This is a driver for the segment LCD found on the Silicon Labs EF32 Giant, Leopard and Wonder Gecko platforms. NOTE: This driver will not work with other platforms, because it contains EFM32-specific code.
Dependents: EFM32 RDA5807M RDS Radio EMF32-Segment-Touch-Demo EMF32_ShowKey blinky_EFM32_Giant ... more
EFM32_SegmentLCD.h
00001 /***************************************************************************//** 00002 * @file EFM32_SegmentLCD.h 00003 * @brief Driver class for the segment LCD's on some of the EFM32 kits. 00004 ******************************************************************************* 00005 * @section License 00006 * <b>(C) Copyright 2015 Silicon Labs, http://www.silabs.com</b> 00007 ******************************************************************************* 00008 * 00009 * Permission is granted to anyone to use this software for any purpose, 00010 * including commercial applications, and to alter it and redistribute it 00011 * freely, subject to the following restrictions: 00012 * 00013 * 1. The origin of this software must not be misrepresented; you must not 00014 * claim that you wrote the original software. 00015 * 2. Altered source versions must be plainly marked as such, and must not be 00016 * misrepresented as being the original software. 00017 * 3. This notice may not be removed or altered from any source distribution. 00018 * 00019 * DISCLAIMER OF WARRANTY/LIMITATION OF REMEDIES: Silicon Labs has no 00020 * obligation to support this Software. Silicon Labs is providing the 00021 * Software "AS IS", with no express or implied warranties of any kind, 00022 * including, but not limited to, any implied warranties of merchantability 00023 * or fitness for any particular purpose or warranties against infringement 00024 * of any proprietary rights of a third party. 00025 * 00026 * Silicon Labs will not be liable for any consequential, incidental, or 00027 * special damages, or any other relief, or for any claim by any third party, 00028 * arising from your use of this Software. 00029 * 00030 ******************************************************************************/ 00031 00032 #ifndef SILABS_SEGMENTLCD_H 00033 #define SILABS_SEGMENTLCD_H 00034 00035 #ifndef TARGET_EFM32 00036 #error "The Silicon Labs SegmentLCD library is specifically designed for EFM32 targets." 00037 #else 00038 #include "platform.h" 00039 #include <mbed.h> 00040 00041 #include "segmentlcd.h" 00042 #include "sleepmodes.h" 00043 00044 namespace silabs { 00045 /** A driver for the Segment LCD present on some EFM32 starter kits 00046 * 00047 * Supports Giant, Leopard and Wonder Gecko STKs 00048 * 00049 * @code 00050 * #include "mbed.h" 00051 * #include "EFM32_SegmentLCD.h" 00052 * 00053 * EFM32_SegmentLCD segmentDisplay; 00054 * 00055 * int main() { 00056 * segmentDisplay.Write("Hello"); 00057 * while(1); 00058 * } 00059 * @endcode 00060 */ 00061 00062 class EFM32_SegmentLCD { 00063 public: 00064 /** 00065 * Constructor. 00066 */ 00067 EFM32_SegmentLCD(); 00068 00069 /** 00070 * Destructor. 00071 */ 00072 ~EFM32_SegmentLCD(); 00073 00074 /** 00075 * Switch off all elements 00076 */ 00077 void AllOff( void ); 00078 00079 /** 00080 * Switch on all elements 00081 */ 00082 void AllOn( void ); 00083 00084 /** 00085 * Switch off (clear) the alphanumeric portion of the display 00086 */ 00087 void AlphaNumberOff(void); 00088 00089 /** 00090 * Switch specified segment on the ring on/off 00091 * 00092 * @param anum ring segment index 00093 * @param on true to turn on, false to turn off 00094 */ 00095 void ARing(int anum, bool on); 00096 00097 /** 00098 * Display a battery level on the LCD. 00099 * 0 = off 00100 * 1 = lowest block 00101 * 2 = lowest + second-to-lowest 00102 * ... 00103 * 00104 * @param batteryLevel Level to show 00105 */ 00106 void Battery(int batteryLevel); 00107 00108 /** 00109 * Display an energy mode ring on the LCD. 00110 * 00111 * @param em energy mode number to display 00112 * @param on true to turn on, false to turn off. 00113 */ 00114 void EnergyMode(int em, bool on); 00115 00116 /** 00117 * Display an unsigned integer on the alphanumeric 00118 * portion of the display as a hex value. 00119 * 00120 * @param num number to display 00121 */ 00122 void LowerHex( uint32_t num ); 00123 00124 /** 00125 * Display a signed integer as decimal number on 00126 * the alphanumeric part of the display. 00127 * 00128 * @param num number to display 00129 */ 00130 void LowerNumber( int num ); 00131 00132 /** 00133 * Display a signed integer on the numeric part 00134 * of the display (clock area). 00135 * max = 9999, min = -9999 00136 */ 00137 void Number(int value); 00138 00139 /** 00140 * Clear the numeric part of the display. 00141 */ 00142 void NumberOff(void); 00143 00144 /** 00145 * Turn a predefined symbol on or off. 00146 * 00147 * @param lcdSymbol predefined symbol in segmentlcdconfig_*.h 00148 * @param on true to turn on, false to turn off. 00149 */ 00150 void Symbol(lcdSymbol s, bool on); 00151 00152 /** 00153 * Display an unsigned short integer as a hex value 00154 * on the numeric part of the display. 00155 * max = FFFF, min = 0 00156 * 00157 * @param value Value to show 00158 */ 00159 void UnsignedHex(uint16_t value); 00160 00161 /** 00162 * Display a 7-character string on the alphanumeric 00163 * portion of the display. 00164 * 00165 * @param string String to show (only the first 7 characters will be shown) 00166 */ 00167 void Write(char *string); 00168 protected: 00169 00170 }; 00171 } 00172 00173 #endif //TARGET_EFM32 00174 #endif //SILABS_SEGMENTLCD_H
Generated on Thu Jul 14 2022 05:15:53 by
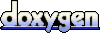