L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_ll_opamp.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_opamp.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of OPAMP LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_OPAMP_H 00040 #define __STM32L4xx_LL_OPAMP_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (OPAMP1) || defined (OPAMP2) 00054 00055 /** @defgroup OPAMP_LL OPAMP 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 /** @defgroup OPAMP_LL_Private_Constants OPAMP Private Constants 00064 * @{ 00065 */ 00066 /* Internal mask for OPAMP power mode: */ 00067 /* To select into literal LL_OPAMP_POWERMODE_x the relevant bits for: */ 00068 /* - OPAMP power mode into the control register */ 00069 /* - OPAMP trimming register offset */ 00070 00071 /* Internal register offset for OPAMP trimming configuration */ 00072 #define OPAMP_POWERMODE_OTR_REGOFFSET ((uint32_t)0x00000000) 00073 #define OPAMP_POWERMODE_LPOTR_REGOFFSET ((uint32_t)0x00000001) 00074 00075 #define OPAMP_POWERMODE_CSR_BIT_MASK (OPAMP_CSR_OPALPM) 00076 #define OPAMP_POWERMODE_OTRX_REGOFFSET_MASK (OPAMP_POWERMODE_LPOTR_REGOFFSET) 00077 00078 /* Internal mask for OPAMP trimming of transistors differential pair NMOS */ 00079 /* or PMOS. */ 00080 /* To select into literal LL_OPAMP_TRIMMING_x the relevant bits for: */ 00081 /* - OPAMP trimming selection of transistors differential pair */ 00082 /* - OPAMP trimming values of transistors differential pair */ 00083 #define OPAMP_TRIMMING_SELECT_MASK (OPAMP1_CSR_CALSEL) 00084 #define OPAMP_TRIMMING_VALUE_MASK (OPAMP_OTR_TRIMOFFSETP | OPAMP_OTR_TRIMOFFSETN) 00085 00086 /** 00087 * @} 00088 */ 00089 00090 00091 /* Private macros ------------------------------------------------------------*/ 00092 /** @defgroup OPAMP_LL_Private_Macros OPAMP Private Macros 00093 * @{ 00094 */ 00095 00096 /** 00097 * @brief Driver macro reserved for internal use: set a pointer to 00098 * a register from a register basis from which an offset 00099 * is applied. 00100 * @param __REG__ Register basis from which the offset is applied. 00101 * @param __REG_OFFSET__ Offset to be applied (unit: number of registers). 00102 * @retval Register address 00103 */ 00104 #define __OPAMP_PTR_REG_OFFSET(__REG__, __REG_OFFSET__) \ 00105 ((uint32_t *)((uint32_t) ((uint32_t)(&(__REG__)) + ((__REG_OFFSET__) << 2)))) 00106 00107 /** 00108 * @} 00109 */ 00110 00111 00112 /* Exported types ------------------------------------------------------------*/ 00113 /* Exported constants --------------------------------------------------------*/ 00114 /** @defgroup OPAMP_LL_Exported_Constants OPAMP Exported Constants 00115 * @{ 00116 */ 00117 00118 /** @defgroup OPAMP_LL_EC_POWERSUPPLY_RANGE POWERSUPPLY RANGE 00119 * @{ 00120 */ 00121 #define LL_OPAMP_POWERSUPPLY_RANGE_LOW ((uint32_t)0x00000000) /*!< Power supply range low. On STM32L4: Vdda lower than 2.4V. */ 00122 #define LL_OPAMP_POWERSUPPLY_RANGE_HIGH (OPAMP1_CSR_OPARANGE) /*!< Power supply range high. On STM32L4: Vdda higher than 2.4V. */ 00123 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup OPAMP_LL_EC_POWERMODE POWERMODE 00129 * @{ 00130 */ 00131 #define LL_OPAMP_POWERMODE_NORMAL (OPAMP_POWERMODE_OTR_REGOFFSET) /*!< OPAMP power mode normal */ 00132 #define LL_OPAMP_POWERMODE_LOWPOWER (OPAMP_POWERMODE_LPOTR_REGOFFSET | OPAMP_CSR_OPALPM) /*!< OPAMP power mode low-power */ 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup OPAMP_LL_EC_MODE MODE 00138 * @{ 00139 */ 00140 #define LL_OPAMP_MODE_FUNCTIONAL ((uint32_t)0x00000000) /*!< OPAMP functional mode */ 00141 #define LL_OPAMP_MODE_CALIBRATION (OPAMP1_CSR_CALON) /*!< OPAMP calibration mode */ 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup OPAMP_LL_EC_FUNCTIONAL_MODE FUNCTIONAL_MODE 00147 * @{ 00148 */ 00149 #define LL_OPAMP_MODE_STANDALONE ((uint32_t)0x00000000) /*!< OPAMP functional mode, OPAMP operation in standalone */ 00150 #define LL_OPAMP_MODE_FOLLOWER (OPAMP_CSR_OPAMODE_1 | OPAMP_CSR_OPAMODE_0) /*!< OPAMP functional mode, OPAMP operation in follower */ 00151 #define LL_OPAMP_MODE_PGA (OPAMP_CSR_OPAMODE_1) /*!< OPAMP functional mode, OPAMP operation in PGA */ 00152 /** 00153 * @} 00154 */ 00155 00156 /** @defgroup OPAMP_LL_EC_MODE_PGA_GAIN MODE PGA GAIN 00157 * @{ 00158 */ 00159 #define LL_OPAMP_PGA_GAIN_2 ((uint32_t)0x00000000) /*!< OPAMP PGA gain 2 */ 00160 #define LL_OPAMP_PGA_GAIN_4 (OPAMP_CSR_PGGAIN_0) /*!< OPAMP PGA gain 4 */ 00161 #define LL_OPAMP_PGA_GAIN_8 (OPAMP_CSR_PGGAIN_1) /*!< OPAMP PGA gain 8 */ 00162 #define LL_OPAMP_PGA_GAIN_16 (OPAMP_CSR_PGGAIN_1 | OPAMP_CSR_PGGAIN_0 ) /*!< OPAMP PGA gain 16 */ 00163 /** 00164 * @} 00165 */ 00166 00167 /** @defgroup OPAMP_LL_EC_INVERTINGINPUT INVERTINGINPUT 00168 * @{ 00169 */ 00170 #define LL_OPAMP_INVERTINGINPUT_IO0 ((uint32_t)0x00000000) /*!< OPAMP inverting input connected to GPIO pin (valid also in PGA mode for filtering) */ 00171 #define LL_OPAMP_INVERTINGINPUT_IO1 (OPAMP1_CSR_VMSEL_0) /*!< OPAMP inverting input (low leakage input) connected to GPIO pin (available only on package BGA132) */ 00172 #define LL_OPAMP_INVERTINGINPUT_CONNECT_NO (OPAMP1_CSR_VMSEL_1) /*!< OPAMP inverting input not externally connected (intended for OPAMP in mode PGA) */ 00173 /** 00174 * @} 00175 */ 00176 00177 /** @defgroup OPAMP_LL_EC_NonInvertingInput NonInvertingInput 00178 * @{ 00179 */ 00180 #define LL_OPAMP_NONINVERTINGINPUT_IO0 ((uint32_t)0x00000000) /*!< OPAMP non inverting input connected to GPIO pin */ 00181 #define LL_OPAMP_NONINVERTINGINPUT_DAC_CH (OPAMP1_CSR_VPSEL) /*!< OPAMP non inverting input connected to DAC1 channel1 output */ 00182 /** 00183 * @} 00184 */ 00185 00186 /** @defgroup OPAMP_LL_EC_TRIMMING_MODE TRIMMING MODE 00187 * @{ 00188 */ 00189 #define LL_OPAMP_TRIMMING_FACTORY ((uint32_t)0x00000000) /*!< Factory trimming */ 00190 #define LL_OPAMP_TRIMMING_USER (OPAMP_CSR_USERTRIM) /*!< User trimming */ 00191 /** 00192 * @} 00193 */ 00194 00195 /** @defgroup OPAMP_LL_EC_TRIMMING_TRANSISTORS_DIFF_PAIR TRIMMING OF TRANSISTORS DIFFERENTIAL PAIR 00196 * @{ 00197 */ 00198 #define LL_OPAMP_TRIMMING_NMOS (OPAMP_OTR_TRIMOFFSETN) /*!< Trimming of transistors differential pair NMOS */ 00199 #define LL_OPAMP_TRIMMING_PMOS (OPAMP_OTR_TRIMOFFSETP | OPAMP1_CSR_CALSEL) /*!< Trimming of transistors differential pair PMOS */ 00200 /** 00201 * @} 00202 */ 00203 00204 /** 00205 * @} 00206 */ 00207 00208 /* Exported macro ------------------------------------------------------------*/ 00209 /** @defgroup OPAMP_LL_Exported_Macros OPAMP Exported Macros 00210 * @{ 00211 */ 00212 /** @defgroup OPAMP_LL_EM_WRITE_READ Common Write and read registers macro 00213 * @{ 00214 */ 00215 /** 00216 * @brief Write a value in OPAMP register 00217 * @param __INSTANCE__ OPAMP Instance 00218 * @param __REG__ Register to be written 00219 * @param __VALUE__ Value to be written in the register 00220 * @retval None 00221 */ 00222 #define LL_OPAMP_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00223 00224 /** 00225 * @brief Read a value in OPAMP register 00226 * @param __INSTANCE__ OPAMP Instance 00227 * @param __REG__ Register to be read 00228 * @retval Register value 00229 */ 00230 #define LL_OPAMP_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00231 /** 00232 * @} 00233 */ 00234 00235 00236 /** 00237 * @} 00238 */ 00239 00240 /* Exported functions --------------------------------------------------------*/ 00241 /** @defgroup OPAMP_LL_Exported_Functions OPAMP Exported Functions 00242 * @{ 00243 */ 00244 00245 /** @defgroup OPAMP_LL_EF_Configuration_global Configuration of OPAMP global parameters 00246 * @{ 00247 */ 00248 /** 00249 * @brief Set OPAMP power range. 00250 * @note The OPAMP power range applies to all OPAMP instances. 00251 * @note The OPAMP power range selection must be performed while 00252 * OPAMPs are powered down. 00253 * @rmtoll CSR OPARANGE LL_OPAMP_SetPowerRange 00254 * @param PowerRange This parameter can be one of the following values: 00255 * @arg @ref LL_OPAMP_POWERSUPPLY_RANGE_LOW 00256 * @arg @ref LL_OPAMP_POWERSUPPLY_RANGE_HIGH 00257 * @retval None 00258 */ 00259 __STATIC_INLINE void LL_OPAMP_SetPowerRange(uint32_t PowerRange) 00260 { 00261 MODIFY_REG(OPAMP1->CSR, OPAMP1_CSR_OPARANGE, PowerRange); 00262 } 00263 00264 /** 00265 * @brief Get OPAMP power range. 00266 * @note The OPAMP power range applies to all OPAMP instances. 00267 * @note The OPAMP power range selection must be performed while 00268 * OPAMPs are powered down. 00269 * @rmtoll CSR OPARANGE LL_OPAMP_GetPowerRange 00270 * @retval Returned value can be one of the following values: 00271 * @arg @ref LL_OPAMP_POWERSUPPLY_RANGE_LOW 00272 * @arg @ref LL_OPAMP_POWERSUPPLY_RANGE_HIGH 00273 */ 00274 __STATIC_INLINE uint32_t LL_OPAMP_GetPowerRange(void) 00275 { 00276 return (uint32_t)(READ_BIT(OPAMP1->CSR, OPAMP1_CSR_OPARANGE)); 00277 } 00278 00279 /** 00280 * @brief Set OPAMP power mode. 00281 * @note The OPAMP must be disabled to change this configuration. 00282 * @rmtoll CSR OPALPM LL_OPAMP_SetPowerMode 00283 * @param OPAMPx OPAMP instance 00284 * @param PowerMode This parameter can be one of the following values: 00285 * @arg @ref LL_OPAMP_POWERMODE_NORMAL 00286 * @arg @ref LL_OPAMP_POWERMODE_LOWPOWER 00287 * @retval None 00288 */ 00289 __STATIC_INLINE void LL_OPAMP_SetPowerMode(OPAMP_TypeDef *OPAMPx, uint32_t PowerMode) 00290 { 00291 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_OPALPM, (PowerMode & OPAMP_POWERMODE_CSR_BIT_MASK)); 00292 } 00293 00294 /** 00295 * @brief Get OPAMP power mode. 00296 * @rmtoll CSR OPALPM LL_OPAMP_GetPowerMode 00297 * @param OPAMPx OPAMP instance 00298 * @retval Returned value can be one of the following values: 00299 * @arg @ref LL_OPAMP_POWERMODE_NORMAL 00300 * @arg @ref LL_OPAMP_POWERMODE_LOWPOWER 00301 */ 00302 __STATIC_INLINE uint32_t LL_OPAMP_GetPowerMode(OPAMP_TypeDef *OPAMPx) 00303 { 00304 register uint32_t power_mode = (READ_BIT(OPAMPx->CSR, OPAMP_CSR_OPALPM)); 00305 00306 return (uint32_t)(power_mode | (power_mode >> (POSITION_VAL(OPAMP_CSR_OPALPM)))); 00307 } 00308 00309 /** 00310 * @brief Set OPAMP mode. 00311 * @note OPAMP mode corresponds to functional or calibration mode: 00312 * - functional mode: OPAMP operation in standalone, follower, ... 00313 * Set functional mode using function 00314 * @ref LL_OPAMP_SetFunctionalMode(). 00315 * - calibration mode: calibration of the selected transistors 00316 * differential pair NMOS or PMOS. 00317 * @rmtoll CSR CALON LL_OPAMP_SetMode 00318 * @param OPAMPx OPAMP instance 00319 * @param Mode This parameter can be one of the following values: 00320 * @arg @ref LL_OPAMP_MODE_FUNCTIONAL 00321 * @arg @ref LL_OPAMP_MODE_CALIBRATION 00322 * @retval None 00323 */ 00324 __STATIC_INLINE void LL_OPAMP_SetMode(OPAMP_TypeDef *OPAMPx, uint32_t Mode) 00325 { 00326 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_CALON, Mode); 00327 } 00328 00329 /** 00330 * @brief Get OPAMP mode. 00331 * @note OPAMP mode corresponds to functional or calibration mode: 00332 * - functional mode: OPAMP operation in standalone, follower, ... 00333 * Set functional mode using function 00334 * @ref LL_OPAMP_SetFunctionalMode(). 00335 * - calibration mode: calibration of the selected transistors 00336 * differential pair NMOS or PMOS. 00337 * @rmtoll CSR CALON LL_OPAMP_GetMode 00338 * @param OPAMPx OPAMP instance 00339 * @retval Returned value can be one of the following values: 00340 * @arg @ref LL_OPAMP_MODE_FUNCTIONAL 00341 * @arg @ref LL_OPAMP_MODE_CALIBRATION 00342 */ 00343 __STATIC_INLINE uint32_t LL_OPAMP_GetMode(OPAMP_TypeDef *OPAMPx) 00344 { 00345 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_CALON)); 00346 } 00347 00348 /** 00349 * @brief Set OPAMP functional mode by setting internal connections. 00350 * OPAMP operation in standalone, follower, ... 00351 * @rmtoll CSR OPAMODE LL_OPAMP_SetFunctionalMode 00352 * @param OPAMPx OPAMP instance 00353 * @param Mode This parameter can be one of the following values: 00354 * @arg @ref LL_OPAMP_MODE_STANDALONE 00355 * @arg @ref LL_OPAMP_MODE_FOLLOWER 00356 * @arg @ref LL_OPAMP_MODE_PGA 00357 * @retval None 00358 */ 00359 __STATIC_INLINE void LL_OPAMP_SetFunctionalMode(OPAMP_TypeDef *OPAMPx, uint32_t Mode) 00360 { 00361 /* Note: Bit OPAMP_CSR_CALON reset to ensure to be in functional mode */ 00362 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_OPAMODE | OPAMP_CSR_CALON, Mode); 00363 } 00364 00365 /** 00366 * @brief Get OPAMP functional mode from setting of internal connections. 00367 * OPAMP operation in standalone, follower, ... 00368 * @rmtoll CSR OPAMODE LL_OPAMP_GetFunctionalMode 00369 * @param OPAMPx OPAMP instance 00370 * @retval Returned value can be one of the following values: 00371 * @arg @ref LL_OPAMP_MODE_STANDALONE 00372 * @arg @ref LL_OPAMP_MODE_FOLLOWER 00373 * @arg @ref LL_OPAMP_MODE_PGA 00374 */ 00375 __STATIC_INLINE uint32_t LL_OPAMP_GetFunctionalMode(OPAMP_TypeDef *OPAMPx) 00376 { 00377 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_OPAMODE)); 00378 } 00379 00380 /** 00381 * @brief Set OPAMP PGA gain. 00382 * @note Preliminarily, OPAMP must be set in mode PGA 00383 * using function @ref LL_OPAMP_SetFunctionalMode(). 00384 * @rmtoll CSR PGGAIN LL_OPAMP_SetPGAGain 00385 * @param OPAMPx OPAMP instance 00386 * @param PGAGain This parameter can be one of the following values: 00387 * @arg @ref LL_OPAMP_PGA_GAIN_2 00388 * @arg @ref LL_OPAMP_PGA_GAIN_4 00389 * @arg @ref LL_OPAMP_PGA_GAIN_8 00390 * @arg @ref LL_OPAMP_PGA_GAIN_16 00391 * @retval None 00392 */ 00393 __STATIC_INLINE void LL_OPAMP_SetPGAGain(OPAMP_TypeDef *OPAMPx, uint32_t PGAGain) 00394 { 00395 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_PGGAIN, PGAGain); 00396 } 00397 00398 /** 00399 * @brief Get OPAMP PGA gain. 00400 * @note Preliminarily, OPAMP must be set in mode PGA 00401 * using function @ref LL_OPAMP_SetFunctionalMode(). 00402 * @rmtoll CSR PGGAIN LL_OPAMP_GetPGAGain 00403 * @param OPAMPx OPAMP instance 00404 * @retval Returned value can be one of the following values: 00405 * @arg @ref LL_OPAMP_PGA_GAIN_2 00406 * @arg @ref LL_OPAMP_PGA_GAIN_4 00407 * @arg @ref LL_OPAMP_PGA_GAIN_8 00408 * @arg @ref LL_OPAMP_PGA_GAIN_16 00409 */ 00410 __STATIC_INLINE uint32_t LL_OPAMP_GetPGAGain(OPAMP_TypeDef *OPAMPx) 00411 { 00412 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_PGGAIN)); 00413 } 00414 00415 /** 00416 * @} 00417 */ 00418 00419 /** @defgroup OPAMP_LL_EF_Configuration_inputs Configuration of OPAMP inputs 00420 * @{ 00421 */ 00422 /** 00423 * @brief Set OPAMP inverting input connection. 00424 * @rmtoll CSR VMSEL LL_OPAMP_SetInvertingInput 00425 * @param OPAMPx OPAMP instance 00426 * @param InvertingInput This parameter can be one of the following values: 00427 * @arg @ref LL_OPAMP_INVERTINGINPUT_IO0 00428 * @arg @ref LL_OPAMP_INVERTINGINPUT_IO1 00429 * @arg @ref LL_OPAMP_INVERTINGINPUT_CONNECT_NO 00430 * @retval None 00431 */ 00432 __STATIC_INLINE void LL_OPAMP_SetInvertingInput(OPAMP_TypeDef *OPAMPx, uint32_t InvertingInput) 00433 { 00434 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_VMSEL, InvertingInput); 00435 } 00436 00437 /** 00438 * @brief Get OPAMP inverting input connection. 00439 * @rmtoll CSR VMSEL LL_OPAMP_GetInvertingInput 00440 * @param OPAMPx OPAMP instance 00441 * @retval Returned value can be one of the following values: 00442 * @arg @ref LL_OPAMP_INVERTINGINPUT_IO0 00443 * @arg @ref LL_OPAMP_INVERTINGINPUT_IO1 00444 * @arg @ref LL_OPAMP_INVERTINGINPUT_CONNECT_NO 00445 */ 00446 __STATIC_INLINE uint32_t LL_OPAMP_GetInvertingInput(OPAMP_TypeDef *OPAMPx) 00447 { 00448 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_VMSEL)); 00449 } 00450 00451 /** 00452 * @brief Set OPAMP non-inverting input connection. 00453 * @rmtoll CSR VPSEL LL_OPAMP_SetNonInvertingInput 00454 * @param OPAMPx OPAMP instance 00455 * @param NonInvertingInput This parameter can be one of the following values: 00456 * @arg @ref LL_OPAMP_NONINVERTINGINPUT_IO0 00457 * @arg @ref LL_OPAMP_NONINVERTINGINPUT_DAC_CH 00458 * @retval None 00459 */ 00460 __STATIC_INLINE void LL_OPAMP_SetNonInvertingInput(OPAMP_TypeDef *OPAMPx, uint32_t NonInvertingInput) 00461 { 00462 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_VPSEL, NonInvertingInput); 00463 } 00464 00465 /** 00466 * @brief Get OPAMP non-inverting input connection. 00467 * @rmtoll CSR VPSEL LL_OPAMP_GetNonInvertingInput 00468 * @param OPAMPx OPAMP instance 00469 * @retval Returned value can be one of the following values: 00470 * @arg @ref LL_OPAMP_NONINVERTINGINPUT_IO0 00471 * @arg @ref LL_OPAMP_NONINVERTINGINPUT_DAC_CH 00472 */ 00473 __STATIC_INLINE uint32_t LL_OPAMP_GetNonInvertingInput(OPAMP_TypeDef *OPAMPx) 00474 { 00475 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_VPSEL)); 00476 } 00477 00478 /** 00479 * @} 00480 */ 00481 00482 /** @defgroup OPAMP_LL_EF_OPAMP_trimming Configuration and operation of OPAMP trimming 00483 * @{ 00484 */ 00485 /** 00486 * @brief Set OPAMP trimming mode. 00487 * @rmtoll CSR USERTRIM LL_OPAMP_SetTrimmingMode 00488 * @param OPAMPx OPAMP instance 00489 * @param TrimmingMode This parameter can be one of the following values: 00490 * @arg @ref LL_OPAMP_TRIMMING_FACTORY 00491 * @arg @ref LL_OPAMP_TRIMMING_USER 00492 * @retval None 00493 */ 00494 __STATIC_INLINE void LL_OPAMP_SetTrimmingMode(OPAMP_TypeDef *OPAMPx, uint32_t TrimmingMode) 00495 { 00496 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_USERTRIM, TrimmingMode); 00497 } 00498 00499 /** 00500 * @brief Get OPAMP trimming mode. 00501 * @rmtoll CSR USERTRIM LL_OPAMP_GetTrimmingMode 00502 * @param OPAMPx OPAMP instance 00503 * @retval Returned value can be one of the following values: 00504 * @arg @ref LL_OPAMP_TRIMMING_FACTORY 00505 * @arg @ref LL_OPAMP_TRIMMING_USER 00506 */ 00507 __STATIC_INLINE uint32_t LL_OPAMP_GetTrimmingMode(OPAMP_TypeDef *OPAMPx) 00508 { 00509 return (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_USERTRIM)); 00510 } 00511 00512 /** 00513 * @brief Set OPAMP offset to calibrate the selected transistors 00514 * differential pair NMOS or PMOS. 00515 * @note Preliminarily, OPAMP must be set in mode calibration 00516 * using function @ref LL_OPAMP_SetMode(). 00517 * @rmtoll CSR CALSEL LL_OPAMP_SetCalibrationSelection 00518 * @param OPAMPx OPAMP instance 00519 * @param TransistorsDiffPair This parameter can be one of the following values: 00520 * @arg @ref LL_OPAMP_TRIMMING_NMOS 00521 * @arg @ref LL_OPAMP_TRIMMING_PMOS 00522 * @retval None 00523 */ 00524 __STATIC_INLINE void LL_OPAMP_SetCalibrationSelection(OPAMP_TypeDef *OPAMPx, uint32_t TransistorsDiffPair) 00525 { 00526 /* Parameter used with mask "OPAMP_TRIMMING_SELECT_MASK" because */ 00527 /* containing other bits reserved for other purpose. */ 00528 MODIFY_REG(OPAMPx->CSR, OPAMP_CSR_CALSEL, (TransistorsDiffPair & OPAMP_TRIMMING_SELECT_MASK)); 00529 } 00530 00531 /** 00532 * @brief Get OPAMP offset to calibrate the selected transistors 00533 * differential pair NMOS or PMOS. 00534 * @note Preliminarily, OPAMP must be set in mode calibration 00535 * using function @ref LL_OPAMP_SetMode(). 00536 * @rmtoll CSR CALSEL LL_OPAMP_GetCalibrationSelection 00537 * @param OPAMPx OPAMP instance 00538 * @retval Returned value can be one of the following values: 00539 * @arg @ref LL_OPAMP_TRIMMING_NMOS 00540 * @arg @ref LL_OPAMP_TRIMMING_PMOS 00541 */ 00542 __STATIC_INLINE uint32_t LL_OPAMP_GetCalibrationSelection(OPAMP_TypeDef *OPAMPx) 00543 { 00544 register uint32_t CalibrationSelection = (uint32_t)(READ_BIT(OPAMPx->CSR, OPAMP_CSR_CALSEL)); 00545 00546 return (CalibrationSelection | 00547 ((OPAMP_OTR_TRIMOFFSETN) << (POSITION_VAL(OPAMP_OTR_TRIMOFFSETP) * (CalibrationSelection && OPAMP1_CSR_CALSEL)))); 00548 } 00549 00550 /** 00551 * @brief Get OPAMP calibration result of toggling output. 00552 * @rmtoll CSR CALOUT LL_OPAMP_IsCalibrationOutputSet 00553 * @param OPAMPx OPAMP instance 00554 * @retval 0 if OPAMP calibration output is reset, 00555 * 1 if OPAMP calibration output is set. 00556 */ 00557 __STATIC_INLINE uint32_t LL_OPAMP_IsCalibrationOutputSet(OPAMP_TypeDef *OPAMPx) 00558 { 00559 return (READ_BIT(OPAMPx->CSR, OPAMP_CSR_CALOUT) == OPAMP_CSR_CALOUT); 00560 } 00561 00562 /** 00563 * @brief Set OPAMP trimming factor for the selected transistors 00564 * differential pair NMOS or PMOS, corresponding to the selected 00565 * power mode. 00566 * @param OPAMPx OPAMP instance 00567 * @param PowerMode This parameter can be one of the following values: 00568 * @arg @ref LL_OPAMP_POWERMODE_NORMAL 00569 * @arg @ref LL_OPAMP_POWERMODE_LOWPOWER 00570 * @param TransistorsDiffPair This parameter can be one of the following values: 00571 * @arg @ref LL_OPAMP_TRIMMING_NMOS 00572 * @arg @ref LL_OPAMP_TRIMMING_PMOS 00573 * @param TrimmingValue 0x00...0x1F 00574 * @retval None 00575 */ 00576 __STATIC_INLINE void LL_OPAMP_SetTrimmingValue(OPAMP_TypeDef* OPAMPx, uint32_t PowerMode, uint32_t TransistorsDiffPair, uint32_t TrimmingValue) 00577 { 00578 register uint32_t *preg = __OPAMP_PTR_REG_OFFSET(OPAMPx->OTR, (PowerMode & OPAMP_POWERMODE_OTRX_REGOFFSET_MASK)); 00579 00580 /* Set bits with position in register depending on parameter */ 00581 /* "TransistorsDiffPair". */ 00582 /* Parameter used with mask "OPAMP_TRIMMING_VALUE_MASK" because */ 00583 /* containing other bits reserved for other purpose. */ 00584 MODIFY_REG(*preg, 00585 (TransistorsDiffPair & OPAMP_TRIMMING_VALUE_MASK), 00586 TrimmingValue << (POSITION_VAL(TransistorsDiffPair & OPAMP_TRIMMING_VALUE_MASK))); 00587 } 00588 00589 /** 00590 * @brief Get OPAMP trimming factor for the selected transistors 00591 * differential pair NMOS or PMOS, corresponding to the selected 00592 * power mode. 00593 * @param OPAMPx OPAMP instance 00594 * @param PowerMode This parameter can be one of the following values: 00595 * @arg @ref LL_OPAMP_POWERMODE_NORMAL 00596 * @arg @ref LL_OPAMP_POWERMODE_LOWPOWER 00597 * @param TransistorsDiffPair This parameter can be one of the following values: 00598 * @arg @ref LL_OPAMP_TRIMMING_NMOS 00599 * @arg @ref LL_OPAMP_TRIMMING_PMOS 00600 * @retval 0x0...0x1F 00601 */ 00602 __STATIC_INLINE uint32_t LL_OPAMP_GetTrimmingValue(OPAMP_TypeDef* OPAMPx, uint32_t PowerMode, uint32_t TransistorsDiffPair) 00603 { 00604 register uint32_t *preg = __OPAMP_PTR_REG_OFFSET(OPAMPx->OTR, (PowerMode & OPAMP_POWERMODE_OTRX_REGOFFSET_MASK)); 00605 00606 /* Retrieve bits with position in register depending on parameter */ 00607 /* "TransistorsDiffPair". */ 00608 /* Parameter used with mask "OPAMP_TRIMMING_VALUE_MASK" because */ 00609 /* containing other bits reserved for other purpose. */ 00610 return (uint32_t)(READ_BIT(*preg, (TransistorsDiffPair & OPAMP_TRIMMING_VALUE_MASK)) 00611 >> (POSITION_VAL((TransistorsDiffPair & OPAMP_TRIMMING_VALUE_MASK))) 00612 ); 00613 } 00614 00615 /** 00616 * @} 00617 */ 00618 00619 /** @defgroup OPAMP_LL_EF_Operation Operation on OPAMP 00620 * @{ 00621 */ 00622 /** 00623 * @brief Enable OPAMP instance. 00624 * @rmtoll CSR OPAMPXEN LL_OPAMP_Enable 00625 * @param OPAMPx OPAMP instance 00626 * @retval None 00627 */ 00628 __STATIC_INLINE void LL_OPAMP_Enable(OPAMP_TypeDef *OPAMPx) 00629 { 00630 SET_BIT(OPAMPx->CSR, OPAMP_CSR_OPAMPxEN); 00631 } 00632 00633 /** 00634 * @brief Disable OPAMP instance. 00635 * @rmtoll CSR OPAMPXEN LL_OPAMP_Disable 00636 * @param OPAMPx OPAMP instance 00637 * @retval None 00638 */ 00639 __STATIC_INLINE void LL_OPAMP_Disable(OPAMP_TypeDef *OPAMPx) 00640 { 00641 CLEAR_BIT(OPAMPx->CSR, OPAMP_CSR_OPAMPxEN); 00642 } 00643 00644 /** 00645 * @brief Get OPAMP instance enable state 00646 * (0: OPAMP is disabled, 1: OPAMP is enabled) 00647 * @rmtoll CSR OPAMPXEN LL_OPAMP_IsEnabled 00648 * @param OPAMPx OPAMP instance 00649 * @retval State of bit (1 or 0). 00650 */ 00651 __STATIC_INLINE uint32_t LL_OPAMP_IsEnabled(OPAMP_TypeDef *OPAMPx) 00652 { 00653 return (READ_BIT(OPAMPx->CSR, OPAMP_CSR_OPAMPxEN) == (OPAMP_CSR_OPAMPxEN)); 00654 } 00655 00656 /** 00657 * @} 00658 */ 00659 00660 /** 00661 * @} 00662 */ 00663 00664 /** 00665 * @} 00666 */ 00667 00668 #endif /* OPAMP1 || OPAMP2 */ 00669 00670 /** 00671 * @} 00672 */ 00673 00674 #ifdef __cplusplus 00675 } 00676 #endif 00677 00678 #endif /* __STM32L4xx_LL_OPAMP_H */ 00679 00680 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00681
Generated on Tue Jul 12 2022 10:58:11 by
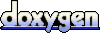