Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_hal_flash.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_flash.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of FLASH HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_HAL_FLASH_H 00040 #define __STM32L4xx_HAL_FLASH_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_HAL_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup FLASH 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup FLASH_Exported_Types FLASH Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief FLASH Erase structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t TypeErase; /*!< Mass erase or page erase. 00068 This parameter can be a value of @ref FLASH_Type_Erase */ 00069 uint32_t Banks; /*!< Select bank to erase. 00070 This parameter must be a value of @ref FLASH_Banks 00071 (FLASH_BANK_BOTH should be used only for mass erase) */ 00072 uint32_t Page; /*!< Initial Flash page to erase when page erase is disabled 00073 This parameter must be a value between 0 and (max number of pages in the bank - 1) 00074 (eg : 255 for 1MB dual bank) */ 00075 uint32_t NbPages; /*!< Number of pages to be erased. 00076 This parameter must be a value between 1 and (max number of pages in the bank - value of initial page)*/ 00077 } FLASH_EraseInitTypeDef; 00078 00079 /** 00080 * @brief FLASH Option Bytes Program structure definition 00081 */ 00082 typedef struct 00083 { 00084 uint32_t OptionType; /*!< Option byte to be configured. 00085 This parameter can be a combination of the values of @ref FLASH_OB_Type */ 00086 uint32_t WRPArea; /*!< Write protection area to be programmed (used for OPTIONBYTE_WRP). 00087 Only one WRP area could be programmed at the same time. 00088 This parameter can be value of @ref FLASH_OB_WRP_Area */ 00089 uint32_t WRPStartOffset; /*!< Write protection start offset (used for OPTIONBYTE_WRP). 00090 This parameter must be a value between 0 and (max number of pages in the bank - 1) 00091 (eg : 25 for 1MB dual bank) */ 00092 uint32_t WRPEndOffset; /*!< Write protection end offset (used for OPTIONBYTE_WRP). 00093 This parameter must be a value between WRPStartOffset and (max number of pages in the bank - 1) */ 00094 uint32_t RDPLevel; /*!< Set the read protection level.. (used for OPTIONBYTE_RDP). 00095 This parameter can be a value of @ref FLASH_OB_Read_Protection */ 00096 uint32_t USERType; /*!< User option byte(s) to be configured (used for OPTIONBYTE_USER). 00097 This parameter can be a combination of @ref FLASH_OB_USER_Type */ 00098 uint32_t USERConfig; /*!< Value of the user option byte (used for OPTIONBYTE_USER). 00099 This parameter can be a combination of @ref FLASH_OB_USER_BOR_LEVEL, 00100 @ref FLASH_OB_USER_nRST_STOP, @ref FLASH_OB_USER_nRST_STANDBY, 00101 @ref FLASH_OB_USER_nRST_SHUTDOWN, @ref FLASH_OB_USER_IWDG_SW, 00102 @ref FLASH_OB_USER_IWDG_STOP, @ref FLASH_OB_USER_IWDG_STANDBY, 00103 @ref FLASH_OB_USER_WWDG_SW, @ref FLASH_OB_USER_BFB2, 00104 @ref FLASH_OB_USER_DUALBANK, @ref FLASH_OB_USER_nBOOT1, 00105 @ref FLASH_OB_USER_SRAM2_PE and @ref FLASH_OB_USER_SRAM2_RST */ 00106 uint32_t PCROPConfig; /*!< Configuration of the PCROP (used for OPTIONBYTE_PCROP). 00107 This parameter must be a combination of @ref FLASH_Banks (except FLASH_BANK_BOTH) 00108 and @ref FLASH_OB_PCROP_RDP */ 00109 uint32_t PCROPStartAddr; /*!< PCROP Start address (used for OPTIONBYTE_PCROP). 00110 This parameter must be a value between begin and end of bank 00111 => Be careful of the bank swapping for the address */ 00112 uint32_t PCROPEndAddr; /*!< PCROP End address (used for OPTIONBYTE_PCROP). 00113 This parameter must be a value between PCROP Start address and end of bank */ 00114 } FLASH_OBProgramInitTypeDef; 00115 00116 /** 00117 * @brief FLASH Procedure structure definition 00118 */ 00119 typedef enum 00120 { 00121 FLASH_PROC_NONE = 0, 00122 FLASH_PROC_PAGE_ERASE, 00123 FLASH_PROC_MASS_ERASE, 00124 FLASH_PROC_PROGRAM, 00125 FLASH_PROC_PROGRAM_LAST 00126 } FLASH_ProcedureTypeDef; 00127 00128 /** 00129 * @brief FLASH handle Structure definition 00130 */ 00131 typedef struct 00132 { 00133 HAL_LockTypeDef Lock; /* FLASH locking object */ 00134 __IO uint32_t ErrorCode; /* FLASH error code */ 00135 __IO FLASH_ProcedureTypeDef ProcedureOnGoing; /* Internal variable to indicate which procedure is ongoing or not in IT context */ 00136 __IO uint32_t Address; /* Internal variable to save address selected for program in IT context */ 00137 __IO uint32_t Bank; /* Internal variable to save current bank selected during erase in IT context */ 00138 __IO uint32_t Page; /* Internal variable to define the current page which is erasing in IT context */ 00139 __IO uint32_t NbPagesToErase; /* Internal variable to save the remaining pages to erase in IT context */ 00140 }FLASH_ProcessTypeDef; 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /* Exported constants --------------------------------------------------------*/ 00147 /** @defgroup FLASH_Exported_Constants FLASH Exported Constants 00148 * @{ 00149 */ 00150 00151 /** @defgroup FLASH_Error FLASH Error 00152 * @{ 00153 */ 00154 #define HAL_FLASH_ERROR_NONE ((uint32_t)0x00000000) 00155 #define HAL_FLASH_ERROR_OP ((uint32_t)0x00000001) 00156 #define HAL_FLASH_ERROR_PROG ((uint32_t)0x00000002) 00157 #define HAL_FLASH_ERROR_WRP ((uint32_t)0x00000004) 00158 #define HAL_FLASH_ERROR_PGA ((uint32_t)0x00000008) 00159 #define HAL_FLASH_ERROR_SIZ ((uint32_t)0x00000010) 00160 #define HAL_FLASH_ERROR_PGS ((uint32_t)0x00000020) 00161 #define HAL_FLASH_ERROR_MIS ((uint32_t)0x00000040) 00162 #define HAL_FLASH_ERROR_FAST ((uint32_t)0x00000080) 00163 #define HAL_FLASH_ERROR_RD ((uint32_t)0x00000100) 00164 #define HAL_FLASH_ERROR_OPTV ((uint32_t)0x00000200) 00165 #define HAL_FLASH_ERROR_ECCD ((uint32_t)0x00000400) 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup FLASH_Type_Erase FLASH Erase Type 00171 * @{ 00172 */ 00173 #define FLASH_TYPEERASE_PAGES ((uint32_t)0x00) /*!<Pages erase only*/ 00174 #define FLASH_TYPEERASE_MASSERASE ((uint32_t)0x01) /*!<Flash mass erase activation*/ 00175 /** 00176 * @} 00177 */ 00178 00179 /** @defgroup FLASH_Banks FLASH Banks 00180 * @{ 00181 */ 00182 #define FLASH_BANK_1 ((uint32_t)0x01) /*!< Bank 1 */ 00183 #define FLASH_BANK_2 ((uint32_t)0x02) /*!< Bank 2 */ 00184 #define FLASH_BANK_BOTH ((uint32_t)(FLASH_BANK_1 | FLASH_BANK_2)) /*!< Bank1 and Bank2 */ 00185 /** 00186 * @} 00187 */ 00188 00189 00190 /** @defgroup FLASH_Type_Program FLASH Program Type 00191 * @{ 00192 */ 00193 #define FLASH_TYPEPROGRAM_DOUBLEWORD ((uint32_t)0x00) /*!<Program a double-word (64-bit) at a specified address.*/ 00194 #define FLASH_TYPEPROGRAM_FAST ((uint32_t)0x01) /*!<Fast program a 32 row double-word (64-bit) at a specified address. 00195 And another 32 row double-word (64-bit) will be programmed */ 00196 #define FLASH_TYPEPROGRAM_FAST_AND_LAST ((uint32_t)0x02) /*!<Fast program a 32 row double-word (64-bit) at a specified address. 00197 And this is the last 32 row double-word (64-bit) programmed */ 00198 /** 00199 * @} 00200 */ 00201 00202 /** @defgroup FLASH_OB_Type FLASH Option Bytes Type 00203 * @{ 00204 */ 00205 #define OPTIONBYTE_WRP ((uint32_t)0x01) /*!< WRP option byte configuration */ 00206 #define OPTIONBYTE_RDP ((uint32_t)0x02) /*!< RDP option byte configuration */ 00207 #define OPTIONBYTE_USER ((uint32_t)0x04) /*!< USER option byte configuration */ 00208 #define OPTIONBYTE_PCROP ((uint32_t)0x08) /*!< PCROP option byte configuration */ 00209 /** 00210 * @} 00211 */ 00212 00213 /** @defgroup FLASH_OB_WRP_Area FLASH WRP Area 00214 * @{ 00215 */ 00216 #define OB_WRPAREA_BANK1_AREAA ((uint32_t)0x00) /*!< Flash Bank 1 Area A */ 00217 #define OB_WRPAREA_BANK1_AREAB ((uint32_t)0x01) /*!< Flash Bank 1 Area B */ 00218 #define OB_WRPAREA_BANK2_AREAA ((uint32_t)0x02) /*!< Flash Bank 2 Area A */ 00219 #define OB_WRPAREA_BANK2_AREAB ((uint32_t)0x04) /*!< Flash Bank 2 Area B */ 00220 /** 00221 * @} 00222 */ 00223 00224 /** @defgroup FLASH_OB_Read_Protection FLASH Option Bytes Read Protection 00225 * @{ 00226 */ 00227 #define OB_RDP_LEVEL_0 ((uint32_t)0xAA) 00228 #define OB_RDP_LEVEL_1 ((uint32_t)0xBB) 00229 #define OB_RDP_LEVEL_2 ((uint32_t)0xCC) /*!< Warning: When enabling read protection level 2 00230 it's no more possible to go back to level 1 or 0 */ 00231 /** 00232 * @} 00233 */ 00234 00235 /** @defgroup FLASH_OB_USER_Type FLASH Option Bytes User Type 00236 * @{ 00237 */ 00238 #define OB_USER_BOR_LEV ((uint32_t)0x0001) /*!< BOR reset Level */ 00239 #define OB_USER_nRST_STOP ((uint32_t)0x0002) /*!< Reset generated when entering the stop mode */ 00240 #define OB_USER_nRST_STDBY ((uint32_t)0x0004) /*!< Reset generated when entering the standby mode */ 00241 #define OB_USER_IWDG_SW ((uint32_t)0x0008) /*!< Independent watchdog selection */ 00242 #define OB_USER_IWDG_STOP ((uint32_t)0x0010) /*!< Independent watchdog counter freeze in stop mode */ 00243 #define OB_USER_IWDG_STDBY ((uint32_t)0x0020) /*!< Independent watchdog counter freeze in standby mode */ 00244 #define OB_USER_WWDG_SW ((uint32_t)0x0040) /*!< Window watchdog selection */ 00245 #define OB_USER_BFB2 ((uint32_t)0x0080) /*!< Dual-bank boot */ 00246 #define OB_USER_DUALBANK ((uint32_t)0x0100) /*!< Dual-Bank on 512KB or 256KB Flash memory devices */ 00247 #define OB_USER_nBOOT1 ((uint32_t)0x0200) /*!< Boot configuration */ 00248 #define OB_USER_SRAM2_PE ((uint32_t)0x0400) /*!< SRAM2 parity check enable */ 00249 #define OB_USER_SRAM2_RST ((uint32_t)0x0800) /*!< SRAM2 Erase when system reset */ 00250 #define OB_USER_nRST_SHDW ((uint32_t)0x1000) /*!< Reset generated when entering the shutdown mode */ 00251 /** 00252 * @} 00253 */ 00254 00255 /** @defgroup FLASH_OB_USER_BOR_LEVEL FLASH Option Bytes User BOR Level 00256 * @{ 00257 */ 00258 #define OB_BOR_LEVEL_0 ((uint32_t)FLASH_OPTR_BOR_LEV_0) /*!< Reset level threshold is around 1.7V */ 00259 #define OB_BOR_LEVEL_1 ((uint32_t)FLASH_OPTR_BOR_LEV_1) /*!< Reset level threshold is around 2.0V */ 00260 #define OB_BOR_LEVEL_2 ((uint32_t)FLASH_OPTR_BOR_LEV_2) /*!< Reset level threshold is around 2.2V */ 00261 #define OB_BOR_LEVEL_3 ((uint32_t)FLASH_OPTR_BOR_LEV_3) /*!< Reset level threshold is around 2.5V */ 00262 #define OB_BOR_LEVEL_4 ((uint32_t)FLASH_OPTR_BOR_LEV_4) /*!< Reset level threshold is around 2.8V */ 00263 /** 00264 * @} 00265 */ 00266 00267 /** @defgroup FLASH_OB_USER_nRST_STOP FLASH Option Bytes User Reset On Stop 00268 * @{ 00269 */ 00270 #define OB_STOP_RST ((uint32_t)0x0000) /*!< Reset generated when entering the stop mode */ 00271 #define OB_STOP_NORST ((uint32_t)FLASH_OPTR_nRST_STOP) /*!< No reset generated when entering the stop mode */ 00272 /** 00273 * @} 00274 */ 00275 00276 /** @defgroup FLASH_OB_USER_nRST_STANDBY FLASH Option Bytes User Reset On Standby 00277 * @{ 00278 */ 00279 #define OB_STANDBY_RST ((uint32_t)0x0000) /*!< Reset generated when entering the standby mode */ 00280 #define OB_STANDBY_NORST ((uint32_t)FLASH_OPTR_nRST_STDBY) /*!< No reset generated when entering the standby mode */ 00281 /** 00282 * @} 00283 */ 00284 00285 /** @defgroup FLASH_OB_USER_nRST_SHUTDOWN FLASH Option Bytes User Reset On Shutdown 00286 * @{ 00287 */ 00288 #define OB_SHUTDOWN_RST ((uint32_t)0x0000) /*!< Reset generated when entering the shutdown mode */ 00289 #define OB_SHUTDOWN_NORST ((uint32_t)FLASH_OPTR_nRST_SHDW) /*!< No reset generated when entering the shutdown mode */ 00290 /** 00291 * @} 00292 */ 00293 00294 /** @defgroup FLASH_OB_USER_IWDG_SW FLASH Option Bytes User IWDG Type 00295 * @{ 00296 */ 00297 #define OB_IWDG_HW ((uint32_t)0x00000) /*!< Hardware independent watchdog */ 00298 #define OB_IWDG_SW ((uint32_t)FLASH_OPTR_IWDG_SW) /*!< Software independent watchdog */ 00299 /** 00300 * @} 00301 */ 00302 00303 /** @defgroup FLASH_OB_USER_IWDG_STOP FLASH Option Bytes User IWDG Mode On Stop 00304 * @{ 00305 */ 00306 #define OB_IWDG_STOP_FREEZE ((uint32_t)0x00000) /*!< Independent watchdog counter is frozen in Stop mode */ 00307 #define OB_IWDG_STOP_RUN ((uint32_t)FLASH_OPTR_IWDG_STOP) /*!< Independent watchdog counter is running in Stop mode */ 00308 /** 00309 * @} 00310 */ 00311 00312 /** @defgroup FLASH_OB_USER_IWDG_STANDBY FLASH Option Bytes User IWDG Mode On Standby 00313 * @{ 00314 */ 00315 #define OB_IWDG_STDBY_FREEZE ((uint32_t)0x00000) /*!< Independent watchdog counter is frozen in Standby mode */ 00316 #define OB_IWDG_STDBY_RUN ((uint32_t)FLASH_OPTR_IWDG_STDBY) /*!< Independent watchdog counter is running in Standby mode */ 00317 /** 00318 * @} 00319 */ 00320 00321 /** @defgroup FLASH_OB_USER_WWDG_SW FLASH Option Bytes User WWDG Type 00322 * @{ 00323 */ 00324 #define OB_WWDG_HW ((uint32_t)0x00000) /*!< Hardware window watchdog */ 00325 #define OB_WWDG_SW ((uint32_t)FLASH_OPTR_WWDG_SW) /*!< Software window watchdog */ 00326 /** 00327 * @} 00328 */ 00329 00330 /** @defgroup FLASH_OB_USER_BFB2 FLASH Option Bytes User BFB2 Mode 00331 * @{ 00332 */ 00333 #define OB_BFB2_DISABLE ((uint32_t)0x000000) /*!< Dual-bank boot disable */ 00334 #define OB_BFB2_ENABLE ((uint32_t)FLASH_OPTR_BFB2) /*!< Dual-bank boot enable */ 00335 /** 00336 * @} 00337 */ 00338 00339 /** @defgroup FLASH_OB_USER_DUALBANK FLASH Option Bytes User Dual-bank Type 00340 * @{ 00341 */ 00342 #define OB_DUALBANK_SINGLE ((uint32_t)0x000000) /*!< 256 KB/512 KB Single-bank Flash */ 00343 #define OB_DUALBANK_DUAL ((uint32_t)FLASH_OPTR_DUALBANK) /*!< 256 KB/512 KB Dual-bank Flash */ 00344 /** 00345 * @} 00346 */ 00347 00348 /** @defgroup FLASH_OB_USER_nBOOT1 FLASH Option Bytes User BOOT1 Type 00349 * @{ 00350 */ 00351 #define OB_BOOT1_SRAM ((uint32_t)0x000000) /*!< Embedded SRAM1 is selected as boot space (if BOOT0=1) */ 00352 #define OB_BOOT1_SYSTEM ((uint32_t)FLASH_OPTR_nBOOT1) /*!< System memory is selected as boot space (if BOOT0=1) */ 00353 /** 00354 * @} 00355 */ 00356 00357 /** @defgroup FLASH_OB_USER_SRAM2_PE FLASH Option Bytes User SRAM2 Parity Check Type 00358 * @{ 00359 */ 00360 #define OB_SRAM2_PARITY_ENABLE ((uint32_t)0x0000000) /*!< SRAM2 parity check enable */ 00361 #define OB_SRAM2_PARITY_DISABLE ((uint32_t)FLASH_OPTR_SRAM2_PE) /*!< SRAM2 parity check disable */ 00362 /** 00363 * @} 00364 */ 00365 00366 /** @defgroup FLASH_OB_USER_SRAM2_RST FLASH Option Bytes User SRAM2 Erase On Reset Type 00367 * @{ 00368 */ 00369 #define OB_SRAM2_RST_ERASE ((uint32_t)0x0000000) /*!< SRAM2 erased when a system reset occurs */ 00370 #define OB_SRAM2_RST_NOT_ERASE ((uint32_t)FLASH_OPTR_SRAM2_RST) /*!< SRAM2 is not erased when a system reset occurs */ 00371 /** 00372 * @} 00373 */ 00374 00375 /** @defgroup FLASH_OB_PCROP_RDP FLASH Option Bytes PCROP On RDP Level Type 00376 * @{ 00377 */ 00378 #define OB_PCROP_RDP_NOT_ERASE ((uint32_t)0x00000000) /*!< PCROP area is not erased when the RDP level 00379 is decreased from Level 1 to Level 0 */ 00380 #define OB_PCROP_RDP_ERASE ((uint32_t)FLASH_PCROP1ER_PCROP_RDP) /*!< PCROP area is erased when the RDP level is 00381 decreased from Level 1 to Level 0 (full mass erase) */ 00382 /** 00383 * @} 00384 */ 00385 00386 /** @defgroup FLASH_Latency FLASH Latency 00387 * @{ 00388 */ 00389 #define FLASH_LATENCY_0 FLASH_ACR_LATENCY_0WS /*!< FLASH Zero wait state */ 00390 #define FLASH_LATENCY_1 FLASH_ACR_LATENCY_1WS /*!< FLASH One wait state */ 00391 #define FLASH_LATENCY_2 FLASH_ACR_LATENCY_2WS /*!< FLASH Two wait states */ 00392 #define FLASH_LATENCY_3 FLASH_ACR_LATENCY_3WS /*!< FLASH Three wait states */ 00393 #define FLASH_LATENCY_4 FLASH_ACR_LATENCY_4WS /*!< FLASH Four wait states */ 00394 /** 00395 * @} 00396 */ 00397 00398 /** @defgroup FLASH_Keys FLASH Keys 00399 * @{ 00400 */ 00401 #define FLASH_KEY1 ((uint32_t)0x45670123) /*!< Flash key1 */ 00402 #define FLASH_KEY2 ((uint32_t)0xCDEF89AB) /*!< Flash key2: used with FLASH_KEY1 00403 to unlock the FLASH registers access */ 00404 00405 #define FLASH_PDKEY1 ((uint32_t)0x04152637) /*!< Flash power down key1 */ 00406 #define FLASH_PDKEY2 ((uint32_t)0xFAFBFCFD) /*!< Flash power down key2: used with FLASH_PDKEY1 00407 to unlock the RUN_PD bit in FLASH_ACR */ 00408 00409 #define FLASH_OPTKEY1 ((uint32_t)0x08192A3B) /*!< Flash option byte key1 */ 00410 #define FLASH_OPTKEY2 ((uint32_t)0x4C5D6E7F) /*!< Flash option byte key2: used with FLASH_OPTKEY1 00411 to allow option bytes operations */ 00412 /** 00413 * @} 00414 */ 00415 00416 /** @defgroup FLASH_Flags FLASH Flags Definition 00417 * @{ 00418 */ 00419 #define FLASH_FLAG_EOP FLASH_SR_EOP /*!< FLASH End of operation flag */ 00420 #define FLASH_FLAG_OPERR FLASH_SR_OPERR /*!< FLASH Operation error flag */ 00421 #define FLASH_FLAG_PROGERR FLASH_SR_PROGERR /*!< FLASH Programming error flag */ 00422 #define FLASH_FLAG_WRPERR FLASH_SR_WRPERR /*!< FLASH Write protection error flag */ 00423 #define FLASH_FLAG_PGAERR FLASH_SR_PGAERR /*!< FLASH Programming alignment error flag */ 00424 #define FLASH_FLAG_SIZERR FLASH_SR_SIZERR /*!< FLASH Size error flag */ 00425 #define FLASH_FLAG_PGSERR FLASH_SR_PGSERR /*!< FLASH Programming sequence error flag */ 00426 #define FLASH_FLAG_MISERR FLASH_SR_MISERR /*!< FLASH Fast programming data miss error flag */ 00427 #define FLASH_FLAG_FASTERR FLASH_SR_FASTERR /*!< FLASH Fast programming error flag */ 00428 #define FLASH_FLAG_RDERR FLASH_SR_RDERR /*!< FLASH PCROP read error flag */ 00429 #define FLASH_FLAG_OPTVERR FLASH_SR_OPTVERR /*!< FLASH Option validity error flag */ 00430 #define FLASH_FLAG_BSY FLASH_SR_BSY /*!< FLASH Busy flag */ 00431 #define FLASH_FLAG_ECCC FLASH_ECCR_ECCC /*!< FLASH ECC correction */ 00432 #define FLASH_FLAG_ECCD FLASH_ECCR_ECCD /*!< FLASH ECC detection */ 00433 00434 #define FLASH_FLAG_ALL_ERRORS (FLASH_FLAG_OPERR | FLASH_FLAG_PROGERR | FLASH_FLAG_WRPERR | \ 00435 FLASH_FLAG_PGAERR | FLASH_FLAG_SIZERR | FLASH_FLAG_PGSERR | \ 00436 FLASH_FLAG_MISERR | FLASH_FLAG_FASTERR | FLASH_FLAG_RDERR | \ 00437 FLASH_FLAG_OPTVERR | FLASH_FLAG_ECCD) 00438 /** 00439 * @} 00440 */ 00441 00442 /** @defgroup FLASH_Interrupt_definition FLASH Interrupts Definition 00443 * @brief FLASH Interrupt definition 00444 * @{ 00445 */ 00446 #define FLASH_IT_EOP FLASH_CR_EOPIE /*!< End of FLASH Operation Interrupt source */ 00447 #define FLASH_IT_OPERR FLASH_CR_ERRIE /*!< Error Interrupt source */ 00448 #define FLASH_IT_RDERR FLASH_CR_RDERRIE /*!< PCROP Read Error Interrupt source*/ 00449 #define FLASH_IT_ECCC (FLASH_ECCR_ECCIE >> 24) /*!< ECC Correction Interrupt source */ 00450 /** 00451 * @} 00452 */ 00453 00454 /** 00455 * @} 00456 */ 00457 00458 /* Exported macros -----------------------------------------------------------*/ 00459 /** @defgroup FLASH_Exported_Macros FLASH Exported Macros 00460 * @brief macros to control FLASH features 00461 * @{ 00462 */ 00463 00464 /** 00465 * @brief Set the FLASH Latency. 00466 * @param __LATENCY__: FLASH Latency 00467 * This parameter can be one of the following values : 00468 * @arg FLASH_LATENCY_0: FLASH Zero wait state 00469 * @arg FLASH_LATENCY_1: FLASH One wait state 00470 * @arg FLASH_LATENCY_2: FLASH Two wait states 00471 * @arg FLASH_LATENCY_3: FLASH Three wait states 00472 * @arg FLASH_LATENCY_4: FLASH Four wait states 00473 * @retval None 00474 */ 00475 #define __HAL_FLASH_SET_LATENCY(__LATENCY__) (FLASH->ACR = IS_FLASH_LATENCY(__LATENCY__) ? \ 00476 (FLASH->ACR & (~FLASH_ACR_LATENCY)) | (__LATENCY__) : FLASH->ACR) 00477 00478 /** 00479 * @brief Get the FLASH Latency. 00480 * @retval FLASH Latency 00481 * This parameter can be one of the following values : 00482 * @arg FLASH_LATENCY_0: FLASH Zero wait state 00483 * @arg FLASH_LATENCY_1: FLASH One wait state 00484 * @arg FLASH_LATENCY_2: FLASH Two wait states 00485 * @arg FLASH_LATENCY_3: FLASH Three wait states 00486 * @arg FLASH_LATENCY_4: FLASH Four wait states 00487 */ 00488 #define __HAL_FLASH_GET_LATENCY() READ_BIT(FLASH->ACR, FLASH_ACR_LATENCY) 00489 00490 /** 00491 * @brief Enable the FLASH prefetch buffer. 00492 * @retval None 00493 */ 00494 #define __HAL_FLASH_PREFETCH_BUFFER_ENABLE() SET_BIT(FLASH->ACR, FLASH_ACR_PRFTEN) 00495 00496 /** 00497 * @brief Disable the FLASH prefetch buffer. 00498 * @retval None 00499 */ 00500 #define __HAL_FLASH_PREFETCH_BUFFER_DISABLE() CLEAR_BIT(FLASH->ACR, FLASH_ACR_PRFTEN) 00501 00502 /** 00503 * @brief Enable the FLASH instruction cache. 00504 * @retval none 00505 */ 00506 #define __HAL_FLASH_INSTRUCTION_CACHE_ENABLE() SET_BIT(FLASH->ACR, FLASH_ACR_ICEN) 00507 00508 /** 00509 * @brief Disable the FLASH instruction cache. 00510 * @retval none 00511 */ 00512 #define __HAL_FLASH_INSTRUCTION_CACHE_DISABLE() CLEAR_BIT(FLASH->ACR, FLASH_ACR_ICEN) 00513 00514 /** 00515 * @brief Enable the FLASH data cache. 00516 * @retval none 00517 */ 00518 #define __HAL_FLASH_DATA_CACHE_ENABLE() SET_BIT(FLASH->ACR, FLASH_ACR_DCEN) 00519 00520 /** 00521 * @brief Disable the FLASH data cache. 00522 * @retval none 00523 */ 00524 #define __HAL_FLASH_DATA_CACHE_DISABLE() CLEAR_BIT(FLASH->ACR, FLASH_ACR_DCEN) 00525 00526 /** 00527 * @brief Reset the FLASH instruction Cache. 00528 * @note This function must be used only when the Instruction Cache is disabled. 00529 * @retval None 00530 */ 00531 #define __HAL_FLASH_INSTRUCTION_CACHE_RESET() SET_BIT(FLASH->ACR, FLASH_ACR_ICRST) 00532 00533 /** 00534 * @brief Reset the FLASH data Cache. 00535 * @note This function must be used only when the data Cache is disabled. 00536 * @retval None 00537 */ 00538 #define __HAL_FLASH_DATA_CACHE_RESET() SET_BIT(FLASH->ACR, FLASH_ACR_DCRST) 00539 00540 /** 00541 * @brief Enable the FLASH power down during Low-power run mode. 00542 * @note Writing this bit to 0 this bit, automatically the keys are 00543 * loss and a new unlock sequence is necessary to re-write it to 1. 00544 */ 00545 #define __HAL_FLASH_POWER_DOWN_ENABLE() do { WRITE_REG(FLASH->PDKEYR, FLASH_PDKEY1); \ 00546 WRITE_REG(FLASH->PDKEYR, FLASH_PDKEY2); \ 00547 SET_BIT(FLASH->ACR, FLASH_ACR_RUN_PD); \ 00548 } while (0) 00549 00550 /** 00551 * @brief Disable the FLASH power down during Low-power run mode. 00552 * @note Writing this bit to 0 this bit, automatically the keys are 00553 * loss and a new unlock sequence is necessary to re-write it to 1. 00554 */ 00555 #define __HAL_FLASH_POWER_DOWN_DISABLE() do { WRITE_REG(FLASH->PDKEYR, FLASH_PDKEY1); \ 00556 WRITE_REG(FLASH->PDKEYR, FLASH_PDKEY2); \ 00557 CLEAR_BIT(FLASH->ACR, FLASH_ACR_RUN_PD); \ 00558 } while (0) 00559 00560 /** 00561 * @brief Enable the FLASH power down during Low-Power sleep mode 00562 * @retval none 00563 */ 00564 #define __HAL_FLASH_SLEEP_POWERDOWN_ENABLE() SET_BIT(FLASH->ACR, FLASH_ACR_SLEEP_PD) 00565 00566 /** 00567 * @brief Disable the FLASH power down during Low-Power sleep mode 00568 * @retval none 00569 */ 00570 #define __HAL_FLASH_SLEEP_POWERDOWN_DISABLE() CLEAR_BIT(FLASH->ACR, FLASH_ACR_SLEEP_PD) 00571 00572 /** 00573 * @} 00574 */ 00575 00576 /** @defgroup FLASH_Interrupt FLASH Interrupts Macros 00577 * @brief macros to handle FLASH interrupts 00578 * @{ 00579 */ 00580 00581 /** 00582 * @brief Enable the specified FLASH interrupt. 00583 * @param __INTERRUPT__: FLASH interrupt 00584 * This parameter can be any combination of the following values: 00585 * @arg FLASH_IT_EOP: End of FLASH Operation Interrupt 00586 * @arg FLASH_IT_OPERR: Error Interrupt 00587 * @arg FLASH_IT_RDERR: PCROP Read Error Interrupt 00588 * @arg FLASH_IT_ECCC: ECC Correction Interrupt 00589 * @retval none 00590 */ 00591 #define __HAL_FLASH_ENABLE_IT(__INTERRUPT__) do { if((__INTERRUPT__) & FLASH_IT_ECCC) { SET_BIT(FLASH->ECCR, FLASH_ECCR_ECCIE); }\ 00592 if((__INTERRUPT__) & (~FLASH_IT_ECCC)) { SET_BIT(FLASH->CR, ((__INTERRUPT__) & (~FLASH_IT_ECCC))); }\ 00593 } while(0) 00594 00595 /** 00596 * @brief Disable the specified FLASH interrupt. 00597 * @param __INTERRUPT__: FLASH interrupt 00598 * This parameter can be any combination of the following values: 00599 * @arg FLASH_IT_EOP: End of FLASH Operation Interrupt 00600 * @arg FLASH_IT_OPERR: Error Interrupt 00601 * @arg FLASH_IT_RDERR: PCROP Read Error Interrupt 00602 * @arg FLASH_IT_ECCC: ECC Correction Interrupt 00603 * @retval none 00604 */ 00605 #define __HAL_FLASH_DISABLE_IT(__INTERRUPT__) do { if((__INTERRUPT__) & FLASH_IT_ECCC) { CLEAR_BIT(FLASH->ECCR, FLASH_ECCR_ECCIE); }\ 00606 if((__INTERRUPT__) & (~FLASH_IT_ECCC)) { CLEAR_BIT(FLASH->CR, ((__INTERRUPT__) & (~FLASH_IT_ECCC))); }\ 00607 } while(0) 00608 00609 /** 00610 * @brief Check whether the specified FLASH flag is set or not. 00611 * @param __FLAG__: specifies the FLASH flag to check. 00612 * This parameter can be one of the following values: 00613 * @arg FLASH_FLAG_EOP: FLASH End of Operation flag 00614 * @arg FLASH_FLAG_OPERR: FLASH Operation error flag 00615 * @arg FLASH_FLAG_PROGERR: FLASH Programming error flag 00616 * @arg FLASH_FLAG_WRPERR: FLASH Write protection error flag 00617 * @arg FLASH_FLAG_PGAERR: FLASH Programming alignment error flag 00618 * @arg FLASH_FLAG_SIZERR: FLASH Size error flag 00619 * @arg FLASH_FLAG_PGSERR: FLASH Programming sequence error flag 00620 * @arg FLASH_FLAG_MISERR: FLASH Fast programming data miss error flag 00621 * @arg FLASH_FLAG_FASTERR: FLASH Fast programming error flag 00622 * @arg FLASH_FLAG_RDERR: FLASH PCROP read error flag 00623 * @arg FLASH_FLAG_OPTVERR: FLASH Option validity error flag 00624 * @arg FLASH_FLAG_BSY: FLASH write/erase operations in progress flag 00625 * @arg FLASH_FLAG_ECCC: FLASH one ECC error has been detected and corrected 00626 * @arg FLASH_FLAG_ECCD: FLASH two ECC errors have been detected 00627 * @retval The new state of FLASH_FLAG (SET or RESET). 00628 */ 00629 #define __HAL_FLASH_GET_FLAG(__FLAG__) (((__FLAG__) & (FLASH_FLAG_ECCC | FLASH_FLAG_ECCD)) ? \ 00630 (READ_BIT(FLASH->ECCR, (__FLAG__)) == (__FLAG__)) : \ 00631 (READ_BIT(FLASH->SR, (__FLAG__)) == (__FLAG__))) 00632 00633 /** 00634 * @brief Clear the FLASH's pending flags. 00635 * @param __FLAG__: specifies the FLASH flags to clear. 00636 * This parameter can be any combination of the following values: 00637 * @arg FLASH_FLAG_EOP: FLASH End of Operation flag 00638 * @arg FLASH_FLAG_OPERR: FLASH Operation error flag 00639 * @arg FLASH_FLAG_PROGERR: FLASH Programming error flag 00640 * @arg FLASH_FLAG_WRPERR: FLASH Write protection error flag 00641 * @arg FLASH_FLAG_PGAERR: FLASH Programming alignment error flag 00642 * @arg FLASH_FLAG_SIZERR: FLASH Size error flag 00643 * @arg FLASH_FLAG_PGSERR: FLASH Programming sequence error flag 00644 * @arg FLASH_FLAG_MISERR: FLASH Fast programming data miss error flag 00645 * @arg FLASH_FLAG_FASTERR: FLASH Fast programming error flag 00646 * @arg FLASH_FLAG_RDERR: FLASH PCROP read error flag 00647 * @arg FLASH_FLAG_OPTVERR: FLASH Option validity error flag 00648 * @arg FLASH_FLAG_ECCC: FLASH one ECC error has been detected and corrected 00649 * @arg FLASH_FLAG_ECCD: FLASH two ECC errors have been detected 00650 * @arg FLASH_FLAG_ALL_ERRORS: FLASH All errors flags 00651 * @retval None 00652 */ 00653 #define __HAL_FLASH_CLEAR_FLAG(__FLAG__) do { if((__FLAG__) & (FLASH_FLAG_ECCC | FLASH_FLAG_ECCD)) { SET_BIT(FLASH->ECCR, ((__FLAG__) & (FLASH_FLAG_ECCC | FLASH_FLAG_ECCD))); }\ 00654 if((__FLAG__) & ~(FLASH_FLAG_ECCC | FLASH_FLAG_ECCD)) { WRITE_REG(FLASH->SR, ((__FLAG__) & ~(FLASH_FLAG_ECCC | FLASH_FLAG_ECCD))); }\ 00655 } while(0) 00656 /** 00657 * @} 00658 */ 00659 00660 /* Include FLASH HAL Extended module */ 00661 #include "stm32l4xx_hal_flash_ex.h" 00662 #include "stm32l4xx_hal_flash_ramfunc.h" 00663 00664 /* Exported functions --------------------------------------------------------*/ 00665 /** @addtogroup FLASH_Exported_Functions 00666 * @{ 00667 */ 00668 00669 /* Program operation functions ***********************************************/ 00670 /** @addtogroup FLASH_Exported_Functions_Group1 00671 * @{ 00672 */ 00673 HAL_StatusTypeDef HAL_FLASH_Program(uint32_t TypeProgram, uint32_t Address, uint64_t Data); 00674 HAL_StatusTypeDef HAL_FLASH_Program_IT(uint32_t TypeProgram, uint32_t Address, uint64_t Data); 00675 /* FLASH IRQ handler method */ 00676 void HAL_FLASH_IRQHandler(void); 00677 /* Callbacks in non blocking modes */ 00678 void HAL_FLASH_EndOfOperationCallback(uint32_t ReturnValue); 00679 void HAL_FLASH_OperationErrorCallback(uint32_t ReturnValue); 00680 /** 00681 * @} 00682 */ 00683 00684 /* Peripheral Control functions **********************************************/ 00685 /** @addtogroup FLASH_Exported_Functions_Group2 00686 * @{ 00687 */ 00688 HAL_StatusTypeDef HAL_FLASH_Unlock(void); 00689 HAL_StatusTypeDef HAL_FLASH_Lock(void); 00690 /* Option bytes control */ 00691 HAL_StatusTypeDef HAL_FLASH_OB_Unlock(void); 00692 HAL_StatusTypeDef HAL_FLASH_OB_Lock(void); 00693 HAL_StatusTypeDef HAL_FLASH_OB_Launch(void); 00694 /** 00695 * @} 00696 */ 00697 00698 /* Peripheral State functions ************************************************/ 00699 /** @addtogroup FLASH_Exported_Functions_Group3 00700 * @{ 00701 */ 00702 uint32_t HAL_FLASH_GetError(void); 00703 /** 00704 * @} 00705 */ 00706 00707 /** 00708 * @} 00709 */ 00710 00711 /* Private constants --------------------------------------------------------*/ 00712 /** @defgroup FLASH_Private_Constants FLASH Private Constants 00713 * @{ 00714 */ 00715 #define FLASH_SIZE_DATA_REGISTER ((uint32_t)0x1FFF75E0) 00716 00717 #define FLASH_SIZE ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) == 0xFFFF)) ? (0x400 << 10) : \ 00718 (((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) << 10)) 00719 00720 #define FLASH_BANK_SIZE (FLASH_SIZE >> 1) 00721 00722 #define FLASH_PAGE_SIZE ((uint32_t)0x800) 00723 00724 #define FLASH_TIMEOUT_VALUE ((uint32_t)50000)/* 50 s */ 00725 /** 00726 * @} 00727 */ 00728 00729 /* Private macros ------------------------------------------------------------*/ 00730 /** @defgroup FLASH_Private_Macros FLASH Private Macros 00731 * @{ 00732 */ 00733 00734 #define IS_FLASH_TYPEERASE(VALUE) (((VALUE) == FLASH_TYPEERASE_PAGES) || \ 00735 ((VALUE) == FLASH_TYPEERASE_MASSERASE)) 00736 00737 #define IS_FLASH_BANK(BANK) (((BANK) == FLASH_BANK_1) || \ 00738 ((BANK) == FLASH_BANK_2) || \ 00739 ((BANK) == FLASH_BANK_BOTH)) 00740 00741 #define IS_FLASH_BANK_EXCLUSIVE(BANK) (((BANK) == FLASH_BANK_1) || \ 00742 ((BANK) == FLASH_BANK_2)) 00743 00744 #define IS_FLASH_TYPEPROGRAM(VALUE) (((VALUE) == FLASH_TYPEPROGRAM_DOUBLEWORD) || \ 00745 ((VALUE) == FLASH_TYPEPROGRAM_FAST) || \ 00746 ((VALUE) == FLASH_TYPEPROGRAM_FAST_AND_LAST)) 00747 00748 #define IS_FLASH_MAIN_MEM_ADDRESS(ADDRESS) (((ADDRESS) >= FLASH_BASE) && ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x400) ? \ 00749 ((ADDRESS) <= FLASH_BASE+0xFFFFF) : ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x200) ? \ 00750 ((ADDRESS) <= FLASH_BASE+0x7FFFF) : ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x100) ? \ 00751 ((ADDRESS) <= FLASH_BASE+0x3FFFF) : ((ADDRESS) <= FLASH_BASE+0xFFFFF))))) 00752 00753 #define IS_FLASH_OTP_ADDRESS(ADDRESS) (((ADDRESS) >= 0x1FFF7000) && ((ADDRESS) <= 0x1FFF73FF)) 00754 00755 #define IS_FLASH_PROGRAM_ADDRESS(ADDRESS) (IS_FLASH_MAIN_MEM_ADDRESS(ADDRESS) || IS_FLASH_OTP_ADDRESS(ADDRESS)) 00756 00757 #define IS_FLASH_PAGE(PAGE) (((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x400) ? ((PAGE) < 256) : \ 00758 ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x200) ? ((PAGE) < 128) : \ 00759 ((((*((uint16_t *)FLASH_SIZE_DATA_REGISTER)) & (0x0FFF)) == 0x100) ? ((PAGE) < 64) : \ 00760 ((PAGE) < 256))))) 00761 00762 #define IS_OPTIONBYTE(VALUE) (((VALUE) <= (OPTIONBYTE_WRP | OPTIONBYTE_RDP | OPTIONBYTE_USER | OPTIONBYTE_PCROP))) 00763 00764 #define IS_OB_WRPAREA(VALUE) (((VALUE) == OB_WRPAREA_BANK1_AREAA) || ((VALUE) == OB_WRPAREA_BANK1_AREAB) || \ 00765 ((VALUE) == OB_WRPAREA_BANK2_AREAA) || ((VALUE) == OB_WRPAREA_BANK2_AREAB)) 00766 00767 #define IS_OB_RDP_LEVEL(LEVEL) (((LEVEL) == OB_RDP_LEVEL_0) ||\ 00768 ((LEVEL) == OB_RDP_LEVEL_1)/* ||\ 00769 ((LEVEL) == OB_RDP_LEVEL_2)*/) 00770 00771 #define IS_OB_USER_TYPE(TYPE) (((TYPE) <= (uint32_t)0x1FFF) && ((TYPE) != 0)) 00772 00773 #define IS_OB_USER_BOR_LEVEL(LEVEL) (((LEVEL) == OB_BOR_LEVEL_0) || ((LEVEL) == OB_BOR_LEVEL_1) || \ 00774 ((LEVEL) == OB_BOR_LEVEL_2) || ((LEVEL) == OB_BOR_LEVEL_3) || \ 00775 ((LEVEL) == OB_BOR_LEVEL_4)) 00776 00777 #define IS_OB_USER_STOP(VALUE) (((VALUE) == OB_STOP_RST) || ((VALUE) == OB_STOP_NORST)) 00778 00779 #define IS_OB_USER_STANDBY(VALUE) (((VALUE) == OB_STANDBY_RST) || ((VALUE) == OB_STANDBY_NORST)) 00780 00781 #define IS_OB_USER_SHUTDOWN(VALUE) (((VALUE) == OB_SHUTDOWN_RST) || ((VALUE) == OB_SHUTDOWN_NORST)) 00782 00783 #define IS_OB_USER_IWDG(VALUE) (((VALUE) == OB_IWDG_HW) || ((VALUE) == OB_IWDG_SW)) 00784 00785 #define IS_OB_USER_IWDG_STOP(VALUE) (((VALUE) == OB_IWDG_STOP_FREEZE) || ((VALUE) == OB_IWDG_STOP_RUN)) 00786 00787 #define IS_OB_USER_IWDG_STDBY(VALUE) (((VALUE) == OB_IWDG_STDBY_FREEZE) || ((VALUE) == OB_IWDG_STDBY_RUN)) 00788 00789 #define IS_OB_USER_WWDG(VALUE) (((VALUE) == OB_WWDG_HW) || ((VALUE) == OB_WWDG_SW)) 00790 00791 #define IS_OB_USER_BFB2(VALUE) (((VALUE) == OB_BFB2_DISABLE) || ((VALUE) == OB_BFB2_ENABLE)) 00792 00793 #define IS_OB_USER_DUALBANK(VALUE) (((VALUE) == OB_DUALBANK_SINGLE) || ((VALUE) == OB_DUALBANK_DUAL)) 00794 00795 #define IS_OB_USER_BOOT1(VALUE) (((VALUE) == OB_BOOT1_SRAM) || ((VALUE) == OB_BOOT1_SYSTEM)) 00796 00797 #define IS_OB_USER_SRAM2_PARITY(VALUE) (((VALUE) == OB_SRAM2_PARITY_ENABLE) || ((VALUE) == OB_SRAM2_PARITY_DISABLE)) 00798 00799 #define IS_OB_USER_SRAM2_RST(VALUE) (((VALUE) == OB_SRAM2_RST_ERASE) || ((VALUE) == OB_SRAM2_RST_NOT_ERASE)) 00800 00801 #define IS_OB_PCROP_RDP(VALUE) (((VALUE) == OB_PCROP_RDP_NOT_ERASE) || ((VALUE) == OB_PCROP_RDP_ERASE)) 00802 00803 #define IS_FLASH_LATENCY(LATENCY) (((LATENCY) == FLASH_LATENCY_0) || \ 00804 ((LATENCY) == FLASH_LATENCY_1) || \ 00805 ((LATENCY) == FLASH_LATENCY_2) || \ 00806 ((LATENCY) == FLASH_LATENCY_3) || \ 00807 ((LATENCY) == FLASH_LATENCY_4)) 00808 /** 00809 * @} 00810 */ 00811 00812 /** 00813 * @} 00814 */ 00815 00816 /** 00817 * @} 00818 */ 00819 00820 #ifdef __cplusplus 00821 } 00822 #endif 00823 00824 #endif /* __STM32L4xx_HAL_FLASH_H */ 00825 00826 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00827
Generated on Tue Jul 12 2022 11:35:09 by
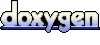