
Gainspan module evaluation with WIZwiki-W7500 using SerialPassthrough.
Dependencies: mbed
Fork of SerialPassthrough_GainspanModule_W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "CBuffer.h" 00003 00004 DigitalOut led1(LED1); 00005 DigitalOut led4(LED4); 00006 00007 RawSerial pc(USBTX, USBRX); // tx, rx 00008 RawSerial dev(D1, D0); // tx, rx for WIZwiki-W75000 00009 00010 #define ASYNC_DEBUG 0 00011 00012 #if ASYNC_DEBUG 00013 //////////////////////////////////////////////////////////////////////////////////////////////// 00014 // mbed Async Debug 00015 Timeout timer_buffer_debug; 00016 CircBuffer<char> async_debugbufer(1024); 00017 00018 void print_debugbuffer() 00019 { 00020 char c=0; 00021 while ( async_debugbufer.available() ) { 00022 async_debugbufer.dequeue(&c); 00023 pc.putc(c); 00024 } 00025 timer_buffer_debug.attach(&print_debugbuffer, 0.1); 00026 } 00027 00028 #include <stdarg.h> 00029 static char debug_line[64]; 00030 void mbed_async_debug(const char *format, ...) 00031 { 00032 va_list args; 00033 00034 va_start(args, format); 00035 00036 vsnprintf(debug_line, sizeof(debug_line), format, args); 00037 int length = strlen(debug_line); 00038 00039 for (int i=0; i<length; i++) 00040 async_debugbufer.queue(debug_line[i]); 00041 00042 va_end(args); 00043 } 00044 00045 // mbed Async Debug Print, You can move below line to mbed-src. 00046 void (*dbg_f)(const char *format, ...); 00047 extern void (*dbg_f)(const char *format, ...); 00048 00049 #endif 00050 00051 void dev_recv() 00052 { 00053 led1 = !led1; 00054 while(dev.readable()) { 00055 pc.putc(dev.getc()); 00056 } 00057 } 00058 00059 void pc_recv() 00060 { 00061 led4 = !led4; 00062 while(pc.readable()) { 00063 dev.putc(pc.getc()); 00064 } 00065 } 00066 00067 int main() 00068 { 00069 for (int i=0; i<10; i++) 00070 { 00071 led1 = !led1; 00072 led4 = !led4; 00073 wait(0.1); 00074 } 00075 00076 pc.baud(115200); 00077 dev.baud(9600); 00078 00079 pc.printf("Serial Passthrough Started. \r\n"); 00080 00081 #if ASYNC_DEBUG 00082 dbg_f = &mbed_async_debug; 00083 timer_buffer_debug.attach(&print_debugbuffer, 0.1); 00084 #endif 00085 00086 pc.attach(&pc_recv, Serial::RxIrq); 00087 dev.attach(&dev_recv, Serial::RxIrq); 00088 00089 while(1) { 00090 wait(1); 00091 } 00092 }
Generated on Fri Jul 22 2022 23:56:12 by
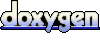