This library can be used in mbed driver or mbed OS2. So If you want to use WizFi310 on mbed OS5, You have to use another WizFi310 library(wizfi310-driver). That is git repository for wizfi310-driver. - https://github.com/ARMmbed/wizfi310-driver
Dependents: KT_IoTMakers_WizFi310_Example WizFi310_STATION_HelloWorld WizFi310_DNS_TCP_HelloWorld WizFi310_Ubidots ... more
WizFi310_msg.cpp
00001 /* 00002 /* Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi310 00021 */ 00022 00023 #include "WizFi310.h" 00024 00025 #ifdef CFG_ENABLE_RTOS 00026 #undef WIZ_DBG 00027 #define WIZ_DBG(x, ...) 00028 #endif 00029 00030 #define RESP_MSG_OK "[OK]" 00031 #define RESP_MSG_ERROR "[ERROR" 00032 #define RESP_MSG_CONNECT "[CONNECT " 00033 #define RESP_MSG_DISCONNECT "[DISCONNECT " 00034 #define RESP_MSG_LISTEN "[LISTEN " 00035 #define RESP_MSG_MQTTCON "[MQTT CONNECT]" 00036 #define RESP_MSG_MQTTDISCON "[MQTT DISCONNECT]" 00037 00038 00039 // This function is operating in ISR. So you can't use debug message. 00040 void WizFi310::recvData ( char c ) 00041 { 00042 static int cid, sub, len, count; 00043 static int is_mqtt_data = 0; 00044 00045 switch(_state.mode) 00046 { 00047 case MODE_COMMAND: 00048 switch(c) 00049 { 00050 case 0: 00051 case 0x0a: // LF 00052 case 0x0d: // CR 00053 break; 00054 00055 case '{': 00056 _state.buf->flush(); 00057 _state.mode = MODE_DATA_RX; 00058 sub = 0; 00059 break; 00060 00061 default: 00062 _state.buf->flush(); 00063 _state.buf->queue(c); 00064 _state.mode = MODE_CMDRESP; 00065 break; 00066 } 00067 break; 00068 00069 case MODE_CMDRESP: 00070 switch(c) 00071 { 00072 case 0: 00073 break; 00074 case 0x0a: // LF 00075 break; 00076 case 0x0d: // CR 00077 if (_flow == 2) setRts(false); // block 00078 _state.mode = MODE_COMMAND; 00079 parseMessage(); 00080 if (_flow == 2) setRts(true); // release 00081 break; 00082 default: 00083 _state.buf->queue(c); 00084 break; 00085 } 00086 break; 00087 00088 case MODE_DATA_RX: 00089 00090 switch(sub) 00091 { 00092 case 0: 00093 // cid 00094 if( (c >= '0') && (c <= '9') ) 00095 { 00096 cid = x2i(c); 00097 } 00098 else if ( c == ',' ) 00099 { 00100 sub++; 00101 count = 0; 00102 len = 0; 00103 } 00104 //daniel add for mqtt 00105 else if ( c == 'Q' ) 00106 { 00107 cid = 0; 00108 is_mqtt_data = 1; 00109 } 00110 // 00111 else 00112 { 00113 _state.mode = MODE_COMMAND; 00114 } 00115 break; 00116 00117 case 1: 00118 // ip 00119 // if ((c >= '0' && c <= '9') || c == '.') 00120 if (((c >= '0' && c <= '9') || c == '.') && is_mqtt_data == 0 ) 00121 { 00122 _con[cid].ip[count] = c; 00123 count++; 00124 } 00125 else if( c == ',' ) 00126 { 00127 _con[cid].ip[count] = '\0'; 00128 _con[cid].port = 0; 00129 sub++; 00130 } 00131 //daniel for mqtt 00132 else if( is_mqtt_data == 1) 00133 { 00134 rcvd_mqtt_topic[count] = c; 00135 count++; 00136 } 00137 // else 00138 else if( is_mqtt_data == 0 ) 00139 { 00140 _state.mode = MODE_COMMAND; 00141 } 00142 break; 00143 00144 case 2: 00145 // port 00146 if ( c >= '0' && c <= '9' ) 00147 { 00148 _con[cid].port = (_con[cid].port * 10) + ( c - '0' ); 00149 } 00150 else if( c == ',') 00151 { 00152 sub++; 00153 count = 0; 00154 } 00155 else 00156 { 00157 _state.mode = MODE_COMMAND; 00158 } 00159 break; 00160 00161 case 3: 00162 // data length 00163 if ( c >= '0' && c <= '9' ) 00164 { 00165 //_con[cid].recv_length = (_con[cid].recv_length * 10) + (c - '0'); 00166 len = (len * 10) + (c - '0'); 00167 } 00168 else if( c == '}' ) 00169 { 00170 sub++; 00171 count = 0; 00172 _con[cid].recv_length = len; 00173 } 00174 else 00175 { 00176 _state.mode = MODE_COMMAND; 00177 } 00178 break; 00179 00180 default: 00181 00182 if(_con[cid].buf != NULL) 00183 { 00184 _con[cid].buf->queue(c); 00185 if(_con[cid].buf->available() > CFG_DATA_SIZE - 16 ) 00186 { 00187 setRts(false); // blcok 00188 _con[cid].received = true; 00189 WIZ_WARN("buf full"); 00190 } 00191 } 00192 _con[cid].recv_length--; 00193 if(_con[cid].recv_length == 0) 00194 { 00195 _con[cid].received = true; 00196 _state.mode = MODE_COMMAND; 00197 } 00198 break; 00199 } 00200 break; 00201 } 00202 } 00203 00204 #define MSG_TABLE_NUM 7 00205 #define RES_TABLE_NUM 7 00206 int WizFi310::parseMessage () { 00207 int i; 00208 char buf[128]; 00209 00210 static const struct MSG_TABLE { 00211 const char msg[24]; 00212 void (WizFi310::*func)(const char *); 00213 } msg_table[MSG_TABLE_NUM] = { 00214 {RESP_MSG_OK, &WizFi310::msgOk}, 00215 {RESP_MSG_ERROR, &WizFi310::msgError}, 00216 {RESP_MSG_CONNECT, &WizFi310::msgConnect}, 00217 {RESP_MSG_DISCONNECT, &WizFi310::msgDisconnect}, 00218 {RESP_MSG_LISTEN, &WizFi310::msgListen}, 00219 {RESP_MSG_MQTTCON, &WizFi310::msgMQTTConnect}, 00220 {RESP_MSG_MQTTDISCON, &WizFi310::msgMQTTDisconnect}, 00221 }; 00222 static const struct RES_TABLE{ 00223 const Response res; 00224 void (WizFi310::*func)(const char *); 00225 }res_table[RES_TABLE_NUM]={ 00226 {RES_NULL, NULL}, 00227 {RES_MACADDRESS, &WizFi310::resMacAddress}, 00228 // {RES_WJOIN, &WizFi310::resWJOIN}, 00229 {RES_CONNECT, &WizFi310::resConnect}, 00230 {RES_SSEND, &WizFi310::resSSEND}, 00231 {RES_FDNS, &WizFi310::resFDNS}, 00232 {RES_SMGMT, &WizFi310::resSMGMT}, 00233 {RES_WSTATUS, &WizFi310::resWSTATUS}, 00234 }; 00235 00236 00237 for( i=0; i<sizeof(buf); i++ ) 00238 { 00239 if( _state.buf->dequeue(&buf[i]) == false ) break; 00240 } 00241 00242 buf[i] = '\0'; 00243 00244 if(_state.res != RES_NULL) 00245 { 00246 for( i=0; i<RES_TABLE_NUM; i++) 00247 { 00248 if(res_table[i].res == _state.res) 00249 { 00250 if(res_table[i].func != NULL) 00251 { 00252 (this->*(res_table[i].func))(buf); 00253 } 00254 00255 if(res_table[i].res == RES_CONNECT && _state.n < 2) 00256 return -1; 00257 } 00258 } 00259 } 00260 00261 for( i=0; i<MSG_TABLE_NUM; i++) 00262 { 00263 if( strncmp(buf, msg_table[i].msg, strlen(msg_table[i].msg)) == 0 ) 00264 { 00265 if(msg_table[i].func != NULL) 00266 { 00267 (this->*(msg_table[i].func))(buf); 00268 } 00269 return 0; 00270 } 00271 } 00272 00273 return -1; 00274 } 00275 00276 00277 void WizFi310::msgOk (const char *buf) 00278 { 00279 _state.ok = true; 00280 } 00281 00282 void WizFi310::msgError (const char *buf) 00283 { 00284 _state.failure = true; 00285 } 00286 00287 void WizFi310::msgConnect (const char *buf) 00288 { 00289 int cid; 00290 00291 //if (buf[9] < '0' || buf[9] > '8' || buf[10] != ']') return; 00292 if( isdigit(buf[9]) == 0 ) return; 00293 00294 cid = x2i(buf[9]); 00295 00296 initCon(cid, true); 00297 _state.cid = cid; 00298 _con[cid].accept = true; 00299 _con[cid].parent = cid; 00300 } 00301 00302 void WizFi310::msgDisconnect (const char *buf) 00303 { 00304 int cid; 00305 00306 //if(buf[12] < '0' || buf[12] > '8' || buf[13] != ']') return; 00307 if( isdigit(buf[12]) == 0 ) return; 00308 00309 cid = x2i(buf[12]); 00310 _con[cid].connected = false; 00311 } 00312 00313 00314 void WizFi310::msgMQTTConnect (const char *buf) 00315 { 00316 int cid = 0; 00317 00318 initCon(cid, true); 00319 _state.cid = cid; 00320 _con[cid].accept = true; 00321 _con[cid].parent = cid; 00322 00323 _con[cid].connected = true; 00324 _state.res = RES_NULL; 00325 _state.ok = true; 00326 } 00327 00328 void WizFi310::msgMQTTDisconnect (const char *buf) 00329 { 00330 int cid = 0; 00331 _con[cid].connected = false; 00332 } 00333 00334 00335 void WizFi310::msgListen (const char *buf) 00336 { 00337 int cid; 00338 00339 //if(buf[8] < '0' || buf[8] > '8' || buf[9] != ']') return; 00340 if( isdigit(buf[8]) == 0 ) return; 00341 00342 cid = x2i(buf[8]); 00343 _state.cid = cid; 00344 } 00345 00346 void WizFi310::resMacAddress (const char *buf) 00347 { 00348 if( buf[2] == ':' && buf[5] == ':') 00349 { 00350 strncpy(_state.mac, buf, sizeof(_state.mac)); 00351 _state.mac[17] = 0; 00352 _state.res = RES_NULL; 00353 00354 if(strncmp(_state.mac,CFG_DEFAULT_MAC,sizeof(CFG_DEFAULT_MAC)) == 0){ 00355 _state.ok = false; 00356 } 00357 _state.ok = true; 00358 } 00359 } 00360 00361 void WizFi310::resConnect (const char *buf) 00362 { 00363 int cid; 00364 00365 if( strstr((char*)buf, RESP_MSG_OK) != NULL){ 00366 _state.n++; 00367 } 00368 else if( strstr((char*)buf, RESP_MSG_CONNECT) != NULL){ 00369 cid = x2i(buf[9]); 00370 _state.cid = cid; 00371 _state.n++; 00372 } 00373 00374 if(_state.n >= 2) 00375 { 00376 _state.res = RES_NULL; 00377 _state.ok = true; 00378 } 00379 } 00380 00381 void WizFi310::resSSEND (const char *buf) 00382 { 00383 if(_state.cid != -1) 00384 { 00385 _state.res = RES_NULL; 00386 _state.ok = true; 00387 } 00388 } 00389 00390 void WizFi310::resFDNS (const char *buf) 00391 { 00392 int i; 00393 00394 for(i=0; i<strlen(buf); i++) 00395 { 00396 if( (buf[i] < '0' || buf[i] > '9') && buf[i] != '.' ) 00397 { 00398 return; 00399 } 00400 } 00401 00402 strncpy(_state.resolv, buf, sizeof(_state.resolv)); 00403 _state.res = RES_NULL; 00404 } 00405 00406 void WizFi310::resSMGMT (const char *buf) 00407 { 00408 int cid, i; 00409 char *c; 00410 00411 // if( (buf[0] < '0' || buf[0] > '8') ) return; 00412 if( isdigit (buf[0])) return; 00413 00414 cid = x2i(buf[0]); 00415 if( cid != _state.cid ) return; 00416 00417 // IP 00418 c = (char*)(buf+6); 00419 for( i=0; i<16; i++ ) 00420 { 00421 if( *(c+i) == ':') 00422 { 00423 _con[cid].ip[i] = '\0'; 00424 i++; 00425 break; 00426 } 00427 if( ( *(c+i) < '0' || *(c+i) > '9') && *(c+i) != '.' ) return; 00428 _con[cid].ip[i] = *(c+i); 00429 } 00430 00431 // Port 00432 c = (c+i); 00433 _con[cid].port = 0; 00434 for( i=0; i<5; i++ ) 00435 { 00436 if( *(c+i) == '/') break; 00437 if( *(c+i) < '0' || *(c+i) > '9' ) return; 00438 00439 _con[cid].port = (_con[cid].port * 10) + ( *(c+i) - '0' ); 00440 } 00441 00442 _state.res = RES_NULL; 00443 } 00444 00445 void WizFi310::resWSTATUS (const char *buf) 00446 { 00447 char* idx_ptr; 00448 00449 if(_state.n == 0) 00450 { 00451 _state.n++; 00452 } 00453 else if(_state.n == 1) 00454 { 00455 idx_ptr = strtok((char*)buf, "/"); // Interface STA or AP 00456 idx_ptr = strtok( NULL, "/"); // SSID 00457 idx_ptr = strtok( NULL, "/"); // IP Addr 00458 memset(_state.ip, 0, sizeof(_state.ip)); 00459 memcpy(_state.ip, idx_ptr, strlen(idx_ptr)+1); 00460 00461 idx_ptr = strtok( NULL, "/"); // Gateway Addr 00462 memset(_state.gateway, 0, sizeof(_state.gateway)); 00463 memcpy(_state.gateway, idx_ptr, strlen(idx_ptr)+1); 00464 00465 _state.res = RES_NULL; 00466 } 00467 00468 }
Generated on Wed Jul 13 2022 18:48:26 by
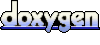