L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_tim_ex.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_tim_ex.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief TIM HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Timer Extended peripheral: 00010 * + Time Hall Sensor Interface Initialization 00011 * + Time Hall Sensor Interface Start 00012 * + Time Complementary signal break and dead time configuration 00013 * + Time Master and Slave synchronization configuration 00014 * + Time Output Compare/PWM Channel Configuration (for channels 5 and 6) 00015 * + Time OCRef clear configuration 00016 * + Timer remapping capabilities configuration 00017 @verbatim 00018 ============================================================================== 00019 ##### TIMER Extended features ##### 00020 ============================================================================== 00021 [..] 00022 The Timer Extended features include: 00023 (#) Complementary outputs with programmable dead-time for : 00024 (++) Output Compare 00025 (++) PWM generation (Edge and Center-aligned Mode) 00026 (++) One-pulse mode output 00027 (#) Synchronization circuit to control the timer with external signals and to 00028 interconnect several timers together. 00029 (#) Break input to put the timer output signals in reset state or in a known state. 00030 (#) Supports incremental (quadrature) encoder and hall-sensor circuitry for 00031 positioning purposes 00032 00033 ##### How to use this driver ##### 00034 ============================================================================== 00035 [..] 00036 (#) Initialize the TIM low level resources by implementing the following functions 00037 depending on the selected feature: 00038 (++) Hall Sensor output : HAL_TIMEx_HallSensor_MspInit() 00039 00040 (#) Initialize the TIM low level resources : 00041 (##) Enable the TIM interface clock using __HAL_RCC_TIMx_CLK_ENABLE(); 00042 (##) TIM pins configuration 00043 (+++) Enable the clock for the TIM GPIOs using the following function: 00044 __HAL_RCC_GPIOx_CLK_ENABLE(); 00045 (+++) Configure these TIM pins in Alternate function mode using HAL_GPIO_Init(); 00046 00047 (#) The external Clock can be configured, if needed (the default clock is the 00048 internal clock from the APBx), using the following function: 00049 HAL_TIM_ConfigClockSource, the clock configuration should be done before 00050 any start function. 00051 00052 (#) Configure the TIM in the desired functioning mode using one of the 00053 initialization function of this driver: 00054 (++) HAL_TIMEx_HallSensor_Init() and HAL_TIMEx_ConfigCommutationEvent(): to use the 00055 Timer Hall Sensor Interface and the commutation event with the corresponding 00056 Interrupt and DMA request if needed (Note that One Timer is used to interface 00057 with the Hall sensor Interface and another Timer should be used to use 00058 the commutation event). 00059 00060 (#) Activate the TIM peripheral using one of the start functions: 00061 (++) Complementary Output Compare : HAL_TIMEx_OCN_Start(), HAL_TIMEx_OCN_Start_DMA(), HAL_TIMEx_OC_Start_IT() 00062 (++) Complementary PWM generation : HAL_TIMEx_PWMN_Start(), HAL_TIMEx_PWMN_Start_DMA(), HAL_TIMEx_PWMN_Start_IT() 00063 (++) Complementary One-pulse mode output : HAL_TIMEx_OnePulseN_Start(), HAL_TIMEx_OnePulseN_Start_IT() 00064 (++) Hall Sensor output : HAL_TIMEx_HallSensor_Start(), HAL_TIMEx_HallSensor_Start_DMA(), HAL_TIMEx_HallSensor_Start_IT(). 00065 00066 00067 @endverbatim 00068 ****************************************************************************** 00069 * @attention 00070 * 00071 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00072 * 00073 * Redistribution and use in source and binary forms, with or without modification, 00074 * are permitted provided that the following conditions are met: 00075 * 1. Redistributions of source code must retain the above copyright notice, 00076 * this list of conditions and the following disclaimer. 00077 * 2. Redistributions in binary form must reproduce the above copyright notice, 00078 * this list of conditions and the following disclaimer in the documentation 00079 * and/or other materials provided with the distribution. 00080 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00081 * may be used to endorse or promote products derived from this software 00082 * without specific prior written permission. 00083 * 00084 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00085 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00086 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00087 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00088 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00089 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00090 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00091 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00092 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00093 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00094 * 00095 ****************************************************************************** 00096 */ 00097 00098 /* Includes ------------------------------------------------------------------*/ 00099 #include "stm32l4xx_hal.h" 00100 00101 /** @addtogroup STM32L4xx_HAL_Driver 00102 * @{ 00103 */ 00104 00105 /** @defgroup TIMEx TIMEx 00106 * @brief TIM Extended HAL module driver 00107 * @{ 00108 */ 00109 00110 #ifdef HAL_TIM_MODULE_ENABLED 00111 00112 /* Private typedef -----------------------------------------------------------*/ 00113 /* Private define ------------------------------------------------------------*/ 00114 #define BDTR_BKF_SHIFT (16) 00115 #define BDTR_BK2F_SHIFT (20) 00116 #define TIMx_ETRSEL_MASK ((uint32_t)0x0001C000) 00117 00118 /* Private macro -------------------------------------------------------------*/ 00119 /* Private variables ---------------------------------------------------------*/ 00120 /* Private function prototypes -----------------------------------------------*/ 00121 static void TIM_OC5_SetConfig(TIM_TypeDef *TIMx, 00122 TIM_OC_InitTypeDef *OC_Config); 00123 00124 static void TIM_OC6_SetConfig(TIM_TypeDef *TIMx, 00125 TIM_OC_InitTypeDef *OC_Config); 00126 00127 static void TIM_CCxNChannelCmd(TIM_TypeDef* TIMx, uint32_t Channel, uint32_t ChannelNState); 00128 00129 /* Private functions ---------------------------------------------------------*/ 00130 /** 00131 * @brief Timer Ouput Compare 5 configuration 00132 * @param TIMx to select the TIM peripheral 00133 * @param OC_Config: The ouput configuration structure 00134 * @retval None 00135 */ 00136 static void TIM_OC5_SetConfig(TIM_TypeDef *TIMx, 00137 TIM_OC_InitTypeDef *OC_Config) 00138 { 00139 uint32_t tmpccmrx = 0; 00140 uint32_t tmpccer = 0; 00141 uint32_t tmpcr2 = 0; 00142 00143 /* Disable the output: Reset the CCxE Bit */ 00144 TIMx->CCER &= ~TIM_CCER_CC5E; 00145 00146 /* Get the TIMx CCER register value */ 00147 tmpccer = TIMx->CCER; 00148 /* Get the TIMx CR2 register value */ 00149 tmpcr2 = TIMx->CR2; 00150 /* Get the TIMx CCMR1 register value */ 00151 tmpccmrx = TIMx->CCMR3; 00152 00153 /* Reset the Output Compare Mode Bits */ 00154 tmpccmrx &= ~(TIM_CCMR3_OC5M); 00155 /* Select the Output Compare Mode */ 00156 tmpccmrx |= OC_Config->OCMode; 00157 00158 /* Reset the Output Polarity level */ 00159 tmpccer &= ~TIM_CCER_CC5P; 00160 /* Set the Output Compare Polarity */ 00161 tmpccer |= (OC_Config->OCPolarity << 16); 00162 00163 if(IS_TIM_BREAK_INSTANCE(TIMx)) 00164 { 00165 /* Reset the Output Compare IDLE State */ 00166 tmpcr2 &= ~TIM_CR2_OIS5; 00167 /* Set the Output Idle state */ 00168 tmpcr2 |= (OC_Config->OCIdleState << 8); 00169 } 00170 /* Write to TIMx CR2 */ 00171 TIMx->CR2 = tmpcr2; 00172 00173 /* Write to TIMx CCMR3 */ 00174 TIMx->CCMR3 = tmpccmrx; 00175 00176 /* Set the Capture Compare Register value */ 00177 TIMx->CCR5 = OC_Config->Pulse; 00178 00179 /* Write to TIMx CCER */ 00180 TIMx->CCER = tmpccer; 00181 } 00182 00183 /** 00184 * @brief Timer Ouput Compare 6 configuration 00185 * @param TIMx to select the TIM peripheral 00186 * @param OC_Config: The ouput configuration structure 00187 * @retval None 00188 */ 00189 static void TIM_OC6_SetConfig(TIM_TypeDef *TIMx, 00190 TIM_OC_InitTypeDef *OC_Config) 00191 { 00192 uint32_t tmpccmrx = 0; 00193 uint32_t tmpccer = 0; 00194 uint32_t tmpcr2 = 0; 00195 00196 /* Disable the output: Reset the CCxE Bit */ 00197 TIMx->CCER &= ~TIM_CCER_CC6E; 00198 00199 /* Get the TIMx CCER register value */ 00200 tmpccer = TIMx->CCER; 00201 /* Get the TIMx CR2 register value */ 00202 tmpcr2 = TIMx->CR2; 00203 /* Get the TIMx CCMR1 register value */ 00204 tmpccmrx = TIMx->CCMR3; 00205 00206 /* Reset the Output Compare Mode Bits */ 00207 tmpccmrx &= ~(TIM_CCMR3_OC6M); 00208 /* Select the Output Compare Mode */ 00209 tmpccmrx |= (OC_Config->OCMode << 8); 00210 00211 /* Reset the Output Polarity level */ 00212 tmpccer &= (uint32_t)~TIM_CCER_CC6P; 00213 /* Set the Output Compare Polarity */ 00214 tmpccer |= (OC_Config->OCPolarity << 20); 00215 00216 if(IS_TIM_BREAK_INSTANCE(TIMx)) 00217 { 00218 /* Reset the Output Compare IDLE State */ 00219 tmpcr2 &= ~TIM_CR2_OIS6; 00220 /* Set the Output Idle state */ 00221 tmpcr2 |= (OC_Config->OCIdleState << 10); 00222 } 00223 00224 /* Write to TIMx CR2 */ 00225 TIMx->CR2 = tmpcr2; 00226 00227 /* Write to TIMx CCMR3 */ 00228 TIMx->CCMR3 = tmpccmrx; 00229 00230 /* Set the Capture Compare Register value */ 00231 TIMx->CCR6 = OC_Config->Pulse; 00232 00233 /* Write to TIMx CCER */ 00234 TIMx->CCER = tmpccer; 00235 } 00236 00237 /* Exported functions --------------------------------------------------------*/ 00238 /** @defgroup TIMEx_Exported_Functions TIM Extended Exported Functions 00239 * @{ 00240 */ 00241 00242 /** @defgroup TIMEx_Exported_Functions_Group1 Extended Timer Hall Sensor functions 00243 * @brief Timer Hall Sensor functions 00244 * 00245 @verbatim 00246 ============================================================================== 00247 ##### Timer Hall Sensor functions ##### 00248 ============================================================================== 00249 [..] 00250 This section provides functions allowing to: 00251 (+) Initialize and configure TIM HAL Sensor. 00252 (+) De-initialize TIM HAL Sensor. 00253 (+) Start the Hall Sensor Interface. 00254 (+) Stop the Hall Sensor Interface. 00255 (+) Start the Hall Sensor Interface and enable interrupts. 00256 (+) Stop the Hall Sensor Interface and disable interrupts. 00257 (+) Start the Hall Sensor Interface and enable DMA transfers. 00258 (+) Stop the Hall Sensor Interface and disable DMA transfers. 00259 00260 @endverbatim 00261 * @{ 00262 */ 00263 /** 00264 * @brief Initializes the TIM Hall Sensor Interface and initialize the associated handle. 00265 * @param htim: TIM Encoder Interface handle 00266 * @param sConfig: TIM Hall Sensor configuration structure 00267 * @retval HAL status 00268 */ 00269 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Init(TIM_HandleTypeDef *htim, TIM_HallSensor_InitTypeDef* sConfig) 00270 { 00271 TIM_OC_InitTypeDef OC_Config; 00272 00273 /* Check the TIM handle allocation */ 00274 if(htim == NULL) 00275 { 00276 return HAL_ERROR; 00277 } 00278 00279 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00280 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 00281 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 00282 assert_param(IS_TIM_IC_POLARITY(sConfig->IC1Polarity)); 00283 assert_param(IS_TIM_IC_PRESCALER(sConfig->IC1Prescaler)); 00284 assert_param(IS_TIM_IC_FILTER(sConfig->IC1Filter)); 00285 00286 if(htim->State == HAL_TIM_STATE_RESET) 00287 { 00288 /* Allocate lock resource and initialize it */ 00289 htim->Lock = HAL_UNLOCKED; 00290 00291 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 00292 HAL_TIMEx_HallSensor_MspInit(htim); 00293 } 00294 00295 /* Set the TIM state */ 00296 htim->State = HAL_TIM_STATE_BUSY; 00297 00298 /* Configure the Time base in the Encoder Mode */ 00299 TIM_Base_SetConfig(htim->Instance, &htim->Init); 00300 00301 /* Configure the Channel 1 as Input Channel to interface with the three Outputs of the Hall sensor */ 00302 TIM_TI1_SetConfig(htim->Instance, sConfig->IC1Polarity, TIM_ICSELECTION_TRC, sConfig->IC1Filter); 00303 00304 /* Reset the IC1PSC Bits */ 00305 htim->Instance->CCMR1 &= ~TIM_CCMR1_IC1PSC; 00306 /* Set the IC1PSC value */ 00307 htim->Instance->CCMR1 |= sConfig->IC1Prescaler; 00308 00309 /* Enable the Hall sensor interface (XOR function of the three inputs) */ 00310 htim->Instance->CR2 |= TIM_CR2_TI1S; 00311 00312 /* Select the TIM_TS_TI1F_ED signal as Input trigger for the TIM */ 00313 htim->Instance->SMCR &= ~TIM_SMCR_TS; 00314 htim->Instance->SMCR |= TIM_TS_TI1F_ED; 00315 00316 /* Use the TIM_TS_TI1F_ED signal to reset the TIM counter each edge detection */ 00317 htim->Instance->SMCR &= ~TIM_SMCR_SMS; 00318 htim->Instance->SMCR |= TIM_SLAVEMODE_RESET; 00319 00320 /* Program channel 2 in PWM 2 mode with the desired Commutation_Delay*/ 00321 OC_Config.OCFastMode = TIM_OCFAST_DISABLE; 00322 OC_Config.OCIdleState = TIM_OCIDLESTATE_RESET; 00323 OC_Config.OCMode = TIM_OCMODE_PWM2; 00324 OC_Config.OCNIdleState = TIM_OCNIDLESTATE_RESET; 00325 OC_Config.OCNPolarity = TIM_OCNPOLARITY_HIGH; 00326 OC_Config.OCPolarity = TIM_OCPOLARITY_HIGH; 00327 OC_Config.Pulse = sConfig->Commutation_Delay; 00328 00329 TIM_OC2_SetConfig(htim->Instance, &OC_Config); 00330 00331 /* Select OC2REF as trigger output on TRGO: write the MMS bits in the TIMx_CR2 00332 register to 101 */ 00333 htim->Instance->CR2 &= ~TIM_CR2_MMS; 00334 htim->Instance->CR2 |= TIM_TRGO_OC2REF; 00335 00336 /* Initialize the TIM state*/ 00337 htim->State= HAL_TIM_STATE_READY; 00338 00339 return HAL_OK; 00340 } 00341 00342 /** 00343 * @brief DeInitialize the TIM Hall Sensor interface 00344 * @param htim: TIM Hall Sensor handle 00345 * @retval HAL status 00346 */ 00347 HAL_StatusTypeDef HAL_TIMEx_HallSensor_DeInit(TIM_HandleTypeDef *htim) 00348 { 00349 /* Check the parameters */ 00350 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00351 00352 htim->State = HAL_TIM_STATE_BUSY; 00353 00354 /* Disable the TIM Peripheral Clock */ 00355 __HAL_TIM_DISABLE(htim); 00356 00357 /* DeInit the low level hardware: GPIO, CLOCK, NVIC */ 00358 HAL_TIMEx_HallSensor_MspDeInit(htim); 00359 00360 /* Change TIM state */ 00361 htim->State = HAL_TIM_STATE_RESET; 00362 00363 /* Release Lock */ 00364 __HAL_UNLOCK(htim); 00365 00366 return HAL_OK; 00367 } 00368 00369 /** 00370 * @brief Initializes the TIM Hall Sensor MSP. 00371 * @param htim: TIM handle 00372 * @retval None 00373 */ 00374 __weak void HAL_TIMEx_HallSensor_MspInit(TIM_HandleTypeDef *htim) 00375 { 00376 /* NOTE : This function should not be modified, when the callback is needed, 00377 the HAL_TIMEx_HallSensor_MspInit could be implemented in the user file 00378 */ 00379 } 00380 00381 /** 00382 * @brief DeInitialize TIM Hall Sensor MSP. 00383 * @param htim: TIM handle 00384 * @retval None 00385 */ 00386 __weak void HAL_TIMEx_HallSensor_MspDeInit(TIM_HandleTypeDef *htim) 00387 { 00388 /* NOTE : This function should not be modified, when the callback is needed, 00389 the HAL_TIMEx_HallSensor_MspDeInit could be implemented in the user file 00390 */ 00391 } 00392 00393 /** 00394 * @brief Starts the TIM Hall Sensor Interface. 00395 * @param htim : TIM Hall Sensor handle 00396 * @retval HAL status 00397 */ 00398 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Start(TIM_HandleTypeDef *htim) 00399 { 00400 /* Check the parameters */ 00401 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00402 00403 /* Enable the Input Capture channels 1 00404 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00405 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 00406 00407 /* Enable the Peripheral */ 00408 __HAL_TIM_ENABLE(htim); 00409 00410 /* Return function status */ 00411 return HAL_OK; 00412 } 00413 00414 /** 00415 * @brief Stops the TIM Hall sensor Interface. 00416 * @param htim : TIM Hall Sensor handle 00417 * @retval HAL status 00418 */ 00419 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Stop(TIM_HandleTypeDef *htim) 00420 { 00421 /* Check the parameters */ 00422 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00423 00424 /* Disable the Input Capture channels 1, 2 and 3 00425 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00426 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 00427 00428 /* Disable the Peripheral */ 00429 __HAL_TIM_DISABLE(htim); 00430 00431 /* Return function status */ 00432 return HAL_OK; 00433 } 00434 00435 /** 00436 * @brief Starts the TIM Hall Sensor Interface in interrupt mode. 00437 * @param htim : TIM Hall Sensor handle 00438 * @retval HAL status 00439 */ 00440 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Start_IT(TIM_HandleTypeDef *htim) 00441 { 00442 /* Check the parameters */ 00443 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00444 00445 /* Enable the capture compare Interrupts 1 event */ 00446 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 00447 00448 /* Enable the Input Capture channels 1 00449 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00450 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 00451 00452 /* Enable the Peripheral */ 00453 __HAL_TIM_ENABLE(htim); 00454 00455 /* Return function status */ 00456 return HAL_OK; 00457 } 00458 00459 /** 00460 * @brief Stops the TIM Hall Sensor Interface in interrupt mode. 00461 * @param htim : TIM handle 00462 * @retval HAL status 00463 */ 00464 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Stop_IT(TIM_HandleTypeDef *htim) 00465 { 00466 /* Check the parameters */ 00467 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00468 00469 /* Disable the Input Capture channels 1 00470 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00471 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 00472 00473 /* Disable the capture compare Interrupts event */ 00474 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 00475 00476 /* Disable the Peripheral */ 00477 __HAL_TIM_DISABLE(htim); 00478 00479 /* Return function status */ 00480 return HAL_OK; 00481 } 00482 00483 /** 00484 * @brief Starts the TIM Hall Sensor Interface in DMA mode. 00485 * @param htim : TIM Hall Sensor handle 00486 * @param pData: The destination Buffer address. 00487 * @param Length: The length of data to be transferred from TIM peripheral to memory. 00488 * @retval HAL status 00489 */ 00490 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Start_DMA(TIM_HandleTypeDef *htim, uint32_t *pData, uint16_t Length) 00491 { 00492 /* Check the parameters */ 00493 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00494 00495 if((htim->State == HAL_TIM_STATE_BUSY)) 00496 { 00497 return HAL_BUSY; 00498 } 00499 else if((htim->State == HAL_TIM_STATE_READY)) 00500 { 00501 if(((uint32_t)pData == 0 ) && (Length > 0)) 00502 { 00503 return HAL_ERROR; 00504 } 00505 else 00506 { 00507 htim->State = HAL_TIM_STATE_BUSY; 00508 } 00509 } 00510 /* Enable the Input Capture channels 1 00511 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00512 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 00513 00514 /* Set the DMA Input Capture 1 Callback */ 00515 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMACaptureCplt; 00516 /* Set the DMA error callback */ 00517 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 00518 00519 /* Enable the DMA channel for Capture 1*/ 00520 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)&htim->Instance->CCR1, (uint32_t)pData, Length); 00521 00522 /* Enable the capture compare 1 Interrupt */ 00523 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 00524 00525 /* Enable the Peripheral */ 00526 __HAL_TIM_ENABLE(htim); 00527 00528 /* Return function status */ 00529 return HAL_OK; 00530 } 00531 00532 /** 00533 * @brief Stops the TIM Hall Sensor Interface in DMA mode. 00534 * @param htim : TIM handle 00535 * @retval HAL status 00536 */ 00537 HAL_StatusTypeDef HAL_TIMEx_HallSensor_Stop_DMA(TIM_HandleTypeDef *htim) 00538 { 00539 /* Check the parameters */ 00540 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 00541 00542 /* Disable the Input Capture channels 1 00543 (in the Hall Sensor Interface the Three possible channels that can be used are TIM_CHANNEL_1, TIM_CHANNEL_2 and TIM_CHANNEL_3) */ 00544 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 00545 00546 00547 /* Disable the capture compare Interrupts 1 event */ 00548 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 00549 00550 /* Disable the Peripheral */ 00551 __HAL_TIM_DISABLE(htim); 00552 00553 /* Return function status */ 00554 return HAL_OK; 00555 } 00556 00557 /** 00558 * @} 00559 */ 00560 00561 /** @defgroup TIMEx_Exported_Functions_Group2 Extended Timer Complementary Output Compare functions 00562 * @brief Timer Complementary Output Compare functions 00563 * 00564 @verbatim 00565 ============================================================================== 00566 ##### Timer Complementary Output Compare functions ##### 00567 ============================================================================== 00568 [..] 00569 This section provides functions allowing to: 00570 (+) Start the Complementary Output Compare/PWM. 00571 (+) Stop the Complementary Output Compare/PWM. 00572 (+) Start the Complementary Output Compare/PWM and enable interrupts. 00573 (+) Stop the Complementary Output Compare/PWM and disable interrupts. 00574 (+) Start the Complementary Output Compare/PWM and enable DMA transfers. 00575 (+) Stop the Complementary Output Compare/PWM and disable DMA transfers. 00576 00577 @endverbatim 00578 * @{ 00579 */ 00580 00581 /** 00582 * @brief Starts the TIM Output Compare signal generation on the complementary 00583 * output. 00584 * @param htim : TIM Output Compare handle 00585 * @param Channel : TIM Channel to be enabled 00586 * This parameter can be one of the following values: 00587 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00588 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00589 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00590 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00591 * @retval HAL status 00592 */ 00593 HAL_StatusTypeDef HAL_TIMEx_OCN_Start(TIM_HandleTypeDef *htim, uint32_t Channel) 00594 { 00595 /* Check the parameters */ 00596 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00597 00598 /* Enable the Capture compare channel N */ 00599 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 00600 00601 /* Enable the Main Ouput */ 00602 __HAL_TIM_MOE_ENABLE(htim); 00603 00604 /* Enable the Peripheral */ 00605 __HAL_TIM_ENABLE(htim); 00606 00607 /* Return function status */ 00608 return HAL_OK; 00609 } 00610 00611 /** 00612 * @brief Stops the TIM Output Compare signal generation on the complementary 00613 * output. 00614 * @param htim : TIM handle 00615 * @param Channel : TIM Channel to be disabled 00616 * This parameter can be one of the following values: 00617 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00618 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00619 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00620 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00621 * @retval HAL status 00622 */ 00623 HAL_StatusTypeDef HAL_TIMEx_OCN_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 00624 { 00625 /* Check the parameters */ 00626 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00627 00628 /* Disable the Capture compare channel N */ 00629 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 00630 00631 /* Disable the Main Ouput */ 00632 __HAL_TIM_MOE_DISABLE(htim); 00633 00634 /* Disable the Peripheral */ 00635 __HAL_TIM_DISABLE(htim); 00636 00637 /* Return function status */ 00638 return HAL_OK; 00639 } 00640 00641 /** 00642 * @brief Starts the TIM Output Compare signal generation in interrupt mode 00643 * on the complementary output. 00644 * @param htim : TIM OC handle 00645 * @param Channel : TIM Channel to be enabled 00646 * This parameter can be one of the following values: 00647 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00648 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00649 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00650 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00651 * @retval HAL status 00652 */ 00653 HAL_StatusTypeDef HAL_TIMEx_OCN_Start_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 00654 { 00655 /* Check the parameters */ 00656 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00657 00658 switch (Channel) 00659 { 00660 case TIM_CHANNEL_1: 00661 { 00662 /* Enable the TIM Output Compare interrupt */ 00663 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 00664 } 00665 break; 00666 00667 case TIM_CHANNEL_2: 00668 { 00669 /* Enable the TIM Output Compare interrupt */ 00670 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 00671 } 00672 break; 00673 00674 case TIM_CHANNEL_3: 00675 { 00676 /* Enable the TIM Output Compare interrupt */ 00677 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC3); 00678 } 00679 break; 00680 00681 case TIM_CHANNEL_4: 00682 { 00683 /* Enable the TIM Output Compare interrupt */ 00684 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC4); 00685 } 00686 break; 00687 00688 default: 00689 break; 00690 } 00691 00692 /* Enable the TIM Break interrupt */ 00693 __HAL_TIM_ENABLE_IT(htim, TIM_IT_BREAK); 00694 00695 /* Enable the Capture compare channel N */ 00696 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 00697 00698 /* Enable the Main Ouput */ 00699 __HAL_TIM_MOE_ENABLE(htim); 00700 00701 /* Enable the Peripheral */ 00702 __HAL_TIM_ENABLE(htim); 00703 00704 /* Return function status */ 00705 return HAL_OK; 00706 } 00707 00708 /** 00709 * @brief Stops the TIM Output Compare signal generation in interrupt mode 00710 * on the complementary output. 00711 * @param htim : TIM Output Compare handle 00712 * @param Channel : TIM Channel to be disabled 00713 * This parameter can be one of the following values: 00714 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00715 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00716 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00717 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00718 * @retval HAL status 00719 */ 00720 HAL_StatusTypeDef HAL_TIMEx_OCN_Stop_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 00721 { 00722 uint32_t tmpccer = 0; 00723 00724 /* Check the parameters */ 00725 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00726 00727 switch (Channel) 00728 { 00729 case TIM_CHANNEL_1: 00730 { 00731 /* Disable the TIM Output Compare interrupt */ 00732 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 00733 } 00734 break; 00735 00736 case TIM_CHANNEL_2: 00737 { 00738 /* Disable the TIM Output Compare interrupt */ 00739 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 00740 } 00741 break; 00742 00743 case TIM_CHANNEL_3: 00744 { 00745 /* Disable the TIM Output Compare interrupt */ 00746 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC3); 00747 } 00748 break; 00749 00750 case TIM_CHANNEL_4: 00751 { 00752 /* Disable the TIM Output Compare interrupt */ 00753 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC4); 00754 } 00755 break; 00756 00757 default: 00758 break; 00759 } 00760 00761 /* Disable the Capture compare channel N */ 00762 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 00763 00764 /* Disable the TIM Break interrupt (only if no more channel is active) */ 00765 tmpccer = htim->Instance->CCER; 00766 if ((tmpccer & (TIM_CCER_CC1NE | TIM_CCER_CC2NE | TIM_CCER_CC3NE)) == RESET) 00767 { 00768 __HAL_TIM_DISABLE_IT(htim, TIM_IT_BREAK); 00769 } 00770 00771 /* Disable the Main Ouput */ 00772 __HAL_TIM_MOE_DISABLE(htim); 00773 00774 /* Disable the Peripheral */ 00775 __HAL_TIM_DISABLE(htim); 00776 00777 /* Return function status */ 00778 return HAL_OK; 00779 } 00780 00781 /** 00782 * @brief Starts the TIM Output Compare signal generation in DMA mode 00783 * on the complementary output. 00784 * @param htim : TIM Output Compare handle 00785 * @param Channel : TIM Channel to be enabled 00786 * This parameter can be one of the following values: 00787 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00788 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00789 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00790 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00791 * @param pData: The source Buffer address. 00792 * @param Length: The length of data to be transferred from memory to TIM peripheral 00793 * @retval HAL status 00794 */ 00795 HAL_StatusTypeDef HAL_TIMEx_OCN_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData, uint16_t Length) 00796 { 00797 /* Check the parameters */ 00798 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00799 00800 if((htim->State == HAL_TIM_STATE_BUSY)) 00801 { 00802 return HAL_BUSY; 00803 } 00804 else if((htim->State == HAL_TIM_STATE_READY)) 00805 { 00806 if(((uint32_t)pData == 0 ) && (Length > 0)) 00807 { 00808 return HAL_ERROR; 00809 } 00810 else 00811 { 00812 htim->State = HAL_TIM_STATE_BUSY; 00813 } 00814 } 00815 switch (Channel) 00816 { 00817 case TIM_CHANNEL_1: 00818 { 00819 /* Set the DMA Period elapsed callback */ 00820 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMADelayPulseCplt; 00821 00822 /* Set the DMA error callback */ 00823 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 00824 00825 /* Enable the DMA channel */ 00826 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)pData, (uint32_t)&htim->Instance->CCR1, Length); 00827 00828 /* Enable the TIM Output Compare DMA request */ 00829 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 00830 } 00831 break; 00832 00833 case TIM_CHANNEL_2: 00834 { 00835 /* Set the DMA Period elapsed callback */ 00836 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMADelayPulseCplt; 00837 00838 /* Set the DMA error callback */ 00839 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 00840 00841 /* Enable the DMA channel */ 00842 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)pData, (uint32_t)&htim->Instance->CCR2, Length); 00843 00844 /* Enable the TIM Output Compare DMA request */ 00845 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 00846 } 00847 break; 00848 00849 case TIM_CHANNEL_3: 00850 { 00851 /* Set the DMA Period elapsed callback */ 00852 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMADelayPulseCplt; 00853 00854 /* Set the DMA error callback */ 00855 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 00856 00857 /* Enable the DMA channel */ 00858 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)pData, (uint32_t)&htim->Instance->CCR3,Length); 00859 00860 /* Enable the TIM Output Compare DMA request */ 00861 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC3); 00862 } 00863 break; 00864 00865 case TIM_CHANNEL_4: 00866 { 00867 /* Set the DMA Period elapsed callback */ 00868 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMADelayPulseCplt; 00869 00870 /* Set the DMA error callback */ 00871 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 00872 00873 /* Enable the DMA channel */ 00874 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)pData, (uint32_t)&htim->Instance->CCR4, Length); 00875 00876 /* Enable the TIM Output Compare DMA request */ 00877 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC4); 00878 } 00879 break; 00880 00881 default: 00882 break; 00883 } 00884 00885 /* Enable the Capture compare channel N */ 00886 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 00887 00888 /* Enable the Main Ouput */ 00889 __HAL_TIM_MOE_ENABLE(htim); 00890 00891 /* Enable the Peripheral */ 00892 __HAL_TIM_ENABLE(htim); 00893 00894 /* Return function status */ 00895 return HAL_OK; 00896 } 00897 00898 /** 00899 * @brief Stops the TIM Output Compare signal generation in DMA mode 00900 * on the complementary output. 00901 * @param htim : TIM Output Compare handle 00902 * @param Channel : TIM Channel to be disabled 00903 * This parameter can be one of the following values: 00904 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00905 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00906 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00907 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00908 * @retval HAL status 00909 */ 00910 HAL_StatusTypeDef HAL_TIMEx_OCN_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 00911 { 00912 /* Check the parameters */ 00913 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 00914 00915 switch (Channel) 00916 { 00917 case TIM_CHANNEL_1: 00918 { 00919 /* Disable the TIM Output Compare DMA request */ 00920 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 00921 } 00922 break; 00923 00924 case TIM_CHANNEL_2: 00925 { 00926 /* Disable the TIM Output Compare DMA request */ 00927 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 00928 } 00929 break; 00930 00931 case TIM_CHANNEL_3: 00932 { 00933 /* Disable the TIM Output Compare DMA request */ 00934 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC3); 00935 } 00936 break; 00937 00938 case TIM_CHANNEL_4: 00939 { 00940 /* Disable the TIM Output Compare interrupt */ 00941 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC4); 00942 } 00943 break; 00944 00945 default: 00946 break; 00947 } 00948 00949 /* Disable the Capture compare channel N */ 00950 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 00951 00952 /* Disable the Main Ouput */ 00953 __HAL_TIM_MOE_DISABLE(htim); 00954 00955 /* Disable the Peripheral */ 00956 __HAL_TIM_DISABLE(htim); 00957 00958 /* Change the htim state */ 00959 htim->State = HAL_TIM_STATE_READY; 00960 00961 /* Return function status */ 00962 return HAL_OK; 00963 } 00964 00965 /** 00966 * @} 00967 */ 00968 00969 /** @defgroup TIMEx_Exported_Functions_Group3 Extended Timer Complementary PWM functions 00970 * @brief Timer Complementary PWM functions 00971 * 00972 @verbatim 00973 ============================================================================== 00974 ##### Timer Complementary PWM functions ##### 00975 ============================================================================== 00976 [..] 00977 This section provides functions allowing to: 00978 (+) Start the Complementary PWM. 00979 (+) Stop the Complementary PWM. 00980 (+) Start the Complementary PWM and enable interrupts. 00981 (+) Stop the Complementary PWM and disable interrupts. 00982 (+) Start the Complementary PWM and enable DMA transfers. 00983 (+) Stop the Complementary PWM and disable DMA transfers. 00984 (+) Start the Complementary Input Capture measurement. 00985 (+) Stop the Complementary Input Capture. 00986 (+) Start the Complementary Input Capture and enable interrupts. 00987 (+) Stop the Complementary Input Capture and disable interrupts. 00988 (+) Start the Complementary Input Capture and enable DMA transfers. 00989 (+) Stop the Complementary Input Capture and disable DMA transfers. 00990 (+) Start the Complementary One Pulse generation. 00991 (+) Stop the Complementary One Pulse. 00992 (+) Start the Complementary One Pulse and enable interrupts. 00993 (+) Stop the Complementary One Pulse and disable interrupts. 00994 00995 @endverbatim 00996 * @{ 00997 */ 00998 00999 /** 01000 * @brief Starts the PWM signal generation on the complementary output. 01001 * @param htim : TIM handle 01002 * @param Channel : TIM Channel to be enabled 01003 * This parameter can be one of the following values: 01004 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01005 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01006 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01007 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01008 * @retval HAL status 01009 */ 01010 HAL_StatusTypeDef HAL_TIMEx_PWMN_Start(TIM_HandleTypeDef *htim, uint32_t Channel) 01011 { 01012 /* Check the parameters */ 01013 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01014 01015 /* Enable the complementary PWM output */ 01016 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 01017 01018 /* Enable the Main Ouput */ 01019 __HAL_TIM_MOE_ENABLE(htim); 01020 01021 /* Enable the Peripheral */ 01022 __HAL_TIM_ENABLE(htim); 01023 01024 /* Return function status */ 01025 return HAL_OK; 01026 } 01027 01028 /** 01029 * @brief Stops the PWM signal generation on the complementary output. 01030 * @param htim : TIM handle 01031 * @param Channel : TIM Channel to be disabled 01032 * This parameter can be one of the following values: 01033 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01034 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01035 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01036 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01037 * @retval HAL status 01038 */ 01039 HAL_StatusTypeDef HAL_TIMEx_PWMN_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 01040 { 01041 /* Check the parameters */ 01042 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01043 01044 /* Disable the complementary PWM output */ 01045 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 01046 01047 /* Disable the Main Ouput */ 01048 __HAL_TIM_MOE_DISABLE(htim); 01049 01050 /* Disable the Peripheral */ 01051 __HAL_TIM_DISABLE(htim); 01052 01053 /* Return function status */ 01054 return HAL_OK; 01055 } 01056 01057 /** 01058 * @brief Starts the PWM signal generation in interrupt mode on the 01059 * complementary output. 01060 * @param htim : TIM handle 01061 * @param Channel : TIM Channel to be disabled 01062 * This parameter can be one of the following values: 01063 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01064 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01065 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01066 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01067 * @retval HAL status 01068 */ 01069 HAL_StatusTypeDef HAL_TIMEx_PWMN_Start_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 01070 { 01071 /* Check the parameters */ 01072 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01073 01074 switch (Channel) 01075 { 01076 case TIM_CHANNEL_1: 01077 { 01078 /* Enable the TIM Capture/Compare 1 interrupt */ 01079 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 01080 } 01081 break; 01082 01083 case TIM_CHANNEL_2: 01084 { 01085 /* Enable the TIM Capture/Compare 2 interrupt */ 01086 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 01087 } 01088 break; 01089 01090 case TIM_CHANNEL_3: 01091 { 01092 /* Enable the TIM Capture/Compare 3 interrupt */ 01093 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC3); 01094 } 01095 break; 01096 01097 case TIM_CHANNEL_4: 01098 { 01099 /* Enable the TIM Capture/Compare 4 interrupt */ 01100 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC4); 01101 } 01102 break; 01103 01104 default: 01105 break; 01106 } 01107 01108 /* Enable the TIM Break interrupt */ 01109 __HAL_TIM_ENABLE_IT(htim, TIM_IT_BREAK); 01110 01111 /* Enable the complementary PWM output */ 01112 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 01113 01114 /* Enable the Main Ouput */ 01115 __HAL_TIM_MOE_ENABLE(htim); 01116 01117 /* Enable the Peripheral */ 01118 __HAL_TIM_ENABLE(htim); 01119 01120 /* Return function status */ 01121 return HAL_OK; 01122 } 01123 01124 /** 01125 * @brief Stops the PWM signal generation in interrupt mode on the 01126 * complementary output. 01127 * @param htim : TIM handle 01128 * @param Channel : TIM Channel to be disabled 01129 * This parameter can be one of the following values: 01130 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01131 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01132 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01133 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01134 * @retval HAL status 01135 */ 01136 HAL_StatusTypeDef HAL_TIMEx_PWMN_Stop_IT (TIM_HandleTypeDef *htim, uint32_t Channel) 01137 { 01138 uint32_t tmpccer = 0; 01139 01140 /* Check the parameters */ 01141 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01142 01143 switch (Channel) 01144 { 01145 case TIM_CHANNEL_1: 01146 { 01147 /* Disable the TIM Capture/Compare 1 interrupt */ 01148 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 01149 } 01150 break; 01151 01152 case TIM_CHANNEL_2: 01153 { 01154 /* Disable the TIM Capture/Compare 2 interrupt */ 01155 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 01156 } 01157 break; 01158 01159 case TIM_CHANNEL_3: 01160 { 01161 /* Disable the TIM Capture/Compare 3 interrupt */ 01162 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC3); 01163 } 01164 break; 01165 01166 case TIM_CHANNEL_4: 01167 { 01168 /* Disable the TIM Capture/Compare 3 interrupt */ 01169 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC4); 01170 } 01171 break; 01172 01173 default: 01174 break; 01175 } 01176 01177 /* Disable the complementary PWM output */ 01178 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 01179 01180 01181 /* Disable the TIM Break interrupt (only if no more channel is active) */ 01182 tmpccer = htim->Instance->CCER; 01183 if ((tmpccer & (TIM_CCER_CC1NE | TIM_CCER_CC2NE | TIM_CCER_CC3NE)) == RESET) 01184 { 01185 __HAL_TIM_DISABLE_IT(htim, TIM_IT_BREAK); 01186 } 01187 01188 /* Disable the Main Ouput */ 01189 __HAL_TIM_MOE_DISABLE(htim); 01190 01191 /* Disable the Peripheral */ 01192 __HAL_TIM_DISABLE(htim); 01193 01194 /* Return function status */ 01195 return HAL_OK; 01196 } 01197 01198 /** 01199 * @brief Starts the TIM PWM signal generation in DMA mode on the 01200 * complementary output 01201 * @param htim : TIM handle 01202 * @param Channel : TIM Channel to be enabled 01203 * This parameter can be one of the following values: 01204 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01205 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01206 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01207 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01208 * @param pData: The source Buffer address. 01209 * @param Length: The length of data to be transferred from memory to TIM peripheral 01210 * @retval HAL status 01211 */ 01212 HAL_StatusTypeDef HAL_TIMEx_PWMN_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData, uint16_t Length) 01213 { 01214 /* Check the parameters */ 01215 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01216 01217 if((htim->State == HAL_TIM_STATE_BUSY)) 01218 { 01219 return HAL_BUSY; 01220 } 01221 else if((htim->State == HAL_TIM_STATE_READY)) 01222 { 01223 if(((uint32_t)pData == 0 ) && (Length > 0)) 01224 { 01225 return HAL_ERROR; 01226 } 01227 else 01228 { 01229 htim->State = HAL_TIM_STATE_BUSY; 01230 } 01231 } 01232 switch (Channel) 01233 { 01234 case TIM_CHANNEL_1: 01235 { 01236 /* Set the DMA Period elapsed callback */ 01237 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMADelayPulseCplt; 01238 01239 /* Set the DMA error callback */ 01240 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 01241 01242 /* Enable the DMA channel */ 01243 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)pData, (uint32_t)&htim->Instance->CCR1, Length); 01244 01245 /* Enable the TIM Capture/Compare 1 DMA request */ 01246 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 01247 } 01248 break; 01249 01250 case TIM_CHANNEL_2: 01251 { 01252 /* Set the DMA Period elapsed callback */ 01253 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMADelayPulseCplt; 01254 01255 /* Set the DMA error callback */ 01256 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 01257 01258 /* Enable the DMA channel */ 01259 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)pData, (uint32_t)&htim->Instance->CCR2, Length); 01260 01261 /* Enable the TIM Capture/Compare 2 DMA request */ 01262 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 01263 } 01264 break; 01265 01266 case TIM_CHANNEL_3: 01267 { 01268 /* Set the DMA Period elapsed callback */ 01269 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMADelayPulseCplt; 01270 01271 /* Set the DMA error callback */ 01272 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 01273 01274 /* Enable the DMA channel */ 01275 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)pData, (uint32_t)&htim->Instance->CCR3,Length); 01276 01277 /* Enable the TIM Capture/Compare 3 DMA request */ 01278 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC3); 01279 } 01280 break; 01281 01282 case TIM_CHANNEL_4: 01283 { 01284 /* Set the DMA Period elapsed callback */ 01285 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMADelayPulseCplt; 01286 01287 /* Set the DMA error callback */ 01288 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 01289 01290 /* Enable the DMA channel */ 01291 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)pData, (uint32_t)&htim->Instance->CCR4, Length); 01292 01293 /* Enable the TIM Capture/Compare 4 DMA request */ 01294 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC4); 01295 } 01296 break; 01297 01298 default: 01299 break; 01300 } 01301 01302 /* Enable the complementary PWM output */ 01303 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_ENABLE); 01304 01305 /* Enable the Main Ouput */ 01306 __HAL_TIM_MOE_ENABLE(htim); 01307 01308 /* Enable the Peripheral */ 01309 __HAL_TIM_ENABLE(htim); 01310 01311 /* Return function status */ 01312 return HAL_OK; 01313 } 01314 01315 /** 01316 * @brief Stops the TIM PWM signal generation in DMA mode on the complementary 01317 * output 01318 * @param htim : TIM handle 01319 * @param Channel : TIM Channel to be disabled 01320 * This parameter can be one of the following values: 01321 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01322 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01323 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01324 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01325 * @retval HAL status 01326 */ 01327 HAL_StatusTypeDef HAL_TIMEx_PWMN_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 01328 { 01329 /* Check the parameters */ 01330 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, Channel)); 01331 01332 switch (Channel) 01333 { 01334 case TIM_CHANNEL_1: 01335 { 01336 /* Disable the TIM Capture/Compare 1 DMA request */ 01337 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 01338 } 01339 break; 01340 01341 case TIM_CHANNEL_2: 01342 { 01343 /* Disable the TIM Capture/Compare 2 DMA request */ 01344 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 01345 } 01346 break; 01347 01348 case TIM_CHANNEL_3: 01349 { 01350 /* Disable the TIM Capture/Compare 3 DMA request */ 01351 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC3); 01352 } 01353 break; 01354 01355 case TIM_CHANNEL_4: 01356 { 01357 /* Disable the TIM Capture/Compare 4 DMA request */ 01358 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC4); 01359 } 01360 break; 01361 01362 default: 01363 break; 01364 } 01365 01366 /* Disable the complementary PWM output */ 01367 TIM_CCxNChannelCmd(htim->Instance, Channel, TIM_CCxN_DISABLE); 01368 01369 /* Disable the Main Ouput */ 01370 __HAL_TIM_MOE_DISABLE(htim); 01371 01372 /* Disable the Peripheral */ 01373 __HAL_TIM_DISABLE(htim); 01374 01375 /* Change the htim state */ 01376 htim->State = HAL_TIM_STATE_READY; 01377 01378 /* Return function status */ 01379 return HAL_OK; 01380 } 01381 01382 /** 01383 * @} 01384 */ 01385 01386 /** @defgroup TIMEx_Exported_Functions_Group4 Extended Timer Complementary One Pulse functions 01387 * @brief Timer Complementary One Pulse functions 01388 * 01389 @verbatim 01390 ============================================================================== 01391 ##### Timer Complementary One Pulse functions ##### 01392 ============================================================================== 01393 [..] 01394 This section provides functions allowing to: 01395 (+) Start the Complementary One Pulse generation. 01396 (+) Stop the Complementary One Pulse. 01397 (+) Start the Complementary One Pulse and enable interrupts. 01398 (+) Stop the Complementary One Pulse and disable interrupts. 01399 01400 @endverbatim 01401 * @{ 01402 */ 01403 01404 /** 01405 * @brief Starts the TIM One Pulse signal generation on the complementary 01406 * output. 01407 * @param htim : TIM One Pulse handle 01408 * @param OutputChannel : TIM Channel to be enabled 01409 * This parameter can be one of the following values: 01410 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01411 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01412 * @retval HAL status 01413 */ 01414 HAL_StatusTypeDef HAL_TIMEx_OnePulseN_Start(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 01415 { 01416 /* Check the parameters */ 01417 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, OutputChannel)); 01418 01419 /* Enable the complementary One Pulse output */ 01420 TIM_CCxNChannelCmd(htim->Instance, OutputChannel, TIM_CCxN_ENABLE); 01421 01422 /* Enable the Main Ouput */ 01423 __HAL_TIM_MOE_ENABLE(htim); 01424 01425 /* Return function status */ 01426 return HAL_OK; 01427 } 01428 01429 /** 01430 * @brief Stops the TIM One Pulse signal generation on the complementary 01431 * output. 01432 * @param htim : TIM One Pulse handle 01433 * @param OutputChannel : TIM Channel to be disabled 01434 * This parameter can be one of the following values: 01435 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01436 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01437 * @retval HAL status 01438 */ 01439 HAL_StatusTypeDef HAL_TIMEx_OnePulseN_Stop(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 01440 { 01441 01442 /* Check the parameters */ 01443 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, OutputChannel)); 01444 01445 /* Disable the complementary One Pulse output */ 01446 TIM_CCxNChannelCmd(htim->Instance, OutputChannel, TIM_CCxN_DISABLE); 01447 01448 /* Disable the Main Ouput */ 01449 __HAL_TIM_MOE_DISABLE(htim); 01450 01451 /* Disable the Peripheral */ 01452 __HAL_TIM_DISABLE(htim); 01453 01454 /* Return function status */ 01455 return HAL_OK; 01456 } 01457 01458 /** 01459 * @brief Starts the TIM One Pulse signal generation in interrupt mode on the 01460 * complementary channel. 01461 * @param htim : TIM One Pulse handle 01462 * @param OutputChannel : TIM Channel to be enabled 01463 * This parameter can be one of the following values: 01464 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01465 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01466 * @retval HAL status 01467 */ 01468 HAL_StatusTypeDef HAL_TIMEx_OnePulseN_Start_IT(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 01469 { 01470 /* Check the parameters */ 01471 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, OutputChannel)); 01472 01473 /* Enable the TIM Capture/Compare 1 interrupt */ 01474 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 01475 01476 /* Enable the TIM Capture/Compare 2 interrupt */ 01477 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 01478 01479 /* Enable the complementary One Pulse output */ 01480 TIM_CCxNChannelCmd(htim->Instance, OutputChannel, TIM_CCxN_ENABLE); 01481 01482 /* Enable the Main Ouput */ 01483 __HAL_TIM_MOE_ENABLE(htim); 01484 01485 /* Return function status */ 01486 return HAL_OK; 01487 } 01488 01489 /** 01490 * @brief Stops the TIM One Pulse signal generation in interrupt mode on the 01491 * complementary channel. 01492 * @param htim : TIM One Pulse handle 01493 * @param OutputChannel : TIM Channel to be disabled 01494 * This parameter can be one of the following values: 01495 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01496 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01497 * @retval HAL status 01498 */ 01499 HAL_StatusTypeDef HAL_TIMEx_OnePulseN_Stop_IT(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 01500 { 01501 /* Check the parameters */ 01502 assert_param(IS_TIM_CCXN_INSTANCE(htim->Instance, OutputChannel)); 01503 01504 /* Disable the TIM Capture/Compare 1 interrupt */ 01505 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 01506 01507 /* Disable the TIM Capture/Compare 2 interrupt */ 01508 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 01509 01510 /* Disable the complementary One Pulse output */ 01511 TIM_CCxNChannelCmd(htim->Instance, OutputChannel, TIM_CCxN_DISABLE); 01512 01513 /* Disable the Main Ouput */ 01514 __HAL_TIM_MOE_DISABLE(htim); 01515 01516 /* Disable the Peripheral */ 01517 __HAL_TIM_DISABLE(htim); 01518 01519 /* Return function status */ 01520 return HAL_OK; 01521 } 01522 01523 /** 01524 * @} 01525 */ 01526 01527 /** @defgroup TIMEx_Exported_Functions_Group5 Extended Peripheral Control functions 01528 * @brief Peripheral Control functions 01529 * 01530 @verbatim 01531 ============================================================================== 01532 ##### Peripheral Control functions ##### 01533 ============================================================================== 01534 [..] 01535 This section provides functions allowing to: 01536 (+) Configure the commutation event in case of use of the Hall sensor interface. 01537 (+) Configure Output channels for OC and PWM mode. 01538 01539 (+) Configure Complementary channels, break features and dead time. 01540 (+) Configure Master synchronization. 01541 (+) Configure timer remapping capabilities. 01542 (+) Enable or disable channel grouping 01543 01544 @endverbatim 01545 * @{ 01546 */ 01547 01548 /** 01549 * @brief Configure the TIM commutation event sequence. 01550 * @note This function is mandatory to use the commutation event in order to 01551 * update the configuration at each commutation detection on the TRGI input of the Timer, 01552 * the typical use of this feature is with the use of another Timer(interface Timer) 01553 * configured in Hall sensor interface, this interface Timer will generate the 01554 * commutation at its TRGO output (connected to Timer used in this function) each time 01555 * the TI1 of the Interface Timer detect a commutation at its input TI1. 01556 * @param htim: TIM handle 01557 * @param InputTrigger : the Internal trigger corresponding to the Timer Interfacing with the Hall sensor 01558 * This parameter can be one of the following values: 01559 * @arg TIM_TS_ITR0: Internal trigger 0 selected 01560 * @arg TIM_TS_ITR1: Internal trigger 1 selected 01561 * @arg TIM_TS_ITR2: Internal trigger 2 selected 01562 * @arg TIM_TS_ITR3: Internal trigger 3 selected 01563 * @arg TIM_TS_NONE: No trigger is needed 01564 * @param CommutationSource : the Commutation Event source 01565 * This parameter can be one of the following values: 01566 * @arg TIM_COMMUTATION_TRGI: Commutation source is the TRGI of the Interface Timer 01567 * @arg TIM_COMMUTATION_SOFTWARE: Commutation source is set by software using the COMG bit 01568 * @retval HAL status 01569 */ 01570 HAL_StatusTypeDef HAL_TIMEx_ConfigCommutationEvent(TIM_HandleTypeDef *htim, uint32_t InputTrigger, uint32_t CommutationSource) 01571 { 01572 /* Check the parameters */ 01573 assert_param(IS_TIM_COMMUTATION_EVENT_INSTANCE(htim->Instance)); 01574 assert_param(IS_TIM_INTERNAL_TRIGGEREVENT_SELECTION(InputTrigger)); 01575 01576 __HAL_LOCK(htim); 01577 01578 if ((InputTrigger == TIM_TS_ITR0) || (InputTrigger == TIM_TS_ITR1) || 01579 (InputTrigger == TIM_TS_ITR2) || (InputTrigger == TIM_TS_ITR3)) 01580 { 01581 /* Select the Input trigger */ 01582 htim->Instance->SMCR &= ~TIM_SMCR_TS; 01583 htim->Instance->SMCR |= InputTrigger; 01584 } 01585 01586 /* Select the Capture Compare preload feature */ 01587 htim->Instance->CR2 |= TIM_CR2_CCPC; 01588 /* Select the Commutation event source */ 01589 htim->Instance->CR2 &= ~TIM_CR2_CCUS; 01590 htim->Instance->CR2 |= CommutationSource; 01591 01592 __HAL_UNLOCK(htim); 01593 01594 return HAL_OK; 01595 } 01596 01597 /** 01598 * @brief Configure the TIM commutation event sequence with interrupt. 01599 * @note This function is mandatory to use the commutation event in order to 01600 * update the configuration at each commutation detection on the TRGI input of the Timer, 01601 * the typical use of this feature is with the use of another Timer(interface Timer) 01602 * configured in Hall sensor interface, this interface Timer will generate the 01603 * commutation at its TRGO output (connected to Timer used in this function) each time 01604 * the TI1 of the Interface Timer detect a commutation at its input TI1. 01605 * @param htim: TIM handle 01606 * @param InputTrigger : the Internal trigger corresponding to the Timer Interfacing with the Hall sensor 01607 * This parameter can be one of the following values: 01608 * @arg TIM_TS_ITR0: Internal trigger 0 selected 01609 * @arg TIM_TS_ITR1: Internal trigger 1 selected 01610 * @arg TIM_TS_ITR2: Internal trigger 2 selected 01611 * @arg TIM_TS_ITR3: Internal trigger 3 selected 01612 * @arg TIM_TS_NONE: No trigger is needed 01613 * @param CommutationSource : the Commutation Event source 01614 * This parameter can be one of the following values: 01615 * @arg TIM_COMMUTATION_TRGI: Commutation source is the TRGI of the Interface Timer 01616 * @arg TIM_COMMUTATION_SOFTWARE: Commutation source is set by software using the COMG bit 01617 * @retval HAL status 01618 */ 01619 HAL_StatusTypeDef HAL_TIMEx_ConfigCommutationEvent_IT(TIM_HandleTypeDef *htim, uint32_t InputTrigger, uint32_t CommutationSource) 01620 { 01621 /* Check the parameters */ 01622 assert_param(IS_TIM_COMMUTATION_EVENT_INSTANCE(htim->Instance)); 01623 assert_param(IS_TIM_INTERNAL_TRIGGEREVENT_SELECTION(InputTrigger)); 01624 01625 __HAL_LOCK(htim); 01626 01627 if ((InputTrigger == TIM_TS_ITR0) || (InputTrigger == TIM_TS_ITR1) || 01628 (InputTrigger == TIM_TS_ITR2) || (InputTrigger == TIM_TS_ITR3)) 01629 { 01630 /* Select the Input trigger */ 01631 htim->Instance->SMCR &= ~TIM_SMCR_TS; 01632 htim->Instance->SMCR |= InputTrigger; 01633 } 01634 01635 /* Select the Capture Compare preload feature */ 01636 htim->Instance->CR2 |= TIM_CR2_CCPC; 01637 /* Select the Commutation event source */ 01638 htim->Instance->CR2 &= ~TIM_CR2_CCUS; 01639 htim->Instance->CR2 |= CommutationSource; 01640 01641 /* Enable the Commutation Interrupt Request */ 01642 __HAL_TIM_ENABLE_IT(htim, TIM_IT_COM); 01643 01644 __HAL_UNLOCK(htim); 01645 01646 return HAL_OK; 01647 } 01648 01649 /** 01650 * @brief Configure the TIM commutation event sequence with DMA. 01651 * @note This function is mandatory to use the commutation event in order to 01652 * update the configuration at each commutation detection on the TRGI input of the Timer, 01653 * the typical use of this feature is with the use of another Timer(interface Timer) 01654 * configured in Hall sensor interface, this interface Timer will generate the 01655 * commutation at its TRGO output (connected to Timer used in this function) each time 01656 * the TI1 of the Interface Timer detect a commutation at its input TI1. 01657 * @note The user should configure the DMA in his own software, in This function only the COMDE bit is set 01658 * @param htim: TIM handle 01659 * @param InputTrigger : the Internal trigger corresponding to the Timer Interfacing with the Hall sensor 01660 * This parameter can be one of the following values: 01661 * @arg TIM_TS_ITR0: Internal trigger 0 selected 01662 * @arg TIM_TS_ITR1: Internal trigger 1 selected 01663 * @arg TIM_TS_ITR2: Internal trigger 2 selected 01664 * @arg TIM_TS_ITR3: Internal trigger 3 selected 01665 * @arg TIM_TS_NONE: No trigger is needed 01666 * @param CommutationSource : the Commutation Event source 01667 * This parameter can be one of the following values: 01668 * @arg TIM_COMMUTATION_TRGI: Commutation source is the TRGI of the Interface Timer 01669 * @arg TIM_COMMUTATION_SOFTWARE: Commutation source is set by software using the COMG bit 01670 * @retval HAL status 01671 */ 01672 HAL_StatusTypeDef HAL_TIMEx_ConfigCommutationEvent_DMA(TIM_HandleTypeDef *htim, uint32_t InputTrigger, uint32_t CommutationSource) 01673 { 01674 /* Check the parameters */ 01675 assert_param(IS_TIM_COMMUTATION_EVENT_INSTANCE(htim->Instance)); 01676 assert_param(IS_TIM_INTERNAL_TRIGGEREVENT_SELECTION(InputTrigger)); 01677 01678 __HAL_LOCK(htim); 01679 01680 if ((InputTrigger == TIM_TS_ITR0) || (InputTrigger == TIM_TS_ITR1) || 01681 (InputTrigger == TIM_TS_ITR2) || (InputTrigger == TIM_TS_ITR3)) 01682 { 01683 /* Select the Input trigger */ 01684 htim->Instance->SMCR &= ~TIM_SMCR_TS; 01685 htim->Instance->SMCR |= InputTrigger; 01686 } 01687 01688 /* Select the Capture Compare preload feature */ 01689 htim->Instance->CR2 |= TIM_CR2_CCPC; 01690 /* Select the Commutation event source */ 01691 htim->Instance->CR2 &= ~TIM_CR2_CCUS; 01692 htim->Instance->CR2 |= CommutationSource; 01693 01694 /* Enable the Commutation DMA Request */ 01695 /* Set the DMA Commutation Callback */ 01696 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferCpltCallback = TIMEx_DMACommutationCplt; 01697 /* Set the DMA error callback */ 01698 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferErrorCallback = TIM_DMAError; 01699 01700 /* Enable the Commutation DMA Request */ 01701 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_COM); 01702 01703 __HAL_UNLOCK(htim); 01704 01705 return HAL_OK; 01706 } 01707 01708 /** 01709 * @brief Initializes the TIM Output Compare Channels according to the specified 01710 * parameters in the TIM_OC_InitTypeDef. 01711 * @param htim: TIM Output Compare handle 01712 * @param sConfig: TIM Output Compare configuration structure 01713 * @param Channel : TIM Channels to configure 01714 * This parameter can be one of the following values: 01715 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01716 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01717 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01718 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01719 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 01720 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 01721 * @arg TIM_CHANNEL_ALL: all output channels supported by the timer instance selected 01722 * @retval HAL status 01723 */ 01724 HAL_StatusTypeDef HAL_TIM_OC_ConfigChannel(TIM_HandleTypeDef *htim, 01725 TIM_OC_InitTypeDef* sConfig, 01726 uint32_t Channel) 01727 { 01728 /* Check the parameters */ 01729 assert_param(IS_TIM_CHANNELS(Channel)); 01730 assert_param(IS_TIM_OC_MODE(sConfig->OCMode)); 01731 assert_param(IS_TIM_OC_POLARITY(sConfig->OCPolarity)); 01732 assert_param(IS_TIM_OCN_POLARITY(sConfig->OCNPolarity)); 01733 assert_param(IS_TIM_OCNIDLE_STATE(sConfig->OCNIdleState)); 01734 assert_param(IS_TIM_OCIDLE_STATE(sConfig->OCIdleState)); 01735 01736 /* Check input state */ 01737 __HAL_LOCK(htim); 01738 01739 htim->State = HAL_TIM_STATE_BUSY; 01740 01741 switch (Channel) 01742 { 01743 case TIM_CHANNEL_1: 01744 { 01745 /* Check the parameters */ 01746 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 01747 01748 /* Configure the TIM Channel 1 in Output Compare */ 01749 TIM_OC1_SetConfig(htim->Instance, sConfig); 01750 } 01751 break; 01752 01753 case TIM_CHANNEL_2: 01754 { 01755 /* Check the parameters */ 01756 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 01757 01758 /* Configure the TIM Channel 2 in Output Compare */ 01759 TIM_OC2_SetConfig(htim->Instance, sConfig); 01760 } 01761 break; 01762 01763 case TIM_CHANNEL_3: 01764 { 01765 /* Check the parameters */ 01766 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 01767 01768 /* Configure the TIM Channel 3 in Output Compare */ 01769 TIM_OC3_SetConfig(htim->Instance, sConfig); 01770 } 01771 break; 01772 01773 case TIM_CHANNEL_4: 01774 { 01775 /* Check the parameters */ 01776 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 01777 01778 /* Configure the TIM Channel 4 in Output Compare */ 01779 TIM_OC4_SetConfig(htim->Instance, sConfig); 01780 } 01781 break; 01782 01783 case TIM_CHANNEL_5: 01784 { 01785 /* Check the parameters */ 01786 assert_param(IS_TIM_CC5_INSTANCE(htim->Instance)); 01787 01788 /* Configure the TIM Channel 5 in Output Compare */ 01789 TIM_OC5_SetConfig(htim->Instance, sConfig); 01790 } 01791 break; 01792 01793 case TIM_CHANNEL_6: 01794 { 01795 /* Check the parameters */ 01796 assert_param(IS_TIM_CC6_INSTANCE(htim->Instance)); 01797 01798 /* Configure the TIM Channel 6 in Output Compare */ 01799 TIM_OC6_SetConfig(htim->Instance, sConfig); 01800 } 01801 break; 01802 01803 default: 01804 break; 01805 } 01806 01807 htim->State = HAL_TIM_STATE_READY; 01808 01809 __HAL_UNLOCK(htim); 01810 01811 return HAL_OK; 01812 } 01813 01814 /** 01815 * @brief Initializes the TIM PWM channels according to the specified 01816 * parameters in the TIM_OC_InitTypeDef. 01817 * @param htim: TIM PWM handle 01818 * @param sConfig: TIM PWM configuration structure 01819 * @param Channel : TIM Channels to be configured 01820 * This parameter can be one of the following values: 01821 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01822 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01823 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01824 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01825 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 01826 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 01827 * @arg TIM_CHANNEL_ALL: all PWM channels supported by the timer instance selected 01828 * @retval HAL status 01829 */ 01830 HAL_StatusTypeDef HAL_TIM_PWM_ConfigChannel(TIM_HandleTypeDef *htim, 01831 TIM_OC_InitTypeDef* sConfig, 01832 uint32_t Channel) 01833 { 01834 /* Check the parameters */ 01835 assert_param(IS_TIM_CHANNELS(Channel)); 01836 assert_param(IS_TIM_PWM_MODE(sConfig->OCMode)); 01837 assert_param(IS_TIM_OC_POLARITY(sConfig->OCPolarity)); 01838 assert_param(IS_TIM_OCN_POLARITY(sConfig->OCNPolarity)); 01839 assert_param(IS_TIM_FAST_STATE(sConfig->OCFastMode)); 01840 assert_param(IS_TIM_OCNIDLE_STATE(sConfig->OCNIdleState)); 01841 assert_param(IS_TIM_OCIDLE_STATE(sConfig->OCIdleState)); 01842 01843 /* Check input state */ 01844 __HAL_LOCK(htim); 01845 01846 htim->State = HAL_TIM_STATE_BUSY; 01847 01848 switch (Channel) 01849 { 01850 case TIM_CHANNEL_1: 01851 { 01852 /* Check the parameters */ 01853 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 01854 01855 /* Configure the Channel 1 in PWM mode */ 01856 TIM_OC1_SetConfig(htim->Instance, sConfig); 01857 01858 /* Set the Preload enable bit for channel1 */ 01859 htim->Instance->CCMR1 |= TIM_CCMR1_OC1PE; 01860 01861 /* Configure the Output Fast mode */ 01862 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC1FE; 01863 htim->Instance->CCMR1 |= sConfig->OCFastMode; 01864 } 01865 break; 01866 01867 case TIM_CHANNEL_2: 01868 { 01869 /* Check the parameters */ 01870 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 01871 01872 /* Configure the Channel 2 in PWM mode */ 01873 TIM_OC2_SetConfig(htim->Instance, sConfig); 01874 01875 /* Set the Preload enable bit for channel2 */ 01876 htim->Instance->CCMR1 |= TIM_CCMR1_OC2PE; 01877 01878 /* Configure the Output Fast mode */ 01879 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC2FE; 01880 htim->Instance->CCMR1 |= sConfig->OCFastMode << 8; 01881 } 01882 break; 01883 01884 case TIM_CHANNEL_3: 01885 { 01886 /* Check the parameters */ 01887 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 01888 01889 /* Configure the Channel 3 in PWM mode */ 01890 TIM_OC3_SetConfig(htim->Instance, sConfig); 01891 01892 /* Set the Preload enable bit for channel3 */ 01893 htim->Instance->CCMR2 |= TIM_CCMR2_OC3PE; 01894 01895 /* Configure the Output Fast mode */ 01896 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC3FE; 01897 htim->Instance->CCMR2 |= sConfig->OCFastMode; 01898 } 01899 break; 01900 01901 case TIM_CHANNEL_4: 01902 { 01903 /* Check the parameters */ 01904 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 01905 01906 /* Configure the Channel 4 in PWM mode */ 01907 TIM_OC4_SetConfig(htim->Instance, sConfig); 01908 01909 /* Set the Preload enable bit for channel4 */ 01910 htim->Instance->CCMR2 |= TIM_CCMR2_OC4PE; 01911 01912 /* Configure the Output Fast mode */ 01913 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC4FE; 01914 htim->Instance->CCMR2 |= sConfig->OCFastMode << 8; 01915 } 01916 break; 01917 01918 case TIM_CHANNEL_5: 01919 { 01920 /* Check the parameters */ 01921 assert_param(IS_TIM_CC5_INSTANCE(htim->Instance)); 01922 01923 /* Configure the Channel 5 in PWM mode */ 01924 TIM_OC5_SetConfig(htim->Instance, sConfig); 01925 01926 /* Set the Preload enable bit for channel5*/ 01927 htim->Instance->CCMR3 |= TIM_CCMR3_OC5PE; 01928 01929 /* Configure the Output Fast mode */ 01930 htim->Instance->CCMR3 &= ~TIM_CCMR3_OC5FE; 01931 htim->Instance->CCMR3 |= sConfig->OCFastMode; 01932 } 01933 break; 01934 01935 case TIM_CHANNEL_6: 01936 { 01937 /* Check the parameters */ 01938 assert_param(IS_TIM_CC6_INSTANCE(htim->Instance)); 01939 01940 /* Configure the Channel 5 in PWM mode */ 01941 TIM_OC6_SetConfig(htim->Instance, sConfig); 01942 01943 /* Set the Preload enable bit for channel6 */ 01944 htim->Instance->CCMR3 |= TIM_CCMR3_OC6PE; 01945 01946 /* Configure the Output Fast mode */ 01947 htim->Instance->CCMR3 &= ~TIM_CCMR3_OC6FE; 01948 htim->Instance->CCMR3 |= sConfig->OCFastMode << 8; 01949 } 01950 break; 01951 01952 default: 01953 break; 01954 } 01955 01956 htim->State = HAL_TIM_STATE_READY; 01957 01958 __HAL_UNLOCK(htim); 01959 01960 return HAL_OK; 01961 } 01962 01963 /** 01964 * @brief Configures the OCRef clear feature 01965 * @param htim: TIM handle 01966 * @param sClearInputConfig: pointer to a TIM_ClearInputConfigTypeDef structure that 01967 * contains the OCREF clear feature and parameters for the TIM peripheral. 01968 * @param Channel: specifies the TIM Channel 01969 * This parameter can be one of the following values: 01970 * @arg TIM_Channel_1: TIM Channel 1 01971 * @arg TIM_Channel_2: TIM Channel 2 01972 * @arg TIM_Channel_3: TIM Channel 3 01973 * @arg TIM_Channel_4: TIM Channel 4 01974 * @arg TIM_Channel_5: TIM Channel 5 01975 * @arg TIM_Channel_6: TIM Channel 6 01976 * @retval None 01977 */ 01978 HAL_StatusTypeDef HAL_TIM_ConfigOCrefClear(TIM_HandleTypeDef *htim, 01979 TIM_ClearInputConfigTypeDef *sClearInputConfig, 01980 uint32_t Channel) 01981 { 01982 uint32_t tmpsmcr = 0; 01983 01984 /* Check the parameters */ 01985 assert_param(IS_TIM_OCXREF_CLEAR_INSTANCE(htim->Instance)); 01986 assert_param(IS_TIM_CLEARINPUT_SOURCE(sClearInputConfig->ClearInputSource)); 01987 01988 /* Check input state */ 01989 __HAL_LOCK(htim); 01990 01991 switch (sClearInputConfig->ClearInputSource) 01992 { 01993 case TIM_CLEARINPUTSOURCE_NONE: 01994 { 01995 /* Get the TIMx SMCR register value */ 01996 tmpsmcr = htim->Instance->SMCR; 01997 01998 /* Clear the OCREF clear selection bit */ 01999 tmpsmcr &= ~TIM_SMCR_OCCS; 02000 02001 /* Clear the ETR Bits */ 02002 tmpsmcr &= ~(TIM_SMCR_ETF | TIM_SMCR_ETPS | TIM_SMCR_ECE | TIM_SMCR_ETP); 02003 02004 /* Set TIMx_SMCR */ 02005 htim->Instance->SMCR = tmpsmcr; 02006 } 02007 break; 02008 02009 case TIM_CLEARINPUTSOURCE_OCREFCLR: 02010 { 02011 /* Clear the OCREF clear selection bit */ 02012 htim->Instance->SMCR &= ~TIM_SMCR_OCCS; 02013 } 02014 break; 02015 02016 case TIM_CLEARINPUTSOURCE_ETR: 02017 { 02018 /* Check the parameters */ 02019 assert_param(IS_TIM_CLEARINPUT_POLARITY(sClearInputConfig->ClearInputPolarity)); 02020 assert_param(IS_TIM_CLEARINPUT_PRESCALER(sClearInputConfig->ClearInputPrescaler)); 02021 assert_param(IS_TIM_CLEARINPUT_FILTER(sClearInputConfig->ClearInputFilter)); 02022 02023 TIM_ETR_SetConfig(htim->Instance, 02024 sClearInputConfig->ClearInputPrescaler, 02025 sClearInputConfig->ClearInputPolarity, 02026 sClearInputConfig->ClearInputFilter); 02027 02028 /* Set the OCREF clear selection bit */ 02029 htim->Instance->SMCR |= TIM_SMCR_OCCS; 02030 } 02031 break; 02032 02033 default: 02034 break; 02035 } 02036 02037 switch (Channel) 02038 { 02039 case TIM_CHANNEL_1: 02040 { 02041 if(sClearInputConfig->ClearInputState != RESET) 02042 { 02043 /* Enable the OCREF clear feature for Channel 1 */ 02044 htim->Instance->CCMR1 |= TIM_CCMR1_OC1CE; 02045 } 02046 else 02047 { 02048 /* Disable the OCREF clear feature for Channel 1 */ 02049 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC1CE; 02050 } 02051 } 02052 break; 02053 case TIM_CHANNEL_2: 02054 { 02055 if(sClearInputConfig->ClearInputState != RESET) 02056 { 02057 /* Enable the OCREF clear feature for Channel 2 */ 02058 htim->Instance->CCMR1 |= TIM_CCMR1_OC2CE; 02059 } 02060 else 02061 { 02062 /* Disable the OCREF clear feature for Channel 2 */ 02063 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC2CE; 02064 } 02065 } 02066 break; 02067 case TIM_CHANNEL_3: 02068 { 02069 if(sClearInputConfig->ClearInputState != RESET) 02070 { 02071 /* Enable the OCREF clear feature for Channel 3 */ 02072 htim->Instance->CCMR2 |= TIM_CCMR2_OC3CE; 02073 } 02074 else 02075 { 02076 /* Disable the OCREF clear feature for Channel 3 */ 02077 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC3CE; 02078 } 02079 } 02080 break; 02081 case TIM_CHANNEL_4: 02082 { 02083 if(sClearInputConfig->ClearInputState != RESET) 02084 { 02085 /* Enable the OCREF clear feature for Channel 4 */ 02086 htim->Instance->CCMR2 |= TIM_CCMR2_OC4CE; 02087 } 02088 else 02089 { 02090 /* Disable the OCREF clear feature for Channel 4 */ 02091 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC4CE; 02092 } 02093 } 02094 break; 02095 case TIM_CHANNEL_5: 02096 { 02097 if(sClearInputConfig->ClearInputState != RESET) 02098 { 02099 /* Enable the OCREF clear feature for Channel 1 */ 02100 htim->Instance->CCMR3 |= TIM_CCMR3_OC5CE; 02101 } 02102 else 02103 { 02104 /* Disable the OCREF clear feature for Channel 1 */ 02105 htim->Instance->CCMR3 &= ~TIM_CCMR3_OC5CE; 02106 } 02107 } 02108 break; 02109 case TIM_CHANNEL_6: 02110 { 02111 if(sClearInputConfig->ClearInputState != RESET) 02112 { 02113 /* Enable the OCREF clear feature for Channel 1 */ 02114 htim->Instance->CCMR3 |= TIM_CCMR3_OC6CE; 02115 } 02116 else 02117 { 02118 /* Disable the OCREF clear feature for Channel 1 */ 02119 htim->Instance->CCMR3 &= ~TIM_CCMR3_OC6CE; 02120 } 02121 } 02122 break; 02123 default: 02124 break; 02125 } 02126 02127 __HAL_UNLOCK(htim); 02128 02129 return HAL_OK; 02130 } 02131 02132 /** 02133 * @brief Configures the TIM in master mode. 02134 * @param htim: TIM handle. 02135 * @param sMasterConfig: pointer to a TIM_MasterConfigTypeDef structure that 02136 * contains the selected trigger output (TRGO) and the Master/Slave 02137 * mode. 02138 * @retval HAL status 02139 */ 02140 HAL_StatusTypeDef HAL_TIMEx_MasterConfigSynchronization(TIM_HandleTypeDef *htim, 02141 TIM_MasterConfigTypeDef * sMasterConfig) 02142 { 02143 uint32_t tmpcr2; 02144 uint32_t tmpsmcr; 02145 02146 /* Check the parameters */ 02147 assert_param(IS_TIM_SYNCHRO_INSTANCE(htim->Instance)); 02148 assert_param(IS_TIM_TRGO_SOURCE(sMasterConfig->MasterOutputTrigger)); 02149 assert_param(IS_TIM_MSM_STATE(sMasterConfig->MasterSlaveMode)); 02150 02151 /* Check input state */ 02152 __HAL_LOCK(htim); 02153 02154 /* Get the TIMx CR2 register value */ 02155 tmpcr2 = htim->Instance->CR2; 02156 02157 /* Get the TIMx SMCR register value */ 02158 tmpsmcr = htim->Instance->SMCR; 02159 02160 /* If the timer supports ADC synchronization through TRGO2, set the master mode selection 2 */ 02161 if (IS_TIM_TRGO2_INSTANCE(htim->Instance)) 02162 { 02163 /* Check the parameters */ 02164 assert_param(IS_TIM_TRGO2_SOURCE(sMasterConfig->MasterOutputTrigger2)); 02165 02166 /* Clear the MMS2 bits */ 02167 tmpcr2 &= ~TIM_CR2_MMS2; 02168 /* Select the TRGO2 source*/ 02169 tmpcr2 |= sMasterConfig->MasterOutputTrigger2; 02170 } 02171 02172 /* Reset the MMS Bits */ 02173 tmpcr2 &= ~TIM_CR2_MMS; 02174 /* Select the TRGO source */ 02175 tmpcr2 |= sMasterConfig->MasterOutputTrigger; 02176 02177 /* Reset the MSM Bit */ 02178 tmpsmcr &= ~TIM_SMCR_MSM; 02179 /* Set master mode */ 02180 tmpsmcr |= sMasterConfig->MasterSlaveMode; 02181 02182 /* Update TIMx CR2 */ 02183 htim->Instance->CR2 = tmpcr2; 02184 02185 /* Update TIMx SMCR */ 02186 htim->Instance->SMCR = tmpsmcr; 02187 02188 __HAL_UNLOCK(htim); 02189 02190 return HAL_OK; 02191 } 02192 02193 /** 02194 * @brief Configures the Break feature, dead time, Lock level, OSSI/OSSR State 02195 * and the AOE(automatic output enable). 02196 * @param htim: TIM handle 02197 * @param sBreakDeadTimeConfig: pointer to a TIM_ConfigBreakDeadConfigTypeDef structure that 02198 * contains the BDTR Register configuration information for the TIM peripheral. 02199 * @retval HAL status 02200 */ 02201 HAL_StatusTypeDef HAL_TIMEx_ConfigBreakDeadTime(TIM_HandleTypeDef *htim, 02202 TIM_BreakDeadTimeConfigTypeDef * sBreakDeadTimeConfig) 02203 { 02204 uint32_t tmpbdtr = 0; 02205 02206 /* Check the parameters */ 02207 assert_param(IS_TIM_BREAK_INSTANCE(htim->Instance)); 02208 assert_param(IS_TIM_OSSR_STATE(sBreakDeadTimeConfig->OffStateRunMode)); 02209 assert_param(IS_TIM_OSSI_STATE(sBreakDeadTimeConfig->OffStateIDLEMode)); 02210 assert_param(IS_TIM_LOCK_LEVEL(sBreakDeadTimeConfig->LockLevel)); 02211 assert_param(IS_TIM_DEADTIME(sBreakDeadTimeConfig->DeadTime)); 02212 assert_param(IS_TIM_BREAK_STATE(sBreakDeadTimeConfig->BreakState)); 02213 assert_param(IS_TIM_BREAK_POLARITY(sBreakDeadTimeConfig->BreakPolarity)); 02214 assert_param(IS_TIM_BREAK_FILTER(sBreakDeadTimeConfig->BreakFilter)); 02215 assert_param(IS_TIM_AUTOMATIC_OUTPUT_STATE(sBreakDeadTimeConfig->AutomaticOutput)); 02216 02217 /* Check input state */ 02218 __HAL_LOCK(htim); 02219 02220 /* Set the Lock level, the Break enable Bit and the Polarity, the OSSR State, 02221 the OSSI State, the dead time value and the Automatic Output Enable Bit */ 02222 if (IS_TIM_BKIN2_INSTANCE(htim->Instance)) 02223 { 02224 assert_param(IS_TIM_BREAK2_STATE(sBreakDeadTimeConfig->Break2State)); 02225 assert_param(IS_TIM_BREAK2_POLARITY(sBreakDeadTimeConfig->Break2Polarity)); 02226 assert_param(IS_TIM_BREAK_FILTER(sBreakDeadTimeConfig->Break2Filter)); 02227 02228 /* Clear the BDTR bits */ 02229 tmpbdtr &= ~(TIM_BDTR_DTG | TIM_BDTR_LOCK | TIM_BDTR_OSSI | 02230 TIM_BDTR_OSSR | TIM_BDTR_BKE | TIM_BDTR_BKP | 02231 TIM_BDTR_AOE | TIM_BDTR_MOE | TIM_BDTR_BKF | 02232 TIM_BDTR_BK2F | TIM_BDTR_BK2E | TIM_BDTR_BK2P); 02233 02234 /* Set the BDTR bits */ 02235 tmpbdtr |= sBreakDeadTimeConfig->DeadTime; 02236 tmpbdtr |= sBreakDeadTimeConfig->LockLevel; 02237 tmpbdtr |= sBreakDeadTimeConfig->OffStateIDLEMode; 02238 tmpbdtr |= sBreakDeadTimeConfig->OffStateRunMode; 02239 tmpbdtr |= sBreakDeadTimeConfig->BreakState; 02240 tmpbdtr |= sBreakDeadTimeConfig->BreakPolarity; 02241 tmpbdtr |= sBreakDeadTimeConfig->AutomaticOutput; 02242 tmpbdtr |= (sBreakDeadTimeConfig->BreakFilter << BDTR_BKF_SHIFT); 02243 tmpbdtr |= (sBreakDeadTimeConfig->Break2Filter << BDTR_BK2F_SHIFT); 02244 tmpbdtr |= sBreakDeadTimeConfig->Break2State; 02245 tmpbdtr |= sBreakDeadTimeConfig->Break2Polarity; 02246 } 02247 else 02248 { 02249 /* Clear the BDTR bits */ 02250 tmpbdtr &= ~(TIM_BDTR_DTG | TIM_BDTR_LOCK | TIM_BDTR_OSSI | 02251 TIM_BDTR_OSSR | TIM_BDTR_BKE | TIM_BDTR_BKP | 02252 TIM_BDTR_AOE | TIM_BDTR_MOE | TIM_BDTR_BKF); 02253 02254 /* Set the BDTR bits */ 02255 tmpbdtr |= sBreakDeadTimeConfig->DeadTime; 02256 tmpbdtr |= sBreakDeadTimeConfig->LockLevel; 02257 tmpbdtr |= sBreakDeadTimeConfig->OffStateIDLEMode; 02258 tmpbdtr |= sBreakDeadTimeConfig->OffStateRunMode; 02259 tmpbdtr |= sBreakDeadTimeConfig->BreakState; 02260 tmpbdtr |= sBreakDeadTimeConfig->BreakPolarity; 02261 tmpbdtr |= sBreakDeadTimeConfig->AutomaticOutput; 02262 tmpbdtr |= (sBreakDeadTimeConfig->BreakFilter << BDTR_BKF_SHIFT); 02263 } 02264 02265 /* Set TIMx_BDTR */ 02266 htim->Instance->BDTR = tmpbdtr; 02267 02268 __HAL_UNLOCK(htim); 02269 02270 return HAL_OK; 02271 } 02272 02273 /** 02274 * @brief Configures the break input source. 02275 * @param htim: TIM handle. 02276 * @param BreakInput: Break input to configure 02277 * This parameter can be one of the following values: 02278 * @arg TIM_BREAKINPUT_BRK: Timer break input 02279 * @arg TIM_BREAKINPUT_BRK2: Timer break 2 input 02280 * @param sBreakInputConfig: Break input source configuration 02281 * @retval HAL status 02282 */ 02283 HAL_StatusTypeDef HAL_TIMEx_ConfigBreakInput(TIM_HandleTypeDef *htim, 02284 uint32_t BreakInput, 02285 TIMEx_BreakInputConfigTypeDef *sBreakInputConfig) 02286 02287 { 02288 uint32_t tmporx = 0; 02289 uint32_t bkin_enable_mask = 0; 02290 uint32_t bkin_polarity_mask = 0; 02291 uint32_t bkin_enable_bitpos = 0; 02292 uint32_t bkin_polarity_bitpos = 0; 02293 02294 /* Check the parameters */ 02295 assert_param(IS_TIM_BREAK_INSTANCE(htim->Instance)); 02296 assert_param(IS_TIM_BREAKINPUT(BreakInput)); 02297 assert_param(IS_TIM_BREAKINPUTSOURCE(sBreakInputConfig->Source)); 02298 assert_param(IS_TIM_BREAKINPUTSOURCE_STATE(sBreakInputConfig->Enable)); 02299 if (sBreakInputConfig->Source != TIM_BREAKINPUTSOURCE_DFSDM) 02300 { 02301 assert_param(IS_TIM_BREAKINPUTSOURCE_POLARITY(sBreakInputConfig->Polarity)); 02302 } 02303 02304 /* Check input state */ 02305 __HAL_LOCK(htim); 02306 02307 switch(sBreakInputConfig->Source) 02308 { 02309 case TIM_BREAKINPUTSOURCE_BKIN: 02310 { 02311 bkin_enable_mask = TIM1_OR2_BKINE; 02312 bkin_enable_bitpos = 0; 02313 bkin_polarity_mask = TIM1_OR2_BKINP; 02314 bkin_polarity_bitpos = 9; 02315 } 02316 break; 02317 case TIM_BREAKINPUTSOURCE_COMP1: 02318 { 02319 bkin_enable_mask = TIM1_OR2_BKCMP1E; 02320 bkin_enable_bitpos = 1; 02321 bkin_polarity_mask = TIM1_OR2_BKCMP1P; 02322 bkin_polarity_bitpos = 10; 02323 } 02324 break; 02325 case TIM_BREAKINPUTSOURCE_COMP2: 02326 { 02327 bkin_enable_mask = TIM1_OR2_BKCMP2E; 02328 bkin_enable_bitpos = 2; 02329 bkin_polarity_mask = TIM1_OR2_BKCMP2P; 02330 bkin_polarity_bitpos = 11; 02331 } 02332 break; 02333 case TIM_BREAKINPUTSOURCE_DFSDM: 02334 { 02335 bkin_enable_mask = TIM1_OR2_BKDFBK0E; 02336 bkin_enable_bitpos = 8; 02337 } 02338 break; 02339 default: 02340 break; 02341 } 02342 02343 switch(BreakInput) 02344 { 02345 case TIM_BREAKINPUT_BRK: 02346 { 02347 /* Get the TIMx_OR2 register value */ 02348 tmporx = htim->Instance->OR2; 02349 02350 /* Enable the break input */ 02351 tmporx &= ~bkin_enable_mask; 02352 tmporx |= (sBreakInputConfig->Enable << bkin_enable_bitpos) & bkin_enable_mask; 02353 02354 /* Set the break input polarity */ 02355 if (sBreakInputConfig->Source != TIM_BREAKINPUTSOURCE_DFSDM) 02356 { 02357 tmporx &= ~bkin_polarity_mask; 02358 tmporx |= (sBreakInputConfig->Polarity << bkin_polarity_bitpos) & bkin_polarity_mask; 02359 } 02360 02361 /* Set TIMx_OR2 */ 02362 htim->Instance->OR2 = tmporx; 02363 } 02364 break; 02365 case TIM_BREAKINPUT_BRK2: 02366 { 02367 /* Get the TIMx_OR3 register value */ 02368 tmporx = htim->Instance->OR3; 02369 02370 /* Enable the break input */ 02371 tmporx &= ~bkin_enable_mask; 02372 tmporx |= (sBreakInputConfig->Enable << bkin_enable_bitpos) & bkin_enable_mask; 02373 02374 /* Set the break input polarity */ 02375 if (sBreakInputConfig->Source != TIM_BREAKINPUTSOURCE_DFSDM) 02376 { 02377 tmporx &= ~bkin_polarity_mask; 02378 tmporx |= (sBreakInputConfig->Polarity << bkin_polarity_bitpos) & bkin_polarity_mask; 02379 } 02380 02381 /* Set TIMx_OR3 */ 02382 htim->Instance->OR3 = tmporx; 02383 } 02384 break; 02385 default: 02386 break; 02387 } 02388 02389 __HAL_UNLOCK(htim); 02390 02391 return HAL_OK; 02392 } 02393 02394 /** 02395 * @brief Configures the TIMx Remapping input capabilities. 02396 * @param htim: TIM handle. 02397 * @param Remap: specifies the TIM remapping source. 02398 * For TIM1, the parameter is a combination of 4 fields (field1 | field2 | field3 | field4): 02399 * field1 can have the following values: 02400 * @arg TIM_TIM1_ETR_ADC1_NONE: TIM1_ETR is not connected to any ADC1 AWD (analog watchdog) 02401 * @arg TIM_TIM1_ETR_ADC1_AWD1: TIM1_ETR is connected to ADC1 AWD1 02402 * @arg TIM_TIM1_ETR_ADC1_AWD2: TIM1_ETR is connected to ADC1 AWD2 02403 * @arg TIM_TIM1_ETR_ADC1_AWD3: TIM1_ETR is connected to ADC1 AWD3 02404 * field2 can have the following values: 02405 * @arg TIM_TIM1_ETR_ADC3_NONE: TIM1_ETR is not connected to any ADC3 AWD (analog watchdog) 02406 * @arg TIM_TIM1_ETR_ADC3_AWD1: TIM1_ETR is connected to ADC3 AWD1 02407 * @arg TIM_TIM1_ETR_ADC3_AWD2: TIM1_ETR is connected to ADC3 AWD2 02408 * @arg TIM_TIM1_ETR_ADC3_AWD3: TIM1_ETR is connected to ADC3 AWD3 02409 * field3 can have the following values: 02410 * @arg TIM_TIM1_TI1_GPIO: TIM1 TI1 is connected to GPIO 02411 * @arg TIM_TIM1_TI1_COMP1: TIM1 TI1 is connected to COMP1 output 02412 * field4 can have the following values: 02413 * @arg TIM_TIM1_ETR_COMP1: TIM1_ETR is connected to COMP1 output 02414 * @arg TIM_TIM1_ETR_COMP2: TIM1_ETR is connected to COMP2 output 02415 * @note When field4 is set to TIM_TIM1_ETR_COMP1 or TIM_TIM1_ETR_COMP2 field1 and field2 values are not significant 02416 * 02417 * For TIM2, the parameter is a combination of 3 fields (field1 | field2 | field3): 02418 * field1 can have the following values: 02419 * @arg TIM_TIM2_ITR1_TIM8_TRGO: TIM2_ITR1 is connected to TIM8_TRGO 02420 * @arg TIM_TIM2_ITR1_OTG_FS_SOF: TIM2_ITR1 is connected to OTG_FS SOF 02421 * field2 can have the following values: 02422 * @arg TIM_TIM2_ETR_GPIO: TIM2_ETR is connected to GPIO 02423 * @arg TIM_TIM2_ETR_LSE: TIM2_ETR is connected to LSE 02424 * @arg TIM_TIM2_ETR_COMP1: TIM2_ETR is connected to COMP1 output 02425 * @arg TIM_TIM2_ETR_COMP2: TIM2_ETR is connected to COMP2 output 02426 * field3 can have the following values: 02427 * @arg TIM_TIM2_TI4_GPIO: TIM2 TI4 is connected to GPIO 02428 * @arg TIM_TIM2_TI4_COMP1: TIM2 TI4 is connected to COMP1 output 02429 * @arg TIM_TIM2_TI4_COMP2: TIM2 TI4 is connected to COMP2 output 02430 * @arg TIM_TIM2_TI4_COMP1_COMP2: TIM2 TI4 is connected to logical OR between COMP1 and COMP2 output 02431 * 02432 * For TIM3, the parameter is a combination 2 fields(field1 | field2): 02433 * field1 can have the following values: 02434 * @arg TIM_TIM3_TI1_GPIO: TIM3 TI1 is connected to GPIO 02435 * @arg TIM_TIM3_TI1_COMP1: TIM3 TI1 is connected to COMP1 output 02436 * @arg TIM_TIM3_TI1_COMP2: TIM3 TI1 is connected to COMP2 output 02437 * @arg TIM_TIM3_TI1_COMP1_COMP2: TIM3 TI1 is connected to logical OR between COMP1 and COMP2 output 02438 * field2 can have the following values: 02439 * @arg TIM_TIM3_ETR_GPIO: TIM3_ETR is connected to GPIO 02440 * @arg TIM_TIM3_ETR_COMP1: TIM3_ETR is connected to COMP1 output 02441 * 02442 * For TIM8, the parameter is a combination of 3 fields (field1 | field2 | field3): 02443 * field1 can have the following values: 02444 * @arg TIM_TIM8_ETR_ADC2_NONE: TIM8_ETR is not connected to any ADC2 AWD (analog watchdog) 02445 * @arg TIM_TIM8_ETR_ADC2_AWD1: TIM8_ETR is connected to ADC2 AWD1 02446 * @arg TIM_TIM8_ETR_ADC2_AWD2: TIM8_ETR is connected to ADC2 AWD2 02447 * @arg TIM_TIM8_ETR_ADC2_AWD3: TIM8_ETR is connected to ADC2 AWD3 02448 * field2 can have the following values: 02449 * @arg TIM_TIM8_ETR_ADC3_NONE: TIM8_ETR is not connected to any ADC3 AWD (analog watchdog) 02450 * @arg TIM_TIM8_ETR_ADC3_AWD1: TIM8_ETR is connected to ADC3 AWD1 02451 * @arg TIM_TIM8_ETR_ADC3_AWD2: TIM8_ETR is connected to ADC3 AWD2 02452 * @arg TIM_TIM8_ETR_ADC3_AWD3: TIM8_ETR is connected to ADC3 AWD3 02453 * field3 can have the following values: 02454 * @arg TIM_TIM8_TI1_GPIO: TIM8 TI1 is connected to GPIO 02455 * @arg TIM_TIM8_TI1_COMP2: TIM8 TI1 is connected to COMP2 output 02456 * field4 can have the following values: 02457 * @arg TIM_TIM8_ETR_COMP1: TIM8_ETR is connected to COMP1 output 02458 * @arg TIM_TIM8_ETR_COMP2: TIM8_ETR is connected to COMP2 output 02459 * @note When field4 is set to TIM_TIM8_ETR_COMP1 or TIM_TIM8_ETR_COMP2 field1 and field2 values are not significant 02460 * 02461 * For TIM15, the parameter is a combination of 3 fields (field1 | field2): 02462 * field1 can have the following values: 02463 * @arg TIM_TIM15_TI1_GPIO: TIM15 TI1 is connected to GPIO 02464 * @arg TIM_TIM15_TI1_LSE: TIM15 TI1 is connected to LSE 02465 * field2 can have the following values: 02466 * @arg TIM_TIM15_ENCODERMODE_NONE: No redirection 02467 * @arg TIM_TIM15_ENCODERMODE_TIM2: TIM2 IC1 and TIM2 IC2 are connected to TIM15 IC1 and TIM15 IC2 respectively 02468 * @arg TIM_TIM15_ENCODERMODE_TIM3: TIM3 IC1 and TIM3 IC2 are connected to TIM15 IC1 and TIM15 IC2 respectively 02469 * @arg TIM_TIM15_ENCODERMODE_TIM4: TIM4 IC1 and TIM4 IC2 are connected to TIM15 IC1 and TIM15 IC2 respectively 02470 * 02471 * For TIM16, the parameter can have the following values: 02472 * @arg TIM_TIM16_TI1_GPIO: TIM16 TI1 is connected to GPIO 02473 * @arg TIM_TIM16_TI1_LSI: TIM16 TI1 is connected to LSI 02474 * @arg TIM_TIM16_TI1_LSE: TIM16 TI1 is connected to LSE 02475 * @arg TIM_TIM16_TI1_RTC: TIM16 TI1 is connected to RTC wakeup interrupt 02476 * 02477 * For TIM17, the parameter can have the following values: 02478 * @arg TIM_TIM17_TI1_GPIO: TIM17 TI1 is connected to GPIO 02479 * @arg TIM_TIM17_TI1_MSI: TIM17 TI1 is connected to MSI 02480 * @arg TIM_TIM17_TI1_HSE_32: TIM17 TI1 is connected to HSE div 32 02481 * @arg TIM_TIM17_TI1_MCO: TIM17 TI1 is connected to MCO 02482 * 02483 * @retval HAL status 02484 */ 02485 HAL_StatusTypeDef HAL_TIMEx_RemapConfig(TIM_HandleTypeDef *htim, uint32_t Remap) 02486 { 02487 uint32_t tmpor1 = 0; 02488 uint32_t tmpor2 = 0; 02489 02490 __HAL_LOCK(htim); 02491 02492 /* Check parameters */ 02493 assert_param(IS_TIM_REMAP_INSTANCE(htim->Instance)); 02494 assert_param(IS_TIM_REMAP(Remap)); 02495 02496 /* Set ETR_SEL bit field (if required) */ 02497 if (IS_TIM_ETRSEL_INSTANCE(htim->Instance)) 02498 { 02499 tmpor2 = htim->Instance->OR2; 02500 tmpor2 &= ~TIMx_ETRSEL_MASK; 02501 tmpor2 |= (Remap & TIMx_ETRSEL_MASK); 02502 02503 /* Set TIMx_OR2 */ 02504 htim->Instance->OR2 = tmpor2; 02505 } 02506 02507 /* Set other remapping capabilities */ 02508 tmpor1 = Remap; 02509 tmpor1 &= ~TIMx_ETRSEL_MASK; 02510 02511 /* Set TIMx_OR1 */ 02512 htim->Instance->OR1 = Remap; 02513 02514 /* Set TIMx_OR1 */ 02515 htim->Instance->OR1 = tmpor1; 02516 02517 htim->State = HAL_TIM_STATE_READY; 02518 02519 __HAL_UNLOCK(htim); 02520 02521 return HAL_OK; 02522 } 02523 02524 /** 02525 * @brief Group channel 5 and channel 1, 2 or 3 02526 * @param htim: TIM handle. 02527 * @param Channels: specifies the reference signal(s) the OC5REF is combined with. 02528 * This parameter can be any combination of the following values: 02529 * TIM_GROUPCH5_NONE: No effect of OC5REF on OC1REFC, OC2REFC and OC3REFC 02530 * TIM_GROUPCH5_OC1REFC: OC1REFC is the logical AND of OC1REFC and OC5REF 02531 * TIM_GROUPCH5_OC2REFC: OC2REFC is the logical AND of OC2REFC and OC5REF 02532 * TIM_GROUPCH5_OC3REFC: OC3REFC is the logical AND of OC3REFC and OC5REF 02533 * @retval HAL status 02534 */ 02535 HAL_StatusTypeDef HAL_TIMEx_GroupChannel5(TIM_HandleTypeDef *htim, uint32_t Channels) 02536 { 02537 /* Check parameters */ 02538 assert_param(IS_TIM_COMBINED3PHASEPWM_INSTANCE(htim->Instance)); 02539 assert_param(IS_TIM_GROUPCH5(Channels)); 02540 02541 /* Process Locked */ 02542 __HAL_LOCK(htim); 02543 02544 htim->State = HAL_TIM_STATE_BUSY; 02545 02546 /* Clear GC5Cx bit fields */ 02547 htim->Instance->CCR5 &= ~(TIM_CCR5_GC5C3|TIM_CCR5_GC5C2|TIM_CCR5_GC5C1); 02548 02549 /* Set GC5Cx bit fields */ 02550 htim->Instance->CCR5 |= Channels; 02551 02552 htim->State = HAL_TIM_STATE_READY; 02553 02554 __HAL_UNLOCK(htim); 02555 02556 return HAL_OK; 02557 } 02558 02559 /** 02560 * @} 02561 */ 02562 02563 /** @defgroup TIMEx_Exported_Functions_Group6 Extended Callbacks functions 02564 * @brief Extended Callbacks functions 02565 * 02566 @verbatim 02567 ============================================================================== 02568 ##### Extended Callbacks functions ##### 02569 ============================================================================== 02570 [..] 02571 This section provides Extended TIM callback functions: 02572 (+) Timer Commutation callback 02573 (+) Timer Break callback 02574 02575 @endverbatim 02576 * @{ 02577 */ 02578 02579 /** 02580 * @brief Hall commutation changed callback in non-blocking mode 02581 * @param htim : TIM handle 02582 * @retval None 02583 */ 02584 __weak void HAL_TIMEx_CommutationCallback(TIM_HandleTypeDef *htim) 02585 { 02586 /* NOTE : This function should not be modified, when the callback is needed, 02587 the HAL_TIMEx_CommutationCallback could be implemented in the user file 02588 */ 02589 } 02590 02591 /** 02592 * @brief Hall Break detection callback in non-blocking mode 02593 * @param htim : TIM handle 02594 * @retval None 02595 */ 02596 __weak void HAL_TIMEx_BreakCallback(TIM_HandleTypeDef *htim) 02597 { 02598 /* NOTE : This function should not be modified, when the callback is needed, 02599 the HAL_TIMEx_BreakCallback could be implemented in the user file 02600 */ 02601 } 02602 02603 /** 02604 * @} 02605 */ 02606 02607 /** @defgroup TIMEx_Exported_Functions_Group7 Extended Peripheral State functions 02608 * @brief Extended Peripheral State functions 02609 * 02610 @verbatim 02611 ============================================================================== 02612 ##### Extended Peripheral State functions ##### 02613 ============================================================================== 02614 [..] 02615 This subsection permits to get in run-time the status of the peripheral 02616 and the data flow. 02617 02618 @endverbatim 02619 * @{ 02620 */ 02621 02622 /** 02623 * @brief Return the TIM Hall Sensor interface handle state. 02624 * @param htim: TIM Hall Sensor handle 02625 * @retval HAL state 02626 */ 02627 HAL_TIM_StateTypeDef HAL_TIMEx_HallSensor_GetState(TIM_HandleTypeDef *htim) 02628 { 02629 return htim->State; 02630 } 02631 02632 /** 02633 * @} 02634 */ 02635 02636 /** 02637 * @brief TIM DMA Commutation callback. 02638 * @param hdma : pointer to DMA handle. 02639 * @retval None 02640 */ 02641 void TIMEx_DMACommutationCplt(DMA_HandleTypeDef *hdma) 02642 { 02643 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 02644 02645 htim->State= HAL_TIM_STATE_READY; 02646 02647 HAL_TIMEx_CommutationCallback(htim); 02648 } 02649 02650 /** 02651 * @brief Enables or disables the TIM Capture Compare Channel xN. 02652 * @param TIMx to select the TIM peripheral 02653 * @param Channel: specifies the TIM Channel 02654 * This parameter can be one of the following values: 02655 * @arg TIM_Channel_1: TIM Channel 1 02656 * @arg TIM_Channel_2: TIM Channel 2 02657 * @arg TIM_Channel_3: TIM Channel 3 02658 * @param ChannelNState: specifies the TIM Channel CCxNE bit new state. 02659 * This parameter can be: TIM_CCxN_ENABLE or TIM_CCxN_Disable. 02660 * @retval None 02661 */ 02662 static void TIM_CCxNChannelCmd(TIM_TypeDef* TIMx, uint32_t Channel, uint32_t ChannelNState) 02663 { 02664 uint32_t tmp = 0; 02665 02666 tmp = TIM_CCER_CC1NE << Channel; 02667 02668 /* Reset the CCxNE Bit */ 02669 TIMx->CCER &= ~tmp; 02670 02671 /* Set or reset the CCxNE Bit */ 02672 TIMx->CCER |= (uint32_t)(ChannelNState << Channel); 02673 } 02674 02675 /** 02676 * @} 02677 */ 02678 02679 #endif /* HAL_TIM_MODULE_ENABLED */ 02680 /** 02681 * @} 02682 */ 02683 02684 /** 02685 * @} 02686 */ 02687 02688 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 02689
Generated on Tue Jul 12 2022 10:58:10 by
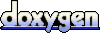