L4 HAL Drivers
Embed:
(wiki syntax)
Show/hide line numbers
stm32l4xx_hal_tim.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_hal_tim.c 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief TIM HAL module driver. 00008 * This file provides firmware functions to manage the following 00009 * functionalities of the Timer (TIM) peripheral: 00010 * + Time Base Initialization 00011 * + Time Base Start 00012 * + Time Base Start Interruption 00013 * + Time Base Start DMA 00014 * + Time Output Compare/PWM Initialization 00015 * + Time Output Compare/PWM Channel Configuration 00016 * + Time Output Compare/PWM Start 00017 * + Time Output Compare/PWM Start Interruption 00018 * + Time Output Compare/PWM Start DMA 00019 * + Time Input Capture Initialization 00020 * + Time Input Capture Channel Configuration 00021 * + Time Input Capture Start 00022 * + Time Input Capture Start Interruption 00023 * + Time Input Capture Start DMA 00024 * + Time One Pulse Initialization 00025 * + Time One Pulse Channel Configuration 00026 * + Time One Pulse Start 00027 * + Time Encoder Interface Initialization 00028 * + Time Encoder Interface Start 00029 * + Time Encoder Interface Start Interruption 00030 * + Time Encoder Interface Start DMA 00031 * + Commutation Event configuration with Interruption and DMA 00032 * + Time OCRef clear configuration 00033 * + Time External Clock configuration 00034 @verbatim 00035 ============================================================================== 00036 ##### TIMER Generic features ##### 00037 ============================================================================== 00038 [..] The Timer features include: 00039 (#) 16-bit up, down, up/down auto-reload counter. 00040 (#) 16-bit programmable prescaler allowing dividing (also on the fly) the 00041 counter clock frequency either by any factor between 1 and 65536. 00042 (#) Up to 4 independent channels for: 00043 (++) Input Capture 00044 (++) Output Compare 00045 (++) PWM generation (Edge and Center-aligned Mode) 00046 (++) One-pulse mode output 00047 00048 ##### How to use this driver ##### 00049 ============================================================================== 00050 [..] 00051 (#) Initialize the TIM low level resources by implementing the following functions 00052 depending on the selected feature: 00053 (++) Time Base : HAL_TIM_Base_MspInit() 00054 (++) Input Capture : HAL_TIM_IC_MspInit() 00055 (++) Output Compare : HAL_TIM_OC_MspInit() 00056 (++) PWM generation : HAL_TIM_PWM_MspInit() 00057 (++) One-pulse mode output : HAL_TIM_OnePulse_MspInit() 00058 (++) Encoder mode output : HAL_TIM_Encoder_MspInit() 00059 00060 (#) Initialize the TIM low level resources : 00061 (##) Enable the TIM interface clock using __HAL_RCC_TIMx_CLK_ENABLE(); 00062 (##) TIM pins configuration 00063 (+++) Enable the clock for the TIM GPIOs using the following function: 00064 __HAL_RCC_GPIOx_CLK_ENABLE(); 00065 (+++) Configure these TIM pins in Alternate function mode using HAL_GPIO_Init(); 00066 00067 (#) The external Clock can be configured, if needed (the default clock is the 00068 internal clock from the APBx), using the following function: 00069 HAL_TIM_ConfigClockSource, the clock configuration should be done before 00070 any start function. 00071 00072 (#) Configure the TIM in the desired functioning mode using one of the 00073 Initialization function of this driver: 00074 (++) HAL_TIM_Base_Init: to use the Timer to generate a simple time base 00075 (++) HAL_TIM_OC_Init and HAL_TIM_OC_ConfigChannel: to use the Timer to generate an 00076 Output Compare signal. 00077 (++) HAL_TIM_PWM_Init and HAL_TIM_PWM_ConfigChannel: to use the Timer to generate a 00078 PWM signal. 00079 (++) HAL_TIM_IC_Init and HAL_TIM_IC_ConfigChannel: to use the Timer to measure an 00080 external signal. 00081 (++) HAL_TIM_OnePulse_Init and HAL_TIM_OnePulse_ConfigChannel: to use the Timer 00082 in One Pulse Mode. 00083 (++) HAL_TIM_Encoder_Init: to use the Timer Encoder Interface. 00084 00085 (#) Activate the TIM peripheral using one of the start functions depending from the feature used: 00086 (++) Time Base : HAL_TIM_Base_Start(), HAL_TIM_Base_Start_DMA(), HAL_TIM_Base_Start_IT() 00087 (++) Input Capture : HAL_TIM_IC_Start(), HAL_TIM_IC_Start_DMA(), HAL_TIM_IC_Start_IT() 00088 (++) Output Compare : HAL_TIM_OC_Start(), HAL_TIM_OC_Start_DMA(), HAL_TIM_OC_Start_IT() 00089 (++) PWM generation : HAL_TIM_PWM_Start(), HAL_TIM_PWM_Start_DMA(), HAL_TIM_PWM_Start_IT() 00090 (++) One-pulse mode output : HAL_TIM_OnePulse_Start(), HAL_TIM_OnePulse_Start_IT() 00091 (++) Encoder mode output : HAL_TIM_Encoder_Start(), HAL_TIM_Encoder_Start_DMA(), HAL_TIM_Encoder_Start_IT(). 00092 00093 (#) The DMA Burst is managed with the two following functions: 00094 HAL_TIM_DMABurst_WriteStart() 00095 HAL_TIM_DMABurst_ReadStart() 00096 00097 @endverbatim 00098 ****************************************************************************** 00099 * @attention 00100 * 00101 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00102 * 00103 * Redistribution and use in source and binary forms, with or without modification, 00104 * are permitted provided that the following conditions are met: 00105 * 1. Redistributions of source code must retain the above copyright notice, 00106 * this list of conditions and the following disclaimer. 00107 * 2. Redistributions in binary form must reproduce the above copyright notice, 00108 * this list of conditions and the following disclaimer in the documentation 00109 * and/or other materials provided with the distribution. 00110 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00111 * may be used to endorse or promote products derived from this software 00112 * without specific prior written permission. 00113 * 00114 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00115 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00116 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00117 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00118 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00119 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00120 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00121 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00122 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00123 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00124 * 00125 ****************************************************************************** 00126 */ 00127 00128 /* Includes ------------------------------------------------------------------*/ 00129 #include "stm32l4xx_hal.h" 00130 00131 /** @addtogroup STM32L4xx_HAL_Driver 00132 * @{ 00133 */ 00134 00135 /** @defgroup TIM TIM 00136 * @brief TIM HAL module driver 00137 * @{ 00138 */ 00139 00140 #ifdef HAL_TIM_MODULE_ENABLED 00141 00142 /* Private typedef -----------------------------------------------------------*/ 00143 /* Private define ------------------------------------------------------------*/ 00144 /* Private macro -------------------------------------------------------------*/ 00145 /* Private variables ---------------------------------------------------------*/ 00146 /* Private function prototypes -----------------------------------------------*/ 00147 static void TIM_TI1_ConfigInputStage(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICFilter); 00148 static void TIM_TI2_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 00149 uint32_t TIM_ICFilter); 00150 static void TIM_TI2_ConfigInputStage(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICFilter); 00151 static void TIM_TI3_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 00152 uint32_t TIM_ICFilter); 00153 static void TIM_TI4_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 00154 uint32_t TIM_ICFilter); 00155 static void TIM_ITRx_SetConfig(TIM_TypeDef* TIMx, uint16_t InputTriggerSource); 00156 static void TIM_DMAPeriodElapsedCplt(DMA_HandleTypeDef *hdma); 00157 static void TIM_DMATriggerCplt(DMA_HandleTypeDef *hdma); 00158 static void TIM_SlaveTimer_SetConfig(TIM_HandleTypeDef *htim, 00159 TIM_SlaveConfigTypeDef * sSlaveConfig); 00160 /* Exported functions --------------------------------------------------------*/ 00161 00162 /** @defgroup TIM_Exported_Functions TIM Exported Functions 00163 * @{ 00164 */ 00165 00166 /** @defgroup TIM_Exported_Functions_Group1 Time Base functions 00167 * @brief Time Base functions 00168 * 00169 @verbatim 00170 ============================================================================== 00171 ##### Time Base functions ##### 00172 ============================================================================== 00173 [..] 00174 This section provides functions allowing to: 00175 (+) Initialize and configure the TIM base. 00176 (+) De-initialize the TIM base. 00177 (+) Start the Time Base. 00178 (+) Stop the Time Base. 00179 (+) Start the Time Base and enable interrupt. 00180 (+) Stop the Time Base and disable interrupt. 00181 (+) Start the Time Base and enable DMA transfer. 00182 (+) Stop the Time Base and disable DMA transfer. 00183 00184 @endverbatim 00185 * @{ 00186 */ 00187 /** 00188 * @brief Initializes the TIM Time base Unit according to the specified 00189 * parameters in the TIM_HandleTypeDef and initialize the associated handle. 00190 * @param htim: TIM Base handle 00191 * @retval HAL status 00192 */ 00193 HAL_StatusTypeDef HAL_TIM_Base_Init(TIM_HandleTypeDef *htim) 00194 { 00195 /* Check the TIM handle allocation */ 00196 if(htim == NULL) 00197 { 00198 return HAL_ERROR; 00199 } 00200 00201 /* Check the parameters */ 00202 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00203 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 00204 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 00205 00206 if(htim->State == HAL_TIM_STATE_RESET) 00207 { 00208 /* Allocate lock resource and initialize it */ 00209 htim->Lock = HAL_UNLOCKED; 00210 00211 /* Init the low level hardware : GPIO, CLOCK, NVIC */ 00212 HAL_TIM_Base_MspInit(htim); 00213 } 00214 00215 /* Set the TIM state */ 00216 htim->State= HAL_TIM_STATE_BUSY; 00217 00218 /* Set the Time Base configuration */ 00219 TIM_Base_SetConfig(htim->Instance, &htim->Init); 00220 00221 /* Initialize the TIM state*/ 00222 htim->State= HAL_TIM_STATE_READY; 00223 00224 return HAL_OK; 00225 } 00226 00227 /** 00228 * @brief DeInitialize the TIM Base peripheral 00229 * @param htim: TIM Base handle 00230 * @retval HAL status 00231 */ 00232 HAL_StatusTypeDef HAL_TIM_Base_DeInit(TIM_HandleTypeDef *htim) 00233 { 00234 /* Check the parameters */ 00235 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00236 00237 htim->State = HAL_TIM_STATE_BUSY; 00238 00239 /* Disable the TIM Peripheral Clock */ 00240 __HAL_TIM_DISABLE(htim); 00241 00242 /* DeInit the low level hardware: GPIO, CLOCK, NVIC */ 00243 HAL_TIM_Base_MspDeInit(htim); 00244 00245 /* Change TIM state */ 00246 htim->State = HAL_TIM_STATE_RESET; 00247 00248 /* Release Lock */ 00249 __HAL_UNLOCK(htim); 00250 00251 return HAL_OK; 00252 } 00253 00254 /** 00255 * @brief Initializes the TIM Base MSP. 00256 * @param htim: TIM handle 00257 * @retval None 00258 */ 00259 __weak void HAL_TIM_Base_MspInit(TIM_HandleTypeDef *htim) 00260 { 00261 /* NOTE : This function should not be modified, when the callback is needed, 00262 the HAL_TIM_Base_MspInit could be implemented in the user file 00263 */ 00264 } 00265 00266 /** 00267 * @brief DeInitialize TIM Base MSP. 00268 * @param htim: TIM handle 00269 * @retval None 00270 */ 00271 __weak void HAL_TIM_Base_MspDeInit(TIM_HandleTypeDef *htim) 00272 { 00273 /* NOTE : This function should not be modified, when the callback is needed, 00274 the HAL_TIM_Base_MspDeInit could be implemented in the user file 00275 */ 00276 } 00277 00278 00279 /** 00280 * @brief Starts the TIM Base generation. 00281 * @param htim : TIM handle 00282 * @retval HAL status 00283 */ 00284 HAL_StatusTypeDef HAL_TIM_Base_Start(TIM_HandleTypeDef *htim) 00285 { 00286 /* Check the parameters */ 00287 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00288 00289 /* Set the TIM state */ 00290 htim->State= HAL_TIM_STATE_BUSY; 00291 00292 /* Enable the Peripheral */ 00293 __HAL_TIM_ENABLE(htim); 00294 00295 /* Change the TIM state*/ 00296 htim->State= HAL_TIM_STATE_READY; 00297 00298 /* Return function status */ 00299 return HAL_OK; 00300 } 00301 00302 /** 00303 * @brief Stops the TIM Base generation. 00304 * @param htim : TIM handle 00305 * @retval HAL status 00306 */ 00307 HAL_StatusTypeDef HAL_TIM_Base_Stop(TIM_HandleTypeDef *htim) 00308 { 00309 /* Check the parameters */ 00310 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00311 00312 /* Set the TIM state */ 00313 htim->State= HAL_TIM_STATE_BUSY; 00314 00315 /* Disable the Peripheral */ 00316 __HAL_TIM_DISABLE(htim); 00317 00318 /* Change the TIM state*/ 00319 htim->State= HAL_TIM_STATE_READY; 00320 00321 /* Return function status */ 00322 return HAL_OK; 00323 } 00324 00325 /** 00326 * @brief Starts the TIM Base generation in interrupt mode. 00327 * @param htim : TIM handle 00328 * @retval HAL status 00329 */ 00330 HAL_StatusTypeDef HAL_TIM_Base_Start_IT(TIM_HandleTypeDef *htim) 00331 { 00332 /* Check the parameters */ 00333 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00334 00335 /* Enable the TIM Update interrupt */ 00336 __HAL_TIM_ENABLE_IT(htim, TIM_IT_UPDATE); 00337 00338 /* Enable the Peripheral */ 00339 __HAL_TIM_ENABLE(htim); 00340 00341 /* Return function status */ 00342 return HAL_OK; 00343 } 00344 00345 /** 00346 * @brief Stops the TIM Base generation in interrupt mode. 00347 * @param htim : TIM handle 00348 * @retval HAL status 00349 */ 00350 HAL_StatusTypeDef HAL_TIM_Base_Stop_IT(TIM_HandleTypeDef *htim) 00351 { 00352 /* Check the parameters */ 00353 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00354 /* Disable the TIM Update interrupt */ 00355 __HAL_TIM_DISABLE_IT(htim, TIM_IT_UPDATE); 00356 00357 /* Disable the Peripheral */ 00358 __HAL_TIM_DISABLE(htim); 00359 00360 /* Return function status */ 00361 return HAL_OK; 00362 } 00363 00364 /** 00365 * @brief Starts the TIM Base generation in DMA mode. 00366 * @param htim : TIM handle 00367 * @param pData: The source Buffer address. 00368 * @param Length: The length of data to be transferred from memory to peripheral. 00369 * @retval HAL status 00370 */ 00371 HAL_StatusTypeDef HAL_TIM_Base_Start_DMA(TIM_HandleTypeDef *htim, uint32_t *pData, uint16_t Length) 00372 { 00373 /* Check the parameters */ 00374 assert_param(IS_TIM_DMA_INSTANCE(htim->Instance)); 00375 00376 if((htim->State == HAL_TIM_STATE_BUSY)) 00377 { 00378 return HAL_BUSY; 00379 } 00380 else if((htim->State == HAL_TIM_STATE_READY)) 00381 { 00382 if((pData == 0 ) && (Length > 0)) 00383 { 00384 return HAL_ERROR; 00385 } 00386 else 00387 { 00388 htim->State = HAL_TIM_STATE_BUSY; 00389 } 00390 } 00391 /* Set the DMA Period elapsed callback */ 00392 htim->hdma[TIM_DMA_ID_UPDATE]->XferCpltCallback = TIM_DMAPeriodElapsedCplt; 00393 00394 /* Set the DMA error callback */ 00395 htim->hdma[TIM_DMA_ID_UPDATE]->XferErrorCallback = TIM_DMAError ; 00396 00397 /* Enable the DMA channel */ 00398 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_UPDATE], (uint32_t)pData, (uint32_t)&htim->Instance->ARR, Length); 00399 00400 /* Enable the TIM Update DMA request */ 00401 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_UPDATE); 00402 00403 /* Enable the Peripheral */ 00404 __HAL_TIM_ENABLE(htim); 00405 00406 /* Return function status */ 00407 return HAL_OK; 00408 } 00409 00410 /** 00411 * @brief Stops the TIM Base generation in DMA mode. 00412 * @param htim : TIM handle 00413 * @retval HAL status 00414 */ 00415 HAL_StatusTypeDef HAL_TIM_Base_Stop_DMA(TIM_HandleTypeDef *htim) 00416 { 00417 /* Check the parameters */ 00418 assert_param(IS_TIM_DMA_INSTANCE(htim->Instance)); 00419 00420 /* Disable the TIM Update DMA request */ 00421 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_UPDATE); 00422 00423 /* Disable the Peripheral */ 00424 __HAL_TIM_DISABLE(htim); 00425 00426 /* Change the htim state */ 00427 htim->State = HAL_TIM_STATE_READY; 00428 00429 /* Return function status */ 00430 return HAL_OK; 00431 } 00432 00433 /** 00434 * @} 00435 */ 00436 00437 /** @defgroup TIM_Exported_Functions_Group2 Time Output Compare functions 00438 * @brief Time Output Compare functions 00439 * 00440 @verbatim 00441 ============================================================================== 00442 ##### Time Output Compare functions ##### 00443 ============================================================================== 00444 [..] 00445 This section provides functions allowing to: 00446 (+) Initialize and configure the TIM Output Compare. 00447 (+) De-initialize the TIM Output Compare. 00448 (+) Start the Time Output Compare. 00449 (+) Stop the Time Output Compare. 00450 (+) Start the Time Output Compare and enable interrupt. 00451 (+) Stop the Time Output Compare and disable interrupt. 00452 (+) Start the Time Output Compare and enable DMA transfer. 00453 (+) Stop the Time Output Compare and disable DMA transfer. 00454 00455 @endverbatim 00456 * @{ 00457 */ 00458 /** 00459 * @brief Initializes the TIM Output Compare according to the specified 00460 * parameters in the TIM_HandleTypeDef and initialize the associated handle. 00461 * @param htim: TIM Output Compare handle 00462 * @retval HAL status 00463 */ 00464 HAL_StatusTypeDef HAL_TIM_OC_Init(TIM_HandleTypeDef* htim) 00465 { 00466 /* Check the TIM handle allocation */ 00467 if(htim == NULL) 00468 { 00469 return HAL_ERROR; 00470 } 00471 00472 /* Check the parameters */ 00473 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00474 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 00475 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 00476 00477 if(htim->State == HAL_TIM_STATE_RESET) 00478 { 00479 /* Allocate lock resource and initialize it */ 00480 htim->Lock = HAL_UNLOCKED; 00481 00482 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 00483 HAL_TIM_OC_MspInit(htim); 00484 } 00485 00486 /* Set the TIM state */ 00487 htim->State= HAL_TIM_STATE_BUSY; 00488 00489 /* Init the base time for the Output Compare */ 00490 TIM_Base_SetConfig(htim->Instance, &htim->Init); 00491 00492 /* Initialize the TIM state*/ 00493 htim->State= HAL_TIM_STATE_READY; 00494 00495 return HAL_OK; 00496 } 00497 00498 /** 00499 * @brief DeInitialize the TIM peripheral 00500 * @param htim: TIM Output Compare handle 00501 * @retval HAL status 00502 */ 00503 HAL_StatusTypeDef HAL_TIM_OC_DeInit(TIM_HandleTypeDef *htim) 00504 { 00505 /* Check the parameters */ 00506 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00507 00508 htim->State = HAL_TIM_STATE_BUSY; 00509 00510 /* Disable the TIM Peripheral Clock */ 00511 __HAL_TIM_DISABLE(htim); 00512 00513 /* DeInit the low level hardware: GPIO, CLOCK, NVIC and DMA */ 00514 HAL_TIM_OC_MspDeInit(htim); 00515 00516 /* Change TIM state */ 00517 htim->State = HAL_TIM_STATE_RESET; 00518 00519 /* Release Lock */ 00520 __HAL_UNLOCK(htim); 00521 00522 return HAL_OK; 00523 } 00524 00525 /** 00526 * @brief Initializes the TIM Output Compare MSP. 00527 * @param htim: TIM handle 00528 * @retval None 00529 */ 00530 __weak void HAL_TIM_OC_MspInit(TIM_HandleTypeDef *htim) 00531 { 00532 /* NOTE : This function should not be modified, when the callback is needed, 00533 the HAL_TIM_OC_MspInit could be implemented in the user file 00534 */ 00535 } 00536 00537 /** 00538 * @brief DeInitialize TIM Output Compare MSP. 00539 * @param htim: TIM handle 00540 * @retval None 00541 */ 00542 __weak void HAL_TIM_OC_MspDeInit(TIM_HandleTypeDef *htim) 00543 { 00544 /* NOTE : This function should not be modified, when the callback is needed, 00545 the HAL_TIM_OC_MspDeInit could be implemented in the user file 00546 */ 00547 } 00548 00549 /** 00550 * @brief Starts the TIM Output Compare signal generation. 00551 * @param htim : TIM Output Compare handle 00552 * @param Channel : TIM Channel to be enabled 00553 * This parameter can be one of the following values: 00554 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00555 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00556 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00557 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00558 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00559 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00560 * @retval HAL status 00561 */ 00562 HAL_StatusTypeDef HAL_TIM_OC_Start(TIM_HandleTypeDef *htim, uint32_t Channel) 00563 { 00564 /* Check the parameters */ 00565 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00566 00567 /* Enable the Output compare channel */ 00568 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 00569 00570 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00571 { 00572 /* Enable the main output */ 00573 __HAL_TIM_MOE_ENABLE(htim); 00574 } 00575 00576 /* Enable the Peripheral */ 00577 __HAL_TIM_ENABLE(htim); 00578 00579 /* Return function status */ 00580 return HAL_OK; 00581 } 00582 00583 /** 00584 * @brief Stops the TIM Output Compare signal generation. 00585 * @param htim : TIM handle 00586 * @param Channel : TIM Channel to be disabled 00587 * This parameter can be one of the following values: 00588 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00589 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00590 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00591 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00592 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00593 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00594 * @retval HAL status 00595 */ 00596 HAL_StatusTypeDef HAL_TIM_OC_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 00597 { 00598 /* Check the parameters */ 00599 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00600 00601 /* Disable the Output compare channel */ 00602 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 00603 00604 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00605 { 00606 /* Disable the Main Ouput */ 00607 __HAL_TIM_MOE_DISABLE(htim); 00608 } 00609 00610 /* Disable the Peripheral */ 00611 __HAL_TIM_DISABLE(htim); 00612 00613 /* Return function status */ 00614 return HAL_OK; 00615 } 00616 00617 /** 00618 * @brief Starts the TIM Output Compare signal generation in interrupt mode. 00619 * @param htim : TIM OC handle 00620 * @param Channel : TIM Channel to be enabled 00621 * This parameter can be one of the following values: 00622 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00623 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00624 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00625 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00626 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00627 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00628 * @retval HAL status 00629 */ 00630 HAL_StatusTypeDef HAL_TIM_OC_Start_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 00631 { 00632 /* Check the parameters */ 00633 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00634 00635 switch (Channel) 00636 { 00637 case TIM_CHANNEL_1: 00638 { 00639 /* Enable the TIM Capture/Compare 1 interrupt */ 00640 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 00641 } 00642 break; 00643 00644 case TIM_CHANNEL_2: 00645 { 00646 /* Enable the TIM Capture/Compare 2 interrupt */ 00647 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 00648 } 00649 break; 00650 00651 case TIM_CHANNEL_3: 00652 { 00653 /* Enable the TIM Capture/Compare 3 interrupt */ 00654 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC3); 00655 } 00656 break; 00657 00658 case TIM_CHANNEL_4: 00659 { 00660 /* Enable the TIM Capture/Compare 4 interrupt */ 00661 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC4); 00662 } 00663 break; 00664 00665 default: 00666 break; 00667 } 00668 00669 /* Enable the Output compare channel */ 00670 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 00671 00672 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00673 { 00674 /* Enable the main output */ 00675 __HAL_TIM_MOE_ENABLE(htim); 00676 } 00677 00678 /* Enable the Peripheral */ 00679 __HAL_TIM_ENABLE(htim); 00680 00681 /* Return function status */ 00682 return HAL_OK; 00683 } 00684 00685 /** 00686 * @brief Stops the TIM Output Compare signal generation in interrupt mode. 00687 * @param htim : TIM Output Compare handle 00688 * @param Channel : TIM Channel to be disabled 00689 * This parameter can be one of the following values: 00690 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00691 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00692 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00693 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00694 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00695 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00696 * @retval HAL status 00697 */ 00698 HAL_StatusTypeDef HAL_TIM_OC_Stop_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 00699 { 00700 /* Check the parameters */ 00701 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00702 00703 switch (Channel) 00704 { 00705 case TIM_CHANNEL_1: 00706 { 00707 /* Disable the TIM Capture/Compare 1 interrupt */ 00708 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 00709 } 00710 break; 00711 00712 case TIM_CHANNEL_2: 00713 { 00714 /* Disable the TIM Capture/Compare 2 interrupt */ 00715 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 00716 } 00717 break; 00718 00719 case TIM_CHANNEL_3: 00720 { 00721 /* Disable the TIM Capture/Compare 3 interrupt */ 00722 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC3); 00723 } 00724 break; 00725 00726 case TIM_CHANNEL_4: 00727 { 00728 /* Disable the TIM Capture/Compare 4 interrupt */ 00729 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC4); 00730 } 00731 break; 00732 00733 default: 00734 break; 00735 } 00736 00737 /* Disable the Output compare channel */ 00738 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 00739 00740 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00741 { 00742 /* Disable the Main Ouput */ 00743 __HAL_TIM_MOE_DISABLE(htim); 00744 } 00745 00746 /* Disable the Peripheral */ 00747 __HAL_TIM_DISABLE(htim); 00748 00749 /* Return function status */ 00750 return HAL_OK; 00751 } 00752 00753 /** 00754 * @brief Starts the TIM Output Compare signal generation in DMA mode. 00755 * @param htim : TIM Output Compare handle 00756 * @param Channel : TIM Channel to be enabled 00757 * This parameter can be one of the following values: 00758 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00759 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00760 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00761 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00762 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00763 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00764 * @param pData: The source Buffer address. 00765 * @param Length: The length of data to be transferred from memory to TIM peripheral 00766 * @retval HAL status 00767 */ 00768 HAL_StatusTypeDef HAL_TIM_OC_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData, uint16_t Length) 00769 { 00770 /* Check the parameters */ 00771 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00772 00773 if((htim->State == HAL_TIM_STATE_BUSY)) 00774 { 00775 return HAL_BUSY; 00776 } 00777 else if((htim->State == HAL_TIM_STATE_READY)) 00778 { 00779 if(((uint32_t)pData == 0 ) && (Length > 0)) 00780 { 00781 return HAL_ERROR; 00782 } 00783 else 00784 { 00785 htim->State = HAL_TIM_STATE_BUSY; 00786 } 00787 } 00788 switch (Channel) 00789 { 00790 case TIM_CHANNEL_1: 00791 { 00792 /* Set the DMA Period elapsed callback */ 00793 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMADelayPulseCplt; 00794 00795 /* Set the DMA error callback */ 00796 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 00797 00798 /* Enable the DMA channel */ 00799 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)pData, (uint32_t)&htim->Instance->CCR1, Length); 00800 00801 /* Enable the TIM Capture/Compare 1 DMA request */ 00802 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 00803 } 00804 break; 00805 00806 case TIM_CHANNEL_2: 00807 { 00808 /* Set the DMA Period elapsed callback */ 00809 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMADelayPulseCplt; 00810 00811 /* Set the DMA error callback */ 00812 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 00813 00814 /* Enable the DMA channel */ 00815 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)pData, (uint32_t)&htim->Instance->CCR2, Length); 00816 00817 /* Enable the TIM Capture/Compare 2 DMA request */ 00818 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 00819 } 00820 break; 00821 00822 case TIM_CHANNEL_3: 00823 { 00824 /* Set the DMA Period elapsed callback */ 00825 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMADelayPulseCplt; 00826 00827 /* Set the DMA error callback */ 00828 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 00829 00830 /* Enable the DMA channel */ 00831 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)pData, (uint32_t)&htim->Instance->CCR3,Length); 00832 00833 /* Enable the TIM Capture/Compare 3 DMA request */ 00834 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC3); 00835 } 00836 break; 00837 00838 case TIM_CHANNEL_4: 00839 { 00840 /* Set the DMA Period elapsed callback */ 00841 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMADelayPulseCplt; 00842 00843 /* Set the DMA error callback */ 00844 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 00845 00846 /* Enable the DMA channel */ 00847 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)pData, (uint32_t)&htim->Instance->CCR4, Length); 00848 00849 /* Enable the TIM Capture/Compare 4 DMA request */ 00850 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC4); 00851 } 00852 break; 00853 00854 default: 00855 break; 00856 } 00857 00858 /* Enable the Output compare channel */ 00859 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 00860 00861 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00862 { 00863 /* Enable the main output */ 00864 __HAL_TIM_MOE_ENABLE(htim); 00865 } 00866 00867 /* Enable the Peripheral */ 00868 __HAL_TIM_ENABLE(htim); 00869 00870 /* Return function status */ 00871 return HAL_OK; 00872 } 00873 00874 /** 00875 * @brief Stops the TIM Output Compare signal generation in DMA mode. 00876 * @param htim : TIM Output Compare handle 00877 * @param Channel : TIM Channel to be disabled 00878 * This parameter can be one of the following values: 00879 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 00880 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 00881 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 00882 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 00883 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 00884 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 00885 * @retval HAL status 00886 */ 00887 HAL_StatusTypeDef HAL_TIM_OC_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 00888 { 00889 /* Check the parameters */ 00890 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 00891 00892 switch (Channel) 00893 { 00894 case TIM_CHANNEL_1: 00895 { 00896 /* Disable the TIM Capture/Compare 1 DMA request */ 00897 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 00898 } 00899 break; 00900 00901 case TIM_CHANNEL_2: 00902 { 00903 /* Disable the TIM Capture/Compare 2 DMA request */ 00904 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 00905 } 00906 break; 00907 00908 case TIM_CHANNEL_3: 00909 { 00910 /* Disable the TIM Capture/Compare 3 DMA request */ 00911 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC3); 00912 } 00913 break; 00914 00915 case TIM_CHANNEL_4: 00916 { 00917 /* Disable the TIM Capture/Compare 4 interrupt */ 00918 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC4); 00919 } 00920 break; 00921 00922 default: 00923 break; 00924 } 00925 00926 /* Disable the Output compare channel */ 00927 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 00928 00929 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 00930 { 00931 /* Disable the Main Ouput */ 00932 __HAL_TIM_MOE_DISABLE(htim); 00933 } 00934 00935 /* Disable the Peripheral */ 00936 __HAL_TIM_DISABLE(htim); 00937 00938 /* Change the htim state */ 00939 htim->State = HAL_TIM_STATE_READY; 00940 00941 /* Return function status */ 00942 return HAL_OK; 00943 } 00944 00945 /** 00946 * @} 00947 */ 00948 00949 /** @defgroup TIM_Exported_Functions_Group3 Time PWM functions 00950 * @brief Time PWM functions 00951 * 00952 @verbatim 00953 ============================================================================== 00954 ##### Time PWM functions ##### 00955 ============================================================================== 00956 [..] 00957 This section provides functions allowing to: 00958 (+) Initialize and configure the TIM OPWM. 00959 (+) De-initialize the TIM PWM. 00960 (+) Start the Time PWM. 00961 (+) Stop the Time PWM. 00962 (+) Start the Time PWM and enable interrupt. 00963 (+) Stop the Time PWM and disable interrupt. 00964 (+) Start the Time PWM and enable DMA transfer. 00965 (+) Stop the Time PWM and disable DMA transfer. 00966 00967 @endverbatim 00968 * @{ 00969 */ 00970 /** 00971 * @brief Initializes the TIM PWM Time Base according to the specified 00972 * parameters in the TIM_HandleTypeDef and initialize the associated handle. 00973 * @param htim: TIM handle 00974 * @retval HAL status 00975 */ 00976 HAL_StatusTypeDef HAL_TIM_PWM_Init(TIM_HandleTypeDef *htim) 00977 { 00978 /* Check the TIM handle allocation */ 00979 if(htim == NULL) 00980 { 00981 return HAL_ERROR; 00982 } 00983 00984 /* Check the parameters */ 00985 assert_param(IS_TIM_INSTANCE(htim->Instance)); 00986 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 00987 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 00988 00989 if(htim->State == HAL_TIM_STATE_RESET) 00990 { 00991 /* Allocate lock resource and initialize it */ 00992 htim->Lock = HAL_UNLOCKED; 00993 00994 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 00995 HAL_TIM_PWM_MspInit(htim); 00996 } 00997 00998 /* Set the TIM state */ 00999 htim->State= HAL_TIM_STATE_BUSY; 01000 01001 /* Init the base time for the PWM */ 01002 TIM_Base_SetConfig(htim->Instance, &htim->Init); 01003 01004 /* Initialize the TIM state*/ 01005 htim->State= HAL_TIM_STATE_READY; 01006 01007 return HAL_OK; 01008 } 01009 01010 /** 01011 * @brief DeInitialize the TIM peripheral 01012 * @param htim: TIM handle 01013 * @retval HAL status 01014 */ 01015 HAL_StatusTypeDef HAL_TIM_PWM_DeInit(TIM_HandleTypeDef *htim) 01016 { 01017 /* Check the parameters */ 01018 assert_param(IS_TIM_INSTANCE(htim->Instance)); 01019 01020 htim->State = HAL_TIM_STATE_BUSY; 01021 01022 /* Disable the TIM Peripheral Clock */ 01023 __HAL_TIM_DISABLE(htim); 01024 01025 /* DeInit the low level hardware: GPIO, CLOCK, NVIC and DMA */ 01026 HAL_TIM_PWM_MspDeInit(htim); 01027 01028 /* Change TIM state */ 01029 htim->State = HAL_TIM_STATE_RESET; 01030 01031 /* Release Lock */ 01032 __HAL_UNLOCK(htim); 01033 01034 return HAL_OK; 01035 } 01036 01037 /** 01038 * @brief Initializes the TIM PWM MSP. 01039 * @param htim: TIM handle 01040 * @retval None 01041 */ 01042 __weak void HAL_TIM_PWM_MspInit(TIM_HandleTypeDef *htim) 01043 { 01044 /* NOTE : This function should not be modified, when the callback is needed, 01045 the HAL_TIM_PWM_MspInit could be implemented in the user file 01046 */ 01047 } 01048 01049 /** 01050 * @brief DeInitialize TIM PWM MSP. 01051 * @param htim: TIM handle 01052 * @retval None 01053 */ 01054 __weak void HAL_TIM_PWM_MspDeInit(TIM_HandleTypeDef *htim) 01055 { 01056 /* NOTE : This function should not be modified, when the callback is needed, 01057 the HAL_TIM_PWM_MspDeInit could be implemented in the user file 01058 */ 01059 } 01060 01061 /** 01062 * @brief Starts the PWM signal generation. 01063 * @param htim : TIM handle 01064 * @param Channel : TIM Channels to be enabled 01065 * This parameter can be one of the following values: 01066 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01067 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01068 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01069 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01070 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 01071 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 01072 * @retval HAL status 01073 */ 01074 HAL_StatusTypeDef HAL_TIM_PWM_Start(TIM_HandleTypeDef *htim, uint32_t Channel) 01075 { 01076 /* Check the parameters */ 01077 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01078 01079 /* Enable the Capture compare channel */ 01080 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01081 01082 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01083 { 01084 /* Enable the main output */ 01085 __HAL_TIM_MOE_ENABLE(htim); 01086 } 01087 01088 /* Enable the Peripheral */ 01089 __HAL_TIM_ENABLE(htim); 01090 01091 /* Return function status */ 01092 return HAL_OK; 01093 } 01094 01095 /** 01096 * @brief Stops the PWM signal generation. 01097 * @param htim : TIM handle 01098 * @param Channel : TIM Channels to be disabled 01099 * This parameter can be one of the following values: 01100 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01101 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01102 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01103 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01104 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 01105 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 01106 * @retval HAL status 01107 */ 01108 HAL_StatusTypeDef HAL_TIM_PWM_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 01109 { 01110 /* Check the parameters */ 01111 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01112 01113 /* Disable the Capture compare channel */ 01114 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01115 01116 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01117 { 01118 /* Disable the Main Ouput */ 01119 __HAL_TIM_MOE_DISABLE(htim); 01120 } 01121 01122 /* Disable the Peripheral */ 01123 __HAL_TIM_DISABLE(htim); 01124 01125 /* Change the htim state */ 01126 htim->State = HAL_TIM_STATE_READY; 01127 01128 /* Return function status */ 01129 return HAL_OK; 01130 } 01131 01132 /** 01133 * @brief Starts the PWM signal generation in interrupt mode. 01134 * @param htim : TIM handle 01135 * @param Channel : TIM Channel to be disabled 01136 * This parameter can be one of the following values: 01137 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01138 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01139 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01140 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01141 * @retval HAL status 01142 */ 01143 HAL_StatusTypeDef HAL_TIM_PWM_Start_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 01144 { 01145 /* Check the parameters */ 01146 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01147 01148 switch (Channel) 01149 { 01150 case TIM_CHANNEL_1: 01151 { 01152 /* Enable the TIM Capture/Compare 1 interrupt */ 01153 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 01154 } 01155 break; 01156 01157 case TIM_CHANNEL_2: 01158 { 01159 /* Enable the TIM Capture/Compare 2 interrupt */ 01160 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 01161 } 01162 break; 01163 01164 case TIM_CHANNEL_3: 01165 { 01166 /* Enable the TIM Capture/Compare 3 interrupt */ 01167 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC3); 01168 } 01169 break; 01170 01171 case TIM_CHANNEL_4: 01172 { 01173 /* Enable the TIM Capture/Compare 4 interrupt */ 01174 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC4); 01175 } 01176 break; 01177 01178 default: 01179 break; 01180 } 01181 01182 /* Enable the Capture compare channel */ 01183 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01184 01185 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01186 { 01187 /* Enable the main output */ 01188 __HAL_TIM_MOE_ENABLE(htim); 01189 } 01190 01191 /* Enable the Peripheral */ 01192 __HAL_TIM_ENABLE(htim); 01193 01194 /* Return function status */ 01195 return HAL_OK; 01196 } 01197 01198 /** 01199 * @brief Stops the PWM signal generation in interrupt mode. 01200 * @param htim : TIM handle 01201 * @param Channel : TIM Channels to be disabled 01202 * This parameter can be one of the following values: 01203 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01204 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01205 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01206 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01207 * @retval HAL status 01208 */ 01209 HAL_StatusTypeDef HAL_TIM_PWM_Stop_IT (TIM_HandleTypeDef *htim, uint32_t Channel) 01210 { 01211 /* Check the parameters */ 01212 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01213 01214 switch (Channel) 01215 { 01216 case TIM_CHANNEL_1: 01217 { 01218 /* Disable the TIM Capture/Compare 1 interrupt */ 01219 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 01220 } 01221 break; 01222 01223 case TIM_CHANNEL_2: 01224 { 01225 /* Disable the TIM Capture/Compare 2 interrupt */ 01226 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 01227 } 01228 break; 01229 01230 case TIM_CHANNEL_3: 01231 { 01232 /* Disable the TIM Capture/Compare 3 interrupt */ 01233 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC3); 01234 } 01235 break; 01236 01237 case TIM_CHANNEL_4: 01238 { 01239 /* Disable the TIM Capture/Compare 4 interrupt */ 01240 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC4); 01241 } 01242 break; 01243 01244 default: 01245 break; 01246 } 01247 01248 /* Disable the Capture compare channel */ 01249 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01250 01251 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01252 { 01253 /* Disable the Main Ouput */ 01254 __HAL_TIM_MOE_DISABLE(htim); 01255 } 01256 01257 /* Disable the Peripheral */ 01258 __HAL_TIM_DISABLE(htim); 01259 01260 /* Return function status */ 01261 return HAL_OK; 01262 } 01263 01264 /** 01265 * @brief Starts the TIM PWM signal generation in DMA mode. 01266 * @param htim : TIM handle 01267 * @param Channel : TIM Channels to be enabled 01268 * This parameter can be one of the following values: 01269 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01270 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01271 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01272 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01273 * @param pData: The source Buffer address. 01274 * @param Length: The length of data to be transferred from memory to TIM peripheral 01275 * @retval HAL status 01276 */ 01277 HAL_StatusTypeDef HAL_TIM_PWM_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData, uint16_t Length) 01278 { 01279 /* Check the parameters */ 01280 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01281 01282 if((htim->State == HAL_TIM_STATE_BUSY)) 01283 { 01284 return HAL_BUSY; 01285 } 01286 else if((htim->State == HAL_TIM_STATE_READY)) 01287 { 01288 if(((uint32_t)pData == 0 ) && (Length > 0)) 01289 { 01290 return HAL_ERROR; 01291 } 01292 else 01293 { 01294 htim->State = HAL_TIM_STATE_BUSY; 01295 } 01296 } 01297 switch (Channel) 01298 { 01299 case TIM_CHANNEL_1: 01300 { 01301 /* Set the DMA Period elapsed callback */ 01302 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMADelayPulseCplt; 01303 01304 /* Set the DMA error callback */ 01305 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 01306 01307 /* Enable the DMA channel */ 01308 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)pData, (uint32_t)&htim->Instance->CCR1, Length); 01309 01310 /* Enable the TIM Capture/Compare 1 DMA request */ 01311 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 01312 } 01313 break; 01314 01315 case TIM_CHANNEL_2: 01316 { 01317 /* Set the DMA Period elapsed callback */ 01318 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMADelayPulseCplt; 01319 01320 /* Set the DMA error callback */ 01321 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 01322 01323 /* Enable the DMA channel */ 01324 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)pData, (uint32_t)&htim->Instance->CCR2, Length); 01325 01326 /* Enable the TIM Capture/Compare 2 DMA request */ 01327 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 01328 } 01329 break; 01330 01331 case TIM_CHANNEL_3: 01332 { 01333 /* Set the DMA Period elapsed callback */ 01334 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMADelayPulseCplt; 01335 01336 /* Set the DMA error callback */ 01337 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 01338 01339 /* Enable the DMA channel */ 01340 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)pData, (uint32_t)&htim->Instance->CCR3,Length); 01341 01342 /* Enable the TIM Output Capture/Compare 3 request */ 01343 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC3); 01344 } 01345 break; 01346 01347 case TIM_CHANNEL_4: 01348 { 01349 /* Set the DMA Period elapsed callback */ 01350 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMADelayPulseCplt; 01351 01352 /* Set the DMA error callback */ 01353 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 01354 01355 /* Enable the DMA channel */ 01356 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)pData, (uint32_t)&htim->Instance->CCR4, Length); 01357 01358 /* Enable the TIM Capture/Compare 4 DMA request */ 01359 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC4); 01360 } 01361 break; 01362 01363 default: 01364 break; 01365 } 01366 01367 /* Enable the Capture compare channel */ 01368 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01369 01370 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01371 { 01372 /* Enable the main output */ 01373 __HAL_TIM_MOE_ENABLE(htim); 01374 } 01375 01376 /* Enable the Peripheral */ 01377 __HAL_TIM_ENABLE(htim); 01378 01379 /* Return function status */ 01380 return HAL_OK; 01381 } 01382 01383 /** 01384 * @brief Stops the TIM PWM signal generation in DMA mode. 01385 * @param htim : TIM handle 01386 * @param Channel : TIM Channels to be disabled 01387 * This parameter can be one of the following values: 01388 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01389 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01390 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01391 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01392 * @retval HAL status 01393 */ 01394 HAL_StatusTypeDef HAL_TIM_PWM_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 01395 { 01396 /* Check the parameters */ 01397 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01398 01399 switch (Channel) 01400 { 01401 case TIM_CHANNEL_1: 01402 { 01403 /* Disable the TIM Capture/Compare 1 DMA request */ 01404 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 01405 } 01406 break; 01407 01408 case TIM_CHANNEL_2: 01409 { 01410 /* Disable the TIM Capture/Compare 2 DMA request */ 01411 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 01412 } 01413 break; 01414 01415 case TIM_CHANNEL_3: 01416 { 01417 /* Disable the TIM Capture/Compare 3 DMA request */ 01418 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC3); 01419 } 01420 break; 01421 01422 case TIM_CHANNEL_4: 01423 { 01424 /* Disable the TIM Capture/Compare 4 interrupt */ 01425 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC4); 01426 } 01427 break; 01428 01429 default: 01430 break; 01431 } 01432 01433 /* Disable the Capture compare channel */ 01434 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01435 01436 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 01437 { 01438 /* Disable the Main Ouput */ 01439 __HAL_TIM_MOE_DISABLE(htim); 01440 } 01441 01442 /* Disable the Peripheral */ 01443 __HAL_TIM_DISABLE(htim); 01444 01445 /* Change the htim state */ 01446 htim->State = HAL_TIM_STATE_READY; 01447 01448 /* Return function status */ 01449 return HAL_OK; 01450 } 01451 01452 /** 01453 * @} 01454 */ 01455 01456 /** @defgroup TIM_Exported_Functions_Group4 Time Input Capture functions 01457 * @brief Time Input Capture functions 01458 * 01459 @verbatim 01460 ============================================================================== 01461 ##### Time Input Capture functions ##### 01462 ============================================================================== 01463 [..] 01464 This section provides functions allowing to: 01465 (+) Initialize and configure the TIM Input Capture. 01466 (+) De-initialize the TIM Input Capture. 01467 (+) Start the Time Input Capture. 01468 (+) Stop the Time Input Capture. 01469 (+) Start the Time Input Capture and enable interrupt. 01470 (+) Stop the Time Input Capture and disable interrupt. 01471 (+) Start the Time Input Capture and enable DMA transfer. 01472 (+) Stop the Time Input Capture and disable DMA transfer. 01473 01474 @endverbatim 01475 * @{ 01476 */ 01477 /** 01478 * @brief Initializes the TIM Input Capture Time base according to the specified 01479 * parameters in the TIM_HandleTypeDef and initialize the associated handle. 01480 * @param htim: TIM Input Capture handle 01481 * @retval HAL status 01482 */ 01483 HAL_StatusTypeDef HAL_TIM_IC_Init(TIM_HandleTypeDef *htim) 01484 { 01485 /* Check the TIM handle allocation */ 01486 if(htim == NULL) 01487 { 01488 return HAL_ERROR; 01489 } 01490 01491 /* Check the parameters */ 01492 assert_param(IS_TIM_INSTANCE(htim->Instance)); 01493 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 01494 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 01495 01496 if(htim->State == HAL_TIM_STATE_RESET) 01497 { 01498 /* Allocate lock resource and initialize it */ 01499 htim->Lock = HAL_UNLOCKED; 01500 01501 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 01502 HAL_TIM_IC_MspInit(htim); 01503 } 01504 01505 /* Set the TIM state */ 01506 htim->State= HAL_TIM_STATE_BUSY; 01507 01508 /* Init the base time for the input capture */ 01509 TIM_Base_SetConfig(htim->Instance, &htim->Init); 01510 01511 /* Initialize the TIM state*/ 01512 htim->State= HAL_TIM_STATE_READY; 01513 01514 return HAL_OK; 01515 } 01516 01517 /** 01518 * @brief DeInitialize the TIM peripheral 01519 * @param htim: TIM Input Capture handle 01520 * @retval HAL status 01521 */ 01522 HAL_StatusTypeDef HAL_TIM_IC_DeInit(TIM_HandleTypeDef *htim) 01523 { 01524 /* Check the parameters */ 01525 assert_param(IS_TIM_INSTANCE(htim->Instance)); 01526 01527 htim->State = HAL_TIM_STATE_BUSY; 01528 01529 /* Disable the TIM Peripheral Clock */ 01530 __HAL_TIM_DISABLE(htim); 01531 01532 /* DeInit the low level hardware: GPIO, CLOCK, NVIC and DMA */ 01533 HAL_TIM_IC_MspDeInit(htim); 01534 01535 /* Change TIM state */ 01536 htim->State = HAL_TIM_STATE_RESET; 01537 01538 /* Release Lock */ 01539 __HAL_UNLOCK(htim); 01540 01541 return HAL_OK; 01542 } 01543 01544 /** 01545 * @brief Initializes the TIM INput Capture MSP. 01546 * @param htim: TIM handle 01547 * @retval None 01548 */ 01549 __weak void HAL_TIM_IC_MspInit(TIM_HandleTypeDef *htim) 01550 { 01551 /* NOTE : This function should not be modified, when the callback is needed, 01552 the HAL_TIM_IC_MspInit could be implemented in the user file 01553 */ 01554 } 01555 01556 /** 01557 * @brief DeInitialize TIM Input Capture MSP. 01558 * @param htim: TIM handle 01559 * @retval None 01560 */ 01561 __weak void HAL_TIM_IC_MspDeInit(TIM_HandleTypeDef *htim) 01562 { 01563 /* NOTE : This function should not be modified, when the callback is needed, 01564 the HAL_TIM_IC_MspDeInit could be implemented in the user file 01565 */ 01566 } 01567 01568 /** 01569 * @brief Starts the TIM Input Capture measurement. 01570 * @param htim : TIM Input Capture handle 01571 * @param Channel : TIM Channels to be enabled 01572 * This parameter can be one of the following values: 01573 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01574 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01575 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01576 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01577 * @retval HAL status 01578 */ 01579 HAL_StatusTypeDef HAL_TIM_IC_Start (TIM_HandleTypeDef *htim, uint32_t Channel) 01580 { 01581 /* Check the parameters */ 01582 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01583 01584 /* Enable the Input Capture channel */ 01585 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01586 01587 /* Enable the Peripheral */ 01588 __HAL_TIM_ENABLE(htim); 01589 01590 /* Return function status */ 01591 return HAL_OK; 01592 } 01593 01594 /** 01595 * @brief Stops the TIM Input Capture measurement. 01596 * @param htim : TIM handle 01597 * @param Channel : TIM Channels to be disabled 01598 * This parameter can be one of the following values: 01599 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01600 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01601 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01602 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01603 * @retval HAL status 01604 */ 01605 HAL_StatusTypeDef HAL_TIM_IC_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 01606 { 01607 /* Check the parameters */ 01608 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01609 01610 /* Disable the Input Capture channel */ 01611 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01612 01613 /* Disable the Peripheral */ 01614 __HAL_TIM_DISABLE(htim); 01615 01616 /* Return function status */ 01617 return HAL_OK; 01618 } 01619 01620 /** 01621 * @brief Starts the TIM Input Capture measurement in interrupt mode. 01622 * @param htim : TIM Input Capture handle 01623 * @param Channel : TIM Channels to be enabled 01624 * This parameter can be one of the following values: 01625 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01626 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01627 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01628 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01629 * @retval HAL status 01630 */ 01631 HAL_StatusTypeDef HAL_TIM_IC_Start_IT (TIM_HandleTypeDef *htim, uint32_t Channel) 01632 { 01633 /* Check the parameters */ 01634 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01635 01636 switch (Channel) 01637 { 01638 case TIM_CHANNEL_1: 01639 { 01640 /* Enable the TIM Capture/Compare 1 interrupt */ 01641 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 01642 } 01643 break; 01644 01645 case TIM_CHANNEL_2: 01646 { 01647 /* Enable the TIM Capture/Compare 2 interrupt */ 01648 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 01649 } 01650 break; 01651 01652 case TIM_CHANNEL_3: 01653 { 01654 /* Enable the TIM Capture/Compare 3 interrupt */ 01655 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC3); 01656 } 01657 break; 01658 01659 case TIM_CHANNEL_4: 01660 { 01661 /* Enable the TIM Capture/Compare 4 interrupt */ 01662 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC4); 01663 } 01664 break; 01665 01666 default: 01667 break; 01668 } 01669 /* Enable the Input Capture channel */ 01670 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01671 01672 /* Enable the Peripheral */ 01673 __HAL_TIM_ENABLE(htim); 01674 01675 /* Return function status */ 01676 return HAL_OK; 01677 } 01678 01679 /** 01680 * @brief Stops the TIM Input Capture measurement in interrupt mode. 01681 * @param htim : TIM handle 01682 * @param Channel : TIM Channels to be disabled 01683 * This parameter can be one of the following values: 01684 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01685 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01686 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01687 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01688 * @retval HAL status 01689 */ 01690 HAL_StatusTypeDef HAL_TIM_IC_Stop_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 01691 { 01692 /* Check the parameters */ 01693 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01694 01695 switch (Channel) 01696 { 01697 case TIM_CHANNEL_1: 01698 { 01699 /* Disable the TIM Capture/Compare 1 interrupt */ 01700 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 01701 } 01702 break; 01703 01704 case TIM_CHANNEL_2: 01705 { 01706 /* Disable the TIM Capture/Compare 2 interrupt */ 01707 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 01708 } 01709 break; 01710 01711 case TIM_CHANNEL_3: 01712 { 01713 /* Disable the TIM Capture/Compare 3 interrupt */ 01714 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC3); 01715 } 01716 break; 01717 01718 case TIM_CHANNEL_4: 01719 { 01720 /* Disable the TIM Capture/Compare 4 interrupt */ 01721 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC4); 01722 } 01723 break; 01724 01725 default: 01726 break; 01727 } 01728 01729 /* Disable the Input Capture channel */ 01730 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01731 01732 /* Disable the Peripheral */ 01733 __HAL_TIM_DISABLE(htim); 01734 01735 /* Return function status */ 01736 return HAL_OK; 01737 } 01738 01739 /** 01740 * @brief Starts the TIM Input Capture measurement on in DMA mode. 01741 * @param htim : TIM Input Capture handle 01742 * @param Channel : TIM Channels to be enabled 01743 * This parameter can be one of the following values: 01744 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01745 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01746 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01747 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01748 * @param pData: The destination Buffer address. 01749 * @param Length: The length of data to be transferred from TIM peripheral to memory. 01750 * @retval HAL status 01751 */ 01752 HAL_StatusTypeDef HAL_TIM_IC_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData, uint16_t Length) 01753 { 01754 /* Check the parameters */ 01755 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01756 assert_param(IS_TIM_DMA_CC_INSTANCE(htim->Instance)); 01757 01758 if((htim->State == HAL_TIM_STATE_BUSY)) 01759 { 01760 return HAL_BUSY; 01761 } 01762 else if((htim->State == HAL_TIM_STATE_READY)) 01763 { 01764 if((pData == 0 ) && (Length > 0)) 01765 { 01766 return HAL_ERROR; 01767 } 01768 else 01769 { 01770 htim->State = HAL_TIM_STATE_BUSY; 01771 } 01772 } 01773 01774 switch (Channel) 01775 { 01776 case TIM_CHANNEL_1: 01777 { 01778 /* Set the DMA Period elapsed callback */ 01779 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMACaptureCplt; 01780 01781 /* Set the DMA error callback */ 01782 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 01783 01784 /* Enable the DMA channel */ 01785 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)&htim->Instance->CCR1, (uint32_t)pData, Length); 01786 01787 /* Enable the TIM Capture/Compare 1 DMA request */ 01788 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 01789 } 01790 break; 01791 01792 case TIM_CHANNEL_2: 01793 { 01794 /* Set the DMA Period elapsed callback */ 01795 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMACaptureCplt; 01796 01797 /* Set the DMA error callback */ 01798 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 01799 01800 /* Enable the DMA channel */ 01801 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)&htim->Instance->CCR2, (uint32_t)pData, Length); 01802 01803 /* Enable the TIM Capture/Compare 2 DMA request */ 01804 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 01805 } 01806 break; 01807 01808 case TIM_CHANNEL_3: 01809 { 01810 /* Set the DMA Period elapsed callback */ 01811 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMACaptureCplt; 01812 01813 /* Set the DMA error callback */ 01814 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 01815 01816 /* Enable the DMA channel */ 01817 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)&htim->Instance->CCR3, (uint32_t)pData, Length); 01818 01819 /* Enable the TIM Capture/Compare 3 DMA request */ 01820 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC3); 01821 } 01822 break; 01823 01824 case TIM_CHANNEL_4: 01825 { 01826 /* Set the DMA Period elapsed callback */ 01827 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMACaptureCplt; 01828 01829 /* Set the DMA error callback */ 01830 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 01831 01832 /* Enable the DMA channel */ 01833 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)&htim->Instance->CCR4, (uint32_t)pData, Length); 01834 01835 /* Enable the TIM Capture/Compare 4 DMA request */ 01836 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC4); 01837 } 01838 break; 01839 01840 default: 01841 break; 01842 } 01843 01844 /* Enable the Input Capture channel */ 01845 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_ENABLE); 01846 01847 /* Enable the Peripheral */ 01848 __HAL_TIM_ENABLE(htim); 01849 01850 /* Return function status */ 01851 return HAL_OK; 01852 } 01853 01854 /** 01855 * @brief Stops the TIM Input Capture measurement on in DMA mode. 01856 * @param htim : TIM Input Capture handle 01857 * @param Channel : TIM Channels to be disabled 01858 * This parameter can be one of the following values: 01859 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 01860 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 01861 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 01862 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 01863 * @retval HAL status 01864 */ 01865 HAL_StatusTypeDef HAL_TIM_IC_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 01866 { 01867 /* Check the parameters */ 01868 assert_param(IS_TIM_CCX_INSTANCE(htim->Instance, Channel)); 01869 assert_param(IS_TIM_DMA_CC_INSTANCE(htim->Instance)); 01870 01871 switch (Channel) 01872 { 01873 case TIM_CHANNEL_1: 01874 { 01875 /* Disable the TIM Capture/Compare 1 DMA request */ 01876 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 01877 } 01878 break; 01879 01880 case TIM_CHANNEL_2: 01881 { 01882 /* Disable the TIM Capture/Compare 2 DMA request */ 01883 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 01884 } 01885 break; 01886 01887 case TIM_CHANNEL_3: 01888 { 01889 /* Disable the TIM Capture/Compare 3 DMA request */ 01890 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC3); 01891 } 01892 break; 01893 01894 case TIM_CHANNEL_4: 01895 { 01896 /* Disable the TIM Capture/Compare 4 DMA request */ 01897 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC4); 01898 } 01899 break; 01900 01901 default: 01902 break; 01903 } 01904 01905 /* Disable the Input Capture channel */ 01906 TIM_CCxChannelCmd(htim->Instance, Channel, TIM_CCx_DISABLE); 01907 01908 /* Disable the Peripheral */ 01909 __HAL_TIM_DISABLE(htim); 01910 01911 /* Change the htim state */ 01912 htim->State = HAL_TIM_STATE_READY; 01913 01914 /* Return function status */ 01915 return HAL_OK; 01916 } 01917 /** 01918 * @} 01919 */ 01920 01921 /** @defgroup TIM_Exported_Functions_Group5 Time One Pulse functions 01922 * @brief Time One Pulse functions 01923 * 01924 @verbatim 01925 ============================================================================== 01926 ##### Time One Pulse functions ##### 01927 ============================================================================== 01928 [..] 01929 This section provides functions allowing to: 01930 (+) Initialize and configure the TIM One Pulse. 01931 (+) De-initialize the TIM One Pulse. 01932 (+) Start the Time One Pulse. 01933 (+) Stop the Time One Pulse. 01934 (+) Start the Time One Pulse and enable interrupt. 01935 (+) Stop the Time One Pulse and disable interrupt. 01936 (+) Start the Time One Pulse and enable DMA transfer. 01937 (+) Stop the Time One Pulse and disable DMA transfer. 01938 01939 @endverbatim 01940 * @{ 01941 */ 01942 /** 01943 * @brief Initializes the TIM One Pulse Time Base according to the specified 01944 * parameters in the TIM_HandleTypeDef and initialize the associated handle. 01945 * @param htim: TIM OnePulse handle 01946 * @param OnePulseMode: Select the One pulse mode. 01947 * This parameter can be one of the following values: 01948 * @arg TIM_OPMODE_SINGLE: Only one pulse will be generated. 01949 * @arg TIM_OPMODE_REPETITIVE: Repetitive pulses will be generated. 01950 * @retval HAL status 01951 */ 01952 HAL_StatusTypeDef HAL_TIM_OnePulse_Init(TIM_HandleTypeDef *htim, uint32_t OnePulseMode) 01953 { 01954 /* Check the TIM handle allocation */ 01955 if(htim == NULL) 01956 { 01957 return HAL_ERROR; 01958 } 01959 01960 /* Check the parameters */ 01961 assert_param(IS_TIM_INSTANCE(htim->Instance)); 01962 assert_param(IS_TIM_COUNTER_MODE(htim->Init.CounterMode)); 01963 assert_param(IS_TIM_CLOCKDIVISION_DIV(htim->Init.ClockDivision)); 01964 assert_param(IS_TIM_OPM_MODE(OnePulseMode)); 01965 01966 if(htim->State == HAL_TIM_STATE_RESET) 01967 { 01968 /* Allocate lock resource and initialize it */ 01969 htim->Lock = HAL_UNLOCKED; 01970 01971 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 01972 HAL_TIM_OnePulse_MspInit(htim); 01973 } 01974 01975 /* Set the TIM state */ 01976 htim->State= HAL_TIM_STATE_BUSY; 01977 01978 /* Configure the Time base in the One Pulse Mode */ 01979 TIM_Base_SetConfig(htim->Instance, &htim->Init); 01980 01981 /* Reset the OPM Bit */ 01982 htim->Instance->CR1 &= ~TIM_CR1_OPM; 01983 01984 /* Configure the OPM Mode */ 01985 htim->Instance->CR1 |= OnePulseMode; 01986 01987 /* Initialize the TIM state*/ 01988 htim->State= HAL_TIM_STATE_READY; 01989 01990 return HAL_OK; 01991 } 01992 01993 /** 01994 * @brief DeInitialize the TIM One Pulse 01995 * @param htim: TIM One Pulse handle 01996 * @retval HAL status 01997 */ 01998 HAL_StatusTypeDef HAL_TIM_OnePulse_DeInit(TIM_HandleTypeDef *htim) 01999 { 02000 /* Check the parameters */ 02001 assert_param(IS_TIM_INSTANCE(htim->Instance)); 02002 02003 htim->State = HAL_TIM_STATE_BUSY; 02004 02005 /* Disable the TIM Peripheral Clock */ 02006 __HAL_TIM_DISABLE(htim); 02007 02008 /* DeInit the low level hardware: GPIO, CLOCK, NVIC */ 02009 HAL_TIM_OnePulse_MspDeInit(htim); 02010 02011 /* Change TIM state */ 02012 htim->State = HAL_TIM_STATE_RESET; 02013 02014 /* Release Lock */ 02015 __HAL_UNLOCK(htim); 02016 02017 return HAL_OK; 02018 } 02019 02020 /** 02021 * @brief Initializes the TIM One Pulse MSP. 02022 * @param htim: TIM handle 02023 * @retval None 02024 */ 02025 __weak void HAL_TIM_OnePulse_MspInit(TIM_HandleTypeDef *htim) 02026 { 02027 /* NOTE : This function should not be modified, when the callback is needed, 02028 the HAL_TIM_OnePulse_MspInit could be implemented in the user file 02029 */ 02030 } 02031 02032 /** 02033 * @brief DeInitialize TIM One Pulse MSP. 02034 * @param htim: TIM handle 02035 * @retval None 02036 */ 02037 __weak void HAL_TIM_OnePulse_MspDeInit(TIM_HandleTypeDef *htim) 02038 { 02039 /* NOTE : This function should not be modified, when the callback is needed, 02040 the HAL_TIM_OnePulse_MspDeInit could be implemented in the user file 02041 */ 02042 } 02043 02044 /** 02045 * @brief Starts the TIM One Pulse signal generation. 02046 * @param htim : TIM One Pulse handle 02047 * @param OutputChannel : TIM Channels to be enabled 02048 * This parameter can be one of the following values: 02049 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02050 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02051 * @retval HAL status 02052 */ 02053 HAL_StatusTypeDef HAL_TIM_OnePulse_Start(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 02054 { 02055 /* Enable the Capture compare and the Input Capture channels 02056 (in the OPM Mode the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) 02057 if TIM_CHANNEL_1 is used as output, the TIM_CHANNEL_2 will be used as input and 02058 if TIM_CHANNEL_1 is used as input, the TIM_CHANNEL_2 will be used as output 02059 in all combinations, the TIM_CHANNEL_1 and TIM_CHANNEL_2 should be enabled together 02060 02061 No need to enable the counter, it's enabled automatically by hardware 02062 (the counter starts in response to a stimulus and generate a pulse */ 02063 02064 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02065 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02066 02067 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 02068 { 02069 /* Enable the main output */ 02070 __HAL_TIM_MOE_ENABLE(htim); 02071 } 02072 02073 /* Return function status */ 02074 return HAL_OK; 02075 } 02076 02077 /** 02078 * @brief Stops the TIM One Pulse signal generation. 02079 * @param htim : TIM One Pulse handle 02080 * @param OutputChannel : TIM Channels to be disable 02081 * This parameter can be one of the following values: 02082 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02083 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02084 * @retval HAL status 02085 */ 02086 HAL_StatusTypeDef HAL_TIM_OnePulse_Stop(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 02087 { 02088 /* Disable the Capture compare and the Input Capture channels 02089 (in the OPM Mode the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) 02090 if TIM_CHANNEL_1 is used as output, the TIM_CHANNEL_2 will be used as input and 02091 if TIM_CHANNEL_1 is used as input, the TIM_CHANNEL_2 will be used as output 02092 in all combinations, the TIM_CHANNEL_1 and TIM_CHANNEL_2 should be disabled together */ 02093 02094 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02095 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02096 02097 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 02098 { 02099 /* Disable the Main Ouput */ 02100 __HAL_TIM_MOE_DISABLE(htim); 02101 } 02102 02103 /* Disable the Peripheral */ 02104 __HAL_TIM_DISABLE(htim); 02105 02106 /* Return function status */ 02107 return HAL_OK; 02108 } 02109 02110 /** 02111 * @brief Starts the TIM One Pulse signal generation in interrupt mode. 02112 * @param htim : TIM One Pulse handle 02113 * @param OutputChannel : TIM Channels to be enabled 02114 * This parameter can be one of the following values: 02115 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02116 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02117 * @retval HAL status 02118 */ 02119 HAL_StatusTypeDef HAL_TIM_OnePulse_Start_IT(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 02120 { 02121 /* Enable the Capture compare and the Input Capture channels 02122 (in the OPM Mode the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) 02123 if TIM_CHANNEL_1 is used as output, the TIM_CHANNEL_2 will be used as input and 02124 if TIM_CHANNEL_1 is used as input, the TIM_CHANNEL_2 will be used as output 02125 in all combinations, the TIM_CHANNEL_1 and TIM_CHANNEL_2 should be enabled together 02126 02127 No need to enable the counter, it's enabled automatically by hardware 02128 (the counter starts in response to a stimulus and generate a pulse */ 02129 02130 /* Enable the TIM Capture/Compare 1 interrupt */ 02131 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 02132 02133 /* Enable the TIM Capture/Compare 2 interrupt */ 02134 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 02135 02136 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02137 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02138 02139 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 02140 { 02141 /* Enable the main output */ 02142 __HAL_TIM_MOE_ENABLE(htim); 02143 } 02144 02145 /* Return function status */ 02146 return HAL_OK; 02147 } 02148 02149 /** 02150 * @brief Stops the TIM One Pulse signal generation in interrupt mode. 02151 * @param htim : TIM One Pulse handle 02152 * @param OutputChannel : TIM Channels to be enabled 02153 * This parameter can be one of the following values: 02154 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02155 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02156 * @retval HAL status 02157 */ 02158 HAL_StatusTypeDef HAL_TIM_OnePulse_Stop_IT(TIM_HandleTypeDef *htim, uint32_t OutputChannel) 02159 { 02160 /* Disable the TIM Capture/Compare 1 interrupt */ 02161 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 02162 02163 /* Disable the TIM Capture/Compare 2 interrupt */ 02164 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 02165 02166 /* Disable the Capture compare and the Input Capture channels 02167 (in the OPM Mode the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) 02168 if TIM_CHANNEL_1 is used as output, the TIM_CHANNEL_2 will be used as input and 02169 if TIM_CHANNEL_1 is used as input, the TIM_CHANNEL_2 will be used as output 02170 in all combinations, the TIM_CHANNEL_1 and TIM_CHANNEL_2 should be disabled together */ 02171 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02172 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02173 02174 if(IS_TIM_BREAK_INSTANCE(htim->Instance) != RESET) 02175 { 02176 /* Disable the Main Ouput */ 02177 __HAL_TIM_MOE_DISABLE(htim); 02178 } 02179 02180 /* Disable the Peripheral */ 02181 __HAL_TIM_DISABLE(htim); 02182 02183 /* Return function status */ 02184 return HAL_OK; 02185 } 02186 02187 /** 02188 * @} 02189 */ 02190 02191 /** @defgroup TIM_Exported_Functions_Group6 Time Encoder functions 02192 * @brief Time Encoder functions 02193 * 02194 @verbatim 02195 ============================================================================== 02196 ##### Time Encoder functions ##### 02197 ============================================================================== 02198 [..] 02199 This section provides functions allowing to: 02200 (+) Initialize and configure the TIM Encoder. 02201 (+) De-initialize the TIM Encoder. 02202 (+) Start the Time Encoder. 02203 (+) Stop the Time Encoder. 02204 (+) Start the Time Encoder and enable interrupt. 02205 (+) Stop the Time Encoder and disable interrupt. 02206 (+) Start the Time Encoder and enable DMA transfer. 02207 (+) Stop the Time Encoder and disable DMA transfer. 02208 02209 @endverbatim 02210 * @{ 02211 */ 02212 /** 02213 * @brief Initializes the TIM Encoder Interface and initialize the associated handle. 02214 * @param htim: TIM Encoder Interface handle 02215 * @param sConfig: TIM Encoder Interface configuration structure 02216 * @retval HAL status 02217 */ 02218 HAL_StatusTypeDef HAL_TIM_Encoder_Init(TIM_HandleTypeDef *htim, TIM_Encoder_InitTypeDef* sConfig) 02219 { 02220 uint32_t tmpsmcr = 0; 02221 uint32_t tmpccmr1 = 0; 02222 uint32_t tmpccer = 0; 02223 02224 /* Check the TIM handle allocation */ 02225 if(htim == NULL) 02226 { 02227 return HAL_ERROR; 02228 } 02229 02230 /* Check the parameters */ 02231 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02232 assert_param(IS_TIM_ENCODER_MODE(sConfig->EncoderMode)); 02233 assert_param(IS_TIM_IC_SELECTION(sConfig->IC1Selection)); 02234 assert_param(IS_TIM_IC_SELECTION(sConfig->IC2Selection)); 02235 assert_param(IS_TIM_IC_POLARITY(sConfig->IC1Polarity)); 02236 assert_param(IS_TIM_IC_POLARITY(sConfig->IC2Polarity)); 02237 assert_param(IS_TIM_IC_PRESCALER(sConfig->IC1Prescaler)); 02238 assert_param(IS_TIM_IC_PRESCALER(sConfig->IC2Prescaler)); 02239 assert_param(IS_TIM_IC_FILTER(sConfig->IC1Filter)); 02240 assert_param(IS_TIM_IC_FILTER(sConfig->IC2Filter)); 02241 02242 if(htim->State == HAL_TIM_STATE_RESET) 02243 { 02244 /* Allocate lock resource and initialize it */ 02245 htim->Lock = HAL_UNLOCKED; 02246 02247 /* Init the low level hardware : GPIO, CLOCK, NVIC and DMA */ 02248 HAL_TIM_Encoder_MspInit(htim); 02249 } 02250 02251 /* Set the TIM state */ 02252 htim->State= HAL_TIM_STATE_BUSY; 02253 02254 /* Reset the SMS bits */ 02255 htim->Instance->SMCR &= ~TIM_SMCR_SMS; 02256 02257 /* Configure the Time base in the Encoder Mode */ 02258 TIM_Base_SetConfig(htim->Instance, &htim->Init); 02259 02260 /* Get the TIMx SMCR register value */ 02261 tmpsmcr = htim->Instance->SMCR; 02262 02263 /* Get the TIMx CCMR1 register value */ 02264 tmpccmr1 = htim->Instance->CCMR1; 02265 02266 /* Get the TIMx CCER register value */ 02267 tmpccer = htim->Instance->CCER; 02268 02269 /* Set the encoder Mode */ 02270 tmpsmcr |= sConfig->EncoderMode; 02271 02272 /* Select the Capture Compare 1 and the Capture Compare 2 as input */ 02273 tmpccmr1 &= ~(TIM_CCMR1_CC1S | TIM_CCMR1_CC2S); 02274 tmpccmr1 |= (sConfig->IC1Selection | (sConfig->IC2Selection << 8)); 02275 02276 /* Set the Capture Compare 1 and the Capture Compare 2 prescalers and filters */ 02277 tmpccmr1 &= ~(TIM_CCMR1_IC1PSC | TIM_CCMR1_IC2PSC); 02278 tmpccmr1 &= ~(TIM_CCMR1_IC1F | TIM_CCMR1_IC2F); 02279 tmpccmr1 |= sConfig->IC1Prescaler | (sConfig->IC2Prescaler << 8); 02280 tmpccmr1 |= (sConfig->IC1Filter << 4) | (sConfig->IC2Filter << 12); 02281 02282 /* Set the TI1 and the TI2 Polarities */ 02283 tmpccer &= ~(TIM_CCER_CC1P | TIM_CCER_CC2P); 02284 tmpccer &= ~(TIM_CCER_CC1NP | TIM_CCER_CC2NP); 02285 tmpccer |= sConfig->IC1Polarity | (sConfig->IC2Polarity << 4); 02286 02287 /* Write to TIMx SMCR */ 02288 htim->Instance->SMCR = tmpsmcr; 02289 02290 /* Write to TIMx CCMR1 */ 02291 htim->Instance->CCMR1 = tmpccmr1; 02292 02293 /* Write to TIMx CCER */ 02294 htim->Instance->CCER = tmpccer; 02295 02296 /* Initialize the TIM state*/ 02297 htim->State= HAL_TIM_STATE_READY; 02298 02299 return HAL_OK; 02300 } 02301 02302 02303 /** 02304 * @brief DeInitialize the TIM Encoder interface 02305 * @param htim: TIM Encoder handle 02306 * @retval HAL status 02307 */ 02308 HAL_StatusTypeDef HAL_TIM_Encoder_DeInit(TIM_HandleTypeDef *htim) 02309 { 02310 /* Check the parameters */ 02311 assert_param(IS_TIM_INSTANCE(htim->Instance)); 02312 02313 htim->State = HAL_TIM_STATE_BUSY; 02314 02315 /* Disable the TIM Peripheral Clock */ 02316 __HAL_TIM_DISABLE(htim); 02317 02318 /* DeInit the low level hardware: GPIO, CLOCK, NVIC */ 02319 HAL_TIM_Encoder_MspDeInit(htim); 02320 02321 /* Change TIM state */ 02322 htim->State = HAL_TIM_STATE_RESET; 02323 02324 /* Release Lock */ 02325 __HAL_UNLOCK(htim); 02326 02327 return HAL_OK; 02328 } 02329 02330 /** 02331 * @brief Initializes the TIM Encoder Interface MSP. 02332 * @param htim: TIM handle 02333 * @retval None 02334 */ 02335 __weak void HAL_TIM_Encoder_MspInit(TIM_HandleTypeDef *htim) 02336 { 02337 /* NOTE : This function should not be modified, when the callback is needed, 02338 the HAL_TIM_Encoder_MspInit could be implemented in the user file 02339 */ 02340 } 02341 02342 /** 02343 * @brief DeInitialize TIM Encoder Interface MSP. 02344 * @param htim: TIM handle 02345 * @retval None 02346 */ 02347 __weak void HAL_TIM_Encoder_MspDeInit(TIM_HandleTypeDef *htim) 02348 { 02349 /* NOTE : This function should not be modified, when the callback is needed, 02350 the HAL_TIM_Encoder_MspDeInit could be implemented in the user file 02351 */ 02352 } 02353 02354 /** 02355 * @brief Starts the TIM Encoder Interface. 02356 * @param htim : TIM Encoder Interface handle 02357 * @param Channel : TIM Channels to be enabled 02358 * This parameter can be one of the following values: 02359 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02360 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02361 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02362 * @retval HAL status 02363 */ 02364 HAL_StatusTypeDef HAL_TIM_Encoder_Start(TIM_HandleTypeDef *htim, uint32_t Channel) 02365 { 02366 /* Check the parameters */ 02367 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02368 02369 /* Enable the encoder interface channels */ 02370 switch (Channel) 02371 { 02372 case TIM_CHANNEL_1: 02373 { 02374 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02375 break; 02376 } 02377 case TIM_CHANNEL_2: 02378 { 02379 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02380 break; 02381 } 02382 default : 02383 { 02384 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02385 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02386 break; 02387 } 02388 } 02389 /* Enable the Peripheral */ 02390 __HAL_TIM_ENABLE(htim); 02391 02392 /* Return function status */ 02393 return HAL_OK; 02394 } 02395 02396 /** 02397 * @brief Stops the TIM Encoder Interface. 02398 * @param htim : TIM Encoder Interface handle 02399 * @param Channel : TIM Channels to be disabled 02400 * This parameter can be one of the following values: 02401 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02402 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02403 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02404 * @retval HAL status 02405 */ 02406 HAL_StatusTypeDef HAL_TIM_Encoder_Stop(TIM_HandleTypeDef *htim, uint32_t Channel) 02407 { 02408 /* Check the parameters */ 02409 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02410 02411 /* Disable the Input Capture channels 1 and 2 02412 (in the EncoderInterface the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) */ 02413 switch (Channel) 02414 { 02415 case TIM_CHANNEL_1: 02416 { 02417 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02418 break; 02419 } 02420 case TIM_CHANNEL_2: 02421 { 02422 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02423 break; 02424 } 02425 default : 02426 { 02427 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02428 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02429 break; 02430 } 02431 } 02432 02433 /* Disable the Peripheral */ 02434 __HAL_TIM_DISABLE(htim); 02435 02436 /* Return function status */ 02437 return HAL_OK; 02438 } 02439 02440 /** 02441 * @brief Starts the TIM Encoder Interface in interrupt mode. 02442 * @param htim : TIM Encoder Interface handle 02443 * @param Channel : TIM Channels to be enabled 02444 * This parameter can be one of the following values: 02445 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02446 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02447 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02448 * @retval HAL status 02449 */ 02450 HAL_StatusTypeDef HAL_TIM_Encoder_Start_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 02451 { 02452 /* Check the parameters */ 02453 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02454 02455 /* Enable the encoder interface channels */ 02456 /* Enable the capture compare Interrupts 1 and/or 2 */ 02457 switch (Channel) 02458 { 02459 case TIM_CHANNEL_1: 02460 { 02461 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02462 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 02463 break; 02464 } 02465 case TIM_CHANNEL_2: 02466 { 02467 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02468 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 02469 break; 02470 } 02471 default : 02472 { 02473 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02474 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02475 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC1); 02476 __HAL_TIM_ENABLE_IT(htim, TIM_IT_CC2); 02477 break; 02478 } 02479 } 02480 02481 /* Enable the Peripheral */ 02482 __HAL_TIM_ENABLE(htim); 02483 02484 /* Return function status */ 02485 return HAL_OK; 02486 } 02487 02488 /** 02489 * @brief Stops the TIM Encoder Interface in interrupt mode. 02490 * @param htim : TIM Encoder Interface handle 02491 * @param Channel : TIM Channels to be disabled 02492 * This parameter can be one of the following values: 02493 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02494 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02495 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02496 * @retval HAL status 02497 */ 02498 HAL_StatusTypeDef HAL_TIM_Encoder_Stop_IT(TIM_HandleTypeDef *htim, uint32_t Channel) 02499 { 02500 /* Check the parameters */ 02501 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02502 02503 /* Disable the Input Capture channels 1 and 2 02504 (in the EncoderInterface the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) */ 02505 if(Channel == TIM_CHANNEL_1) 02506 { 02507 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02508 02509 /* Disable the capture compare Interrupts 1 */ 02510 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 02511 } 02512 else if(Channel == TIM_CHANNEL_2) 02513 { 02514 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02515 02516 /* Disable the capture compare Interrupts 2 */ 02517 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 02518 } 02519 else 02520 { 02521 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02522 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02523 02524 /* Disable the capture compare Interrupts 1 and 2 */ 02525 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC1); 02526 __HAL_TIM_DISABLE_IT(htim, TIM_IT_CC2); 02527 } 02528 02529 /* Disable the Peripheral */ 02530 __HAL_TIM_DISABLE(htim); 02531 02532 /* Change the htim state */ 02533 htim->State = HAL_TIM_STATE_READY; 02534 02535 /* Return function status */ 02536 return HAL_OK; 02537 } 02538 02539 /** 02540 * @brief Starts the TIM Encoder Interface in DMA mode. 02541 * @param htim : TIM Encoder Interface handle 02542 * @param Channel : TIM Channels to be enabled 02543 * This parameter can be one of the following values: 02544 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02545 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02546 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02547 * @param pData1: The destination Buffer address for IC1. 02548 * @param pData2: The destination Buffer address for IC2. 02549 * @param Length: The length of data to be transferred from TIM peripheral to memory. 02550 * @retval HAL status 02551 */ 02552 HAL_StatusTypeDef HAL_TIM_Encoder_Start_DMA(TIM_HandleTypeDef *htim, uint32_t Channel, uint32_t *pData1, uint32_t *pData2, uint16_t Length) 02553 { 02554 /* Check the parameters */ 02555 assert_param(IS_TIM_DMA_CC_INSTANCE(htim->Instance)); 02556 02557 if((htim->State == HAL_TIM_STATE_BUSY)) 02558 { 02559 return HAL_BUSY; 02560 } 02561 else if((htim->State == HAL_TIM_STATE_READY)) 02562 { 02563 if((((pData1 == 0) || (pData2 == 0) )) && (Length > 0)) 02564 { 02565 return HAL_ERROR; 02566 } 02567 else 02568 { 02569 htim->State = HAL_TIM_STATE_BUSY; 02570 } 02571 } 02572 02573 switch (Channel) 02574 { 02575 case TIM_CHANNEL_1: 02576 { 02577 /* Set the DMA Period elapsed callback */ 02578 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMACaptureCplt; 02579 02580 /* Set the DMA error callback */ 02581 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 02582 02583 /* Enable the DMA channel */ 02584 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)&htim->Instance->CCR1, (uint32_t )pData1, Length); 02585 02586 /* Enable the TIM Input Capture DMA request */ 02587 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 02588 02589 /* Enable the Peripheral */ 02590 __HAL_TIM_ENABLE(htim); 02591 02592 /* Enable the Capture compare channel */ 02593 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02594 } 02595 break; 02596 02597 case TIM_CHANNEL_2: 02598 { 02599 /* Set the DMA Period elapsed callback */ 02600 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMACaptureCplt; 02601 02602 /* Set the DMA error callback */ 02603 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError; 02604 /* Enable the DMA channel */ 02605 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)&htim->Instance->CCR2, (uint32_t)pData2, Length); 02606 02607 /* Enable the TIM Input Capture DMA request */ 02608 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 02609 02610 /* Enable the Peripheral */ 02611 __HAL_TIM_ENABLE(htim); 02612 02613 /* Enable the Capture compare channel */ 02614 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02615 } 02616 break; 02617 02618 case TIM_CHANNEL_ALL: 02619 { 02620 /* Set the DMA Period elapsed callback */ 02621 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMACaptureCplt; 02622 02623 /* Set the DMA error callback */ 02624 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 02625 02626 /* Enable the DMA channel */ 02627 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)&htim->Instance->CCR1, (uint32_t)pData1, Length); 02628 02629 /* Set the DMA Period elapsed callback */ 02630 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMACaptureCplt; 02631 02632 /* Set the DMA error callback */ 02633 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 02634 02635 /* Enable the DMA channel */ 02636 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)&htim->Instance->CCR2, (uint32_t)pData2, Length); 02637 02638 /* Enable the Peripheral */ 02639 __HAL_TIM_ENABLE(htim); 02640 02641 /* Enable the Capture compare channel */ 02642 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_ENABLE); 02643 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_ENABLE); 02644 02645 /* Enable the TIM Input Capture DMA request */ 02646 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC1); 02647 /* Enable the TIM Input Capture DMA request */ 02648 __HAL_TIM_ENABLE_DMA(htim, TIM_DMA_CC2); 02649 } 02650 break; 02651 02652 default: 02653 break; 02654 } 02655 /* Return function status */ 02656 return HAL_OK; 02657 } 02658 02659 /** 02660 * @brief Stops the TIM Encoder Interface in DMA mode. 02661 * @param htim : TIM Encoder Interface handle 02662 * @param Channel : TIM Channels to be enabled 02663 * This parameter can be one of the following values: 02664 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02665 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02666 * @arg TIM_CHANNEL_ALL: TIM Channel 1 and TIM Channel 2 are selected 02667 * @retval HAL status 02668 */ 02669 HAL_StatusTypeDef HAL_TIM_Encoder_Stop_DMA(TIM_HandleTypeDef *htim, uint32_t Channel) 02670 { 02671 /* Check the parameters */ 02672 assert_param(IS_TIM_DMA_CC_INSTANCE(htim->Instance)); 02673 02674 /* Disable the Input Capture channels 1 and 2 02675 (in the EncoderInterface the two possible channels that can be used are TIM_CHANNEL_1 and TIM_CHANNEL_2) */ 02676 if(Channel == TIM_CHANNEL_1) 02677 { 02678 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02679 02680 /* Disable the capture compare DMA Request 1 */ 02681 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 02682 } 02683 else if(Channel == TIM_CHANNEL_2) 02684 { 02685 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02686 02687 /* Disable the capture compare DMA Request 2 */ 02688 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 02689 } 02690 else 02691 { 02692 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_1, TIM_CCx_DISABLE); 02693 TIM_CCxChannelCmd(htim->Instance, TIM_CHANNEL_2, TIM_CCx_DISABLE); 02694 02695 /* Disable the capture compare DMA Request 1 and 2 */ 02696 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC1); 02697 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_CC2); 02698 } 02699 02700 /* Disable the Peripheral */ 02701 __HAL_TIM_DISABLE(htim); 02702 02703 /* Change the htim state */ 02704 htim->State = HAL_TIM_STATE_READY; 02705 02706 /* Return function status */ 02707 return HAL_OK; 02708 } 02709 02710 /** 02711 * @} 02712 */ 02713 /** @defgroup TIM_Exported_Functions_Group7 TIM IRQ handler management 02714 * @brief IRQ handler management 02715 * 02716 @verbatim 02717 ============================================================================== 02718 ##### IRQ handler management ##### 02719 ============================================================================== 02720 [..] 02721 This section provides Timer IRQ handler function. 02722 02723 @endverbatim 02724 * @{ 02725 */ 02726 /** 02727 * @brief This function handles TIM interrupts requests. 02728 * @param htim: TIM handle 02729 * @retval None 02730 */ 02731 void HAL_TIM_IRQHandler(TIM_HandleTypeDef *htim) 02732 { 02733 /* Capture compare 1 event */ 02734 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_CC1) != RESET) 02735 { 02736 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_CC1) !=RESET) 02737 { 02738 { 02739 __HAL_TIM_CLEAR_IT(htim, TIM_IT_CC1); 02740 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_1; 02741 02742 /* Input capture event */ 02743 if((htim->Instance->CCMR1 & TIM_CCMR1_CC1S) != 0x00) 02744 { 02745 HAL_TIM_IC_CaptureCallback(htim); 02746 } 02747 /* Output compare event */ 02748 else 02749 { 02750 HAL_TIM_OC_DelayElapsedCallback(htim); 02751 HAL_TIM_PWM_PulseFinishedCallback(htim); 02752 } 02753 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 02754 } 02755 } 02756 } 02757 /* Capture compare 2 event */ 02758 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_CC2) != RESET) 02759 { 02760 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_CC2) !=RESET) 02761 { 02762 __HAL_TIM_CLEAR_IT(htim, TIM_IT_CC2); 02763 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_2; 02764 /* Input capture event */ 02765 if((htim->Instance->CCMR1 & TIM_CCMR1_CC2S) != 0x00) 02766 { 02767 HAL_TIM_IC_CaptureCallback(htim); 02768 } 02769 /* Output compare event */ 02770 else 02771 { 02772 HAL_TIM_OC_DelayElapsedCallback(htim); 02773 HAL_TIM_PWM_PulseFinishedCallback(htim); 02774 } 02775 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 02776 } 02777 } 02778 /* Capture compare 3 event */ 02779 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_CC3) != RESET) 02780 { 02781 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_CC3) !=RESET) 02782 { 02783 __HAL_TIM_CLEAR_IT(htim, TIM_IT_CC3); 02784 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_3; 02785 /* Input capture event */ 02786 if((htim->Instance->CCMR2 & TIM_CCMR2_CC3S) != 0x00) 02787 { 02788 HAL_TIM_IC_CaptureCallback(htim); 02789 } 02790 /* Output compare event */ 02791 else 02792 { 02793 HAL_TIM_OC_DelayElapsedCallback(htim); 02794 HAL_TIM_PWM_PulseFinishedCallback(htim); 02795 } 02796 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 02797 } 02798 } 02799 /* Capture compare 4 event */ 02800 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_CC4) != RESET) 02801 { 02802 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_CC4) !=RESET) 02803 { 02804 __HAL_TIM_CLEAR_IT(htim, TIM_IT_CC4); 02805 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_4; 02806 /* Input capture event */ 02807 if((htim->Instance->CCMR2 & TIM_CCMR2_CC4S) != 0x00) 02808 { 02809 HAL_TIM_IC_CaptureCallback(htim); 02810 } 02811 /* Output compare event */ 02812 else 02813 { 02814 HAL_TIM_OC_DelayElapsedCallback(htim); 02815 HAL_TIM_PWM_PulseFinishedCallback(htim); 02816 } 02817 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 02818 } 02819 } 02820 /* TIM Update event */ 02821 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_UPDATE) != RESET) 02822 { 02823 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_UPDATE) !=RESET) 02824 { 02825 __HAL_TIM_CLEAR_IT(htim, TIM_IT_UPDATE); 02826 HAL_TIM_PeriodElapsedCallback(htim); 02827 } 02828 } 02829 /* TIM Break input event */ 02830 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_BREAK) != RESET) 02831 { 02832 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_BREAK) !=RESET) 02833 { 02834 __HAL_TIM_CLEAR_IT(htim, TIM_IT_BREAK); 02835 HAL_TIMEx_BreakCallback(htim); 02836 } 02837 } 02838 /* TIM Trigger detection event */ 02839 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_TRIGGER) != RESET) 02840 { 02841 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_TRIGGER) !=RESET) 02842 { 02843 __HAL_TIM_CLEAR_IT(htim, TIM_IT_TRIGGER); 02844 HAL_TIM_TriggerCallback(htim); 02845 } 02846 } 02847 /* TIM commutation event */ 02848 if(__HAL_TIM_GET_FLAG(htim, TIM_FLAG_COM) != RESET) 02849 { 02850 if(__HAL_TIM_GET_IT_SOURCE(htim, TIM_IT_COM) !=RESET) 02851 { 02852 __HAL_TIM_CLEAR_IT(htim, TIM_FLAG_COM); 02853 HAL_TIMEx_CommutationCallback(htim); 02854 } 02855 } 02856 } 02857 02858 /** 02859 * @} 02860 */ 02861 02862 /** @defgroup TIM_Exported_Functions_Group8 Peripheral Control functions 02863 * @brief Peripheral Control functions 02864 * 02865 @verbatim 02866 ============================================================================== 02867 ##### Peripheral Control functions ##### 02868 ============================================================================== 02869 [..] 02870 This section provides functions allowing to: 02871 (+) Configure The Input Output channels for OC, PWM, IC or One Pulse mode. 02872 (+) Configure External Clock source. 02873 (+) Configure Complementary channels, break features and dead time. 02874 (+) Configure Master and the Slave synchronization. 02875 (+) Configure the DMA Burst Mode. 02876 02877 @endverbatim 02878 * @{ 02879 */ 02880 02881 /** 02882 * @brief Initializes the TIM Output Compare Channels according to the specified 02883 * parameters in the TIM_OC_InitTypeDef. 02884 * @param htim: TIM Output Compare handle 02885 * @param sConfig: TIM Output Compare configuration structure 02886 * @param Channel : TIM Channels to be enabled 02887 * This parameter can be one of the following values: 02888 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02889 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02890 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 02891 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 02892 * @arg TIM_CHANNEL_5: TIM Channel 5 selected 02893 * @arg TIM_CHANNEL_6: TIM Channel 6 selected 02894 * @retval HAL status 02895 */ 02896 __weak HAL_StatusTypeDef HAL_TIM_OC_ConfigChannel(TIM_HandleTypeDef *htim, TIM_OC_InitTypeDef* sConfig, uint32_t Channel) 02897 { 02898 /* Check the parameters */ 02899 assert_param(IS_TIM_CHANNELS(Channel)); 02900 assert_param(IS_TIM_OC_MODE(sConfig->OCMode)); 02901 assert_param(IS_TIM_OC_POLARITY(sConfig->OCPolarity)); 02902 assert_param(IS_TIM_OCN_POLARITY(sConfig->OCNPolarity)); 02903 assert_param(IS_TIM_OCNIDLE_STATE(sConfig->OCNIdleState)); 02904 assert_param(IS_TIM_OCIDLE_STATE(sConfig->OCIdleState)); 02905 02906 /* Check input state */ 02907 __HAL_LOCK(htim); 02908 02909 htim->State = HAL_TIM_STATE_BUSY; 02910 02911 switch (Channel) 02912 { 02913 case TIM_CHANNEL_1: 02914 { 02915 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 02916 /* Configure the TIM Channel 1 in Output Compare */ 02917 TIM_OC1_SetConfig(htim->Instance, sConfig); 02918 } 02919 break; 02920 02921 case TIM_CHANNEL_2: 02922 { 02923 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02924 /* Configure the TIM Channel 2 in Output Compare */ 02925 TIM_OC2_SetConfig(htim->Instance, sConfig); 02926 } 02927 break; 02928 02929 case TIM_CHANNEL_3: 02930 { 02931 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 02932 /* Configure the TIM Channel 3 in Output Compare */ 02933 TIM_OC3_SetConfig(htim->Instance, sConfig); 02934 } 02935 break; 02936 02937 case TIM_CHANNEL_4: 02938 { 02939 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 02940 /* Configure the TIM Channel 4 in Output Compare */ 02941 TIM_OC4_SetConfig(htim->Instance, sConfig); 02942 } 02943 break; 02944 02945 default: 02946 break; 02947 } 02948 htim->State = HAL_TIM_STATE_READY; 02949 02950 __HAL_UNLOCK(htim); 02951 02952 return HAL_OK; 02953 } 02954 02955 /** 02956 * @brief Initializes the TIM Input Capture Channels according to the specified 02957 * parameters in the TIM_IC_InitTypeDef. 02958 * @param htim: TIM IC handle 02959 * @param sConfig: TIM Input Capture configuration structure 02960 * @param Channel : TIM Channels to be enabled 02961 * This parameter can be one of the following values: 02962 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 02963 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 02964 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 02965 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 02966 * @retval HAL status 02967 */ 02968 HAL_StatusTypeDef HAL_TIM_IC_ConfigChannel(TIM_HandleTypeDef *htim, TIM_IC_InitTypeDef* sConfig, uint32_t Channel) 02969 { 02970 /* Check the parameters */ 02971 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 02972 assert_param(IS_TIM_IC_POLARITY(sConfig->ICPolarity)); 02973 assert_param(IS_TIM_IC_SELECTION(sConfig->ICSelection)); 02974 assert_param(IS_TIM_IC_PRESCALER(sConfig->ICPrescaler)); 02975 assert_param(IS_TIM_IC_FILTER(sConfig->ICFilter)); 02976 02977 __HAL_LOCK(htim); 02978 02979 htim->State = HAL_TIM_STATE_BUSY; 02980 02981 if (Channel == TIM_CHANNEL_1) 02982 { 02983 /* TI1 Configuration */ 02984 TIM_TI1_SetConfig(htim->Instance, 02985 sConfig->ICPolarity, 02986 sConfig->ICSelection, 02987 sConfig->ICFilter); 02988 02989 /* Reset the IC1PSC Bits */ 02990 htim->Instance->CCMR1 &= ~TIM_CCMR1_IC1PSC; 02991 02992 /* Set the IC1PSC value */ 02993 htim->Instance->CCMR1 |= sConfig->ICPrescaler; 02994 } 02995 else if (Channel == TIM_CHANNEL_2) 02996 { 02997 /* TI2 Configuration */ 02998 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 02999 03000 TIM_TI2_SetConfig(htim->Instance, 03001 sConfig->ICPolarity, 03002 sConfig->ICSelection, 03003 sConfig->ICFilter); 03004 03005 /* Reset the IC2PSC Bits */ 03006 htim->Instance->CCMR1 &= ~TIM_CCMR1_IC2PSC; 03007 03008 /* Set the IC2PSC value */ 03009 htim->Instance->CCMR1 |= (sConfig->ICPrescaler << 8); 03010 } 03011 else if (Channel == TIM_CHANNEL_3) 03012 { 03013 /* TI3 Configuration */ 03014 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 03015 03016 TIM_TI3_SetConfig(htim->Instance, 03017 sConfig->ICPolarity, 03018 sConfig->ICSelection, 03019 sConfig->ICFilter); 03020 03021 /* Reset the IC3PSC Bits */ 03022 htim->Instance->CCMR2 &= ~TIM_CCMR2_IC3PSC; 03023 03024 /* Set the IC3PSC value */ 03025 htim->Instance->CCMR2 |= sConfig->ICPrescaler; 03026 } 03027 else 03028 { 03029 /* TI4 Configuration */ 03030 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 03031 03032 TIM_TI4_SetConfig(htim->Instance, 03033 sConfig->ICPolarity, 03034 sConfig->ICSelection, 03035 sConfig->ICFilter); 03036 03037 /* Reset the IC4PSC Bits */ 03038 htim->Instance->CCMR2 &= ~TIM_CCMR2_IC4PSC; 03039 03040 /* Set the IC4PSC value */ 03041 htim->Instance->CCMR2 |= (sConfig->ICPrescaler << 8); 03042 } 03043 03044 htim->State = HAL_TIM_STATE_READY; 03045 03046 __HAL_UNLOCK(htim); 03047 03048 return HAL_OK; 03049 } 03050 03051 /** 03052 * @brief Initializes the TIM PWM channels according to the specified 03053 * parameters in the TIM_OC_InitTypeDef. 03054 * @param htim: TIM handle 03055 * @param sConfig: TIM PWM configuration structure 03056 * @param Channel : TIM Channels to be enabled 03057 * This parameter can be one of the following values: 03058 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 03059 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 03060 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 03061 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 03062 * @retval HAL status 03063 */ 03064 __weak HAL_StatusTypeDef HAL_TIM_PWM_ConfigChannel(TIM_HandleTypeDef *htim, TIM_OC_InitTypeDef* sConfig, uint32_t Channel) 03065 { 03066 __HAL_LOCK(htim); 03067 03068 /* Check the parameters */ 03069 assert_param(IS_TIM_CHANNELS(Channel)); 03070 assert_param(IS_TIM_PWM_MODE(sConfig->OCMode)); 03071 assert_param(IS_TIM_OC_POLARITY(sConfig->OCPolarity)); 03072 assert_param(IS_TIM_OCN_POLARITY(sConfig->OCNPolarity)); 03073 assert_param(IS_TIM_FAST_STATE(sConfig->OCFastMode)); 03074 assert_param(IS_TIM_OCNIDLE_STATE(sConfig->OCNIdleState)); 03075 assert_param(IS_TIM_OCIDLE_STATE(sConfig->OCIdleState)); 03076 03077 htim->State = HAL_TIM_STATE_BUSY; 03078 03079 switch (Channel) 03080 { 03081 case TIM_CHANNEL_1: 03082 { 03083 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 03084 /* Configure the Channel 1 in PWM mode */ 03085 TIM_OC1_SetConfig(htim->Instance, sConfig); 03086 03087 /* Set the Preload enable bit for channel1 */ 03088 htim->Instance->CCMR1 |= TIM_CCMR1_OC1PE; 03089 03090 /* Configure the Output Fast mode */ 03091 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC1FE; 03092 htim->Instance->CCMR1 |= sConfig->OCFastMode; 03093 } 03094 break; 03095 03096 case TIM_CHANNEL_2: 03097 { 03098 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 03099 /* Configure the Channel 2 in PWM mode */ 03100 TIM_OC2_SetConfig(htim->Instance, sConfig); 03101 03102 /* Set the Preload enable bit for channel2 */ 03103 htim->Instance->CCMR1 |= TIM_CCMR1_OC2PE; 03104 03105 /* Configure the Output Fast mode */ 03106 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC2FE; 03107 htim->Instance->CCMR1 |= sConfig->OCFastMode << 8; 03108 } 03109 break; 03110 03111 case TIM_CHANNEL_3: 03112 { 03113 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 03114 /* Configure the Channel 3 in PWM mode */ 03115 TIM_OC3_SetConfig(htim->Instance, sConfig); 03116 03117 /* Set the Preload enable bit for channel3 */ 03118 htim->Instance->CCMR2 |= TIM_CCMR2_OC3PE; 03119 03120 /* Configure the Output Fast mode */ 03121 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC3FE; 03122 htim->Instance->CCMR2 |= sConfig->OCFastMode; 03123 } 03124 break; 03125 03126 case TIM_CHANNEL_4: 03127 { 03128 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 03129 /* Configure the Channel 4 in PWM mode */ 03130 TIM_OC4_SetConfig(htim->Instance, sConfig); 03131 03132 /* Set the Preload enable bit for channel4 */ 03133 htim->Instance->CCMR2 |= TIM_CCMR2_OC4PE; 03134 03135 /* Configure the Output Fast mode */ 03136 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC4FE; 03137 htim->Instance->CCMR2 |= sConfig->OCFastMode << 8; 03138 } 03139 break; 03140 03141 default: 03142 break; 03143 } 03144 03145 htim->State = HAL_TIM_STATE_READY; 03146 03147 __HAL_UNLOCK(htim); 03148 03149 return HAL_OK; 03150 } 03151 03152 /** 03153 * @brief Initializes the TIM One Pulse Channels according to the specified 03154 * parameters in the TIM_OnePulse_InitTypeDef. 03155 * @param htim: TIM One Pulse handle 03156 * @param sConfig: TIM One Pulse configuration structure 03157 * @param OutputChannel : TIM Channels to be enabled 03158 * This parameter can be one of the following values: 03159 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 03160 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 03161 * @param InputChannel : TIM Channels to be enabled 03162 * This parameter can be one of the following values: 03163 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 03164 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 03165 * @retval HAL status 03166 */ 03167 HAL_StatusTypeDef HAL_TIM_OnePulse_ConfigChannel(TIM_HandleTypeDef *htim, TIM_OnePulse_InitTypeDef* sConfig, uint32_t OutputChannel, uint32_t InputChannel) 03168 { 03169 TIM_OC_InitTypeDef temp1; 03170 03171 /* Check the parameters */ 03172 assert_param(IS_TIM_OPM_CHANNELS(OutputChannel)); 03173 assert_param(IS_TIM_OPM_CHANNELS(InputChannel)); 03174 03175 if(OutputChannel != InputChannel) 03176 { 03177 __HAL_LOCK(htim); 03178 03179 htim->State = HAL_TIM_STATE_BUSY; 03180 03181 /* Extract the Ouput compare configuration from sConfig structure */ 03182 temp1.OCMode = sConfig->OCMode; 03183 temp1.Pulse = sConfig->Pulse; 03184 temp1.OCPolarity = sConfig->OCPolarity; 03185 temp1.OCNPolarity = sConfig->OCNPolarity; 03186 temp1.OCIdleState = sConfig->OCIdleState; 03187 temp1.OCNIdleState = sConfig->OCNIdleState; 03188 03189 switch (OutputChannel) 03190 { 03191 case TIM_CHANNEL_1: 03192 { 03193 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 03194 03195 TIM_OC1_SetConfig(htim->Instance, &temp1); 03196 } 03197 break; 03198 case TIM_CHANNEL_2: 03199 { 03200 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 03201 03202 TIM_OC2_SetConfig(htim->Instance, &temp1); 03203 } 03204 break; 03205 default: 03206 break; 03207 } 03208 switch (InputChannel) 03209 { 03210 case TIM_CHANNEL_1: 03211 { 03212 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 03213 03214 TIM_TI1_SetConfig(htim->Instance, sConfig->ICPolarity, 03215 sConfig->ICSelection, sConfig->ICFilter); 03216 03217 /* Reset the IC1PSC Bits */ 03218 htim->Instance->CCMR1 &= ~TIM_CCMR1_IC1PSC; 03219 03220 /* Select the Trigger source */ 03221 htim->Instance->SMCR &= ~TIM_SMCR_TS; 03222 htim->Instance->SMCR |= TIM_TS_TI1FP1; 03223 03224 /* Select the Slave Mode */ 03225 htim->Instance->SMCR &= ~TIM_SMCR_SMS; 03226 htim->Instance->SMCR |= TIM_SLAVEMODE_TRIGGER; 03227 } 03228 break; 03229 case TIM_CHANNEL_2: 03230 { 03231 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 03232 03233 TIM_TI2_SetConfig(htim->Instance, sConfig->ICPolarity, 03234 sConfig->ICSelection, sConfig->ICFilter); 03235 03236 /* Reset the IC2PSC Bits */ 03237 htim->Instance->CCMR1 &= ~TIM_CCMR1_IC2PSC; 03238 03239 /* Select the Trigger source */ 03240 htim->Instance->SMCR &= ~TIM_SMCR_TS; 03241 htim->Instance->SMCR |= TIM_TS_TI2FP2; 03242 03243 /* Select the Slave Mode */ 03244 htim->Instance->SMCR &= ~TIM_SMCR_SMS; 03245 htim->Instance->SMCR |= TIM_SLAVEMODE_TRIGGER; 03246 } 03247 break; 03248 03249 default: 03250 break; 03251 } 03252 03253 htim->State = HAL_TIM_STATE_READY; 03254 03255 __HAL_UNLOCK(htim); 03256 03257 return HAL_OK; 03258 } 03259 else 03260 { 03261 return HAL_ERROR; 03262 } 03263 } 03264 03265 /** 03266 * @brief Configure the DMA Burst to transfer Data from the memory to the TIM peripheral 03267 * @param htim: TIM handle 03268 * @param BurstBaseAddress: TIM Base address from when the DMA will starts the Data write 03269 * This parameters can be on of the following values: 03270 * @arg TIM_DMABASE_CR1 03271 * @arg TIM_DMABASE_CR2 03272 * @arg TIM_DMABASE_SMCR 03273 * @arg TIM_DMABASE_DIER 03274 * @arg TIM_DMABASE_SR 03275 * @arg TIM_DMABASE_EGR 03276 * @arg TIM_DMABASE_CCMR1 03277 * @arg TIM_DMABASE_CCMR2 03278 * @arg TIM_DMABASE_CCER 03279 * @arg TIM_DMABASE_CNT 03280 * @arg TIM_DMABASE_PSC 03281 * @arg TIM_DMABASE_ARR 03282 * @arg TIM_DMABASE_RCR 03283 * @arg TIM_DMABASE_CCR1 03284 * @arg TIM_DMABASE_CCR2 03285 * @arg TIM_DMABASE_CCR3 03286 * @arg TIM_DMABASE_CCR4 03287 * @arg TIM_DMABASE_BDTR 03288 * @arg TIM_DMABASE_DCR 03289 * @param BurstRequestSrc: TIM DMA Request sources 03290 * This parameters can be on of the following values: 03291 * @arg TIM_DMA_UPDATE: TIM update Interrupt source 03292 * @arg TIM_DMA_CC1: TIM Capture Compare 1 DMA source 03293 * @arg TIM_DMA_CC2: TIM Capture Compare 2 DMA source 03294 * @arg TIM_DMA_CC3: TIM Capture Compare 3 DMA source 03295 * @arg TIM_DMA_CC4: TIM Capture Compare 4 DMA source 03296 * @arg TIM_DMA_COM: TIM Commutation DMA source 03297 * @arg TIM_DMA_TRIGGER: TIM Trigger DMA source 03298 * @param BurstBuffer: The Buffer address. 03299 * @param BurstLength: DMA Burst length. This parameter can be one value 03300 * between: TIM_DMABurstLength_1Transfer and TIM_DMABurstLength_18Transfers. 03301 * @retval HAL status 03302 */ 03303 HAL_StatusTypeDef HAL_TIM_DMABurst_WriteStart(TIM_HandleTypeDef *htim, uint32_t BurstBaseAddress, uint32_t BurstRequestSrc, 03304 uint32_t* BurstBuffer, uint32_t BurstLength) 03305 { 03306 /* Check the parameters */ 03307 assert_param(IS_TIM_DMABURST_INSTANCE(htim->Instance)); 03308 assert_param(IS_TIM_DMA_BASE(BurstBaseAddress)); 03309 assert_param(IS_TIM_DMA_SOURCE(BurstRequestSrc)); 03310 assert_param(IS_TIM_DMA_LENGTH(BurstLength)); 03311 03312 if((htim->State == HAL_TIM_STATE_BUSY)) 03313 { 03314 return HAL_BUSY; 03315 } 03316 else if((htim->State == HAL_TIM_STATE_READY)) 03317 { 03318 if((BurstBuffer == 0 ) && (BurstLength > 0)) 03319 { 03320 return HAL_ERROR; 03321 } 03322 else 03323 { 03324 htim->State = HAL_TIM_STATE_BUSY; 03325 } 03326 } 03327 switch(BurstRequestSrc) 03328 { 03329 case TIM_DMA_UPDATE: 03330 { 03331 /* Set the DMA Period elapsed callback */ 03332 htim->hdma[TIM_DMA_ID_UPDATE]->XferCpltCallback = TIM_DMAPeriodElapsedCplt; 03333 03334 /* Set the DMA error callback */ 03335 htim->hdma[TIM_DMA_ID_UPDATE]->XferErrorCallback = TIM_DMAError ; 03336 03337 /* Enable the DMA channel */ 03338 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_UPDATE], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03339 } 03340 break; 03341 case TIM_DMA_CC1: 03342 { 03343 /* Set the DMA Period elapsed callback */ 03344 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMADelayPulseCplt; 03345 03346 /* Set the DMA error callback */ 03347 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 03348 03349 /* Enable the DMA channel */ 03350 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03351 } 03352 break; 03353 case TIM_DMA_CC2: 03354 { 03355 /* Set the DMA Period elapsed callback */ 03356 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMADelayPulseCplt; 03357 03358 /* Set the DMA error callback */ 03359 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 03360 03361 /* Enable the DMA channel */ 03362 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03363 } 03364 break; 03365 case TIM_DMA_CC3: 03366 { 03367 /* Set the DMA Period elapsed callback */ 03368 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMADelayPulseCplt; 03369 03370 /* Set the DMA error callback */ 03371 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 03372 03373 /* Enable the DMA channel */ 03374 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03375 } 03376 break; 03377 case TIM_DMA_CC4: 03378 { 03379 /* Set the DMA Period elapsed callback */ 03380 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMADelayPulseCplt; 03381 03382 /* Set the DMA error callback */ 03383 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 03384 03385 /* Enable the DMA channel */ 03386 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03387 } 03388 break; 03389 case TIM_DMA_COM: 03390 { 03391 /* Set the DMA Period elapsed callback */ 03392 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferCpltCallback = TIMEx_DMACommutationCplt; 03393 03394 /* Set the DMA error callback */ 03395 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferErrorCallback = TIM_DMAError ; 03396 03397 /* Enable the DMA channel */ 03398 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_COMMUTATION], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03399 } 03400 break; 03401 case TIM_DMA_TRIGGER: 03402 { 03403 /* Set the DMA Period elapsed callback */ 03404 htim->hdma[TIM_DMA_ID_TRIGGER]->XferCpltCallback = TIM_DMATriggerCplt; 03405 03406 /* Set the DMA error callback */ 03407 htim->hdma[TIM_DMA_ID_TRIGGER]->XferErrorCallback = TIM_DMAError ; 03408 03409 /* Enable the DMA channel */ 03410 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_TRIGGER], (uint32_t)BurstBuffer, (uint32_t)&htim->Instance->DMAR, ((BurstLength) >> 8) + 1); 03411 } 03412 break; 03413 default: 03414 break; 03415 } 03416 /* configure the DMA Burst Mode */ 03417 htim->Instance->DCR = BurstBaseAddress | BurstLength; 03418 03419 /* Enable the TIM DMA Request */ 03420 __HAL_TIM_ENABLE_DMA(htim, BurstRequestSrc); 03421 03422 htim->State = HAL_TIM_STATE_READY; 03423 03424 /* Return function status */ 03425 return HAL_OK; 03426 } 03427 03428 /** 03429 * @brief Stops the TIM DMA Burst mode 03430 * @param htim: TIM handle 03431 * @param BurstRequestSrc: TIM DMA Request sources to disable 03432 * @retval HAL status 03433 */ 03434 HAL_StatusTypeDef HAL_TIM_DMABurst_WriteStop(TIM_HandleTypeDef *htim, uint32_t BurstRequestSrc) 03435 { 03436 /* Check the parameters */ 03437 assert_param(IS_TIM_DMA_SOURCE(BurstRequestSrc)); 03438 03439 /* Abort the DMA transfer (at least disable the DMA channel) */ 03440 switch(BurstRequestSrc) 03441 { 03442 case TIM_DMA_UPDATE: 03443 { 03444 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_UPDATE]); 03445 } 03446 break; 03447 case TIM_DMA_CC1: 03448 { 03449 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC1]); 03450 } 03451 break; 03452 case TIM_DMA_CC2: 03453 { 03454 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC2]); 03455 } 03456 break; 03457 case TIM_DMA_CC3: 03458 { 03459 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC3]); 03460 } 03461 break; 03462 case TIM_DMA_CC4: 03463 { 03464 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC4]); 03465 } 03466 break; 03467 case TIM_DMA_COM: 03468 { 03469 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_COMMUTATION]); 03470 } 03471 break; 03472 case TIM_DMA_TRIGGER: 03473 { 03474 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_TRIGGER]); 03475 } 03476 break; 03477 default: 03478 break; 03479 } 03480 03481 /* Disable the TIM Update DMA request */ 03482 __HAL_TIM_DISABLE_DMA(htim, BurstRequestSrc); 03483 03484 /* Return function status */ 03485 return HAL_OK; 03486 } 03487 03488 /** 03489 * @brief Configure the DMA Burst to transfer Data from the TIM peripheral to the memory 03490 * @param htim: TIM handle 03491 * @param BurstBaseAddress: TIM Base address from when the DMA will starts the Data read 03492 * This parameters can be on of the following values: 03493 * @arg TIM_DMABASE_CR1 03494 * @arg TIM_DMABASE_CR2 03495 * @arg TIM_DMABASE_SMCR 03496 * @arg TIM_DMABASE_DIER 03497 * @arg TIM_DMABASE_SR 03498 * @arg TIM_DMABASE_EGR 03499 * @arg TIM_DMABASE_CCMR1 03500 * @arg TIM_DMABASE_CCMR2 03501 * @arg TIM_DMABASE_CCER 03502 * @arg TIM_DMABASE_CNT 03503 * @arg TIM_DMABASE_PSC 03504 * @arg TIM_DMABASE_ARR 03505 * @arg TIM_DMABASE_RCR 03506 * @arg TIM_DMABASE_CCR1 03507 * @arg TIM_DMABASE_CCR2 03508 * @arg TIM_DMABASE_CCR3 03509 * @arg TIM_DMABASE_CCR4 03510 * @arg TIM_DMABASE_BDTR 03511 * @arg TIM_DMABASE_DCR 03512 * @param BurstRequestSrc: TIM DMA Request sources 03513 * This parameters can be on of the following values: 03514 * @arg TIM_DMA_UPDATE: TIM update Interrupt source 03515 * @arg TIM_DMA_CC1: TIM Capture Compare 1 DMA source 03516 * @arg TIM_DMA_CC2: TIM Capture Compare 2 DMA source 03517 * @arg TIM_DMA_CC3: TIM Capture Compare 3 DMA source 03518 * @arg TIM_DMA_CC4: TIM Capture Compare 4 DMA source 03519 * @arg TIM_DMA_COM: TIM Commutation DMA source 03520 * @arg TIM_DMA_TRIGGER: TIM Trigger DMA source 03521 * @param BurstBuffer: The Buffer address. 03522 * @param BurstLength: DMA Burst length. This parameter can be one value 03523 * between: TIM_DMABurstLength_1Transfer and TIM_DMABurstLength_18Transfers. 03524 * @retval HAL status 03525 */ 03526 HAL_StatusTypeDef HAL_TIM_DMABurst_ReadStart(TIM_HandleTypeDef *htim, uint32_t BurstBaseAddress, uint32_t BurstRequestSrc, 03527 uint32_t *BurstBuffer, uint32_t BurstLength) 03528 { 03529 /* Check the parameters */ 03530 assert_param(IS_TIM_DMABURST_INSTANCE(htim->Instance)); 03531 assert_param(IS_TIM_DMA_BASE(BurstBaseAddress)); 03532 assert_param(IS_TIM_DMA_SOURCE(BurstRequestSrc)); 03533 assert_param(IS_TIM_DMA_LENGTH(BurstLength)); 03534 03535 if((htim->State == HAL_TIM_STATE_BUSY)) 03536 { 03537 return HAL_BUSY; 03538 } 03539 else if((htim->State == HAL_TIM_STATE_READY)) 03540 { 03541 if((BurstBuffer == 0 ) && (BurstLength > 0)) 03542 { 03543 return HAL_ERROR; 03544 } 03545 else 03546 { 03547 htim->State = HAL_TIM_STATE_BUSY; 03548 } 03549 } 03550 switch(BurstRequestSrc) 03551 { 03552 case TIM_DMA_UPDATE: 03553 { 03554 /* Set the DMA Period elapsed callback */ 03555 htim->hdma[TIM_DMA_ID_UPDATE]->XferCpltCallback = TIM_DMAPeriodElapsedCplt; 03556 03557 /* Set the DMA error callback */ 03558 htim->hdma[TIM_DMA_ID_UPDATE]->XferErrorCallback = TIM_DMAError ; 03559 03560 /* Enable the DMA channel */ 03561 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_UPDATE], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03562 } 03563 break; 03564 case TIM_DMA_CC1: 03565 { 03566 /* Set the DMA Period elapsed callback */ 03567 htim->hdma[TIM_DMA_ID_CC1]->XferCpltCallback = TIM_DMACaptureCplt; 03568 03569 /* Set the DMA error callback */ 03570 htim->hdma[TIM_DMA_ID_CC1]->XferErrorCallback = TIM_DMAError ; 03571 03572 /* Enable the DMA channel */ 03573 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC1], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03574 } 03575 break; 03576 case TIM_DMA_CC2: 03577 { 03578 /* Set the DMA Period elapsed callback */ 03579 htim->hdma[TIM_DMA_ID_CC2]->XferCpltCallback = TIM_DMACaptureCplt; 03580 03581 /* Set the DMA error callback */ 03582 htim->hdma[TIM_DMA_ID_CC2]->XferErrorCallback = TIM_DMAError ; 03583 03584 /* Enable the DMA channel */ 03585 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC2], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03586 } 03587 break; 03588 case TIM_DMA_CC3: 03589 { 03590 /* Set the DMA Period elapsed callback */ 03591 htim->hdma[TIM_DMA_ID_CC3]->XferCpltCallback = TIM_DMACaptureCplt; 03592 03593 /* Set the DMA error callback */ 03594 htim->hdma[TIM_DMA_ID_CC3]->XferErrorCallback = TIM_DMAError ; 03595 03596 /* Enable the DMA channel */ 03597 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC3], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03598 } 03599 break; 03600 case TIM_DMA_CC4: 03601 { 03602 /* Set the DMA Period elapsed callback */ 03603 htim->hdma[TIM_DMA_ID_CC4]->XferCpltCallback = TIM_DMACaptureCplt; 03604 03605 /* Set the DMA error callback */ 03606 htim->hdma[TIM_DMA_ID_CC4]->XferErrorCallback = TIM_DMAError ; 03607 03608 /* Enable the DMA channel */ 03609 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_CC4], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03610 } 03611 break; 03612 case TIM_DMA_COM: 03613 { 03614 /* Set the DMA Period elapsed callback */ 03615 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferCpltCallback = TIMEx_DMACommutationCplt; 03616 03617 /* Set the DMA error callback */ 03618 htim->hdma[TIM_DMA_ID_COMMUTATION]->XferErrorCallback = TIM_DMAError ; 03619 03620 /* Enable the DMA channel */ 03621 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_COMMUTATION], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03622 } 03623 break; 03624 case TIM_DMA_TRIGGER: 03625 { 03626 /* Set the DMA Period elapsed callback */ 03627 htim->hdma[TIM_DMA_ID_TRIGGER]->XferCpltCallback = TIM_DMATriggerCplt; 03628 03629 /* Set the DMA error callback */ 03630 htim->hdma[TIM_DMA_ID_TRIGGER]->XferErrorCallback = TIM_DMAError ; 03631 03632 /* Enable the DMA channel */ 03633 HAL_DMA_Start_IT(htim->hdma[TIM_DMA_ID_TRIGGER], (uint32_t)&htim->Instance->DMAR, (uint32_t)BurstBuffer, ((BurstLength) >> 8) + 1); 03634 } 03635 break; 03636 default: 03637 break; 03638 } 03639 03640 /* configure the DMA Burst Mode */ 03641 htim->Instance->DCR = BurstBaseAddress | BurstLength; 03642 03643 /* Enable the TIM DMA Request */ 03644 __HAL_TIM_ENABLE_DMA(htim, BurstRequestSrc); 03645 03646 htim->State = HAL_TIM_STATE_READY; 03647 03648 /* Return function status */ 03649 return HAL_OK; 03650 } 03651 03652 /** 03653 * @brief Stop the DMA burst reading 03654 * @param htim: TIM handle 03655 * @param BurstRequestSrc: TIM DMA Request sources to disable. 03656 * @retval HAL status 03657 */ 03658 HAL_StatusTypeDef HAL_TIM_DMABurst_ReadStop(TIM_HandleTypeDef *htim, uint32_t BurstRequestSrc) 03659 { 03660 /* Check the parameters */ 03661 assert_param(IS_TIM_DMA_SOURCE(BurstRequestSrc)); 03662 03663 /* Abort the DMA transfer (at least disable the DMA channel) */ 03664 switch(BurstRequestSrc) 03665 { 03666 case TIM_DMA_UPDATE: 03667 { 03668 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_UPDATE]); 03669 } 03670 break; 03671 case TIM_DMA_CC1: 03672 { 03673 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC1]); 03674 } 03675 break; 03676 case TIM_DMA_CC2: 03677 { 03678 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC2]); 03679 } 03680 break; 03681 case TIM_DMA_CC3: 03682 { 03683 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC3]); 03684 } 03685 break; 03686 case TIM_DMA_CC4: 03687 { 03688 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_CC4]); 03689 } 03690 break; 03691 case TIM_DMA_COM: 03692 { 03693 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_COMMUTATION]); 03694 } 03695 break; 03696 case TIM_DMA_TRIGGER: 03697 { 03698 HAL_DMA_Abort(htim->hdma[TIM_DMA_ID_TRIGGER]); 03699 } 03700 break; 03701 default: 03702 break; 03703 } 03704 03705 /* Disable the TIM Update DMA request */ 03706 __HAL_TIM_DISABLE_DMA(htim, BurstRequestSrc); 03707 03708 /* Return function status */ 03709 return HAL_OK; 03710 } 03711 03712 /** 03713 * @brief Generate a software event 03714 * @param htim: TIM handle 03715 * @param EventSource: specifies the event source. 03716 * This parameter can be one of the following values: 03717 * @arg TIM_EVENTSOURCE_UPDATE: Timer update Event source 03718 * @arg TIM_EVENTSOURCE_CC1: Timer Capture Compare 1 Event source 03719 * @arg TIM_EVENTSOURCE_CC2: Timer Capture Compare 2 Event source 03720 * @arg TIM_EVENTSOURCE_CC3: Timer Capture Compare 3 Event source 03721 * @arg TIM_EVENTSOURCE_CC4: Timer Capture Compare 4 Event source 03722 * @arg TIM_EVENTSOURCE_COM: Timer COM event source 03723 * @arg TIM_EVENTSOURCE_TRIGGER: Timer Trigger Event source 03724 * @arg TIM_EVENTSOURCE_BREAK: Timer Break event source 03725 * @arg TIM_EVENTSOURCE_BREAK2: Timer Break2 event source 03726 * @retval None 03727 */ 03728 03729 HAL_StatusTypeDef HAL_TIM_GenerateEvent(TIM_HandleTypeDef *htim, uint32_t EventSource) 03730 { 03731 /* Check the parameters */ 03732 assert_param(IS_TIM_INSTANCE(htim->Instance)); 03733 assert_param(IS_TIM_EVENT_SOURCE(EventSource)); 03734 03735 /* Process Locked */ 03736 __HAL_LOCK(htim); 03737 03738 /* Change the TIM state */ 03739 htim->State = HAL_TIM_STATE_BUSY; 03740 03741 /* Set the event sources */ 03742 htim->Instance->EGR = EventSource; 03743 03744 /* Change the TIM state */ 03745 htim->State = HAL_TIM_STATE_READY; 03746 03747 __HAL_UNLOCK(htim); 03748 03749 /* Return function status */ 03750 return HAL_OK; 03751 } 03752 03753 /** 03754 * @brief Configures the OCRef clear feature 03755 * @param htim: TIM handle 03756 * @param sClearInputConfig: pointer to a TIM_ClearInputConfigTypeDef structure that 03757 * contains the OCREF clear feature and parameters for the TIM peripheral. 03758 * @param Channel: specifies the TIM Channel 03759 * This parameter can be one of the following values: 03760 * @arg TIM_CHANNEL_1: TIM Channel 1 03761 * @arg TIM_CHANNEL_2: TIM Channel 2 03762 * @arg TIM_CHANNEL_3: TIM Channel 3 03763 * @arg TIM_CHANNEL_4: TIM Channel 4 03764 * @retval HAL status 03765 */ 03766 __weak HAL_StatusTypeDef HAL_TIM_ConfigOCrefClear(TIM_HandleTypeDef *htim, TIM_ClearInputConfigTypeDef * sClearInputConfig, uint32_t Channel) 03767 { 03768 /* Check the parameters */ 03769 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 03770 assert_param(IS_TIM_CHANNELS(Channel)); 03771 assert_param(IS_TIM_CLEARINPUT_SOURCE(sClearInputConfig->ClearInputSource)); 03772 03773 /* Process Locked */ 03774 __HAL_LOCK(htim); 03775 03776 htim->State = HAL_TIM_STATE_BUSY; 03777 03778 if(sClearInputConfig->ClearInputSource == TIM_CLEARINPUTSOURCE_ETR) 03779 { 03780 /* Check the parameters */ 03781 assert_param(IS_TIM_CLEARINPUT_POLARITY(sClearInputConfig->ClearInputPolarity)); 03782 assert_param(IS_TIM_CLEARINPUT_PRESCALER(sClearInputConfig->ClearInputPrescaler)); 03783 assert_param(IS_TIM_CLEARINPUT_FILTER(sClearInputConfig->ClearInputFilter)); 03784 03785 TIM_ETR_SetConfig(htim->Instance, 03786 sClearInputConfig->ClearInputPrescaler, 03787 sClearInputConfig->ClearInputPolarity, 03788 sClearInputConfig->ClearInputFilter); 03789 } 03790 03791 switch (Channel) 03792 { 03793 case TIM_CHANNEL_1: 03794 { 03795 if(sClearInputConfig->ClearInputState != RESET) 03796 { 03797 /* Enable the OCREF clear feature for Channel 1 */ 03798 htim->Instance->CCMR1 |= TIM_CCMR1_OC1CE; 03799 } 03800 else 03801 { 03802 /* Disable the OCREF clear feature for Channel 1 */ 03803 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC1CE; 03804 } 03805 } 03806 break; 03807 case TIM_CHANNEL_2: 03808 { 03809 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 03810 if(sClearInputConfig->ClearInputState != RESET) 03811 { 03812 /* Enable the OCREF clear feature for Channel 2 */ 03813 htim->Instance->CCMR1 |= TIM_CCMR1_OC2CE; 03814 } 03815 else 03816 { 03817 /* Disable the OCREF clear feature for Channel 2 */ 03818 htim->Instance->CCMR1 &= ~TIM_CCMR1_OC2CE; 03819 } 03820 } 03821 break; 03822 case TIM_CHANNEL_3: 03823 { 03824 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 03825 if(sClearInputConfig->ClearInputState != RESET) 03826 { 03827 /* Enable the OCREF clear feature for Channel 3 */ 03828 htim->Instance->CCMR2 |= TIM_CCMR2_OC3CE; 03829 } 03830 else 03831 { 03832 /* Disable the OCREF clear feature for Channel 3 */ 03833 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC3CE; 03834 } 03835 } 03836 break; 03837 case TIM_CHANNEL_4: 03838 { 03839 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 03840 if(sClearInputConfig->ClearInputState != RESET) 03841 { 03842 /* Enable the OCREF clear feature for Channel 4 */ 03843 htim->Instance->CCMR2 |= TIM_CCMR2_OC4CE; 03844 } 03845 else 03846 { 03847 /* Disable the OCREF clear feature for Channel 4 */ 03848 htim->Instance->CCMR2 &= ~TIM_CCMR2_OC4CE; 03849 } 03850 } 03851 break; 03852 default: 03853 break; 03854 } 03855 03856 htim->State = HAL_TIM_STATE_READY; 03857 03858 __HAL_UNLOCK(htim); 03859 03860 return HAL_OK; 03861 } 03862 03863 /** 03864 * @brief Configures the clock source to be used 03865 * @param htim: TIM handle 03866 * @param sClockSourceConfig: pointer to a TIM_ClockConfigTypeDef structure that 03867 * contains the clock source information for the TIM peripheral. 03868 * @retval HAL status 03869 */ 03870 HAL_StatusTypeDef HAL_TIM_ConfigClockSource(TIM_HandleTypeDef *htim, TIM_ClockConfigTypeDef * sClockSourceConfig) 03871 { 03872 uint32_t tmpsmcr = 0; 03873 03874 /* Process Locked */ 03875 __HAL_LOCK(htim); 03876 03877 htim->State = HAL_TIM_STATE_BUSY; 03878 03879 /* Check the parameters */ 03880 assert_param(IS_TIM_CLOCKSOURCE(sClockSourceConfig->ClockSource)); 03881 assert_param(IS_TIM_CLOCKPOLARITY(sClockSourceConfig->ClockPolarity)); 03882 assert_param(IS_TIM_CLOCKPRESCALER(sClockSourceConfig->ClockPrescaler)); 03883 assert_param(IS_TIM_CLOCKFILTER(sClockSourceConfig->ClockFilter)); 03884 03885 /* Reset the SMS, TS, ECE, ETPS and ETRF bits */ 03886 tmpsmcr = htim->Instance->SMCR; 03887 tmpsmcr &= ~(TIM_SMCR_SMS | TIM_SMCR_TS); 03888 tmpsmcr &= ~(TIM_SMCR_ETF | TIM_SMCR_ETPS | TIM_SMCR_ECE | TIM_SMCR_ETP); 03889 htim->Instance->SMCR = tmpsmcr; 03890 03891 switch (sClockSourceConfig->ClockSource) 03892 { 03893 case TIM_CLOCKSOURCE_INTERNAL: 03894 { 03895 assert_param(IS_TIM_INSTANCE(htim->Instance)); 03896 /* Disable slave mode to clock the prescaler directly with the internal clock */ 03897 htim->Instance->SMCR &= ~TIM_SMCR_SMS; 03898 } 03899 break; 03900 03901 case TIM_CLOCKSOURCE_ETRMODE1: 03902 { 03903 /* Check whether or not the timer instance supports external trigger input mode 1 (ETRF)*/ 03904 assert_param(IS_TIM_CLOCKSOURCE_ETRMODE1_INSTANCE(htim->Instance)); 03905 03906 /* Configure the ETR Clock source */ 03907 TIM_ETR_SetConfig(htim->Instance, 03908 sClockSourceConfig->ClockPrescaler, 03909 sClockSourceConfig->ClockPolarity, 03910 sClockSourceConfig->ClockFilter); 03911 /* Get the TIMx SMCR register value */ 03912 tmpsmcr = htim->Instance->SMCR; 03913 /* Reset the SMS and TS Bits */ 03914 tmpsmcr &= ~(TIM_SMCR_SMS | TIM_SMCR_TS); 03915 /* Select the External clock mode1 and the ETRF trigger */ 03916 tmpsmcr |= (TIM_SLAVEMODE_EXTERNAL1 | TIM_CLOCKSOURCE_ETRMODE1); 03917 /* Write to TIMx SMCR */ 03918 htim->Instance->SMCR = tmpsmcr; 03919 } 03920 break; 03921 03922 case TIM_CLOCKSOURCE_ETRMODE2: 03923 { 03924 /* Check whether or not the timer instance supports external trigger input mode 2 (ETRF)*/ 03925 assert_param(IS_TIM_CLOCKSOURCE_ETRMODE2_INSTANCE(htim->Instance)); 03926 03927 /* Configure the ETR Clock source */ 03928 TIM_ETR_SetConfig(htim->Instance, 03929 sClockSourceConfig->ClockPrescaler, 03930 sClockSourceConfig->ClockPolarity, 03931 sClockSourceConfig->ClockFilter); 03932 /* Enable the External clock mode2 */ 03933 htim->Instance->SMCR |= TIM_SMCR_ECE; 03934 } 03935 break; 03936 03937 case TIM_CLOCKSOURCE_TI1: 03938 { 03939 /* Check whether or not the timer instance supports external clock mode 1 */ 03940 assert_param(IS_TIM_CLOCKSOURCE_TIX_INSTANCE(htim->Instance)); 03941 03942 TIM_TI1_ConfigInputStage(htim->Instance, 03943 sClockSourceConfig->ClockPolarity, 03944 sClockSourceConfig->ClockFilter); 03945 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_TI1); 03946 } 03947 break; 03948 03949 case TIM_CLOCKSOURCE_TI2: 03950 { 03951 /* Check whether or not the timer instance supports external clock mode 1 (ETRF)*/ 03952 assert_param(IS_TIM_CLOCKSOURCE_TIX_INSTANCE(htim->Instance)); 03953 03954 TIM_TI2_ConfigInputStage(htim->Instance, 03955 sClockSourceConfig->ClockPolarity, 03956 sClockSourceConfig->ClockFilter); 03957 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_TI2); 03958 } 03959 break; 03960 03961 case TIM_CLOCKSOURCE_TI1ED: 03962 { 03963 /* Check whether or not the timer instance supports external clock mode 1 */ 03964 assert_param(IS_TIM_CLOCKSOURCE_TIX_INSTANCE(htim->Instance)); 03965 03966 TIM_TI1_ConfigInputStage(htim->Instance, 03967 sClockSourceConfig->ClockPolarity, 03968 sClockSourceConfig->ClockFilter); 03969 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_TI1ED); 03970 } 03971 break; 03972 03973 case TIM_CLOCKSOURCE_ITR0: 03974 { 03975 /* Check whether or not the timer instance supports internal trigger input */ 03976 assert_param(IS_TIM_CLOCKSOURCE_ITRX_INSTANCE(htim->Instance)); 03977 03978 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_ITR0); 03979 } 03980 break; 03981 03982 case TIM_CLOCKSOURCE_ITR1: 03983 { 03984 /* Check whether or not the timer instance supports internal trigger input */ 03985 assert_param(IS_TIM_CLOCKSOURCE_ITRX_INSTANCE(htim->Instance)); 03986 03987 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_ITR1); 03988 } 03989 break; 03990 03991 case TIM_CLOCKSOURCE_ITR2: 03992 { 03993 /* Check whether or not the timer instance supports internal trigger input */ 03994 assert_param(IS_TIM_CLOCKSOURCE_ITRX_INSTANCE(htim->Instance)); 03995 03996 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_ITR2); 03997 } 03998 break; 03999 04000 case TIM_CLOCKSOURCE_ITR3: 04001 { 04002 /* Check whether or not the timer instance supports internal trigger input */ 04003 assert_param(IS_TIM_CLOCKSOURCE_ITRX_INSTANCE(htim->Instance)); 04004 04005 TIM_ITRx_SetConfig(htim->Instance, TIM_CLOCKSOURCE_ITR3); 04006 } 04007 break; 04008 04009 default: 04010 break; 04011 } 04012 htim->State = HAL_TIM_STATE_READY; 04013 04014 __HAL_UNLOCK(htim); 04015 04016 return HAL_OK; 04017 } 04018 04019 /** 04020 * @brief Selects the signal connected to the TI1 input: direct from CH1_input 04021 * or a XOR combination between CH1_input, CH2_input & CH3_input 04022 * @param htim: TIM handle. 04023 * @param TI1_Selection: Indicate whether or not channel 1 is connected to the 04024 * output of a XOR gate. 04025 * This parameter can be one of the following values: 04026 * @arg TIM_TI1SELECTION_CH1: The TIMx_CH1 pin is connected to TI1 input 04027 * @arg TIM_TI1SELECTION_XORCOMBINATION: The TIMx_CH1, CH2 and CH3 04028 * pins are connected to the TI1 input (XOR combination) 04029 * @retval HAL status 04030 */ 04031 HAL_StatusTypeDef HAL_TIM_ConfigTI1Input(TIM_HandleTypeDef *htim, uint32_t TI1_Selection) 04032 { 04033 uint32_t tmpcr2 = 0; 04034 04035 /* Check the parameters */ 04036 assert_param(IS_TIM_XOR_INSTANCE(htim->Instance)); 04037 assert_param(IS_TIM_TI1SELECTION(TI1_Selection)); 04038 04039 /* Get the TIMx CR2 register value */ 04040 tmpcr2 = htim->Instance->CR2; 04041 04042 /* Reset the TI1 selection */ 04043 tmpcr2 &= ~TIM_CR2_TI1S; 04044 04045 /* Set the TI1 selection */ 04046 tmpcr2 |= TI1_Selection; 04047 04048 /* Write to TIMxCR2 */ 04049 htim->Instance->CR2 = tmpcr2; 04050 04051 return HAL_OK; 04052 } 04053 04054 /** 04055 * @brief Configures the TIM in Slave mode 04056 * @param htim: TIM handle. 04057 * @param sSlaveConfig: pointer to a TIM_SlaveConfigTypeDef structure that 04058 * contains the selected trigger (internal trigger input, filtered 04059 * timer input or external trigger input) and the ) and the Slave 04060 * mode (Disable, Reset, Gated, Trigger, External clock mode 1). 04061 * @retval HAL status 04062 */ 04063 HAL_StatusTypeDef HAL_TIM_SlaveConfigSynchronization(TIM_HandleTypeDef *htim, TIM_SlaveConfigTypeDef * sSlaveConfig) 04064 { 04065 /* Check the parameters */ 04066 assert_param(IS_TIM_SLAVE_INSTANCE(htim->Instance)); 04067 assert_param(IS_TIM_SLAVE_MODE(sSlaveConfig->SlaveMode)); 04068 assert_param(IS_TIM_TRIGGER_SELECTION(sSlaveConfig->InputTrigger)); 04069 04070 __HAL_LOCK(htim); 04071 04072 htim->State = HAL_TIM_STATE_BUSY; 04073 04074 TIM_SlaveTimer_SetConfig(htim, sSlaveConfig); 04075 04076 /* Disable Trigger Interrupt */ 04077 __HAL_TIM_DISABLE_IT(htim, TIM_IT_TRIGGER); 04078 04079 /* Disable Trigger DMA request */ 04080 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_TRIGGER); 04081 04082 htim->State = HAL_TIM_STATE_READY; 04083 04084 __HAL_UNLOCK(htim); 04085 04086 return HAL_OK; 04087 } 04088 04089 /** 04090 * @brief Configures the TIM in Slave mode in interrupt mode 04091 * @param htim: TIM handle. 04092 * @param sSlaveConfig: pointer to a TIM_SlaveConfigTypeDef structure that 04093 * contains the selected trigger (internal trigger input, filtered 04094 * timer input or external trigger input) and the ) and the Slave 04095 * mode (Disable, Reset, Gated, Trigger, External clock mode 1). 04096 * @retval HAL status 04097 */ 04098 HAL_StatusTypeDef HAL_TIM_SlaveConfigSynchronization_IT(TIM_HandleTypeDef *htim, 04099 TIM_SlaveConfigTypeDef * sSlaveConfig) 04100 { 04101 /* Check the parameters */ 04102 assert_param(IS_TIM_SLAVE_INSTANCE(htim->Instance)); 04103 assert_param(IS_TIM_SLAVE_MODE(sSlaveConfig->SlaveMode)); 04104 assert_param(IS_TIM_TRIGGER_SELECTION(sSlaveConfig->InputTrigger)); 04105 04106 __HAL_LOCK(htim); 04107 04108 htim->State = HAL_TIM_STATE_BUSY; 04109 04110 TIM_SlaveTimer_SetConfig(htim, sSlaveConfig); 04111 04112 /* Enable Trigger Interrupt */ 04113 __HAL_TIM_ENABLE_IT(htim, TIM_IT_TRIGGER); 04114 04115 /* Disable Trigger DMA request */ 04116 __HAL_TIM_DISABLE_DMA(htim, TIM_DMA_TRIGGER); 04117 04118 htim->State = HAL_TIM_STATE_READY; 04119 04120 __HAL_UNLOCK(htim); 04121 04122 return HAL_OK; 04123 } 04124 04125 /** 04126 * @brief Read the captured value from Capture Compare unit 04127 * @param htim: TIM handle. 04128 * @param Channel : TIM Channels to be enabled 04129 * This parameter can be one of the following values: 04130 * @arg TIM_CHANNEL_1: TIM Channel 1 selected 04131 * @arg TIM_CHANNEL_2: TIM Channel 2 selected 04132 * @arg TIM_CHANNEL_3: TIM Channel 3 selected 04133 * @arg TIM_CHANNEL_4: TIM Channel 4 selected 04134 * @retval Captured value 04135 */ 04136 uint32_t HAL_TIM_ReadCapturedValue(TIM_HandleTypeDef *htim, uint32_t Channel) 04137 { 04138 uint32_t tmpreg = 0; 04139 04140 __HAL_LOCK(htim); 04141 04142 switch (Channel) 04143 { 04144 case TIM_CHANNEL_1: 04145 { 04146 /* Check the parameters */ 04147 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 04148 04149 /* Return the capture 1 value */ 04150 tmpreg = htim->Instance->CCR1; 04151 04152 break; 04153 } 04154 case TIM_CHANNEL_2: 04155 { 04156 /* Check the parameters */ 04157 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04158 04159 /* Return the capture 2 value */ 04160 tmpreg = htim->Instance->CCR2; 04161 04162 break; 04163 } 04164 04165 case TIM_CHANNEL_3: 04166 { 04167 /* Check the parameters */ 04168 assert_param(IS_TIM_CC3_INSTANCE(htim->Instance)); 04169 04170 /* Return the capture 3 value */ 04171 tmpreg = htim->Instance->CCR3; 04172 04173 break; 04174 } 04175 04176 case TIM_CHANNEL_4: 04177 { 04178 /* Check the parameters */ 04179 assert_param(IS_TIM_CC4_INSTANCE(htim->Instance)); 04180 04181 /* Return the capture 4 value */ 04182 tmpreg = htim->Instance->CCR4; 04183 04184 break; 04185 } 04186 04187 default: 04188 break; 04189 } 04190 04191 __HAL_UNLOCK(htim); 04192 return tmpreg; 04193 } 04194 04195 /** 04196 * @} 04197 */ 04198 04199 /** @defgroup TIM_Exported_Functions_Group9 TIM Callbacks functions 04200 * @brief TIM Callbacks functions 04201 * 04202 @verbatim 04203 ============================================================================== 04204 ##### TIM Callbacks functions ##### 04205 ============================================================================== 04206 [..] 04207 This section provides TIM callback functions: 04208 (+) Timer Period elapsed callback 04209 (+) Timer Output Compare callback 04210 (+) Timer Input capture callback 04211 (+) Timer Trigger callback 04212 (+) Timer Error callback 04213 04214 @endverbatim 04215 * @{ 04216 */ 04217 04218 /** 04219 * @brief Period elapsed callback in non-blocking mode 04220 * @param htim : TIM handle 04221 * @retval None 04222 */ 04223 __weak void HAL_TIM_PeriodElapsedCallback(TIM_HandleTypeDef *htim) 04224 { 04225 /* NOTE : This function should not be modified, when the callback is needed, 04226 the __HAL_TIM_PeriodElapsedCallback could be implemented in the user file 04227 */ 04228 04229 } 04230 /** 04231 * @brief Output Compare callback in non-blocking mode 04232 * @param htim : TIM OC handle 04233 * @retval None 04234 */ 04235 __weak void HAL_TIM_OC_DelayElapsedCallback(TIM_HandleTypeDef *htim) 04236 { 04237 /* NOTE : This function should not be modified, when the callback is needed, 04238 the __HAL_TIM_OC_DelayElapsedCallback could be implemented in the user file 04239 */ 04240 } 04241 /** 04242 * @brief Input Capture callback in non-blocking mode 04243 * @param htim : TIM IC handle 04244 * @retval None 04245 */ 04246 __weak void HAL_TIM_IC_CaptureCallback(TIM_HandleTypeDef *htim) 04247 { 04248 /* NOTE : This function should not be modified, when the callback is needed, 04249 the __HAL_TIM_IC_CaptureCallback could be implemented in the user file 04250 */ 04251 } 04252 04253 /** 04254 * @brief PWM Pulse finished callback in non-blocking mode 04255 * @param htim : TIM handle 04256 * @retval None 04257 */ 04258 __weak void HAL_TIM_PWM_PulseFinishedCallback(TIM_HandleTypeDef *htim) 04259 { 04260 /* NOTE : This function should not be modified, when the callback is needed, 04261 the __HAL_TIM_PWM_PulseFinishedCallback could be implemented in the user file 04262 */ 04263 } 04264 04265 /** 04266 * @brief Hall Trigger detection callback in non-blocking mode 04267 * @param htim : TIM handle 04268 * @retval None 04269 */ 04270 __weak void HAL_TIM_TriggerCallback(TIM_HandleTypeDef *htim) 04271 { 04272 /* NOTE : This function should not be modified, when the callback is needed, 04273 the HAL_TIM_TriggerCallback could be implemented in the user file 04274 */ 04275 } 04276 04277 /** 04278 * @brief Timer error callback in non-blocking mode 04279 * @param htim : TIM handle 04280 * @retval None 04281 */ 04282 __weak void HAL_TIM_ErrorCallback(TIM_HandleTypeDef *htim) 04283 { 04284 /* NOTE : This function should not be modified, when the callback is needed, 04285 the HAL_TIM_ErrorCallback could be implemented in the user file 04286 */ 04287 } 04288 04289 /** 04290 * @} 04291 */ 04292 04293 /** @defgroup TIM_Exported_Functions_Group10 Peripheral State functions 04294 * @brief Peripheral State functions 04295 * 04296 @verbatim 04297 ============================================================================== 04298 ##### Peripheral State functions ##### 04299 ============================================================================== 04300 [..] 04301 This subsection permits to get in run-time the status of the peripheral 04302 and the data flow. 04303 04304 @endverbatim 04305 * @{ 04306 */ 04307 04308 /** 04309 * @brief Return the TIM Base handle state. 04310 * @param htim: TIM Base handle 04311 * @retval HAL state 04312 */ 04313 HAL_TIM_StateTypeDef HAL_TIM_Base_GetState(TIM_HandleTypeDef *htim) 04314 { 04315 return htim->State; 04316 } 04317 04318 /** 04319 * @brief Return the TIM OC handle state. 04320 * @param htim: TIM Ouput Compare handle 04321 * @retval HAL state 04322 */ 04323 HAL_TIM_StateTypeDef HAL_TIM_OC_GetState(TIM_HandleTypeDef *htim) 04324 { 04325 return htim->State; 04326 } 04327 04328 /** 04329 * @brief Return the TIM PWM handle state. 04330 * @param htim: TIM handle 04331 * @retval HAL state 04332 */ 04333 HAL_TIM_StateTypeDef HAL_TIM_PWM_GetState(TIM_HandleTypeDef *htim) 04334 { 04335 return htim->State; 04336 } 04337 04338 /** 04339 * @brief Return the TIM Input Capture handle state. 04340 * @param htim: TIM IC handle 04341 * @retval HAL state 04342 */ 04343 HAL_TIM_StateTypeDef HAL_TIM_IC_GetState(TIM_HandleTypeDef *htim) 04344 { 04345 return htim->State; 04346 } 04347 04348 /** 04349 * @brief Return the TIM One Pulse Mode handle state. 04350 * @param htim: TIM OPM handle 04351 * @retval HAL state 04352 */ 04353 HAL_TIM_StateTypeDef HAL_TIM_OnePulse_GetState(TIM_HandleTypeDef *htim) 04354 { 04355 return htim->State; 04356 } 04357 04358 /** 04359 * @brief Return the TIM Encoder Mode handle state. 04360 * @param htim: TIM Encoder handle 04361 * @retval HAL state 04362 */ 04363 HAL_TIM_StateTypeDef HAL_TIM_Encoder_GetState(TIM_HandleTypeDef *htim) 04364 { 04365 return htim->State; 04366 } 04367 04368 /** 04369 * @} 04370 */ 04371 04372 /** 04373 * @brief TIM DMA error callback 04374 * @param hdma : pointer to DMA handle. 04375 * @retval None 04376 */ 04377 void TIM_DMAError(DMA_HandleTypeDef *hdma) 04378 { 04379 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 04380 04381 htim->State= HAL_TIM_STATE_READY; 04382 04383 HAL_TIM_ErrorCallback(htim); 04384 } 04385 04386 /** 04387 * @brief TIM DMA Delay Pulse complete callback. 04388 * @param hdma : pointer to DMA handle. 04389 * @retval None 04390 */ 04391 void TIM_DMADelayPulseCplt(DMA_HandleTypeDef *hdma) 04392 { 04393 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 04394 04395 htim->State= HAL_TIM_STATE_READY; 04396 04397 if (hdma == htim->hdma[TIM_DMA_ID_CC1]) 04398 { 04399 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_1; 04400 } 04401 else if (hdma == htim->hdma[TIM_DMA_ID_CC2]) 04402 { 04403 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_2; 04404 } 04405 else if (hdma == htim->hdma[TIM_DMA_ID_CC3]) 04406 { 04407 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_3; 04408 } 04409 else if (hdma == htim->hdma[TIM_DMA_ID_CC4]) 04410 { 04411 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_4; 04412 } 04413 04414 HAL_TIM_PWM_PulseFinishedCallback(htim); 04415 04416 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 04417 } 04418 /** 04419 * @brief TIM DMA Capture complete callback. 04420 * @param hdma : pointer to DMA handle. 04421 * @retval None 04422 */ 04423 void TIM_DMACaptureCplt(DMA_HandleTypeDef *hdma) 04424 { 04425 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 04426 04427 htim->State= HAL_TIM_STATE_READY; 04428 04429 if (hdma == htim->hdma[TIM_DMA_ID_CC1]) 04430 { 04431 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_1; 04432 } 04433 else if (hdma == htim->hdma[TIM_DMA_ID_CC2]) 04434 { 04435 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_2; 04436 } 04437 else if (hdma == htim->hdma[TIM_DMA_ID_CC3]) 04438 { 04439 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_3; 04440 } 04441 else if (hdma == htim->hdma[TIM_DMA_ID_CC4]) 04442 { 04443 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_4; 04444 } 04445 04446 HAL_TIM_IC_CaptureCallback(htim); 04447 04448 htim->Channel = HAL_TIM_ACTIVE_CHANNEL_CLEARED; 04449 } 04450 04451 /** 04452 * @brief TIM DMA Period Elapse complete callback. 04453 * @param hdma : pointer to DMA handle. 04454 * @retval None 04455 */ 04456 static void TIM_DMAPeriodElapsedCplt(DMA_HandleTypeDef *hdma) 04457 { 04458 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 04459 04460 htim->State= HAL_TIM_STATE_READY; 04461 04462 HAL_TIM_PeriodElapsedCallback(htim); 04463 } 04464 04465 /** 04466 * @brief TIM DMA Trigger callback. 04467 * @param hdma : pointer to DMA handle. 04468 * @retval None 04469 */ 04470 static void TIM_DMATriggerCplt(DMA_HandleTypeDef *hdma) 04471 { 04472 TIM_HandleTypeDef* htim = ( TIM_HandleTypeDef* )((DMA_HandleTypeDef* )hdma)->Parent; 04473 04474 htim->State= HAL_TIM_STATE_READY; 04475 04476 HAL_TIM_TriggerCallback(htim); 04477 } 04478 04479 /** 04480 * @brief Time Base configuration 04481 * @param TIMx: TIM peripheral 04482 * @param Structure: TIM Base configuration structure 04483 * @retval None 04484 */ 04485 void TIM_Base_SetConfig(TIM_TypeDef *TIMx, TIM_Base_InitTypeDef *Structure) 04486 { 04487 uint32_t tmpcr1 = 0; 04488 tmpcr1 = TIMx->CR1; 04489 04490 /* Set TIM Time Base Unit parameters ---------------------------------------*/ 04491 if (IS_TIM_COUNTER_MODE_SELECT_INSTANCE(TIMx)) 04492 { 04493 /* Select the Counter Mode */ 04494 tmpcr1 &= ~(TIM_CR1_DIR | TIM_CR1_CMS); 04495 tmpcr1 |= Structure->CounterMode; 04496 } 04497 04498 if(IS_TIM_CLOCK_DIVISION_INSTANCE(TIMx)) 04499 { 04500 /* Set the clock division */ 04501 tmpcr1 &= ~TIM_CR1_CKD; 04502 tmpcr1 |= (uint32_t)Structure->ClockDivision; 04503 } 04504 04505 TIMx->CR1 = tmpcr1; 04506 04507 /* Set the Autoreload value */ 04508 TIMx->ARR = (uint32_t)Structure->Period ; 04509 04510 /* Set the Prescaler value */ 04511 TIMx->PSC = (uint32_t)Structure->Prescaler; 04512 04513 if (IS_TIM_REPETITION_COUNTER_INSTANCE(TIMx)) 04514 { 04515 /* Set the Repetition Counter value */ 04516 TIMx->RCR = Structure->RepetitionCounter; 04517 } 04518 04519 /* Generate an update event to reload the Prescaler 04520 and the repetition counter(only for TIM1 and TIM8) value immediately */ 04521 TIMx->EGR = TIM_EGR_UG; 04522 } 04523 04524 /** 04525 * @brief Time Ouput Compare 1 configuration 04526 * @param TIMx to select the TIM peripheral 04527 * @param OC_Config: The ouput configuration structure 04528 * @retval None 04529 */ 04530 void TIM_OC1_SetConfig(TIM_TypeDef *TIMx, TIM_OC_InitTypeDef *OC_Config) 04531 { 04532 uint32_t tmpccmrx = 0; 04533 uint32_t tmpccer = 0; 04534 uint32_t tmpcr2 = 0; 04535 04536 /* Disable the Channel 1: Reset the CC1E Bit */ 04537 TIMx->CCER &= ~TIM_CCER_CC1E; 04538 04539 /* Get the TIMx CCER register value */ 04540 tmpccer = TIMx->CCER; 04541 /* Get the TIMx CR2 register value */ 04542 tmpcr2 = TIMx->CR2; 04543 04544 /* Get the TIMx CCMR1 register value */ 04545 tmpccmrx = TIMx->CCMR1; 04546 04547 /* Reset the Output Compare Mode Bits */ 04548 tmpccmrx &= ~TIM_CCMR1_OC1M; 04549 tmpccmrx &= ~TIM_CCMR1_CC1S; 04550 /* Select the Output Compare Mode */ 04551 tmpccmrx |= OC_Config->OCMode; 04552 04553 /* Reset the Output Polarity level */ 04554 tmpccer &= ~TIM_CCER_CC1P; 04555 /* Set the Output Compare Polarity */ 04556 tmpccer |= OC_Config->OCPolarity; 04557 04558 if(IS_TIM_CCXN_INSTANCE(TIMx, TIM_CHANNEL_1)) 04559 { 04560 /* Check parameters */ 04561 assert_param(IS_TIM_OCN_POLARITY(OC_Config->OCNPolarity)); 04562 04563 /* Reset the Output N Polarity level */ 04564 tmpccer &= ~TIM_CCER_CC1NP; 04565 /* Set the Output N Polarity */ 04566 tmpccer |= OC_Config->OCNPolarity; 04567 /* Reset the Output N State */ 04568 tmpccer &= ~TIM_CCER_CC1NE; 04569 } 04570 04571 if(IS_TIM_BREAK_INSTANCE(TIMx)) 04572 { 04573 /* Check parameters */ 04574 assert_param(IS_TIM_OCNIDLE_STATE(OC_Config->OCNIdleState)); 04575 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04576 04577 /* Reset the Output Compare and Output Compare N IDLE State */ 04578 tmpcr2 &= ~TIM_CR2_OIS1; 04579 tmpcr2 &= ~TIM_CR2_OIS1N; 04580 /* Set the Output Idle state */ 04581 tmpcr2 |= OC_Config->OCIdleState; 04582 /* Set the Output N Idle state */ 04583 tmpcr2 |= OC_Config->OCNIdleState; 04584 } 04585 /* Write to TIMx CR2 */ 04586 TIMx->CR2 = tmpcr2; 04587 04588 /* Write to TIMx CCMR1 */ 04589 TIMx->CCMR1 = tmpccmrx; 04590 04591 /* Set the Capture Compare Register value */ 04592 TIMx->CCR1 = OC_Config->Pulse; 04593 04594 /* Write to TIMx CCER */ 04595 TIMx->CCER = tmpccer; 04596 } 04597 04598 /** 04599 * @brief Time Ouput Compare 2 configuration 04600 * @param TIMx to select the TIM peripheral 04601 * @param OC_Config: The ouput configuration structure 04602 * @retval None 04603 */ 04604 void TIM_OC2_SetConfig(TIM_TypeDef *TIMx, TIM_OC_InitTypeDef *OC_Config) 04605 { 04606 uint32_t tmpccmrx = 0; 04607 uint32_t tmpccer = 0; 04608 uint32_t tmpcr2 = 0; 04609 04610 /* Disable the Channel 2: Reset the CC2E Bit */ 04611 TIMx->CCER &= ~TIM_CCER_CC2E; 04612 04613 /* Get the TIMx CCER register value */ 04614 tmpccer = TIMx->CCER; 04615 /* Get the TIMx CR2 register value */ 04616 tmpcr2 = TIMx->CR2; 04617 04618 /* Get the TIMx CCMR1 register value */ 04619 tmpccmrx = TIMx->CCMR1; 04620 04621 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 04622 tmpccmrx &= ~TIM_CCMR1_OC2M; 04623 tmpccmrx &= ~TIM_CCMR1_CC2S; 04624 04625 /* Select the Output Compare Mode */ 04626 tmpccmrx |= (OC_Config->OCMode << 8); 04627 04628 /* Reset the Output Polarity level */ 04629 tmpccer &= ~TIM_CCER_CC2P; 04630 /* Set the Output Compare Polarity */ 04631 tmpccer |= (OC_Config->OCPolarity << 4); 04632 04633 if(IS_TIM_CCXN_INSTANCE(TIMx, TIM_CHANNEL_2)) 04634 { 04635 assert_param(IS_TIM_OCN_POLARITY(OC_Config->OCNPolarity)); 04636 assert_param(IS_TIM_OCNIDLE_STATE(OC_Config->OCNIdleState)); 04637 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04638 04639 /* Reset the Output N Polarity level */ 04640 tmpccer &= ~TIM_CCER_CC2NP; 04641 /* Set the Output N Polarity */ 04642 tmpccer |= (OC_Config->OCNPolarity << 4); 04643 /* Reset the Output N State */ 04644 tmpccer &= ~TIM_CCER_CC2NE; 04645 04646 } 04647 04648 if(IS_TIM_BREAK_INSTANCE(TIMx)) 04649 { 04650 /* Check parameters */ 04651 assert_param(IS_TIM_OCNIDLE_STATE(OC_Config->OCNIdleState)); 04652 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04653 04654 /* Reset the Output Compare and Output Compare N IDLE State */ 04655 tmpcr2 &= ~TIM_CR2_OIS2; 04656 tmpcr2 &= ~TIM_CR2_OIS2N; 04657 /* Set the Output Idle state */ 04658 tmpcr2 |= (OC_Config->OCIdleState << 2); 04659 /* Set the Output N Idle state */ 04660 tmpcr2 |= (OC_Config->OCNIdleState << 2); 04661 } 04662 04663 /* Write to TIMx CR2 */ 04664 TIMx->CR2 = tmpcr2; 04665 04666 /* Write to TIMx CCMR1 */ 04667 TIMx->CCMR1 = tmpccmrx; 04668 04669 /* Set the Capture Compare Register value */ 04670 TIMx->CCR2 = OC_Config->Pulse; 04671 04672 /* Write to TIMx CCER */ 04673 TIMx->CCER = tmpccer; 04674 } 04675 04676 /** 04677 * @brief Time Ouput Compare 3 configuration 04678 * @param TIMx to select the TIM peripheral 04679 * @param OC_Config: The ouput configuration structure 04680 * @retval None 04681 */ 04682 void TIM_OC3_SetConfig(TIM_TypeDef *TIMx, TIM_OC_InitTypeDef *OC_Config) 04683 { 04684 uint32_t tmpccmrx = 0; 04685 uint32_t tmpccer = 0; 04686 uint32_t tmpcr2 = 0; 04687 04688 /* Disable the Channel 3: Reset the CC2E Bit */ 04689 TIMx->CCER &= ~TIM_CCER_CC3E; 04690 04691 /* Get the TIMx CCER register value */ 04692 tmpccer = TIMx->CCER; 04693 /* Get the TIMx CR2 register value */ 04694 tmpcr2 = TIMx->CR2; 04695 04696 /* Get the TIMx CCMR2 register value */ 04697 tmpccmrx = TIMx->CCMR2; 04698 04699 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 04700 tmpccmrx &= ~TIM_CCMR2_OC3M; 04701 tmpccmrx &= ~TIM_CCMR2_CC3S; 04702 /* Select the Output Compare Mode */ 04703 tmpccmrx |= OC_Config->OCMode; 04704 04705 /* Reset the Output Polarity level */ 04706 tmpccer &= ~TIM_CCER_CC3P; 04707 /* Set the Output Compare Polarity */ 04708 tmpccer |= (OC_Config->OCPolarity << 8); 04709 04710 if(IS_TIM_CCXN_INSTANCE(TIMx, TIM_CHANNEL_3)) 04711 { 04712 assert_param(IS_TIM_OCN_POLARITY(OC_Config->OCNPolarity)); 04713 assert_param(IS_TIM_OCNIDLE_STATE(OC_Config->OCNIdleState)); 04714 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04715 04716 /* Reset the Output N Polarity level */ 04717 tmpccer &= ~TIM_CCER_CC3NP; 04718 /* Set the Output N Polarity */ 04719 tmpccer |= (OC_Config->OCNPolarity << 8); 04720 /* Reset the Output N State */ 04721 tmpccer &= ~TIM_CCER_CC3NE; 04722 } 04723 04724 if(IS_TIM_BREAK_INSTANCE(TIMx)) 04725 { 04726 /* Check parameters */ 04727 assert_param(IS_TIM_OCNIDLE_STATE(OC_Config->OCNIdleState)); 04728 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04729 04730 /* Reset the Output Compare and Output Compare N IDLE State */ 04731 tmpcr2 &= ~TIM_CR2_OIS3; 04732 tmpcr2 &= ~TIM_CR2_OIS3N; 04733 /* Set the Output Idle state */ 04734 tmpcr2 |= (OC_Config->OCIdleState << 4); 04735 /* Set the Output N Idle state */ 04736 tmpcr2 |= (OC_Config->OCNIdleState << 4); 04737 } 04738 04739 /* Write to TIMx CR2 */ 04740 TIMx->CR2 = tmpcr2; 04741 04742 /* Write to TIMx CCMR2 */ 04743 TIMx->CCMR2 = tmpccmrx; 04744 04745 /* Set the Capture Compare Register value */ 04746 TIMx->CCR3 = OC_Config->Pulse; 04747 04748 /* Write to TIMx CCER */ 04749 TIMx->CCER = tmpccer; 04750 } 04751 04752 /** 04753 * @brief Time Ouput Compare 4 configuration 04754 * @param TIMx to select the TIM peripheral 04755 * @param OC_Config: The ouput configuration structure 04756 * @retval None 04757 */ 04758 void TIM_OC4_SetConfig(TIM_TypeDef *TIMx, TIM_OC_InitTypeDef *OC_Config) 04759 { 04760 uint32_t tmpccmrx = 0; 04761 uint32_t tmpccer = 0; 04762 uint32_t tmpcr2 = 0; 04763 04764 /* Disable the Channel 4: Reset the CC4E Bit */ 04765 TIMx->CCER &= ~TIM_CCER_CC4E; 04766 04767 /* Get the TIMx CCER register value */ 04768 tmpccer = TIMx->CCER; 04769 /* Get the TIMx CR2 register value */ 04770 tmpcr2 = TIMx->CR2; 04771 04772 /* Get the TIMx CCMR2 register value */ 04773 tmpccmrx = TIMx->CCMR2; 04774 04775 /* Reset the Output Compare mode and Capture/Compare selection Bits */ 04776 tmpccmrx &= ~TIM_CCMR2_OC4M; 04777 tmpccmrx &= ~TIM_CCMR2_CC4S; 04778 04779 /* Select the Output Compare Mode */ 04780 tmpccmrx |= (OC_Config->OCMode << 8); 04781 04782 /* Reset the Output Polarity level */ 04783 tmpccer &= ~TIM_CCER_CC4P; 04784 /* Set the Output Compare Polarity */ 04785 tmpccer |= (OC_Config->OCPolarity << 12); 04786 04787 if(IS_TIM_BREAK_INSTANCE(TIMx)) 04788 { 04789 assert_param(IS_TIM_OCIDLE_STATE(OC_Config->OCIdleState)); 04790 04791 /* Reset the Output Compare IDLE State */ 04792 tmpcr2 &= ~TIM_CR2_OIS4; 04793 /* Set the Output Idle state */ 04794 tmpcr2 |= (OC_Config->OCIdleState << 6); 04795 } 04796 04797 /* Write to TIMx CR2 */ 04798 TIMx->CR2 = tmpcr2; 04799 04800 /* Write to TIMx CCMR2 */ 04801 TIMx->CCMR2 = tmpccmrx; 04802 04803 /* Set the Capture Compare Register value */ 04804 TIMx->CCR4 = OC_Config->Pulse; 04805 04806 /* Write to TIMx CCER */ 04807 TIMx->CCER = tmpccer; 04808 } 04809 04810 static void TIM_SlaveTimer_SetConfig(TIM_HandleTypeDef *htim, 04811 TIM_SlaveConfigTypeDef * sSlaveConfig) 04812 { 04813 uint32_t tmpsmcr = 0; 04814 uint32_t tmpccmr1 = 0; 04815 uint32_t tmpccer = 0; 04816 04817 /* Get the TIMx SMCR register value */ 04818 tmpsmcr = htim->Instance->SMCR; 04819 04820 /* Reset the Trigger Selection Bits */ 04821 tmpsmcr &= ~TIM_SMCR_TS; 04822 /* Set the Input Trigger source */ 04823 tmpsmcr |= sSlaveConfig->InputTrigger; 04824 04825 /* Reset the slave mode Bits */ 04826 tmpsmcr &= ~TIM_SMCR_SMS; 04827 /* Set the slave mode */ 04828 tmpsmcr |= sSlaveConfig->SlaveMode; 04829 04830 /* Write to TIMx SMCR */ 04831 htim->Instance->SMCR = tmpsmcr; 04832 04833 /* Configure the trigger prescaler, filter, and polarity */ 04834 switch (sSlaveConfig->InputTrigger) 04835 { 04836 case TIM_TS_ETRF: 04837 { 04838 /* Check the parameters */ 04839 assert_param(IS_TIM_CLOCKSOURCE_ETRMODE1_INSTANCE(htim->Instance)); 04840 assert_param(IS_TIM_TRIGGERPRESCALER(sSlaveConfig->TriggerPrescaler)); 04841 assert_param(IS_TIM_TRIGGERPOLARITY(sSlaveConfig->TriggerPolarity)); 04842 assert_param(IS_TIM_TRIGGERFILTER(sSlaveConfig->TriggerFilter)); 04843 /* Configure the ETR Trigger source */ 04844 TIM_ETR_SetConfig(htim->Instance, 04845 sSlaveConfig->TriggerPrescaler, 04846 sSlaveConfig->TriggerPolarity, 04847 sSlaveConfig->TriggerFilter); 04848 } 04849 break; 04850 04851 case TIM_TS_TI1F_ED: 04852 { 04853 /* Check the parameters */ 04854 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 04855 assert_param(IS_TIM_TRIGGERFILTER(sSlaveConfig->TriggerFilter)); 04856 04857 /* Disable the Channel 1: Reset the CC1E Bit */ 04858 tmpccer = htim->Instance->CCER; 04859 htim->Instance->CCER &= ~TIM_CCER_CC1E; 04860 tmpccmr1 = htim->Instance->CCMR1; 04861 04862 /* Set the filter */ 04863 tmpccmr1 &= ~TIM_CCMR1_IC1F; 04864 tmpccmr1 |= ((sSlaveConfig->TriggerFilter) << 4); 04865 04866 /* Write to TIMx CCMR1 and CCER registers */ 04867 htim->Instance->CCMR1 = tmpccmr1; 04868 htim->Instance->CCER = tmpccer; 04869 04870 } 04871 break; 04872 04873 case TIM_TS_TI1FP1: 04874 { 04875 /* Check the parameters */ 04876 assert_param(IS_TIM_CC1_INSTANCE(htim->Instance)); 04877 assert_param(IS_TIM_TRIGGERPOLARITY(sSlaveConfig->TriggerPolarity)); 04878 assert_param(IS_TIM_TRIGGERFILTER(sSlaveConfig->TriggerFilter)); 04879 04880 /* Configure TI1 Filter and Polarity */ 04881 TIM_TI1_ConfigInputStage(htim->Instance, 04882 sSlaveConfig->TriggerPolarity, 04883 sSlaveConfig->TriggerFilter); 04884 } 04885 break; 04886 04887 case TIM_TS_TI2FP2: 04888 { 04889 /* Check the parameters */ 04890 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04891 assert_param(IS_TIM_TRIGGERPOLARITY(sSlaveConfig->TriggerPolarity)); 04892 assert_param(IS_TIM_TRIGGERFILTER(sSlaveConfig->TriggerFilter)); 04893 04894 /* Configure TI2 Filter and Polarity */ 04895 TIM_TI2_ConfigInputStage(htim->Instance, 04896 sSlaveConfig->TriggerPolarity, 04897 sSlaveConfig->TriggerFilter); 04898 } 04899 break; 04900 04901 case TIM_TS_ITR0: 04902 { 04903 /* Check the parameter */ 04904 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04905 } 04906 break; 04907 04908 case TIM_TS_ITR1: 04909 { 04910 /* Check the parameter */ 04911 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04912 } 04913 break; 04914 04915 case TIM_TS_ITR2: 04916 { 04917 /* Check the parameter */ 04918 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04919 } 04920 break; 04921 04922 case TIM_TS_ITR3: 04923 { 04924 /* Check the parameter */ 04925 assert_param(IS_TIM_CC2_INSTANCE(htim->Instance)); 04926 } 04927 break; 04928 04929 default: 04930 break; 04931 } 04932 } 04933 04934 /** 04935 * @brief Configure the TI1 as Input. 04936 * @param TIMx to select the TIM peripheral. 04937 * @param TIM_ICPolarity : The Input Polarity. 04938 * This parameter can be one of the following values: 04939 * @arg TIM_ICPolarity_Rising 04940 * @arg TIM_ICPolarity_Falling 04941 * @arg TIM_ICPolarity_BothEdge 04942 * @param TIM_ICSelection: specifies the input to be used. 04943 * This parameter can be one of the following values: 04944 * @arg TIM_ICSelection_DirectTI: TIM Input 1 is selected to be connected to IC1. 04945 * @arg TIM_ICSelection_IndirectTI: TIM Input 1 is selected to be connected to IC2. 04946 * @arg TIM_ICSelection_TRC: TIM Input 1 is selected to be connected to TRC. 04947 * @param TIM_ICFilter: Specifies the Input Capture Filter. 04948 * This parameter must be a value between 0x00 and 0x0F. 04949 * @retval None 04950 * @note TIM_ICFilter and TIM_ICPolarity are not used in INDIRECT mode as TI2FP1 04951 * (on channel2 path) is used as the input signal. Therefore CCMR1 must be 04952 * protected against un-initialized filter and polarity values. 04953 */ 04954 void TIM_TI1_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 04955 uint32_t TIM_ICFilter) 04956 { 04957 uint32_t tmpccmr1 = 0; 04958 uint32_t tmpccer = 0; 04959 04960 /* Disable the Channel 1: Reset the CC1E Bit */ 04961 TIMx->CCER &= ~TIM_CCER_CC1E; 04962 tmpccmr1 = TIMx->CCMR1; 04963 tmpccer = TIMx->CCER; 04964 04965 /* Select the Input */ 04966 if(IS_TIM_CC2_INSTANCE(TIMx) != RESET) 04967 { 04968 tmpccmr1 &= ~TIM_CCMR1_CC1S; 04969 tmpccmr1 |= TIM_ICSelection; 04970 } 04971 else 04972 { 04973 tmpccmr1 |= TIM_CCMR1_CC1S_0; 04974 } 04975 04976 /* Set the filter */ 04977 tmpccmr1 &= ~TIM_CCMR1_IC1F; 04978 tmpccmr1 |= ((TIM_ICFilter << 4) & TIM_CCMR1_IC1F); 04979 04980 /* Select the Polarity and set the CC1E Bit */ 04981 tmpccer &= ~(TIM_CCER_CC1P | TIM_CCER_CC1NP); 04982 tmpccer |= (TIM_ICPolarity & (TIM_CCER_CC1P | TIM_CCER_CC1NP)); 04983 04984 /* Write to TIMx CCMR1 and CCER registers */ 04985 TIMx->CCMR1 = tmpccmr1; 04986 TIMx->CCER = tmpccer; 04987 } 04988 04989 /** 04990 * @brief Configure the Polarity and Filter for TI1. 04991 * @param TIMx to select the TIM peripheral. 04992 * @param TIM_ICPolarity : The Input Polarity. 04993 * This parameter can be one of the following values: 04994 * @arg TIM_ICPolarity_Rising 04995 * @arg TIM_ICPolarity_Falling 04996 * @arg TIM_ICPolarity_BothEdge 04997 * @param TIM_ICFilter: Specifies the Input Capture Filter. 04998 * This parameter must be a value between 0x00 and 0x0F. 04999 * @retval None 05000 */ 05001 static void TIM_TI1_ConfigInputStage(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICFilter) 05002 { 05003 uint32_t tmpccmr1 = 0; 05004 uint32_t tmpccer = 0; 05005 05006 /* Disable the Channel 1: Reset the CC1E Bit */ 05007 tmpccer = TIMx->CCER; 05008 TIMx->CCER &= ~TIM_CCER_CC1E; 05009 tmpccmr1 = TIMx->CCMR1; 05010 05011 /* Set the filter */ 05012 tmpccmr1 &= ~TIM_CCMR1_IC1F; 05013 tmpccmr1 |= (TIM_ICFilter << 4); 05014 05015 /* Select the Polarity and set the CC1E Bit */ 05016 tmpccer &= ~(TIM_CCER_CC1P | TIM_CCER_CC1NP); 05017 tmpccer |= TIM_ICPolarity; 05018 05019 /* Write to TIMx CCMR1 and CCER registers */ 05020 TIMx->CCMR1 = tmpccmr1; 05021 TIMx->CCER = tmpccer; 05022 } 05023 05024 /** 05025 * @brief Configure the TI2 as Input. 05026 * @param TIMx to select the TIM peripheral 05027 * @param TIM_ICPolarity : The Input Polarity. 05028 * This parameter can be one of the following values: 05029 * @arg TIM_ICPolarity_Rising 05030 * @arg TIM_ICPolarity_Falling 05031 * @arg TIM_ICPolarity_BothEdge 05032 * @param TIM_ICSelection: specifies the input to be used. 05033 * This parameter can be one of the following values: 05034 * @arg TIM_ICSelection_DirectTI: TIM Input 2 is selected to be connected to IC2. 05035 * @arg TIM_ICSelection_IndirectTI: TIM Input 2 is selected to be connected to IC1. 05036 * @arg TIM_ICSelection_TRC: TIM Input 2 is selected to be connected to TRC. 05037 * @param TIM_ICFilter: Specifies the Input Capture Filter. 05038 * This parameter must be a value between 0x00 and 0x0F. 05039 * @retval None 05040 * @note TIM_ICFilter and TIM_ICPolarity are not used in INDIRECT mode as TI1FP2 05041 * (on channel1 path) is used as the input signal. Therefore CCMR1 must be 05042 * protected against un-initialized filter and polarity values. 05043 */ 05044 static void TIM_TI2_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 05045 uint32_t TIM_ICFilter) 05046 { 05047 uint32_t tmpccmr1 = 0; 05048 uint32_t tmpccer = 0; 05049 05050 /* Disable the Channel 2: Reset the CC2E Bit */ 05051 TIMx->CCER &= ~TIM_CCER_CC2E; 05052 tmpccmr1 = TIMx->CCMR1; 05053 tmpccer = TIMx->CCER; 05054 05055 /* Select the Input */ 05056 tmpccmr1 &= ~TIM_CCMR1_CC2S; 05057 tmpccmr1 |= (TIM_ICSelection << 8); 05058 05059 /* Set the filter */ 05060 tmpccmr1 &= ~TIM_CCMR1_IC2F; 05061 tmpccmr1 |= ((TIM_ICFilter << 12) & TIM_CCMR1_IC2F); 05062 05063 /* Select the Polarity and set the CC2E Bit */ 05064 tmpccer &= ~(TIM_CCER_CC2P | TIM_CCER_CC2NP); 05065 tmpccer |= ((TIM_ICPolarity << 4) & (TIM_CCER_CC2P | TIM_CCER_CC2NP)); 05066 05067 /* Write to TIMx CCMR1 and CCER registers */ 05068 TIMx->CCMR1 = tmpccmr1 ; 05069 TIMx->CCER = tmpccer; 05070 } 05071 05072 /** 05073 * @brief Configure the Polarity and Filter for TI2. 05074 * @param TIMx to select the TIM peripheral. 05075 * @param TIM_ICPolarity : The Input Polarity. 05076 * This parameter can be one of the following values: 05077 * @arg TIM_ICPolarity_Rising 05078 * @arg TIM_ICPolarity_Falling 05079 * @arg TIM_ICPolarity_BothEdge 05080 * @param TIM_ICFilter: Specifies the Input Capture Filter. 05081 * This parameter must be a value between 0x00 and 0x0F. 05082 * @retval None 05083 */ 05084 static void TIM_TI2_ConfigInputStage(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICFilter) 05085 { 05086 uint32_t tmpccmr1 = 0; 05087 uint32_t tmpccer = 0; 05088 05089 /* Disable the Channel 2: Reset the CC2E Bit */ 05090 TIMx->CCER &= ~TIM_CCER_CC2E; 05091 tmpccmr1 = TIMx->CCMR1; 05092 tmpccer = TIMx->CCER; 05093 05094 /* Set the filter */ 05095 tmpccmr1 &= ~TIM_CCMR1_IC2F; 05096 tmpccmr1 |= (TIM_ICFilter << 12); 05097 05098 /* Select the Polarity and set the CC2E Bit */ 05099 tmpccer &= ~(TIM_CCER_CC2P | TIM_CCER_CC2NP); 05100 tmpccer |= (TIM_ICPolarity << 4); 05101 05102 /* Write to TIMx CCMR1 and CCER registers */ 05103 TIMx->CCMR1 = tmpccmr1 ; 05104 TIMx->CCER = tmpccer; 05105 } 05106 05107 /** 05108 * @brief Configure the TI3 as Input. 05109 * @param TIMx to select the TIM peripheral 05110 * @param TIM_ICPolarity : The Input Polarity. 05111 * This parameter can be one of the following values: 05112 * @arg TIM_ICPolarity_Rising 05113 * @arg TIM_ICPolarity_Falling 05114 * @arg TIM_ICPolarity_BothEdge 05115 * @param TIM_ICSelection: specifies the input to be used. 05116 * This parameter can be one of the following values: 05117 * @arg TIM_ICSelection_DirectTI: TIM Input 3 is selected to be connected to IC3. 05118 * @arg TIM_ICSelection_IndirectTI: TIM Input 3 is selected to be connected to IC4. 05119 * @arg TIM_ICSelection_TRC: TIM Input 3 is selected to be connected to TRC. 05120 * @param TIM_ICFilter: Specifies the Input Capture Filter. 05121 * This parameter must be a value between 0x00 and 0x0F. 05122 * @retval None 05123 * @note TIM_ICFilter and TIM_ICPolarity are not used in INDIRECT mode as TI3FP4 05124 * (on channel1 path) is used as the input signal. Therefore CCMR2 must be 05125 * protected against un-initialized filter and polarity values. 05126 */ 05127 static void TIM_TI3_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 05128 uint32_t TIM_ICFilter) 05129 { 05130 uint32_t tmpccmr2 = 0; 05131 uint32_t tmpccer = 0; 05132 05133 /* Disable the Channel 3: Reset the CC3E Bit */ 05134 TIMx->CCER &= ~TIM_CCER_CC3E; 05135 tmpccmr2 = TIMx->CCMR2; 05136 tmpccer = TIMx->CCER; 05137 05138 /* Select the Input */ 05139 tmpccmr2 &= ~TIM_CCMR2_CC3S; 05140 tmpccmr2 |= TIM_ICSelection; 05141 05142 /* Set the filter */ 05143 tmpccmr2 &= ~TIM_CCMR2_IC3F; 05144 tmpccmr2 |= ((TIM_ICFilter << 4) & TIM_CCMR2_IC3F); 05145 05146 /* Select the Polarity and set the CC3E Bit */ 05147 tmpccer &= ~(TIM_CCER_CC3P | TIM_CCER_CC3NP); 05148 tmpccer |= ((TIM_ICPolarity << 8) & (TIM_CCER_CC3P | TIM_CCER_CC3NP)); 05149 05150 /* Write to TIMx CCMR2 and CCER registers */ 05151 TIMx->CCMR2 = tmpccmr2; 05152 TIMx->CCER = tmpccer; 05153 } 05154 05155 /** 05156 * @brief Configure the TI4 as Input. 05157 * @param TIMx to select the TIM peripheral 05158 * @param TIM_ICPolarity : The Input Polarity. 05159 * This parameter can be one of the following values: 05160 * @arg TIM_ICPolarity_Rising 05161 * @arg TIM_ICPolarity_Falling 05162 * @arg TIM_ICPolarity_BothEdge 05163 * @param TIM_ICSelection: specifies the input to be used. 05164 * This parameter can be one of the following values: 05165 * @arg TIM_ICSelection_DirectTI: TIM Input 4 is selected to be connected to IC4. 05166 * @arg TIM_ICSelection_IndirectTI: TIM Input 4 is selected to be connected to IC3. 05167 * @arg TIM_ICSelection_TRC: TIM Input 4 is selected to be connected to TRC. 05168 * @param TIM_ICFilter: Specifies the Input Capture Filter. 05169 * This parameter must be a value between 0x00 and 0x0F. 05170 * @note TIM_ICFilter and TIM_ICPolarity are not used in INDIRECT mode as TI4FP3 05171 * (on channel1 path) is used as the input signal. Therefore CCMR2 must be 05172 * protected against un-initialized filter and polarity values. 05173 * @retval None 05174 */ 05175 static void TIM_TI4_SetConfig(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICSelection, 05176 uint32_t TIM_ICFilter) 05177 { 05178 uint32_t tmpccmr2 = 0; 05179 uint32_t tmpccer = 0; 05180 05181 /* Disable the Channel 4: Reset the CC4E Bit */ 05182 TIMx->CCER &= ~TIM_CCER_CC4E; 05183 tmpccmr2 = TIMx->CCMR2; 05184 tmpccer = TIMx->CCER; 05185 05186 /* Select the Input */ 05187 tmpccmr2 &= ~TIM_CCMR2_CC4S; 05188 tmpccmr2 |= (TIM_ICSelection << 8); 05189 05190 /* Set the filter */ 05191 tmpccmr2 &= ~TIM_CCMR2_IC4F; 05192 tmpccmr2 |= ((TIM_ICFilter << 12) & TIM_CCMR2_IC4F); 05193 05194 /* Select the Polarity and set the CC4E Bit */ 05195 tmpccer &= ~(TIM_CCER_CC4P | TIM_CCER_CC4NP); 05196 tmpccer |= ((TIM_ICPolarity << 12) & (TIM_CCER_CC4P | TIM_CCER_CC4NP)); 05197 05198 /* Write to TIMx CCMR2 and CCER registers */ 05199 TIMx->CCMR2 = tmpccmr2; 05200 TIMx->CCER = tmpccer ; 05201 } 05202 05203 /** 05204 * @brief Selects the Input Trigger source 05205 * @param TIMx to select the TIM peripheral 05206 * @param InputTriggerSource: The Input Trigger source. 05207 * This parameter can be one of the following values: 05208 * @arg TIM_TS_ITR0: Internal Trigger 0 05209 * @arg TIM_TS_ITR1: Internal Trigger 1 05210 * @arg TIM_TS_ITR2: Internal Trigger 2 05211 * @arg TIM_TS_ITR3: Internal Trigger 3 05212 * @arg TIM_TS_TI1F_ED: TI1 Edge Detector 05213 * @arg TIM_TS_TI1FP1: Filtered Timer Input 1 05214 * @arg TIM_TS_TI2FP2: Filtered Timer Input 2 05215 * @arg TIM_TS_ETRF: External Trigger input 05216 * @retval None 05217 */ 05218 static void TIM_ITRx_SetConfig(TIM_TypeDef *TIMx, uint16_t InputTriggerSource) 05219 { 05220 uint32_t tmpsmcr = 0; 05221 05222 /* Get the TIMx SMCR register value */ 05223 tmpsmcr = TIMx->SMCR; 05224 /* Reset the TS Bits */ 05225 tmpsmcr &= ~TIM_SMCR_TS; 05226 /* Set the Input Trigger source and the slave mode*/ 05227 tmpsmcr |= InputTriggerSource | TIM_SLAVEMODE_EXTERNAL1; 05228 /* Write to TIMx SMCR */ 05229 TIMx->SMCR = tmpsmcr; 05230 } 05231 /** 05232 * @brief Configures the TIMx External Trigger (ETR). 05233 * @param TIMx to select the TIM peripheral 05234 * @param TIM_ExtTRGPrescaler: The external Trigger Prescaler. 05235 * This parameter can be one of the following values: 05236 * @arg TIM_ExtTRGPSC_DIV1: ETRP Prescaler OFF. 05237 * @arg TIM_ExtTRGPSC_DIV2: ETRP frequency divided by 2. 05238 * @arg TIM_ExtTRGPSC_DIV4: ETRP frequency divided by 4. 05239 * @arg TIM_ExtTRGPSC_DIV8: ETRP frequency divided by 8. 05240 * @param TIM_ExtTRGPolarity: The external Trigger Polarity. 05241 * This parameter can be one of the following values: 05242 * @arg TIM_ExtTRGPolarity_Inverted: active low or falling edge active. 05243 * @arg TIM_ExtTRGPolarity_NonInverted: active high or rising edge active. 05244 * @param ExtTRGFilter: External Trigger Filter. 05245 * This parameter must be a value between 0x00 and 0x0F 05246 * @retval None 05247 */ 05248 void TIM_ETR_SetConfig(TIM_TypeDef* TIMx, uint32_t TIM_ExtTRGPrescaler, 05249 uint32_t TIM_ExtTRGPolarity, uint32_t ExtTRGFilter) 05250 { 05251 uint32_t tmpsmcr = 0; 05252 05253 tmpsmcr = TIMx->SMCR; 05254 05255 /* Reset the ETR Bits */ 05256 tmpsmcr &= ~(TIM_SMCR_ETF | TIM_SMCR_ETPS | TIM_SMCR_ECE | TIM_SMCR_ETP); 05257 05258 /* Set the Prescaler, the Filter value and the Polarity */ 05259 tmpsmcr |= (uint32_t)(TIM_ExtTRGPrescaler | (TIM_ExtTRGPolarity | (ExtTRGFilter << 8))); 05260 05261 /* Write to TIMx SMCR */ 05262 TIMx->SMCR = tmpsmcr; 05263 } 05264 05265 /** 05266 * @brief Enables or disables the TIM Capture Compare Channel x. 05267 * @param TIMx to select the TIM peripheral 05268 * @param Channel: specifies the TIM Channel 05269 * This parameter can be one of the following values: 05270 * @arg TIM_CHANNEL_1: TIM Channel 1 05271 * @arg TIM_CHANNEL_2: TIM Channel 2 05272 * @arg TIM_CHANNEL_3: TIM Channel 3 05273 * @arg TIM_CHANNEL_4: TIM Channel 4 05274 * @param ChannelState: specifies the TIM Channel CCxE bit new state. 05275 * This parameter can be: TIM_CCx_ENABLE or TIM_CCx_Disable. 05276 * @retval None 05277 */ 05278 void TIM_CCxChannelCmd(TIM_TypeDef* TIMx, uint32_t Channel, uint32_t ChannelState) 05279 { 05280 uint32_t tmp = 0; 05281 05282 /* Check the parameters */ 05283 assert_param(IS_TIM_CC1_INSTANCE(TIMx)); 05284 assert_param(IS_TIM_CHANNELS(Channel)); 05285 05286 tmp = TIM_CCER_CC1E << Channel; 05287 05288 /* Reset the CCxE Bit */ 05289 TIMx->CCER &= ~tmp; 05290 05291 /* Set or reset the CCxE Bit */ 05292 TIMx->CCER |= (uint32_t)(ChannelState << Channel); 05293 } 05294 05295 05296 /** 05297 * @} 05298 */ 05299 05300 #endif /* HAL_TIM_MODULE_ENABLED */ 05301 /** 05302 * @} 05303 */ 05304 05305 /** 05306 * @} 05307 */ 05308 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 05309
Generated on Tue Jul 12 2022 10:58:10 by
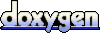