L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_ll_lpuart.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_lpuart.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of LPUART LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_LPUART_H 00040 #define __STM32L4xx_LL_LPUART_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (LPUART1) 00054 00055 /** @defgroup LPUART_LL LPUART 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 /** @defgroup LPUART_LL_Private_Constants LPUART Private Constants 00064 * @{ 00065 */ 00066 00067 /* Defines used for the bit position in the register and perform offsets*/ 00068 #define LPUART_POSITION_CR1_DEDT (uint32_t)POSITION_VAL(USART_CR1_DEDT) 00069 #define LPUART_POSITION_CR1_DEAT (uint32_t)POSITION_VAL(USART_CR1_DEAT) 00070 #define LPUART_POSITION_CR2_ADD (uint32_t)POSITION_VAL(USART_CR2_ADD) 00071 00072 /* Defines used in Baudrate related macros and corresponding register setting computation */ 00073 #define LPUART_LPUARTDIV_FREQ_MUL (uint32_t)(256) 00074 #define LPUART_BRR_MASK (uint32_t)(0x000FFFFF) 00075 /** 00076 * @} 00077 */ 00078 00079 00080 /* Private macros ------------------------------------------------------------*/ 00081 00082 /* Exported types ------------------------------------------------------------*/ 00083 /* Exported constants --------------------------------------------------------*/ 00084 /** @defgroup LPUART_LL_Exported_Constants LPUART Exported Constants 00085 * @{ 00086 */ 00087 00088 /** @defgroup LPUART_LL_EC_CLEAR_FLAG Clear Flags Defines 00089 * @brief Flags defines which can be used with LL_LPUART_WriteReg function 00090 * @{ 00091 */ 00092 #define LL_LPUART_ICR_PECF USART_ICR_PECF 00093 #define LL_LPUART_ICR_FECF USART_ICR_FECF 00094 #define LL_LPUART_ICR_NCF USART_ICR_NCF 00095 #define LL_LPUART_ICR_ORECF USART_ICR_ORECF 00096 #define LL_LPUART_ICR_IDLECF USART_ICR_IDLECF 00097 #define LL_LPUART_ICR_TCCF USART_ICR_TCCF 00098 #define LL_LPUART_ICR_CTSCF USART_ICR_CTSCF 00099 #define LL_LPUART_ICR_CMCF USART_ICR_CMCF 00100 #define LL_LPUART_ICR_WUCF USART_ICR_WUCF 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup LPUART_LL_EC_GET_FLAG Get Flags Defines 00106 * @brief Flags defines which can be used with LL_LPUART_ReadReg function 00107 * @{ 00108 */ 00109 #define LL_LPUART_ISR_PE USART_ISR_PE 00110 #define LL_LPUART_ISR_FE USART_ISR_FE 00111 #define LL_LPUART_ISR_NE USART_ISR_NE 00112 #define LL_LPUART_ISR_ORE USART_ISR_ORE 00113 #define LL_LPUART_ISR_IDLE USART_ISR_IDLE 00114 #define LL_LPUART_ISR_RXNE USART_ISR_RXNE 00115 #define LL_LPUART_ISR_TC USART_ISR_TC 00116 #define LL_LPUART_ISR_TXE USART_ISR_TXE 00117 #define LL_LPUART_ISR_CTSIF USART_ISR_CTSIF 00118 #define LL_LPUART_ISR_CTS USART_ISR_CTS 00119 #define LL_LPUART_ISR_BUSY USART_ISR_BUSY 00120 #define LL_LPUART_ISR_CMF USART_ISR_CMF 00121 #define LL_LPUART_ISR_SBKF USART_ISR_SBKF 00122 #define LL_LPUART_ISR_RWU USART_ISR_RWU 00123 #define LL_LPUART_ISR_WUF USART_ISR_WUF 00124 #define LL_LPUART_ISR_TEACK USART_ISR_TEACK 00125 #define LL_LPUART_ISR_REACK USART_ISR_REACK 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup LPUART_LL_EC_IT IT Defines 00131 * @brief IT defines which can be used with LL_LPUART_ReadReg and LL_LPUART_WriteReg functions 00132 * @{ 00133 */ 00134 #define LL_LPUART_CR1_IDLEIE USART_CR1_IDLEIE 00135 #define LL_LPUART_CR1_RXNEIE USART_CR1_RXNEIE 00136 #define LL_LPUART_CR1_TCIE USART_CR1_TCIE 00137 #define LL_LPUART_CR1_TXEIE USART_CR1_TXEIE 00138 #define LL_LPUART_CR1_PEIE USART_CR1_PEIE 00139 #define LL_LPUART_CR1_CMIE USART_CR1_CMIE 00140 #define LL_LPUART_CR3_EIE USART_CR3_EIE 00141 #define LL_LPUART_CR3_CTSIE USART_CR3_CTSIE 00142 #define LL_LPUART_CR3_WUFIE USART_CR3_WUFIE 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup LPUART_LL_EC_DIRECTION DIRECTION 00148 * @{ 00149 */ 00150 #define LL_LPUART_DIRECTION_NONE (uint32_t)0x00000000 /*!< Transmitter and Receiver are disabled */ 00151 #define LL_LPUART_DIRECTION_RX USART_CR1_RE /*!< Transmitter is disabled and Receiver is enabled */ 00152 #define LL_LPUART_DIRECTION_TX USART_CR1_TE /*!< Transmitter is enabled and Receiver is disabled */ 00153 #define LL_LPUART_DIRECTION_TX_RX (USART_CR1_TE |USART_CR1_RE) /*!< Transmitter and Receiver are enabled */ 00154 /** 00155 * @} 00156 */ 00157 00158 /** @defgroup LPUART_LL_EC_PARITY PARITY 00159 * @{ 00160 */ 00161 #define LL_LPUART_PARITY_NONE (uint32_t)0x00000000 /*!< Parity control disabled */ 00162 #define LL_LPUART_PARITY_EVEN USART_CR1_PCE /*!< Parity control enabled and Even Parity is selected */ 00163 #define LL_LPUART_PARITY_ODD (USART_CR1_PCE | USART_CR1_PS) /*!< Parity control enabled and Odd Parity is selected */ 00164 /** 00165 * @} 00166 */ 00167 00168 /** @defgroup LPUART_LL_EC_WAKEUP WAKEUP 00169 * @{ 00170 */ 00171 #define LL_LPUART_WAKEUP_IDLELINE (uint32_t)0x00000000 /*!< LPUART wakeup from Mute mode on Idle Line */ 00172 #define LL_LPUART_WAKEUP_ADDRESSMARK USART_CR1_WAKE /*!< LPUART wakeup from Mute mode on Address Mark */ 00173 /** 00174 * @} 00175 */ 00176 00177 /** @defgroup LPUART_LL_EC_DATAWIDTH DATAWIDTH 00178 * @{ 00179 */ 00180 #define LL_LPUART_DATAWIDTH_7B USART_CR1_M1 /*!< 7 bits word length : Start bit, 7 data bits, n stop bits */ 00181 #define LL_LPUART_DATAWIDTH_8B (uint32_t)0x00000000 /*!< 8 bits word length : Start bit, 8 data bits, n stop bits */ 00182 #define LL_LPUART_DATAWIDTH_9B USART_CR1_M0 /*!< 9 bits word length : Start bit, 9 data bits, n stop bits */ 00183 /** 00184 * @} 00185 */ 00186 00187 /** @defgroup LPUART_LL_EC_STOPBITS STOPBITS 00188 * @{ 00189 */ 00190 #define LL_LPUART_STOPBITS_1 (uint32_t)0x00000000 /*!< 1 stop bit */ 00191 #define LL_LPUART_STOPBITS_2 USART_CR2_STOP_1 /*!< 2 stop bits */ 00192 /** 00193 * @} 00194 */ 00195 00196 /** @defgroup LPUART_LL_EC_TXRX TXRX 00197 * @{ 00198 */ 00199 #define LL_LPUART_TXRX_STANDARD (uint32_t)0x00000000 /*!< TX/RX pins are used as defined in standard pinout */ 00200 #define LL_LPUART_TXRX_SWAPPED (USART_CR2_SWAP) /*!< TX and RX pins functions are swapped. */ 00201 /** 00202 * @} 00203 */ 00204 00205 /** @defgroup LPUART_LL_EC_RXPIN_LEVEL RXPIN LEVEL 00206 * @{ 00207 */ 00208 #define LL_LPUART_RXPIN_LEVEL_STANDARD (uint32_t)0x00000000 /*!< RX pin signal works using the standard logic levels */ 00209 #define LL_LPUART_RXPIN_LEVEL_INVERTED (USART_CR2_RXINV) /*!< RX pin signal values are inverted. */ 00210 /** 00211 * @} 00212 */ 00213 00214 /** @defgroup LPUART_LL_EC_TXPIN_LEVEL TXPIN LEVEL 00215 * @{ 00216 */ 00217 #define LL_LPUART_TXPIN_LEVEL_STANDARD (uint32_t)0x00000000 /*!< TX pin signal works using the standard logic levels */ 00218 #define LL_LPUART_TXPIN_LEVEL_INVERTED (USART_CR2_TXINV) /*!< TX pin signal values are inverted. */ 00219 /** 00220 * @} 00221 */ 00222 00223 /** @defgroup LPUART_LL_EC_BINARY_LOGIC BINARY LOGIC 00224 * @{ 00225 */ 00226 #define LL_LPUART_BINARY_LOGIC_POSITIVE (uint32_t)0x00000000 /*!< Logical data from the data register are send/received in positive/direct logic. (1=H, 0=L) */ 00227 #define LL_LPUART_BINARY_LOGIC_NEGATIVE USART_CR2_DATAINV /*!< Logical data from the data register are send/received in negative/inverse logic. (1=L, 0=H). The parity bit is also inverted. */ 00228 /** 00229 * @} 00230 */ 00231 00232 /** @defgroup LPUART_LL_EC_BITORDER BITORDER 00233 * @{ 00234 */ 00235 #define LL_LPUART_BITORDER_LSBFIRST (uint32_t)0x00000000 /*!< data is transmitted/received with data bit 0 first, following the start bit */ 00236 #define LL_LPUART_BITORDER_MSBFIRST USART_CR2_MSBFIRST /*!< data is transmitted/received with the MSB first, following the start bit */ 00237 /** 00238 * @} 00239 */ 00240 00241 /** @defgroup LPUART_LL_EC_ADDRESS_DETECT ADDRESS DETECT 00242 * @{ 00243 */ 00244 #define LL_LPUART_ADDRESS_DETECT_4B (uint32_t)0x00000000 /*!< 4-bit address detection method selected */ 00245 #define LL_LPUART_ADDRESS_DETECT_7B USART_CR2_ADDM7 /*!< 7-bit address detection (in 8-bit data mode) method selected */ 00246 /** 00247 * @} 00248 */ 00249 00250 /** @defgroup LPUART_LL_EC_HWCONTROL HWCONTROL 00251 * @{ 00252 */ 00253 #define LL_LPUART_HWCONTROL_NONE (uint32_t)0x00000000 /*!< CTS and RTS hardware flow control disabled */ 00254 #define LL_LPUART_HWCONTROL_RTS USART_CR3_RTSE /*!< RTS output enabled, data is only requested when there is space in the receive buffer */ 00255 #define LL_LPUART_HWCONTROL_CTS USART_CR3_CTSE /*!< CTS mode enabled, data is only transmitted when the nCTS input is asserted (tied to 0) */ 00256 #define LL_LPUART_HWCONTROL_RTS_CTS (USART_CR3_RTSE | USART_CR3_CTSE) /*!< CTS and RTS hardware flow control enabled */ 00257 /** 00258 * @} 00259 */ 00260 00261 /** @defgroup LPUART_LL_EC_WAKEUP_ON WAKEUP ON 00262 * @{ 00263 */ 00264 #define LL_LPUART_WAKEUP_ON_ADDRESS (uint32_t)0x00000000 /*!< Wakeup active on address match */ 00265 #define LL_LPUART_WAKEUP_ON_STARTBIT USART_CR3_WUS_1 /*!< Wakeup active on Start bit detection */ 00266 #define LL_LPUART_WAKEUP_ON_RXNE (USART_CR3_WUS_0 | USART_CR3_WUS_1) /*!< Wakeup active on RXNE */ 00267 /** 00268 * @} 00269 */ 00270 00271 /** @defgroup LPUART_LL_EC_DE_POLARITY DE POLARITY 00272 * @{ 00273 */ 00274 #define LL_LPUART_DE_POLARITY_HIGH (uint32_t)0x00000000 /*!< DE signal is active high */ 00275 #define LL_LPUART_DE_POLARITY_LOW USART_CR3_DEP /*!< DE signal is active low */ 00276 /** 00277 * @} 00278 */ 00279 00280 /** @defgroup LPUART_LL_EC_DMA_REG_DATA DMA register data 00281 * @{ 00282 */ 00283 #define LL_LPUART_DMA_REG_DATA_TRANSMIT (uint32_t)0 /*!< Get address of data register used for transmission */ 00284 #define LL_LPUART_DMA_REG_DATA_RECEIVE (uint32_t)1 /*!< Get address of data register used for reception */ 00285 /** 00286 * @} 00287 */ 00288 00289 /** 00290 * @} 00291 */ 00292 00293 /* Exported macro ------------------------------------------------------------*/ 00294 /** @defgroup LPUART_LL_Exported_Macros LPUART Exported Macros 00295 * @{ 00296 */ 00297 00298 /** @defgroup LPUART_LL_EM_WRITE_READ Common Write and read registers Macros 00299 * @{ 00300 */ 00301 00302 /** 00303 * @brief Write a value in LPUART register 00304 * @param __INSTANCE__ LPUART Instance 00305 * @param __REG__ Register to be written 00306 * @param __VALUE__ Value to be written in the register 00307 * @retval None 00308 */ 00309 #define LL_LPUART_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00310 00311 /** 00312 * @brief Read a value in LPUART register 00313 * @param __INSTANCE__ LPUART Instance 00314 * @param __REG__ Register to be read 00315 * @retval Register value 00316 */ 00317 #define LL_LPUART_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00318 /** 00319 * @} 00320 */ 00321 00322 /** @defgroup LPUART_LL_EM_Exported_Macros_Helper Helper Macros 00323 * @{ 00324 */ 00325 00326 /** 00327 * @brief Compute LPUARTDIV value according to Peripheral Clock and 00328 * expected Baudrate (20-bit value of LPUARTDIV is returned) 00329 * @param __PERIPHCLK__ Peripheral Clock frequency used for LPUART Instance 00330 * @param __BAUDRATE__ Baudrate value to achieve 00331 * @retval LPUARTDIV value to be used for BRR register filling 00332 */ 00333 #define __LL_LPUART_DIV(__PERIPHCLK__, __BAUDRATE__) ((((uint64_t)(__PERIPHCLK__)*LPUART_LPUARTDIV_FREQ_MUL)/(__BAUDRATE__)) & LPUART_BRR_MASK) 00334 00335 /** 00336 * @} 00337 */ 00338 00339 /** 00340 * @} 00341 */ 00342 00343 /* Exported functions --------------------------------------------------------*/ 00344 /** @defgroup LPUART_LL_Exported_Functions LPUART Exported Functions 00345 * @{ 00346 */ 00347 00348 /** @defgroup LPUART_LL_EF_Configuration Configuration functions 00349 * @{ 00350 */ 00351 00352 /** 00353 * @brief LPUART Enable 00354 * @rmtoll CR1 UE LL_LPUART_Enable 00355 * @param LPUARTx LPUART Instance 00356 * @retval None 00357 */ 00358 __STATIC_INLINE void LL_LPUART_Enable(USART_TypeDef *LPUARTx) 00359 { 00360 SET_BIT(LPUARTx->CR1, USART_CR1_UE); 00361 } 00362 00363 /** 00364 * @brief LPUART Disable 00365 * @note When LPUART is disabled, LPUART prescalers and outputs are stopped immediately, 00366 * and current operations are discarded. The configuration of the LPUART is kept, but all the status 00367 * flags, in the LPUARTx_ISR are set to their default values. 00368 * @note In order to go into low-power mode without generating errors on the line, 00369 * the TE bit must be reset before and the software must wait 00370 * for the TC bit in the LPUART_ISR to be set before resetting the UE bit. 00371 * The DMA requests are also reset when UE = 0 so the DMA channel must 00372 * be disabled before resetting the UE bit. 00373 * @rmtoll CR1 UE LL_LPUART_Disable 00374 * @param LPUARTx LPUART Instance 00375 * @retval None 00376 */ 00377 __STATIC_INLINE void LL_LPUART_Disable(USART_TypeDef *LPUARTx) 00378 { 00379 CLEAR_BIT(LPUARTx->CR1, USART_CR1_UE); 00380 } 00381 00382 /** 00383 * @brief Indicate if LPUART is enabled 00384 * @rmtoll CR1 UE LL_LPUART_IsEnabled 00385 * @param LPUARTx LPUART Instance 00386 * @retval State of bit (1 or 0). 00387 */ 00388 __STATIC_INLINE uint32_t LL_LPUART_IsEnabled(USART_TypeDef *LPUARTx) 00389 { 00390 return (READ_BIT(LPUARTx->CR1, USART_CR1_UE) == (USART_CR1_UE)); 00391 } 00392 00393 /** 00394 * @brief LPUART enabled in STOP Mode 00395 * @note When this function is enabled, LPUART is able to wake up the MCU from Stop mode, provided that 00396 * LPUART clock selection is HSI or LSE in RCC. 00397 * @rmtoll CR1 UESM LL_LPUART_EnableInStopMode 00398 * @param LPUARTx LPUART Instance 00399 * @retval None 00400 */ 00401 __STATIC_INLINE void LL_LPUART_EnableInStopMode(USART_TypeDef *LPUARTx) 00402 { 00403 SET_BIT(LPUARTx->CR1, USART_CR1_UESM); 00404 } 00405 00406 /** 00407 * @brief LPUART disabled in STOP Mode 00408 * @note When this function is disabled, LPUART is not able to wake up the MCU from Stop mode 00409 * @rmtoll CR1 UESM LL_LPUART_DisableInStopMode 00410 * @param LPUARTx LPUART Instance 00411 * @retval None 00412 */ 00413 __STATIC_INLINE void LL_LPUART_DisableInStopMode(USART_TypeDef *LPUARTx) 00414 { 00415 CLEAR_BIT(LPUARTx->CR1, USART_CR1_UESM); 00416 } 00417 00418 /** 00419 * @brief Indicate if LPUART is enabled in STOP Mode 00420 * (able to wake up MCU from Stop mode or not) 00421 * @rmtoll CR1 UESM LL_LPUART_IsEnabledInStopMode 00422 * @param LPUARTx LPUART Instance 00423 * @retval State of bit (1 or 0). 00424 */ 00425 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledInStopMode(USART_TypeDef *LPUARTx) 00426 { 00427 return (READ_BIT(LPUARTx->CR1, USART_CR1_UESM) == (USART_CR1_UESM)); 00428 } 00429 00430 /** 00431 * @brief Receiver Enable (Receiver is enabled and begins searching for a start bit) 00432 * @rmtoll CR1 RE LL_LPUART_EnableDirectionRx 00433 * @param LPUARTx LPUART Instance 00434 * @retval None 00435 */ 00436 __STATIC_INLINE void LL_LPUART_EnableDirectionRx(USART_TypeDef *LPUARTx) 00437 { 00438 SET_BIT(LPUARTx->CR1, USART_CR1_RE); 00439 } 00440 00441 /** 00442 * @brief Receiver Disable 00443 * @rmtoll CR1 RE LL_LPUART_DisableDirectionRx 00444 * @param LPUARTx LPUART Instance 00445 * @retval None 00446 */ 00447 __STATIC_INLINE void LL_LPUART_DisableDirectionRx(USART_TypeDef *LPUARTx) 00448 { 00449 CLEAR_BIT(LPUARTx->CR1, USART_CR1_RE); 00450 } 00451 00452 /** 00453 * @brief Transmitter Enable 00454 * @rmtoll CR1 TE LL_LPUART_EnableDirectionTx 00455 * @param LPUARTx LPUART Instance 00456 * @retval None 00457 */ 00458 __STATIC_INLINE void LL_LPUART_EnableDirectionTx(USART_TypeDef *LPUARTx) 00459 { 00460 SET_BIT(LPUARTx->CR1, USART_CR1_TE); 00461 } 00462 00463 /** 00464 * @brief Transmitter Disable 00465 * @rmtoll CR1 TE LL_LPUART_DisableDirectionTx 00466 * @param LPUARTx LPUART Instance 00467 * @retval None 00468 */ 00469 __STATIC_INLINE void LL_LPUART_DisableDirectionTx(USART_TypeDef *LPUARTx) 00470 { 00471 CLEAR_BIT(LPUARTx->CR1, USART_CR1_TE); 00472 } 00473 00474 /** 00475 * @brief Configure simultaneously enabled/disabled states 00476 * of Transmitter and Receiver 00477 * @rmtoll CR1 RE LL_LPUART_SetTransferDirection\n 00478 * CR1 TE LL_LPUART_SetTransferDirection 00479 * @param LPUARTx LPUART Instance 00480 * @param Direction This parameter can be one of the following values: 00481 * @arg @ref LL_LPUART_DIRECTION_NONE 00482 * @arg @ref LL_LPUART_DIRECTION_RX 00483 * @arg @ref LL_LPUART_DIRECTION_TX 00484 * @arg @ref LL_LPUART_DIRECTION_TX_RX 00485 * @retval None 00486 */ 00487 __STATIC_INLINE void LL_LPUART_SetTransferDirection(USART_TypeDef *LPUARTx, uint32_t Direction) 00488 { 00489 MODIFY_REG(LPUARTx->CR1, USART_CR1_RE | USART_CR1_TE, Direction); 00490 } 00491 00492 /** 00493 * @brief Return enabled/disabled states of Transmitter and Receiver 00494 * @rmtoll CR1 RE LL_LPUART_GetTransferDirection\n 00495 * CR1 TE LL_LPUART_GetTransferDirection 00496 * @param LPUARTx LPUART Instance 00497 * @retval Returned value can be one of the following values: 00498 * @arg @ref LL_LPUART_DIRECTION_NONE 00499 * @arg @ref LL_LPUART_DIRECTION_RX 00500 * @arg @ref LL_LPUART_DIRECTION_TX 00501 * @arg @ref LL_LPUART_DIRECTION_TX_RX 00502 */ 00503 __STATIC_INLINE uint32_t LL_LPUART_GetTransferDirection(USART_TypeDef *LPUARTx) 00504 { 00505 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_RE | USART_CR1_TE)); 00506 } 00507 00508 /** 00509 * @brief Configure Parity (enabled/disabled and parity mode if enabled) 00510 * @note This function selects if hardware parity control (generation and detection) is enabled or disabled. 00511 * When the parity control is enabled (Odd or Even), computed parity bit is inserted at the MSB position 00512 * (depending on data width) and parity is checked on the received data. 00513 * @rmtoll CR1 PS LL_LPUART_SetParity\n 00514 * CR1 PCE LL_LPUART_SetParity 00515 * @param LPUARTx LPUART Instance 00516 * @param ParityMode This parameter can be one of the following values: 00517 * @arg @ref LL_LPUART_PARITY_NONE 00518 * @arg @ref LL_LPUART_PARITY_EVEN 00519 * @arg @ref LL_LPUART_PARITY_ODD 00520 * @retval None 00521 */ 00522 __STATIC_INLINE void LL_LPUART_SetParity(USART_TypeDef *LPUARTx, uint32_t ParityMode) 00523 { 00524 MODIFY_REG(LPUARTx->CR1, USART_CR1_PS | USART_CR1_PCE, ParityMode); 00525 } 00526 00527 /** 00528 * @brief Return Parity configuration (enabled/disabled and parity mode if enabled) 00529 * @rmtoll CR1 PS LL_LPUART_GetParity\n 00530 * CR1 PCE LL_LPUART_GetParity 00531 * @param LPUARTx LPUART Instance 00532 * @retval Returned value can be one of the following values: 00533 * @arg @ref LL_LPUART_PARITY_NONE 00534 * @arg @ref LL_LPUART_PARITY_EVEN 00535 * @arg @ref LL_LPUART_PARITY_ODD 00536 */ 00537 __STATIC_INLINE uint32_t LL_LPUART_GetParity(USART_TypeDef *LPUARTx) 00538 { 00539 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_PS | USART_CR1_PCE)); 00540 } 00541 00542 /** 00543 * @brief Set Receiver Wakeup method from Mute mode. 00544 * @rmtoll CR1 WAKE LL_LPUART_SetWakeUpMethod 00545 * @param LPUARTx LPUART Instance 00546 * @param Method This parameter can be one of the following values: 00547 * @arg @ref LL_LPUART_WAKEUP_IDLELINE 00548 * @arg @ref LL_LPUART_WAKEUP_ADDRESSMARK 00549 * @retval None 00550 */ 00551 __STATIC_INLINE void LL_LPUART_SetWakeUpMethod(USART_TypeDef *LPUARTx, uint32_t Method) 00552 { 00553 MODIFY_REG(LPUARTx->CR1, USART_CR1_WAKE, Method); 00554 } 00555 00556 /** 00557 * @brief Return Receiver Wakeup method from Mute mode 00558 * @rmtoll CR1 WAKE LL_LPUART_GetWakeUpMethod 00559 * @param LPUARTx LPUART Instance 00560 * @retval Returned value can be one of the following values: 00561 * @arg @ref LL_LPUART_WAKEUP_IDLELINE 00562 * @arg @ref LL_LPUART_WAKEUP_ADDRESSMARK 00563 */ 00564 __STATIC_INLINE uint32_t LL_LPUART_GetWakeUpMethod(USART_TypeDef *LPUARTx) 00565 { 00566 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_WAKE)); 00567 } 00568 00569 /** 00570 * @brief Set Word length (nb of data bits, excluding start and stop bits) 00571 * @rmtoll CR1 M LL_LPUART_SetDataWidth 00572 * @param LPUARTx LPUART Instance 00573 * @param DataWidth This parameter can be one of the following values: 00574 * @arg @ref LL_LPUART_DATAWIDTH_7B 00575 * @arg @ref LL_LPUART_DATAWIDTH_8B 00576 * @arg @ref LL_LPUART_DATAWIDTH_9B 00577 * @retval None 00578 */ 00579 __STATIC_INLINE void LL_LPUART_SetDataWidth(USART_TypeDef *LPUARTx, uint32_t DataWidth) 00580 { 00581 MODIFY_REG(LPUARTx->CR1, USART_CR1_M, DataWidth); 00582 } 00583 00584 /** 00585 * @brief Return Word length (i.e. nb of data bits, excluding start and stop bits) 00586 * @rmtoll CR1 M LL_LPUART_GetDataWidth 00587 * @param LPUARTx LPUART Instance 00588 * @retval Returned value can be one of the following values: 00589 * @arg @ref LL_LPUART_DATAWIDTH_7B 00590 * @arg @ref LL_LPUART_DATAWIDTH_8B 00591 * @arg @ref LL_LPUART_DATAWIDTH_9B 00592 */ 00593 __STATIC_INLINE uint32_t LL_LPUART_GetDataWidth(USART_TypeDef *LPUARTx) 00594 { 00595 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_M)); 00596 } 00597 00598 /** 00599 * @brief Allow switch between Mute Mode and Active mode 00600 * @rmtoll CR1 MME LL_LPUART_EnableMuteMode 00601 * @param LPUARTx LPUART Instance 00602 * @retval None 00603 */ 00604 __STATIC_INLINE void LL_LPUART_EnableMuteMode(USART_TypeDef *LPUARTx) 00605 { 00606 SET_BIT(LPUARTx->CR1, USART_CR1_MME); 00607 } 00608 00609 /** 00610 * @brief Prevent Mute Mode use. Set Receiver in active mode permanently. 00611 * @rmtoll CR1 MME LL_LPUART_DisableMuteMode 00612 * @param LPUARTx LPUART Instance 00613 * @retval None 00614 */ 00615 __STATIC_INLINE void LL_LPUART_DisableMuteMode(USART_TypeDef *LPUARTx) 00616 { 00617 CLEAR_BIT(LPUARTx->CR1, USART_CR1_MME); 00618 } 00619 00620 /** 00621 * @brief Indicate if switch between Mute Mode and Active mode is allowed 00622 * @rmtoll CR1 MME LL_LPUART_IsEnabledMuteMode 00623 * @param LPUARTx LPUART Instance 00624 * @retval State of bit (1 or 0). 00625 */ 00626 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledMuteMode(USART_TypeDef *LPUARTx) 00627 { 00628 return (READ_BIT(LPUARTx->CR1, USART_CR1_MME) == (USART_CR1_MME)); 00629 } 00630 00631 /** 00632 * @brief Set the length of the stop bits 00633 * @rmtoll CR2 STOP LL_LPUART_SetStopBitsLength 00634 * @param LPUARTx LPUART Instance 00635 * @param StopBits This parameter can be one of the following values: 00636 * @arg @ref LL_LPUART_STOPBITS_1 00637 * @arg @ref LL_LPUART_STOPBITS_2 00638 * @retval None 00639 */ 00640 __STATIC_INLINE void LL_LPUART_SetStopBitsLength(USART_TypeDef *LPUARTx, uint32_t StopBits) 00641 { 00642 MODIFY_REG(LPUARTx->CR2, USART_CR2_STOP, StopBits); 00643 } 00644 00645 /** 00646 * @brief Retrieve the length of the stop bits 00647 * @rmtoll CR2 STOP LL_LPUART_GetStopBitsLength 00648 * @param LPUARTx LPUART Instance 00649 * @retval Returned value can be one of the following values: 00650 * @arg @ref LL_LPUART_STOPBITS_1 00651 * @arg @ref LL_LPUART_STOPBITS_2 00652 */ 00653 __STATIC_INLINE uint32_t LL_LPUART_GetStopBitsLength(USART_TypeDef *LPUARTx) 00654 { 00655 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_STOP)); 00656 } 00657 00658 /** 00659 * @brief Configure Character frame format (Datawidth, Parity control, Stop Bits) 00660 * @note Call of this function is equivalent to following function call sequence : 00661 * - Data Width configuration using @ref LL_LPUART_SetDataWidth() function 00662 * - Parity Control and mode configuration using @ref LL_LPUART_SetParity() function 00663 * - Stop bits configuration using @ref LL_LPUART_SetStopBitsLength() function 00664 * @rmtoll CR1 PS LL_LPUART_ConfigCharacter\n 00665 * CR1 PCE LL_LPUART_ConfigCharacter\n 00666 * CR1 M LL_LPUART_ConfigCharacter\n 00667 * CR2 STOP LL_LPUART_ConfigCharacter 00668 * @param LPUARTx LPUART Instance 00669 * @param DataWidth This parameter can be one of the following values: 00670 * @arg @ref LL_LPUART_DATAWIDTH_7B 00671 * @arg @ref LL_LPUART_DATAWIDTH_8B 00672 * @arg @ref LL_LPUART_DATAWIDTH_9B 00673 * @param ParityMode This parameter can be one of the following values: 00674 * @arg @ref LL_LPUART_PARITY_NONE 00675 * @arg @ref LL_LPUART_PARITY_EVEN 00676 * @arg @ref LL_LPUART_PARITY_ODD 00677 * @param StopBits This parameter can be one of the following values: 00678 * @arg @ref LL_LPUART_STOPBITS_1 00679 * @arg @ref LL_LPUART_STOPBITS_2 00680 * @retval None 00681 */ 00682 __STATIC_INLINE void LL_LPUART_ConfigCharacter(USART_TypeDef *LPUARTx, uint32_t DataWidth, uint32_t ParityMode, 00683 uint32_t StopBits) 00684 { 00685 MODIFY_REG(LPUARTx->CR1, USART_CR1_PS | USART_CR1_PCE | USART_CR1_M, ParityMode | DataWidth); 00686 MODIFY_REG(LPUARTx->CR2, USART_CR2_STOP, StopBits); 00687 } 00688 00689 /** 00690 * @brief Configure TX/RX pins swapping setting. 00691 * @rmtoll CR2 SWAP LL_LPUART_SetTXRXSwap 00692 * @param LPUARTx LPUART Instance 00693 * @param SwapConfig This parameter can be one of the following values: 00694 * @arg @ref LL_LPUART_TXRX_STANDARD 00695 * @arg @ref LL_LPUART_TXRX_SWAPPED 00696 * @retval None 00697 */ 00698 __STATIC_INLINE void LL_LPUART_SetTXRXSwap(USART_TypeDef *LPUARTx, uint32_t SwapConfig) 00699 { 00700 MODIFY_REG(LPUARTx->CR2, USART_CR2_SWAP, SwapConfig); 00701 } 00702 00703 /** 00704 * @brief Retrieve TX/RX pins swapping configuration. 00705 * @rmtoll CR2 SWAP LL_LPUART_GetTXRXSwap 00706 * @param LPUARTx LPUART Instance 00707 * @retval Returned value can be one of the following values: 00708 * @arg @ref LL_LPUART_TXRX_STANDARD 00709 * @arg @ref LL_LPUART_TXRX_SWAPPED 00710 */ 00711 __STATIC_INLINE uint32_t LL_LPUART_GetTXRXSwap(USART_TypeDef *LPUARTx) 00712 { 00713 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_SWAP)); 00714 } 00715 00716 /** 00717 * @brief Configure RX pin active level logic 00718 * @rmtoll CR2 RXINV LL_LPUART_SetRXPinLevel 00719 * @param LPUARTx LPUART Instance 00720 * @param PinInvMethod This parameter can be one of the following values: 00721 * @arg @ref LL_LPUART_RXPIN_LEVEL_STANDARD 00722 * @arg @ref LL_LPUART_RXPIN_LEVEL_INVERTED 00723 * @retval None 00724 */ 00725 __STATIC_INLINE void LL_LPUART_SetRXPinLevel(USART_TypeDef *LPUARTx, uint32_t PinInvMethod) 00726 { 00727 MODIFY_REG(LPUARTx->CR2, USART_CR2_RXINV, PinInvMethod); 00728 } 00729 00730 /** 00731 * @brief Retrieve RX pin active level logic configuration 00732 * @rmtoll CR2 RXINV LL_LPUART_GetRXPinLevel 00733 * @param LPUARTx LPUART Instance 00734 * @retval Returned value can be one of the following values: 00735 * @arg @ref LL_LPUART_RXPIN_LEVEL_STANDARD 00736 * @arg @ref LL_LPUART_RXPIN_LEVEL_INVERTED 00737 */ 00738 __STATIC_INLINE uint32_t LL_LPUART_GetRXPinLevel(USART_TypeDef *LPUARTx) 00739 { 00740 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_RXINV)); 00741 } 00742 00743 /** 00744 * @brief Configure TX pin active level logic 00745 * @rmtoll CR2 TXINV LL_LPUART_SetTXPinLevel 00746 * @param LPUARTx LPUART Instance 00747 * @param PinInvMethod This parameter can be one of the following values: 00748 * @arg @ref LL_LPUART_TXPIN_LEVEL_STANDARD 00749 * @arg @ref LL_LPUART_TXPIN_LEVEL_INVERTED 00750 * @retval None 00751 */ 00752 __STATIC_INLINE void LL_LPUART_SetTXPinLevel(USART_TypeDef *LPUARTx, uint32_t PinInvMethod) 00753 { 00754 MODIFY_REG(LPUARTx->CR2, USART_CR2_TXINV, PinInvMethod); 00755 } 00756 00757 /** 00758 * @brief Retrieve TX pin active level logic configuration 00759 * @rmtoll CR2 TXINV LL_LPUART_GetTXPinLevel 00760 * @param LPUARTx LPUART Instance 00761 * @retval Returned value can be one of the following values: 00762 * @arg @ref LL_LPUART_TXPIN_LEVEL_STANDARD 00763 * @arg @ref LL_LPUART_TXPIN_LEVEL_INVERTED 00764 */ 00765 __STATIC_INLINE uint32_t LL_LPUART_GetTXPinLevel(USART_TypeDef *LPUARTx) 00766 { 00767 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_TXINV)); 00768 } 00769 00770 /** 00771 * @brief Configure Binary data logic. 00772 * 00773 * @note Allow to define how Logical data from the data register are send/received : 00774 * either in positive/direct logic (1=H, 0=L) or in negative/inverse logic (1=L, 0=H) 00775 * @rmtoll CR2 DATAINV LL_LPUART_SetBinaryDataLogic 00776 * @param LPUARTx LPUART Instance 00777 * @param DataLogic This parameter can be one of the following values: 00778 * @arg @ref LL_LPUART_BINARY_LOGIC_POSITIVE 00779 * @arg @ref LL_LPUART_BINARY_LOGIC_NEGATIVE 00780 * @retval None 00781 */ 00782 __STATIC_INLINE void LL_LPUART_SetBinaryDataLogic(USART_TypeDef *LPUARTx, uint32_t DataLogic) 00783 { 00784 MODIFY_REG(LPUARTx->CR2, USART_CR2_DATAINV, DataLogic); 00785 } 00786 00787 /** 00788 * @brief Retrieve Binary data configuration 00789 * @rmtoll CR2 DATAINV LL_LPUART_GetBinaryDataLogic 00790 * @param LPUARTx LPUART Instance 00791 * @retval Returned value can be one of the following values: 00792 * @arg @ref LL_LPUART_BINARY_LOGIC_POSITIVE 00793 * @arg @ref LL_LPUART_BINARY_LOGIC_NEGATIVE 00794 */ 00795 __STATIC_INLINE uint32_t LL_LPUART_GetBinaryDataLogic(USART_TypeDef *LPUARTx) 00796 { 00797 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_DATAINV)); 00798 } 00799 00800 /** 00801 * @brief Configure transfer bit order (either Less or Most Significant Bit First) 00802 * @note MSB First means data is transmitted/received with the MSB first, following the start bit. 00803 * LSB First means data is transmitted/received with data bit 0 first, following the start bit. 00804 * @rmtoll CR2 MSBFIRST LL_LPUART_SetTransferBitOrder 00805 * @param LPUARTx LPUART Instance 00806 * @param BitOrder This parameter can be one of the following values: 00807 * @arg @ref LL_LPUART_BITORDER_LSBFIRST 00808 * @arg @ref LL_LPUART_BITORDER_MSBFIRST 00809 * @retval None 00810 */ 00811 __STATIC_INLINE void LL_LPUART_SetTransferBitOrder(USART_TypeDef *LPUARTx, uint32_t BitOrder) 00812 { 00813 MODIFY_REG(LPUARTx->CR2, USART_CR2_MSBFIRST, BitOrder); 00814 } 00815 00816 /** 00817 * @brief Return transfer bit order (either Less or Most Significant Bit First) 00818 * @note MSB First means data is transmitted/received with the MSB first, following the start bit. 00819 * LSB First means data is transmitted/received with data bit 0 first, following the start bit. 00820 * @rmtoll CR2 MSBFIRST LL_LPUART_GetTransferBitOrder 00821 * @param LPUARTx LPUART Instance 00822 * @retval Returned value can be one of the following values: 00823 * @arg @ref LL_LPUART_BITORDER_LSBFIRST 00824 * @arg @ref LL_LPUART_BITORDER_MSBFIRST 00825 */ 00826 __STATIC_INLINE uint32_t LL_LPUART_GetTransferBitOrder(USART_TypeDef *LPUARTx) 00827 { 00828 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_MSBFIRST)); 00829 } 00830 00831 /** 00832 * @brief Set Address of the LPUART node. 00833 * 00834 * @note This is used in multiprocessor communication during Mute mode or Stop mode, 00835 * for wakeup with address mark detection. 00836 * @note 4bits address node is used when 4-bit Address Detection is selected in ADDM7. 00837 * (b7-b4 should be set to 0) 00838 * 8bits address node is used when 7-bit Address Detection is selected in ADDM7. 00839 * (This is used in multiprocessor communication during Mute mode or Stop mode, 00840 * for wakeup with 7-bit address mark detection. 00841 * The MSB of the character sent by the transmitter should be equal to 1. 00842 * It may also be used for character detection during normal reception, 00843 * Mute mode inactive (for example, end of block detection in ModBus protocol). 00844 * In this case, the whole received character (8-bit) is compared to the ADD[7:0] 00845 * value and CMF flag is set on match) 00846 * @rmtoll CR2 ADD LL_LPUART_ConfigNodeAddress\n 00847 * CR2 ADDM7 LL_LPUART_ConfigNodeAddress 00848 * @param LPUARTx LPUART Instance 00849 * @param AddressLen This parameter can be one of the following values: 00850 * @arg @ref LL_LPUART_ADDRESS_DETECT_4B 00851 * @arg @ref LL_LPUART_ADDRESS_DETECT_7B 00852 * @param NodeAddress 4 or 7 bit Address of the LPUART node. 00853 * @retval None 00854 */ 00855 __STATIC_INLINE void LL_LPUART_ConfigNodeAddress(USART_TypeDef *LPUARTx, uint32_t AddressLen, uint32_t NodeAddress) 00856 { 00857 MODIFY_REG(LPUARTx->CR2, USART_CR2_ADD | USART_CR2_ADDM7, 00858 (uint32_t)(AddressLen | (NodeAddress << LPUART_POSITION_CR2_ADD))); 00859 } 00860 00861 /** 00862 * @brief Return 8 bit Address of the LPUART node as set in ADD field of CR2. 00863 * @note If 4-bit Address Detection is selected in ADDM7, 00864 * only 4bits (b3-b0) of returned value are relevant (b31-b4 are not relevant) 00865 * If 7-bit Address Detection is selected in ADDM7, 00866 * only 8bits (b7-b0) of returned value are relevant (b31-b8 are not relevant) 00867 * @rmtoll CR2 ADD LL_LPUART_GetNodeAddress 00868 * @param LPUARTx LPUART Instance 00869 * @retval Address of the LPUART node (0..255) 00870 */ 00871 __STATIC_INLINE uint32_t LL_LPUART_GetNodeAddress(USART_TypeDef *LPUARTx) 00872 { 00873 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_ADD) >> LPUART_POSITION_CR2_ADD); 00874 } 00875 00876 /** 00877 * @brief Return Length of Node Address used in Address Detection mode (7-bit or 4-bit) 00878 * @rmtoll CR2 ADDM7 LL_LPUART_GetNodeAddressLen 00879 * @param LPUARTx LPUART Instance 00880 * @retval Returned value can be one of the following values: 00881 * @arg @ref LL_LPUART_ADDRESS_DETECT_4B 00882 * @arg @ref LL_LPUART_ADDRESS_DETECT_7B 00883 */ 00884 __STATIC_INLINE uint32_t LL_LPUART_GetNodeAddressLen(USART_TypeDef *LPUARTx) 00885 { 00886 return (uint32_t)(READ_BIT(LPUARTx->CR2, USART_CR2_ADDM7)); 00887 } 00888 00889 /** 00890 * @brief Enable RTS HW Flow Control 00891 * @rmtoll CR3 RTSE LL_LPUART_EnableRTSHWFlowCtrl 00892 * @param LPUARTx LPUART Instance 00893 * @retval None 00894 */ 00895 __STATIC_INLINE void LL_LPUART_EnableRTSHWFlowCtrl(USART_TypeDef *LPUARTx) 00896 { 00897 SET_BIT(LPUARTx->CR3, USART_CR3_RTSE); 00898 } 00899 00900 /** 00901 * @brief Disable RTS HW Flow Control 00902 * @rmtoll CR3 RTSE LL_LPUART_DisableRTSHWFlowCtrl 00903 * @param LPUARTx LPUART Instance 00904 * @retval None 00905 */ 00906 __STATIC_INLINE void LL_LPUART_DisableRTSHWFlowCtrl(USART_TypeDef *LPUARTx) 00907 { 00908 CLEAR_BIT(LPUARTx->CR3, USART_CR3_RTSE); 00909 } 00910 00911 /** 00912 * @brief Enable CTS HW Flow Control 00913 * @rmtoll CR3 CTSE LL_LPUART_EnableCTSHWFlowCtrl 00914 * @param LPUARTx LPUART Instance 00915 * @retval None 00916 */ 00917 __STATIC_INLINE void LL_LPUART_EnableCTSHWFlowCtrl(USART_TypeDef *LPUARTx) 00918 { 00919 SET_BIT(LPUARTx->CR3, USART_CR3_CTSE); 00920 } 00921 00922 /** 00923 * @brief Disable CTS HW Flow Control 00924 * @rmtoll CR3 CTSE LL_LPUART_DisableCTSHWFlowCtrl 00925 * @param LPUARTx LPUART Instance 00926 * @retval None 00927 */ 00928 __STATIC_INLINE void LL_LPUART_DisableCTSHWFlowCtrl(USART_TypeDef *LPUARTx) 00929 { 00930 CLEAR_BIT(LPUARTx->CR3, USART_CR3_CTSE); 00931 } 00932 00933 /** 00934 * @brief Configure HW Flow Control mode (both CTS and RTS) 00935 * @rmtoll CR3 RTSE LL_LPUART_SetHWFlowCtrl\n 00936 * CR3 CTSE LL_LPUART_SetHWFlowCtrl 00937 * @param LPUARTx LPUART Instance 00938 * @param HWFlowCtrlMode This parameter can be one of the following values: 00939 * @arg @ref LL_LPUART_HWCONTROL_NONE 00940 * @arg @ref LL_LPUART_HWCONTROL_RTS 00941 * @arg @ref LL_LPUART_HWCONTROL_CTS 00942 * @arg @ref LL_LPUART_HWCONTROL_RTS_CTS 00943 * @retval None 00944 */ 00945 __STATIC_INLINE void LL_LPUART_SetHWFlowCtrl(USART_TypeDef *LPUARTx, uint32_t HWFlowCtrlMode) 00946 { 00947 MODIFY_REG(LPUARTx->CR3, USART_CR3_RTSE | USART_CR3_CTSE, HWFlowCtrlMode); 00948 } 00949 00950 /** 00951 * @brief Return HW Flow Control configuration (both CTS and RTS) 00952 * @rmtoll CR3 RTSE LL_LPUART_GetHWFlowCtrl\n 00953 * CR3 CTSE LL_LPUART_GetHWFlowCtrl 00954 * @param LPUARTx LPUART Instance 00955 * @retval Returned value can be one of the following values: 00956 * @arg @ref LL_LPUART_HWCONTROL_NONE 00957 * @arg @ref LL_LPUART_HWCONTROL_RTS 00958 * @arg @ref LL_LPUART_HWCONTROL_CTS 00959 * @arg @ref LL_LPUART_HWCONTROL_RTS_CTS 00960 */ 00961 __STATIC_INLINE uint32_t LL_LPUART_GetHWFlowCtrl(USART_TypeDef *LPUARTx) 00962 { 00963 return (uint32_t)(READ_BIT(LPUARTx->CR3, USART_CR3_RTSE | USART_CR3_CTSE)); 00964 } 00965 00966 /** 00967 * @brief Enable Overrun detection 00968 * @rmtoll CR3 OVRDIS LL_LPUART_EnableOverrunDetect 00969 * @param LPUARTx LPUART Instance 00970 * @retval None 00971 */ 00972 __STATIC_INLINE void LL_LPUART_EnableOverrunDetect(USART_TypeDef *LPUARTx) 00973 { 00974 CLEAR_BIT(LPUARTx->CR3, USART_CR3_OVRDIS); 00975 } 00976 00977 /** 00978 * @brief Disable Overrun detection 00979 * @rmtoll CR3 OVRDIS LL_LPUART_DisableOverrunDetect 00980 * @param LPUARTx LPUART Instance 00981 * @retval None 00982 */ 00983 __STATIC_INLINE void LL_LPUART_DisableOverrunDetect(USART_TypeDef *LPUARTx) 00984 { 00985 SET_BIT(LPUARTx->CR3, USART_CR3_OVRDIS); 00986 } 00987 00988 /** 00989 * @brief Indicate if Overrun detection is enabled 00990 * @rmtoll CR3 OVRDIS LL_LPUART_IsEnabledOverrunDetect 00991 * @param LPUARTx LPUART Instance 00992 * @retval State of bit (1 or 0). 00993 */ 00994 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledOverrunDetect(USART_TypeDef *LPUARTx) 00995 { 00996 return (READ_BIT(LPUARTx->CR3, USART_CR3_OVRDIS) != USART_CR3_OVRDIS); 00997 } 00998 00999 /** 01000 * @brief Select event type for Wake UP Interrupt Flag (WUS[1:0] bits) 01001 * @rmtoll CR3 WUS LL_LPUART_SetWKUPType 01002 * @param LPUARTx LPUART Instance 01003 * @param Type This parameter can be one of the following values: 01004 * @arg @ref LL_LPUART_WAKEUP_ON_ADDRESS 01005 * @arg @ref LL_LPUART_WAKEUP_ON_STARTBIT 01006 * @arg @ref LL_LPUART_WAKEUP_ON_RXNE 01007 * @retval None 01008 */ 01009 __STATIC_INLINE void LL_LPUART_SetWKUPType(USART_TypeDef *LPUARTx, uint32_t Type) 01010 { 01011 MODIFY_REG(LPUARTx->CR3, USART_CR3_WUS, Type); 01012 } 01013 01014 /** 01015 * @brief Return event type for Wake UP Interrupt Flag (WUS[1:0] bits) 01016 * @rmtoll CR3 WUS LL_LPUART_GetWKUPType 01017 * @param LPUARTx LPUART Instance 01018 * @retval Returned value can be one of the following values: 01019 * @arg @ref LL_LPUART_WAKEUP_ON_ADDRESS 01020 * @arg @ref LL_LPUART_WAKEUP_ON_STARTBIT 01021 * @arg @ref LL_LPUART_WAKEUP_ON_RXNE 01022 */ 01023 __STATIC_INLINE uint32_t LL_LPUART_GetWKUPType(USART_TypeDef *LPUARTx) 01024 { 01025 return (uint32_t)(READ_BIT(LPUARTx->CR3, USART_CR3_WUS)); 01026 } 01027 01028 /** 01029 * @brief Configure LPUART BRR register for achieving expected Baudrate value. 01030 * 01031 * @note Compute and set LPUARTDIV value in BRR Register (full BRR content) 01032 * according to used Peripheral Clock and expected BaudRate values 01033 * @note Provided that LPUARTx_BRR must be > = 0x300 and LPUART_BRR is 20-bit, 01034 * a care should be taken when generating high baudrates using high PeriphClk 01035 * values. PeriphClk must be in the range [3 x BaudRate, 4096 x BaudRate]. 01036 * @rmtoll BRR BRR LL_LPUART_SetBaudRate 01037 * @param LPUARTx LPUART Instance 01038 * @param PeriphClk Peripheral Clock 01039 * @param BaudRate Baudrate 01040 * @retval None 01041 */ 01042 __STATIC_INLINE void LL_LPUART_SetBaudRate(USART_TypeDef *LPUARTx, uint32_t PeriphClk, uint32_t BaudRate) 01043 { 01044 LPUARTx->BRR = __LL_LPUART_DIV(PeriphClk, BaudRate); 01045 } 01046 01047 /** 01048 * @brief Return current Baudrate value, according to LPUARTDIV present in BRR register 01049 * (full BRR content), and to used Peripheral Clock values 01050 * @rmtoll BRR BRR LL_LPUART_GetBaudRate 01051 * @param LPUARTx LPUART Instance 01052 * @param PeriphClk Peripheral Clock 01053 * @retval BaudRate 01054 */ 01055 __STATIC_INLINE uint32_t LL_LPUART_GetBaudRate(USART_TypeDef *LPUARTx, uint32_t PeriphClk) 01056 { 01057 return (uint32_t)(((uint64_t)(PeriphClk) * LPUART_LPUARTDIV_FREQ_MUL) / LPUARTx->BRR); 01058 } 01059 01060 /** 01061 * @} 01062 */ 01063 01064 /** @defgroup LPUART_LL_EF_Configuration_HalfDuplex Configuration functions related to Half Duplex feature 01065 * @{ 01066 */ 01067 01068 /** 01069 * @brief Enable Single Wire Half-Duplex mode 01070 * @rmtoll CR3 HDSEL LL_LPUART_EnableHalfDuplex 01071 * @param LPUARTx LPUART Instance 01072 * @retval None 01073 */ 01074 __STATIC_INLINE void LL_LPUART_EnableHalfDuplex(USART_TypeDef *LPUARTx) 01075 { 01076 SET_BIT(LPUARTx->CR3, USART_CR3_HDSEL); 01077 } 01078 01079 /** 01080 * @brief Disable Single Wire Half-Duplex mode 01081 * @rmtoll CR3 HDSEL LL_LPUART_DisableHalfDuplex 01082 * @param LPUARTx LPUART Instance 01083 * @retval None 01084 */ 01085 __STATIC_INLINE void LL_LPUART_DisableHalfDuplex(USART_TypeDef *LPUARTx) 01086 { 01087 CLEAR_BIT(LPUARTx->CR3, USART_CR3_HDSEL); 01088 } 01089 01090 /** 01091 * @brief Indicate if Single Wire Half-Duplex mode is enabled 01092 * @rmtoll CR3 HDSEL LL_LPUART_IsEnabledHalfDuplex 01093 * @param LPUARTx LPUART Instance 01094 * @retval State of bit (1 or 0). 01095 */ 01096 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledHalfDuplex(USART_TypeDef *LPUARTx) 01097 { 01098 return (READ_BIT(LPUARTx->CR3, USART_CR3_HDSEL) == (USART_CR3_HDSEL)); 01099 } 01100 01101 /** 01102 * @} 01103 */ 01104 01105 /** @defgroup LPUART_LL_EF_Configuration_DE Configuration functions related to Driver Enable feature 01106 * @{ 01107 */ 01108 01109 /** 01110 * @brief Set DEDT (Driver Enable Deassertion Time), Time value expressed on 5 bits ([4:0] bits). 01111 * @rmtoll CR1 DEDT LL_LPUART_SetDEDeassertionTime 01112 * @param LPUARTx LPUART Instance 01113 * @param Time 0..31 01114 * @retval None 01115 */ 01116 __STATIC_INLINE void LL_LPUART_SetDEDeassertionTime(USART_TypeDef *LPUARTx, uint32_t Time) 01117 { 01118 MODIFY_REG(LPUARTx->CR1, USART_CR1_DEDT, Time << LPUART_POSITION_CR1_DEDT); 01119 } 01120 01121 /** 01122 * @brief Return DEDT (Driver Enable Deassertion Time) 01123 * @rmtoll CR1 DEDT LL_LPUART_GetDEDeassertionTime 01124 * @param LPUARTx LPUART Instance 01125 * @retval Time value expressed on 5 bits ([4:0] bits) : 0..31 01126 */ 01127 __STATIC_INLINE uint32_t LL_LPUART_GetDEDeassertionTime(USART_TypeDef *LPUARTx) 01128 { 01129 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_DEDT) >> LPUART_POSITION_CR1_DEDT); 01130 } 01131 01132 /** 01133 * @brief Set DEAT (Driver Enable Assertion Time), Time value expressed on 5 bits ([4:0] bits). 01134 * @rmtoll CR1 DEAT LL_LPUART_SetDEAssertionTime 01135 * @param LPUARTx LPUART Instance 01136 * @param Time 0..31 01137 * @retval None 01138 */ 01139 __STATIC_INLINE void LL_LPUART_SetDEAssertionTime(USART_TypeDef *LPUARTx, uint32_t Time) 01140 { 01141 MODIFY_REG(LPUARTx->CR1, USART_CR1_DEAT, Time << LPUART_POSITION_CR1_DEAT); 01142 } 01143 01144 /** 01145 * @brief Return DEAT (Driver Enable Assertion Time) 01146 * @rmtoll CR1 DEAT LL_LPUART_GetDEAssertionTime 01147 * @param LPUARTx LPUART Instance 01148 * @retval Time value expressed on 5 bits ([4:0] bits) : 0..31 01149 */ 01150 __STATIC_INLINE uint32_t LL_LPUART_GetDEAssertionTime(USART_TypeDef *LPUARTx) 01151 { 01152 return (uint32_t)(READ_BIT(LPUARTx->CR1, USART_CR1_DEAT) >> LPUART_POSITION_CR1_DEAT); 01153 } 01154 01155 /** 01156 * @brief Enable Driver Enable (DE) Mode 01157 * @rmtoll CR3 DEM LL_LPUART_EnableDEMode 01158 * @param LPUARTx LPUART Instance 01159 * @retval None 01160 */ 01161 __STATIC_INLINE void LL_LPUART_EnableDEMode(USART_TypeDef *LPUARTx) 01162 { 01163 SET_BIT(LPUARTx->CR3, USART_CR3_DEM); 01164 } 01165 01166 /** 01167 * @brief Disable Driver Enable (DE) Mode 01168 * @rmtoll CR3 DEM LL_LPUART_DisableDEMode 01169 * @param LPUARTx LPUART Instance 01170 * @retval None 01171 */ 01172 __STATIC_INLINE void LL_LPUART_DisableDEMode(USART_TypeDef *LPUARTx) 01173 { 01174 CLEAR_BIT(LPUARTx->CR3, USART_CR3_DEM); 01175 } 01176 01177 /** 01178 * @brief Indicate if Driver Enable (DE) Mode is enabled 01179 * @rmtoll CR3 DEM LL_LPUART_IsEnabledDEMode 01180 * @param LPUARTx LPUART Instance 01181 * @retval State of bit (1 or 0). 01182 */ 01183 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledDEMode(USART_TypeDef *LPUARTx) 01184 { 01185 return (READ_BIT(LPUARTx->CR3, USART_CR3_DEM) == (USART_CR3_DEM)); 01186 } 01187 01188 /** 01189 * @brief Select Driver Enable Polarity 01190 * @rmtoll CR3 DEP LL_LPUART_SetDESignalPolarity 01191 * @param LPUARTx LPUART Instance 01192 * @param Polarity This parameter can be one of the following values: 01193 * @arg @ref LL_LPUART_DE_POLARITY_HIGH 01194 * @arg @ref LL_LPUART_DE_POLARITY_LOW 01195 * @retval None 01196 */ 01197 __STATIC_INLINE void LL_LPUART_SetDESignalPolarity(USART_TypeDef *LPUARTx, uint32_t Polarity) 01198 { 01199 MODIFY_REG(LPUARTx->CR3, USART_CR3_DEP, Polarity); 01200 } 01201 01202 /** 01203 * @brief Return Driver Enable Polarity 01204 * @rmtoll CR3 DEP LL_LPUART_GetDESignalPolarity 01205 * @param LPUARTx LPUART Instance 01206 * @retval Returned value can be one of the following values: 01207 * @arg @ref LL_LPUART_DE_POLARITY_HIGH 01208 * @arg @ref LL_LPUART_DE_POLARITY_LOW 01209 */ 01210 __STATIC_INLINE uint32_t LL_LPUART_GetDESignalPolarity(USART_TypeDef *LPUARTx) 01211 { 01212 return (uint32_t)(READ_BIT(LPUARTx->CR3, USART_CR3_DEP)); 01213 } 01214 01215 /** 01216 * @} 01217 */ 01218 01219 /** @defgroup LPUART_LL_EF_FLAG_Management FLAG_Management 01220 * @{ 01221 */ 01222 01223 /** 01224 * @brief Check if the LPUART Parity Error Flag is set or not 01225 * @rmtoll ISR PE LL_LPUART_IsActiveFlag_PE 01226 * @param LPUARTx LPUART Instance 01227 * @retval State of bit (1 or 0). 01228 */ 01229 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_PE(USART_TypeDef *LPUARTx) 01230 { 01231 return (READ_BIT(LPUARTx->ISR, USART_ISR_PE) == (USART_ISR_PE)); 01232 } 01233 01234 /** 01235 * @brief Check if the LPUART Framing Error Flag is set or not 01236 * @rmtoll ISR FE LL_LPUART_IsActiveFlag_FE 01237 * @param LPUARTx LPUART Instance 01238 * @retval State of bit (1 or 0). 01239 */ 01240 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_FE(USART_TypeDef *LPUARTx) 01241 { 01242 return (READ_BIT(LPUARTx->ISR, USART_ISR_FE) == (USART_ISR_FE)); 01243 } 01244 01245 /** 01246 * @brief Check if the LPUART Noise detected Flag is set or not 01247 * @rmtoll ISR NE LL_LPUART_IsActiveFlag_NE 01248 * @param LPUARTx LPUART Instance 01249 * @retval State of bit (1 or 0). 01250 */ 01251 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_NE(USART_TypeDef *LPUARTx) 01252 { 01253 return (READ_BIT(LPUARTx->ISR, USART_ISR_NE) == (USART_ISR_NE)); 01254 } 01255 01256 /** 01257 * @brief Check if the LPUART OverRun Error Flag is set or not 01258 * @rmtoll ISR ORE LL_LPUART_IsActiveFlag_ORE 01259 * @param LPUARTx LPUART Instance 01260 * @retval State of bit (1 or 0). 01261 */ 01262 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_ORE(USART_TypeDef *LPUARTx) 01263 { 01264 return (READ_BIT(LPUARTx->ISR, USART_ISR_ORE) == (USART_ISR_ORE)); 01265 } 01266 01267 /** 01268 * @brief Check if the LPUART IDLE line detected Flag is set or not 01269 * @rmtoll ISR IDLE LL_LPUART_IsActiveFlag_IDLE 01270 * @param LPUARTx LPUART Instance 01271 * @retval State of bit (1 or 0). 01272 */ 01273 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_IDLE(USART_TypeDef *LPUARTx) 01274 { 01275 return (READ_BIT(LPUARTx->ISR, USART_ISR_IDLE) == (USART_ISR_IDLE)); 01276 } 01277 01278 /** 01279 * @brief Check if the LPUART Read Data Register Not Empty Flag is set or not 01280 * @rmtoll ISR RXNE LL_LPUART_IsActiveFlag_RXNE 01281 * @param LPUARTx LPUART Instance 01282 * @retval State of bit (1 or 0). 01283 */ 01284 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_RXNE(USART_TypeDef *LPUARTx) 01285 { 01286 return (READ_BIT(LPUARTx->ISR, USART_ISR_RXNE) == (USART_ISR_RXNE)); 01287 } 01288 01289 /** 01290 * @brief Check if the LPUART Transmission Complete Flag is set or not 01291 * @rmtoll ISR TC LL_LPUART_IsActiveFlag_TC 01292 * @param LPUARTx LPUART Instance 01293 * @retval State of bit (1 or 0). 01294 */ 01295 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_TC(USART_TypeDef *LPUARTx) 01296 { 01297 return (READ_BIT(LPUARTx->ISR, USART_ISR_TC) == (USART_ISR_TC)); 01298 } 01299 01300 /** 01301 * @brief Check if the LPUART Transmit Data Register Empty Flag is set or not 01302 * @rmtoll ISR TXE LL_LPUART_IsActiveFlag_TXE 01303 * @param LPUARTx LPUART Instance 01304 * @retval State of bit (1 or 0). 01305 */ 01306 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_TXE(USART_TypeDef *LPUARTx) 01307 { 01308 return (READ_BIT(LPUARTx->ISR, USART_ISR_TXE) == (USART_ISR_TXE)); 01309 } 01310 01311 /** 01312 * @brief Check if the LPUART CTS interrupt Flag is set or not 01313 * @rmtoll ISR CTSIF LL_LPUART_IsActiveFlag_nCTS 01314 * @param LPUARTx LPUART Instance 01315 * @retval State of bit (1 or 0). 01316 */ 01317 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_nCTS(USART_TypeDef *LPUARTx) 01318 { 01319 return (READ_BIT(LPUARTx->ISR, USART_ISR_CTSIF) == (USART_ISR_CTSIF)); 01320 } 01321 01322 /** 01323 * @brief Check if the LPUART CTS Flag is set or not 01324 * @rmtoll ISR CTS LL_LPUART_IsActiveFlag_CTS 01325 * @param LPUARTx LPUART Instance 01326 * @retval State of bit (1 or 0). 01327 */ 01328 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_CTS(USART_TypeDef *LPUARTx) 01329 { 01330 return (READ_BIT(LPUARTx->ISR, USART_ISR_CTS) == (USART_ISR_CTS)); 01331 } 01332 01333 /** 01334 * @brief Check if the LPUART Busy Flag is set or not 01335 * @rmtoll ISR BUSY LL_LPUART_IsActiveFlag_BUSY 01336 * @param LPUARTx LPUART Instance 01337 * @retval State of bit (1 or 0). 01338 */ 01339 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_BUSY(USART_TypeDef *LPUARTx) 01340 { 01341 return (READ_BIT(LPUARTx->ISR, USART_ISR_BUSY) == (USART_ISR_BUSY)); 01342 } 01343 01344 /** 01345 * @brief Check if the LPUART Character Match Flag is set or not 01346 * @rmtoll ISR CMF LL_LPUART_IsActiveFlag_CM 01347 * @param LPUARTx LPUART Instance 01348 * @retval State of bit (1 or 0). 01349 */ 01350 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_CM(USART_TypeDef *LPUARTx) 01351 { 01352 return (READ_BIT(LPUARTx->ISR, USART_ISR_CMF) == (USART_ISR_CMF)); 01353 } 01354 01355 /** 01356 * @brief Check if the LPUART Send Break Flag is set or not 01357 * @rmtoll ISR SBKF LL_LPUART_IsActiveFlag_SBK 01358 * @param LPUARTx LPUART Instance 01359 * @retval State of bit (1 or 0). 01360 */ 01361 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_SBK(USART_TypeDef *LPUARTx) 01362 { 01363 return (READ_BIT(LPUARTx->ISR, USART_ISR_SBKF) == (USART_ISR_SBKF)); 01364 } 01365 01366 /** 01367 * @brief Check if the LPUART Receive Wake Up from mute mode Flag is set or not 01368 * @rmtoll ISR RWU LL_LPUART_IsActiveFlag_RWU 01369 * @param LPUARTx LPUART Instance 01370 * @retval State of bit (1 or 0). 01371 */ 01372 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_RWU(USART_TypeDef *LPUARTx) 01373 { 01374 return (READ_BIT(LPUARTx->ISR, USART_ISR_RWU) == (USART_ISR_RWU)); 01375 } 01376 01377 /** 01378 * @brief Check if the LPUART Wake Up from stop mode Flag is set or not 01379 * @rmtoll ISR WUF LL_LPUART_IsActiveFlag_WKUP 01380 * @param LPUARTx LPUART Instance 01381 * @retval State of bit (1 or 0). 01382 */ 01383 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_WKUP(USART_TypeDef *LPUARTx) 01384 { 01385 return (READ_BIT(LPUARTx->ISR, USART_ISR_WUF) == (USART_ISR_WUF)); 01386 } 01387 01388 /** 01389 * @brief Check if the LPUART Transmit Enable Acknowledge Flag is set or not 01390 * @rmtoll ISR TEACK LL_LPUART_IsActiveFlag_TEACK 01391 * @param LPUARTx LPUART Instance 01392 * @retval State of bit (1 or 0). 01393 */ 01394 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_TEACK(USART_TypeDef *LPUARTx) 01395 { 01396 return (READ_BIT(LPUARTx->ISR, USART_ISR_TEACK) == (USART_ISR_TEACK)); 01397 } 01398 01399 /** 01400 * @brief Check if the LPUART Receive Enable Acknowledge Flag is set or not 01401 * @rmtoll ISR REACK LL_LPUART_IsActiveFlag_REACK 01402 * @param LPUARTx LPUART Instance 01403 * @retval State of bit (1 or 0). 01404 */ 01405 __STATIC_INLINE uint32_t LL_LPUART_IsActiveFlag_REACK(USART_TypeDef *LPUARTx) 01406 { 01407 return (READ_BIT(LPUARTx->ISR, USART_ISR_REACK) == (USART_ISR_REACK)); 01408 } 01409 01410 /** 01411 * @brief Clear Parity Error Flag 01412 * @rmtoll ICR PECF LL_LPUART_ClearFlag_PE 01413 * @param LPUARTx LPUART Instance 01414 * @retval None 01415 */ 01416 __STATIC_INLINE void LL_LPUART_ClearFlag_PE(USART_TypeDef *LPUARTx) 01417 { 01418 WRITE_REG(LPUARTx->ICR, USART_ICR_PECF); 01419 } 01420 01421 /** 01422 * @brief Clear Framing Error Flag 01423 * @rmtoll ICR FECF LL_LPUART_ClearFlag_FE 01424 * @param LPUARTx LPUART Instance 01425 * @retval None 01426 */ 01427 __STATIC_INLINE void LL_LPUART_ClearFlag_FE(USART_TypeDef *LPUARTx) 01428 { 01429 WRITE_REG(LPUARTx->ICR, USART_ICR_FECF); 01430 } 01431 01432 /** 01433 * @brief Clear Noise detected Flag 01434 * @rmtoll ICR NCF LL_LPUART_ClearFlag_NE 01435 * @param LPUARTx LPUART Instance 01436 * @retval None 01437 */ 01438 __STATIC_INLINE void LL_LPUART_ClearFlag_NE(USART_TypeDef *LPUARTx) 01439 { 01440 WRITE_REG(LPUARTx->ICR, USART_ICR_NCF); 01441 } 01442 01443 /** 01444 * @brief Clear OverRun Error Flag 01445 * @rmtoll ICR ORECF LL_LPUART_ClearFlag_ORE 01446 * @param LPUARTx LPUART Instance 01447 * @retval None 01448 */ 01449 __STATIC_INLINE void LL_LPUART_ClearFlag_ORE(USART_TypeDef *LPUARTx) 01450 { 01451 WRITE_REG(LPUARTx->ICR, USART_ICR_ORECF); 01452 } 01453 01454 /** 01455 * @brief Clear IDLE line detected Flag 01456 * @rmtoll ICR IDLECF LL_LPUART_ClearFlag_IDLE 01457 * @param LPUARTx LPUART Instance 01458 * @retval None 01459 */ 01460 __STATIC_INLINE void LL_LPUART_ClearFlag_IDLE(USART_TypeDef *LPUARTx) 01461 { 01462 WRITE_REG(LPUARTx->ICR, USART_ICR_IDLECF); 01463 } 01464 01465 /** 01466 * @brief Clear Transmission Complete Flag 01467 * @rmtoll ICR TCCF LL_LPUART_ClearFlag_TC 01468 * @param LPUARTx LPUART Instance 01469 * @retval None 01470 */ 01471 __STATIC_INLINE void LL_LPUART_ClearFlag_TC(USART_TypeDef *LPUARTx) 01472 { 01473 WRITE_REG(LPUARTx->ICR, USART_ICR_TCCF); 01474 } 01475 01476 /** 01477 * @brief Clear CTS Interrupt Flag 01478 * @rmtoll ICR CTSCF LL_LPUART_ClearFlag_nCTS 01479 * @param LPUARTx LPUART Instance 01480 * @retval None 01481 */ 01482 __STATIC_INLINE void LL_LPUART_ClearFlag_nCTS(USART_TypeDef *LPUARTx) 01483 { 01484 WRITE_REG(LPUARTx->ICR, USART_ICR_CTSCF); 01485 } 01486 01487 /** 01488 * @brief Clear Character Match Flag 01489 * @rmtoll ICR CMCF LL_LPUART_ClearFlag_CM 01490 * @param LPUARTx LPUART Instance 01491 * @retval None 01492 */ 01493 __STATIC_INLINE void LL_LPUART_ClearFlag_CM(USART_TypeDef *LPUARTx) 01494 { 01495 WRITE_REG(LPUARTx->ICR, USART_ICR_CMCF); 01496 } 01497 01498 /** 01499 * @brief Clear Wake Up from stop mode Flag 01500 * @rmtoll ICR WUCF LL_LPUART_ClearFlag_WKUP 01501 * @param LPUARTx LPUART Instance 01502 * @retval None 01503 */ 01504 __STATIC_INLINE void LL_LPUART_ClearFlag_WKUP(USART_TypeDef *LPUARTx) 01505 { 01506 WRITE_REG(LPUARTx->ICR, USART_ICR_WUCF); 01507 } 01508 01509 /** 01510 * @} 01511 */ 01512 01513 /** @defgroup LPUART_LL_EF_IT_Management IT_Management 01514 * @{ 01515 */ 01516 01517 /** 01518 * @brief Enable IDLE Interrupt 01519 * @rmtoll CR1 IDLEIE LL_LPUART_EnableIT_IDLE 01520 * @param LPUARTx LPUART Instance 01521 * @retval None 01522 */ 01523 __STATIC_INLINE void LL_LPUART_EnableIT_IDLE(USART_TypeDef *LPUARTx) 01524 { 01525 SET_BIT(LPUARTx->CR1, USART_CR1_IDLEIE); 01526 } 01527 01528 /** 01529 * @brief Enable RX Not Empty Interrupt 01530 * @rmtoll CR1 RXNEIE LL_LPUART_EnableIT_RXNE 01531 * @param LPUARTx LPUART Instance 01532 * @retval None 01533 */ 01534 __STATIC_INLINE void LL_LPUART_EnableIT_RXNE(USART_TypeDef *LPUARTx) 01535 { 01536 SET_BIT(LPUARTx->CR1, USART_CR1_RXNEIE); 01537 } 01538 01539 /** 01540 * @brief Enable Transmission Complete Interrupt 01541 * @rmtoll CR1 TCIE LL_LPUART_EnableIT_TC 01542 * @param LPUARTx LPUART Instance 01543 * @retval None 01544 */ 01545 __STATIC_INLINE void LL_LPUART_EnableIT_TC(USART_TypeDef *LPUARTx) 01546 { 01547 SET_BIT(LPUARTx->CR1, USART_CR1_TCIE); 01548 } 01549 01550 /** 01551 * @brief Enable TX Empty Interrupt 01552 * @rmtoll CR1 TXEIE LL_LPUART_EnableIT_TXE 01553 * @param LPUARTx LPUART Instance 01554 * @retval None 01555 */ 01556 __STATIC_INLINE void LL_LPUART_EnableIT_TXE(USART_TypeDef *LPUARTx) 01557 { 01558 SET_BIT(LPUARTx->CR1, USART_CR1_TXEIE); 01559 } 01560 01561 /** 01562 * @brief Enable Parity Error Interrupt 01563 * @rmtoll CR1 PEIE LL_LPUART_EnableIT_PE 01564 * @param LPUARTx LPUART Instance 01565 * @retval None 01566 */ 01567 __STATIC_INLINE void LL_LPUART_EnableIT_PE(USART_TypeDef *LPUARTx) 01568 { 01569 SET_BIT(LPUARTx->CR1, USART_CR1_PEIE); 01570 } 01571 01572 /** 01573 * @brief Enable Character Match Interrupt 01574 * @rmtoll CR1 CMIE LL_LPUART_EnableIT_CM 01575 * @param LPUARTx LPUART Instance 01576 * @retval None 01577 */ 01578 __STATIC_INLINE void LL_LPUART_EnableIT_CM(USART_TypeDef *LPUARTx) 01579 { 01580 SET_BIT(LPUARTx->CR1, USART_CR1_CMIE); 01581 } 01582 01583 /** 01584 * @brief Enable Error Interrupt 01585 * @note When set, Error Interrupt Enable Bit is enabling interrupt generation in case of a framing 01586 * error, overrun error or noise flag (FE=1 or ORE=1 or NF=1 in the LPUARTx_ISR register). 01587 * 0: Interrupt is inhibited 01588 * 1: An interrupt is generated when FE=1 or ORE=1 or NF=1 in the LPUARTx_ISR register. 01589 * @rmtoll CR3 EIE LL_LPUART_EnableIT_ERROR 01590 * @param LPUARTx LPUART Instance 01591 * @retval None 01592 */ 01593 __STATIC_INLINE void LL_LPUART_EnableIT_ERROR(USART_TypeDef *LPUARTx) 01594 { 01595 SET_BIT(LPUARTx->CR3, USART_CR3_EIE); 01596 } 01597 01598 /** 01599 * @brief Enable CTS Interrupt 01600 * @rmtoll CR3 CTSIE LL_LPUART_EnableIT_CTS 01601 * @param LPUARTx LPUART Instance 01602 * @retval None 01603 */ 01604 __STATIC_INLINE void LL_LPUART_EnableIT_CTS(USART_TypeDef *LPUARTx) 01605 { 01606 SET_BIT(LPUARTx->CR3, USART_CR3_CTSIE); 01607 } 01608 01609 /** 01610 * @brief Enable WakeUp from Stop Mode Interrupt 01611 * @rmtoll CR3 WUFIE LL_LPUART_EnableIT_WKUP 01612 * @param LPUARTx LPUART Instance 01613 * @retval None 01614 */ 01615 __STATIC_INLINE void LL_LPUART_EnableIT_WKUP(USART_TypeDef *LPUARTx) 01616 { 01617 SET_BIT(LPUARTx->CR3, USART_CR3_WUFIE); 01618 } 01619 01620 /** 01621 * @brief Disable IDLE Interrupt 01622 * @rmtoll CR1 IDLEIE LL_LPUART_DisableIT_IDLE 01623 * @param LPUARTx LPUART Instance 01624 * @retval None 01625 */ 01626 __STATIC_INLINE void LL_LPUART_DisableIT_IDLE(USART_TypeDef *LPUARTx) 01627 { 01628 CLEAR_BIT(LPUARTx->CR1, USART_CR1_IDLEIE); 01629 } 01630 01631 /** 01632 * @brief Disable RX Not Empty Interrupt 01633 * @rmtoll CR1 RXNEIE LL_LPUART_DisableIT_RXNE 01634 * @param LPUARTx LPUART Instance 01635 * @retval None 01636 */ 01637 __STATIC_INLINE void LL_LPUART_DisableIT_RXNE(USART_TypeDef *LPUARTx) 01638 { 01639 CLEAR_BIT(LPUARTx->CR1, USART_CR1_RXNEIE); 01640 } 01641 01642 /** 01643 * @brief Disable Transmission Complete Interrupt 01644 * @rmtoll CR1 TCIE LL_LPUART_DisableIT_TC 01645 * @param LPUARTx LPUART Instance 01646 * @retval None 01647 */ 01648 __STATIC_INLINE void LL_LPUART_DisableIT_TC(USART_TypeDef *LPUARTx) 01649 { 01650 CLEAR_BIT(LPUARTx->CR1, USART_CR1_TCIE); 01651 } 01652 01653 /** 01654 * @brief Disable TX Empty Interrupt 01655 * @rmtoll CR1 TXEIE LL_LPUART_DisableIT_TXE 01656 * @param LPUARTx LPUART Instance 01657 * @retval None 01658 */ 01659 __STATIC_INLINE void LL_LPUART_DisableIT_TXE(USART_TypeDef *LPUARTx) 01660 { 01661 CLEAR_BIT(LPUARTx->CR1, USART_CR1_TXEIE); 01662 } 01663 01664 /** 01665 * @brief Disable Parity Error Interrupt 01666 * @rmtoll CR1 PEIE LL_LPUART_DisableIT_PE 01667 * @param LPUARTx LPUART Instance 01668 * @retval None 01669 */ 01670 __STATIC_INLINE void LL_LPUART_DisableIT_PE(USART_TypeDef *LPUARTx) 01671 { 01672 CLEAR_BIT(LPUARTx->CR1, USART_CR1_PEIE); 01673 } 01674 01675 /** 01676 * @brief Disable Character Match Interrupt 01677 * @rmtoll CR1 CMIE LL_LPUART_DisableIT_CM 01678 * @param LPUARTx LPUART Instance 01679 * @retval None 01680 */ 01681 __STATIC_INLINE void LL_LPUART_DisableIT_CM(USART_TypeDef *LPUARTx) 01682 { 01683 CLEAR_BIT(LPUARTx->CR1, USART_CR1_CMIE); 01684 } 01685 01686 /** 01687 * @brief Disable Error Interrupt 01688 * @note When set, Error Interrupt Enable Bit is enabling interrupt generation in case of a framing 01689 * error, overrun error or noise flag (FE=1 or ORE=1 or NF=1 in the LPUARTx_ISR register). 01690 * 0: Interrupt is inhibited 01691 * 1: An interrupt is generated when FE=1 or ORE=1 or NF=1 in the LPUARTx_ISR register. 01692 * @rmtoll CR3 EIE LL_LPUART_DisableIT_ERROR 01693 * @param LPUARTx LPUART Instance 01694 * @retval None 01695 */ 01696 __STATIC_INLINE void LL_LPUART_DisableIT_ERROR(USART_TypeDef *LPUARTx) 01697 { 01698 CLEAR_BIT(LPUARTx->CR3, USART_CR3_EIE); 01699 } 01700 01701 /** 01702 * @brief Disable CTS Interrupt 01703 * @rmtoll CR3 CTSIE LL_LPUART_DisableIT_CTS 01704 * @param LPUARTx LPUART Instance 01705 * @retval None 01706 */ 01707 __STATIC_INLINE void LL_LPUART_DisableIT_CTS(USART_TypeDef *LPUARTx) 01708 { 01709 CLEAR_BIT(LPUARTx->CR3, USART_CR3_CTSIE); 01710 } 01711 01712 /** 01713 * @brief Disable WakeUp from Stop Mode Interrupt 01714 * @rmtoll CR3 WUFIE LL_LPUART_DisableIT_WKUP 01715 * @param LPUARTx LPUART Instance 01716 * @retval None 01717 */ 01718 __STATIC_INLINE void LL_LPUART_DisableIT_WKUP(USART_TypeDef *LPUARTx) 01719 { 01720 CLEAR_BIT(LPUARTx->CR3, USART_CR3_WUFIE); 01721 } 01722 01723 /** 01724 * @brief Check if the LPUART IDLE Interrupt source is enabled or disabled. 01725 * @rmtoll CR1 IDLEIE LL_LPUART_IsEnabledIT_IDLE 01726 * @param LPUARTx LPUART Instance 01727 * @retval State of bit (1 or 0). 01728 */ 01729 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_IDLE(USART_TypeDef *LPUARTx) 01730 { 01731 return (READ_BIT(LPUARTx->CR1, USART_CR1_IDLEIE) == (USART_CR1_IDLEIE)); 01732 } 01733 01734 /** 01735 * @brief Check if the LPUART RX Not Empty Interrupt is enabled or disabled. 01736 * @rmtoll CR1 RXNEIE LL_LPUART_IsEnabledIT_RXNE 01737 * @param LPUARTx LPUART Instance 01738 * @retval State of bit (1 or 0). 01739 */ 01740 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_RXNE(USART_TypeDef *LPUARTx) 01741 { 01742 return (READ_BIT(LPUARTx->CR1, USART_CR1_RXNEIE) == (USART_CR1_RXNEIE)); 01743 } 01744 01745 /** 01746 * @brief Check if the LPUART Transmission Complete Interrupt is enabled or disabled. 01747 * @rmtoll CR1 TCIE LL_LPUART_IsEnabledIT_TC 01748 * @param LPUARTx LPUART Instance 01749 * @retval State of bit (1 or 0). 01750 */ 01751 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_TC(USART_TypeDef *LPUARTx) 01752 { 01753 return (READ_BIT(LPUARTx->CR1, USART_CR1_TCIE) == (USART_CR1_TCIE)); 01754 } 01755 01756 /** 01757 * @brief Check if the LPUART TX Empty Interrupt is enabled or disabled. 01758 * @rmtoll CR1 TXEIE LL_LPUART_IsEnabledIT_TXE 01759 * @param LPUARTx LPUART Instance 01760 * @retval State of bit (1 or 0). 01761 */ 01762 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_TXE(USART_TypeDef *LPUARTx) 01763 { 01764 return (READ_BIT(LPUARTx->CR1, USART_CR1_TXEIE) == (USART_CR1_TXEIE)); 01765 } 01766 01767 /** 01768 * @brief Check if the LPUART Parity Error Interrupt is enabled or disabled. 01769 * @rmtoll CR1 PEIE LL_LPUART_IsEnabledIT_PE 01770 * @param LPUARTx LPUART Instance 01771 * @retval State of bit (1 or 0). 01772 */ 01773 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_PE(USART_TypeDef *LPUARTx) 01774 { 01775 return (READ_BIT(LPUARTx->CR1, USART_CR1_PEIE) == (USART_CR1_PEIE)); 01776 } 01777 01778 /** 01779 * @brief Check if the LPUART Character Match Interrupt is enabled or disabled. 01780 * @rmtoll CR1 CMIE LL_LPUART_IsEnabledIT_CM 01781 * @param LPUARTx LPUART Instance 01782 * @retval State of bit (1 or 0). 01783 */ 01784 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_CM(USART_TypeDef *LPUARTx) 01785 { 01786 return (READ_BIT(LPUARTx->CR1, USART_CR1_CMIE) == (USART_CR1_CMIE)); 01787 } 01788 01789 /** 01790 * @brief Check if the LPUART Error Interrupt is enabled or disabled. 01791 * @rmtoll CR3 EIE LL_LPUART_IsEnabledIT_ERROR 01792 * @param LPUARTx LPUART Instance 01793 * @retval State of bit (1 or 0). 01794 */ 01795 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_ERROR(USART_TypeDef *LPUARTx) 01796 { 01797 return (READ_BIT(LPUARTx->CR3, USART_CR3_EIE) == (USART_CR3_EIE)); 01798 } 01799 01800 /** 01801 * @brief Check if the LPUART CTS Interrupt is enabled or disabled. 01802 * @rmtoll CR3 CTSIE LL_LPUART_IsEnabledIT_CTS 01803 * @param LPUARTx LPUART Instance 01804 * @retval State of bit (1 or 0). 01805 */ 01806 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_CTS(USART_TypeDef *LPUARTx) 01807 { 01808 return (READ_BIT(LPUARTx->CR3, USART_CR3_CTSIE) == (USART_CR3_CTSIE)); 01809 } 01810 01811 /** 01812 * @brief Check if the LPUART WakeUp from Stop Mode Interrupt is enabled or disabled. 01813 * @rmtoll CR3 WUFIE LL_LPUART_IsEnabledIT_WKUP 01814 * @param LPUARTx LPUART Instance 01815 * @retval State of bit (1 or 0). 01816 */ 01817 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledIT_WKUP(USART_TypeDef *LPUARTx) 01818 { 01819 return (READ_BIT(LPUARTx->CR3, USART_CR3_WUFIE) == (USART_CR3_WUFIE)); 01820 } 01821 01822 /** 01823 * @} 01824 */ 01825 01826 /** @defgroup LPUART_LL_EF_DMA_Management DMA_Management 01827 * @{ 01828 */ 01829 01830 /** 01831 * @brief Enable DMA Mode for reception 01832 * @rmtoll CR3 DMAR LL_LPUART_EnableDMAReq_RX 01833 * @param LPUARTx LPUART Instance 01834 * @retval None 01835 */ 01836 __STATIC_INLINE void LL_LPUART_EnableDMAReq_RX(USART_TypeDef *LPUARTx) 01837 { 01838 SET_BIT(LPUARTx->CR3, USART_CR3_DMAR); 01839 } 01840 01841 /** 01842 * @brief Disable DMA Mode for reception 01843 * @rmtoll CR3 DMAR LL_LPUART_DisableDMAReq_RX 01844 * @param LPUARTx LPUART Instance 01845 * @retval None 01846 */ 01847 __STATIC_INLINE void LL_LPUART_DisableDMAReq_RX(USART_TypeDef *LPUARTx) 01848 { 01849 CLEAR_BIT(LPUARTx->CR3, USART_CR3_DMAR); 01850 } 01851 01852 /** 01853 * @brief Check if DMA Mode is enabled for reception 01854 * @rmtoll CR3 DMAR LL_LPUART_IsEnabledDMAReq_RX 01855 * @param LPUARTx LPUART Instance 01856 * @retval State of bit (1 or 0). 01857 */ 01858 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledDMAReq_RX(USART_TypeDef *LPUARTx) 01859 { 01860 return (READ_BIT(LPUARTx->CR3, USART_CR3_DMAR) == (USART_CR3_DMAR)); 01861 } 01862 01863 /** 01864 * @brief Enable DMA Mode for transmission 01865 * @rmtoll CR3 DMAT LL_LPUART_EnableDMAReq_TX 01866 * @param LPUARTx LPUART Instance 01867 * @retval None 01868 */ 01869 __STATIC_INLINE void LL_LPUART_EnableDMAReq_TX(USART_TypeDef *LPUARTx) 01870 { 01871 SET_BIT(LPUARTx->CR3, USART_CR3_DMAT); 01872 } 01873 01874 /** 01875 * @brief Disable DMA Mode for transmission 01876 * @rmtoll CR3 DMAT LL_LPUART_DisableDMAReq_TX 01877 * @param LPUARTx LPUART Instance 01878 * @retval None 01879 */ 01880 __STATIC_INLINE void LL_LPUART_DisableDMAReq_TX(USART_TypeDef *LPUARTx) 01881 { 01882 CLEAR_BIT(LPUARTx->CR3, USART_CR3_DMAT); 01883 } 01884 01885 /** 01886 * @brief Check if DMA Mode is enabled for transmission 01887 * @rmtoll CR3 DMAT LL_LPUART_IsEnabledDMAReq_TX 01888 * @param LPUARTx LPUART Instance 01889 * @retval State of bit (1 or 0). 01890 */ 01891 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledDMAReq_TX(USART_TypeDef *LPUARTx) 01892 { 01893 return (READ_BIT(LPUARTx->CR3, USART_CR3_DMAT) == (USART_CR3_DMAT)); 01894 } 01895 01896 /** 01897 * @brief Enable DMA Disabling on Reception Error 01898 * @rmtoll CR3 DDRE LL_LPUART_EnableDMADeactOnRxErr 01899 * @param LPUARTx LPUART Instance 01900 * @retval None 01901 */ 01902 __STATIC_INLINE void LL_LPUART_EnableDMADeactOnRxErr(USART_TypeDef *LPUARTx) 01903 { 01904 SET_BIT(LPUARTx->CR3, USART_CR3_DDRE); 01905 } 01906 01907 /** 01908 * @brief Disable DMA Disabling on Reception Error 01909 * @rmtoll CR3 DDRE LL_LPUART_DisableDMADeactOnRxErr 01910 * @param LPUARTx LPUART Instance 01911 * @retval None 01912 */ 01913 __STATIC_INLINE void LL_LPUART_DisableDMADeactOnRxErr(USART_TypeDef *LPUARTx) 01914 { 01915 CLEAR_BIT(LPUARTx->CR3, USART_CR3_DDRE); 01916 } 01917 01918 /** 01919 * @brief Indicate if DMA Disabling on Reception Error is disabled 01920 * @rmtoll CR3 DDRE LL_LPUART_IsEnabledDMADeactOnRxErr 01921 * @param LPUARTx LPUART Instance 01922 * @retval State of bit (1 or 0). 01923 */ 01924 __STATIC_INLINE uint32_t LL_LPUART_IsEnabledDMADeactOnRxErr(USART_TypeDef *LPUARTx) 01925 { 01926 return (READ_BIT(LPUARTx->CR3, USART_CR3_DDRE) == (USART_CR3_DDRE)); 01927 } 01928 01929 /** 01930 * @brief Get the LPUART data register address used for DMA transfer 01931 * @rmtoll RDR RDR LL_LPUART_DMA_GetRegAddr\n 01932 * @rmtoll TDR TDR LL_LPUART_DMA_GetRegAddr 01933 * @param LPUARTx LPUART Instance 01934 * @param Direction This parameter can be one of the following values: 01935 * @arg @ref LL_LPUART_DMA_REG_DATA_TRANSMIT 01936 * @arg @ref LL_LPUART_DMA_REG_DATA_RECEIVE 01937 * @retval Address of data register 01938 */ 01939 __STATIC_INLINE uint32_t LL_LPUART_DMA_GetRegAddr(USART_TypeDef *LPUARTx, uint32_t Direction) 01940 { 01941 register uint32_t data_reg_addr = 0; 01942 01943 if (Direction == LL_LPUART_DMA_REG_DATA_TRANSMIT) 01944 { 01945 /* return address of TDR register */ 01946 data_reg_addr = (uint32_t)&(LPUARTx->TDR); 01947 } 01948 else 01949 { 01950 /* return address of RDR register */ 01951 data_reg_addr = (uint32_t)&(LPUARTx->RDR); 01952 } 01953 01954 return data_reg_addr; 01955 } 01956 01957 /** 01958 * @} 01959 */ 01960 01961 /** @defgroup LPUART_LL_EF_Data_Management Data_Management 01962 * @{ 01963 */ 01964 01965 /** 01966 * @brief Read Receiver Data register (Receive Data value, 8 bits) 01967 * @rmtoll RDR RDR LL_LPUART_ReceiveData8 01968 * @param LPUARTx LPUART Instance 01969 * @retval 0..0xFF 01970 */ 01971 __STATIC_INLINE uint8_t LL_LPUART_ReceiveData8(USART_TypeDef *LPUARTx) 01972 { 01973 return (uint8_t)(READ_BIT(LPUARTx->RDR, USART_RDR_RDR)); 01974 } 01975 01976 /** 01977 * @brief Read Receiver Data register (Receive Data value, 9 bits) 01978 * @rmtoll RDR RDR LL_LPUART_ReceiveData9 01979 * @param LPUARTx LPUART Instance 01980 * @retval 0..0x1FF 01981 */ 01982 __STATIC_INLINE uint16_t LL_LPUART_ReceiveData9(USART_TypeDef *LPUARTx) 01983 { 01984 return (uint16_t)(READ_BIT(LPUARTx->RDR, USART_RDR_RDR)); 01985 } 01986 01987 /** 01988 * @brief Write in Transmitter Data Register (Transmit Data value, 8 bits) 01989 * @rmtoll TDR TDR LL_LPUART_TransmitData8 01990 * @param LPUARTx LPUART Instance 01991 * @param Value 0..0xFF 01992 * @retval None 01993 */ 01994 __STATIC_INLINE void LL_LPUART_TransmitData8(USART_TypeDef *LPUARTx, uint8_t Value) 01995 { 01996 LPUARTx->TDR = Value; 01997 } 01998 01999 /** 02000 * @brief Write in Transmitter Data Register (Transmit Data value, 9 bits) 02001 * @rmtoll TDR TDR LL_LPUART_TransmitData9 02002 * @param LPUARTx LPUART Instance 02003 * @param Value 0..0x1FF 02004 * @retval None 02005 */ 02006 __STATIC_INLINE void LL_LPUART_TransmitData9(USART_TypeDef *LPUARTx, uint16_t Value) 02007 { 02008 LPUARTx->TDR = Value & 0x1FF; 02009 } 02010 02011 /** 02012 * @} 02013 */ 02014 02015 /** @defgroup LPUART_LL_EF_Execution Execution 02016 * @{ 02017 */ 02018 02019 /** 02020 * @brief Request Break sending 02021 * @rmtoll RQR SBKRQ LL_LPUART_RequestBreakSending 02022 * @param LPUARTx LPUART Instance 02023 * @retval None 02024 */ 02025 __STATIC_INLINE void LL_LPUART_RequestBreakSending(USART_TypeDef *LPUARTx) 02026 { 02027 SET_BIT(LPUARTx->RQR, USART_RQR_SBKRQ); 02028 } 02029 02030 /** 02031 * @brief Put LPUART in mute mode and set the RWU flag 02032 * @rmtoll RQR MMRQ LL_LPUART_RequestEnterMuteMode 02033 * @param LPUARTx LPUART Instance 02034 * @retval None 02035 */ 02036 __STATIC_INLINE void LL_LPUART_RequestEnterMuteMode(USART_TypeDef *LPUARTx) 02037 { 02038 SET_BIT(LPUARTx->RQR, USART_RQR_MMRQ); 02039 } 02040 02041 /** 02042 * @brief Request a Receive Data flush 02043 * @rmtoll RQR RXFRQ LL_LPUART_RequestRxDataFlush 02044 * @param LPUARTx LPUART Instance 02045 * @retval None 02046 */ 02047 __STATIC_INLINE void LL_LPUART_RequestRxDataFlush(USART_TypeDef *LPUARTx) 02048 { 02049 SET_BIT(LPUARTx->RQR, USART_RQR_RXFRQ); 02050 } 02051 02052 /** 02053 * @} 02054 */ 02055 02056 02057 /** 02058 * @} 02059 */ 02060 02061 /** 02062 * @} 02063 */ 02064 02065 #endif /* LPUART1 */ 02066 02067 /** 02068 * @} 02069 */ 02070 02071 #ifdef __cplusplus 02072 } 02073 #endif 02074 02075 #endif /* __STM32L4xx_LL_LPUART_H */ 02076 02077 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 02078
Generated on Tue Jul 12 2022 10:58:11 by
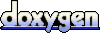