
use serial connected to PC communicate with cloud, and send command to actor and receive date from sensor
Dependencies: MbedJSONValue mbed nRF24L01
main.cpp
00001 #include "mbed.h" 00002 #include "MbedJSONValue.h" 00003 #include <string> 00004 //------------------------------------ 00005 // Hyperterminal configuration 00006 // 9600 bauds, 8-bit data, no parity 00007 //------------------------------------ 00008 00009 void Jserialize(void); 00010 void Jparse(void); 00011 00012 Serial pc(SERIAL_TX, SERIAL_RX); 00013 00014 DigitalOut myled(LED1); 00015 00016 int main() 00017 { 00018 int i = 1; 00019 Jserialize(); 00020 Jserialize(); 00021 while(1) { 00022 wait(1); 00023 pc.printf("This program runs since %d seconds.\r\n", i++); 00024 myled = !myled; 00025 } 00026 } 00027 00028 void Jserialize(void) 00029 { 00030 00031 MbedJSONValue demo; 00032 std::string s; 00033 00034 //fill the object 00035 demo["my_array"][0] = "demo_string"; 00036 demo["my_array"][1] = 10; 00037 demo["my_boolean"] = false; 00038 00039 //serialize it into a JSON string 00040 s = demo.serialize(); 00041 printf("json: %s\r\n", s.c_str()); 00042 } 00043 00044 void Jparse(void) 00045 { 00046 MbedJSONValue demo; 00047 00048 const char * json = "{\"my_array\": [\"demo_string\", 10], \"my_boolean\": true}"; 00049 00050 //parse the previous string and fill the object demo 00051 parse(demo, json); 00052 00053 std::string my_str; 00054 int my_int; 00055 bool my_bool; 00056 00057 my_str = demo["my_array"][0].get<std::string>(); 00058 my_int = demo["my_array"][1].get<int>(); 00059 my_bool = demo["my_boolean"].get<bool>(); 00060 00061 printf("my_str: %s\r\n", my_str.c_str()); 00062 printf("my_int: %d\r\n", my_int); 00063 printf("my_bool: %s\r\n", my_bool ? "true" : "false"); 00064 }
Generated on Wed Jul 13 2022 12:09:01 by
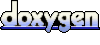