance->TXDR, hi2c->XferSize); 03550 03551 /* Send Slave Address */ 03552 /* Set NBYTES to write and reload if size > 255 */ 03553 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03554 { 03555 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 03556 } 03557 else 03558 { 03559 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 03560 } 03561 03562 /* Wait until TXIS flag is set */ 03563 if(I2C_WaitOnTXISFlagUntilTimeout(hi2c, I2C_TIMEOUT_TXIS) != HAL_OK) 03564 { 03565 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03566 /* Wait until STOPF flag is reset */ 03567 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03568 { 03569 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03570 { 03571 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03572 } 03573 else 03574 { 03575 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03576 } 03577 } 03578 03579 /* Clear STOP Flag */ 03580 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03581 03582 /* Clear Configuration Register 2 */ 03583 I2C_RESET_CR2(hi2c); 03584 03585 hi2c->XferCount = 0; 03586 03587 hi2c->State = HAL_I2C_STATE_READY; 03588 HAL_I2C_ErrorCallback(hi2c); 03589 } 03590 else 03591 { 03592 /* Enable DMA Request */ 03593 hi2c->Instance->CR1 |= I2C_CR1_TXDMAEN; 03594 } 03595 } 03596 } 03597 else 03598 { 03599 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03600 /* Wait until STOPF flag is reset */ 03601 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03602 { 03603 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03604 { 03605 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03606 } 03607 else 03608 { 03609 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03610 } 03611 } 03612 03613 /* Clear STOP Flag */ 03614 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03615 03616 /* Clear Configuration Register 2 */ 03617 I2C_RESET_CR2(hi2c); 03618 03619 /* Disable DMA Request */ 03620 hi2c->Instance->CR1 &= ~I2C_CR1_TXDMAEN; 03621 03622 hi2c->XferCount = 0; 03623 03624 hi2c->State = HAL_I2C_STATE_READY; 03625 03626 /* Check if Errors has been detected during transfer */ 03627 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03628 { 03629 HAL_I2C_ErrorCallback(hi2c); 03630 } 03631 else 03632 { 03633 HAL_I2C_MemTxCpltCallback(hi2c); 03634 } 03635 } 03636 } 03637 03638 /** 03639 * @brief DMA I2C Memory Read process complete callback 03640 * @param hdma: DMA handle 03641 * @retval None 03642 */ 03643 static void I2C_DMAMemReceiveCplt(DMA_HandleTypeDef *hdma) 03644 { 03645 I2C_HandleTypeDef* hi2c = ( I2C_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 03646 uint16_t DevAddress; 03647 03648 /* Check if last DMA request was done with RELOAD */ 03649 /* Set NBYTES to write and reload if size > 255 */ 03650 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03651 { 03652 /* Wait until TCR flag is set */ 03653 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_TCR, RESET, I2C_TIMEOUT_TCR) != HAL_OK) 03654 { 03655 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03656 } 03657 03658 /* Disable DMA Request */ 03659 hi2c->Instance->CR1 &= ~I2C_CR1_RXDMAEN; 03660 03661 /* Check if Errors has been detected during transfer */ 03662 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03663 { 03664 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03665 /* Wait until STOPF flag is reset */ 03666 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03667 { 03668 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03669 { 03670 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03671 } 03672 else 03673 { 03674 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03675 } 03676 } 03677 03678 /* Clear STOP Flag */ 03679 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03680 03681 /* Clear Configuration Register 2 */ 03682 I2C_RESET_CR2(hi2c); 03683 03684 hi2c->XferCount = 0; 03685 03686 hi2c->State = HAL_I2C_STATE_READY; 03687 HAL_I2C_ErrorCallback(hi2c); 03688 } 03689 else 03690 { 03691 hi2c->pBuffPtr += hi2c->XferSize; 03692 hi2c->XferCount -= hi2c->XferSize; 03693 if(hi2c->XferCount > 255) 03694 { 03695 hi2c->XferSize = 255; 03696 } 03697 else 03698 { 03699 hi2c->XferSize = hi2c->XferCount; 03700 } 03701 03702 DevAddress = (hi2c->Instance->CR2 & I2C_CR2_SADD); 03703 03704 /* Enable the DMA channel */ 03705 HAL_DMA_Start_IT(hi2c->hdmarx, (uint32_t)&hi2c->Instance->RXDR, (uint32_t)hi2c->pBuffPtr, hi2c->XferSize); 03706 03707 /* Send Slave Address */ 03708 /* Set NBYTES to write and reload if size > 255 */ 03709 if( (hi2c->XferSize == 255) && (hi2c->XferSize < hi2c->XferCount) ) 03710 { 03711 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_RELOAD_MODE, I2C_NO_STARTSTOP); 03712 } 03713 else 03714 { 03715 I2C_TransferConfig(hi2c,DevAddress,hi2c->XferSize, I2C_AUTOEND_MODE, I2C_NO_STARTSTOP); 03716 } 03717 03718 /* Wait until RXNE flag is set */ 03719 if(I2C_WaitOnFlagUntilTimeout(hi2c, I2C_FLAG_RXNE, RESET, I2C_TIMEOUT_RXNE) != HAL_OK) 03720 { 03721 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03722 } 03723 03724 /* Check if Errors has been detected during transfer */ 03725 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03726 { 03727 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03728 /* Wait until STOPF flag is reset */ 03729 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03730 { 03731 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03732 { 03733 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03734 } 03735 else 03736 { 03737 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03738 } 03739 } 03740 03741 /* Clear STOP Flag */ 03742 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03743 03744 /* Clear Configuration Register 2 */ 03745 I2C_RESET_CR2(hi2c); 03746 03747 hi2c->XferCount = 0; 03748 03749 hi2c->State = HAL_I2C_STATE_READY; 03750 HAL_I2C_ErrorCallback(hi2c); 03751 } 03752 else 03753 { 03754 /* Enable DMA Request */ 03755 hi2c->Instance->CR1 |= I2C_CR1_RXDMAEN; 03756 } 03757 } 03758 } 03759 else 03760 { 03761 /* No need to Check TC flag, with AUTOEND mode the stop is automatically generated */ 03762 /* Wait until STOPF flag is reset */ 03763 if(I2C_WaitOnSTOPFlagUntilTimeout(hi2c, I2C_TIMEOUT_STOPF) != HAL_OK) 03764 { 03765 if(hi2c->ErrorCode == HAL_I2C_ERROR_AF) 03766 { 03767 hi2c->ErrorCode |= HAL_I2C_ERROR_AF; 03768 } 03769 else 03770 { 03771 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03772 } 03773 } 03774 03775 /* Clear STOP Flag */ 03776 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03777 03778 /* Clear Configuration Register 2 */ 03779 I2C_RESET_CR2(hi2c); 03780 03781 /* Disable DMA Request */ 03782 hi2c->Instance->CR1 &= ~I2C_CR1_RXDMAEN; 03783 03784 hi2c->XferCount = 0; 03785 03786 hi2c->State = HAL_I2C_STATE_READY; 03787 03788 /* Check if Errors has been detected during transfer */ 03789 if(hi2c->ErrorCode != HAL_I2C_ERROR_NONE) 03790 { 03791 HAL_I2C_ErrorCallback(hi2c); 03792 } 03793 else 03794 { 03795 HAL_I2C_MemRxCpltCallback(hi2c); 03796 } 03797 } 03798 } 03799 03800 /** 03801 * @brief DMA I2C communication error callback. 03802 * @param hdma : DMA handle 03803 * @retval None 03804 */ 03805 static void I2C_DMAError(DMA_HandleTypeDef *hdma) 03806 { 03807 I2C_HandleTypeDef* hi2c = ( I2C_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 03808 03809 /* Disable Acknowledge */ 03810 hi2c->Instance->CR2 |= I2C_CR2_NACK; 03811 03812 hi2c->XferCount = 0; 03813 03814 hi2c->State = HAL_I2C_STATE_READY; 03815 03816 hi2c->ErrorCode |= HAL_I2C_ERROR_DMA; 03817 03818 HAL_I2C_ErrorCallback(hi2c); 03819 } 03820 03821 /** 03822 * @brief This function handles I2C Communication Timeout. 03823 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 03824 * the configuration information for the specified I2C. 03825 * @param Flag: specifies the I2C flag to check. 03826 * @param Status: The new Flag status (SET or RESET). 03827 * @param Timeout: Timeout duration 03828 * @retval HAL status 03829 */ 03830 static HAL_StatusTypeDef I2C_WaitOnFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Flag, FlagStatus Status, uint32_t Timeout) 03831 { 03832 uint32_t tickstart = HAL_GetTick(); 03833 03834 /* Wait until flag is set */ 03835 if(Status == RESET) 03836 { 03837 while(__HAL_I2C_GET_FLAG(hi2c, Flag) == RESET) 03838 { 03839 /* Check for the Timeout */ 03840 if(Timeout != HAL_MAX_DELAY) 03841 { 03842 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 03843 { 03844 hi2c->State= HAL_I2C_STATE_READY; 03845 /* Process Unlocked */ 03846 __HAL_UNLOCK(hi2c); 03847 return HAL_TIMEOUT; 03848 } 03849 } 03850 } 03851 } 03852 else 03853 { 03854 while(__HAL_I2C_GET_FLAG(hi2c, Flag) != RESET) 03855 { 03856 /* Check for the Timeout */ 03857 if(Timeout != HAL_MAX_DELAY) 03858 { 03859 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 03860 { 03861 hi2c->State= HAL_I2C_STATE_READY; 03862 /* Process Unlocked */ 03863 __HAL_UNLOCK(hi2c); 03864 return HAL_TIMEOUT; 03865 } 03866 } 03867 } 03868 } 03869 return HAL_OK; 03870 } 03871 03872 /** 03873 * @brief This function handles I2C Communication Timeout for specific usage of TXIS flag. 03874 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 03875 * the configuration information for the specified I2C. 03876 * @param Timeout: Timeout duration 03877 * @retval HAL status 03878 */ 03879 static HAL_StatusTypeDef I2C_WaitOnTXISFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout) 03880 { 03881 uint32_t tickstart = HAL_GetTick(); 03882 03883 while(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_TXIS) == RESET) 03884 { 03885 /* Check if a NACK is detected */ 03886 if(I2C_IsAcknowledgeFailed(hi2c, Timeout) != HAL_OK) 03887 { 03888 return HAL_ERROR; 03889 } 03890 03891 /* Check for the Timeout */ 03892 if(Timeout != HAL_MAX_DELAY) 03893 { 03894 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 03895 { 03896 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03897 hi2c->State= HAL_I2C_STATE_READY; 03898 03899 /* Process Unlocked */ 03900 __HAL_UNLOCK(hi2c); 03901 03902 return HAL_TIMEOUT; 03903 } 03904 } 03905 } 03906 return HAL_OK; 03907 } 03908 03909 /** 03910 * @brief This function handles I2C Communication Timeout for specific usage of STOP flag. 03911 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 03912 * the configuration information for the specified I2C. 03913 * @param Timeout: Timeout duration 03914 * @retval HAL status 03915 */ 03916 static HAL_StatusTypeDef I2C_WaitOnSTOPFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout) 03917 { 03918 uint32_t tickstart = 0x00; 03919 tickstart = HAL_GetTick(); 03920 03921 while(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == RESET) 03922 { 03923 /* Check if a NACK is detected */ 03924 if(I2C_IsAcknowledgeFailed(hi2c, Timeout) != HAL_OK) 03925 { 03926 return HAL_ERROR; 03927 } 03928 03929 /* Check for the Timeout */ 03930 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 03931 { 03932 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03933 hi2c->State= HAL_I2C_STATE_READY; 03934 03935 /* Process Unlocked */ 03936 __HAL_UNLOCK(hi2c); 03937 03938 return HAL_TIMEOUT; 03939 } 03940 } 03941 return HAL_OK; 03942 } 03943 03944 /** 03945 * @brief This function handles I2C Communication Timeout for specific usage of RXNE flag. 03946 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 03947 * the configuration information for the specified I2C. 03948 * @param Timeout: Timeout duration 03949 * @retval HAL status 03950 */ 03951 static HAL_StatusTypeDef I2C_WaitOnRXNEFlagUntilTimeout(I2C_HandleTypeDef *hi2c, uint32_t Timeout) 03952 { 03953 uint32_t tickstart = 0x00; 03954 tickstart = HAL_GetTick(); 03955 03956 while(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_RXNE) == RESET) 03957 { 03958 /* Check if a STOPF is detected */ 03959 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == SET) 03960 { 03961 /* Clear STOP Flag */ 03962 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 03963 03964 /* Clear Configuration Register 2 */ 03965 I2C_RESET_CR2(hi2c); 03966 03967 hi2c->ErrorCode = HAL_I2C_ERROR_NONE; 03968 hi2c->State= HAL_I2C_STATE_READY; 03969 03970 /* Process Unlocked */ 03971 __HAL_UNLOCK(hi2c); 03972 03973 return HAL_ERROR; 03974 } 03975 03976 /* Check for the Timeout */ 03977 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 03978 { 03979 hi2c->ErrorCode |= HAL_I2C_ERROR_TIMEOUT; 03980 hi2c->State= HAL_I2C_STATE_READY; 03981 03982 /* Process Unlocked */ 03983 __HAL_UNLOCK(hi2c); 03984 03985 return HAL_TIMEOUT; 03986 } 03987 } 03988 return HAL_OK; 03989 } 03990 03991 /** 03992 * @brief This function handles Acknowledge failed detection during an I2C Communication. 03993 * @param hi2c : Pointer to a I2C_HandleTypeDef structure that contains 03994 * the configuration information for the specified I2C. 03995 * @param Timeout: Timeout duration 03996 * @retval HAL status 03997 */ 03998 static HAL_StatusTypeDef I2C_IsAcknowledgeFailed(I2C_HandleTypeDef *hi2c, uint32_t Timeout) 03999 { 04000 uint32_t tickstart = 0x00; 04001 tickstart = HAL_GetTick(); 04002 04003 if(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_AF) == SET) 04004 { 04005 /* Generate stop if necessary only in case of I2C peripheral in MASTER mode */ 04006 if((hi2c->State == HAL_I2C_STATE_MASTER_BUSY_TX) || (hi2c->State == HAL_I2C_STATE_MEM_BUSY_TX) 04007 || (hi2c->State == HAL_I2C_STATE_MEM_BUSY_RX)) 04008 { 04009 /* No need to generate the STOP condition if AUTOEND mode is enabled */ 04010 /* Generate the STOP condition only in case of SOFTEND mode is enabled */ 04011 if((hi2c->Instance->CR2 & I2C_AUTOEND_MODE) != I2C_AUTOEND_MODE) 04012 { 04013 /* Generate Stop */ 04014 hi2c->Instance->CR2 |= I2C_CR2_STOP; 04015 } 04016 } 04017 04018 /* Wait until STOP Flag is reset */ 04019 /* AutoEnd should be initiate after AF */ 04020 while(__HAL_I2C_GET_FLAG(hi2c, I2C_FLAG_STOPF) == RESET) 04021 { 04022 /* Check for the Timeout */ 04023 if(Timeout != HAL_MAX_DELAY) 04024 { 04025 if((Timeout == 0)||((HAL_GetTick() - tickstart ) > Timeout)) 04026 { 04027 hi2c->State= HAL_I2C_STATE_READY; 04028 /* Process Unlocked */ 04029 __HAL_UNLOCK(hi2c); 04030 return HAL_TIMEOUT; 04031 } 04032 } 04033 } 04034 04035 /* Clear NACKF Flag */ 04036 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_AF); 04037 04038 /* Clear STOP Flag */ 04039 __HAL_I2C_CLEAR_FLAG(hi2c, I2C_FLAG_STOPF); 04040 04041 /* Clear Configuration Register 2 */ 04042 I2C_RESET_CR2(hi2c); 04043 04044 hi2c->ErrorCode = HAL_I2C_ERROR_AF; 04045 hi2c->State= HAL_I2C_STATE_READY; 04046 04047 /* Process Unlocked */ 04048 __HAL_UNLOCK(hi2c); 04049 04050 return HAL_ERROR; 04051 } 04052 return HAL_OK; 04053 } 04054 04055 /** 04056 * @brief Handles I2Cx communication when starting transfer or during transfer (TC or TCR flag are set). 04057 * @param hi2c: I2C handle. 04058 * @param DevAddress: specifies the slave address to be programmed. 04059 * @param Size: specifies the number of bytes to be programmed. 04060 * This parameter must be a value between 0 and 255. 04061 * @param Mode: new state of the I2C START condition generation. 04062 * This parameter can be one of the following values: 04063 * @arg I2C_RELOAD_MODE: Enable Reload mode . 04064 * @arg I2C_AUTOEND_MODE: Enable Automatic end mode. 04065 * @arg I2C_SOFTEND_MODE: Enable Software end mode. 04066 * @param Request: new state of the I2C START condition generation. 04067 * This parameter can be one of the following values: 04068 * @arg I2C_NO_STARTSTOP: Don't Generate stop and start condition. 04069 * @arg I2C_GENERATE_STOP: Generate stop condition (Size should be set to 0). 04070 * @arg I2C_GENERATE_START_READ: Generate Restart for read request. 04071 * @arg I2C_GENERATE_START_WRITE: Generate Restart for write request. 04072 * @retval None 04073 */ 04074 static void I2C_TransferConfig(I2C_HandleTypeDef *hi2c, uint16_t DevAddress, uint8_t Size, uint32_t Mode, uint32_t Request) 04075 { 04076 uint32_t tmpreg = 0; 04077 04078 /* Check the parameters */ 04079 assert_param(IS_I2C_ALL_INSTANCE(hi2c->Instance)); 04080 assert_param(IS_TRANSFER_MODE(Mode)); 04081 assert_param(IS_TRANSFER_REQUEST(Request)); 04082 04083 /* Get the CR2 register value */ 04084 tmpreg = hi2c->Instance->CR2; 04085 04086 /* clear tmpreg specific bits */ 04087 tmpreg &= (uint32_t)~((uint32_t)(I2C_CR2_SADD | I2C_CR2_NBYTES | I2C_CR2_RELOAD | I2C_CR2_AUTOEND | I2C_CR2_RD_WRN | I2C_CR2_START | I2C_CR2_STOP)); 04088 04089 /* update tmpreg */ 04090 tmpreg |= (uint32_t)(((uint32_t)DevAddress & I2C_CR2_SADD) | (((uint32_t)Size << 16 ) & I2C_CR2_NBYTES) | \ 04091 (uint32_t)Mode | (uint32_t)Request); 04092 04093 /* update CR2 register */ 04094 hi2c->Instance->CR2 = tmpreg; 04095 } 04096 04097 /** 04098 * @} 04099 */ 04100 04101 #endif /* HAL_I2C_MODULE_ENABLED */ 04102 /** 04103 * @} 04104 */ 04105 04106 /** 04107 * @} 04108 */ 04109 04110 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 04111
Generated on Tue Jul 12 2022 11:35:11 by
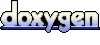