Library for interfacing to Sparkfun Weather Meters.
Dependents: WeatherStation Deneme testgeneral ... more
WeatherMeters.h
00001 /* Copyright 2012 Adam Green (http://mbed.org/users/AdamGreen/) 00002 00003 Licensed under the Apache License, Version 2.0 (the "License"); 00004 you may not use this file except in compliance with the License. 00005 You may obtain a copy of the License at 00006 00007 http://www.apache.org/licenses/LICENSE-2.0 00008 00009 Unless required by applicable law or agreed to in writing, software 00010 distributed under the License is distributed on an "AS IS" BASIS, 00011 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 See the License for the specific language governing permissions and 00013 limitations under the License. 00014 */ 00015 /* Header file for class to interface with Sparkfun Weather Meters: 00016 http://www.sparkfun.com/products/8942 00017 */ 00018 #ifndef _WEATHER_METERS_H_ 00019 #define _WEATHER_METERS_H_ 00020 00021 #include "WindVane.h" 00022 #include "RainGauge.h" 00023 #include "Anemometer.h" 00024 00025 00026 namespace AFP 00027 { 00028 00029 /** A class to interface with the Sparkfun Weather Meters. 00030 http://www.sparkfun.com/products/8942 00031 00032 This set of weather meters from Sparkfun includes anemometer (wind speed), 00033 wind vane, and rain gauge. All of the sensors use reed switches so that 00034 the electronics can detect motion/location in the sensor hardware via 00035 magnets placed in the sensors without actually exposing the electonic 00036 switches themselves to the elements. A reed switch closes as the 00037 anemometer spins and the rain gauge empties. The wind vane includes 00038 8 reed switches, each with a different valued resistor attached so that 00039 the voltage will vary depending on where the magnet in the vane is 00040 located. 00041 * Example: 00042 * @code 00043 #include <mbed.h> 00044 #include "WeatherMeters.h" 00045 00046 int main() 00047 { 00048 CWeatherMeters WeatherMeters(p9, p10, p15); 00049 for (;;) 00050 { 00051 CWeatherMeters::SMeasurements Measurements; 00052 00053 WeatherMeters.GetMeasurements(&Measurements); 00054 00055 printf("Direction: %s\n", Measurements.WindDirectionString); 00056 printf("Depth: %f\n",Measurements.Rainfall); 00057 printf("Current speed: %f\n", Measurements.WindSpeed); 00058 printf("Maximum speed: %f\n", Measurements.MaximumWindSpeed); 00059 00060 wait(1.0f); 00061 } 00062 } 00063 * @endcode 00064 */ 00065 class CWeatherMeters 00066 { 00067 protected: 00068 CAnemometer m_Anemometer; 00069 CRainGauge m_RainGauge; 00070 CWindVane m_WindVane; 00071 00072 public: 00073 /** A structure in which the current measurements registered by the weather 00074 meters are returned from the GetMeasurements() method. 00075 */ 00076 struct SMeasurements 00077 { 00078 const char* WindDirectionString; /**< String representing current wind direction. Example: "North" */ 00079 float WindDirectionAngle; /**< Wind direction in degrees from north. */ 00080 float WindSpeed; /**< Current wind speed in km/hour. */ 00081 float MaximumWindSpeed; /**< Maximum wind speed measured since last reset. Measured in km/hour. */ 00082 float Rainfall; /**< Amount of rainfall since last reset. Measured in mm. */ 00083 }; 00084 00085 /** Create a CWeatherMeters object, binds it to the specified input pins, 00086 and initializes the required interrupt handlers. 00087 00088 @param AnemometerPin The mbed pin which is connected to the anemometer 00089 sensor. Must be a pin on which the mbed supports InterruptIn. 00090 @param RainGauagePin The mbed pin which is connected to the rain gauge 00091 sensor. Must be a pin on which the mbed supports InterruptIn. 00092 @param WindVanePin The mbed pin which is connected to the wind vane 00093 sensor. Must be a pin on which the mbed supports AnalogIn. 00094 @param WindVaneSeriesResistance The value of the resistance which is 00095 in series with the wind vane sensor and along with the 00096 varying resistance of the wind vane sensor itself, acts as a 00097 voltage divider. 00098 */ 00099 CWeatherMeters(PinName AnemometerPin, 00100 PinName RainGaugePin, 00101 PinName WindVanePin, 00102 float WindVaneSeriesResistance = 10000.0f); 00103 00104 /** Gets current weather meter measurements. 00105 00106 NOTE: Should call this method on a regular basis (every 15 minutes or 00107 less) to keep internal timers from overflowing. 00108 00109 @param pMeasurements points to the measurement structure to be filled in 00110 with the current measurement from the sensors. The historical 00111 values in this structure such as rainfall and maximum wind 00112 speed can be reset by calls to ResetRainfall(), 00113 ResetMaximumWindSpeed(), and Reset(). 00114 */ 00115 void GetMeasurements(SMeasurements* pMeasurements); 00116 00117 /** Resets all historical measurements: rainfall and maximum wind speed. */ 00118 void Reset(); 00119 00120 /** Resets the cumulative rainfall measurement. */ 00121 void ResetRainfall(); 00122 00123 /** Resets the maximum wind speed measurement. */ 00124 void ResetMaximumWindSpeed(); 00125 }; 00126 00127 } // namespace AFP 00128 using namespace AFP; 00129 00130 #endif // _WEATHER_METERS_H_
Generated on Fri Jul 15 2022 00:08:29 by
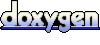