Thinger.io Client Library for ARM mbed platform. This is a generic library that provides a base class that can be used to other develop hardware specific libraries.
Fork of ThingerClient by
thinger_message.hpp
00001 // The MIT License (MIT) 00002 // 00003 // Copyright (c) 2015 THINGER LTD 00004 // Author: alvarolb@gmail.com (Alvaro Luis Bustamante) 00005 // 00006 // Permission is hereby granted, free of charge, to any person obtaining a copy 00007 // of this software and associated documentation files (the "Software"), to deal 00008 // in the Software without restriction, including without limitation the rights 00009 // to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 // copies of the Software, and to permit persons to whom the Software is 00011 // furnished to do so, subject to the following conditions: 00012 // 00013 // The above copyright notice and this permission notice shall be included in 00014 // all copies or substantial portions of the Software. 00015 // 00016 // THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 // IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 // FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 // AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 // LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 // OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 // THE SOFTWARE. 00023 00024 #ifndef THINGER_MESSAGE_HPP 00025 #define THINGER_MESSAGE_HPP 00026 00027 #include "pson.h" 00028 00029 namespace thinger{ 00030 00031 enum message_type{ 00032 MESSAGE = 1, 00033 KEEP_ALIVE = 2 00034 }; 00035 00036 class thinger_message{ 00037 00038 public: 00039 00040 // fields for a thinger message (encoded as in protocol buffers) 00041 enum fields{ 00042 STREAM_ID = 1, 00043 SIGNAL_FLAG = 2, 00044 UNUSED = 3, 00045 RESOURCE = 4, 00046 UNUSED2 = 5, 00047 PSON_PAYLOAD = 6 00048 }; 00049 00050 // flags for describing a thinger message 00051 enum signal_flag { 00052 // GENERAL USED FLAGS 00053 REQUEST_OK = 1, // the request with the given stream id was successful 00054 REQUEST_ERROR = 2, // the request with the given stream id failed 00055 00056 // SENT BY THE SERVER 00057 NONE = 0, // default resource action: just execute the given resource 00058 START_STREAM = 3, // enable a streaming resource (with stream_id, and resource filled, sample interval (in payload) is optional) 00059 STOP_STREAM = 4, // stop the streaming resource (with stream_id, and resource filled) 00060 00061 // SENT BY DEVICE 00062 AUTH = 5, 00063 STREAM_EVENT = 6, // means that the message data is related to a stream event 00064 STREAM_SAMPLE = 7, // means that the message is related to a periodical streaming sample 00065 CALL_ENDPOINT = 8 // call the endpoint with the provided name (endpoint in resource, value passed in payload) 00066 }; 00067 00068 public: 00069 00070 /** 00071 * Initialize a default response message setting the same stream id of the source message, 00072 * and initializing the signal flag to ok. All remaining data or fields are empty 00073 */ 00074 thinger_message(thinger_message& other) : 00075 stream_id(other.stream_id), 00076 flag(REQUEST_OK), 00077 resource(NULL), 00078 data(NULL), 00079 data_allocated(false) 00080 {} 00081 00082 /** 00083 * Initialize a default empty message 00084 */ 00085 thinger_message() : 00086 stream_id(0), 00087 flag(NONE), 00088 resource(NULL), 00089 data(NULL), 00090 data_allocated(false) 00091 {} 00092 00093 ~thinger_message(){ 00094 // deallocate resource 00095 destroy(resource, protoson::pool); 00096 // deallocate paylaod if was allocated here 00097 if(data_allocated){ 00098 destroy(data, protoson::pool); 00099 } 00100 } 00101 00102 private: 00103 /// used for identifying a unique stream 00104 uint16_t stream_id; 00105 /// used for setting a stream signal 00106 signal_flag flag; 00107 /// used to identify a device resource 00108 protoson::pson* resource; 00109 /// used to fill a data payload in the message 00110 protoson::pson* data; 00111 /// flag to determine when the payload has been reserved 00112 bool data_allocated; 00113 00114 public: 00115 00116 uint16_t get_stream_id(){ 00117 return stream_id; 00118 } 00119 00120 signal_flag get_signal_flag(){ 00121 return flag; 00122 } 00123 00124 bool has_data(){ 00125 return data!=NULL; 00126 } 00127 00128 bool has_resource(){ 00129 return resource!=NULL; 00130 } 00131 00132 public: 00133 void set_stream_id(uint16_t stream_id) { 00134 thinger_message::stream_id = stream_id; 00135 } 00136 00137 void set_signal_flag(signal_flag const &flag) { 00138 thinger_message::flag = flag; 00139 } 00140 00141 public: 00142 00143 void operator=(const char* str){ 00144 ((protoson::pson &) * this) = str; 00145 } 00146 00147 operator protoson::pson&(){ 00148 if(data==NULL){ 00149 data = new (protoson::pool) protoson::pson; 00150 data_allocated = true; 00151 } 00152 return *data; 00153 } 00154 00155 protoson::pson_array& resources(){ 00156 return (protoson::pson_array&)get_resources(); 00157 } 00158 00159 protoson::pson& get_resources(){ 00160 if(resource==NULL){ 00161 resource = new (protoson::pool) protoson::pson; 00162 } 00163 return *resource; 00164 } 00165 00166 protoson::pson& get_data(){ 00167 return *this; 00168 } 00169 00170 void set_data(protoson::pson& pson_data){ 00171 if(data==NULL){ 00172 data = &pson_data; 00173 data_allocated = false; 00174 } 00175 } 00176 00177 }; 00178 } 00179 00180 #endif
Generated on Wed Jul 13 2022 01:45:11 by
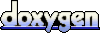