The Ethernet-Board
Dependencies: EthernetInterface HTTPClient MODSERIAL mbed-dev mbed-rtos wolfSSL
Fork of sose2016_tr_oauth_pop by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "HTTPClient.h" 00004 #include "MODSERIAL.h" 00005 #include "base64.h" 00006 00007 #define SEND_BUF_SIZE 4096 00008 00009 DigitalOut R(LED_RED); 00010 DigitalOut G(LED_GREEN); 00011 DigitalOut B(LED_BLUE); 00012 00013 MODSERIAL serial(USBTX, USBRX,4096); // tx, rx 00014 EthernetInterface eth; 00015 HTTPClient http; 00016 00017 SPISlave device(D11, D12, D13, D10); 00018 00019 char str2[32000]; 00020 00021 char * token; 00022 00023 int length_countdown = 4; 00024 00025 char length_bytes[4]; 00026 int length = 0; 00027 int i = 0; 00028 00029 bool onRead(char ch) 00030 { 00031 if (ch == 0x11) NVIC_SystemReset(); // Reset 00032 00033 // If length didn't get read yet... 00034 if (length_countdown > 0){ 00035 length_countdown--; 00036 length_bytes[3-length_countdown] = ch; 00037 00038 // if last lengthbyte got read... 00039 if (length_countdown == 0){ 00040 00041 //Set length and malloc the token. 00042 length = length_bytes[0] + (length_bytes[1] << 8) + (length_bytes[2] << 16) + (length_bytes[3] << 24); 00043 i = length; 00044 token = (char*) malloc(sizeof(char)*length+1); 00045 } 00046 return false; 00047 } 00048 00049 //Read token 00050 memset(token+(length-i),ch,1); 00051 i--; 00052 00053 //If token got read... 00054 if (i == 0 and length_countdown == 0){ 00055 //Set 0x00 byte to the end and return true. 00056 memset(token+length,0x00,1); 00057 length_countdown = 4; 00058 return true; 00059 } 00060 return false; 00061 } 00062 00063 void encodeToken(){ 00064 size_t len; 00065 char * ptr = strtok(token,"|"); 00066 00067 char * t1 = base64_encode((const unsigned char*)ptr,strlen(ptr),&len); 00068 ptr = strtok(NULL,"|"); 00069 char * t2 = base64_encode((const unsigned char*)ptr,strlen(ptr),&len); 00070 ptr = strtok(NULL,"|"); 00071 00072 free(token); 00073 token = (char *) malloc(strlen(t1)+strlen(t2)+strlen(ptr)+3); 00074 sprintf(token,"%s.%s.%s",t1,t2,ptr); 00075 00076 free(t1); 00077 free(t2); 00078 free(ptr); 00079 base64_cleanup(); 00080 } 00081 00082 int main() { 00083 setbuf(stdout, NULL); 00084 eth.init(); 00085 eth.connect(); 00086 R=!R;G=!G;B=!B; 00087 wait(1); 00088 00089 serial.printf("Start"); 00090 00091 //Init SPI 00092 device.format(8,3); 00093 device.frequency(2000000); 00094 00095 00096 00097 while(1){ 00098 00099 // Recieve Token 00100 while(1){ 00101 if(device.receive()) { 00102 if (onRead(device.read())) break; 00103 } 00104 } 00105 00106 //serial.printf("%s\r\n\r\n",token); 00107 00108 // Encode the first two parts of the message to get the valid token 00109 encodeToken(); 00110 00111 // Light Blue 00112 B=!B; 00113 00114 // Token Read, light Yellow 00115 R=!R;G=!G;B=!B; 00116 00117 // Send token to Server 00118 HTTPMap map; 00119 HTTPText inText(str2, 32000); 00120 map.put("client_id", "a1b2c3"); 00121 map.put("client_secret", "drickyoughurt"); 00122 map.put("token_type_hint", "pop_token"); 00123 map.put("token", token); 00124 00125 int ret = http.post("https://kongsugar.de/introspection", map, &inText); 00126 00127 free(token); 00128 00129 if (!ret) 00130 { 00131 // Unlock, Light Green 5s 00132 R=!R;wait(5);G=!G; 00133 } 00134 else 00135 { 00136 // Light Red 5s 00137 G=!G;wait(5);R=!R; 00138 } 00139 00140 // Bugfix, nervous user send token multiple times! 00141 memset(&str2[0], 0, sizeof(str2)); 00142 serial.rxBufferFlush(); 00143 } 00144 }
Generated on Wed Jul 13 2022 07:41:08 by
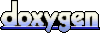