d
Dependencies: AX12_final MX106_not_working comunication_1 mbed-dev
Fork of MX106-finaltest by
MX.h
00001 #ifndef MX_H 00002 #define MX_H 00003 00004 #define MX_REG_ID 0x3 00005 #define MX_REG_CW_LIMIT 0x06 00006 #define MX_REG_CCW_LIMIT 0x08 00007 #define MX_REG_GOAL_POSITION 0x1E 00008 #define MX_REG_MOVING_SPEED 0x20 00009 #define MX_REG_VOLTS 0x2A 00010 #define MX_REG_AMPERE 0x44 00011 #define MX_REG_TEMP 0x2B 00012 #define MX_REG_PGAIN 0x1C 00013 #define MX_REG_IGAIN 0x1B 00014 #define MX_REG_DGAIN 0X1A 00015 #define MX_REG_MOVING 0x2E 00016 #define MX_REG_MAXTORQUE 0x22 00017 #define MX_REG_POSITION 0x24 00018 #define MX_RESOLUTION 0.088 00019 //bit per degrees 4095/360° 00020 #define MX_REG_MOTORONOFF 0x18 00021 #define MX_BIT_DEG 11.375 00022 #define MX_DEBUG 1 00023 #define MX_TRIGGER_DEBUG 1 00024 #define MX_REG_CURRENT 0x44 00025 #define MX_REG_TEMP 0x2B 00026 00027 #define MX_READ_DEBUG 1 00028 #define AX12_WRITE_DEBUG 1 00029 #define AX12_READ_DEBUG 1 00030 #define AX12_TRIGGER_DEBUG 1 00031 #define AX12_DEBUG 1 00032 00033 #define AX12_REG_ID 0x3 00034 #define AX12_REG_CW_LIMIT 0x06 00035 #define AX12_REG_CCW_LIMIT 0x08 00036 #define AX12_REG_GOAL_POSITION 0x1E 00037 #define AX12_REG_MOVING_SPEED 0x20 00038 #define AX12_REG_VOLTS 0x2A 00039 #define AX12_REG_TEMP 0x2B 00040 #define AX12_REG_MOVING 0x2E 00041 #define AX12_REG_POSITION 0x24 00042 #define AX12_REG_MAXTORQUE 0x22 00043 #define AX12_REG_MOTORONOFF 0x18 00044 #define AX12_MODE_POSITION 0 00045 #define AX12_MODE_ROTATION 1 00046 00047 #define AX12_CW 1 00048 #define AX12_CCW 1 00049 #define goal_resolution 0.08789 00050 00051 #define READ_DEBUG 1 00052 00053 #include "Joint.h" 00054 00055 // TODO: spostare i metodi uguali da MX106, MX64 in questo file e in MX.cpp. 00056 00057 class MX : public Joint 00058 { 00059 00060 public: 00061 MX(communication_1& line, int ID, float gear_train); 00062 virtual ~MX(); 00063 void setID(int newID); 00064 int getID(); 00065 00066 /** Set the mode of the servo 00067 * @param mode 00068 * 0 = Positional, default 00069 * 1 = Continuous rotation 00070 */ 00071 virtual void setMode(int mode); 00072 00073 void setCWLimitUnits(short limit); 00074 void setCCWLimitUnits(short limit); 00075 00076 /** Set the clockwise limit of the servo 00077 * 00078 * @param degrees, 0-300 00079 */ 00080 void setCWLimit(float degrees); 00081 /** Set the counter-clockwise limit of the servo 00082 * 00083 * @param degrees, 0-300 00084 */ 00085 void setCCWLimit(float degrees); 00086 00087 /** Set the speed of the servo in continuous rotation mode 00088 * 00089 * @param speed, -1.0 to 1.0 00090 * -1.0 = full speed counter clock wise 00091 * 1.0 = full speed clock wise 00092 */ 00093 //void setCRSpeed(float speed); 00094 // 00095 void setGoalPosition(float degrees); 00096 float getPosition(); 00097 00098 /** Set goal angle in integer degrees, in positional mode 00099 * 00100 * @param degrees 0-300 00101 * @param flags, defaults to 0 00102 * flags[0] = blocking, return when goal position reached 00103 * flags[1] = register, activate with a broadcast trigger 00104 * 00105 */ 00106 int setGoal(float degrees, int flags = 0); 00107 void setMaxSpeed(float degreeS); 00108 void setCRSpeed(float degreeS); 00109 void setSpeed(float degreeS); 00110 float getTemp(); 00111 float getCurrent(); 00112 float getVolts(); 00113 virtual float getPGain(); 00114 virtual float getIGain(); 00115 virtual float getDGain(); 00116 virtual void setPGain(float gain); 00117 virtual void setIGain(float gain); 00118 virtual void setDGain(float gain); 00119 void setMaxTorque(float torque); 00120 void setMotorEnabled(bool enabled); 00121 bool isMoving(); 00122 00123 protected: 00124 communication_1& _line; 00125 float _gear_train; 00126 int _ID; 00127 int _mode; 00128 float maxSpeedDegreeS; 00129 float limitCWDegrees; 00130 float limitCCWDegrees;; 00131 }; 00132 00133 #endif
Generated on Fri Jul 15 2022 02:02:32 by
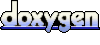